Unlocking the Power of Python Modules: A Programmer's In-Depth Guide
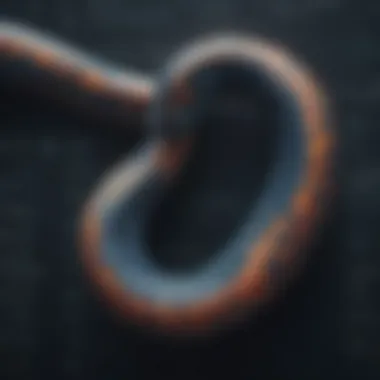
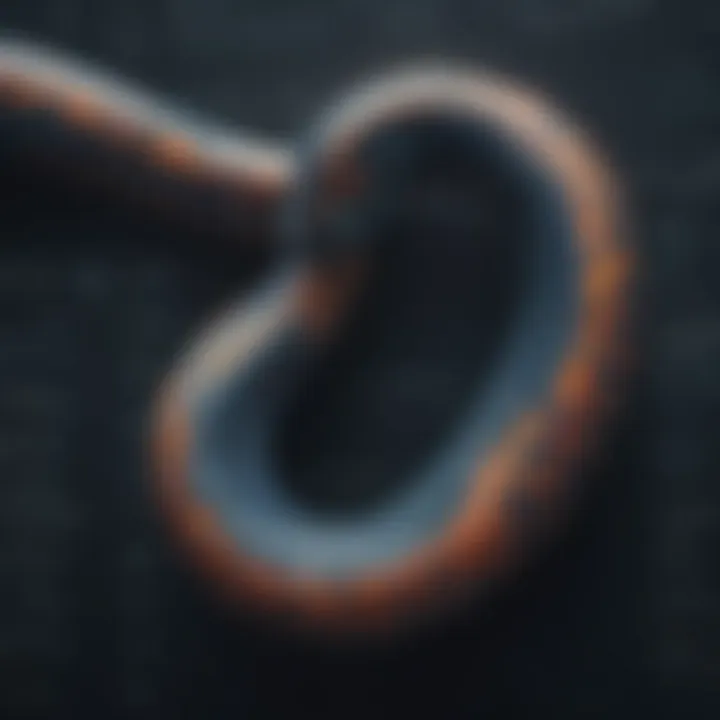
Coding Challenges
In the realm of Python programming, mastering Python modules is paramount for any programmer seeking to enhance code organization, efficiency, and collaboration. Weekly coding challenges serve as an excellent platform to put your Python module knowledge into practice and refine your programming skills. These challenges typically present varying levels of complexity, allowing programmers to tackle diverse problems using Python modules. By engaging in these coding challenges, programmers can deepen their understanding of Python modules and explore innovative ways to optimize their code structure.
Problem Solutions and Explanations
Each coding challenge provides an opportunity to apply Python modules in solving real-world programming problems. As programmers work through these challenges, they develop a repertoire of solutions utilizing different Python modules, gaining invaluable hands-on experience in leveraging these tools effectively. After completing a challenge, detailed explanations of the solutions further enhance the learning process, demystifying complex algorithms and strategies employed. This section offers a comprehensive breakdown of the thought processes behind each solution, guiding programmers to grasp the nuances of Python module application.
Tips and Strategies for Coding Challenges
Navigating coding challenges requires not only proficiency in Python modules but also strategic thinking and problem-solving acumen. Here, we offer a collection of expert-backed tips and strategies to assist programmers in approaching coding challenges strategically. From optimizing code efficiency to debugging techniques, these insights aim to equip programmers with the essential skills needed to excel in coding challenges. By incorporating these tips into their practice, programmers can enhance their overall performance and deepen their understanding of Python modules' functionality.
Community Participation Highlights
Engaging with a vibrant coding community can further enrich the coding challenge experience. Through collaboration and sharing of insights, programmers can explore diverse perspectives on Python module usage and discover innovative approaches to problem-solving. Community participation highlights showcase exemplary solutions, foster discussions on best practices, and create a conducive environment for knowledge exchange. By actively participating in the coding community, programmers can elevate their skills, expand their technical repertoire, and forge valuable connections within the programming landscape.
Technology Trends
Python modules continue to evolve alongside technological advancements, shaping the landscape of programming and software development. Stay abreast of the latest technological innovations that intersect with Python modules, influencing coding practices and methodologies worldwide. Delve into emerging technologies that leverage Python modules as core components, revolutionizing industries and empowering programmers to embark on groundbreaking projects. Explore how technology trends intersect with Python modules, driving innovation, and pushing the boundaries of what's achievable in the programming realm.
Introduction to Python Modules
In the realm of Python programming, understanding Python modules is paramount for efficient code organization, reuse, and collaboration. Python modules serve as discrete units of code that can be imported and utilized within other Python programs. By breaking down the complexities of Python modules, programmers can streamline their workflows, enhance code reusability, and encourage modular programming practices for scalability and maintainability in software projects. This section sheds light on the essential concepts surrounding Python modules, laying the groundwork for exploring their functionalities and applications.
Definition of Python Modules
What are Python modules?
Revolving at the core of Python programming, Python modules represent encapsulated units of code that consist of classes, functions, and variables. Separating code into modules enables better organization and abstraction, fostering a modular programming approach that promotes code reusability and maintainability. Python modules encapsulate related functionalities, allowing for logical grouping and separation in a software project. The modularity of Python modules simplifies code management and promotes clean, scalable codebases, making them integral components in software development.
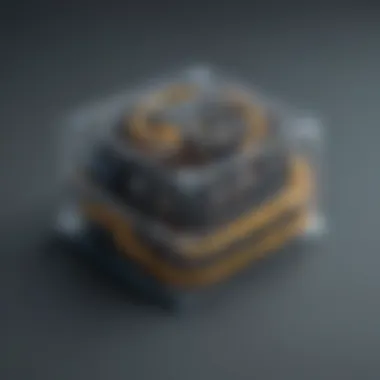
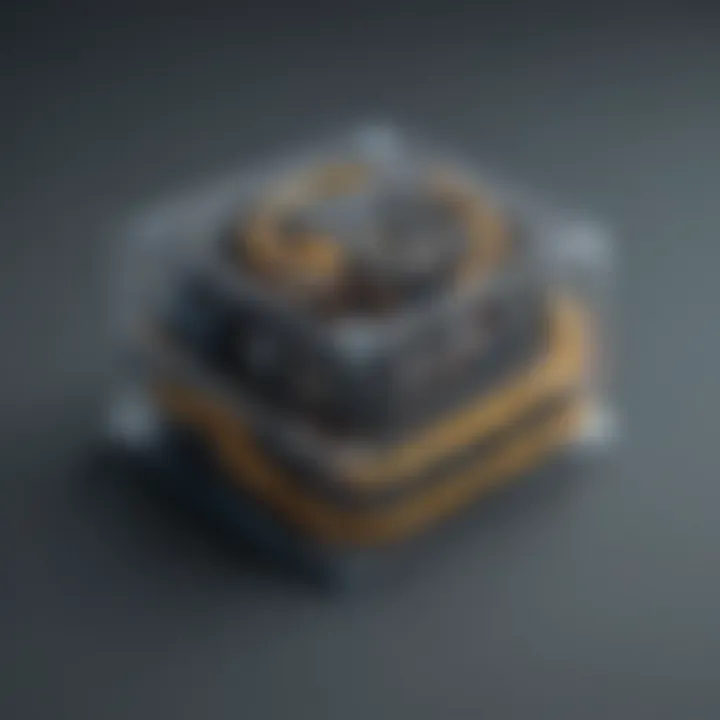
Key characteristics of Python modules
Python modules possess distinctive characteristics that set them apart as fundamental building blocks in Python programming. One key characteristic is modularity, which allows programmers to break down complex systems into manageable components. This modularity fosters code reusability, easing maintenance and updates within a project. Another critical feature is encapsulation, which shields module contents from external interference, ensuring data security and integrity. Additionally, Python modules encourage abstraction by hiding implementation details and promoting a clear interface for interaction, enhancing code readability and maintainability.
Importance of Modular Programming
Benefits of modular programming
Embracing modular programming practices provides several benefits to programmers and software projects. Modular programming promotes code reuse, reducing redundancy and promoting efficiency in development. By breaking down a program into modular components, developers can focus on specific functionalities, enhancing code clarity and maintainability. The reusability of modules across projects accelerates development cycles and fosters a systematic approach to software design, facilitating collaboration and code sharing among team members.
How Python modules enhance code organization
Python modules play a pivotal role in enhancing code organization by allowing programmers to structure code into logical units. By breaking down code into modules, developers can segregate functionalities, making codebases more manageable and comprehensible. Organized code structures facilitated by Python modules enable developers to navigate through codebases effortlessly, locate specific functionalities, and implement changes efficiently. Moreover, modular code organization simplifies debugging processes and promotes code maintenance, underpinning the importance of Python modules in software development projects.
Creating and Importing Python Modules
In this section of the comprehensive guide on Understanding Python Modules, we delve into the crucial aspect of creating and importing Python modules. The creation and importing of modules play a pivotal role in Python programming by facilitating code organization, reusability, and scalability. When programmers develop applications, creating modular code that can be easily imported and utilized across various parts of the program is essential for maintaining a structured and efficient codebase.
Defining a Python Module
When exploring the syntax for creating a Python module, programmers encounter a fundamental building block of Python programming. The syntax for creating a module involves encapsulating Python code within a .py file, where functions, classes, and variables can be defined. This methodology promotes code modularity and encapsulation, allowing programmers to break down their code into manageable components that serve specific functionalities.
Best practices for module naming
Another critical aspect to consider when defining Python modules is adhering to best practices for module naming. By following established conventions for naming modules, programmers streamline collaboration and code maintenance. Clear and descriptive module names enhance code readability, making it easier for developers to understand the purpose and functionality of each module within a project.
Importing Modules in Python
In the context of Python programming, importing modules is a fundamental mechanism for integrating external code into a project. The 'import' statement in Python enables developers to access functions, classes, and variables defined in separate modules, enhancing code reusability and minimizing redundancy. Effective import practices ensure that developers can leverage pre-existing code libraries and functionalities to expedite the development process.
Using the 'import' statement
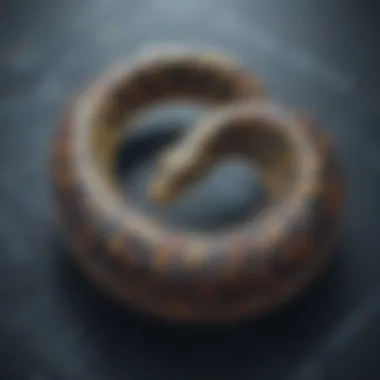
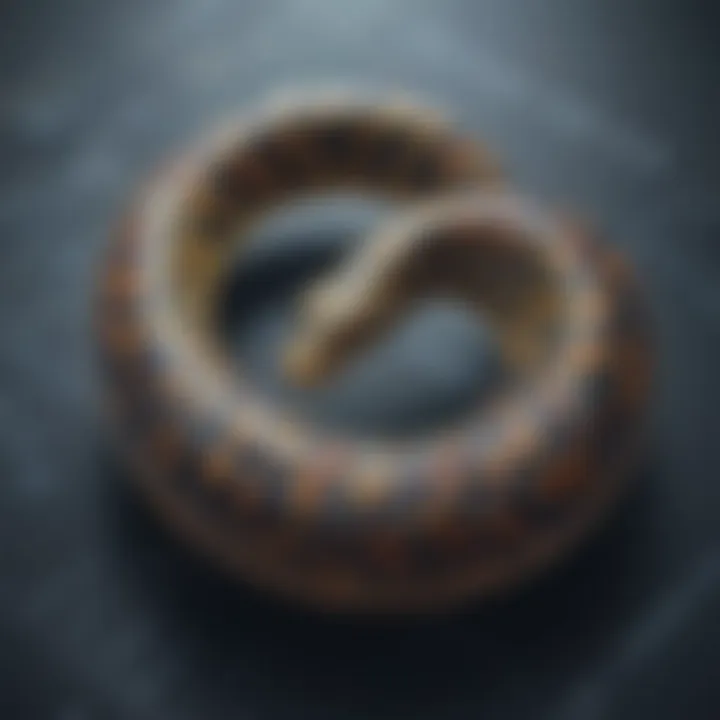
The utilization of the 'import' statement empowers programmers to bring external modules into their Python scripts effortlessly. By importing entire modules, developers can access all the resources contained within them, providing a convenient way to incorporate external functionalities into their projects. This practice promotes code efficiency and encourages the reuse of existing code components across multiple projects.
Importing specific functionsclasses from a module
In Python programming, importing specific functions or classes from a module offers a more targeted approach to utilizing external code. By selectively importing only the necessary components from a module, developers can optimize resource utilization and minimize potential namespace conflicts. This granular import strategy enhances code clarity and reduces the risk of unintended interactions between different parts of a program.
Exploring Module Functionality:
Exploring Module Functionality plays a crucial role in this comprehensive guide as it delves into the intricate details of Python modules. By understanding this aspect, programmers can enhance their code organization and improve reusability. Exploring Module Functionality sheds light on the inner workings of modules, providing insights into accessing attributes and utilizing packages and submodules effectively. This section covers key elements such as module introspection through the 'dir()' function and the importance of nesting modules within packages for streamlined code management. By exploring module functionality, programmers can maximize the potential of Python modules and optimize their coding projects.
Accessing Module Attributes:
The 'dir()' function for module introspection:
The 'dir()' function for module introspection is a fundamental aspect of exploring module functionality. This function allows programmers to introspect a module, gaining valuable information about its attributes and structure. The 'dir()' function assists in understanding the contents of a module, facilitating efficient code navigation and development. Its key characteristic lies in providing a comprehensive list of attributes within a module, aiding programmers in utilizing the module effectively. The unique feature of the 'dir()' function is its ability to reveal hidden attributes and methods, enabling programmers to access all relevant information within a module. While beneficial for exploring module functionality, the 'dir()' function may require careful interpretation to avoid overwhelming details.
Retrieving information about a module:
Retrieving information about a module is essential for exploring module functionality comprehensively. This aspect allows programmers to gather pertinent details about a module, including its properties, functions, and classes. By retrieving information about a module, programmers can gain a deeper understanding of its structure and functionality, enabling efficient utilization in their coding projects. The key characteristic of retrieving information lies in providing a detailed overview of the module's components, empowering programmers to make informed decisions during code implementation. The unique feature of this process is its ability to offer insights into a module's specifications, aiding in seamless integration within a larger codebase. While advantageous for exploring module functionality, retrieving information may require meticulous attention to detail to extract relevant data effectively.
Module Packages and Submodules:
Nesting modules within packages:
Nesting modules within packages is a vital component of exploring module functionality in this guide. This practice involves organizing related modules into a hierarchical structure, enhancing code manageability and scalability. Nesting modules within packages simplifies code navigation and promotes modular design, making it a popular choice for structuring complex projects. The key characteristic of nesting modules within packages is its ability to group related functionality together, promoting code reusability and clarity. The unique feature of this practice is its facilitation of modular architecture within Python projects, allowing for flexible and efficient code structuring. While advantageous for exploring module functionality, nesting modules within packages may require careful planning to maintain a well-structured and coherent codebase.
Creating submodules for enhanced code organization:
Creating submodules for enhanced code organization contributes significantly to exploring module functionality. This approach involves breaking down a module into smaller, specialized modules, enhancing code organization and promoting modular design principles. Creating submodules enables programmers to compartmentalize functionality based on specific features or tasks, streamlining code management and improving readability. The key characteristic of creating submodules is its capacity to isolate distinct functionalities within a module, facilitating code reuse and maintenance. The unique feature of this approach is its support for modular programming paradigms, allowing for the independent development and testing of module components. While beneficial for exploring module functionality, creating submodules may require careful consideration of module dependencies and interactions to ensure seamless integration within a codebase.
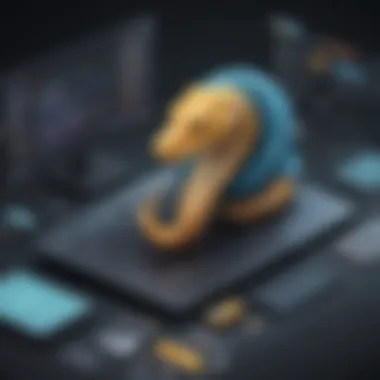
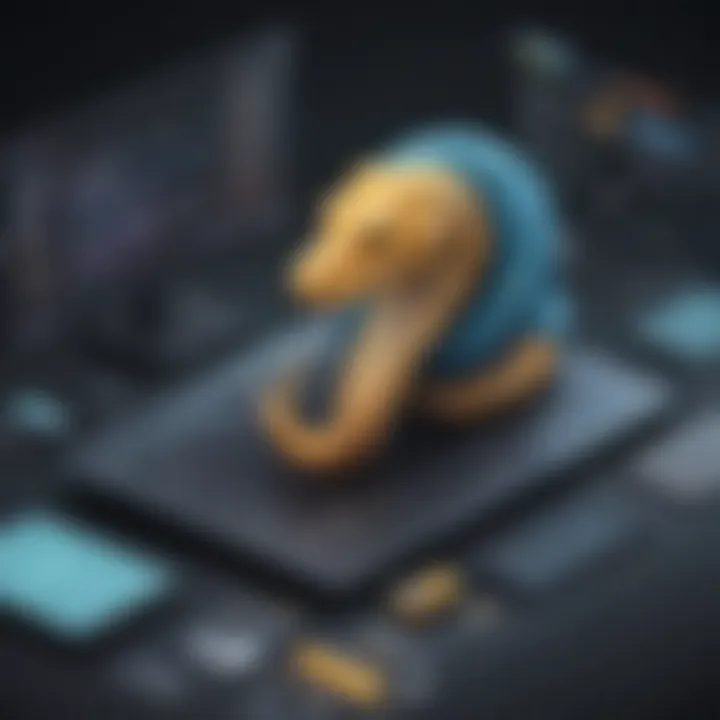
Advanced Module Techniques
In this article, we delve into the critical realm of Advanced Module Techniques, a pivotal component in understanding the intricate workings of Python modules. These techniques go beyond the basics, offering programmers a deeper insight into optimizing code functionality, increasing efficiency, and enhancing overall project management. By mastering Advanced Module Techniques, individuals can streamline their coding practices, improve code maintainability, and facilitate seamless collaboration among team members. The importance of mastering these techniques cannot be overstated, as they play a crucial role in elevating one's Python programming skills to a more sophisticated level, allowing for enhanced code modularity and reusability.
Module Aliasing and Reloading
Assigning aliases to modules
Discussing the concept of assigning aliases to modules is paramount in harnessing the power of Python modules effectively. By assigning aliases, programmers can create shorthand references to modules, simplifying code readability and promoting a more efficient coding environment. The key characteristic of assigning aliases lies in its ability to shorten lengthy module names without compromising clarity or functionality. This efficient strategy is widely favored in this article for its role in improving code aesthetics, reducing typing efforts, and increasing code conciseness. Despite its simplicity, the unique feature of assigning aliases significantly contributes to enhancing code organization and facilitating quicker reference to essential modules within a project, showcasing clear advantages in terms of code maintenance and readability.
Reloading modules for code updates
Exploring the practice of reloading modules for code updates provides programmers with a powerful tool for implementing real-time changes to their codebase. The key characteristic of reloading modules lies in its capability to update code dynamically during script execution, enabling developers to test new code iterations seamlessly without restarting the entire program. This approach proves to be beneficial in this article for its contribution towards facilitating agile development practices, enhancing debugging processes, and promoting rapid code refinements. The unique feature of reloading modules offers programmers the flexibility to adapt code changes swiftly, providing valuable advantages in scenarios where constant code modifications are necessary, ultimately fostering a more efficient and productive programming environment.
Optimizing Module Performance
Optimizing module performance is a critical aspect in the realm of Python programming. By focusing on enhancing the efficiency and speed of modules, programmers can significantly improve the execution and functionality of their codebase. In this section, we will delve into key strategies and techniques that aim to optimize module performance, thereby elevating the overall quality of Python programs.
Module Caching and Compilation
Understanding module caching mechanisms
When discussing module performance optimization, understanding module caching mechanisms plays a pivotal role. Module caching involves the storage of imported modules in memory to expedite the loading process. By caching modules, Python can recall them swiftly when required, reducing the time taken to access and execute code segments. This approach significantly enhances the operational speed of modules and is a popular choice among developers looking to boost the efficiency of their Python projects.
Compiling modules for improved performance
Compiling modules is another crucial aspect of optimizing module performance. By compiling modules, Python translates source code into bytecode, facilitating faster execution and improving overall performance. The compiled bytecode can be directly executed by the Python interpreter, eliminating the need for repetitive translation during code execution. This compilation process results in accelerated runtime speeds and enhanced efficiency, making it a favorable option for developers seeking to maximize the performance of their Python applications.
Avoiding Common Module Pitfalls
Issues to watch out for when working with Python modules
While working with Python modules, it is essential to be aware of common pitfalls that may impede the smooth functioning of your code. Issues such as namespace conflicts, circular dependencies, or outdated modules can pose challenges during development. By identifying and addressing these issues proactively, programmers can streamline their workflow and maintain the integrity of their projects. Being vigilant and understanding potential pitfalls ensures a seamless coding experience and fosters code stability.
Strategies for troubleshooting module-related errors
In the event of module-related errors, having effective strategies for troubleshooting is paramount. By utilizing debugging tools, logging mechanisms, and systematic error analysis, developers can efficiently identify and resolve issues within their modules. Implementing thorough testing procedures and error-handling protocols can minimize downtime and optimize code performance. These strategies aid in fortifying the reliability and functionality of Python modules, empowering programmers to deliver robust and error-free applications.