Mastering App Development with Java: A Deep Dive
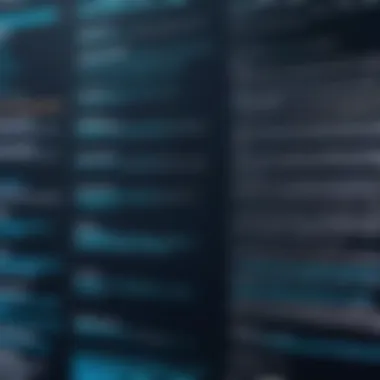
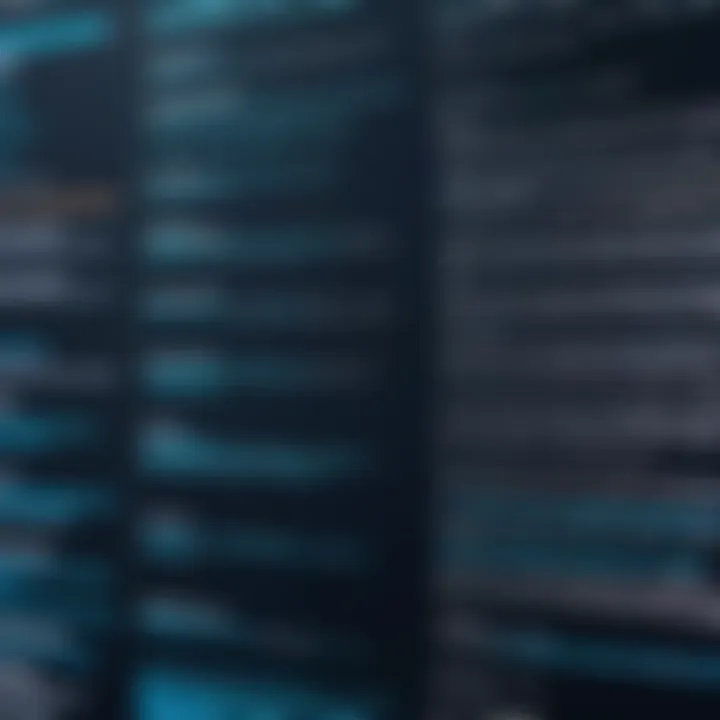
Intro
Java is a powerful programming language well-regarded for its versatility and depth, making it an optimal choice for app development across various platforms. This article covers the complex topics related to app development using Java, addressing both fundamental and advanced aspects.
With its rich ecosystem of frameworks and tools, developers can create mobile applications, web services, and enterprise solutions seamlessly. Moreover, Java continues to evolve, adapting to current technological demands.
Our detailed exploration will span essential elements such as coding challenges, current technology trends, valued resources, and core computer science concepts grounding Java's capabilities. Whether you are an experienced programmer or just beginning, this guide will provide insightful knowledge conducive to both skill enhancement and practical application.
Coding Challenges
Weekly Coding Challenges
In the realm of programming, maintaining consistent skill improvement is crucial. Weekly coding challenges provide a platform for developers to refine their coding proficiency by engaging with practical problems. Various platforms, such as HackerRank and CodeSignal, offer Java-specific challenges that help build and test your foundational skills.
Problem Solutions and Explanations
Engaging with coding challenges like these will lead you to various problem solutions. Understanding those solutions enhances your problem-solving abilities. By dissecting the logic and approaches taken to solve these issues, developers gain deeper insights into Java’s intricate functions.
Tips and Strategies for Coding Challenges
Solving coding challenges effectively requires certain strategies. One must:
- Understand the problem clearly
- Start with a brute force solution and optimize later
- Test solutions with various edge cases
- Read others’ work to gain new perspectives
Through thoughtful analysis of these steps, developers enhance their coding efficiency.
Community Participation Highlights
Focus on participating in developer communities through platforms like Reddit and Stack Overflow. Discussing coding concepts and sharing solutions can lead to new insights and foster relationships with peers engaged in similar pursuits.
Technology Trends
Latest Technological Innovations
As the tech landscape shifts, understanding recent technological innovations is imperative. Java remains relevant as it evolves alongside emerging technologies, such as cloud computing and blockchain.
Emerging Technologies to Watch
Be aware of upcoming trends like reactive programming and microservices architecture. Their adoption is boosting application efficiency and performance without sacrificing reliability.
Technology Impact on Society
The application of these emerging technologies affects society – from improving how businesses operate to enhancing user experiences. Java’s adaptability places it as a fundamental tool in addressing these societal changes.
Expert Opinions and Analysis
Insights from seasoned developers provide a compass for understanding these technological dynamics. Following thought leaders in Java through blogs and social media can toll your ability to foresee where the language and its applications will trend.
Coding Resources
Programming Language Guides
Developers seeking clarity can benefit from guides on distinct Java features. These texts provide structured ways of approaching large-scale software outcomes while considering best practices of the language.
Tools and Software Reviews
Software tools such as IntelliJ IDEA and Eclipse play pivotal roles in Java development. Comprehensive reviews help choose the right tools that suit individual or project needs.
Tutorials and How-To Articles
Online tutorials offer hands-on experience. They cover relevant Java frameworks, such as Spring and Hibernate, ensuring developers can navigate these assets effectively.
Online Learning Platforms Comparison
Platforms like Coursera, Udemy, and edX differ in delivery style and curriculum quality. Evaluating them contributes to advancing your development journey.
Computer Science Concepts
Algorithms and Data Structures Primers
Solid foundation in computer science principles, especially algorithms and data structures, are crucial. Understanding these optimizes Java app development significantly, ultimately improving performance.
Artificial Intelligence and Machine Learning Basics
As AI and ML grow, so does the integration of these technologies with Java. Gaining knowledge in these areas broadens the horizons of any app developer.
Networking and Security Fundamentals
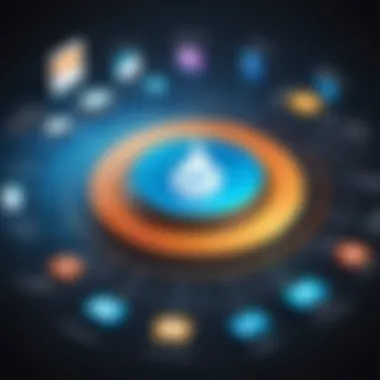
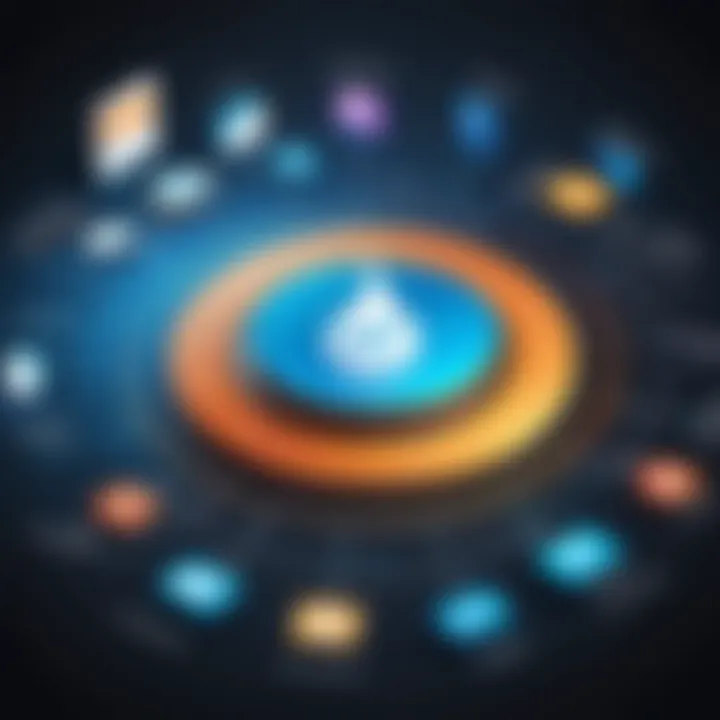
With more applications connected over networks, graps of networking principles and security is vital. Java supports the development of secure applications; thus, understanding these fundamentals enhances one’s credibility.
Quantum Computing and Future Technologies
The landscape of computer sciences is transitioning to integrate quantum computing. Java's adaptability may play a crucial role in building applications in this revolutionary arena.
Java remains a potent tool for designing resilient applications. By engaging actively with the coding community, you can consistently enhance your skills, keeping abreast of prevailing trends and expanding your horizons.
Preface to Java in App Development
Java has carved a significant niche in the app development landscape. Its platform independence, rich ecosystem, and robust performance contribute to its ongoing relevance. This section addresses Java’s importance, specifically within modern applications. It focuses on elements that accentuate Java's capabilities, the benefits for developers, and critical considerations in its adoption.
The Evolution of Java
Java originated in the mid-1990s, designed to be a versatile programming language suitable for a wide range of applications. Its early implementation laid the groundwork for portability, which allowed developers to run applications on multiple platforms without modifications. Over time, Java has seen numerous updates, enhancing its functionality and efficiency. Key milestones include:
- Java 1.0 (1996): The first version, focused on layout and functionality.
- Java 2 (1998): Introduced Swing for rich GUI development and the Collections Framework.
- Java 5 (2004): Brought generics, annotations, and enumerations to enhance type safety.
- Java 8 (2014): Added lambda expressions and the Stream API, making programming more intuitive and efficient.
These updates helped foster community engagement around Java, ensuring it remains a preferred choice when handling complex and scalable applications.
Java's Role in Modern Application Development
Java plays a significant role in the contemporary app development scene. It offers a multitude of frameworks and libraries that streamline complex tasks. Its key contributions include:
- Versatility: It powers everything from mobile applications on Android to enterprise-level applications.
- Rich Ecosystem: Java provides mature tools like Spring, Hibernate, and Java EE, which mitigate development challenges and increase productivity.
- Strong Community Support: With a vast number of developers globally, there’s a wealth of shared knowledge, documentation, and forums available to troubleshoot and expand skills.
Java’s stability and adaptability make it uniquely positioned to meet various development needs. Its continuing evolution cannot be overlooked; adapting to changing technology demands has solidified its place as a long-term choice for developers.
Fundamental Concepts of Java Programming
Understanding the fundamental concepts of Java programming is essential for anyone venturing into app development with Java. These basic elements are crucial because they establish a strong foundation, ensuring developers can construct applications that are efficient and maintainable. Mastering the syntax, semantics, object-oriented principles, and data structures equips both novice and experienced programmers with the skills needed to tackle complex projects. In this section, we will delve into these fundamental aspects—with clarity on their significance and practical applications also noted.
Syntax and Semantics
The syntax is the set of rules that define the combinations of symbols that are correctly structured programs. In simple terms, it is akin to grammar in a spoken language. Adhering to syntax rules is non-negotiable; otherwise, the code will not compile. Java has a clear and strict syntax, which is one of the reasons it is favored in education.
Is it only important to learn the syntax? No. Equally vital is semantics, which refers to the meaning of those syntactical constructs—how the specific combination of words in the code translates to action when run on the Java Virtual Machine (JVM). Developers must understand not just how to write valid syntax but also what that syntax means in the context of programming logic.
For example, the command correctly creates a variable named with the integer value of 5. But until a programmer understands what class means, how data is actually stored, and how basic data types interact, they remain limited in effectiveness.
Object-Oriented Programming Principles
Java is built on the principles of object-oriented programming (OOP). These include encapsulation, inheritance, polymorphism, and abstraction. Each of these concepts plays a pivotal role in software development:
- Encapsulation: This hides the internal object details and only exposes the features and functions that developers need.
- Inheritance: This allows one class to derive properties and behaviors from another class, leading to code reuse.
- Polymorphism: This feature enables objects to be treated as instances of their parent class while allowing for method overriding.
- Abstraction: This focuses on exposing the essential features and ignoring the complex details.
These principles lead to clean and reusable code, making it understandable and manageable, particularly in larger applications. They also drive a designer’s ability to model real-world components seamlessly.
Data Structures in Java
Effective programming also relies heavily on understanding data structures, which are fundamental components for storing and organizing data in a program. Java offers various built-in data structures that are critical when developing applications.
Some common Java data structures include:
- Arrays: A fundamental structure that holds a fixed-size sequence of elements.
- ArrayList: A resizable array implementation from the Java Collections Framework.
- LinkedList: A linear data structure that consists of nodes where each node points to the next node.
- HashMap: A collection that stores items in key-value pairs, ideal for quickly accessing data.
- Stack and Queue: LIFO and FIFO structures that are beneficial in numerous algorithms.
Understanding these structures helps developers apply the right efficiency tools, enhance data retrieval time, and effectively manage memory. Choosing the most appropriate data structure for a specific scenario can drastically boost application performance.
The mastery of fundamental concepts solidifies your ability to design robust applications in Java, ensuring clarity, efficiency, and maintainability in programming tasks.
Common Java Development Tools
In the realm of Java application development, tools play a vital role in ensuring that the process is efficient, organized, and fruitful. These tools not only help streamline workflow but also enhance productivity, making it essential for developers to utilize them effectively. Understanding these tools is fundamental for both aspiring and seasoned programmers, as they shape the overall programming experience.
Integrated Development Environments (IDEs)
Integrated Development Environments, or IDEs, provide a comprehensive platform for developers to write, test, and debug their Java code. IDEs combine various development tools into a single, user-friendly interface. This integration brings many benefits such as syntax highlighting, code completion, and runtime error identification.
Popular IDEs like IntelliJ IDEA, Eclipse, and NetBeans are commonly used in the Java community. Each offers unique features:
- IntelliJ IDEA is well-liked for its intelligent code assistance and refactoring capabilities.
- Eclipse provides a versatile environment often favored for plugin development due to its extensibility.
- NetBeans is known for its simplicity, making it beginner-friendly while supporting full coding support.
Utilizing an IDE can significantly decrease the time you spend debugging and writing code. Enhanced navigation features such as automatic indexing allow developers to jump to function definitions quickly, thus improving productivity.
Build Automation Tools
Build automation tools are critical for managing the lifecycle of applications, particularly as projects grow in complexity. These tools automate tasks such as compiling source code, packaging binaries, running tests, and deploying software. Automated builds reduce the likelihood of human error and ensure consistent deployment from development to production.
Common tools include Apache Maven and Gradle. Maven focuses on project management, emphasizing standardized project structure and dependencies. Gradle, on the other hand, combines features of both Maven and Ant, allowing for custom scripts that tailor the build process to specific project requirements.
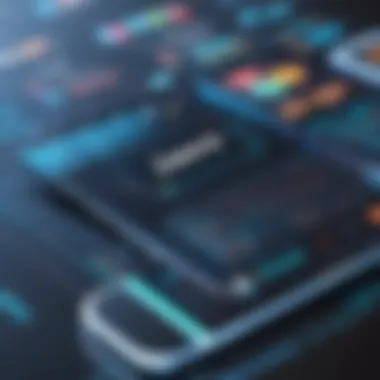
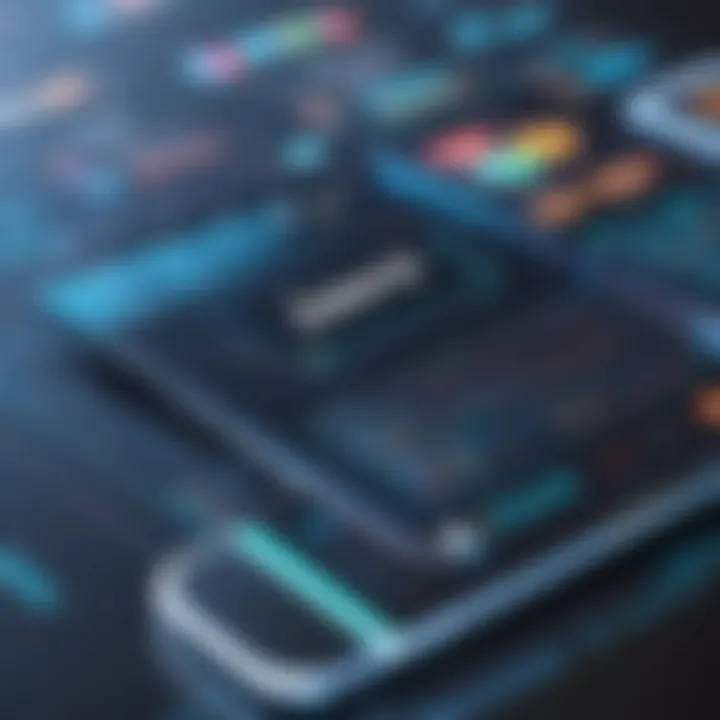
Using such tools also opens avenues for continuous integration and delivery. Therefore, they are no longer optional but essential for efficient project turnaround.
Version Control Systems
Version control systems (VCS) are indispensable in modern software development. They enable programmers to track changes, revert to previous states, and collaborate more effectively. In Java programming, using a VCS is critical for handling code alterations across team members.
Git is one widely-used system that allows multiple developers to work on the same project without conflicts. Popular hosting services, like GitHub and GitLab, facilitate code sharing and project management.
Using VCS reduces the potential for data loss and fosters transparency within the coding environment.
In summary, relying on these common Java development tools positions developers for success, regardless of their experience level. They allow programmers to focus more on writing code instead of menial tasks, ultimately contributing to higher quality applications and quicker delivery to users.
Employing common tools not only improves code management but enhances the collaborative experience, cultivating a cohesive development culture.
Frameworks for Java Application Development
Frameworks play a critical role in Java application development, providing a structured foundation upon which developers can build robust applications. They offer pre-written code, components, and tools, enabling developers to focus on the unique aspects of their application rather than dealing extensively with the underlying codebase. In this section, we will delve into three significant frameworks used in Java application development: Spring Framework, JavaServer Faces (JSF), and Hibernate, examining their specific advantages and common use cases.
Spring Framework
The Spring Framework is widely recognized for its ability to simplify enterprise application development. It is modular and flexible, allowing developers to use only the parts they need. This core feature addresses the concerns of overhead and resource management while encouraging clean code organization through Inversion of Control (IoC) and Aspect-Oriented Programming (AOP).
Some key benefits include:
- Integration: Spring integrates smoothly with various data access technologies, like JDBC and ORM frameworks.
- Dependency Injection: This design pattern helps in creating loosely coupled applications, making maintenance easier.
- Microservices Support: It has become central to developing microservices, supporting scalability and manageability.
Example Code Snippet
This illustrates how easily objects can be managed with the Spring framework.
JavaServer Faces (JSF)
JavaServer Faces is primarily focused on facilitating user interface development for Java applications. It follows a component-based architecture, allowing developers to create user interfaces through reusable UI components. This framework integrates well with various JSF libraries and supports seamless data-driven application development.
Key features include:
- Rich Component Set: JSF comes equipped with a vast array of pre-built UI components, which enhances the graphical user interface.
- Ajax Support: The framework supports Ajax, enabling dynamic web applications and improving user experience.
- Standardization: JSF adheres to Java EE standards, which eases the integration process within various Java enterprise environments.
Hibernate for Database Management
Hibernate is a prominent Object-Relational Mapping (ORM) tool in Java, simplifying database interactions. The main goal of Hibernate is to bridge the gap between database tables and Java objects, allowing developers to work at a higher abstraction level without needing to understand the underlying complexities of SQL.
Advantages of using Hibernate include:
- Database Independence: Code becomes more portable as Hibernate can work with multiple databases without needing significant changes.
- Lazy Loading: This feature improves performance by loading child objects only when needed, which expands application efficiency.
- Caching: Hibernate offers a sophisticated caching mechanism, enabling better resource allocation and faster query execution.
The effective use of frameworks like Spring, JSF, and Hibernate not only simplifies the application development process but also ensures that developers adhere to best practices, thus fostering the creation of maintainable and adaptable applications in a constantly evolving ecosystem.
Harnessing the power of these frameworks ultimately leads to increased productivity and efficient application management.
By utilizing robust Java frameworks conducive to application development, programmers can elevate their project outcomes while optimizing for scalability and performance.
Mobile App Development with Java
Mobile app development plays a crucial role in modern tech environments. Java, as one of the primary programming languages for developing mobile applications, offers versatility and stability. Understanding how to utilize Java for mobile apps allows developers to create robust applications that can reach a vast audience.
Java's compatibility with different devices ensures a broader reach. Many mobile users primarily utilize Android devices, and since Android's official language is Java, mastery of it can significantly enhance a developer's skill set. This section focuses on key elements of mobile app development that are vital, including the benefits and considerations of Java in this domain.
Android Development Overview
Android, being an open-source platform. adapts Java efficiently. From its inception, Android has used Java as its principal language, since it offers vibrant development infrastructure. Developers can tap into a large pool of resources, community support, and libraries that extend Java's capabilities, helping streamline values throughout all stages of the app lifecycle.
In addition, Android Studio, Google’s official Integrated Development Environment (IDE) for Android development, provides all necessary tools for creativity of apps in it. Setting up the environment can be quick and requires easily installed SDKs along with code samples. This allows both aspiring developers and veterans to work toward building unique mobile applications while varifying their functionality.
Using Java for Android Applications
When using Java, developers gain access to a variety of language features, durability in handling various app functionalities, and detailed documentation. With a robust standard traditionally utilized in enterprise settings, Java provides stability- brandished tools usable across many devices.
Think performatively: developers can leverage advances features like:
- Object-Oriented Programming principles to create maintainable code.
- Pre-built Android classes to readily handle user interface and interactions.
- Rich APIs across Google services that simplify integrating added functionalities.
Moreover, utilizing Java APIs allows frameworks and libraries to optimize various mobile features.
Mobile Development Best Practices
Engaging in mobile application development using Java demands awareness of some best practices. Some considerations that enhance usability and performance include:
- Efficient Memory Use: Always be conscious of creating efficient data structures. Feigning excessive memory footprints can result in crashes. All developers should conduct memory monitoring during and after app creation.
- Emphasis on User Experience: Design interfaces that are user-friendly. Functional but minimalist interfaces often yield increased satisfaction among users.
- Testing Continuously: Establishing a habit of testing early and often reduces ambiguity in your application's performance. Tools like JUnit for Java testing mark usefulness in ensuring all parts work collaboratively.

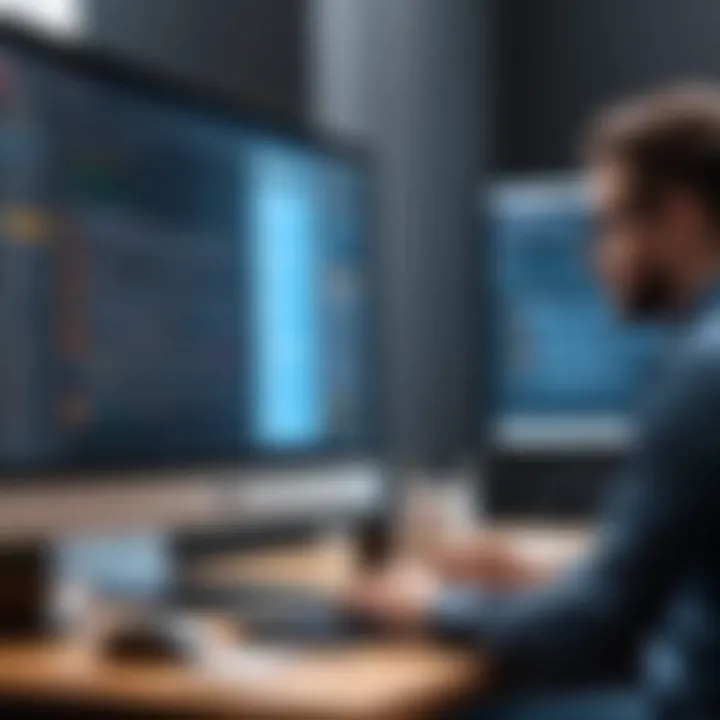
While delving into mobile app intersection with Java, developers ensure they focus on writing practical, maintainable, and user-centered apps that can thrive and evolve with user expectations and feedback based in their unique needs.
Optimizing app shrinkage with respect to user behavior leads users enjoy applications cater fundamentals of Java prominently.
Web Applications and Java
Java holds a pivotal role in web application development, providing a strong foundation for robust and scalable applications. Its platform independence and versatility cater to a wide spectrum of web solutions—from small projects to large enterprise systems. Leveraging Java in web app development leads to not just functional applications but also enhances the user experience through its rich features and libraries. Developers often choose Java for its ability to deliver reliable performance, something that becomes vital with the increasing demands of modern web applications.
There are essential aspects attached to employing Java for web development. Its extensive ecosystem includes frameworks and libraries that facilitate rapid development. This empowers developers to build sophisticated web applications while managing code complexity efficiently. Furthermore, Java’s object-oriented programming principles make the organization of large code bases feasible, which plays a crucial role in medium to large projects in today's everyday software solutions.
Java Servlets and JSP
Java Servlets and JavaServer Pages (JSP) are foundational technologies in Java web development. Servlets are Java classes that handle requests and responses within an application. They enable developers to write server-side code that can dynamically produce content, enhancing the interactivity of web applications. Every interaction from a user triggers a servlet, which processes the input and generates an appropriate response, thus facilitating a seamless user experience.
JSP, on the other hand, is a technology that simplifies the development of dynamic web content. With JSP, developers can embed Java code directly into HTML, making it possible to leverage the power of Java in the front end. This separation allows for cleaner code and easier maintenance since UI designers can focus on the presentation without dealing with complex Java logic.
Utilizing both Servlets and JSP together is common, promoting a Model-View-Controller (MVC) architecture. This architecture separates application logic from the user interface, allowing for greater flexibility and ease of updates.
The combination of Java Servlets and JSP is invaluable in creating dynamic web applications that require interaction with users, databases, or other services.
RESTful Web Services with Java
RESTful web services have transformed how applications communicate. By adhering to REST principles, Java helps create scalable and efficient services. REST (Representational State Transfer) stands for a set of guidelines that enable seamless interactions over the web. These services operate using standard HTTP methods, promoting a stateless communication model. This means every request from a client to the server must contain all the data needed to understand and process it, leading to improved performance.
In Java, developers utilize libraries such as Spring Boot and JAX-RS to construct these services effortlessly. This facilitates the construction of APIs that various clients, including mobile applications and web applications, can consume. The simplicity and efficiency introduced through RESTful services in Java ensure that developers can focus on building rich user experiences without worrying excessively about complex back-end issues.
Furthermore, proper authentication and resource management are vital considerations when developing RESTful APIs in Java. Using technologies like JWT (JSON Web Token) can add a layer of security thus enabling a safer environment for application users. The flexibility, scalability, and performance of RESTful services solidify Java's place in modern application ecosystems.
Challenges in Java App Development
Understanding the challenges in Java app development is essential for both new and seasoned developers. This section examines the significance of these challenges and offers insights into addressing them to enhance development processes. The increasing complexity of software requirements calls for a nuanced approach to app development. Recognizing potential hurdles enables proactive measures which can lead to more efficient and robust applications.
Performance Issues
Performance is crucial in app development. Inadequate performance can lead to slow applications that frustrate users. With Java, developers often face issues such as memory leaks and excessive garbage collection. These issues typically arise from improper coding practices. Memory leaks occur when applications hold onto memory resources that are no longer needed. This persistent allocation can significantly degrade performance potentially crashing apps.
Another common performance concern involves the runtime speed of Java applications. Although Java's Just-In-Time (JIT) compilation enhances execution speed, not all situations benefit from this feature. Benchmarking performance using tools like JMH (Java Microbenchmark Harness) can prove sensitive to tuning. Developing performance benchmarks enables developers to gauge potential issues early and adjust accordingly.
Security Considerations
Security is at the forefront of any application development endeavor. Java, while robust, poses specific security risks if certain protocols are overlooked. Java applications are subject to issues such as injection attacks, unauthorized access, and cross-site scripting. These vulnerabilities can emanate from poorly sanitized inputs. Thus, implementing solid input validation becomes necessary to minimize risks.
Another serious security concern centers around secure communications. Utilizing Java APIs like Java Secure Socket Extension (JSSE) can mitigate risk by ensuring that data transmissions are encrypted. It enables secure channels of communication essential for protecting sensitive data. Adopting best practices for safeguarding resources and employing comprehensive error handling mechanisms promotest overall application security.
Debugging and Troubleshooting
No app development lifecycle is complete without the need for debugging. Java offers numerous tools and methods to assist with identifying bugs. Yet, developers may encounter difficulties in tracking issues due to Java's dynamic nature. For instance, stack traces can provide critical insight but also prove overwhelming if not parsed correctly.
Employing integrated development environments (IDEs) like IntelliJ IDEA or Eclipse can simplify the debugging prospects significantly. These environments offer visual aids that allow developers to inspect current states, evaluate variables, and identify problematic lines of code efficiently. Maintaining a discipline of writing unit tests can greatly reduce troubleshooting's head performance and complexity in the later stages of development.
Pro Tip: Documenting coding decisions, pitfalls encountered, and resolutions can streamline debugging processes in future projects.
Future Trends in Java Development
The landscape of technology is ever-changing, and Java is no exception. Understanding future trends in Java development is critical for developers. They need to anticipate changes and adapt. This section will highlight what to expect in the coming years, including advances in tools, practices, and technologies.
Emerging Technologies
The advent of new technologies signals significant opportunities for Java developers. As these technologies evolve, they shape the frameworks and methodologies used in Java application development. Notable emerging technologies include:
- Artificial Intelligence (AI): Integrating AI in Java applications can enhance user experiences and functionality. Developers are utilizing libraries such as Deeplearning4j and Weka for machine learning.
- Internet of Things (IoT): Java is establishing itself as a leading language for IoT development. Its portability is crucial for diverse platforms used in IoT devices.
- Blockchain: This technology is gaining traction in the Java community. With Java frameworks like Hyperledger Fabric, developers can build secure blockchain solutions.
The importance of adapting to these technologies lies in the potential for enriched functionalities, user engagement, and application performance. The future of Java development will undoubtedly intertwine with these innovations.
Java in Cloud Computing
Cloud computing is rapidly changing how applications are built, deployed, and scaled. Java has a prominent role in this transition. Utilizing Java in cloud computing offers numerous advantages:
- Scalability and Flexibility: Java applications can easily scale horizontally. Developers can use cloud providers like Amazon Web Services or Google Cloud Platform to deploy microservices architectures that improve application scalability.
- Cross-Platform Compatibility: Java's
End
The conclusion serves as a critical chapter in this article, synthesizing diverse aspects of Java app development. It is a culmination of the discussions held in previous sections, an opportunity to reinforce the significance of Java in current and future tech landscapes.
The Enduring Relevance of Java
Despite the evolving technology spectrum and the rise of numerous programming languages, Java maintains a compelling presence in the development community. This language provides unique benefits, enhancing its appeal for aspiring and seasoned developers alike.
- Cross-Platform Functionality: Java's 'write once, run anywhere' philosophy enables developers to create applications that function seamlessly across multiple operating systems. This feature is pivotal for businesses aiming to reach broad audiences.
- Robust Security Features: Given the increase in cybersecurity threats, Java's in-built security architecture offers protective measures that can safeguard applications against risks.
- Rich Ecosystem: With extensive libraries, frameworks, and development tools at its disposal, Java emerges as a versatile choice. Outfits like the Spring Framework and Hibernate exemplify this support, catering to various programming demands.
- Active Community Support: Java benefits from a large, vibrant community. This ecosystem provides a reservoir of knowledge and resources that developers can tap into whenever facing challenges.
- Continued Relevance in Education: Many computer science courses prominently feature Java, establishing its importance among the next generation of programmers. This wide adoption in education reinforces its accessibility and utility.
In summary, the conclusion captures the essence of Java’s durability in app development. A combination of its unique features and a strong community propels Java forward in the tech field, ensuring versatillity and relevance long into the future.
Java remains a foundation upon which many successful applications are built, emphasizing its place within modern software development.