Automated Testing Tools in Python: A Comprehensive Guide
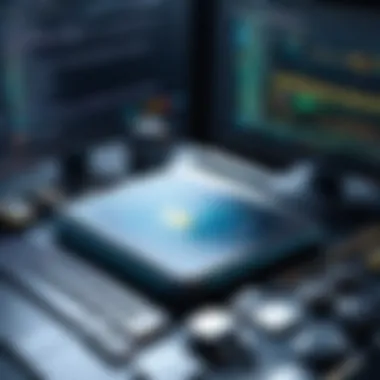
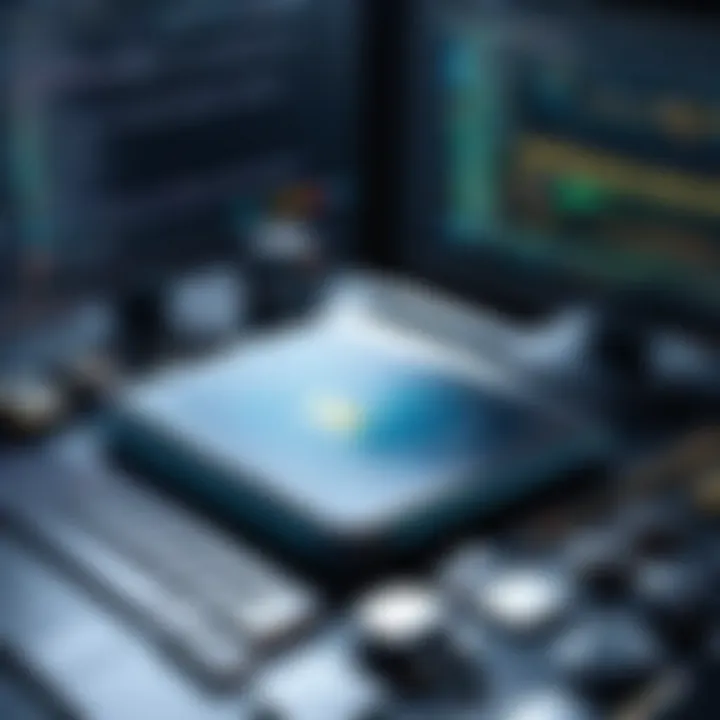
Intro
In the fast-paced world of software development, testing is a critical component that cannot be overlooked. It acts as the line of defense, ensuring that applications function as intended and meet user expectations. In recent years, automated testing tools have gained significant traction, particularly in the Python ecosystem. They are not just conveniences; they offer measurable efficiency and a means to improve code quality without the constant manual labor.
With a plethora of automated testing tools available, developers are spoilt for choice. However, this abundance can also lead to difficulties in selecting the right tools tailored to specific project needs. This article breaks down the landscape of these tools, pinpointing key features, benefits, and ideal use cases.
Moreover, we'll explore how these automation tools fit into the broader strategy of software development and quality assurance, including their roles in team collaboration. By dissecting popular tools and technologies utilized in the Python arena, this guide will provide insights that are essential for anyone looking to enhance their testing strategies efficiently.
So, whether you are a seasoned coder or a novice aiming to sharpen your skills, understanding automated testing will set you up for success in tackling challenges head-on.
Understanding Automated Testing
Automated testing has become a key player in modern software development, significantly influencing how teams deliver quality applications. The use of automated testing tools helps to streamline workflows, reduce manual errors, and enable quicker feedback cycles. This approach is not merely a trend; it’s a necessity for developers who want to maintain the integrity of their code while facing the increasing pressures of tight deadlines and complex project requirements.
The stakes are high when it comes to software failure, which is why understanding automated testing is vital. It allows teams to identify issues earlier in the development process, dramatically decreasing the risks associated with software releases.
Definition and Importance
To grasp the essence of automated testing, one must first define what it means. Automated testing refers to the process of using specialized software to control the execution of tests and compare the actual outcomes to the predicted ones. This is different from manual testing, where testers execute cases by hand. The primary aim here is to enhance productivity, save time, and improve accuracy.
The importance of automated testing cannot be overstated. By automating repetitive testing tasks, developers can allocate their time to more strategic activities. It creates a systematic approach to testing, allowing for continuous integration and continuous deployment (CI/CD) practices that modern software development demands.
Types of Testing
Automated testing encapsulates various types of testing, each serving its unique purpose. Understanding these types can equip developers with the knowledge to select the appropriate tests for their specific circumstances.
- Unit Testing: Unit testing involves testing individual components or functions of the software to validate their correctness, often in isolation from other parts of the application. The beauty of unit testing is its ability to catch bugs at the earliest stage possible, which often translates to lower costs in later development stages. However, unit tests require considerable precision as they need strong coverage of the code to be effective.
- Integration Testing: Integration testing evaluates how various modules or services interact with each other. This form of testing is essential because errors often arise from the way components blend, rather than from an individual piece. A common pitfall is underestimating the complexity of integration, which can lead to oversights in resource consumption.
- Functional Testing: Functional testing assesses a specific function of the software against its requirements. This is valuable for ensuring that the software does what it’s meant to do. However, it's crucial to keep in mind that functional tests might miss broader system interactions. The key characteristic here is focusing on outputs with given inputs, and it's often favored for its straightforwardness.
- Regression Testing: This type of testing checks that recent changes haven’t adversely affected existing features. In fast-paced development environments where code changes and iterations happen frequently, regression testing becomes indispensable. The unique advantage of regression tests is they enable teams to ensure code stability over time, albeit at the cost of potentially increasing the test suite's complexity.
Understanding these types of testing lays a solid foundation for leveraging automated testing tools effectively. With a clear grasp of what each type entails, developers can make informed choices that align with their project requirements and goals. Whether you are an aspiring programmer or a seasoned professional, mastering the art of testing is crucial in the ever-evolving landscape of software development.
Foreword to Python as a Testing Tool
Python has carved out a significant niche in the realm of software development, especially in testing practices. In this segment, we’ll explore why Python is regarded as one of the go-to programming languages for testers and developers alike. Understanding its pivotal role as a testing tool can lead programmers to more efficient and effective testing processes.
One of the primary reasons to choose Python is its readability and simplicity. For newcomers, getting accustomed to testing principles is smoother with Python's clear syntax. Experienced programmers, on the other hand, appreciate Python's versatility—integrating seamlessly with various frameworks and libraries. Importantly, the wide array of tools available makes it an even more attractive choice for automated testing.
Another noteworthy consideration is the thriving community around Python. This community continually contributes to a wealth of resources, libraries, and plugins tailored specifically for testing. When choosing Python, you’re not just selecting a tool; you are tapping into a vast ecosystem packed with support and innovation. This can be invaluable as software requirements evolve over time.
Why Choose Python?
Python's strength in automated testing cannot be overstated. Its syntax allows teams to quickly write and modify test cases, shortening the feedback loop and allowing for rapid iterations. Additionally, the language's ability to handle many types of testing—from unit tests to integration tests—ensures that it can adapt as project requirements shift. Often, the integration of Python in a continuous integration and continuous deployment (CI/CD) pipeline maximizes efficiency in updating or testing software.
Moreover, the popularity of Python among various programming disciplines means that teams can leverage a common language for both development and testing. This reduces the friction that often arises from having multiple languages and frameworks in play within a single project.
Python Libraries for Testing
Python’s robust library ecosystem is a driving force behind its popularity as a testing tool. Let's delve into some of the key libraries that empower Python developers with effective testing capabilities.
pytest
pytest stands out as one of the most used testing frameworks in Python. Users often rave about its straightforward syntax and powerful features that simplify the testing process.
Its key characteristic is the ability to write simple test cases using plain assert statements. This makes it easy for anyone familiar with Python to understand. One unique feature is its support for fixtures, which allow for setup and teardown of test environments in a clean manner. This is particularly advantageous when you need to run multiple tests that require the same setup.
Key Takeaway: pytest’s plugin architecture means that you can easily extend its functionalities. This allows teams to adapt the tool to their specific needs without reinventing the wheel.
unittest
unittest, which is part of the standard library, provides a more traditional approach to testing with its xUnit style. Its structure may seem rigid at first, but it encourages discipline in writing robust tests.
A significant advantage is that it offers a rich set of assertions, making it possible to check various aspects of your application. One downside, however, is that its syntax may appear a bit cumbersome compared to more modern alternatives like pytest. Still, for those used to framework-driven development, it is a solid choice.
doctest
doctest serves a niche purpose but can be extremely useful in specific scenarios. Its defining feature lies in the ability to embed test cases within the documentation of your modules. This means your examples not only serve as documentation but are also actively verified against the code.
While this keeps your examples verifiable, it may limit complex test writing as it focuses on simple input and output verification. Thus, it's more of a complement to other methods rather than a standalone tool for comprehensive testing.
In summary, the selection of Python as a testing tool is a choice rich with potential and consideration. With libraries like pytest, unittest, and doctest at your disposal, Python enables various testing methodologies that can elevate software development and quality assurance practices.
Key Automated Testing Tools in Python
When it comes to automated testing in Python, the tools available can make or break the efficiency of the development process. The right tool can streamline testing, ensuring that bugs are caught early and that features are implemented correctly. This section dives deep into some of the key automated testing tools in Python, explaining their significance and how they can impact your projects. Each tool has specifications that cater to different requirements, making it crucial for developers to choose wisely based on their needs.
pytest
Basic Features
pytest stands out due to its simplicity and flexibility. One of its key characteristics is the ease of writing test cases. With minimal boilerplate code required, developers can focus solely on the logic that needs testing.
For instance, comparisons using assertions are straightforward. If a test fails, pytest provides clear information on what went wrong, which is invaluable during debugging. Furthermore, it supports fixtures that allow for setup and teardown operations, making tests cleaner and more efficient.
The unique advantage of pytest is its ability to handle complex testing scenarios with ease. It’s portable across different testing types, from unit to functional testing. However, beginners might feel overwhelmed initially due to the wide array of functionalities available.
Plugins and Extensions
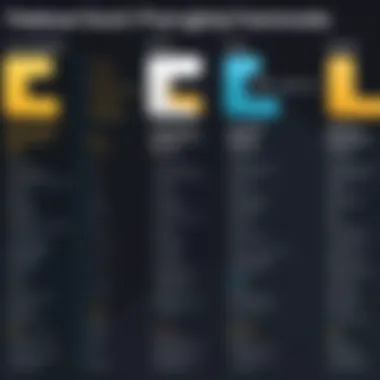
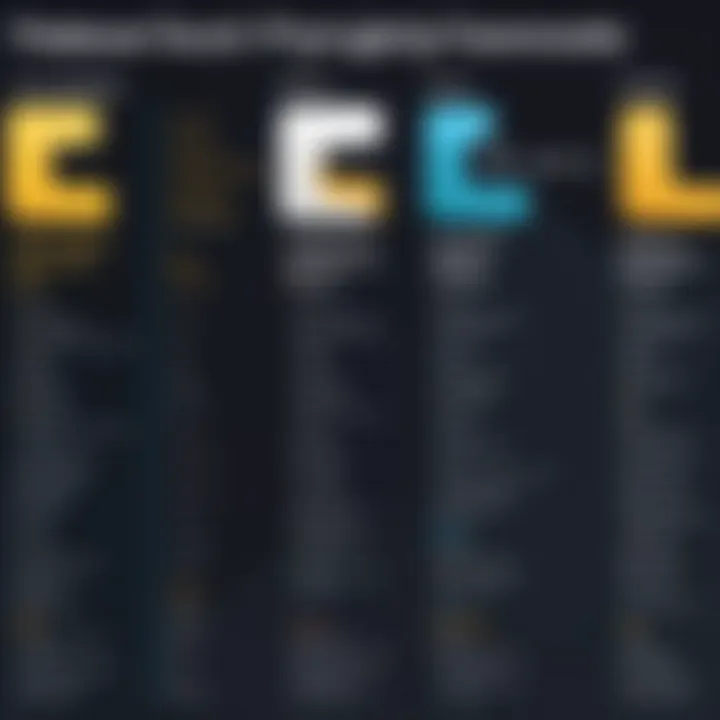
The plugin system in pytest is another significant asset. It allows users to extend the capabilities as per their project's needs. With hundreds of plugins out there, integrating features like HTML reporting, mocking, or parallel testing has never been easier.
One popular plugin is pytest-cov, which helps in measuring code coverage during testing. This functionality ensures that tests not only pass but also adequately cover the critical parts of the code.
But, while the plugin flexibility is great, maintaining compatibility and ensuring that the plugins are up to date can sometimes be a hassle, especially in larger projects where multiple dependencies are in play.
Best Practices
Writing effective test cases in pytest is paramount. Adhering to best practices ensures the longevity and maintainability of tests. This includes naming conventions like using prefix for functions, which makes it easy for pytest to identify them as test cases.
Another practice is to keep your test cases isolated. Dependencies in tests can lead to flaky tests, where results vary due to state changes or execution order. Each test should stand on its own, not relying on prior successful runs. Moreover, documenting tests adds clarity, making it easier for team members to understand their purpose.
Despite its advantages, it’s essential for developers to regularly refactor tests to keep them relevant as the codebase evolves.
Robot Framework
Overview and Capabilities
Robot Framework emerges as a powerful tool boasts easy readability which appeals especially to teams that include non-technical members. With a keyword-driven approach, the tests become almost self-documenting. This is particularly beneficial when onboarding new team members since tests can be understood even without in-depth programming knowledge.
The capability to integrate with other libraries is another strong point, as it allows seamless automation of various applications beyond just Python projects. Its design is modular, so adding functionalities when the project grows isn’t problematic.
However, the reliance on a specific syntax can be a learning curve for those who are more familiar with traditional programming languages.
Built-in Libraries
Robot Framework is enriched with built-in libraries that cover a vast range of functionalities. Common libraries include the OperatingSystem library for file manipulation and the Selenium library which facilitates web testing.
Using these libraries means test writing becomes faster, and the tests themselves become more robust, accommodating many potential user interactions with the system.
Nonetheless, developers might find the built-in libraries somewhat limiting for highly customized functionalities that they might need to implement.
Use Cases
A primary strength of Robot Framework is its adaptability in various contexts. It’s particularly favored in acceptance level testing, where stakeholders want a clear picture of feature functionalities.
Many organizations use it for testing web applications, mobile applications, and even complex enterprise systems. It’s also beneficial in environments where different teams might not share the same technical background.
However, for very detailed unit tests, teams might need alternative tools or mix and match with other frameworks to meet comprehensive testing needs.
Selenium with Python
Web Application Testing
Selenium with Python is a heavyweight contender in the ring of web application testing. The flexibility to script tests not only in Python but in multiple languages empowers teams who may work across various tech stacks.
A major characteristic of Selenium is its ability to interact with web elements, allowing for automatic interactions, which can significantly reduce manual testing efforts. It faithfully mimics user behavior, delivering accurate results.
While its flexibility is commendable, sometimes this robustness can lead to more complicated setup processes, which might intimidate teams unaccustomed to automated testing tools.
Browser Automation
Beyond the realm of testing, Selenium shines in browser automation tasks. Whether it's automating data entry or scraping, its comprehensive API offers developers a multitude of options.
This functionality can save businesses considerable time, as repetitive tasks once done manually can be handed over to automation. However, automation can lead to maintenance issues, as web interfaces constantly evolve, which means that tests will need continuous updates.
Integration with pytest
Integrating Selenium with pytest allows teams to harness the strengths of both tools effectively. The combination promotes better test organization and seamless report generation,
The synergy between these tools also means that developers can write more maintainable and reusable automation scripts, which is vital in dynamic environments where code changes frequently. Nonetheless, careful attention to maintaining the right versions of both tools is necessary to ensure compatibility and smooth operation.
Locust
Load Testing
In today’s high-demand environments, load testing has gained prominence, and Locust delivers this with style. The ability to simulate hundreds, if not thousands, of user interactions means developers can stress-test applications before they go live.
A standout characteristic of Locust is its simplicity in creating load tests using plain Python code, making it very accessible.
However, for teams unfamiliar with Python, there may be a slight learning curve. Still, once implemented, its straightforward nature makes it easy to set up and modify tests.
Testing Web Applications
Locust excels at testing web applications under varying conditions. Developers can deploy testing scenarios that mimic real-world usage, which provides vital details about how an application will perform under pressure.
By tracking metrics such as response times and error rates, teams can determine application responsiveness well before users ever interact with it. While it encapsulates detailed functionality, results can sometimes generate a false sense of security if not interpreted cautiously.
Distributed Testing
The ability to carry out distributed testing sets Locust apart. This means testing across various machines can be done simultaneously, thus simulating heavy traffic more realistically.
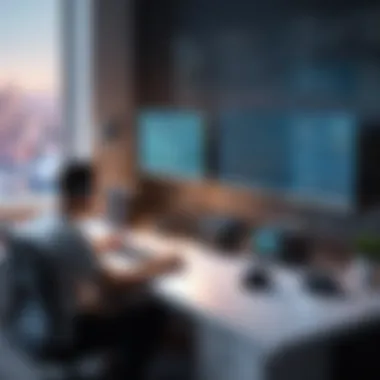
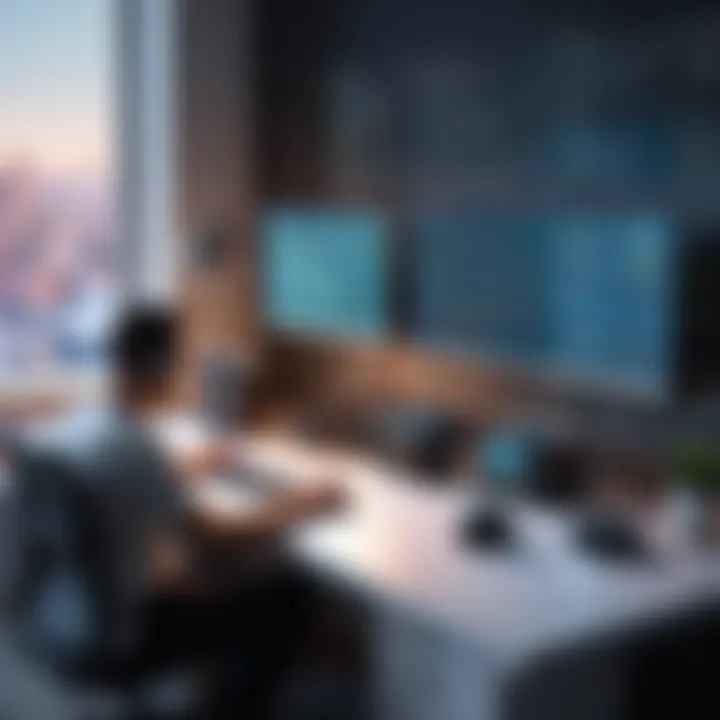
As the testing demands grow or environments become more complex, this feature proves crucial. Yet, coordinating distributed setups often requires more oversight and management, which might be a concern for smaller teams or projects.
Locust provides a compelling option for teams looking to test the scalability of applications with a straightforward setup, but effective monitoring is key to leveraging its full potential.
Choosing the Right Tool
Selecting the appropriate automated testing tool is not just an afterthought; it's a critical part of the testing process that can determine the effectiveness of your software development lifecycle. A fitting tool can enhance productivity, reduce bugs, and ensure a smooth path from development to deployment. While the plethora of options may seem overwhelming, understanding the nuances of your project and team's capabilities can guide you towards the right choice.
This section will cover the key elements necessary for making an informed decision about testing tools, focusing on three primary factors: project requirements, team expertise, and tool compatibility.
Factors to Consider
Project Requirements
When diving into project requirements, one must consider how the nature of a project dictates the kind of testing needed. Every project comes with its own set of requirements—be it the level of functionality to be tested, the target platforms, or even the timelines for delivery.
A significant characteristic of project requirements is specificity. Clearly defined requirements help in choosing tools that tailor specifically to those needs. For instance, a web application might benefit from Selenium, specifically designed for browser automation testing. This ensures that the tool’s capabilities align with what the project demands, making it all the more beneficial. Likewise, if the project involves microservices, a tool like pytest could easily accommodate unit and integration testing.
On the flip side, vague project requirements lead to a mismatch between the tool and the testing needs. If you’re biting off a lot without a clear structure, the risk of choosing an ineffective tool rises significantly.
Team Expertise
Team expertise speaks volumes when selecting the right automated testing tool. The skills and familiarity of the development and testing team can greatly influence the tool choice. A group comfortable with Python will naturally lean towards Python-centric tools like pytest or unittest. The idea here is not just to choose a tool but to leverage the existing knowledge base within the team.
One of the benefits of aligning tool selection with team expertise is the potential for a quicker onboarding process. If the team is already skilled in a particular tool, they're likely to hit the ground running. Conversely, opting for a tool that requires a steep learning curve could lead to delays and frustration, wasting valuable time and resources in the process.
However, relying solely on team familiarity may limit opportunities for growth. Sometimes it's worth considering a tool that is slightly outside the comfort zone if it promises significant advantages in the longer run.
Tool Compatibility
Last but not least, we've got tool compatibility. This factor refers to how well an automated testing tool integrates with the existing tech stack. When tools work seamlessly with other applications, libraries, or frameworks in use, the whole testing process becomes smoother.
A main characteristic of compatibility is flexibility. Ideally, you want a testing tool that can be effortlessly integrated into CI/CD pipelines, version control systems, or cloud environments. An example includes Jenkins with pytest, which solidifies a robust workflow for continuous testing.
Now, a key downside exists when tools do not mesh well with your existing stack. Incompatibility can lead to frustration as it not only extends the testing phase but can also introduce bugs that weren’t there in the first place. So, when in doubt, compatibility should be one of those red flags that catches your eye during the selection process.
Making an Informed Decision
Armed with the knowledge of project requirements, team expertise, and tool compatibility, you’re now more equipped to make an informed decision regarding your automated testing tool. Each choice shapes the effectiveness of your testing strategy. If the process feels a bit overwhelming, remember: alignment with your project goals and understanding your team's strengths will pave the way to success.
"Choosing the right testing tool is akin to a carpenter selecting the right hammer; the right fit can make the work seamless, while the wrong choice can lead to more headaches than solutions."
Review your options carefully, weigh the pros and cons, and make a choice that serves both your project’s needs and your team's expertise.
Integration Techniques
Understanding integration techniques is crucial for optimizing the effectiveness of automated testing tools within Python projects. These techniques focus on bringing together various components of a development and testing ecosystem to ensure seamless functionality. Proper integration can significantly improve the development pipeline, leading to faster delivery and higher quality software. The importance of these techniques cannot be overstated, as they directly influence how well automated tests are executed and maintained.
Setting up an effective testing environment dives into various setups and practices that can enhance the automation process. This involves configuring systems, installing necessary tools, and preparing the environment in a way that reduces friction when writing and executing tests. Meanwhile, continuous integration allows teams to merge their code changes frequently. Automated tests then run with each integration, which not only catches errors early but also ensures that new changes don’t break existing code.
Setting Up Testing Environments
The foundation of successful automated testing lies in how well the testing environments are set up. In simple terms, one must create a space that mirrors production as closely as possible. This kind of environment ensures that tests behave consistently with what end-users experience.
A few considerations when setting up include:
- Isolation: Protect the environments to avoid interference from unrelated processes that can lead to flaky tests.
- Dependencies: Use tools like to manage and install dependencies, ensuring all required libraries are accounted for.
- Environment Variables: Setting appropriate configurations to mimic different scenarios helps in evaluating how the application reacts under various conditions.
Utilizing containerization tools, like Docker, can also play a significant role, allowing developers to package applications reliably, making it easier to maintain consistency across environments.
Continuous Integration with Test Automation
Continuous integration (CI) plays a pivotal role in the efficiency of software development. It stresses the importance of validating every change that pushes into the main repository. Ideally, this practice involves automatically running tests to ensure that everything works as intended after those changes.
Version Control Systems
Version control systems, such as Git, represent the backbone of CI processes. They provide a structured way to manage code changes, allowing developers to track alterations and collaborate efficiently. The key characteristic of version control systems is their ability to maintain a history of changes, which is essential for both accountability and coordination among team members.
One notable feature of version control systems is branching. This allows developers to work on separate features independently without disrupting the main codebase. The merge process brings all alterations together, which is further validated through automated tests during the CI phase. However, one downside is dealing with merge conflicts, which can arise when multiple changes collide.
/ Pipelines
CI/CD pipelines take the benefits of continuous integration a step further by automating the deployment process. After the testing stages validate the code, CI/CD pipelines ensure that the newly tested code is seamlessly deployed to production. The noteworthy aspect of CI/CD pipelines is the automation of not just the testing but the entire deployment lifecycle, making it a popular choice for modern development teams.
A unique feature of CI/CD pipelines is that they can incorporate multiple stages, such as building, testing, and deploying, in a single framework. This interlinks various processes, making it clear and straightforward to visualize progress. Advantages here include reduced manual errors, faster releases, and continuous feedback on the application’s health. However, teams must be cautious about improperly managing the pipeline, which could lead to complicatons and slow down the workflow.
Continuous integration coupled with effective testing environments drives not just productivity but also a culture of quality in software development.
Establishing these integration techniques lays a solid foundation for automated testing in Python, ensuring that the team can respond swiftly to changes while maintaining high software testing standards.
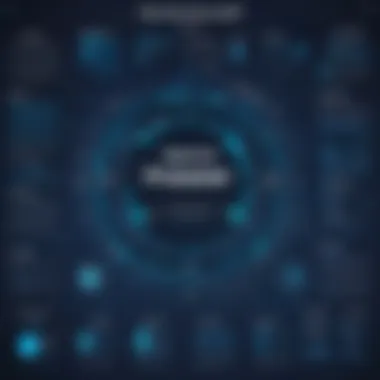
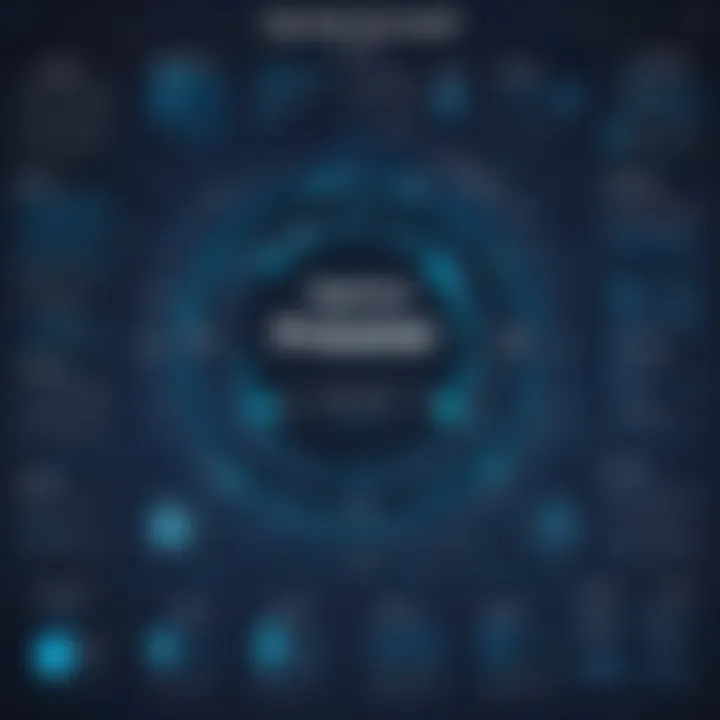
Writing Effective Test Cases
Writing effective test cases is a cornerstone of any automated testing framework, particularly in Python. These test cases serve as the blueprint for verifying that software applications work as intended. They not only help identify bugs and issues but also facilitate better communication among team members about the functionality and quality of the product.
There are several crucial elements to consider when crafting test cases. Effective test cases should be clear and concise, focusing on a single objective. This clarity helps prevent confusion, ensuring that anyone reviewing the test knows what functionality is being validated. Additionally, they should be repeatable, allowing for consistent results over multiple test runs. This repeatability is vital in continuous integration environments where tests are automated and run frequently.
Benefits of well-structured test cases are manifold. They help in accelerating the debugging process by narrowing down the source of a bug through targeted tests. Moreover, they can improve collaboration since all team members can easily understand what is being tested, and they serve as a form of documentation for the application’s requirements.
Before we dive deeper, it's important to touch on some key considerations when writing these test cases. Think about maintainability and scalability; as projects evolve, test cases should be easy to update without starting from scratch. Furthermore, having a consistent naming convention for test cases can enhance organization and clarity.
"Clear and concise test cases can save developers from hours of debugging later on."
Best Practices for Test Case Design
When designing test cases, adhering to best practices can significantly affect their effectiveness and usability. Here are a few guidelines to consider:
- Use Descriptive Names: Test case names should clearly indicate what they are validating. Instead of naming a test , opt for something like .
- Keep It Simple: Tests should verify one specific scenario. Avoid complex conditions that can make it hard to determine what has failed.
- Use Assertions Wisely: Assertions are the backbone of test cases; choose the right assertions to validate outputs robustly. Python's framework, for instance, supports various assertions that help in comparing expected outcomes with actual results.
- Document Assumptions: If a test case makes any assumptions about the application state or the environment, document them within the test case using comments. This can be invaluable for future debugging.
Another aspect to ponder is the setup and teardown methods. They prepare the environment for tests and clean up afterward. Using these methods judiciously can prevent side-effects between tests, keeping your testing run smooth.
Common Pitfalls to Avoid
Even seasoned programmers can stumble upon certain pitfalls when writing test cases. Here are a, few to watch for:
- Over-Complicating Tests: It's tempting to create tests that cover multiple scenarios. However, too much complexity can lead to misinterpretation of results and may obscure the underlying issues.
- Neglecting Edge Cases: A common oversight is failing to test edge cases, which may include unusual inputs or unusual application states. It’s crucial to incorporate these cases to ensure robust coverage.
- Ignoring Test Maintenance: As the application evolves, so too should your test cases. Regularly review and update them to keep them relevant; otherwise, they risk becoming irrelevant or misleading.
- Failure to Isolate Tests: Each test should run independently of others. This way, the outcome of one does not influence another’s results, which can skew the overall testing assessment.
By adhering to these best practices and being mindful of common pitfalls, you can enhance the quality and effectiveness of your testing efforts. Writing effective test cases is not merely a task but an investment in the software's overall quality.
Community and Support
Community and support play an integral role in the arena of automated testing tools in Python. For developers, especially those who may be starting on their journey, fostering connections with others who share similar interests can significantly enhance their learning, skill set, and problem-solving capabilities. Having access to a robust community can help demystify challenges, create networks for collaboration, and provide platforms for sharing best practices. This collective knowledge is invaluable, making it easier to navigate the complexities of automated testing.
A vibrant community can often spell the difference between a programmer who's floundering through research and one who efficiently finds solutions. Through forums, social media groups, and discussion boards, users can seek assistance, share experiences, and engage in dialogue that hones their skills—transforming troubleshooting into a learning experience. Moreover, new insights gained from community interactions can guide users in selecting appropriate tools based on firsthand experiences from others.
In essence, the support structures surrounding Python automated testing tools are essential for nurturing talent, fostering an ecosystem of continuous improvement, and promoting the sustained growth of technology enthusiasts and professionals alike.
Active Python Testing Communities
Active Python testing communities thrive in various online spaces, offering support and resources for users. Platforms such as Reddit have dedicated threads and subreddits, like r/Python, where one can find discussions tailored to specific needs. These online communities foster collaboration among experienced developers and novices, alike.
Engaging in these spaces allows for the exchange of ideas, feedback on testing techniques, and sharing of code snippets that tackle real-world problems. Additionally, many established testing communities often host virtual meetups where experts share knowledge about new tools or updates. This active exchange of information makes the learning curve less steep and encourages contributors to continually enhance their skills.
Resources for Learning and Improvement
Online Tutorials
Online tutorials act as vital tools of empowerment in the world of Python automated testing. They present concepts in digestible chunks, making it easier for users to absorb new information. Typically, platforms may like YouTube or Coursera host step-by-step video tutorials, allowing learners to follow along visually.
One key characteristic of these tutorials is their accessibility; learners can work at their own pace, pausing and rewatching sections as needed. However, while many tutorials provide practical insights, they can sometimes become outdated quickly, given the rapidly evolving field of technology.
Overall, online tutorials can be an excellent resource for those seeking an engaging and visual approach to learning Python testing tools, yet users must remain discerning about the date and relevance of the content.
Documentation
Documentation serves as the backbone for any programming tool, and this holds true for Python testing tools as well. Comprehensive and well-organized documentation, such as that provided by libraries like pytest, is crucial in understanding how to utilize specific features effectively.
The key characteristic of good documentation is clarity. Clear examples and thorough explanations help users grasp complex concepts without feeling overwhelmed. However, it’s not uncommon for documentation to assume a certain level of knowledge, which can be a barrier for beginners trying to dive in.
Hence, while documentation is an excellent asset for understanding the depth of the tools available, new programmers might need to supplement it with community support or tutorials for comprehensive learning.
Forums
Forums function as interactive spaces where users can ask questions and share their experiences regarding automated testing in Python. These platforms allow for real-time problem solving and support, creating a hub for collaboration. Sites like Stack Overflow have dedicated sections for Python-related queries, where users can rapidly identify solutions to particular challenges they face.
One unique feature of forums is that they often hold a treasure trove of archived discussions. Users can search for previously answered questions, accessing a wealth of shared knowledge without having to start their inquiries from scratch. However, the downside is the possibility of encountering outdated or incorrect information, highlighting the importance of verifying the insights gleaned from these discussions.
Future Trends in Automated Testing
Automated testing is evolving quickly to meet the demands of modern software development. As projects become increasingly complex, the importance of understanding future trends in automated testing cannot be overstated. Recognizing these trends not only helps teams stay relevant but also improves their overall efficiency and effectiveness. These advancements pave the way for smarter testing, better resource allocation, and enhanced collaboration among developers.
Emerging Technologies
In the realm of automated testing, several new technologies are making waves. Here are a few worth keeping an eye on:
- Containerization and Virtualization: Tools like Docker and Kubernetes allow testers to create isolated environments for testing. This ensures consistent results across different platforms and reduces the typical environment setup time.
- Low-Code and No-Code Testing: These platforms empower non-technical stakeholders to contribute to the testing process. With user-friendly interfaces, they can create and manage tests, which speeds up the testing cycle dramatically. For instance, tools like TestProject or Katalon Studio take this approach.
- Blockchain for Testing: This emerging technology aims to enhance the integrity and security of applications by providing an immutable ledger of transactions. It is particularly useful in testing decentralized apps where data cannot be altered.
Stay aware that new tools and technologies are springing up like mushrooms after rain, so keeping abreast of what’s available is vital for testing success.
Role of AI in Testing
Artificial Intelligence is not just a fad; it's transforming how we think about testing. Here’s how AI is becoming an integral part of the testing landscape:
- Test Case Generation: AI can analyze requirements and automatically generate test cases. This makes it easier to ensure that all scenarios are covered without the usual manual overhead. Tools like Test.ai use machine learning models to facilitate this process.
- Predictive Analysis: By leveraging historical data, AI tools can predict potential problem areas in the software and suggest where to focus testing efforts. This proactive approach can save time and resources.
- Self-Healing Tests: AI systems are capable of adapting to changes automatically. Instead of failing due to trivial alterations in the application, self-healing tests can adjust themselves to keep on running smoothly, greatly reducing maintenance efforts.
"AI is reshaping the testing landscape, making it more efficient and less time-consuming, which allows teams to focus on enhancing code quality rather than merely finding bugs."
As we look ahead, it's crystal clear that automation—and especially AI—will continue to refine and redefine the way we approach testing. Understanding these trends not only equips companies with the tools to optimize their testing efforts but also places them at the forefront of technological innovation.