Mastering Class Definitions in Python Programming
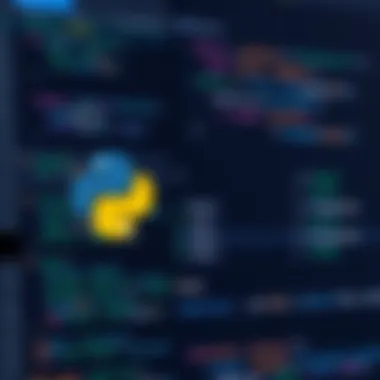
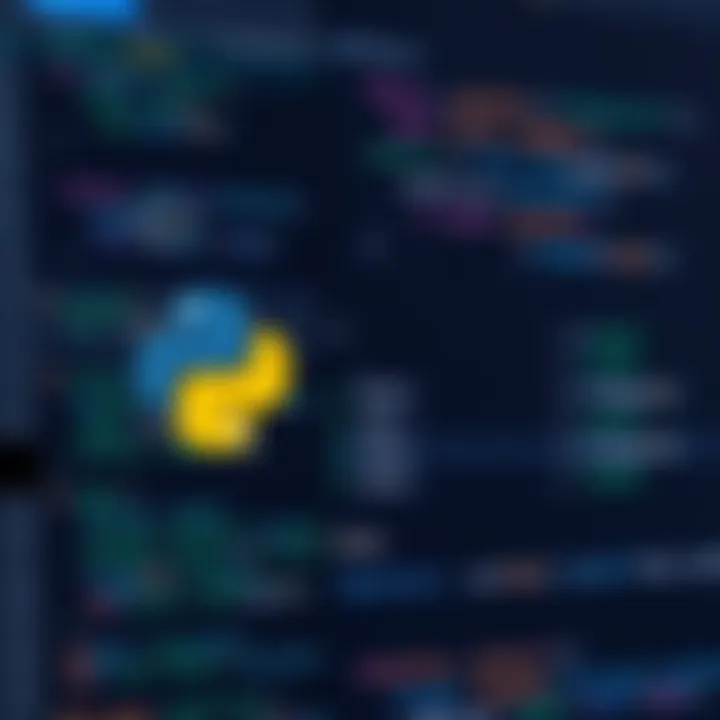
Intro
Understanding how to define classes in Python is far from a mere academic exercise. Itâs like learning to craft your own toolbox â each tool represents a unique class, tailored for a specific job. In Python, classes lay down the backbone of object-oriented programming, allowing developers to encapsulate data and the functions manipulating that data. In todayâs programming landscape, where the demand for clean and manageable code continues to rise, mastering class definitions stands as a foundational skill for every programmer.
From novice coders to seasoned IT professionals, everyone can find value in the structural elegance classes provide. They arenât just a fancy way of organizing code; theyâre a gateway to understanding core principles like encapsulation, abstraction, and inheritance.
As we embark on this exploration, we aim to dissect not just the how, but the why behind class definitions in Python. By diving into the syntax, principles, and some best practices, weâll not only clarify any clouds lurking around this topic but also provide a space for learning about comparisons with other programming paradigms.
Python's versatility often garners attention, but without grasping the crux of its class structures, itâs akin to possessing a high-powered engine without knowing how to drive. Letâs start peeling back the layers and setting the wheels in motion.
Intro to Classes in Python
In the realm of programming, the concept of classes plays a pivotal role, particularly in languages like Python. This section introduces the foundational elements of classes, delving into their significance within the context of object-oriented programming. Classes serve as blueprints for creating objects, encapsulating both data and behavior together, which aids in organizing complex code in a more manageable manner.
When you start to look at programming, it's like trying to assemble a jigsaw puzzle. Each piece of the puzzle can represent different functionalities or data, but how do they all fit together? That's where classes come in. They allow developers to group related data and functions, creating modular and reusable chunks of code. This approach not only enhances readability but also promotes collaboration among various parts of a project.
Defining What a Class Is
A class in Python is essentially a user-defined prototype that defines the attributes and methods of objects created from the class. To put it simply, a class is a way of bundling data and functionality together. For instance, consider a class named . This class might encapsulate attributes like
along with methods that define behaviors, such as
- .
This encapsulation allows us to create multiple instances of , each with different values for those attributes, like one red and another blue . Thus, classes serve both as templates and as containers for data, yielding a perfect blend of structure and flexibility in our programming endeavors.
"Classes enable the transformation of ideas into coded reality. They are the vessels that carry our logic and data throughout the digital landscape."
Purpose of Classes in Programming
The purpose of classes extends beyond mere organization; they introduce several beneficial concepts that redefine how we interact with code. A primary advantage is abstraction, allowing programmers to focus on the high-level functionalities without needing to delve into every detail. This leads to increased efficiency as one can work with complex systems while managing complexity seamlessly.
Another key aspect of classes is inheritance, which enables a new class to adopt properties and behaviors from an existing class. This capability is akin to a family heritage, where children inherit traits from their parents. For example, if we have a class, we could create subclasses such as and , each inheriting shared attributes while also introducing specific functionalities unique to their type.
Basic Syntax of Class Definition
The basic syntax of class definition in Python is fundamental to understanding how objects are structured and utilized within the language. This topic may seem a bit dry at first glance but, trust me, grasping the intricacies of class syntax can open up a world of possibilities in object-oriented programming. This section covers specific elements, the benefits of mastering syntax, and considerations that any programmer should keep in mind.
Clearly, Python's syntax is renowned for its readability and simplicity. It allows programmers to focus more on solving problems than on the intricacies of the language itself. This user-friendliness extends into class definitions, making it easier for novices to establish a solid foundation in programming.
Key Points About Basic Syntax:
- Code readability: Python strives for clarity. The way classes are defined reflects this philosophy through an intuitively structured format.
- Ease of use: With fewer keywords and straightforward indentation, beginners can grasp class definitions without feeling overwhelmed.
- Flexibility: Once you understand the basic syntax, modifying and extending classes becomes a manageable task, even for more complex systems.
However, several aspects warrant closer examination. For instance, while it may be tempting to view the class definition as a mere technicality, it serves as the blueprint for behavior and properties of the relevant objects. This relationship between syntax and object behavior underscores why every detail matters.
"Good coding style aids in the maintainability of code, making it easier for others (and yourself) to read later."
Structure of a Class Declaration
In Python, a class is declared using the keyword, followed by the class name and a colon. Itâs somewhat like putting a sign out front to indicate what lies within. Here's a simple structure of a class declaration:
In this structure:
- Class Name: By Pythonâs conventions, class names should follow the CapWords approach, which improves readability. For example, is preferred over .
- Attributes: These define properties associated with the class. They can be categorized as class attributes (shared among all instances) or instance attributes (specific to each instance).
- Methods: Methods are simply functions defined inside the class that define behaviors. They can take additional parameters, but they should always include as the first parameter to refer to the instance calling the method.
Initialization Method Explained
The initialization method, or method as it is known in Python, plays a crucial role in class definitions. Think of this method as the welcome mat into the world of your object. It is automatically invoked whenever a new instance of the class is created, serving to initialize any attributes you've defined.
Here's how the method is structured:
- Parameters: As depicted, can accept parameters, which makes it dynamic. By passing values during the creation of an instance, you fine-tune object characteristics right from the get-go.
- Self Parameter: It's noteworthy that the parameter refers to the instance being created, acting much like a dictionary to store object attributes. One can understand how this allows each object to maintain its unique state.
Hence, good utilization of the initialization method ensures that every time a new object spins up, it arrives ready to roll, fully equipped with the necessary attributes.
The elegance in Python's class definition, although initially seeming straightforward, packs a punch once understood. By mastering the syntax, programmers not only grasp how to implement object-oriented principles but create efficient, readable, and maintainable code.
Understanding Attributes and Methods
When we talk about classes in Python, attributes and methods are like the bread and butter of object-oriented programming. They essentially define what each class can do and what information each instance holds. Recognizing the distinction between attributes and methods is crucial for anyone who wants to harness the full power of classes. After all, itâs not just about defining a class; itâs also about how to interact with it effectively.
Class Attributes vs Instance Attributes
To dive deeper, letâs break down what class attributes and instance attributes are. Simply put, class attributes are variables that belong to the class itself rather than a specific instance. This means that they are shared across all instances of that class. For example, if you have a class with a class attribute called set to , every instance of will have equal to .
On the other hand, instance attributes are tied to individual objects. Each instance can hold different values. If you have two instances of the class, one could represent a red sedan and another a blue coupe, each with its specific attributes like or . Hereâs a clearer picture:
In this example, is a class attribute, while and are instance attributes. Understanding this distinction helps in managing data and ensuring that each object's state is unique when needed, yet still offering shared characteristics.
Benefits of Understanding Attributes
Grasping class and instance attributes aids in better designing classes. Here are some benefits:
- Memory Efficiency: Class attributes reduce redundancy, saving memory when the same value is to be shared.
- Organizational Clarity: Knowing the difference facilitates clearer code organization, improving readability.
- Behavior Preservation: It helps in keeping the behavior consistent across instances, especially for shared properties.
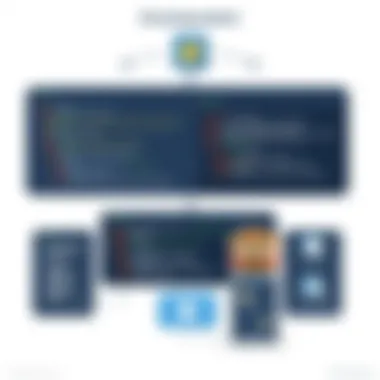
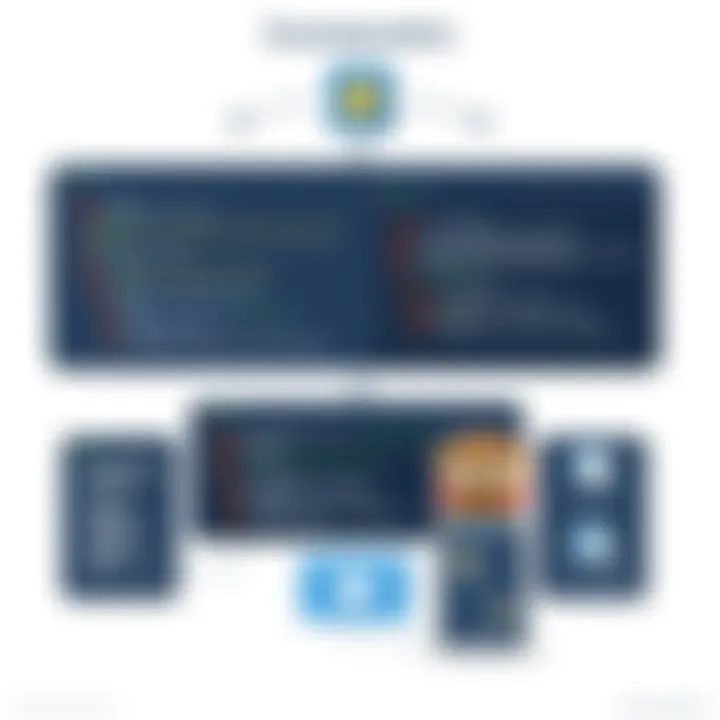
Defining and Calling Methods
Methods in Python classes are essentially functions that define the behavior of class objects. They encapsulate the actions an object of the class can perform, interacting with both attributes and other methods. The most common method in any Python class is the method, which is called when an object is instantiated.
When defining methods, itâs vital to remember that they take the instance of the class as the first argument, conventionally named . This allows each method to access the instanceâs attributes and other methods. For instance, in the class above, we could add a method to display the car details:
This outputs: This car is a red sedan with 4 wheels.
This clearly illustrates that methods allow for functionalities that can manipulate an objectâs internal state or perform operations relevant to that object. The ability to create methods enhances the interactivity of your class, enabling it to respond to input or execute specific tasks based on its attributes.
Considerations When Working with Methods
While working with methods, keep a few key points in mind:
- Naming Conventions: Use descriptive names. This makes it easier to understand what each method does without delving into the code.
- Maintainability: Keep methods focused on a single action or task for greater maintainability and reusability.
- Encapsulation: This principle encourages the use of methods to interact with object attributes rather than accessing them directly, aligning with best practices and promoting cleaner code.
Ultimately, understanding attributes and methods is not just a fundamental part of using classes in Python, it's also a cornerstone of writing clear, efficient, and maintainable code. As you continue to explore these concepts, youâll find they provide the building blocks necessary for more advanced topics in object-oriented programming.
Encapsulation and Data Hiding
Encapsulation is one of the fundamental concepts of object-oriented programming, especially in Python. At its core, encapsulation is all about bundling the data (attributes) and methods (functions) that operate on that data into a single unit or class. This not only helps in organizing code, but it also plays a pivotal role in maintaining the integrity of the data within the class. By controlling access to the class's internal representation, encapsulation allows for data hiding, which can prevent unwanted interference and misuse of class data.
When we discuss data hiding, we're looking at how data within a class can be protected from outside interference. It's much like keeping valuable items locked away. The benefits of encapsulation and data hiding include:
- Prevention of unintended access: By limiting visibility to certain details, you ensure that the class's integrity remains intact.
- Ease of maintenance: When the internal implementation changes, as long as the interface remains consistent, external code doesn't need to change.
- Reduced complexity: Focusing only on the public interface can simplify how other parts of the program interact with the class.
Think of it this way: if you bought a car, you wouldn't need to know how the engine works to drive it. That's encapsulation at play. So, managing what can and cannot be accessed becomes crucial to a smooth running program.
Importance of Encapsulation
In Python, encapsulation is imperative for a few reasons. First, it allows data and behavior to be kept together in a single entity. This not only makes logical sense but also enhances readability. More often than not, when you come across an object, you want to know how to interact with it without diving into the nitty-gritty of its internal workings.
Moreover, encapsulation holds a special place in fostering safe programming practices by:
- Preventing the accidental modification of data. Using the or keywords allows developers to control how attributes are accessed.
- Enforcing a clear separation between interface and implementation. This clear delineation is vital as it outlines what functions are available to the rest of the program.
- Facilitating debugging and testing. Fewer dependencies mean simpler isolation of issues down the line.
In essence, encapsulation elevates code quality by enforcing good practices, which results in fewer bugs and a more robust codebase.
Private and Protected Attributes
In Python, class attributes are typically public by default, which means they can be accessed from outside the class. However, encapsulation allows for a deeper level of protection through private and protected attributes.
Private attributes are indicated by a double underscore prefix (e.g., ). The idea here is that these attributes should not be accessible or modifiable from outside the class. While Python relies on convention for access control, it still provides mechanisms to discourage external access.
On the other hand, protected attributes, signaled by a single underscore prefix (e.g., ), indicate a signal that this attribute is meant as an internal variable of the class. While still technically accessible from outside, it's a strong suggestion not to access these attributes directly.
To summarize:
- Private Attributes:
- Protected Attributes:
- Cannot be accessed directly from outside the class.
- Useful for internal logic where control of modification is crucial.
- Should be treated with care, indicating they're not for consumption outside the class but are still accessible.
In practice, encapsulating attributes leads to better design. It provides a clear contract of what attributes are safe to use and which ones should remain hidden. Thus, encapsulation combined with the distinction between private and protected attributes creates a disciplined coding environment and fosters a culture of respecting data integrity.
Inheritance in Python Classes
Inheritance is a fundamental concept in object-oriented programming, particularly in Python. It enables new classes to adopt properties and methods from existing classes, streamlining design and promoting code reusability. In this section, we will explore the essence of inheritance, its benefits, and critical aspects that programmers should be mindful of.
Concept of Inheritance
At its core, inheritance stands as a link between two classes, allowing one class to inherit the characteristics of another. The class that inherits the properties is known as the subclass, whereas the class being inherited from is called the parent class or superclass.
For instance, consider a scenario where you have a base class named . From this class, you can create subclasses like and , which inherit standard attributes like , , and methods like and . This structure not only defines a clear relationship between the classes but also enhances maintainability of the code, as changes made in the parent class reflect in the subclasses.
One practical benefit of inheritance is that it allows developers to build upon existing code without having to rewrite it from scratch. This leads to efficiency in coding and significantly reduces potential bugs. Thus, when used wisely, inheritance can be a tremendous asset in your programming toolkit.
Overriding Methods in Subclasses
Overriding methods is another powerful facet of inheritance. A subclass can provide its specific implementation of a method that is already defined in its superclass. This means if you have a method in the class, such as , the subclass can override this method to provide unique behavior tailored to a car, for example, activating ignition systems, while retaining the original method form in .
Here's a brief illustration:
When you create instances of or and call the method, you will see distinctly different behaviors.
Understanding method overriding is crucial. It grants subclasses the flexibility to modify or enhance inherited behaviors, resulting in more tailored and efficient applications.
Thus, programmers must use overriding cautiously, ensuring that the overriding methods align well with the intended behavior of the subclass while maintaining the essence of the parent class. Awareness of issues like the diamond problem in multiple inheritance can prevent potential pitfalls when designing class hierarchies.
In summary, inheritance not only provides a structured way to organize code but also fosters greater agility in programming. It allows for building on existing foundations while facilitating the unique needs of specific situations.
Polymorphism and Its Application
Polymorphism stands as one of the cornerstones of object-oriented programming in Python. It allows objects of different classes to be treated as objects of a common superclass. This capability brings about several benefits including code reusability and flexibility. Imagine creating a single function that can operate on objects of various classes without needing to rewrite code. This is where the beauty of polymorphism plays out, making it an indispensable tool in a programmer's toolkit.
Understanding Polymorphism
At its core, polymorphism means "many shapes." In Python, it manifests in various forms, notably through method overriding and duck typing. When a subclass provides a specific implementation of a method that is already defined in its superclass, this is termed method overriding. On the other hand, duck typing leans heavily on the idea that if an object behaves as a certain type, it can be treated as that type irrespective of its actual type.
Key Concepts of Polymorphism:
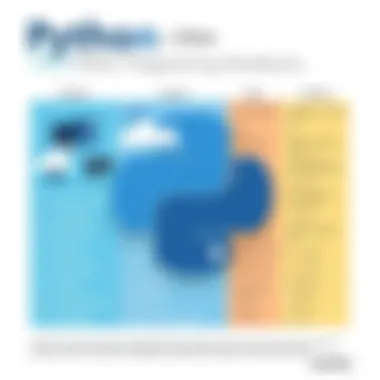
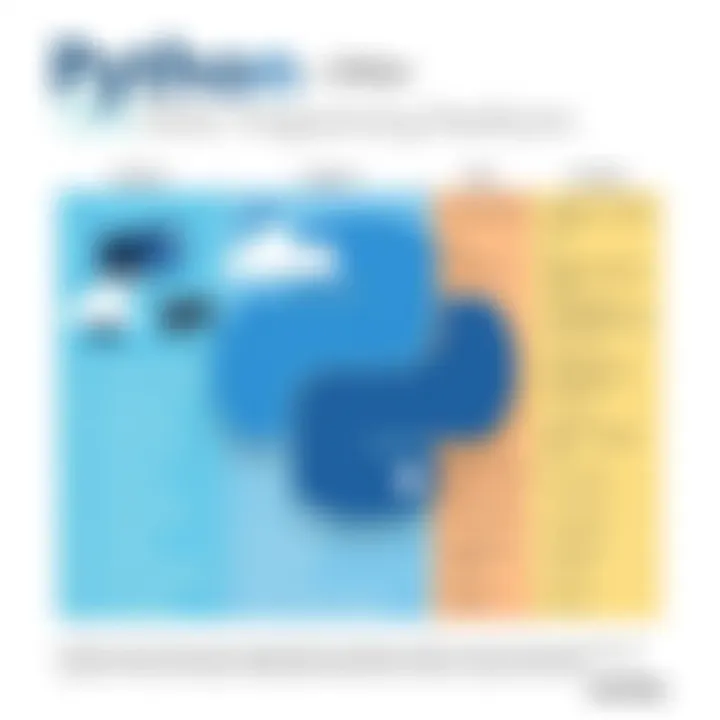
- Method Overriding: Allows subclasses to define their behavior for a method originally provided by a superclass.
- Duck Typing: Focuses on what an object can do, rather than what it is. For instance, if a function requires an object to have a specific method, it only checks if that method exists.
Considering these concepts, the implementation of polymorphism can greatly streamline your code. Instead of writing multiple functions to handle different object types, a single function can handle all compatible types. This not only reduces redundancy but also enhances maintainability.
Examples of Polymorphism in Classes
To illustrate polymorphism with practical examples, letâs take a look at a scenario where multiple geometric shapes share a common behaviorâcalculating area.
Consider the Following Code:
The example above showcases how the function can accept different shapesâ and âwithout needing to worry about the specific types being passed in. Each shape class implements its own version of the method. As a result, when we call or , the appropriate method gets invoked due to polymorphism.
Polymorphism not only simplifies code but also adheres to the principle of programming to an interface, promoting better software design.
In summary, polymorphism enriches your programming experience by allowing for flexible and generalizable code. It ensures that your classes can communicate in a seamless manner, making your code cleaner and more effective while lowering the barrier to debugging and updates. With polymorphism, the landscape of whatâs possible in class interactions expands significantly.
Class Interactions and Relationships
In Python programming, understanding how classes interact with each other is crucial for creating robust, maintainable software. The relationships between classes can greatly influence the architecture of a project. So, letâs break down the different types of class interactions: association, aggregation, and composition. Each of these forms a unique way of connecting classes, providing varying degrees of dependency and organization.
Association, Aggregation, and Composition
Association is the broadest form of relationship between classes. In practice, this means that one class uses or interacts with another, but neither class is tightly coupled to the other. For instance, imagine a class and a class. A teacher can be associated with a school, but the teacher doesnât necessarily need to be a part of that schoolâs implementation. This comes in handy when you want to maintain loose coupling while still allowing interaction.
Aggregation can be thought of as a specialized form of association. It implies a whole-part relationship where a class can exist independently of its parent class. If we consider a class that aggregates objects, the books can exist without the library. If the library closes down, the books are still intact and can be placed elsewhere. This provides an organizational structure while keeping components modular and flexible.
On the other hand, Composition introduces a tighter bond between classes. Here, the lifecycle of the component classes depends on the parent class. For example, a class can own objects. If the car is destroyed, the engine cannot exist independently; it is designed to work solely within the context of that car. This demonstrates a strong relationship where one class strictly owns the lifetime of another. Thus, it becomes evident that knowing when to use association, aggregation, or composition can help in building a sound architecture that maximizes reusability and minimizes code rigidity.
Understanding Class Dependency
Class dependency reflects how classes rely on one another. Itâs key to managing changes over time in any codebase. When one class is dependent on another, a change can ripple through your system and create maintenance headaches. This is where understanding class interactions becomes vital because you want to minimize dependencies while allowing necessary interactions.
One approach is to use dependency injection, allowing you to pass in dependencies from the outside rather than hardcoding them inside a class. This way, you can change dependencies with greater ease without breaking the functionality of your classes. Furthermore, striving for dependency inversionâan essential principle in solid architectureâencourages the design of systems that are less prone to changes.
Moreover, employing design patterns like the strategy or observer pattern can help manage dependencies more effectively. By doing so, classes can interact while keeping them decoupled from each other. This is beneficial in complex systems where changes in one part of the application shouldnât send shockwaves through others. Ultimately, understanding these dependencies not only fosters better code organization but also aids in the evolution of the application as requirements change over time.
Best Practices for Class Design
Designing classes in Python requires a thoughtful approach; poor designs can lead to software that's harder to maintain and extend. By adhering to a few best practices, programmers can improve code quality and promote a better software structure. These practices revolve around clarity, reusability, and simplicity. Every developer, regardless of experience level, should consider these aspects when defining classes, as they significantly impact the longevity and comprehensibility of the code.
Principles for Clean Class Structure
A clean class structure is not just a matter of aestheticsâit's about functionality and maintainability. The following principles can guide developers:
- Single Responsibility Principle: Each class should have only one responsibility. This makes it easier to understand and manage. If a class starts handling multiple responsibilities, it may become more complex than necessary.
- Clear Naming Conventions: Class names should be descriptive. If you name a class , it should clearly relate to employee properties and behaviors, avoiding vague names that donât reflect its purpose. This assists not only current developers but also future maintainers who may work with the code.
- Favor Composition Over Inheritance: While inheritance has its uses, excessive reliance can lead to a tangled web of classes. Composition, on the other hand, allows the creation of complex types by combining simpler ones, promoting flexibility.
- Limit Class Size: Aiming for smaller classes encourages focused functionality. Classes that do too much can become unwieldy, making them challenging to work with and understand.
- Encapsulate Behavior: Methods should be designed to operate on class attributes tightly associated with them. This encapsulation allows for safer interaction with an object's state, reducing the potential for bugs.
Implementing these principles can solidify a codebase and improve its maintainability over time.
"Good programs are not just written; they are rewritten."
â Unknown
Common Pitfalls in Class Definition
Even seasoned developers can stumble into traps when defining classes. Recognizing these pitfalls is crucial for anyone looking to refine their programming skills while working with classes:
- Overcomplicated Class Definitions: Sometimes, developers can over-engineer their classes, adding unnecessary complexity. Aim to keep it simple. If a class has multiple constructors or methods that could be more coherent, it may be time to reevaluate.
- Ignoring Documentation: A lack of documentation can lead to confusion down the road. Classes should come with docstrings detailing purpose, methods, and attributes for future reference. This becomes vital in team environments.
- Not Using Access Modifiers: Failing to leverage private and protected attributes can lead to unwanted interference with an object's state. Understanding when and how to protect data is essential for encapsulation.
- Unnecessary Dependencies: Heavy reliance on other classes can create a fragile structure. Striving for low coupling between classes enhances code resilience and flexibility.
- Neglecting Tests: Classes should be tested independently to ensure that they function correctly. Skipping tests can lead to unforeseen bugs when classes interact.
Avoiding these pitfalls not only saves time but also enhances code readability and reliability. Ultimately, by being aware of common missteps, programmers can direct their efforts toward more robust class designs.
Error Handling in Classes
Error handling within classes is a vital aspect of robust software development, allowing programmers to create applications that can gracefully manage unexpected situations. In the realm of Python programming, effective error handling ensures that your class-based structures not only function as intended but also provide the flexibility to handle exceptions without crashing the application. This topic is especially important in classes, as they can encapsulate both data and methods, making it crucial to implement error management that fosters reliability.
The ability to anticipate problems and manage them appropriately is a skill every aspiring and experienced programmer should cultivate. By addressing various possible errors, you maintain control over your program's flow and user experience, turning potential failures into manageable parts of the logic.
Using Try-Except Blocks
Try-except blocks are the fundamental building blocks of error handling in Python. They allow developers to test a block of code for errors, helping in isolating and managing exception scenarios without crashing the whole program. The typical structure is straightforward:
When using try-except blocks in your classes, there's a natural tendency to encapsulate logic within methods. For instance, if you have a method that performs a file operation, wrapping that operation within a try-except block can save you from unforeseen issues such as file accessibility problems:
In this case, users of the class are spared from harsh program failures, and instead, receive meaningful feedback when something goes amiss.
Creating Custom Exceptions
Custom exceptions are another powerful tool in the Python arsenal for tailored error handling. By defining your own exception types, you can provide more specific and informative error messages, enhancing both debugging and user experience.
Creating a custom exception is as simple as inheriting from the built-in class. Hereâs an example:
Then, you can raise this exception within your class whenever a specific error condition is encountered.
This level of specificity can be invaluable. When one of your methods raises a , the calling code is informed that a particular error occurred, enabling better error handling strategies.
"Error handling is not just about catching errors; it's about defining the story your program tells when things don't go as planned."
Real-World Applications of Classes
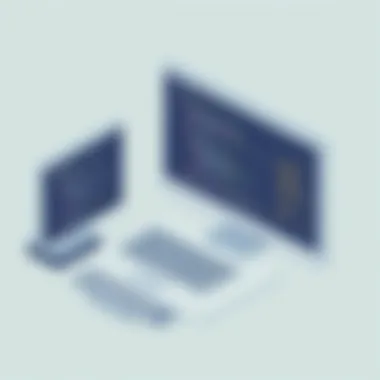
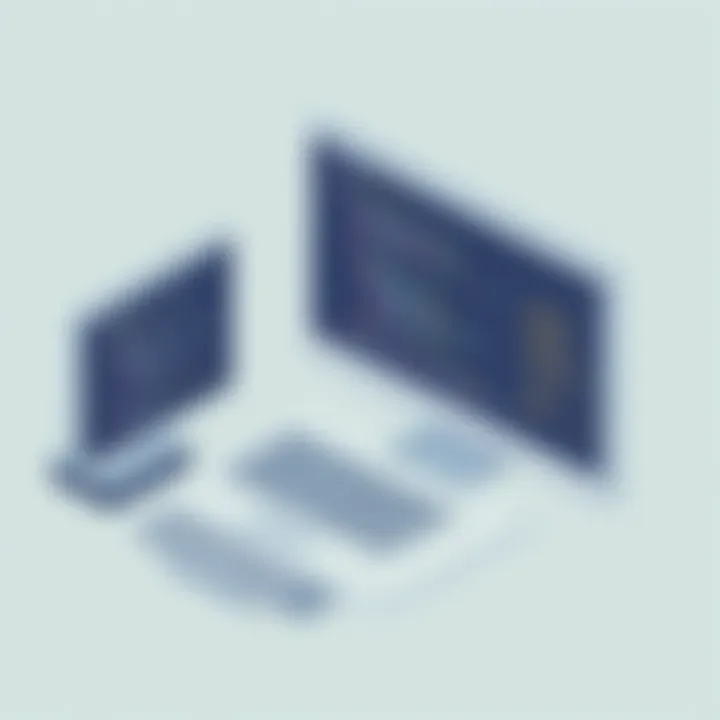
Classes in Python are much more than mere structures; they serve as the backbone of modern software development. They allow programmers to create well-organized, modular, and reusable code, making it easier to manage large codebases. In practical terms, understanding and applying class definitions can greatly enhance the efficiency of a programming project.
By using classes, developers can simulate real-world entities, encapsulating their attributes and behaviors. This approach leads to code that is not just functional but also aligned with logical representations of the problems being solved. Moreover, classes facilitate scalability and maintenance, which are crucial in any significant software endeavor.
One specific aspect worth highlighting is the promotion of code reuse. For instance, once a class is defined, it can be used as a blueprint for creating multiple objects without duplicating code. This leads to cleaner and more efficient programming practices.
"In coding, clarity is king. Classes help ensure that the intricate web of relationships and functionalities remains comprehensible."
Case Study: Class-Based Project
Letâs consider a hypothetical project for an online bookstore. A well-structured class design for this type of application can encompass various elements, from product management to user interactions. At its core, a class might define properties such as title, author, price, and availability.
In the case of this bookstore, you'd probably have additional classes for , , and . Each of these can interact seamlessly through methods and attributes defined in their respective classes. The relationships between these classes illustrate the power of object-oriented design: for instance, an class can hold multiple instances, exploring the concept of aggregation.
Roles of Classes in Software Development
In the realm of software development, classes fill several essential roles. First and foremost, they act as blueprints for creating objects. The use of classes can lead to better-organized code, which is especially important in large teams or projects.
- Modularity: Classes allow developers to break down complex problems into smaller, manageable parts. This modularity not only makes the code easier to comprehend but also simplifies testing and debugging.
- Encapsulation: By keeping data and methods related to a class together, it promotes data protection and prevents unintended interference with the internal workings of an object.
- Abstraction: Classes enable programmers to focus more on the high-level operations without delving into the complexities beneath. For example, users interacting with a class do not need to know about how the borrowing mechanism works internally.
- Inheritance: This powerful feature allows a new class to inherit properties and methods from existing classes, enhancing code reusability and simplicity in design.
- Polymorphism: It gives classes a flexible nature where they can behave in different ways based on the object type. This is vital for designing systems with varied behavior across similar entities.
Through these roles, classes contribute significantly to a well-structured coding approach. In terms of performance and maintainability, they can reduce the possibility of errors while providing clear pathways for expansion. Thus, their real-world applications extend far beyond mere syntaxâthey embody fundamental principles that guide effective software engineering.
Classes vs Other Programming Structures
Understanding the nuances of classes in the grand tapestry of programming structures is vital. It helps to grasp not just how they function, but why they are often chosen over other paradigms. Classes offer an organized and efficient way to encapsulate data and behavior, which can typically lead to clearer and more maintainable code. This makes them a preferred choice in object-oriented programming, especially in Python.
Comparing Classes to Functions
When one considers classes, it's hard not to draw comparisons with functions. Both play pivotal roles in programming, yet they serve fundamentally different purposes.
- Purpose and Structure: Functions are primarily used for executing a specific task or calculation. They take inputs, process them, and return outputs. In contrast, classes bundle data (attributes) and methods (functions) within single entities. This encapsulation allows classes to manage related functionalities and attributes more cohesively.
- Scope of Use: Functions are generally stateless, meaning they donât retain data between calls. Every time a function is invoked, it's like starting fresh. Classes, however, can maintain state through instance variables, providing a context that functions lack. This statefulness is beneficial when managing a collection of related data and behaviors.
- Reusability and Extensibility: While functions can be reused, classes take reusability a step further. They allow for inheritance, meaning one class can inherit attributes and methods from another. This makes it easier to extend functionalities without duplicating code.
In short, while functions are like tools in a toolbox, classes are like entire toolboxes. To illustrate:
Classes in Functional Programming Context
When diving into the world of functional programming, the role of classes becomes rather intriguing. Functional programming emphasizes immutability and stateless functions, often seeing classes as unwelcome baggage. They view functions as first-class citizens; however, classes can play a complementary role in certain situations.
- Hybrid Approach: Some languages that support both paradigms, such as Python, allow developers to mix functional elements with OOP. For example, you might have a class that manages configurations but use pure functions for data processing.
- State Management: Classes can help manage state in an environment where functions focus on passing data without retaining it. They can encapsulate behaviors that need to maintain context, bridging the gap between pure functions and stateful operations.
- Enhanced Readability and Organization: In scenarios requiring more structured approaches, classes can break down complex functionality into manageable pieces, enhancing code clarity. Just as you keep your clothes organized in a closet, classes keep related functions and data neatly bundled.
In summary, while classes and functions serve different purposes, they can coexist harmoniously. The choice between using classes or functions, or a blend of both, ultimately hinges on the context of your application and what best suits your programming style.
When mastering programming, understanding the relationship between classes and functions can reshape how you approach problem-solving.
Advanced Topics in Class Definition
In the realm of Python, diving into advanced topics surrounding class definitions sheds light on powerful tools that enhance programming efficiency and capability. Especially for those who have a grasp on the basics, understanding these advanced elements can considerably elevate oneâs programming skills. Two such pivotal concepts are metaclasses and class decorators. Each serves distinct purposes but collectively they open a doorway to more flexible, dynamic, and elevated programming practices.
Metaclasses and Their Utility
Metaclasses can be somewhat of a gray area for many programmers; they are objects of class creation. To put it simply, whereas a class defines how an instance behaves, a metaclass defines how a class behaves. This may sound like abstract mumbo-jumbo, but letâs untangle it.
When you create a class in Python, you usually use the default metaclass, which is . However, when you need more control over how classes are instantiated or modified, metaclasses come into play. They provide a way to intercept the class creation process and can add attributes or methods or even modify class properties before it's created.
Consider this example:
This snippet illustrates a metaclass that, upon class creation, adds a new attribute to . By utilizing metaclasses, programmers can define unique behaviors or properties that cater to specific needs, crafting a more tailored and efficient class structure.
Class Decorators as a Design Tool
Now, class decorators are yet another fascinating aspect of Python classes that should not be overlooked. A class decorator is a function that can modify or enhance a class definition. This concept blurs the boundaries between functions and classes, affording flexibility in programming practices.
Imagine you want to add a method to a class without actually modifying the class definition itself. This can be achieved through a class decorator. Here's a simple illustration:
In this code, the function serves as a decorator that injects a new method into . The ability to extend classes in such a manner allows for cleaner and more manageable code, especially as complex systems scale.
By incorporating these advanced topics, programmers can take their understanding of Python to the next level, enhancing both their effectiveness and creativity in software development.
"Exploring advanced topics in Python class definitions can unlock unique programming capabilities, allowing for designs that were previously thought to be beyond reach."
To summarize, metaclasses and class decorators provide a toolkit for those seeking to refine their code like a craftsman. By using these advanced concepts judiciously, one can construct more innovative, readable, and maintainable code in their Python projects. Understanding these elements not only aids in broadening oneâs skill set but also enhances oneâs approach to problem-solving through programming.
Closure and Further Reading
In wrapping up this exploration of class definitions in Python, it's essential to recognize the significance of this framework within programming. Classes serve as a backbone for organizing code, fostering maintainability, and enhancing readability. They empower developers to create intricate systems where data and functions are cohesively bundled. Without a firm grasp on how to define and utilize classes effectively, programmers risk introducing confusion and inefficiency into their codebases.
Understanding class definitions doesn't merely stop at syntax; it extends into mastering the principles behind encapsulation, inheritance, and polymorphism, which can significantly influence the quality of software development. Mastering these topics not only strengthens one's programming skills but also equips aspiring developers and seasoned pros alike with tools to create scalable and robust applications.
Moreover, the ever-evolving tech landscape demands a commitment to continuous learning. Thus, further reading becomes indispensable for anyone venturing into or already immersed in Python development. Engaging with supplementary materials enriches the learning experience, deepens comprehension, and keeps professionals updated on the latest best practices.
"Learning never exhausts the mind." - Leonardo da Vinci
Summary of Key Insights
In this article, weâve delved into the essentials of defining classes in Python, highlighting the following key insights:
- The Role of Classes: Classes organize software projects, encapsulating data and behavior to reflect real-world entities.
- Syntax Matters: A proper understanding of class syntax is foundational for writing effective Python code.
- Attributes and Methods: Differentiating between class and instance attributes, alongside defining methods, provides clarity on object behavior.
- Encapsulation: It is vital for protecting data integrity through visibility rules.
- Inheritance and Polymorphism: These principles allow code reuse and flexibility, enhancing development efficiency.
Recommended Resources for Learners
To deepen your understanding of class definitions and enhance your programming skills, consider exploring the following resources:
- Python Documentation: python.org offers a comprehensive guide on classes and objects.
- Wikipedia: The Wikipedia page on Object-Oriented Programming provides context and history.
- Stack Overflow: Engaging with the community on platforms like reddit.com can offer insights and practical advice.
- Books: Python Crash Course by Eric Matthes is an excellent entry point for beginners.