Exploring Push Notifications in Android Without Firebase
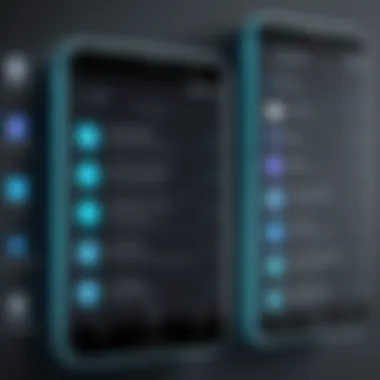
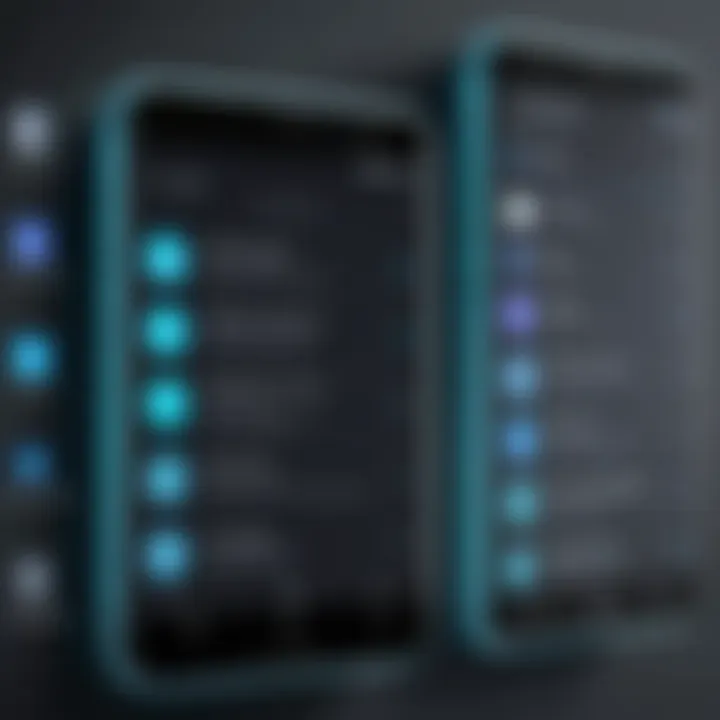
Intro
In the ever-evolving landscape of mobile app development, the ability to send push notifications is a critical feature that enhances user engagement. For many developers, Firebase provides a straightforward solution, but it isn't the only route to explore. Implementing push notifications without Firebase opens doors to a multitude of alternatives, each coming with its own set of challenges and benefits.
This guide focuses on methods that leverage REST APIs, WebSockets, and local mechanisms to create a seamless notification experience on Android devices. It's a brave new world out there, and as more developers seek to break free from Firebase's infrastructure, exploring different avenues becomes vital.
From performance considerations to security implications, this article aims to equip you with a comprehensive understanding of how to effectively implement push notifications in your Android applications. Letās dive in.
Intro to Push Notifications on Android
Push notifications have evolved into a vital tool for engaging users in todayās mobile-driven world. Understanding their role in Android applications is essential, especially if one wishes to implement a system without relying on Firebase. Mobile apps face fierce competition for user attention, and notifications serve as a powerful ally to keep an app at the forefront of users' minds. They bridge the gap between the app and the user, offering timely updates, reminders, and personalized content that can significantly enhance user experience.
When developers create a push notification system, they must consider factors such as user interaction, message delivery reliability, and the potential for notifications to impact device performance.
Understanding Push Notifications
At its core, a push notification is a message sent from a server to a user's device, alerting them about some content or event related to the app. Unlike traditional SMS or email, these notifications can reach users directly on their home screen, allowing for better visibility and immediacy.
The mechanism behind push notifications often involves a request-response protocol where the app registers with a push service, obtaining unique tokens for message delivery. These tokens act as an address, helping the server reach the intended device. The beauty of push notifications lies in their ability to convey crucial information in a concise manner, making them an effective means of communication.
A key point to remember is that push notifications require an established internet connection. Without it, users may miss important updates, which can be frustrating. Developers must weigh the pros and cons of this approach, especially in areas with poor connectivity.
Common Uses of Push Notifications
Push notifications aren't just a digital nudge; they can serve numerous practical purposes across various industries. Hereās a closer look at how theyāre commonly utilized:
- Marketing Promotions: Retail and e-commerce apps use notifications to send out discounts, sales alerts, and personalized recommendations based on user behavior. This can entice users to return to the app and make a purchase.
- Content Updates: News applications frequently push notifications to alert users about breaking news or important updates, ensuring they stay informed.
- Reminders and Alerts: Calendar and task management apps leverage notifications to remind users of appointments, deadlines, and important eventsāall aimed at enhancing productivity.
- Social Interactions: Social media platforms send notifications when someone likes, comments, or shares a post, helping to stimulate user engagement and interaction within the community.
- User Engagement: Many gaming apps use push notifications to remind players about events, achievements, or invitations from friends, helping companies maximize user retention.
These examples illustrate that when used judiciously, push notifications can create a positive user experience and foster stronger engagement with the app. The trick is to stride the fine line between being informative and overwhelming.
In summary, understanding push notifications is fundamental when developing for Android, especially when considering alternatives to Firebase. The power of notifications lies in their ability to connect, inform, and inspire users to act.
The Role of Firebase in Android Notifications
Firebase has carved a niche in the realm of mobile development, particularly with push notifications. Its prominence stems from Firebase Cloud Messaging (FCM), a robust service that facilitates the effortless transmission of notifications to Android devices. Understanding its role is paramount, especially for developers contemplating alternatives.
Firebase provides a powerful suite of tools for developers looking to engage users through notifications. With FCM, sending messages becomes a cakewalk, thanks to its integration with various Firebase services. For example, you can easily sync notifications with analytics tools, tailoring your messages based on user behavior. This offers significant advantages in creating targeted and effective engagement strategies.
However, while Firebase is a robust solution, it isn't a one-size-fits-all answer. The service brings forth certain complexities and considerations, especially when dealing with sensitive applications or custom needs. Itās crucial to weigh these elements before committing to Firebase alone.
Advantages of Firebase Cloud Messaging
- Seamless Integration: FCM is designed to work hand-in-hand with other Firebase services. This allows developers to create a more unified application environment. The integration helps in measuring analytics on notification interactions, leading to better strategies.
- Scalability: For applications with growing user bases, FCM offers a scalable solution where the infrastructure handles increased traffic effectively. Developers can send messages without worrying about the underlying mechanics.
- Versatile Messaging Options: Firebase allows both upstream and downstream messaging. Developers can send messages from the server to the client and vice versa. Moreover, notifications can be sent not just to Android but also across other platforms, such as iOS and web applications. This makes it a versatile option for multi-platform apps.
- Free Tier Availability: Firebase provides a generous free tier, making it accessible for startups and independent developers looking to implement push notifications without a hefty investment upfront.
In summary, Firebase Cloud Messaging presents developers with a convenient and extensive toolkit for implementing notifications, fostering user engagement. Yet, developers should recognize that the simplicity may come at the cost of flexibility, particularly in niche scenarios.
Limitations of Firebase for Certain Applications
While Firebase presents a solid case for being a go-to solution, itās essential to acknowledge its limitations. Not every application will benefit from its features, and in some cases, it may even introduce constraints that could hinder development.
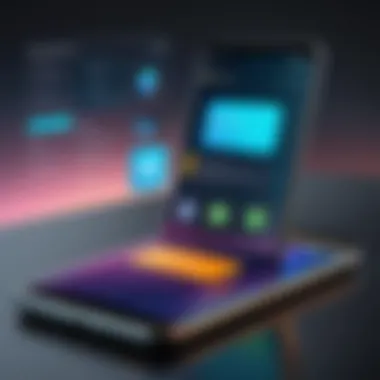
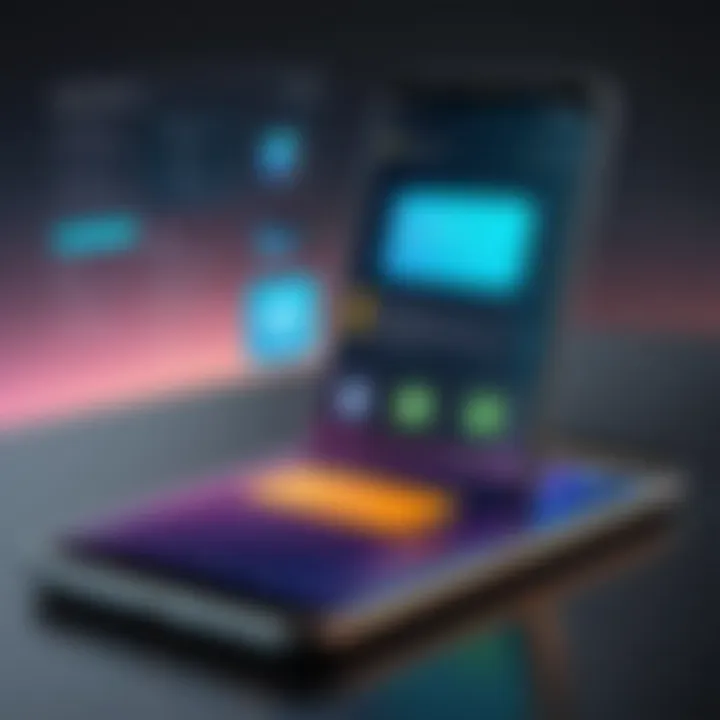
- Dependency on Internet Connection: FCM relies heavily on internet connectivity to deliver notifications. For applications that must operate offline or in low connectivity areas, this presents a significant drawback.
- Privacy Concerns: Data privacy continues to be a topic of concern for many users. When using Firebase, user data is processed through Googleās infrastructure, which may not align with the data protection policies of certain organizations, particularly in regions with strict regulations.
- Limited Customization Options: While FCM excels at basic notification functionalities, advanced customization can be a burden to achieve. Developers seeking distinctive designs and interactive functionalities might find FCM lacking in certain areas.
- Potential Learning Curve: Even though FCM is built for ease of use, integrating it with other Firebase features may require some learning, thus delaying development for new users.
Overall, while Firebase provides a powerful push notifications framework, it is imperative that developers assess their specific needs against its limitations. For some use cases, employing alternatives may provide greater flexibility and control.
Alternatives to Firebase for Android Push Notifications
The realm of push notifications is as vast as it is vital for modern mobile applications. While Firebase provides a robust solution for many, relying solely on it can limit creativity and flexibility. Exploring alternatives to Firebase for Android push notifications opens new doors for developers looking to tailor their implementations to meet specific project requirements. The alternatives not only empower developers but also enhance the user experience through customized notifications that resonate more with the target audience. It's a bit like having a toolboxādifferent tools serve different purposes.
Using REST APIs for Notifications
Designing a REST API
Designing a REST API is a fundamental step in providing a seamless notification service. A well-architected REST API stands out due to its stateless interactions and reliance on standard HTTP methods. This makes it easier to manage and scale as user demands grow, and lets developers focus on building robust applications without being bogged down by backend complexities. Incorporating security features such as OAuth for ensuring safe communication can further bolster the architecture. However, it comes with its own baggage such as requiring additional setup and maintenance efforts.
Integration with Android
When talking about integrating a REST API with Android, it's all about establishing easy, efficient communication channels between your app and the API. By using libraries like Retrofit or Volley, developers can make the interaction feel as seamless as a well-oiled machine. This integration allows for real-time notifications, which are crucial for user engagement. While itās a popular choice, developers should be aware that network latency can sometimes lead to delays in notifications, which may hinder the user experience.
Example Code Snippet
Providing an example code snippet is like giving a lifebuoy to someone who is swimming against the tide of confusion. A simple code snippet to trigger a notification could look something like this:
This snippet effectively illustrates how developers can trigger notifications through a REST API call. The clarity of implementation is one of its most appealing aspects, but itās important to also note that a robust backend is necessary to handle a large influx of notifications.
Implementing WebSockets
Setting Up a WebSocket Server
Setting up a WebSocket server provides a real-time communication pathway, which is ideal for applications requiring instant updates. The key characteristic is its ability to maintain a persistent connection, unlike traditional HTTP requests that are more transactional. This persistent nature allows for a constant flow of informationālike a river that never runs dry. However, creating a WebSocket server demands a good understanding of sockets and protocol handling which might be a hurdle for some.
Handling WebSocket Connections
Effectively managing WebSocket connections can be seen as conducting an orchestraāeach connection needs to be properly maintained for the music to flow harmoniously. The asynchronous nature of WebSocket means messages can be sent and received without the back-and-forth handshake typical of HTTP. This allows for speedy notifications which are vital in applications such as chat apps or live score updates, but it also means that developers must implement robust error handling to avoid disruptions in service.
Pros and Cons of WebSockets
WebSockets bring a unique set of advantages and disadvantages to the table. On one side, their capacity for real-time communication attracts many developers who are after immediacy in their apps. But on the flip side, they can introduce complexities like handling user load and ensuring security, since each socket connection remains open. Determining whether WebSockets are right for your app often boils down to a strategic evaluation of these pros and cons.
Local Notifications in Android
Creating Local Notification Channels
Creating local notification channels is a crucial step for managing how notifications appear on a device. With Android's notification intents, developers can craft distinct channels for different types of notificationsālike setting different playlists for different moods. This enhances user control over what notifications they receive and ensures they can tailor their experience. However, one downside is that developers need to manage these channels effectively, which may require additional code and testing to get right.
Triggering Notifications
Triggering notifications locally might sound simple, but thereās more to it beneath the surface. Developers can schedule notifications to appear at specific times or trigger them based on other events within the app. This adaptability often leads to higher user engagement, yet it is crucial to give careful consideration to timing and the relevance of the notifications. Too many notifications can overwhelm users, leading to them disabling notifications altogether.
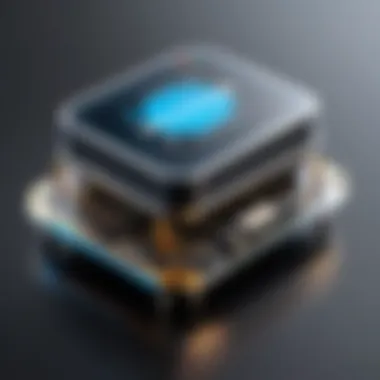
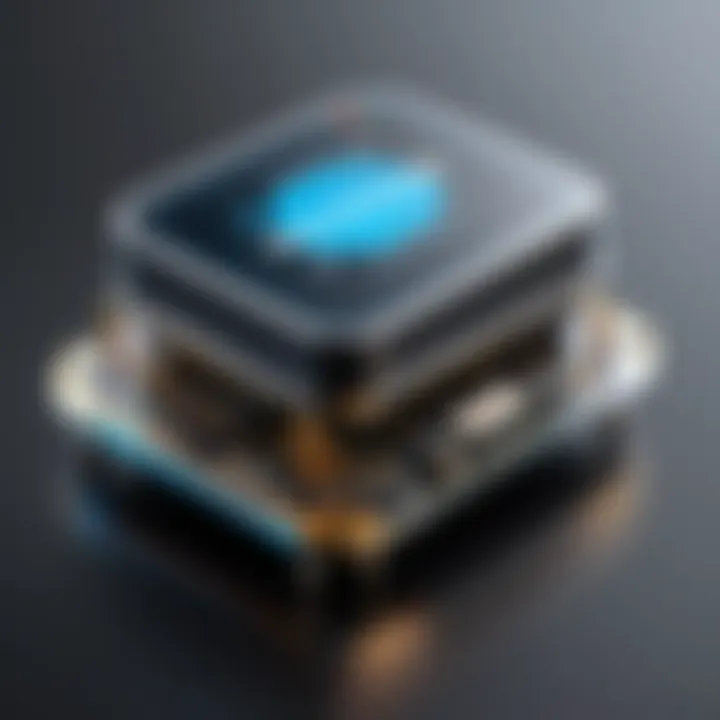
Restrictions on Local Notifications
Understanding the restrictions on local notifications is crucial to avoid running into situations where notifications fail to deliver or appear incorrectly. Things such as user settings, device limitations, or permissions can affect how and when notifications are shown. Being aware of these nuances helps developers to strategize effectively, leading to a smoother user experience. All in all, while local notifications offer a quick and versatile solution, staying on top of these restrictions is key to maintaining effectiveness.
Security Considerations
In the landscape of mobile applications, pushing notifications stands out as an essential feature, but itās not just about delivering messages. When implementing Android push notifications, security considerations cannot be an afterthought. Without the safety net provided by services like Firebase, developers must tread carefully to ensure that the communication channels between their servers and the devices remain secure. Additionally, protecting user privacy and managing permissions are fundamental aspects that can significantly influence user trust and app adoption.
Data Security in Message Transmission
When it comes to transmitting messages, ensuring the security of data in transit is paramount. Utilizing encryption protocols such as HTTPS is a good starting point. This method protects data from being intercepted during transmission. Here are some key aspects to consider:
- Use TLS/SSL: Always encrypt your data using Transport Layer Security (TLS) or Secure Sockets Layer (SSL). This will guard against eavesdropping and man-in-the-middle attacks, which are all too common in today's digital realm.
- Payload Sanitization: Validate and sanitize any data sent in notifications. Malicious users often attempt to inject harmful payloads into notifications to exploit vulnerabilities in the application.
- Implement Token Authentication: Instead of relying on static keys, use dynamic tokens for authentication to ensure that only legitimate devices receive notifications. This reduces risks associated with stolen credentials.
Employing these strategies not only secures the data but also builds a robust foundation for trust, which is crucial when users interact with your application.
User Privacy and Permissions
As data privacy regulations become increasingly stringent, developers must prioritize user privacy when it comes to push notifications. Obtaining explicit permission from users is not only a good practice, but also often a legal requirement under various privacy laws such as GDPR or CCPA.
To enhance user privacy, consider the following approaches:
- Clearly Define Permissions: When requesting permission to send notifications, be transparent about why the app needs this access. Users appreciate clarity and are more inclined to grant permissions when they feel informed.
- Offer Customization: Allow users to customize their notification settings. Giving them control over what they receive and when can lead to a better user experience.
- Regularly Review Permissions: Make it easy for users to modify the permission settings from within the app. This empowers users to feel secure about their data.
Protecting user privacy not only complies with legal requirements but also fosters a loyal user base. Itās about creating an environment that respects individual rights, which, in turn, can directly affect the longevity of your app in a competitive market.
"In the age of information, data security is not just a necessity; itās a fundamental right."
Incorporating these considerations ensures that you are on the right path. As developers, our duty extends beyond functionality; it fundamentally includes the trust we build with our users.
Challenges in Implementing Custom Solutions
In the journey of developing push notifications on Android without Firebase, several challenges may arise. These challenges are critical to consider as they can impact the effectiveness and reliability of your notification system. A well-thought-out approach will help mitigate issues and enhance user experience. Here, we will delve into specific elements of these challenges and their considerations, focusing on dealing with message delivery failures and device-specific issues.
Handling Message Delivery Failures
Message delivery failures can be downright frustrating. When users opt in for notifications, they expect timely communication, whether itās a message from a friend or an update on their favorite app. However, several factors can disrupt this seamless flow.
- Network Reliability: Not every user will have a stable internet connection. Messages might pile up in the queue when a user is on a poor network, resulting in delivery absences when connectivity returns.
- Server Overload: If your notification server is running at full capacity or experiences downtime, messages may not reach their destination. This can happen during peak hours, causing a backlog of notifications.
- Device Settings: Users might inadvertently mute notifications or restrict background data for specific apps. It's important to educate users on managing their device settings to ensure they remain in the loop.
To combat these issues, implementing robust fallback mechanisms can be a lifesaver. For instance, consider retrying message delivery after a set period or utilizing a delay strategy, which can significantly reduce the impact of these failures. A summary of key strategies includes:
- Implementing exponential backoff for retries.
- Providing user feedback on delivery status can help maintain transparency.
- Ensuring your server infrastructure can auto-scale to handle spikes in traffic.
"Itās not just about sending messages; it's about ensuring they are received and understood."
Dealing with Device-Specific Issues
In the world of Android, fragmentation is both a blessing and a curse. Different manufacturers and versions of Android operating systems result in varied behaviors. This can complicate your notification implementation strategy.
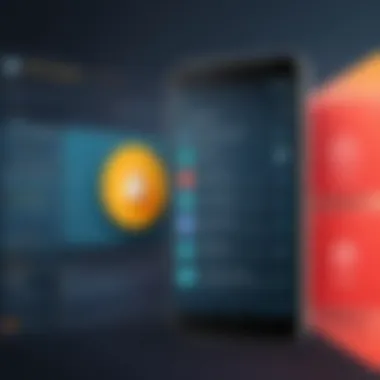
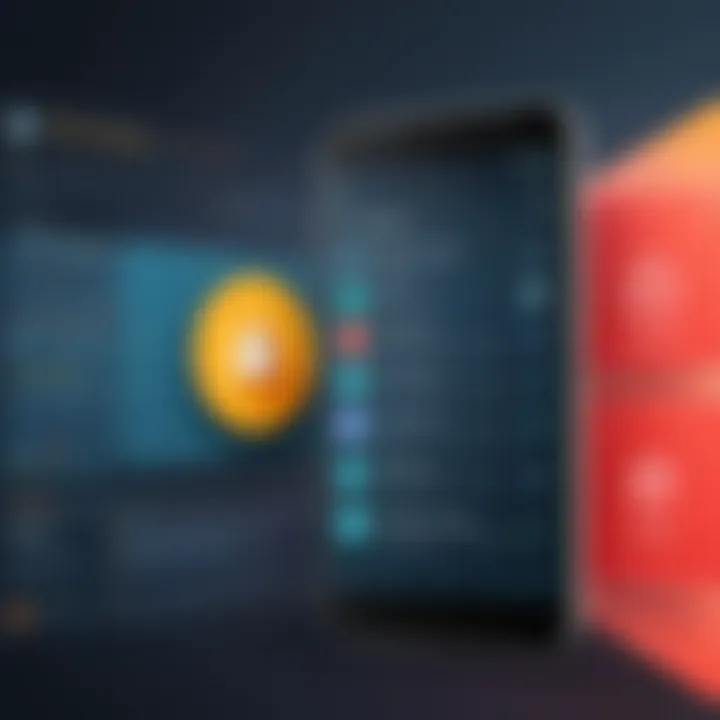
- OS Versions: Not all users are on the latest Android version. Features available in the latest release may not work on older versions, leading to inconsistent user experiences.
- Custom OEM Implementations: Manufacturers like Samsung, Xiaomi, and Huawei sometimes modify how notifications function, which could cause unexpected behavior in your app.
- Permissions and User Preferences: Users may have personalization features that differ across devices, affecting how, or whether, they receive notifications. This can range from modifying notification tones to changing display settings.
To address these issues, conducting thorough testing across a range of devices and OS versions is paramount. Creating a compatibility matrix that outlines the notification compatibility with different versions and manufacturers can also be beneficial.
In summary, a few practical tactics include:
- Establishing clear documentation for users regarding compatible devices.
- Regular updates and patches for your notification logic to adapt to new Android features.
- Utilizing analytics to understand which versions and devices most of your users use.
Through careful navigation of these challenges, developers can significantly improve the robustness and reach of their custom push notification implementations. By being proactive in identifying and correcting potential issues, youāll ensure a smoother notification experience for users.
Best Practices for Notifications
When it comes to sending push notifications, adhering to a set of best practices is crucial for ensuring that your messages not only reach your users but also engage them effectively. Well-crafted notifications can significantly impact user engagement and retention. But how do you navigate the minefield of notification strategies without falling into the traps of annoyance and irrelevance? Let's delve into this topic.
Designing Effective Notification Messages
Creating effective notification messages demands a careful balance between clarity and brevity. Users typically scan notifications quickly; therefore, itās essential that the message conveys the intended information in as few words as possible. Consider using an active voice. Instead of saying, "Your discount is available now," you might opt for, "Claim your discount now!" This small change adds an element of urgency and clarity.
It's key to keep the content relevant. Context matters. For instance, sending a notification about a flash sale on warm clothing during a summer heatwave might not trigger the interest you seek. Target your audience's immediate interests based on their previous interactions with your application.
Incorporate rich media where feasibleāimages or action buttons can heighten engagement. According to some studies, users are more inclined to interact with a notification that includes a visual element.
Also, consider personalizing messages. Users appreciate when it feels like a notification is tailored just for them. A simple, "Hey Sarah, your favorite shoes are on sale," can go a long way compared to a generic message. Using data analytics to understand user behavior can assist in crafting messages that resonate with them.
"Effective push notifications respect the userās space while inviting them back to engage."
User Customization Options
A key aspect of user satisfaction with push notifications is offering customization options. Not everyone wants the same experience, and providing users with the freedom to choose their notification preferences can greatly enhance user satisfaction. This includes allowing users to select the types of notifications they want to receiveānews updates, offers, reminders, or even personalized content.
Beyond types, users should also have a say in the frequency of notifications. Bombarding users with too many messages can lead to annoyance and, subsequently, uninstalls. Therefore, giving users the power to adjust settings according to their comfort level can maintain user interest and minimize churn.
Consider implementing a simple settings menu within your app, where users can manage alerts easily. Options might include:
- Mute notifications for a period
- Opt into specific topics or types
- Receive a summary notification instead of individual messages
This level of control not only increases user trust but also makes them more likely to stay engaged with your application. After all, when users feel they are in control, they are more likely to enjoy their interaction with your app.
In sum, the best practices for notifications revolve around creating content that is concise, relevant, and personalized while offering users options to tailor their experience. By following these guidelines, you can foster a healthier relationship between your application and its users, all while ensuring effective communication.
The End
In wrapping up the discussion on implementing push notifications in Android without the crutch of Firebase, itās crucial to reflect on the key elements that make these alternatives not just feasible, but often beneficial. Understanding that developers can harness the power of push notifications through REST APIs, WebSockets, and local notifications opens up a world of flexibility. The versatility of these methods allows for tailoring solutions that match specific application needs perfectly.
Recap of Key Points
When it comes to summarizing the insights shared throughout this article, a handful of points stand out:
- Diverse Alternatives: Developers are not limited to Firebase but have options like REST APIs and WebSockets that cater to varying requirements.
- Security Matters: It's essential to address data privacy and security by implementing encryption for data transmission.
- Customization: Users appreciate the ability to customize their notification settings, and apps that offer this feature see better engagement.
- Challenges Ahead: Implementing custom solutions comes with its own set of challenges like handling message failures and addressing device-specific quirks.
Recapping these points drives home the importance of adaptability in the ever-evolving tech landscape.
Future Directions in Push Notification Technology
As push notification technology continues to mature, several trends are worth keeping an eye on:
- Increased Personalization: Notifications will likely become more user-centric, with the help of machine learning algorithms that offer tailored content.
- Integration of AI: With the rise of artificial intelligence, companies are exploring how to predict user behavior to enhance notification relevance.
- Cross-Platform Solutions: Future developments may lean towards unified solutions that allow notifications across multiple operating systems without major discrepancies.
- Focus on User Experience: As users grow more discerning, a shift towards less intrusive, more meaningful notifications will likely become the norm.