C# for Programmers: An In-Depth Exploration of Concepts
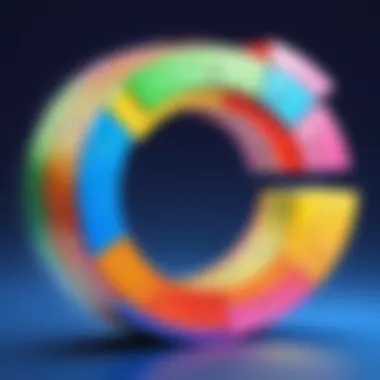
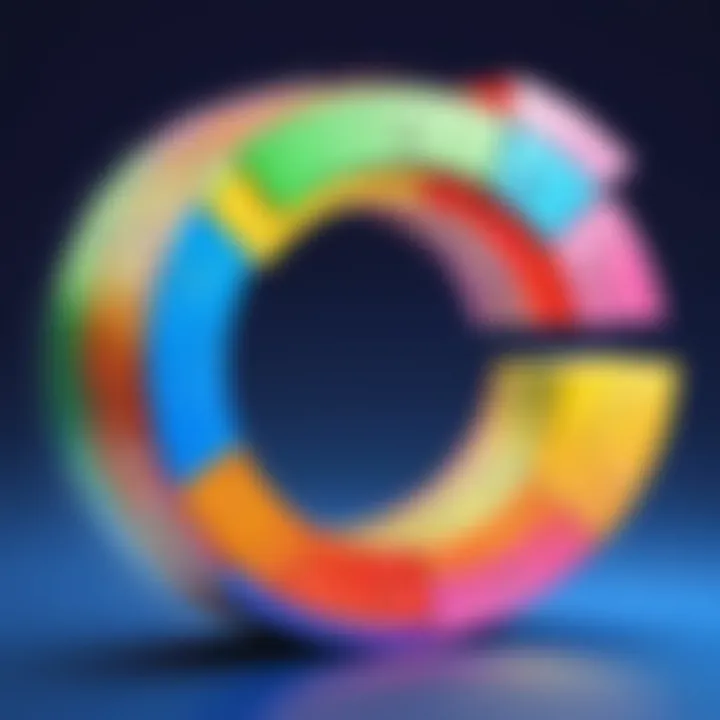
Intro
C# is a versatile programming language targeted towards modern application development. It has become a foundational tool for many programmers and organizations, known for its strong typing, advanced features, and ability to integrate seamlessly with various platforms. Our aim is to explore the depths of C#, from essential principles to advanced concepts, enhancing the reader's skill set.
Understanding C# requires more than knowing syntax; it necessitates grasping fundamental programming constructs, best practices, and how to effectively utilize its rich ecosystem. The use of C spans various areas, including web development, mobile applications, and even game design. Each of these domains can benefit greatly from a programmer's solid understanding of the language.
By examining the core features of C#, developers can build efficient software systems. Delving into its object-oriented aspects, one can appreciate the language's structure that promotes code reusability and clean design. Moreover, with the continuous evolution of technology, keeping updated with recent advancements becomes vital.
In this article, we will guide you through the intricacies of C#, touching upon crucial coding challenges, contemporary technologies that complement C#, valuable resources for learning, and foundational computer science aspects tied to the language.
Coding Challenges
Coding challenges form the backbone of a skilled programmer's toolkit. They enhance problem-solving skills and help in understanding C# in a practical context.
Weekly Coding Challenges
Engaging in regular coding challenges propels one’s programming abilities. Platforms such as the leaderboards on Codewars or LeetCode provide motivation through friendly competitions. Each problem serves as a new puzzle to solve, reinforcing techniques and sharpening logical reasoning.
Problem Solutions and Explanations
Generally, approaching coding challenges involves iteration. For example, problems regarding string manipulation or data sorting are common. Here, it is useful to implement efficient algorithms to ensure optimized solutions. Once a challenge is solved, breaking down the solution enhances understanding. It’s essential to revisit the logic and explore alternative approaches.
“Programming is not about typing, it’s about thinking.” — Casey Patten
Tips and Strategies for Coding Challenges
To excel in coding challenges, consider the following strategies:
- Start simple: Tackle easier problems first. This builds confidence.
- Write pseudocode: Outline your thoughts in plain language before coding.
- Test thoroughly: Validate outcomes with a variety of test cases.
- Learn from peers: Examine others' code solutions; insights can lead to new understandings.
Community Participation Highlights
One valuable aspect of programming is community interaction. Websites like Reddit or specialized forums display diverse approaches to coding challenges. Involvement in community discussions and reviews not only enriches knowledge but can also provide support in overcoming obstacles in one’s learning journey.
Understanding C# through the lens of coding challenges ensures a deep dive into the language. Tackling various tasks reveals the spectrum of C#'s functions and reinforces knowledge gain. This exploration sets the groundwork for further refined insights into emerging technology trends, guides, and crucial computer science concepts.
Prologue to
C# serves as a pivotal language in the realm of programming, offering a blend of simplicity, power, and versatility. Understanding the foundations and the evolution of C equips programmers with the knowledge needed to leverage its features in a variety of applications. This section introduces C considering its historical background and current significance.
History and Evolution
C# was created by Microsoft in the early 2000s as part of its .NET initiative. The first version of C was released alongside .NET Framework in 2002. It drew principles from other languages such as Java, C++, and Visual Basic, aimed at creating a user-friendly environment.
The language has evolved extensively. Major versions have introduced new features such as generics in C# 2.0, LINQ in C 3.0, and asynchronous programming with async/await in C 5. Every iteration strengthened its ecosystem, making it relevant for modern development practices.
These refinements have transformed C# from a language tailored for Windows applications into a versatile entity used across various platforms, including web, cloud, and game development. Recognizing this growth is key for anyone pursuing a career in tech, as it highlights the significance of adaptability in programming.
Current Relevance in Programming
At present, C# remains a critical language for developers across sectors. It is predominantly used in enterprises for backend systems and web applications through ASP.NET. The ongoing advancement of technologies like Azure has further bolstered C#'s role, making it an integral tool for cloud-based solutions.
The gaming industry also relies on C#, notably with game engines like Unity, which is a leading platform for game development. Unity enables users to create rich, 2D, and 3D experiences using C#, grounding the language's practical application in a thriving field.
C#'s relevance is sustained not only by its uses in existing frameworks but also by supportive communities on forums such as Reddit, where programmers exchange knowledge and experiences. The evolution of C# reflects the need for continuous learning, making it a valuable asset in a programmer's career.
In summary, C#'s journey from its inception to current-day relevance epitomizes its importance in the ever-evolving landscape of technology. Exploring C# equips both aspiring and experienced programmers with the skills to capitalize on its robust capabilities.
Language Fundamentals
C# is a language that stands out in the world of programming due to its versatility. Understanding the fundamentals is essential for any programmer who wants to excel in C#. This section covers the basis of the C language, breaking down its syntax, data types, and control structures. By grasping these core elements, one builds a strong foundation that contributes to more advanced topics later on.
Syntax Overview
C# has a clear and modern syntax, making it relatively easy to read and write compared to some other programming languages. It follows a C-style syntax, which is not only familiar to many programmers but also consistent.
Every C# program executes within a class, identified by the keyword. The main execution point is defined using the method. Basic syntax involves defining a namespace, class, and methods. To demonstrate:
Some important syntax rules to keep in mind:
- Semicolons () are used to indicate the end of a statement.
- Keywords (like , , and ) dictate the visibility and accessibility of classes and methods.
Having a strong grip on syntax lays the groundwork for learning more complex constructs in C#.
Data Types and Variables
C# employs a rich set of data types for varied applications. Understanding these types is vital as they define the kind of data you can work with. C is a statically typed language, meaning all variables must have an assigned data type before they are used, and type checks are performed at compile time.
Key data types include:
- int: Represents integer types.
- double: For floating-point numbers.
- string: Holds sequences of characters.
- bool: Represents true or false values.
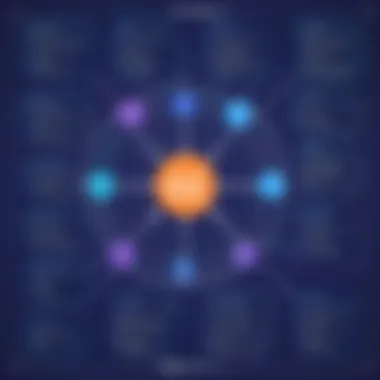
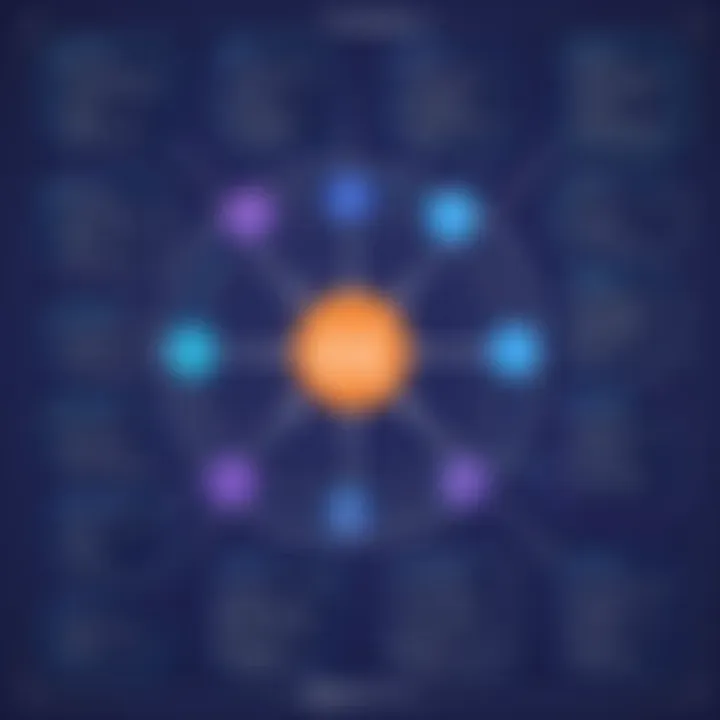
When declaring variables, you need to specify the data type:
Aside from primitive types, C# supports complex data types like arrays and collections, allowing for more structured data organization. Using the appropriate data type improves efficiency and optimizes memory usage.
Control Structures
Control structures direct the flow of the program based on conditions. Understanding these structures is fundamental to building dynamic applications. C# includes a variety of control structures like conditionals and loops, which provide flexibility in the execution of code.
If-Else Statement
The statement evaluates a condition and executes a block of code if true. This provides a way to introduce decision-making in your program:
Loops
Loops enable code to run multiple times, thus reducing redundancy. The loop, loop, and loop are common ways to iterate over a collection or execute a block a specified number of times.
Understanding these control structures helps create infinitely versatile programs capable of responding to different scenarios and user input.
By mastering C# Language Fundamentals, programmers can leverage the full power of C effectively in any software development environment.
Object-Oriented Programming in
Object-oriented programming (OOP) in C# is a fundamental paradigm that shapes how software is designed and developed. By utilizing OOP principles, developers can create modular, reusable, and scalable code. The significance of OOP in C revolves around encapsulation, inheritance, and polymorphism. These principles help in managing large codebases and making programs more understandable. As C is mainly used in large applications, mastering its OOP aspects offers a competitive advantage.
Classes and Objects
Defining Classes
Defining classes in C# is central to the object-oriented paradigm. A class acts as a blueprint for creating objects, which are instances of the class. This foundational concept allows for code reuse and organization. With proper class definitions, programmers can encapsulate data and behaviors relevant to the problem being solved.
One key characteristic of classes is their ability to model complex systems. They keep related properties and methods together, leading to enhanced readability and maintenance. When classes are defined properly, developers can efficiently manage interactions within large applications. This encapsulation not only simplifies coding tasks but also lowers the potential for errors during the development life cycle.
But, one downside to be aware of is the complexity that can arise if classes are not organized thoughtfully. Classes that become too large or attempt to handle too many responsibilities can make maintenance cumbersome, challenging even seasoned developers.
Creating Objects
Creating objects is straightforward in C#. Objects bring life to classes, transforming cold definitions into functional components in your program. Creating an object involves instantiating a class, utilizing its constructor to set initial values or state. This initiation allows for interaction with the programming constructs stored in memory.
The primary characteristic that makes object creation appealing is its clarity. Each object operates based on its defined structure, instantiating state and behaviors that derive from its class. This principle supports organized programming, ensuring newer developers understand how to structure their work from the ground up.
However, instantiating excessive objects wastefully can introduce inefficiencies in memory allocation. Therefore, while utilizing objects enhances usability, careful management is essential to optimize resource usage.
Inheritance and Polymorphism
Understanding Inheritance
Inheritance in C# permits a new class to derive properties and methods from another. This allows developers to create a hierarchy amongst classes that promotes reuse. Users can define a general base class and create derived classes with specialized features. One key characteristic is that code can be inherited easily, reducing redundancy.
This is a beneficial approach in structuring applications, as it streamlines the process of extending functionality. Still, over-using inheritance can lead to tight coupling between classes, which may cause difficulties during system updates or debugging related issues.
Implementing Polymorphism
Polymorphism enables methods to do different things based on the object that is calling them. Through polymorphism, a single interface can lead to a variety of implementations. This enhances flexibility, allowing for dynamic programming tactics when creating complex systems.
The primary characteristic here is that it provides a mechanism for code extensibility. Its benefit is that programmers can introduce new class behaviors into a program without altering the original software. However, understanding polymorphism thoroughly can require a significant cognitive load, which might confuse beginner developers.
Encapsulation and Abstraction
Using Access Modifiers
Access modifiers in C# enhance encapsulation by controlling visibility. These modifiers, such as public, private, and protected, help to define who can access a class's attributes and methods. One of the major aspects is that encapsulation aids in restricting access to the underlying system to promote security and maintainability.
This is valuable as it reduces the risk of errors caused by unintended interactions with class internals. A disadvantage, however, lies in the complexity they introduce; when visibility rules become convoluted, they can create harder navigation for developers needing to interface with the codebase.
Abstract Classes and Interfaces
Abstract classes and interfaces provide means to achieve abstraction in programming. An abstract class can define methods that must be implemented by derived classes, allowing for a consistent interface without the need for full implementation. Interfaces operate similarly but do not provide any stain code and only describe behavior.
The key characteristic beneficial to developers is the flexibility of implementation. They are highly useful in collaborative projects where multiple developers need to adhere to shared principles. Nevertheless, they require discipline, and misuse could result in indirectly inducing confusion within teams, especially among novices.
Advanced
Concepts
Expanding your expertise in C# necessitates a comprehensive understanding of its advanced concepts. This knowledge unlocks the full potential of the language, allowing developers to write efficient, sophisticated code. Understanding advanced topics enhances not only application performance but also improves data handling and user interactions in software solutions.
Delegates and Events
Delegates are a foundational element of C# programming, acting as type-safe function pointers. They enable methods to be passed as parameters, fostering flexibility and reusability in code. An important usage scenario is in event handling, where delegates provide a means to respond to events in an organized manner. For instance, when a button is clicked in a user interface, that action can trigger a method directly tied to the button via a delegate.
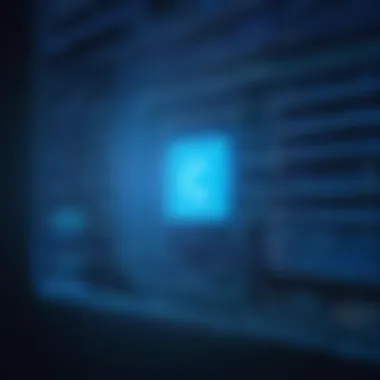
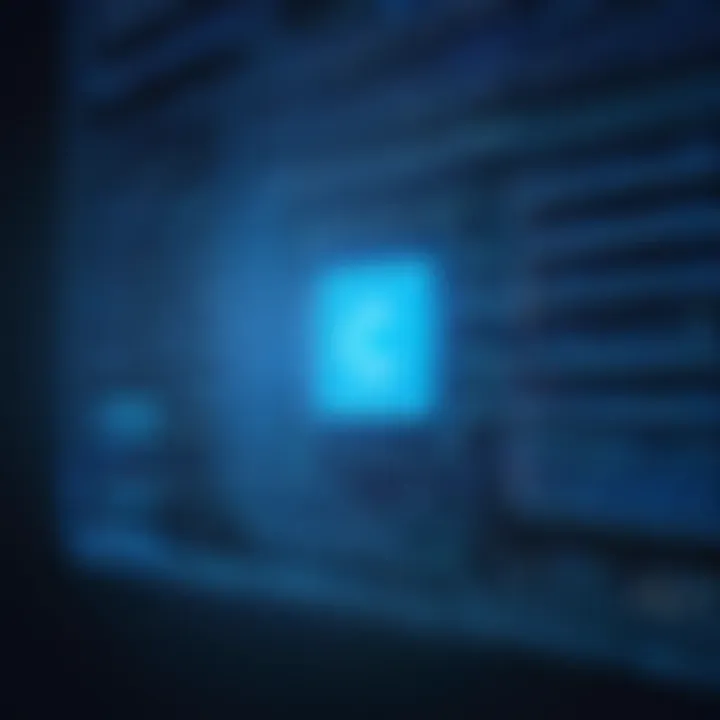
Events, closely related to delegates, provide a published-subscribe model where methods subscribe to specific actions. This structure naturally leads to a more decoupled design.
Advantages to understanding delegates and events include:
- Encapsulation of code behavior: Method encapsulation makes it easier to manage complex interactions.
- Improved code readability: Using delegates can simplify the main program flow by separating behaviors.
- Facilitated event-driven programming: Many modern applications follow an event-driven model, which relies heavily on these concepts.
LINQ and Data Manipulation
Language Integrated Query, or LINQ, revolutionizes data manipulation in C#. It provides a consistent model for querying various data sources, ranging from databases to in-memory collections, enabling programmers to integrate declarative data access behavior. This capability promotes concise code and enables deep integration with other C# features.
LINQ supports syntax both in SQL-like and method-based formats, appealing to various coding styles. Some key benefits of using LINQ include:
- Readable queries: LINQ brings clarity to data retrieval, making it easier to construct and debug.
- Strongly typed checks: Compile-time checks reduce runtime errors related to queries.
- Integration: Eases the integration with complex data sources without sacrificing speed.
Here's a brief example of LINQ usage in finding elements within a numerical array:
This clear, expressive syntax captures the essence of what data manipulation with LINQ aims for.
Asynchronous Programming
As C# has matured, asynchronous programming has taken center stage. Understanding how to leverage async and await keywords greatly enhances performance, especially in applications that conduct operations prone to latency. This area is crucial for developers aiming to create responsive applications, as it frees the main thread to continue running while background tasks execute.
In asynchronous programming, developers must grasp how tasks function.
Consider the benefits:
- Responsiveness: Users benefit from smoother application interactions.
- Resource Optimization: Threads are managed efficiently, enhancing application performance under load.
- Comprehensive error handling: Offers structured error management mechanisms.
An important code segment might look like this:
This shows not just the efficiency gained, but also the clarity that can be achieved through asynchronous methods.
Working with
Frameworks
Understanding frameworks is key when programming in C#. They provide tools and libraries that accelerate development. Whether for web applications, game design, or desktop solutions, selecting the right framework can significantly impact efficiency and functionality.
Frameworks encapsulate common functionalities, allowing developers to concentrate on intricate algorithms and features rather than reinventing the wheel. They follow best practices, which can lead to cleaner code and improved collaboration among team members.
However, framework selection requires careful consideration in relation to project requirements, performance, and community support.
.
NET Framework vs .NET Core
When discussing C# frameworks, one immediately encounters the .NET Framework and .NET Core. The traditional .NET Framework is primarily for Windows applications. Certainly it features a comprehensive library and supports various programming languages. Despite its capabilities, its dependence on Windows limits its versatility.
.NET Core, conversely, is open-source and cross-platform. This versatility allows developers to build applications that run not just on Windows, but also on macOS and Linux. The performance of .NET Core is impressive, often preferable in modern development settings.
Key Differences:
- Compatibility: .NET Core offers broader platform support.
- Performance: .NET Core often exceeds .NET Framework in speed.
- Development Tools: Both have a rich set of tools, but .NET Core stays more current in updates.
ASP.
NET for Web Development
ASP.NET revolutionizes the approach to building web applications with C#. It extends the .NET insights into web app development. With flexibility and powerful features, ASP.NET is ideal for dynamic webpage creation and can also serve as an API backend.
Key elements of ASP.NET include:
- Robust Security Features: Many built-in safeguards secure data effectively.
- Separation of Concerns: MVC (Model-View-Controller) framework encourages organized code, improving maintenance.
- Support for Various Formats: RESTful services can effortlessly deliver XML, JSON, or other data formats.
Its versatility makes ASP.NET an essential competence for web developers working with C#.
Unity for Game Development
Unity is an extraordinarily popular framework among game developers. Its use of C# enhances game logic, graphics, and physics interactions effectively. Unity supports various platforms—from consoles to mobile devices—which broadens a developer's reach.
Key advantages of Unity include:
- Cross-Platform Compatibility: Write code once, deploy everywhere, which saves considerable time.
- Asset Store: A rich library of assets can help designers and developers save from scratch.
- Multiplayer Services: Build multiplayer experiences with unity’s built-in tools.
Due to its user-friendly interface and robust community, Unity remains a preferred choice in game development environments.
Testing and Debugging in
Testing and debugging are critical elements in the C# programming process. They ensure that the code not only works as intended but also meets the requirements effectively. A robust testing and debugging strategy can significantly reduce errors and improve performance. Developers who invest time in these practices will benefit from increased productivity and reduced future maintenance costs. Therefore, understanding how to conduct testing and debugging is essential for both new and seasoned programmers.
Unit Testing Principles
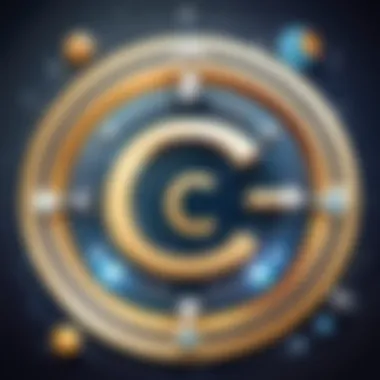
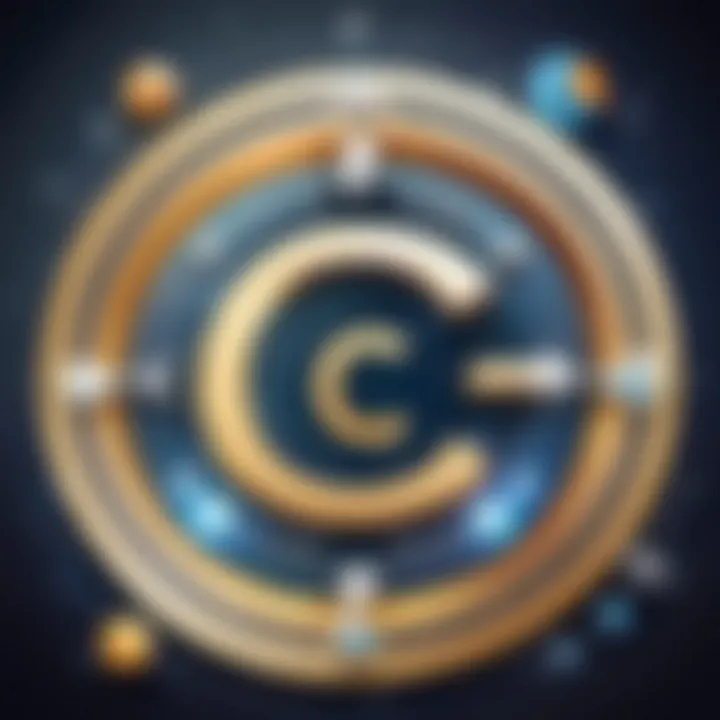
Unit testing focuses on verifying the smallest parts of an application, like individual functions or methods, in isolation. Each test determines whether the function behaves as expected given certain inputs. The principles of unit testing include:
- Test Coverage: Aim for a high percentage of your functions tested.
- Test Independence: Ensure that each test runs independently and does not affect another.
- Naming Conventions: Use clear and descriptive names for tests to explain what they verify.
- Automated Testing: Utilize testing frameworks, like NUnit or xUnit, to automate tests.
Unit tests not only can find bugs early but also serve as documentation for code functionality. This practice ensures that alterations in the codebase retain the intended behavior. A significant aspect of unit testing is when programmers refactor code. They can run existing tests to ensure nothing breaks, maintaining a high standard of quality while enhancing code over time.
Common Debugging Techniques
Debugging is the process of identifying and resolving issues in the code effectively. C# provides various techniques and tools that programmers can use to make this task manageable.
- Breakpoints: These allow programmers to pause the execution of code at critical points. By inspecting variables and the flow of execution at these breakpoints, you isolate the issue efficiently.
- Step Over and Step Into: When stepping through code, you can control execution flow and see if the methods behave as expected. Step Over allows you to skip code execution at a method call, while Step Into executes the funciton,
- Debugging Console: Utilize the console window for outputting values and understanding the runtime state of your application.
- Error Handling: Proper exception handling can simplify debugging by recording important information about errors, instead of allowing them to crash the application.
To take advantage of debugging tools effectively, it is important to structure your code into smaller chunks. A clear organization helps identify issues faster and focus on the part of the code that caused the malfunction. Implementing these techniques systematically can help developers refine their skills, ultimately leading to higher code quality.
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it." - Brian W. Kernighan
Best Practices for
Programming
Best practices in C# programming are essential for developing efficient, robust, and maintainable software. Following these practices enhances code quality, facilitates collaboration, and improves project manageability. Understanding and applying best practices ensures that code is easier to read, test, and modify.
Code Readability and Maintainability
Code readability directly impacts its maintainability. Highly readable code allows programmers, including those who may not be the original authors, to easily understand the logic, structure, and intent behind the code. Here are key points to consider:
- Consistent Naming Conventions: Use meaningful names for variables, methods, and classes. A well-chosen name should give an immediate idea of its functionality or the data it holds. For instance, prefer over a vague .
- Clear Code Structure: Organize code logically using regions, namespaces, and appropriate file structures. This reduces cognitive load for someone navigating the code for the first time.
- Comment Sparingly but Effectively: Aim to write self-explanatory code, however, where necessary, include comments to clarify complex logic. Avoid over-commenting; redundant comments can clutter the code.
- Consistent Formatting: Applying consistent indentation and spacing is critical. Ensure each member follows the same formatting style across the project, making it visually coherent.
Utilizing tools such as ReSharper or CodeMaid can assist in maintaining code quality and consistency. They can automatically reformat code for readability, ensuring that even larger chunks remain accessible.
Performance Optimization Tips
Optimizing performance is key for building high-efficiency applications. In C#, several techniques can be employed to ensure your code runs smoothly and optimally:
- Use StringBuilder for String Manipulation: When performing multiple string modifications, prefer over regular string concatenation. This can dramatically reduce memory overhead and improve performance due to its mutable properties.
- Minimize Object Creation: Excessive object creation can lead to heap fragmentation and garbage collection overhead. Reuse objects when feasible, especially in performance-critical loops.
- Profile and Benchmark Your Code: Utilize tools such as BenchmarkDotNet to measure performance impacts of code changes. Understanding where bottlenecks lie allows for targeted optimizations.
- Employ Lazy Initialization: Use lazy loading for initializing heavy resources only when they are needed. This can speed up your application’s startup time, making it feel more responsive to the user.
Each performance tip stems from practical scenarios that C# developers face regularly. Creating a culture of performance consideration among teams favors sustainable practices in the long term.
Applying the principles outlined not only keeps code manageable but also improves its execution and developer efficiency over time.
Community and Resources
C# is more than a programming language; it is a vibrant community of developers, learners, and enthusiasts. Engaging with this community is crucial for anyone looking to enhance their skills or stay updated with the latest trends and technologies. The C community provides invaluable resources such as forums, discussion groups, and events where programmers can share experiences, seek help, and collaborate on projects. The connections formed within these communities can lead to mentorship opportunities and collaborative endeavors, crucial for personal and professional growth.
Key Online Forums and Groups
Joining online forums and groups dedicated to C# can vastly enhance your programming journey. Here are notable resources that you can explore:
- Stack Overflow: A question-and-answer site, it is one of the most active forums where developers post questions related to C#. Users can get rapid responses and solutions from experienced peers.
- Reddit: Subreddits such as r/csharp are excellent for discussion about C#. You can find tips, tutorials, and community challenges suitable for different skill levels.
- Microsoft Developer Network: A central hub for learning and collaboration on C#, this is where Microsoft provides official documentation, updates, and best practices.
- GitHub: Engaging in projects via GitHub can improve your coding skills and allow you to learn by collaborating with others. You can explore existing repositories or start your own C# project.
Participating in these forums allows for direct interaction with a variety of programmers, and your understanding of C# can deepen through collaborative problem-solving and shared projects.
Recommended Learning Resources
To build proficient C# skills and advance your programming career, accessing structured resources is essential. Below are some recommended materials that valuable for programmers:
- Microsoft Learn: Official and comprehensive. The tutorials cover everything from fundamentals to advanced topics in C#. This is a state-of-the-art resource.
- Pluralsight: Offers courses designed by industry professionals. Its C# pathway presents a systematic approach to learning with various projects.
- Books: Titles like C# in Depth by Jon Skeet and Pro C 9 by Andrew Troelsen provide in-depth knowledge and are critical for serious learners.
- YouTube Tutorials: Channels focusing on C# can deliver practical guidance on specific concepts like LINQ or asynchronous programming.
- Local Meetups/Groups: Finding a local user group or attending C# conferences can place you in direct contact with experts and other learners.
By leveraging these resources, you can significantly improve your C# programming skills and connect with like-minded individuals passionate about technology.
Engaging with the C# community can help developers find guidance, inspire creativity, and encourage lifelong learning in a constantly evolving tech landscape.
Ending
The conclusion of this article highlights the significance of C# as a programming language and encapsulates the essential aspects discussed throughout the text. As C remains a pivotal component within many software development projects, understanding its core principles and diverse capabilities signifies a key step for any programmer. This structural wrap-up aims to consolidate learning, reinforcing the knowledge and skills aimed at practical applications.
Recap of Key Points
The examination of C# includes notable tenets that stand out. Recognizing core language fundamentals such as syntax, data types, and control structures serves as the foundation. Additionally, object-oriented principles are vital. Developers must appreciate the nuances of classes and objects, and the significance of encapsulation and polymorphism.
From advanced concepts like LINQ to asynchronous programming, the ability to leverage frameworks like ASP.NET gives programmers distinct advantages in web and application development. Best practices in coding further elevate maintainability and performance, fostering clean, efficient, and sustainable code.
- Key Takeaways:
- Understand basic and advanced C# concepts.
- Learn about OOP principles that shape C# programming.
- Optimize code for clarity and performance.
By reinforcing these key points, programmers enhance their skills and readiness for real-world applications of C#.
Future of
and Programming Trends
Looking forward, C# is poised to navigate the evolving landscape of technology. The rise in demand for cloud computing, machine learning, and cross-platform applications positions C favorably, particularly with technologies like .NET 6 and .NET MAUI expanding its capabilities.
As community contributions and open-source initiatives continue to shape C#, collaboration may bring unprecedented innovations in language features and enhancements.
Factors impacting the future programming trends include:
- Maintaining backward compatibility with legacy applications.
- Growing emphasis on cloud-based solutions like Azure.
- Integrating artificial intelligence and data science capabilities directly within C# solutions.
In summary, C# is not just surviving but thriving. It enhances its relevance and utility amid constant change marks the future of software development.