Beginner's Guide to Learning Node.js
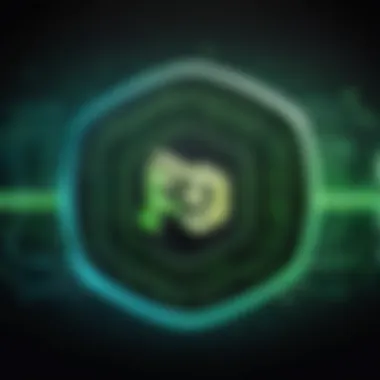
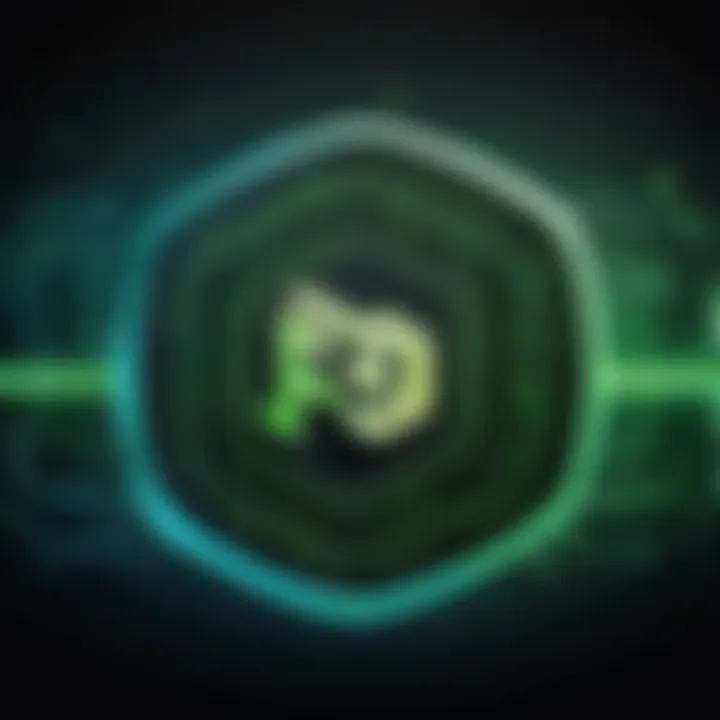
Intro
Node.js has emerged as a key player in the world of web development. As an open-source JavaScript run-time environment, it allows developers to build scalable network applications efficiently. This article aims to serve as a comprehensive guide tailored for beginners keen on mastering Node.js. Let's explore the fundamental building blocks that will set you on the path to becoming proficient in this dynamic platform.
Coding Challenges
Engaging with coding challenges is a pivotal part of the learning process for Node.js beginners. These challenges not only enhance coding skills but also provide practical experience in using Node.js within various scenarios.
Weekly Coding Challenges
Participating in weekly coding tasks keeps your mind sharp and fosters continuous learning. Websites like HackerRank and LeetCode frequently publish coding challenges that cater specifically to JavaScript and Node.js users. Engaging with these can solidify your skills and provide insights into common pitfalls developers face.
Problem Solutions and Explanations
When tackling coding challenges, understanding the solutions is crucial. Each problem often has multiple solutions, showcasing different approaches you can take. Take time to read through discussions and explanations on platforms like Reddit or Stack Overflow, which can illuminate methodologies that you may have overlooked.
Tips and Strategies for Coding Challenges
- Break down problems: Start by analyzing the problem statement and breaking it into manageable parts.
- Practice regularly: Consistency is essential—set aside dedicated time each week for coding.
- Review your code: After a solution is achieved, review it for optimization opportunities. This can improve your understanding of effective coding practices.
Community Participation Highlights
The Node.js community is vibrant and supportive. Participating in coding competitions or collaborative projects helps you stay engaged. Many GitHub repositories showcase Node.js projects where you can contribute, test your skills, and connect with fellow beginners and experienced developers.
Resources for Learning Node.js
In addition to coding challenges, having access to quality resources is vital for your learning journey. Here are some recommended sources:
Programming Language Guides
Books and online guides can be invaluable. "Node.js Design Patterns" by Mario Casciaro and "Eloquent JavaScript" by Marijn Haverbeke are excellent starting points.
Tools and Software Reviews
Familiarizing yourself with tools such as Visual Studio Code, npm (Node Package Manager), and Postman can enhance your development process. Each tool comes with unique features that can aid in debugging, package management, and API development.
Tutorials and How-To Articles
Online tutorials serve as practical step-by-step guides. Websites like NodeSchool offer interactive lessons that cover various aspects of Node.js, including modules and frameworks.
Online Learning Platforms Comparison
Several platforms provide structured learning paths for Node.js. For instance, Codecademy and Udemy offer courses tailored to beginners, each varying in approach and depth. Comparing these platforms can help determine which aligns best with your learning style.
In summary, this guide will navigate you through learning Node.js effectively, equipping you with the necessary tools and strategies to succeed. Understanding coding challenges, utilizing relevant resources, and engaging with the community will significantly contribute to your growth as a Node.js programmer.
Prelims to Node.js
Node.js has gained significant traction in the software development community for its efficiency and versatility. It is crucial in this article to introduce Node.js as it serves as the foundation for various web applications and services. Understanding its core features and functionalities will equip beginners with the necessary knowledge to navigate the landscape of modern web development.
The importance of learning Node.js lies in its event-driven architecture, which allows for high concurrency and responsiveness. It operates on a single-threaded model, making it ideal for developing scalable network applications that can handle multiple requests simultaneously. This efficiency leads to reduced server response times and enhanced user experience, making Node.js a popular choice for both startups and established enterprises.
Moreover, Node.js utilizes JavaScript, which is the most widely used programming language. This offers a transition opportunity for web developers who are already familiar with JavaScript on the client side. Consequently, leveraging Node.js allows developers to use the same language for both front-end and back-end development, promoting enhanced productivity and a cohesive workflow.
Additionally, the thriving ecosystem of packages available through npm (Node Package Manager) significantly streamlines the development process. New developers can access a wide variety of libraries and frameworks to accelerate their projects. The community support and extensive documentation further foster a welcoming environment for learners.
"Node.js empowers developers to build real-time applications with ease, leveraging JavaScript across the stack."
In summary, this introduction sets the stage for understanding Node.js’s importance in contemporary development. The subsequent sections will offer deeper insights into its architecture, capabilities, and practical applications.
Setting Up Your Environment
Setting up your environment is a critical step in your Node.js journey. It creates the foundation for your development work, ensuring you have the necessary tools and configurations to effectively write, test, and run your applications. This section will provide you with insight on installing Node.js and selecting an appropriate Integrated Development Environment (IDE) or text editor. A well-structured environment not only enhances productivity but also makes learning and troubleshooting more manageable.
Installing Node.js
Installing Node.js is the first action to take when setting up your environment. Node.js is an open-source, cross-platform JavaScript runtime that allows developers to run JavaScript server-side. It is crucial to download the latest stable version, as this will include important features as well as bug fixes. You can find the installer on the official Node.js website at nodejs.org.
Steps for installation:
- Choose the appropriate installer for your operating system (Windows, macOS, or Linux).
- Follow the installation wizard's prompts. Make sure to check the option to add Node.js to your system path. This step ensures that you can run Node.js commands from the terminal or command prompt.
- Verify the installation by opening a terminal or command prompt and typing . This command will display the installed version of Node.js.
Benefits of Installing Node.js:
- JavaScript on the server: Run server-side JavaScript, allowing for a seamless developer experience.
- Speed: Its performance, powered by the V8 engine, is notable, making it suitable for data-intensive applications.
- Vast Ecosystem: Gain access to npm, the Node package manager, which hosts a wealth of libraries and frameworks.
Choosing an IDE or Text Editor
Once Node.js is installed, the next step is to choose an IDE or text editor. There are many available options, each with its unique features and capabilities. The selection largely depends on personal preference, the complexity of projects, and specific requirements.
When evaluating options, consider the following:
- Feature set: Look for essential features like syntax highlighting, debugging tools, and code completion.
- Community Support: Tools with a larger user base tend to have more resources available, from plugins to forums.
- Customization: The ability to adjust settings and plugins according to your workflow can greatly improve your efficiency.
Some popular tools include:
- Visual Studio Code: Highly versatile and extensible, ideal for both beginners and advanced users.
- Sublime Text: A light editor known for speed and simplicity.
- Atom: Open-source, allows customization along with community-driven packages.
"A well-configured environment is as important as code itself. It can make or break your development experience."
With the environment in place, you can now move forward to grasp the core concepts of Node.js, leading you towards building impactful applications.
Core Concepts of Node.js
Understanding the core concepts of Node.js is essential for anyone interested in this powerful platform. Node.js is built on several foundational principles that define its functionality and efficiency. Delving into these concepts equips beginners with the necessary knowledge to utilize Node.js effectively in various development scenarios. It is essential to grasp these key elements to make informed decisions in coding practices, architectural design, and the integration of Node.js with other technologies.
Event-Driven Architecture
Event-driven architecture (EDA) is a crucial component of Node.js. This design pattern allows programs to respond to events or changes in state. In Node.js, developers can create applications that react to user inputs or server triggers without blocking the execution of other code. With EDA, the application remains responsive, allowing for better user experiences.
Key benefits of EDA in Node.js include:
- Scalability: EDA supports massive numbers of simultaneous connections. It enables Node.js applications to manage multiple requests efficiently. This is vital for modern web applications that serve high traffic.
- Efficiency: Since Node.js uses an event loop, it can manage I/O operations without waiting for them to complete. This leads to faster load times and a smoother experience for users.
- Flexibility: Building applications around events allows developers to adapt easily to changing requirements. They can add, modify, or remove event listeners as needed.
Understanding this architecture leads to more effective coding practices. Developers should consider how events flow through their applications to maximize performance and maintainability.
Non-Blocking /O
Non-blocking I/O (input/output) is another fundamental concept in Node.js. Traditional I/O operations can block execution until the operation completes. This leads to latency issues, especially when dealing with network requests or file operations. Non-blocking I/O, however, allows for concurrent execution, keeping the application running smoothly even during lengthy operations.
With non-blocking I/O, the event-driven model shines. Here are some key aspects:
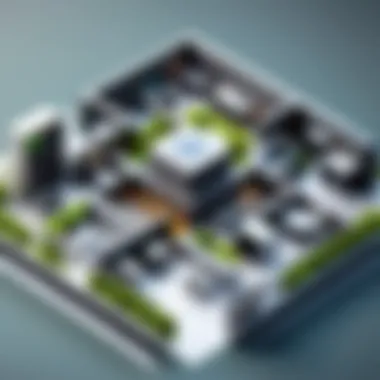
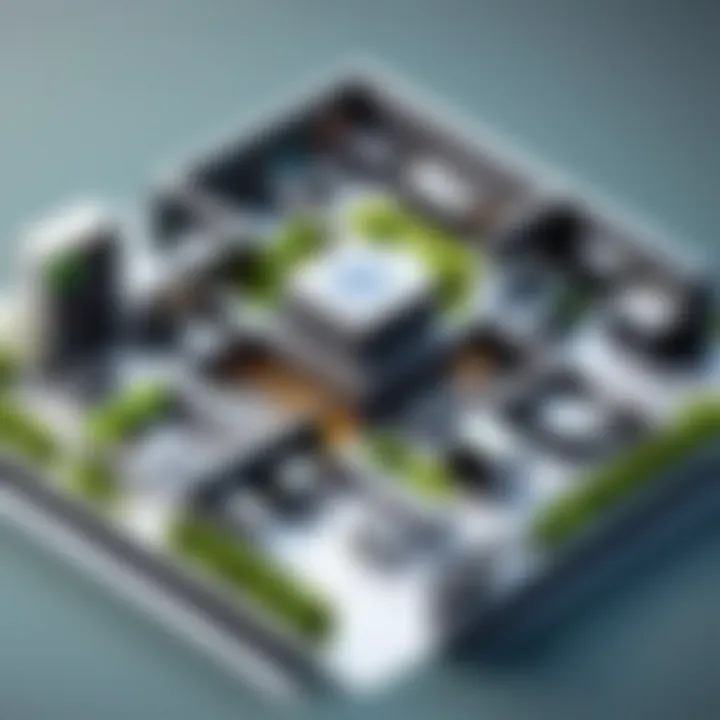
- Concurrency: Multiple operations can take place simultaneously without waiting for each to finish. This is particularly useful for web servers that handle various requests.
- Performance Optimization: Developers can enhance application performance by minimizing wait times. Non-blocking I/O enables tasks to run in the background, leading to a more responsive application.
- Simplified Code: The asynchronous nature of non-blocking I/O helps in writing cleaner and more concise code. It reduces the complexity often associated with synchronous programming.
In practice, it is common to use callback functions, promises, or async/await syntax to implement non-blocking I/O in Node.js. Understanding how to leverage these techniques can significantly improve a developer's ability to create fast and scalable applications.
In summary, the core concepts of Node.js, particularly event-driven architecture and non-blocking I/O, are vital for realizing the platform's full potential. Mastery of these principles will pave the way for success in developing high-performance applications.
Building Your First Application
Building your first application in Node.js is a significant milestone in the learning process. It encapsulates the fundamental principles of programming with this platform and lays the groundwork for more complex projects. Understanding how to create a simple application teaches you about servers, request handling, and fundamental web technologies. This section provides a step-by-step guide to establishing a solid foundation in these areas.
Creating a Basic Server
Creating a basic server is the first practical exercise you will undertake. This task is crucial, as it illustrates how Node.js operates in a real-world context. Establishing a server enables you to respond to web requests, which is a foundational capability in web development.
To start, you need to utilize the built-in HTTP module in Node.js. This is where you will learn the syntax and structure of Node.js. The process is straightforward. First, you will import the http module, then create a server that listens on a specific port. Here is a simple example:
In this snippet, you create an HTTP server that responds to every request with "Hello World". This exercise introduces you to asynchronous programming and callbacks in Node.js, critical aspects you will use throughout your projects.
Handling Requests and Responses
Handling requests and responses is an essential skill in Node.js application development. It involves managing incoming data from clients and sending back appropriate responses.
An effective Node.js application will typically define how to process various types of requests. In your basic server example, request processing is done inside the callback function. You can examine the object to understand the HTTP request, including the request method and the URL. Meanwhile, the object is used to form your response, controlling the status code and the data sent back to the client.
For instance, here is how you might enhance the previous server application to differentiate responses based on the URL:
In this case, the server checks the URL for specific paths and responds accordingly. This functionality is vital, as it mimics the behavior of real-world applications where different routes serve different types of content. It might seem simple, but mastering request and response handling is fundamental for developing more advanced applications.
"Understanding how to handle requests and responses is crucial to becoming proficient in Node.js."
By successfully mastering these concepts of creating a server and handling requests, you pave the way for more sophisticated applications and gain confidence in utilizing Node.js tools.
Using npm and Package Management
Understanding npm and package management is critical for anyone learning Node.js. It streamlines the process of managing external code libraries, increasing efficiency in both development and maintenance. npm, or Node Package Manager, is a central part of the Node.js ecosystem, allowing developers to access a vast repository of libraries and tools. This eliminates the need to write common functionalities from scratch, thus accelerating the development process.
What is npm?
npm is the default package manager for Node.js. It facilitates the installation, updating, and uninstallation of packages, which are community-developed libraries that extend the functionality of Node applications. Each package is stored in a public registry, ensuring that developers can find and utilize a plethora of resources with ease.
The benefits of using npm include:
- Simplified Dependency Management: Developers can maintain their project’s dependencies with ease, ensuring that all necessary libraries are up to date.
- Version Control: npm allows the installation of specific versions of packages, preventing compatibility issues that could otherwise arise in projects.
- Community Contributions: It has a large community of contributors who continually update packages, enhancing functionality and security.
npm allows developers to focus more on building their applications than on managing dependencies. To put it simply, npm is a crucial tool in a Node.js developer's toolkit.
Installing and Managing Packages
To begin using npm, you first need to install Node.js, as npm is bundled with it. Once Node.js is installed, you can access npm through the command line. Here are the basic commands for managing packages:
- Installing a Package: You can install packages using the following command:This command adds the specified package to your node_modules directory and updates your package.json file.
- Uninstalling a Package: To remove a package, use:
- Updating Packages: Keeping packages updated is important for security and performance. To update all packages to their latest versions, execute:
- Listing Installed Packages: To see what packages are currently installed, you can use:
These commands underscore the importance of npm in simplifying package management for developers. Effective use of npm ensures that Node.js projects remain maintainable and scalable.
"npm allows developers to efficiently manage libraries, focusing more on development rather than resource management."
By leveraging npm, developers can enhance their productivity and streamline workflow within the Node.js environment. The significance of understanding npm cannot be overstated, especially for those forging their path in the realm of Node.js development.
Exploring Built-in Modules
Exploring built-in modules in Node.js is fundamental for any developer. These modules provide essential functionalities that help create robust applications without requiring extensive external libraries. Utilising built-in modules enhances productivity, as it allows you to focus on application development instead of repeatedly implementing common features. Node.js offers numerous built-in modules, each serving distinct purposes, which helps to streamline the development process.
File System Module
The File System module, accessed via the variable, is one of the most utilized built-in modules in Node.js. It allows developers to interact with the file system on their machines. This module supports a range of operations, such as reading, writing, and deleting files. For example, if you need to read a file’s contents using this module, you would do the following:
By leveraging the File System module, you can handle numerous file operations easily. It simplifies how you manage file-related tasks within your application. Developers can ensure efficient data handling, making their applications more interactive and responsive. As you explore this module, consider the asynchronous and synchronous methods it offers, depending on your application needs.
HTTP Module
The HTTP module is another critical built-in module. It enables developers to create web servers and work with HTTP requests and responses. This module is vital for any application that requires server-side functionality. An example of creating a simple server using the HTTP module is shown below:
In this code, a basic HTTP server listens for incoming requests on port 3000. When a request is received, it responds with the text "Hello World!". By mastering the HTTP module, you can start building more complex applications that communicate through web protocols. The importance of understanding how to manage requests and responses cannot be overstated, as it is one of the core aspects of web development in Node.js.
Embracing built-in modules like File System and HTTP not only simplifies coding tasks but also promotes better application design through modular development.
In summary, exploring built-in modules is crucial as they form the backbone of many Node.js applications. The more familiar you become with these tools, the more efficient and powerful your code will be.
Error Handling in Node.js
Error handling is a critical aspect of programming in Node.js that often determines the robustness and reliability of applications. In a non-blocking, event-driven environment like Node.js, an error can propagate quickly if not properly managed. The unique nature of Node.js, which relies heavily on asynchronous operations, makes it essential for developers to grasp the subtleties of handling errors. Addressing errors effectively prevents unexpected crashes and ensures a smoother user experience. This section will examine common error types and best practices for implementing error handling in Node.js.
Common Error Types
Errors in Node.js can be broadly categorized into several types. Understanding these common error types allows developers to better anticipate and manage issues that may arise during application runtime.
- ReferenceError: This error occurs when the code references a variable that does not exist in the current scope. It can be frustrating for beginners as it often signifies a typo or oversight.
- TypeError: This type of error arises when a value is not of the expected type. For example, attempting to call a string as if it were a function will trigger a TypeError.
- SyntaxError: Syntax errors are encountered when the code is malformed or not according to JavaScript's syntax rules. This could be missing brackets or using improper statements.
- RangeError: This occurs when a value is not within a set or allowable range. For instance, trying to create an array with a negative length will result in a RangeError.
- Custom Errors: Developers can also create custom error types to suit specific needs within applications, making error management more contextually relevant.
Implementing Error Handling Practices
Properly handling errors in Node.js requires systematic practices. Implementing these practices can significantly improve the robustness of applications and enhance user satisfaction.
- Try-Catch Blocks: This standard error handling structure allows developers to manage synchronous code easily. It can capture errors and handle them gracefully instead of allowing the application to crash.

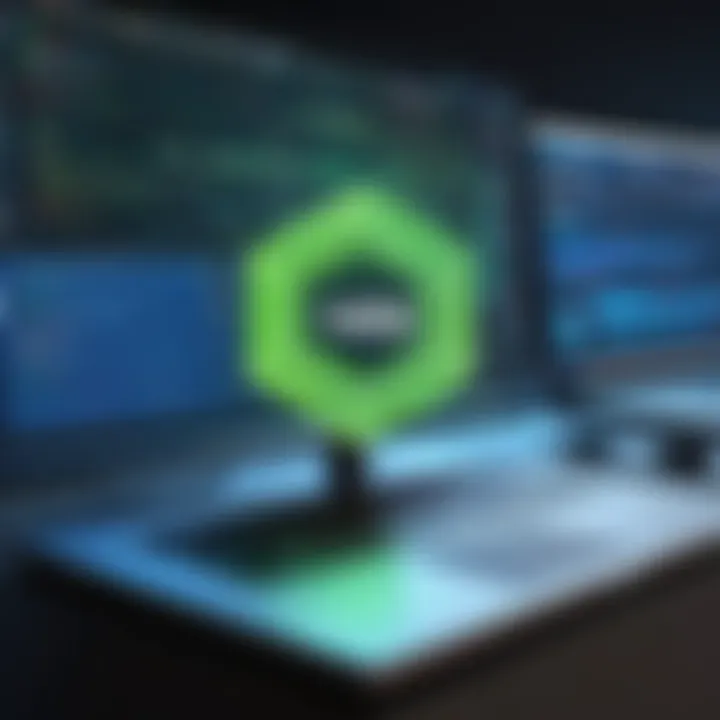
- Error First Callbacks: In asynchronous code, following the convention of using error-first callbacks is crucial. This means the first argument of the callback function should always represent an error, if any. It helps in identifying problems in asynchronous operations more efficiently.
- Using Promises: When working with Promises, errors can be caught using the method. This provides a cleaner way to manage errors compared to traditional callbacks.
- Global Error Handling: To catch unhandled errors, developers can set up global error handlers. This ensures that any uncaught errors are logged, allowing for investigation and fixes later. Leveraging tools like can help detect these.
- Logging Errors: Regularly logging errors is vital for ongoing maintenance of the application. Tools like Winston or Bunyan can help in maintaining structured log files.
It is crucial to remind yourself that effective error handling not only enhances the stability of an application but also contributes significantly to its maintainability.
With these practices in place, Node.js developers can handle issues more effectively, ensuring that applications remain functional even in the face of errors. Proper error handling promotes a culture of robust programming and contributes to the overall health of software projects.
Prelude to Asynchronous Programming
Asynchronous programming is a crucial concept in Node.js, allowing it to achieve high performance and responsiveness. Understanding this concept is vital for beginners because it directly affects how applications handle tasks and user interactions. In contrast to synchronous programming, where tasks are executed one after another, asynchronous programming allows operations to run concurrently. This feature particularly benefits I/O-bound tasks, reducing wait times and improving user experience.
In Node.js, nearly everything is asynchronous. The importance of asynchronous programming cannot be overstated as it enables developers to create applications that are efficient and capable of handling a large number of operations simultaneously.
Callbacks
Callbacks are functions passed as arguments to other functions and executed after a specific task is completed. They serve as a fundamental building block of asynchronous programming in Node.js. By using callbacks, developers can write non-blocking code, which means that the main program flow isn't interrupted while waiting for an operation to finish.
For example, consider reading a file using the module. Instead of freezing the application while the file is being read, a callback can notify the program once the file has been loaded. The syntax is simple but requires careful attention to avoid common pitfalls like callback hell, a scenario where callbacks are nested within callbacks, making the code hard to read and maintain.
Here's a basic example of using a callback with the module:
In this case, once the file reading is done, the callback function either handles an error or processes the data. This pattern illustrates the power of callbacks while also highlighting the importance of graceful error handling within asynchronous tasks.
Promises and Async/Await
Promises represent the next step in controlling asynchronous operations. A promise is an object that may produce a value in the future, either resolved or rejected. It simplifies error handling and leads to cleaner, more manageable code compared to callbacks. Promises are especially useful when dealing with multiple asynchronous operations.
A basic example of a promise in Node.js is shown below:
In this structure, is called when the promise is resolved, and handles any errors. This way of handling asynchronous operations allows developers to chain multiple operations easily, leading to more readable code.
The syntax takes this a step further, making asynchronous code appear synchronous. By declaring a function as , you can use the keyword to pause execution until the promise is resolved. This simplification allows for straightforward error handling through blocks.
Example:
By utilizing async/await, developers can write more intuitive code, making it easier to follow the logic of asynchronous operations. Both promises and async/await are invaluable tools in a Node.js developer's toolkit, allowing efficient error handling and cleanup of otherwise confusing callback scenarios.
Working with Databases
Databases are essential for any application that needs to store and manipulate data. For Node.js beginners, understanding how to work with databases is vital. This knowledge enables you to build dynamic applications that can handle data efficiently. By working with databases, you facilitate the storage and retrieval of user data, which is fundamental to developing web and mobile applications.
In the realm of Node.js, choosing the right database can significantly impact your application's performance and scalability. You can work with various types of databases, including relational databases like MySQL and PostgreSQL, or NoSQL databases such as MongoDB and CouchDB. Each of these databases has unique features and benefits that can suit different use cases.
When working with databases, you should consider factors such as:
- Data structure: How you organize your data will impact how efficient your database queries are.
- Scalability: Flexibility to grow the database as your application demands increase.
- Performance: Speed of data retrieval and storage operations.
In essence, engaging with databases is about leveraging data to enhance the functionality and usability of your applications, making it a crucial component of your Node.js development journey.
Connecting to a Database
Connecting to a database is the first step in utilizing its features. In Node.js, connecting to a database can be achieved through various libraries depending on the type of database you choose. For instance, to connect to a MongoDB database, you would commonly use the library. Here’s a basic outline of how you can establish a connection:
This code snippet illustrates how to connect to a local MongoDB database named . The function returns a promise that resolves upon a successful connection or rejects with an error, which is critical for error handling.
CRUD Operations
CRUD stands for Create, Read, Update, and Delete. These are the four basic operations that all databases must support. It is imperative for Node.js developers to master these operations, as they form the foundation for interacting with your database.
- Create: This operation adds a new record to the database.
- Read: This operation retrieves records from the database.
- Update: This operation modifies existing records in the database.
- Delete: This operation removes records from the database.
In the context of MongoDB, working with CRUD operations can be done easily using . Here is a simple example demonstrating all four operations:
The ability to perform these CRUD operations is fundamental for effective database management, empowering developers to create robust applications.
By mastering the connection to your chosen database and implementing CRUD operations, you will unlock the ability to build meaningful and interactive applications. As you develop your skills, testing and refining these operations will enhance your understanding of how data flows within your Node.js applications.
Security Best Practices
Understanding security best practices in the context of Node.js is crucial for developers at all levels. Node.js is a powerful tool, but with this power comes the responsibility to ensure that applications are secure. Cybersecurity threats are increasing, and a single vulnerability in your application can lead to serious consequences, including data breaches and loss of user trust. Hence, developers need to be proactive in understanding these threats and learning how to mitigate them.
Security practices not only protect your application but also improve its performance and reliability. Implementing the right measures can prevent unauthorized access, data leaks, and other malicious activities that may compromise both the application and its users.
In the following sections, we will explore some common vulnerabilities associated with Node.js applications and discuss practical methods for implementing robust security measures.
Understanding Common Vulnerabilities
Node.js applications face a range of security vulnerabilities. Recognizing these vulnerabilities is the first step towards safeguarding your code. Some of the most common issues include:
- Injection Attacks: This involves inserting malicious code into an application, which can lead to unauthorized data access or manipulation. SQL injection is a notable example, where attackers manipulate SQL queries to gain control over the database.
- Cross-Site Scripting (XSS): This vulnerability allows attackers to inject scripts into web pages viewed by users. This can result in the execution of malicious scripts, often leading to session hijacking or data theft.
- Insecure Dependencies: Node.js relies heavily on third-party packages. Using outdated or unverified packages can introduce vulnerabilities into your application. It is essential to regularly evaluate and update your dependencies.
- Denial of Service (DoS): Attackers can overwhelm an application with excessive requests, causing it to crash or become unresponsive.
Understanding these vulnerabilities allows developers to anticipate potential risks and take steps to mitigate them. Regular code audits, dependency checks, and security training for developers are effective ways to enhance security.
Implementing Security Measures
To fortify your Node.js applications against common vulnerabilities, implementing specific security measures is essential. Here are several strategies:
- Input Validation: Implement strict validation rules for user input. This includes using libraries such as to sanitize and validate input data before processing.
- Use Environment Variables: Store sensitive information like API keys and database credentials in environment variables rather than hardcoding them into your application. Libraries like can help manage these variables securely.
- Employ Security Headers: Add security headers to responses to protect against various attacks. Packages like can automatically configure secure HTTP headers in your Express applications.
- Monitor Dependencies Regularly: Use tools like or to regularly check for known vulnerabilities in your package dependencies. Address any issues promptly.
- Implement Rate Limiting: To mitigate denial of service attacks, implement rate limiting to restrict the number of requests a single client can make in a given timeframe. Middleware like can be useful here.
- Error Handling: Use proper error handling to avoid exposing stack traces or sensitive information in the browser. This can prevent attackers from gaining insight into your code structure.
By prioritizing these security practices, developers can significantly enhance the resilience of their Node.js applications, ensuring a safer experience for both developers and users.
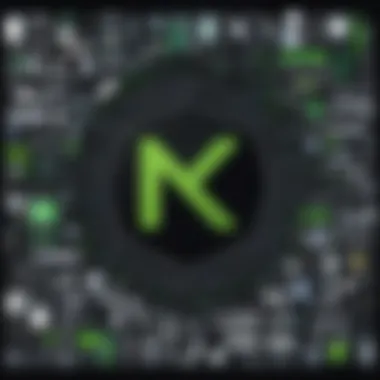
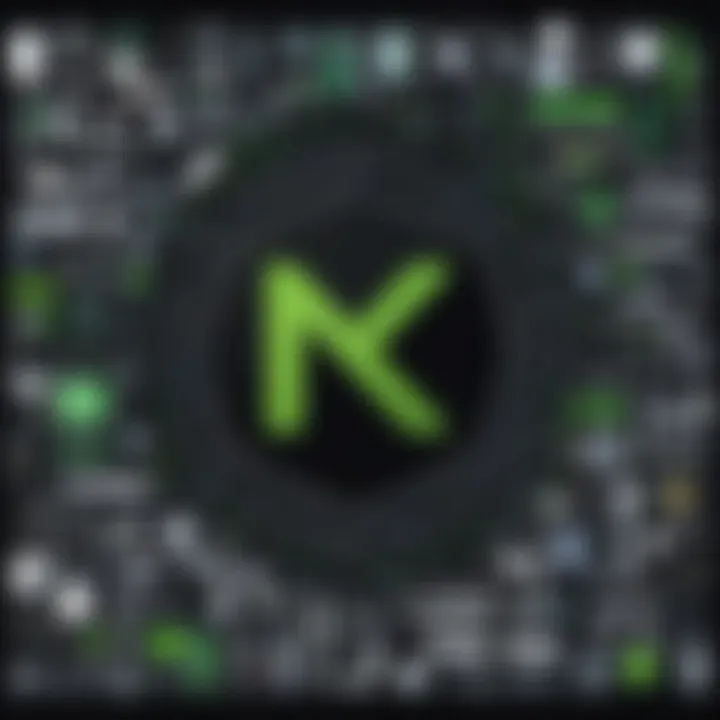
Testing and Debugging Node.js Applications
Testing and debugging are essential skills for any software developer. In Node.js, these practices ensure that applications function correctly and reliably. Both testing and debugging help identify and fix issues early in the development process, which can save time and resources in the long run. Through systematic testing, developers can validate that their code behaves as expected. On the other hand, debugging allows for the exploration of unknown issues that arise during execution.
Engaging in these practices not only enhances code quality but also improves the overall user experience. Since Node.js is primarily used in server-side development, employing proper testing ensures that the backend services are robust and free from defects that could lead to user frustration or data loss.
Debugging Techniques
Debugging in Node.js involves a mix of techniques that allow developers to trace and identify faults within their applications. One common method is using the built-in debugger tool that Node.js provides. To start debugging a script with the built-in debugger, you can run the application using the command:
This opens an interactive debugger in the console, providing breakpoints, stepping through code, and inspecting variables' current states. Another technique involves using tools like Chrome DevTools, which can facilitate debugging of Node.js applications with an easier user interface. Developers can connect their Node.js application to Chrome via the flag and use the DevTools to step through the code visually.
Additionally, developers can leverage logging methods, such as , to output variable values and execution flow. Though simple, this method can be surprisingly effective in highlighting where a program fails to perform as anticipated. Moreover, more sophisticated logging libraries like Winston can be used to provide more structured logging.
Unit Testing Frameworks
Unit testing is crucial to ensuring that individual components of the application work as intended. There are several frameworks available for performing unit testing in Node.js, making it a versatile option for developers. Mocha, for instance, is a popular testing framework offering flexibility and a variety of assertion libraries. It allows developers to define test cases in a straightforward manner, defining what's expected and assessing output accurately.
Another prevalent choice is Jest, developed by Facebook. Jest is known for its ease of use and comes with built-in test runners and coverage reporting, making it ideal for beginners. It supports asynchronous testing, snapshots, and has great documentation to get started quickly.
Important to mention is Chai, which is often used with Mocha for assertions. Chai provides a variety of assertion styles and includes many helpful plugins.
It is vital to write tests before coding the application. This can lead to higher quality code and better project management.
Moreover, continuously integrating testing into the development workflow, often termed continuous integration (CI), ensures that new changes do not break existing functionality. Overall, understanding and implementing effective testing and debugging techniques in Node.js can significantly improve code quality and maintainability.
Deploying Node.js Applications
Deploying Node.js applications is a significant step within the development lifecycle. It marks the transition from a local development environment to a production setting where real users will interact with your application. Understanding this process is essential for beginners and seasoned developers alike.
The deployment process involves several key elements. Not only must the application be packaged correctly, but it must also be optimized for performance and security. Beginners should grasp the importance of this aspect as a poorly deployed application can lead to downtime, security vulnerabilities, and other operational issues.
Moreover, deploying applications effectively can enhance their scalability and maintainability. By choosing the right strategies, developers can ensure their applications handle variable loads and can be updated with minimal disruption. This section discusses specific deployment strategies and the practical use of cloud services to ease the deployment process.
Deployment Strategies
Effective deployment strategies are crucial to ensuring that Node.js applications run smoothly in production environments. Here are several common strategies that developers should consider:
- Containerization: Using tools like Docker allows applications to run in isolated environments. This helps in maintaining consistency across development and production. Containerization can streamline deployments and make scaling easier.
- Continuous Integration/Continuous Deployment (CI/CD): Implementing CI/CD practices automates testing and deployment processes. Using CI tools such as Jenkins or GitHub Actions enables developers to push code reliably and frequently, ensuring that applications are always up-to-date.
- Load Balancing: Distributing traffic among multiple servers ensures that no single server gets overwhelmed. This increases performance and provides redundancy. Tools like Nginx or HAProxy are commonly used in this context.
- Rolling Deployments: This strategy involves gradually releasing updates to a small subset of users before rolling it out to everyone. This minimizes the risk of widespread failures and allows teams to monitor the new release closely before full deployment.
Each deployment strategy has its benefits and should align with specific project requirements and team capacities. Beginners should experiment with several approaches to gain insights into the deployment landscapes.
Using Cloud Services
Adopting cloud services simplifies the complexities of deploying Node.js applications. Cloud providers offer a multitude of features that cater to different aspects of deployment, such as infrastructure management, scalability, and serverless architectures. Here are some notable advantages of using cloud services:
- Ease of Setup: Many cloud platforms, such as Heroku, AWS, or Google Cloud, provide streamlined processes for deploying applications, allowing developers to focus more on coding than on configuring servers.
- Scalability: Cloud services can automatically scale resources up or down based on user demand. This feature ensures applications can maintain performance levels even during traffic spikes.
- Security Features: Cloud providers often include built-in security tools and practices. This can help safeguard applications against potential threats without requiring extensive in-house security expertise.
- Cost Efficiency: With pay-as-you-go models, developers only pay for the resources they utilize. This is particularly beneficial for startups and small teams looking to manage budgets effectively.
"Deployment is an interesting exercise in balancing risk, speed, and cost."
By developing a good grasp of deployment concepts and practices, beginners can transition smoothly from development to production, ensuring their applications reach their users effectively.
Prologue to Frameworks and Libraries
Understanding frameworks and libraries is essential for any developer venturing into Node.js. This area of knowledge facilitates the creation of scalable and maintainable applications. A framework often provides a structured way of organizing code, whereas libraries offer reusable code pieces that enhance the functionality of applications. Aspiring programmers can leverage these tools to streamline development processes, reduce coding errors, and focus on building features rather than reinventing the wheel.
In the Node.js ecosystem, there are several popular frameworks and libraries that cater to various needs. Recognizing the significance of these resources allows developers to choose the right tools for their projects. The adoption of frameworks and libraries can significantly enhance productivity, streamline workflows, and ensure adherence to best practices, which ultimately results in delivering superior software solutions.
Overview of Popular Frameworks
When discussing frameworks for Node.js, several standout options come to mind. Express.js is perhaps the most widely used framework, providing a minimalist and flexible structure for developing web applications. Next, there is NestJS, which utilizes TypeScript to offer a robust architecture, making it suitable for building scalable server-side applications. Another notable mention is Koa.js, designed by the creators of Express, emphasizing a smaller footprint and modern features such as async/await.
Additional frameworks worth noting include Hapi.js, known for its rich functionality and support for building powerful applications, and Sails.js, which brings the conventions of MVC into the Node.js environment. Each framework offers distinct advantages, and their choice largely depends on the project's requirements and the developer's familiarity with the technologies involved.
Selecting the Right Framework
Choosing the right framework is a critical decision that can influence the project's success. Developers should consider several factors when making this choice. First, evaluate the size and complexity of the application. For smaller projects, a lightweight framework like Express.js may suffice. In contrast, larger applications may benefit from the organized structure offered by NestJS or Sails.js.
Next, consider the community support and ecosystem surrounding a framework. A vibrant community indicates a wealth of resources, libraries, and plugins that can be invaluable for troubleshooting and extending the functionality of the framework.
Lastly, assess your team's expertise with the frameworks under consideration. Familiarity can drastically reduce development time and increase efficiency.
In summary, getting acquainted with frameworks and libraries in Node.js not only boosts productivity but also ensures quality and maintainability across projects. It is a fundamental aspect of becoming an effective developer.
Real-World Applications of Node.js
Understanding the real-world applications of Node.js is crucial for beginners who want to see its practical benefits and versatility in software development. Node.js has gained substantial traction in recent years among developers and companies alike due to its event-driven architecture and non-blocking I/O capabilities. This architecture makes it well-suited for I/O-intensive operations, such as web applications that require real-time client-server interaction.
By exploring real-world cases and industry trends, beginners can better appreciate not only the technical strengths of Node.js but also how it fits into the broader context of modern development practices. Professionals in various sectors, including finance, e-commerce, and social media, have successfully implemented Node.js to enhance performance and scalability.
Case Studies
Several prominent companies have embraced Node.js, showcasing its practical uses and benefits across different industries. For example, Netflix utilizes Node.js to handle high volume network requests, enabling smooth streaming experiences for millions of users. By leveraging Node.js, Netflix can manage server-side rendering with ease, which significantly improves page load time and user engagement.
Another instance is LinkedIn, which migrated its mobile application from Ruby on Rails to Node.js. This transition resulted in a substantial performance boost, as it cut down on server response time, thus providing users with a more streamlined and responsive application.
Moreover, companies like PayPal have reported improvements in performance and speed when they shifted to Node.js for their web services. The use of shared code between client and server sides led to greater flexibility and faster feature delivery, ultimately enhancing user satisfaction.
Industry Trends
The demand for real-time applications has surged, and in this regard, Node.js plays a pivotal role. Recent trends indicate a growing interest in using Node.js for developing microservices architecture. This trend allows organizations to break down monolithic applications into smaller, manageable services that can be developed, deployed, and scaled independently.
Another notable industry trend is the rise of serverless architecture. With platforms like AWS Lambda supporting Node.js, developers can build applications without the need to manage server infrastructure. This encourages a focus on writing code and achieving quick iterations while reducing operational overhead.
Furthermore, Node.js is becoming a preferred choice for Internet of Things (IoT) applications, where it can handle numerous concurrent connections efficiently. As IoT devices proliferate, the ability of Node.js to manage high throughput and low-latency communication will be invaluable for developers working in this space.
In summary, real-world applications of Node.js showcase its capabilities and relevance in today's technology landscape. By studying these case studies and industry trends, beginners can gain insights into how to utilize Node.js effectively to meet their project requirements.
Finale and Future Learning Paths
The conclusion represents a pivotal moment in your journey of learning Node.js. It allows you to reflect on what you have learned while setting a path for future exploration. Understanding the consolidation of knowledge is crucial. The format and versatility of Node.js can be leveraging for vast types of applications, from web servers to complex backend systems. By revisiting core principles, you can further solidify your foundation in programming.
Reviewing Key Concepts
It is essential to recap the principal ideas discussed throughout the learning path. Node.js stands out for its non-blocking I/O model which enhances scalability. Your exposure to concepts such as event-driven architecture, asynchronous programming, and npm management lay the groundwork for more complex projects. Reviewing these concepts is advantageous not just for retention, but for preparing you for practical, real-world application.
"By understanding the underlying structures of Node.js, you are better prepared to tackle challenges that arise in software development."
Continuing Your Node.js Journey
Looking beyond this introduction is crucial for those aspiring to deepen their Node.js skills. As you progress, consider delving into numerous frameworks like Express.js or NestJS, which are designed to streamline Node.js development. Engaging with the community through platforms like Reddit or Stack Overflow can expose you to various best practices and real-life solutions.
It is also prudent to keep practicing. Building projects, regardless of scale, refines your abilities and cements your understanding of the platform. Explore advanced topics such as microservices architecture, API development, or cloud deployment techniques that remain pivotal in current environments. Continuous learning, whether through courses, podcasts, or developer meetups, will further enhance your journey.
In sum, embracing ongoing education and practice in Node.js will unlock a multitude of opportunities, making you a versatile developer in the tech landscape.