Exploring Android Development with Visual Studio
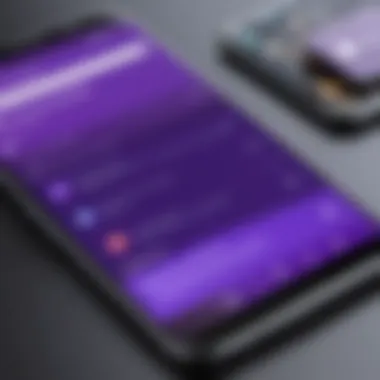
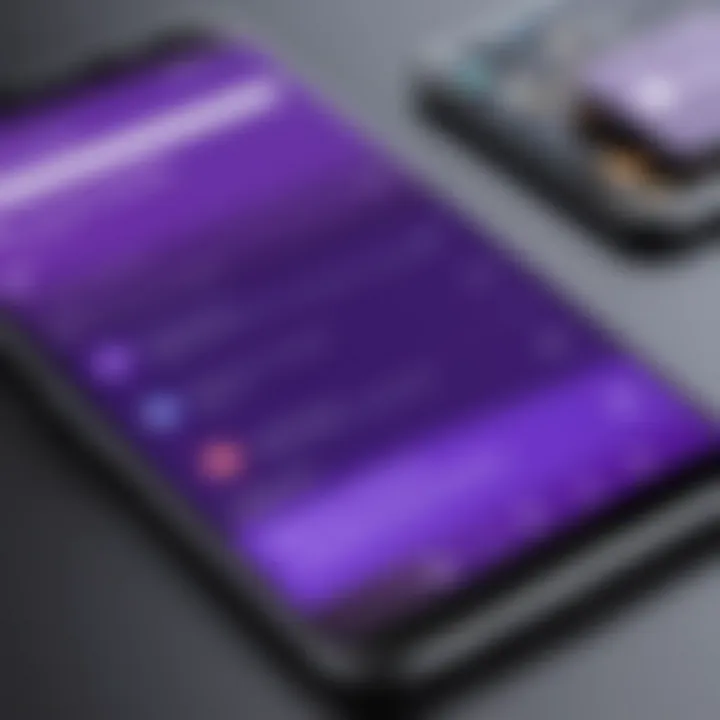
Intro
In recent years, Android development has seen a significant shift in the tools and frameworks available to developers. Among these tools, Visual Studio emerges as a powerful option, particularly when integrated with Xamarin. This combination allows for a more seamless development experience, enabling programmers to create robust Android applications using C#. The intent of this article is not just to inform but to provide a comprehensive guide to using Visual Studio for Android app development.
Key Points to Discuss
The article will explore several critical dimensions of Android development using Visual Studio.
- Initial Setup: Getting Visual Studio ready for Android development, including configuration and required components.
- Xamarin Integration: Understanding how Xamarin extends the capabilities of Visual Studio to create cross-platform applications.
- Mobile Design Considerations: Key considerations, such as UI/UX guidelines, that enhance the user experience in mobile applications.
- Coding Best Practices: Techniques and practices that can improve the quality and performance of the code.
- Performance Optimization: Strategies to ensure applications run efficiently on various devices.
- Debugging Techniques: Approaches for troubleshooting and resolving issues during development.
- Deployment Strategies: Various methods to effectively deploy the application once development is complete.
- Community and Resources: Insightful resources and community contributions to aid developers in their journey.
With this structure, the article aims to equip both novice and more experienced developers with the necessary knowledge and tools to effectively handle Android development in Visual Studio. The exploration will be thorough, ensuring a clear understanding of each topic while avoiding common pitfalls in mobile app development.
Preamble to Android Development
Android development is a crucial area in the realm of software engineering. With over two billion active Android devices globally, the demand for Android applications is ever-growing. This section serves as an entry point to explore the fundamentals of Android development, emphasizing its significance in today's mobile-centric world.
Understanding the importance of Android development is essential for both new and seasoned developers. As technology evolves, so do the tools and frameworks available for development. Being proficient in Android development opens various career opportunities. It allows developers to create applications that enhance user experience and meet modern needs.
In this article, we will cover why Android development matters and how it can benefit developers. Learn about the tools tailored for Android, including Visual Studio and its integration with Xamarin for streamlined app development.
Historical Context of Android Development
The history of Android development dates back to 2003 when Android Inc. was founded. Initially, Android was meant for digital cameras but quickly shifted focus to mobile devices. In 2005, Google acquired Android Inc., launching Android as an open-source platform in 2008.
Over the years, Android has seen numerous updates, evolving from a novice platform to a frontrunner in mobile OS. Each Android version has introduced features that improve functionality, security, and user experience. The Android SDK enables developers to build applications using Java and Kotlin. Such historical context provides background on how Android has grown, impacting the mobile development landscape significantly.
The Role of Visual Studio in Modern Development
In the contemporary development ecosystem, Visual Studio stands out for its robust capabilities. It provides a comprehensive integrated development environment (IDE) for a range of applications, including Android.
With Visual Studio, developers can harness powerful debugging tools, sophisticated code editing features, and extensive project management capabilities. Visual Studio also seamlessly integrates with Xamarin, facilitating cross-platform development. This enables developers to write code in C#, reuse components across platforms, and hence accelerate the development process.
Moreover, the inclusion of tools such as the Android Emulator enhances the testing phase of development. Developers can simulate different devices and Android OS versions with ease, ensuring their applications perform optimally across varying conditions.
"A proficient development environment like Visual Studio can be a game-changer. It streamlines the process, making Android development more accessible and efficient."
In summary, Visual Studio isn’t just a tool; it serves as a critical enabler for Android developers. As we progress in the article, we will delve deeper into setting it up, exploring its features, and understanding how it enhances Android development.
Setting Up Visual Studio for Android Development
Setting up Visual Studio for Android development is a crucial foundational step for any developer aiming to work within the Android ecosystem. This setup process not only optimizes the development experience but also equips developers with essential tools that streamline coding, testing, and deployment. Visual Studio integrates various features and functionalities, making it a preferred choice for many. Understanding how to properly configure the environment can lead to smoother project workflows, increased productivity, and a better overall understanding of Android development practices.
Installing Visual Studio and Necessary Components
To begin the installation of Visual Studio, visit the official Microsoft website. Once on the site, download the installer for the Community, Professional, or Enterprise edition depending on your needs. During the installation process, you will encounter the option to select workloads. For Android development, ensure to check the Mobile development with .NET workload. This option installs Visual Studio, along with Xamarin and all other necessary components for Android development.
- After selecting the relevant workload, proceed to install.
- Also, download additional necessary SDKs by checking related options.
Completing the installation may take some time depending on your system configuration. Once finished, launch Visual Studio and verify the installation by creating a new Android project. This check helps confirm that the required components are correctly installed and functioning.
Configuring the Android Emulator
The Android Emulator is a fundamental tool for testing applications in a virtual environment. After installing Visual Studio, you must configure the Android Emulator to simulate various device specifications. To begin, locate the Android Emulator component in the Visual Studio Installer or package manager.
- Select the appropriate API level for your target devices. Google provides different API levels to emulate various Android versions.
- Choose the device specification that corresponds to the devices your app will support.
Once setup is done, open the Android Device Manager within Visual Studio. Here, you can create or launch your configured virtual device. Testing your application on an emulator helps catch issues before deployment, making it an essential routine in mobile development.
Integrating Xamarin with Visual Studio
Xamarin is a prominent framework within Visual Studio that facilitates cross-platform development. It allows developers to write shared code for both Android and iOS applications using C#. To integrate Xamarin effectively, make sure that it is selected during the Visual Studio installation. If it wasn't included initially, you can add it through the Visual Studio Installer later.
Once Xamarin is installed, create a new project by selecting -> .
During project setup, you can choose between various project templates. The ideal option often depends on the specifics of your application:
- Blank App (for building from scratch)
- Tabbed App (for applications requiring tabbed navigation)
- Master-Detail App (suitable for data-driven applications)
After setting up a Xamarin project, explore Xamarin's libraries and components, which allow for easier UI design and functionality. This integration simplifies the process of maintaining a single code base for multiple platforms, enhancing efficiency in the development workflow.
"The efforts put into setting up your development environment reflect directly on the ease and effectiveness of your development tasks."
Through careful setup of Visual Studio for Android development, programmers can harness powerful tools that significantly enhance their productivity and ease the path toward delivering high-quality applications.
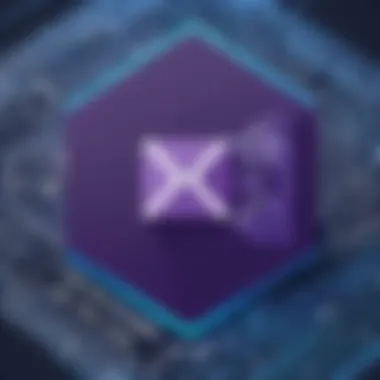
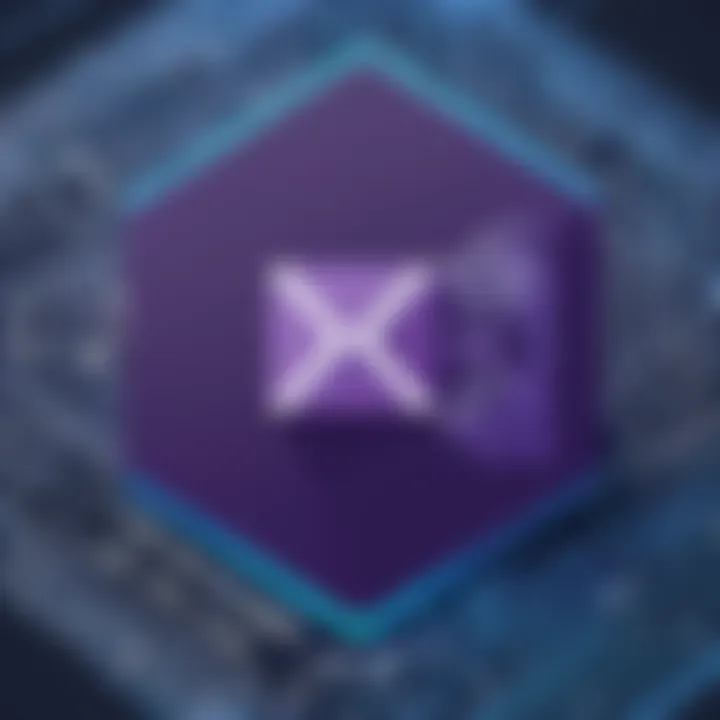
Understanding Android Application Architecture
The importance of understanding Android application architecture cannot be understated in the realm of development. A robust framework establishes a solid foundation for building applications that are not only efficient but also maintainable over time. By grasping the components and design principles involved, developers can make informed decisions that affect both the functionality and usability of their applications. This section delves into critical elements that collectively contribute to an application's architecture, ultimately enhancing the development process.
Overview of Android Architecture Components
Android architecture consists of several key components that work together cohesively. Here is a breakdown of these essential elements:
- Activities: They represent the user interface screen. Activities manage the UI elements and user interactions.
- Fragments: Fragments are modular sections of an activity that can be reused across different screens. This allows for more dynamic UIs.
- Services: Services run in the background without user interface components. They perform long-running operations. Examples include downloading files or playing music.
- Content Providers: They manage application data. Applications can share data with other apps through content providers safely.
- Broadcast Receivers: These components respond to system-wide broadcast messages. They help apps listen for events such as battery low warnings or network connectivity changes.
Each of these components interacts within the Android framework, facilitating a smooth development process. By understanding these components and their functions, developers can design applications that are both powerful and user-friendly.
Navigating the Android Manifest File
The Android manifest file is crucial in the application development. This XML file serves as a blueprint for the app and outlines essential characteristics, such as:
- Package Name: This unique identifier distinguishes your app from others in the Google Play Store.
- Permissions: The manifest specifies what system features the app can access. For example, if your app needs access to the device's camera or internet, these permissions must be declared in the manifest file.
- Activities and Services: You must declare your activities and any services your app will use. This information informs the Android system about what components your app has and how to interact with them.
Here is a simple example of an Android manifest entry:
This entry defines the application's package, permissions, and the main activity. Each attribute in the manifest helps the Android system understand how to manage and interact with the application effectively. Understanding the Android manifest file is essential, as errors in this file can lead to application malfunction or failure to execute.
The Android manifest file serves as the critical guide for the app, detailing how it will operate within the Android ecosystem.
Development Fundamentals
The importance of development fundamentals in Android development using Visual Studio cannot be understated. This area serves as the backbone for effective mobile application design, coding practices, and overall project success. A solid understanding of development fundamentals ensures that programmers, whether novice or experienced, can create efficient, maintainable, and high-quality applications.
In a thriving ecosystem like Android, developers must grasp the nuances of coding conventions, debugging techniques, and user interface design principles. By mastering these elements, programmers can improve the robustness of their applications while enhancing user experience. The need for cross-platform compatibility is increasingly significant, especially when using tools like Visual Studio. Hence, establishing a firm foundation in these fundamentals will guide developers towards making informed decisions throughout the development process.
Recognizing the best practices in coding not only boosts productivity but also streamlines collaboration within teams. Establishing standards will mitigate common pitfalls and contribute to a more efficient development life cycle.
Furthermore, development fundamentals encompass a myriad of considerations such as:
- Version control: Understanding systems like Git can facilitate smoother project management by tracking changes and enabling collaborative work.
- Documentation: Writing clear documentation aids in maintaining the code and ensures better comprehension among team members.
- Testing techniques: Implementing robust testing methods leads to fewer bugs and enhances software reliability.
These aspects, combined, empower developers to face the ever-evolving challenges of software development. They serve as critical touchpoints through which one approaches problem-solving and innovation in the realm of Android development.
Coding Practices for Android with Visual Studio
When it comes to coding practices for Android development in Visual Studio, several guidelines stand out. First and foremost, adhering to a consistent coding style significantly helps in maintaining readability. Using fully descriptive variable names and organizing code into clear, logical structures allows other developers to navigate your code easily.
Moreover, it is prudent to utilize comments judiciously. While an ideally constructed code should be self-explanatory, certain complex logic may require clarification. Regularly refactoring the code can also substantially enhance its quality. Clean code is not just about eliminating bugs; it is about improving maintainability.
Another key practice is to embrace modular programming. Breaking the application code into smaller, reusable components promotes organization and reduces redundancy. Furthermore, this approach enables easier testing and debugging.
The integration of linting tools can also help in identifying potential errors or style violations before they escalate. Ultimately, following these coding practices contributes to a higher-quality product and facilitates collaboration among team members.
Utilizing Xamarin.Forms for UI Development
Xamarin.Forms plays a crucial role in UI development for Android applications within Visual Studio. This framework allows developers to create cross-platform applications with a native look and feel, using a single codebase. Leveraging Xamarin.Forms can drastically reduce the time spent coding and maintaining UI elements across various platforms.
With its comprehensive library of pre-built components, Xamarin.Forms simplifies the process of designing user interfaces. These UI controls are designed with responsiveness in mind, adapting seamlessly to different screen sizes and orientations.
One of the standout benefits of Xamarin.Forms is its capability to bind data efficiently. This framework utilizes data binding to connect UI components to data sources, ensuring that the UI reflects the current state of the data without requiring extensive boilerplate code.
Another consideration is the layout options available in Xamarin.Forms. Developers can use several layout patterns such as StackLayout, Grid, and AbsoluteLayout. Each serves different situations and helps in organizing the UI elements effectively, enhancing user interaction.
Key Tools in Visual Studio for Android Development
Understanding the key tools available in Visual Studio for Android development is critical for both efficiency and effectiveness in application creation. These tools aid in streamlining workflows, enhance collaboration, and improve the overall quality of applications. Visual Studio, equipped with specialized functionalities, provides a robust environment for developers to work within, offering a balance between flexibility and power when coding and managing Android projects.
Using the Android Device Monitor
The Android Device Monitor is an essential tool within Visual Studio that facilitates the management and debugging of Android devices. This tool enables developers to monitor the performance of their applications on physical devices or emulators in real time. It allows observation of CPU usage, memory consumption, and network activity, which is vital for identifying performance bottlenecks.
Among the benefits of utilizing the Android Device Monitor are:
- Real-Time Performance Tracking: Developers can see how their app interacts with the device resources and gain insights into areas that may require optimization.
- Logcat Viewer: This feature allows viewing system messages, including stack traces when an error occurs, which is incredibly useful during the debugging process.
- Snapshot Capabilities: Taking snapshots of device states helps in understanding how the app behaves under different conditions. This is particularly useful for testing various network states or user interactions.
The Android Device Monitor can be accessed directly within Visual Studio, making it convenient for developers to switch between coding and monitoring without disrupting their workflow. This integration ensures a seamless development experience.
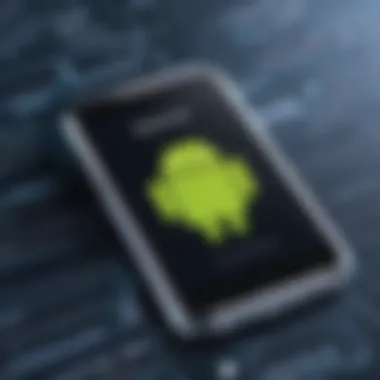
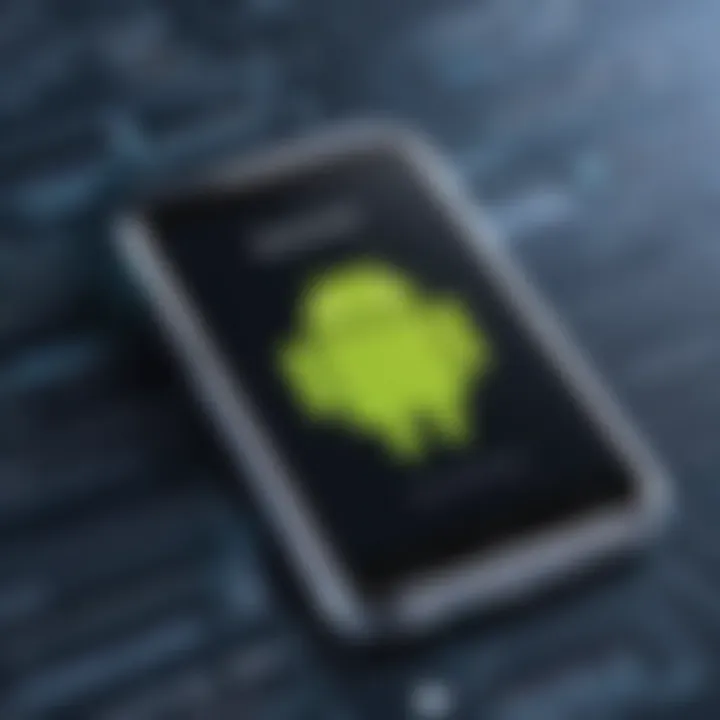
Implementing Code Analysis Tools
Another crucial aspect of Android development in Visual Studio is implementing code analysis tools. Code analysis is the process of reviewing code to identify potential errors, enforce coding standards, and improve code quality. Visual Studio provides several built-in tools that developers can leverage for this purpose, such as Roslyn analyzers and FxCop analyzers.
These tools come with multiple advantages:
- Error Detection: They help in catching common programming pitfalls before they escalate into more significant issues during runtime.
- Standard Compliance: Developers can ensure that their code adheres to established coding guidelines and practices, promoting maintainability and readability over time.
- Refactoring Suggestions: Code analysis tools often provide suggestions for refactoring, which aids in improving code structure and quality.
To implement these tools, a simple setup in Visual Studio allows integration into the project workflow, making it easier for developers to receive feedback on their code as they type. This immediate feedback loop can significantly enhance the learning curve for new programmers while ensuring that seasoned developers maintain high standards.
"The key to successful Android development is not only in writing code but ensuring that the code is clean, efficient and maintains a high standard of quality."
In summary, the tools like the Android Device Monitor and code analysis tools are indispensable in any Android developer's toolkit. They not only support the technical aspects of development but also foster a culture of continuous improvement within the coding practices.
Debugging and Testing Strategies
Debugging and testing are vital components in the landscape of any software development process. They ensure that the application functions correctly, meets user expectations, and maintains performance standards. In Android development with Visual Studio, a judicious approach to debugging and testing can dramatically enhance the quality of mobile applications. Effective strategies can lead to early detection of bugs, ensuring stability and reliability in the final product.
The integration of debugging and testing tools within Visual Studio provides a structured environment for developers. This is particularly significant when working with Android, where device fragmentation can sometimes complicate testing efforts. Understanding common debugging techniques and automating testing processes can save time and resources while reducing the likelihood of post-release issues.
It is important to approach debugging with a mindset of problem-solving and inquiry. The systematic elimination of potential issues allows developers to pinpoint the root causes of defects, fostering a reliable final product.
Common Debugging Techniques in Visual Studio
Within Visual Studio, there are several debugging techniques that developers can utilize to effectively isolate and resolve issues in their Android applications.
- Breakpoints: Setting breakpoints in the code allows developers to pause execution at critical lines of code. This enables step-by-step code execution, providing insights into variable values and runtime states.
- Watch Window: By adding variables to a watch window, it becomes easier to track changes in their values during execution. This helps in understanding how data flows within the application.
- Immediate Window: This tool allows developers to execute commands directly during debugging. By inspecting values or even modifying them on the fly, developers can understand the behavior of their application more thoroughly.
- Debugging Exceptions: Visual Studio can be configured to catch specific exceptions or break on all exceptions. This provides an immediate insight when an error occurs, allowing the developer to analyse the stack trace and understand the issue better.
Debugging is not about proving that you wrote working code; it's about understanding why code behaves the way it does.
- Remote Debugging: For applications running on devices, Visual Studio supports remote debugging. This is particularly useful to debug issues that occur on an actual device rather than an emulator, which can differ in performance and behavior.
Understanding and applying these techniques will lead to more efficient problem-solving in the development cycle and ultimately a more stable application.
Automated Testing Approaches for Android Apps
Automated testing is an essential practice that streamlines the process of validating code at various stages of development. In the context of Android development with Visual Studio, several automated testing approaches offer a robust framework.
- Unit Testing: This involves testing individual components or functions of the application to ensure they perform as expected. Unit test frameworks like NUnit can be integrated into Visual Studio to automate these tests.
- UI Testing: Tools like Appium or Xamarin.UITest can be leveraged for automated UI testing. These tools simulate user interactions to test the application’s interface and ensure it behaves as intended across different screen sizes and orientations.
- Integration Testing: This form of testing focuses on the interactions between various components of the application. Automating this process can help to verify that modules work together seamlessly.
- Continuous Integration/Continuous Deployment (CI/CD): Setting up a CI/CD pipeline can be beneficial for automating the testing and deployment process. Tools such as Azure DevOps can be used to integrate with Visual Studio, allowing automated tests to run every time new code is pushed, ensuring that regressions are caught early.
Utilizing automated testing approaches significantly reduces the time spent on manual testing and enhances the ability to manage complex applications. This creates a reliable workflow in the development process, fostering strong and resilient Android applications.
Performance Optimization Techniques
Performance optimization in Android development is crucial. It ensures that applications run smoothly and efficiently on various devices. When applications are optimized, they use fewer resources, which leads to a better user experience. Users prefer applications that are responsive and do not drain battery life quickly.
Key aspects of performance optimization include resource management, efficient coding practices, and continuous monitoring. In this section, we will discuss two fundamental areas: Monitoring Application Performance and Memory Management Best Practices.
Monitoring Application Performance
Monitoring application performance involves tracking how well your app runs in real-time and under various conditions. Tools like Android Profiler in Visual Studio provide insights into CPU usage, memory consumption, and network activity. Effective monitoring allows you to detect performance bottlenecks early in the development process.
- Identify Performance Issues: Regular monitoring helps recognize areas where speed can be improved. For instance, if the application lags during certain processes, it might be due to excessive resource usage or inefficient algorithms.
- Test Under Various Conditions: It is essential to test your application under different scenarios. Usage patterns can differ widely among users, and your application should perform well regardless of the circumstances.
A critical takeaway here is that continuous performance monitoring should be part of the development lifecycle. Actively tracking performance leads to timely adjustments that enhance the overall quality of the software.
Memory Management Best Practices
Memory management is another pivotal aspect of performance optimization. Poor memory management can lead to issues like low performance, crashes, and a poor user experience.
To improve memory management, consider the following practices:
- Avoid Memory Leaks: Be cautious about unused references. Use weak references where applicable. Regularly review and optimize how your code uses memory resources.
- Proper Usage of Android Lifecycle: Implement and manage components according to their lifecycle. This means releasing resources when an activity is paused and recreating them when necessary to avoid unnecessary memory use.
- Profile Memory Use: Utilize tools within Visual Studio to analyze memory allocation. Identifying which parts of your code consume the most memory can provide direction to improve those areas.
Using proper memory management techniques not only improves app performance but also enhances usability, allowing developers to create robust applications that manage resources wisely.
"Optimization is key to delivering a superior product. It should be considered at every stage of development."
By integrating performance optimization techniques throughout the development process, developers can ensure that their applications are both efficient and user-friendly.
Deployment Considerations
Deployment is a pivotal stage in the Android development lifecycle. This section elaborates on key considerations that developers must navigate when deploying their applications. It encompasses packaging applications for release, ensuring compliance with platform standards, and preparing for distribution. The objective here is to equip developers with knowledge to streamline the deployment process and minimize potential pitfalls.
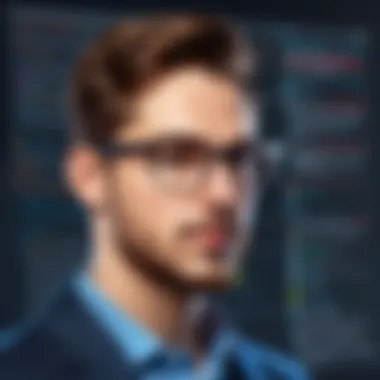
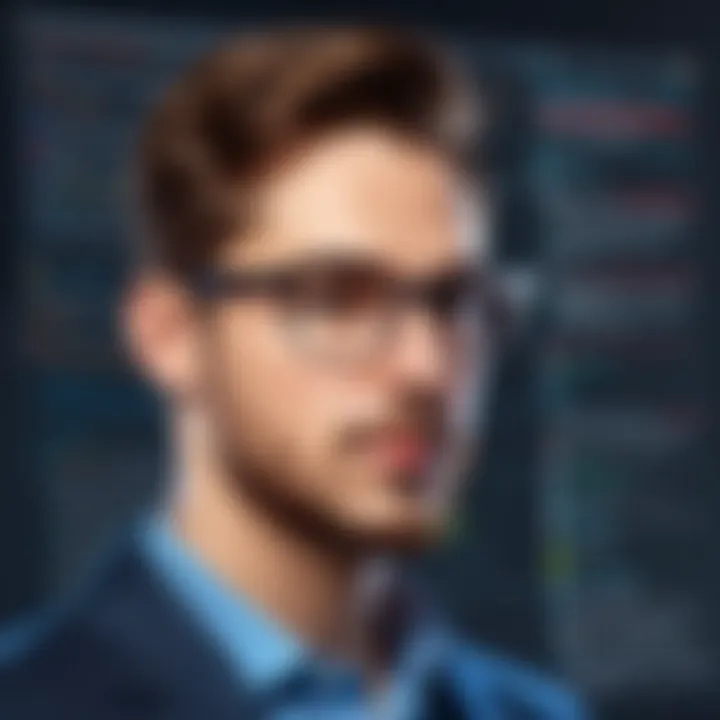
A significant aspect of deployment involves understanding how to package Android applications effectively. This ensures that users receive a functional and optimized version of the application without unnecessary bloat. Proper configuration and testing during packaging mitigates issues that may arise post-deployment. Furthermore, adhering to Google Play Store guidelines plays a crucial role in enhancing app visibility and user experience. A well-structured deployment strategy contributes to an application’s overall success.
Another topic under this section is publishing applications on the Google Play Store. This platform serves as the primary distribution channel for Android apps, thus familiarity with its publishing processes is critical. Carefully analyzing each step helps developers release their applications successfully, increasing reach and user engagement. Ensuring compliant and optimized packaging and knowing the criteria for visibility can boost the chances of an app thriving in a competitive marketplace.
By mastering deployment considerations, developers improve not just their technical acumen, but also their business understanding. This leads to creating applications that are more appealing to end-users.
Packaging Android Applications for Release
Packaging is the process that converts your code into an installable format for Android devices. The most common format is the APK (Android Package Kit). An essential first step is to configure build settings within Visual Studio. This includes specifying the application version, setting the appropriate build type, and enabling all necessary permissions.
When packaging the Android application:
- Use ProGuard: This tool helps in removing unused code and optimizing bytecode.
- Set version code and version name: This identification is critical for updates.
- Shrink your resources: Remove unnecessary resources that bloats the APK size.
- Test the release build: Ensure it functions as intended under real-world conditions.
In Visual Studio, packaging can be accomplished through project properties. Simply navigate to the Android Options, where you can define packaging settings. Once your app is ready for release, a suitable APK file is created.
Publishing Apps on Google Play Store
Publishing on the Google Play Store is a straightforward yet structured process that ensures your app reaches a wide audience. Before publishing, several considerations must be taken into account:
- Account Setup: Create a Google Play Developer account, which has a one-time registration fee.
- Compliance: Ensure that your app conforms to all Google Play policies to avoid rejection.
- Store Listing: Craft an engaging description, choose relevant category, and provide high-quality screenshots.
- Track App Performance Post-Publication: Use the Google Play Console for analytics and user feedback to iteratively improve your app.
The actual publishing process requires uploading your created APK along with filling necessary information. After submission, the app undergoes a review process before going live. The experience of successfully publishing an app can be significant for a developer, marking the culmination of hard work and creativity.
Successful deployment not only involves launching an app but also ensuring its presence in a saturated market with strategic marketing and user engagement.
In summary, focusing on deployment considerations empowers developers to launch their applications effectively and position them for success in the complex Android ecosystem. Understanding both packaging and publishing details reinforces skills crucial for a thriving development career.
Exploring Community and Resources
The exploration of community and resources is vital for anyone delving into Android development with Visual Studio. Community support enhances learning, fosters networking, and facilitates collaboration. Resources provide the necessary knowledge that allows developers to stay updated with trends and best practices. By engaging with both, individuals can significantly enhance their skills and knowledge base.
Online Communities for Android Developers
Online communities serve as a critical lifeline for developers at all levels. These spaces are avenues for sharing insights, troubleshooting, and exchanging ideas. Platforms like Reddit offer subreddit discussions specific to Android development, where users can post questions and receive answers from experienced members. This kind of direct interaction can accelerate learning and problem-solving.
Consider forums such as Stack Overflow, which is specifically designed for developers seeking quick solutions to technical challenges. By browsing through the questions and answers, one can gain insights into common issues faced by others. Another excellent community resource is Facebook groups dedicated to Android development. Here, members share tutorials, code snippets, and project ideas, making it a rich source of inspiration and support.
- Benefits of Online Communities:
- Real-time feedback on development challenges.
- Networking opportunities with like-minded individuals.
- Access to a variety of learning resources and shared experiences.
- Exposure to industry news and best practices.
Utilizing Learning Resources and Documentation
The significance of accessing learning resources and documentation cannot be overstated. Official documentation from Microsoft, particularly for Visual Studio, is an essential asset. It provides in-depth guides on features, best practices, and configurations. This resource helps developers understand the capabilities of Visual Studio within the context of Android development. Further, engaging with tutorials on platforms like Microsoft Learn can provide structured learning paths tailored for Android developers.
Moreover, books and e-books on Android development with Visual Studio are available for those who prefer comprehensive reading. These texts can offer granular insights into coding practices, architectural principles, and even case studies from real-world applications.
Being part of educational platforms like Coursera or Udemy can also be beneficial. These platforms often have specialized courses focused on Visual Studio and Android development, providing opportunities for hands-on learning experiences. Here are some points to remember when utilizing learning resources:
- Key Considerations:
- Ensure the resources are updated to reflect the latest versions of tools and practices.
- Look for community reviews or recommended lists for credibility.
- Balance self-learning with community interaction for well-rounded development.
"Engaging deeply with community and educational resources can be the turning point in a developer's journey, facilitating growth and innovation in their projects."
Through harnessing the strengths of communities and resources, aspiring and experienced programmers alike can navigate the complexities of Android development effectively.
Culmination and Future Trends in Android Development
As we conclude our exploration of Android development with Visual Studio, it is imperative to emphasize the transformative nature of this domain. Android development, particularly using Visual Studio, is not merely about creating applications but about fostering innovation and addressing user needs. The convergence of technology advancements with practical application underscores the future potential of this field.
Emerging Technologies and Their Impact
Emerging technologies are redefining the landscape of Android development. The integration of cloud computing allows for enhanced storage solutions and scalability. With Artificial Intelligence (AI), developers can create more intuitive applications that adapt to user preferences. Furthermore, Internet of Things (IoT) continues to permeate the mobile ecosystem. Apps that interact with various smart devices are on the rise, creating a demand for developers who can navigate these complexities effectively.
"Emerging technologies are not just trends; they shape the future of development in crucial ways."
Blockchain technology also presents exciting opportunities. It enhances security protocols, especially for applications that require transactions. As developers consider these technologies, the focus should be on understanding their mechanisms and implications. This knowledge lays the groundwork for creating robust applications that utilize these innovations wisely.
The Evolving Role of Visual Studio in the Developer Ecosystem
Visual Studio serves as a cornerstone in the developer ecosystem. Its role is evolving to accommodate new development methodologies and tools. The recent updates enhance its collaborative features, allowing teams to work seamlessly—regardless of geographical boundaries.
Moreover, as cross-platform development gains traction, Visual Studio facilitates integration with various frameworks, including Xamarin. This cross-compatibility enhances the productivity of developers, enabling them to work on multiple platforms from a single codebase.
The integration of AI tools within Visual Studio is also noteworthy. These tools streamline coding processes and debugging, allowing developers to focus more on creative problem solving rather than repetitive tasks. As Visual Studio continues to adapt to these trends, it remains a pivotal resource for both novice and experienced developers.
In summary, the future of Android development with Visual Studio is bright. As emerging technologies intertwine with traditional development practices, the scope for innovation and improvement remains vast. Developers equipped with the knowledge and skills to adapt to these changes will undoubtedly lead the charge in this dynamic field.