Comprehensive Guide to C Programming: From Basics to Mastery
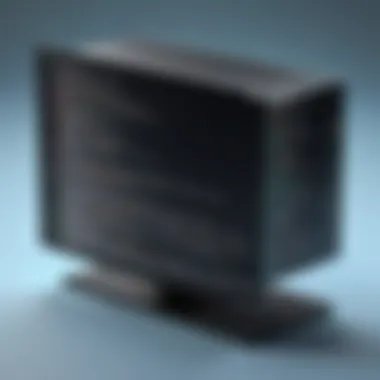
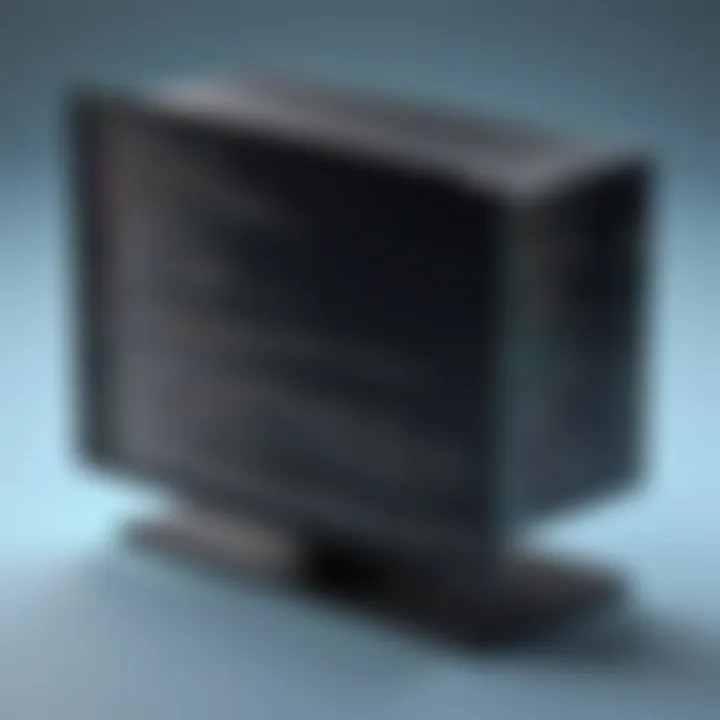
Intro
C programming is a foundational skill in the computer science field. It has a profound influence on many languages that arose after it, such as C++, Java, and Python. This comprehensive guide serves as a framework that bridges the gap between basic syntax to advanced programming techniques, catering to both beginners and seasoned professionals.
Understanding C programming is essential for problem-solving and developing algorithms that are efficient. Furthermore, it allows programmers to understand memory management and optimization strategies that are critical in high-performance applications. Throughout this guide, readers will explore practical applications and theoretical concepts that are vital for coding success.
By delving into essential elements like data types, control structures, and functions, one can build a robust knowledge base. Moreover, the intricate aspects such as pointers, memory allocation, and file handling will be examined thoroughly. Each segment of this guide emphasizes practical implementation, enabling readers to apply what they've learned more effectively.
Arming both novices and experienced programmers with the tools and insights necessary for effective coding practices is the goal of this narrative. Whether you're coding for fun, academic purposes, or professional projects, this guide gives a solid stepping stone to mastery in C programming.
Preamble to Programming
The field of programming is vast and continually evolving. C programming represents a foundational pillar within this domain. It not only serves as a starting point for many aspiring developers but also underpins various programming languages that followed it. The significance of understanding C goes beyond mere syntax; it instills a fundamental grasp of programming logic, memory management, and system-level interactions. By delving into C programming, one can uncover how software communicates with hardware, making it invaluable for disciplines ranging from system design to embedded system development.
History and Evolution of
The C programming language has a storied history that spans several decades. Developed in the early 1970s by Dennis Ritchie at Bell Labs, it was originally created to enhance the UNIX operating system. C represented a new breed of programming languages—it combined the low-level capabilities of assembly language with high-level language features, allowing for greater efficiency in coding. By adopting a structured approach, it simplified complex programming tasks while enabling systems programmers to control hardware more effectively.
Over the years, C evolved significantly. The first standardized version, ANSI C, emerged in 1989. This standardization process addressed portability concerns and solidified C as a versatile tool for software development. It provided a framework that programmers could rely upon across various platforms.
C has influenced many other languages such as C++, Java, and Python. Its simple yet powerful constructs continue to resonate within the programming community. The language has undergone updates, with C99 and C11 introducing new features, but the core principles remain unchanged. The historical progression of C underscores its role as a fundamental language that all programmers should explore.
Significance of in Modern Computing
In contemporary computing, C retains its relevance despite the emergence of languages like Python or Java. Its significance can be seen in various facets of technology. Firstly, C is often the language of choice for system programming. Operating systems, embedded systems, and device drivers commonly utilize C due to its direct memory access capabilities. Many high-performance applications, particularly those that require real-time processing, benefit immensely from the efficiency C offers.
Secondly, the conceptual frameworks learned from C programming are applicable to many modern programming languages. Understanding pointers, memory allocation, and the stack versus heap concept aids programmers in mastering other languages quickly. C encourages clarity and precision in code, promoting good programming habits.
Finally, the widespread use of C in education reflects its importance. Computer science programs around the world introduce C as a primary language, enabling students to understand the underlying principles of computing.
"C remains fundamental in establishing the base upon which higher-level languages build. Its knowledge equips both beginners and professionals with a toolkit for navigating the complex landscape of modern programming."
Basic Syntax and Structure
Understanding the basic syntax and structure of a programming language is crucial for effective coding. In C, these elements form the foundation upon which more complex constructs are built. Correct syntax ensures that the code is not only valid but also functional. Without a grasp of these fundamentals, a programmer may struggle to troubleshoot errors or optimize their code, leading to frustration and inefficiency.
Understanding the Program Components
C programs consist of various components that work together to perform a task. Each component serves a specific purpose and follows predefined rules.
- Preprocessor Directives: These are commands that are processed before compilation. They include directives like for including library files and for defining macros. They set the stage for the compilation process.
- Functions: Functions are the building blocks of C programs. The main function, , is the starting point of execution. Other functions can be defined to encapsulate specific tasks, promoting modularity and code reuse.
- Variables: Variables store data that can change during program execution. Each variable must be declared before use, specifying its type (e.g., , , ). This declaration informs the compiler of how much memory to allocate.
- Statements: C statements execute actions. They can include declarations, assignments, and control structures. Each statement generally ends with a semicolon, marking the conclusion of that instruction.
- Comments: Comments are ignored by the compiler but provide valuable documentation within the code. They can help explain logic or outline the function of certain code segments, making it easier for others to understand.
Understanding how these components interact is key to writing effective C code. Each part has its own rules and syntax that must be followed, and recognizing how they integrate into a complete C program is essential for both new and experienced programmers.
Common Syntax Rules
Adhering to common syntax rules in C is paramount for writing correct and maintainable code. Here are some basic rules to keep in mind:
- Case Sensitivity: C is case sensitive. For example, and refer to two different identifiers.
- Semicolon Termination: All statements must end with a semicolon. Omitting this can result in compilation errors.
- Brackets Usage: C uses curly braces to define the beginning and end of functions, loops, and conditional blocks. It is crucial that each opening brace has a corresponding closing brace.
- Data Type Declaration: Variables must be declared before use, and their type should match the kind of data they will hold. This prevents type-related errors during runtime.
- Function Calls: Functions must be called within the scope where they are defined. Proper usage of arguments in function calls is also necessary to ensure that the code works as intended.
In summary, familiarizing oneself with these syntax rules is integral to mastering C programming. Incorrect syntax can lead to unexpected behaviors or, even worse, cause the program to fail to compile, underscoring its importance in the learning process.
"Proper syntax enables programmers to translate their thoughts into functional code with precision and ease."
By learning and applying these rules, aspiring and experienced programmers can enhance their coding skills and develop a deeper understanding of how C operates.
Data Types and Variables
Understanding data types and variables is critical in C programming. Data types define the kind of data that can be stored and manipulated within a program. Variables, on the other hand, are used to represent data in memory. The carefull choice of appropriate data types leads to more efficient programming. They affect memory usage, performance, and correctness of your program. Here are some key elements to consider:
- Memory Efficiency: Choosing the right data type can save memory. For instance, using a instead of an when only a character is needed uses less space.
- Type Safety: This ensures that the operations performed on data are valid. For example, trying to perform arithmetic operations on strings can lead to errors.
- Performance: Data types can affect how quickly a program runs. Using the appropriate types is crucial for optimizing performance.
Overall, understanding data types and variables paves the way for sound programming methodology.
Primitive Data Types
C supports several primitive data types essential for performing basic operations. These include:
- int: Represents integers, both positive and negative. Typically 4 bytes.
- char: Stores individual characters, usually 1 byte.
- float: Used for single-precision floating-point numbers, generally 4 bytes.
- double: Represents double-precision floating-point numbers, usually 8 bytes.
Each of these types holds specific characteristics, leading to distinct scenarios in programming. For example, using for counting iterations in a loop is effective, while is ideal for scientific calculations where decimals are involved.
Type Modifiers
Type modifiers refine the properties of base data types in C. These modifiers provide greater flexibility and control over the size and range of data types. Key type modifiers include:
- signed: Allows the data type to hold both positive and negative values.
- unsigned: Prevents negative values, thus doubling the maximum positive value that can be stored.
- short: Reduces the size of , typically to 2 bytes, which is useful for conserving memory.
- long: Increases the size of data types, often resulting in 8 bytes for .
This specificity allows programmers to choose the best possible representation for their data, enhancing the efficiency of the application.
User-defined Data Types
User-defined data types in C enable programmers to create more complex data structures. This is vital for managing and organizing data effectively in larger projects. Common user-defined types include:
- Structures: Allow grouping of different data types under a single name, useful for representing records.
- Unions: Save memory by allowing different data types to occupy the same memory location.
- Enums: Provide a way to define variables that can be assigned a set of predefined constants, increasing code readability.
By employing user-defined data types, programmers can reflect real-world entities and enhance program clarity.
"Choosing the right data type is key to writing efficient C code."
Control Structures
Control structures are a fundamental aspect of C programming. They determine the flow of control in a program, enabling developers to dictate how and when certain blocks of code are executed. This section will focus on two primary categories: conditional statements and looping mechanisms. Each plays a crucial role in creating dynamic and responsive applications.
Conditional Statements
Conditional statements allow programmers to execute specific code segments based on certain conditions. This is essential in creating programs that respond differently under varying circumstances.
The most common forms include the , , and statements. Here’s a brief explanation of each:
- if statement: This evaluates a condition. If true, the code block corresponding to the is executed.
- else if statement: If the initial condition fails, this provides an alternative condition to evaluate.
- else statement: This executes when all previous conditions fail.
For example:
Using conditional statements efficiently is vital. They help to break complex logic into manageable components, facilitating easier debugging and enhancement of program functionality. However, one must consider how deeply nested conditional statements can hurt readability. Therefore, it is often wise to keep them as straightforward as possible.
Looping Mechanisms
Looping mechanisms are critical for executing a block of code multiple times until a specified condition is met. C offers several types of loops, namely the , , and loops. Each serves its purpose and can be chosen based on specific needs.
- for loop: This loop is typically used when the number of iterations is known beforehand. It consists of three parts: initialization, condition, and increment/decrement.Example:
- while loop: This loop is used when the number of iterations is unknown. It continues to execute as long as a specified condition remains true.Example:
- do while loop: Similar to the loop, but it guarantees that the code block will execute at least once, as the condition is checked after the execution.Example:
Choosing the correct looping mechanism can affect performance and clarity. For instance, using a loop for a fixed number of iterations can be more intuitive than a loop, which can lead to confusion if not handled properly. In summary, understanding control structures not only aids in writing effective C programs but also enhances overall programming skills.
Functions in
Functions are essential components of the C programming language. They allow for modular programming, where code can be organized into blocks that perform specific tasks. This organization improves code readability and reusability, making it easier for programmers to manage complex projects. By using functions, developers can define a sequence of statements that can be executed multiple times, reducing code duplication and enhancing maintainability.
Functions also facilitate easier debugging. Errors in specific functions can be isolated without affecting the entire program. This sense of abstraction provides clarity for both new and seasoned programmers.
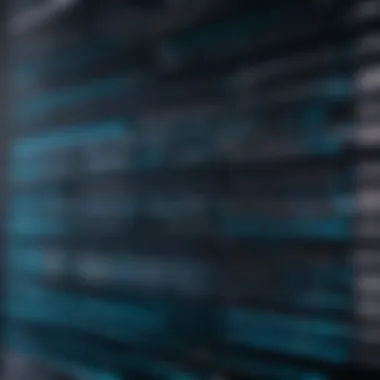
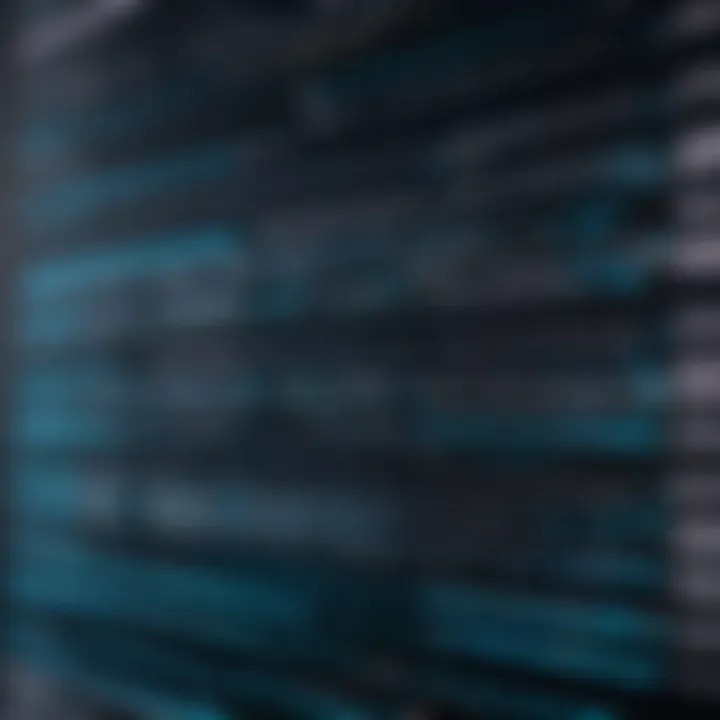
Defining and Calling Functions
In C, defining a function involves outlining its return type, name, and parameters. The structure is simple:
For example:
Once a function is defined, calling it is straightforward. You simply use the function name, followed by parentheses, passing any required arguments:
This will call the function with arguments 5 and 3 and store the result in .
Function Pointers
Function pointers are another critical aspect of C. They allow you to store the address of a function, enabling dynamic function calling. A function pointer can be declared as follows:
For instance, if we have a function , we can create a pointer to it:
The real power of function pointers lies in their flexibility. They can be used for callbacks, allowing for code designed to be executed in the context of another function. This means that functions can be passed as parameters to other functions, leading to more generic and reusable code patterns.
Moreover, they are fundamental in implementing data structures like linked lists or trees, where you need the capability to call different functions based on dynamic conditions. Overall, function pointers enhance the capability and flexibility of C programming.
Pointers and Memory Management
Memory management is one of the key responsibilities of a programmer. In C programming, the concept of pointers plays a critical role in how we handle memory. Understanding pointers alongside memory management gives programmers the ability to optimize performance and efficiently utilize resources. This section emphasizes the relevance and intricacies of pointers and dynamic memory allocation, marking them as essential skills for both novice and experienced developers.
Understanding Pointers
Pointers are variables that store memory addresses. This feature is fundamental in C programming. They allow developers to directly reference memory locations, making it possible to manipulate data structures like arrays and linked lists efficiently. The ability to use pointers can lead to more optimized code and can reduce memory usage via indirect data access.
Here are some key points about pointers:
- Memory Efficiency: By manipulating memory directly, pointers can significantly enhance the performance of a program.
- Dynamic Data Structures: Pointers enable complex data structures such as trees and graphs that can grow and shrink in size during runtime.
- Function Arguments: They allow functions to modify variables passed by reference, avoiding unnecessary copies in memory.
When declaring a pointer, it is important to use the correct types. For example:
In this example, is a pointer to an integer. Mismanagement of pointers can lead to undefined behavior, crashes, or memory leaks. Therefore, careful handling is crucial.
Dynamic Memory Allocation
Dynamic memory allocation gives the programmer control over memory management at runtime. Unlike static memory allocation, which reserves memory at compile time, dynamic memory allocation allows for creating variables in memory during the program's execution.
C provides several functions for dynamic memory allocation, most notably:
- : Allocates a specified number of bytes and returns a pointer to the first byte.
- : Similar to but initializes the allocated memory to zero.
- : Resizes previously allocated memory while preserving its content.
- : Deallocates memory that was previously allocated.
Here’s an example of dynamic memory allocation using :
It is essential to check if the allocation was successful. If fails, it returns , and attempting to use such a pointer will result in runtime errors.
Benefits of Dynamic Memory Allocation
- Flexibility: Programmers can allocate memory as needed, which is particularly useful for large data sets.
- Performance: It can lead to more efficient memory usage, as allocation occurs only when necessary.
- Control: The programmer has full control over memory allocation and can optimize programs as needed.
Considerations
When using pointers and managing memory dynamically, several considerations must be kept in mind:
- Memory Leaks: Failing to deallocate memory with will lead to memory leaks.
- Dangling Pointers: These occur when memory has been freed, but a pointer still points to that location, potentially causing unpredictable behavior.
- Segmentation Faults: Improper use of pointers can lead to read or write access violations in memory, causing the program to crash.
Using pointers and understanding dynamic memory allocation are vital parts of effective C programming. By mastering these concepts, programmers can build efficient, scalable applications.
Arrays and Strings
Arrays and strings are foundational concepts in C programming that impact both performance and functionality. Understanding how to utilize arrays effectively is crucial for efficient data management and manipulation. Arrays are a collection of variables of the same type, which can be accessed using an index. This structured way of storing data improves both access speed and memory usage. On the other hand, strings, while often treated as just a sequence of characters, represent a more complex level of data handling that includes memory allocation and textual manipulation. The relevance of mastering these concepts cannot be understated, especially in data processing and algorithm development scenarios.
Declaring and Initializing Arrays
Declaring an array in C is straightforward. The syntax requires specifying the type of elements it holds and the array's name, followed by the size of the array in square brackets. For instance:
Initialization can occur simultaneously or afterwards. For example:
Alternatively, elements can later be assigned individually:
Arrays in C have some important characteristics. First, the size is fixed once declared, which means you cannot change its size dynamically during runtime. Second, arrays are efficient in storing large amounts of data because they store contiguous memory locations. One limitation to consider is that, unlike higher-level languages, C does not automatically check for array bounds, leading to potential segmentation faults if accessed beyond allocated size. This can cause programs to behave unpredictably. Therefore, proper management and access to array elements are necessary to ensure stability in coding.
String Manipulation Functions
In C, strings are defined as an array of characters, terminated by a null character . This representation means that functions to manipulate strings rely on the common array operations. The C standard library provides a variety of functions for string operations, found primarily in the header file. Some essential functions include:
- : Calculates the length of a string without counting the null terminator.
- : Copies one string to another, maintaining the null terminator.
- : Compares two strings lexicographically.
- : Concatenates two strings, appending one to the other.
Here’s an example that demonstrates some string functions:
In the example above, the and functions are utilized to manipulate strings effectively. Understanding how these functions work is vital for string handling. However, it is essential to manage memory correctly when using strings in C to prevent overflows and ensure safe string operations.
Important: Remember, C does not provide a built-in way to automatically manage strings. Always ensure buffers are adequately sized to prevent memory issues.
Structures and Unions
Structures and unions are essential elements in C programming, providing a means to group related data types together. This topic gains significance as programmers often need to manage and manipulate complex data in a structured way. Using structures and unions facilitates effective data organization, enhances code readability, and allows for sophisticated data management strategies.
At the core of structures is the concept of grouping different data types into a single unit, where each member can be of a different type. This is particularly useful when you want to represent a real-world entity, such as a student with properties like name, ID, and grades.
Unions, by contrast, serve a different purpose. They also allow for the grouping of different data types, but they share the same memory space among their members, essentially allowing the storage of one value at a time. Understanding when to use structures versus unions can greatly improve memory efficiency and performance in programs.
Defining Structures
In C, a structure is defined using the keyword, followed by the structure name and the member variables within curly braces. Member variables can be of different types, including primitive types and other structures. Here’s an example:
In this example, the structure contains three members: , , and . With structures, you can create variables that store data about individual students, making it easier to manage complex datasets. To create a structure variable, you simply declare it as below:
The structures can also be initialized at the time of declaration. For example:
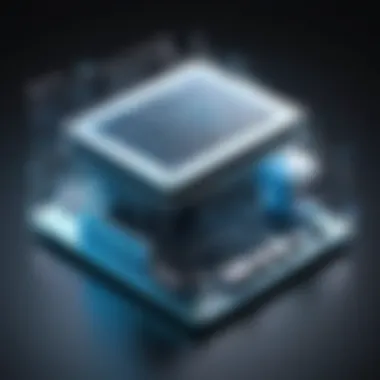
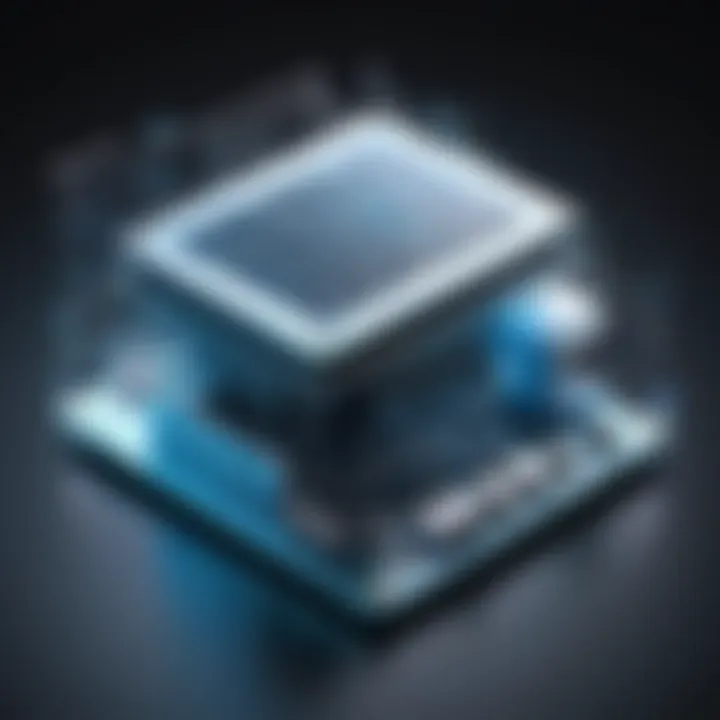
This initialization allows direct assignment of values to the members of the structure. Moreover, accessing members of a structure is straightforward using the dot operator. For instance, will reference the grade of .
Using Unions
Unions are defined in a similar way to structures but use the keyword. The key difference lies in memory management. While multiple members can be declared in a union, they share the same memory location. This means a union can only store one member value at a time, which can lead to memory savings in certain situations.
An example of defining a union is shown below:
In this case, the union can hold an integer, a float, or a character, but only one of these types at any given time. When a union is large (with many members), it allows for more efficient memory usage. Accessing a union's member is also done using the dot operator, similar to structures. Here’s how you can use it:
When you assign a new value to , the previously stored is overwritten. Thus, careful management is necessary while using unions.
Remember: Unions are helpful when only one field needs to be accessed at a time, providing both flexibility and memory efficiency.
In summary, both structures and unions are crucial for organizing data in C programming. They facilitate the development of complex programs by allowing related data to be handled together, thus improving program readability and efficiency.
File Input and Output
The topic of File Input and Output (I/O) is essential in C programming as it facilitates interaction between programs and external data. By understanding file I/O, programmers can read data from files, manipulate it, and save changes back to disk. This capability is critical for applications that require data persistence, such as database management systems or simple file processors. Mastery of file I/O enhances the versatility of C programs, making them more applicable in real-world scenarios.
Reading from Files
Reading from files involves opening the file, reading its contents, and then closing it. C provides several library functions to accomplish file reading. The process usually requires a few key steps:
- Opening the file: Use to open a file in read mode. If the file does not exist or cannot be opened, the function returns a NULL pointer.
- Reading the data: Functions like , , or can be utilized to extract data from the file.
- Closing the file: It is good practice to close the file using to free system resources.
Here’s a simple example that demonstrates reading from a text file:
This code opens a file named , reads each character until reaching the end of the file (EOF), and then outputs it to the console.
Writing to Files
Writing to files is equally important and follows a similar process. The steps include opening the file in write mode, writing data, and subsequently closing the file. Here’s how it generally works:
- Opening the file: Use to open a file in write mode. If the file does not exist, it will be created. Note that opening in write mode will overwrite any existing content in the file.
- Writing data: Functions like , , or can be utilized to write data into the file.
- Closing the file: Always close the file with to ensure all data is properly written and resources are released.
Below is an example of how to write to a file:
In this code snippet, a new file called is created, and a line of text is written into it.
Understanding file I/O is crucial for programming. It allows for the storage and retrieval of data, enabling the persistence that is often required in software applications.
"File I/O is like the bridge that connects a program with its environment, making data management feasible."
By mastering these techniques, programmers can enhance their applications, ensuring they can handle user data effectively.
Preprocessor Directives
Preprocessor directives play a crucial role in the C programming language. They are commands that the compiler processes before the actual compilation of the code begins. This phase is important because it allows for conditional compilation, macros, and file inclusion, which are essential for creating efficient and manageable code. The proper usage of preprocessor directives can lead to better organization and optimization of programs.
One of the key benefits of preprocessor directives is that they help manage and reduce code complexity. By enabling conditional compilation, developers can include or exclude portions of code depending on specific conditions. This functionality is particularly useful when working on cross-platform projects, allowing the same codebase to adapt to different environments without significant alterations.
In addition, preprocessor directives allow for macro development that simplifies repetitive tasks within the code. These macros can act as shortcuts for defining constants, functions, or any expressions that are used multiple times. Thus, preprocessor directives not only enhance readability but also promote code reusability.
Defining Macros
Macros are a powerful feature facilitated by preprocessor directives. They enable the definition of code snippets or constants that can be reused throughout the program. Macros are defined using the directive, which specifies a name for the macro and its value or expression.
For example:
This line creates a macro called that can be used in place of its defined value. Whenever the compiler sees , it replaces it with . This is beneficial for maintaining consistency, especially in large projects, as it eliminates hard-coded values within the code. If there's a need to change the value of a constant, only the macro needs retracing.
It's important to use macros carefully. Overusing them can lead to debugging challenges, as errors related to macros can be harder to trace than standard code. Moreover, macros do not obey scope rules; they exist throughout the file after their definition. This can lead to unexpected results if not managed well.
Conditional Compilation
Conditional compilation is another vital aspect of preprocessor directives. By utilizing , , , , and , programmers can include or exclude code segments depending on defined conditions. This capability is particularly helpful in configuring code that may need to behave differently in various settings or platforms.
For instance:
In this example, if the macro is defined, the line will execute. If not, it will be ignored during compilation. This approach can streamline output during testing phases, allowing developers to toggle debugging information without altering the core logic of the program.
Conditional compilation can significantly optimize program performance and simplify execution paths.
Overall, the correct use of preprocessor directives can enhance coding efficiency, foster maintainability, and improve the overall quality of C programming. By understanding and implementing these directives effectively, programmers can cultivate a robust coding practice that is adaptable to various programming environments.
Debugging and Error Handling
Debugging and error handling represent vital components in the lifecycle of C programming. As programmers, the complexity of the code can introduce various challenges, including logic errors and runtime errors. The ability to efficiently debug one’s code not only enhances the overall quality but also streamlines development processes. This section delves into common errors encountered in C and effective techniques for debugging them.
Common Errors in
Errors in C programming typically fall into several categories. Recognizing these categories is the first step toward efficient debugging. Some of the most frequent errors include:
- Syntax Errors: These occur when the code violates the grammar rules of C. Missing semicolons or improperly matched brackets are common culprits.
- Logical Errors: Unlike syntax errors, these do not prevent the code from running, but they result in incorrect behavior. Such errors can be tricky to identify since the program compiles and runs without crashing.
- Runtime Errors: These arise during program execution. Issues such as division by zero or accessing an array out of bounds can lead to serious problems that abrupt program flow.
- Compilation Errors: These errors happen when the compiler fails to translate the code into machine language due to syntactical mistakes or type mismatches.
"Understanding the types of errors is crucial for effective debugging."
Understanding these errors allows for a more focused debugging strategy. A good practice is to implement rigorous testing, especially for programs with complex logic.
Debugging Techniques
Debugging requires a thoughtful approach. Here are several techniques that can enhance your debugging efforts:
- Print Statements: Inserting print statements throughout your code can help track the flow of execution and variable states.
- Using a Debugger: Tools such as GDB (GNU Debugger) provide advanced features that allow stepping through the code, inspecting variables, and observing how the program behaves in real-time.
- Unit Testing: Writing tests for individual components of code helps ensure that each part behaves as expected. This method can prevent errors from spreading throughout the codebase.
- Code Reviews: Engaging in peer review processes of code can uncover problems early. Another set of eyes may spot issues that the original programmer might miss.
- Static Code Analysis: Tools that analyze code without executing it can help detect potential issues early in the development cycle.
By employing these techniques, programmers can significantly reduce the time spent debugging while increasing their understanding of the code's behavior. This ultimately results in higher-quality software and a more streamlined development process.
Standard Libraries
C Standard Libraries play a crucial role in C programming by offering a set of pre-written functions that assist programmers in performing common tasks. These libraries enable code reuse, streamline development, and promote effective management of system resources. They are essential for both novices and experienced developers as they provide standardized methods to access common operations like input/output handling, string manipulation, and mathematical computations.
Using the C Standard Libraries promotes efficiency and reduces the likelihood of errors. Rather than writing code from scratch for functionalities like file handling or string operations, programmers can utilize these libraries, ensure optimal performance and reliability, and benefit from community-tested functions. Additionally, they help maintain code portability across various systems, making it easier to share and maintain software.
The C Standard Libraries are a foundation that allows developers to focus on problem-solving rather than reinventing the wheel.
Preface to Standard Libraries
Standard libraries in C can be divided into several categories, including input/output libraries, string handling libraries, mathematical libraries, and more. Each library addresses specific domains of programming, providing essential functions that cater to varied needs.
Some common libraries include:
- stdio.h: Supports input and output functions such as printf() and scanf().
- stdlib.h: Provides functions for memory allocation, process control, and conversions.
- string.h: Offers functions for manipulating C strings, including concatenation and comparison.
- math.h: Contains mathematical functions like sin(), cos(), and sqrt().
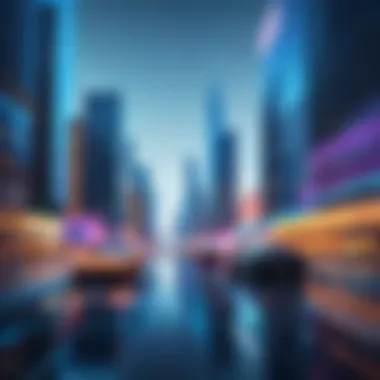
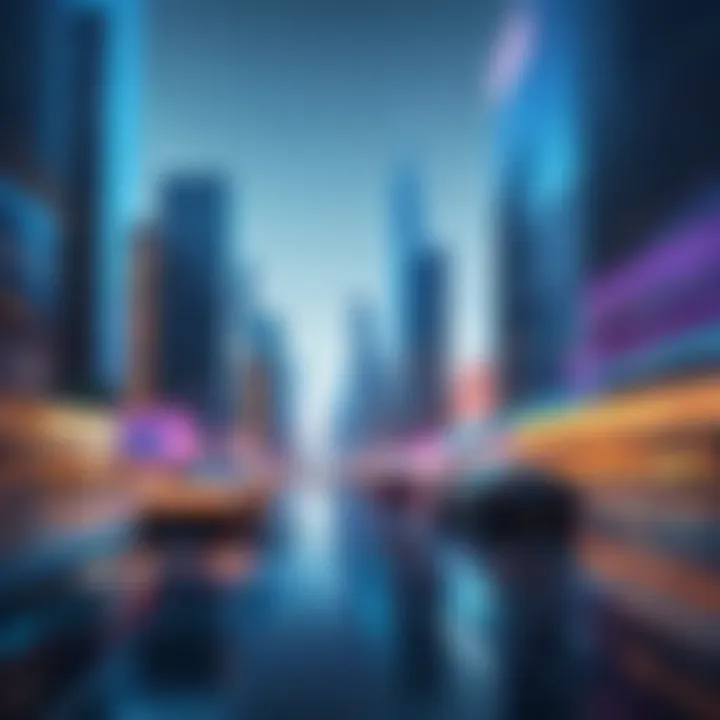
By utilizing these libraries, programmers can access a well-documented interface for performing operations efficiently, ensuring both ease of use and reliability.
Additionally, when incorporating libraries into a program, clarity is maintained in the code structure as the focus shifts from implementing basic functions to leveraging standard ones. This highlights the effectiveness of library functions, making the code clearer and more concise.
Frequently Used Libraries
Several standard libraries are used extensively in C programming. Understanding these libraries is important for any programmer aiming to master C.
- stdio.hThe header file stdio.h> is fundamental. It includes functions for basic input and output. Functions such as printf() for printing to the console and scanf() for reading user input are essential tools for any C program.
- stdlib.hThis library provides utilities for memory allocation and conversion functions. Functions like malloc() and free() are essential for dynamic memory management, while atoi() and atof() facilitate conversion between strings and numeric types.
- string.hString operations are simplified with string.h>. This library provides functions to handle C-style strings, including functions for copying, concatenation, and comparison. Each function allows for efficient manipulation of character arrays, a common data type in C programming.
- math.hFor mathematical operations, math.h> is indispensable. It includes functions for performing complex calculations, including trigonometric functions and logarithms, thus enhancing the mathematical capabilities of your programs.
Using these libraries not only speeds up development but also helps to enforce coding standards within teams. Many organizations prefer to rely on standard libraries for their reliability, performance, and community support.
Advanced Programming Techniques
Advanced C programming techniques are essential for programmers looking to optimize performance, utilize system resources efficiently, and develop complex applications. Understanding these concepts allows engineers to write code that is not only functional but also scalable and robust. In this section, we will focus on two pivotal elements: multithreading and inter-process communication (IPC). Each plays a significant role in modern software development, particularly in environments requiring concurrent processing and communication between different processes.
Multithreading in
Multithreading refers to the ability of a program to execute multiple threads concurrently. Each thread runs independently but shares the same resources, making multithreading a highly efficient strategy for maximizing CPU utilization. In C, multithreading can be implemented using the POSIX Threads (pthread) library, which provides a uniform interface for creating and managing threads.
The benefits of multithreading include:
- Improved Performance: Tasks that can be executed in parallel enhance the overall efficiency of applications. This can lead to faster execution times, especially for CPU-bound operations.
- Better Resource Management: Multithreading allows for better utilization of system resources. Threads can share memory and other resources that would otherwise require separate processes.
- Responsiveness: In user interface applications, multithreading can keep the interface responsive while performing background computations.
Considerations when working with multithreading include:
- Data Consistency: Proper synchronization mechanisms like mutex locks are essential to prevent data races and ensure that shared resources are accessed safely.
- Thread Overhead: Creating and managing threads has overhead. Therefore, it is important to assess if multithreading provides substantial benefits for the specific application.
Example code snippet for creating a simple thread:
Inter-process Communication
Inter-process communication enables the exchange of data between multiple independent processes. Since processes do not share memory space like threads, IPC mechanisms become necessary for their communication. C provides several IPC methods, such as pipes, message queues, and shared memory.
The significance of IPC includes:
- Modularity: It promotes application modularity, allowing different components of a software to communicate while running as isolated processes.
- Resource Allocation: It helps in optimizing resource allocation, especially in systems with multiple users or heavy tasks.
- Scalability: Applications can be designed to scale by adding more processes without major modifications to existing code.
Common IPC methods are as follows:
- Pipes: Allow data to flow in one direction between processes.
- Message Queues: Send and receive messages in a FIFO manner, providing a way for processes to communicate asynchronously.
- Shared Memory: A segment of memory shared among processes, making data access faster.
When implementing IPC, programmers must consider the following:
- Synchronization: Proper handling is required to prevent collisions or data inconsistencies between processes.
- Complexity: Managing IPC can introduce extra complexity to program design. Thus, careful planning is needed.
"Efficient handling of multithreading and inter-process communication techniques can lead to significant enhancements in software performance and scalability."
Language in Embedded Systems
The C programming language plays a pivotal role in the world of embedded systems. These systems include a broad range of devices from home appliances to complex machinery in industrial environments. The efficiency, speed, and low-level access provided by C make it a favorable choice for programming these systems. In this section, we will delve deeper into the significance and characteristics of C in this domain.
Embedded Systems Overview
Embedded systems are designed to perform specific tasks or functions within larger systems. Unlike general-purpose computers, they have restricted computing resources and often operate in real-time.
Key Characteristics of Embedded Systems:
- Dedicated Functionality: Each embedded system does one or a few specific tasks very well.
- Real-Time Operation: Timeliness is critical. Many applications require immediate responses.
- Resource Constraints: Limited processing power, memory, and storage are common.
- Integration with Hardware: Operating closely with hardware components is essential for efficiency.
C is directly tied to the hardware due to its close proximity to assembly languages. This allows for fine-tuned control over the hardware which is often necessary for embedded applications. By writing efficient and compact code, developers can fully utilize the available resources, leading to improved performance and accuracy in applications.
for Microcontrollers
Microcontrollers are small computing devices embedded in various products, ranging from toys to medical equipment. They often run on real-time operating systems, or no operating system at all, making C an ideal choice. Here are some benefits of using C for microcontroller programming:
- Efficiency: C is known for generating efficient machine code. This is crucial for devices where performance is key.
- Portability: Code written in C can be easily transported to other platforms with slight modifications. This flexibility is beneficial for various microcontroller architectures.
- Access to Low-Level System Functions: C enables programmers to manipulate hardware directly, which is essential for device control.
- Rich Libraries: A comprehensive set of libraries support interfacing with hardware, simplifying development.
Using C allows developers to create robust applications while ensuring the intended functionality of microcontrollers. Furthermore, as embedded systems are an integral part of Internet of Things technology, proficiency in C can greatly enhance a programmer's skill set in the current market.
"C provides the necessary tools to communicate and control hardware effectively, creating a bridge between software and physical systems."
In summary, C programming is critical for the development of embedded systems and microcontrollers. Its combination of efficiency and low-level hardware access equips developers to effectively address the unique challenges presented in this domain.
Comparison with Other Programming Languages
Understanding how C programming compares to other languages is crucial. It offers insights into its strengths and weaknesses. C is a foundational language in computing. Its principles inform the design of many modern programming languages. Thus, comparing C with other languages like C++ and Python is relevant for both budding developers and seasoned professionals.
vs. ++
C++ is often seen as an extension of C, but it introduces object-oriented programming (OOP) paradigms that C does not possess. Here are some key points of distinction:
- Object-Oriented Features: C++ supports classes and objects. This allows developers to organize code better and encapsulate functionality. C, being a procedural language, lacks these constructs.
- Memory Management: Both languages offer manual memory management, but C++ also introduces constructors and destructors. These aid in automatically managing resource allocation and deallocation, which is not the case in C.
- Standard Template Library (STL): C++ has the STL, which provides a wealth of data structures and algorithms. This enhances productivity and efficiency when programming. C relies mostly on built-in libraries.
- Performance: While C generally has a slight performance advantage due to its low-level capabilities, well-optimized C++ code can rival it closely. Compilers for both languages can yield efficient machine code.
In scenarios where high-level programming and object-oriented design are necessary, C++ can be more favorable. Conversely, in systems programming and when dealing with hardware, many prefer C due to its simplicity and control.
vs. Python
Python is significantly different from C in terms of design philosophy and application. The differences are as follows:
- Syntax and Readability: Python is known for its clear syntax. It emphasizes readability and simplicity, making it ideal for beginners. C has a more complex syntax, which can be less intuitive for novices.
- Typing System: C uses static typing, which requires variable type to be declared explicitly. Python employs dynamic typing, allowing for more flexibility but potentially introducing runtime errors that might be caught at compile time in C.
- Performance: C is compiled directly to machine code, which generally results in superior performance. Python is an interpreted language, leading to slower execution. This is critical in performance-sensitive applications.
- Use Cases: C is widely used for system-level programming, embedded systems, and applications requiring close interaction with hardware. Python excels in web development, data analysis, and scripting.
The choice between C and Python often depends on the project requirements. For maximum performance and hardware access, C remains unmatched. For rapid application development and ease of use, Python is usually preferred.
"Understanding the comparison of programming languages helps developers choose the right tool for the job, enhancing both productivity and efficiency."
Future of Programming Language
The C programming language is an enduring pillar in the world of computing, celebrated for its performance and efficiency. Its significance extends well beyond its age, shaping many modern programming languages and systems. As technology rapidly evolves, examining the future of C becomes essential. This section will discuss emerging trends and the role of C in academia and industry, providing insights that highlight why C remains relevant today.
Emerging Trends
As programming paradigms shift, C continues to adapt. Some emerging trends impacting C are:
- Integration with Modern Technologies: C is increasingly used in embedded systems with rising demands for IoT. Applications designed in C can provide the necessary low-level control while ensuring efficient resource management.
- Security and Safety: With the increasing focus on cybersecurity, C's performance in myriads of applications raises concerns about vulnerabilities in memory management. There is a movement towards safer coding practices including tools for static code analysis and memory safety.
- Compilers and Tooling Enhancements: Compilers are evolving, enhancing the optimization of C code. This allows developers to produce faster and more efficient software. Recent developments in tools such as LLVM are promising when it comes to generating optimized code.
- Concurrent and Parallel Programming: As hardware advances toward multi-core processors, the programming community is focusing on concurrent programming. This is not only an addition but also an evolution of existing C paradigms.
"The enduring strength of C lies in its ability to evolve while maintaining its core efficiency."
These trends indicate that although C is often considered a legacy language, it is anything but obsolete. The language is transitioning to meet the demands of modern technology while retaining its foundational virtues.
Language in Academia and Industry
C has a strong foothold in both academia and industry, and its usage is far from diminishing. In academia, C serves as a fundamental tool for teaching programming. It lays the foundation for understanding more complex languages and computer science principles.
In industry, C is prevalent in the following areas:
- Operating Systems: Many systems like Linux are built using C, showcasing its capability to manage hardware resources effectively.
- Embedded Systems: Devices ranging from simple appliances to complex machinery commonly use C. Its performance and limited resource requirements suit these applications well.
- Game Development: Performance-critical applications, such as game engines, often capitalize on C's efficiency.
- Financial Systems: High-frequency trading systems leverage C for its speed and deterministic behavior.
Overall, C paves the way for future innovations. Its relationships with emerging technology ensure its longevity in a fast-paced field, making it an invaluable asset for aspiring programmers and IT professionals.
Epilogue
The conclusion of this comprehensive guide is pivotal as it ties together all the threads explored throughout the article. The importance of understanding the concluding remarks cannot be overstated. A well-rounded conclusion encapsulates the core insights and lessons presented, while also providing direction for future exploration and learning. It is in this part of the text where both novices and seasoned programmers find value in reflecting on what they have learned.
Summary of Key Points
In summation, the journey through C programming revealed critical components and advanced concepts that are vital for effective programming practices. Important topics included the structure of C programs, data types, control mechanisms, functions, and pointers. Each of these elements is integral to writing efficient and effective code in C. By mastering the syntax and semantics, individuals can appreciate the power and versatility of this language. Moreover, it demonstrated how C holds an important place in both historical and contemporary contexts of computing.
Final Thoughts
To conclude, delving into C programming equips programmers with a strong foundation that extends beyond simple syntax. It fosters a deeper understanding of how computers work, and how software interacts with hardware. This not only enhances one's programming abilities but also sharpens analytical skills, which are crucial in solving complex problems.
As technology continues to evolve, the relevance of C remains evident in both academic and industrial spheres. Thus, embracing continuous learning and experimentation will further empower aspiring programmers to excel in their craft. Individuals should remain curious and open to exploring the vast resources available to them, such as forums on Reddit or articles on Wikipedia, to stay updated in this ever-changing field.