Master Ruby Programming: A Complete Guide for Developers
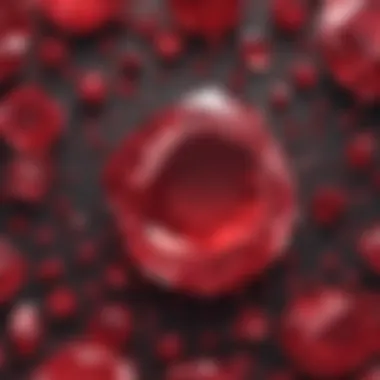
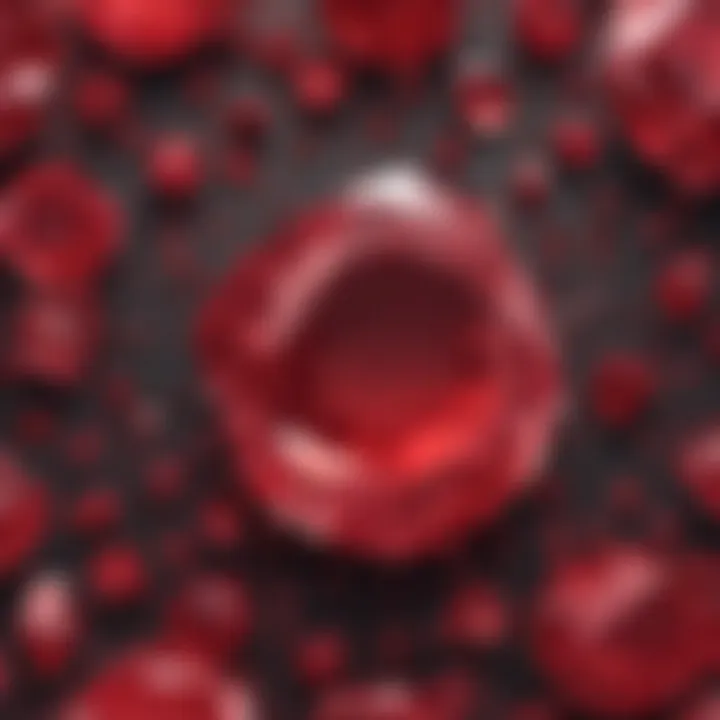
Coding Challenges
As we embark on our journey towards mastering Ruby programming, it is essential to test our understanding and skills through weekly coding challenges. These challenges serve as a practical application of the concepts discussed in the course, allowing us to apply our knowledge in solving real-world problems. By actively participating in these coding challenges, we enhance our problem-solving abilities, logic reasoning, and programming proficiency. Subsequently, exploring the problem solutions and explanations for each challenge enables a deeper comprehension of the Ruby syntax, data structures, and algorithms involved. Furthermore, delving into tips and strategies for coding challenges equips us with valuable techniques to approach and tackle complex coding scenarios effectively. The sense of community participation in these challenges fosters a collaborative learning environment, where individuals can share insights, learn from each other, and collectively improve their Ruby programming skills.
Introduction to Ruby Programming
Understanding Ruby Basics
Overview of Ruby Language
The overview of the Ruby language provides a comprehensive look at the syntax and structure that define Ruby. This section delves into the unique features of Ruby, such as its object-oriented nature, simplicity, and flexibility. Understanding the core aspects of Ruby's design philosophy and syntax is essential for programming proficiency. Exploring the nuances of Ruby's language constructs offers insights into why Ruby remains a popular choice among developers.
Setting up Ruby Environment
Setting up the Ruby environment is a crucial initial step for any developer venturing into Ruby programming. This subsection focuses on the tools and configurations required to create a conducive development environment. Emphasizing the importance of a well-configured environment ensures smooth coding processes and efficient debugging practices. Despite potential challenges, optimizing the Ruby environment can significantly enhance productivity and code quality.
Variables and Data Types
Variables and data types form the backbone of any programming language, including Ruby. This segment delves into the different variable types available in Ruby and their respective use cases. Understanding data types and variable declarations is vital for effective data manipulation and storage within Ruby programs. Mastery of variables and data types empowers developers to write efficient and reliable code.
Operators and Expressions
Operators and expressions play a pivotal role in conducting operations and evaluations within Ruby programs. This section elucidates the various operators supported by Ruby, along with their precedence and functionality. Exploring different expressions and their impact on program flow equips developers with the tools to create logical and efficient code structures. The strategic use of operators enhances code readability and functionality.
Control Structures and Loops
Conditional Statements
Conditional statements allow developers to introduce decision-making logic into their Ruby programs. This subsection scrutinizes the different conditional constructs available in Ruby, highlighting their syntax and usage. Employing well-defined conditional statements enables developers to execute specific code blocks based on predefined conditions, enhancing program flexibility and control flow.
Looping Constructs
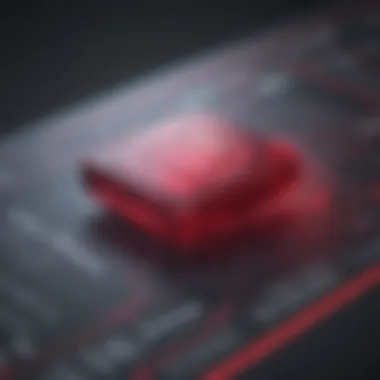
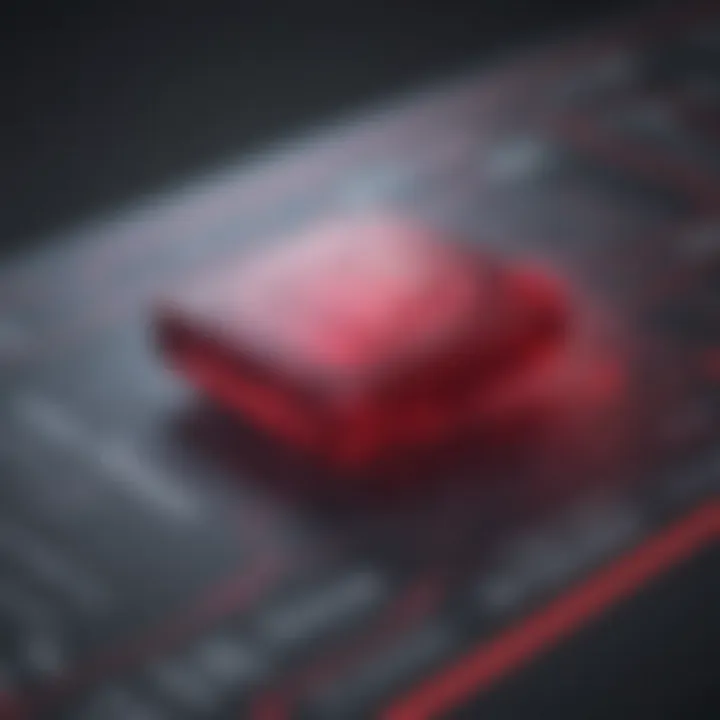
Looping constructs offer developers the ability to iterate over data structures or execute specific code iteratively. This part of the section delves into the various loop types supported by Ruby, elucidating their differences and best use cases. Effectively implementing looping constructs optimizes code repetition and fosters efficient data processing within Ruby programs.
Iterators and Enumerators
Iterators and enumerators provide a convenient mechanism for traversing and manipulating collections in Ruby. This segment explores the intricacies of iterators and enumerators, emphasizing their role in simplifying iterative operations. Leveraging iterators and enumerators enhances code readability and conciseness, facilitating streamlined data processing and manipulation.
Methods and Functions in Ruby
Defining and Calling Methods
Methods encapsulate reusable blocks of code in Ruby, facilitating code organization and reusability. This subsection delves into the process of defining and invoking methods in Ruby, outlining best practices and conventions. Understanding the intricacies of method definition and invocation enhances code modularization and simplifies program maintenance.
Method Arguments and Return Values
Method arguments and return values are essential components of method invocation in Ruby. This section explores the nuances of passing arguments to methods and handling return values effectively. Mastery of method arguments and return values allows developers to create versatile and adaptable functions within their Ruby programs.
Built-in vs. Custom Methods
Distinguishing between built-in and custom methods in Ruby is vital for leveraging existing functionalities and creating bespoke solutions. This part of the section elucidates the benefits and limitations of both built-in and custom methods. Striking a balance between utilizing built-in methods for efficiency and crafting custom methods for specific requirements empowers developers to write robust and flexible Ruby code.
Advanced Ruby Concepts
Object-Oriented Programming in Ruby
Object-Oriented Programming (OOP) in Ruby is a fundamental paradigm that emphasizes the use of classes and objects to structure code efficiently. Classes are blueprints for creating objects, encapsulating data, and behavior within a single entity. Objects, on the other hand, are instances of classes that interact with each other through methods. The power of OOP lies in its ability to organize code logically, promote code reusability, and enhance maintainability.
Classes and Objects
Classes and objects are integral components of OOP in Ruby, allowing developers to encapsulate properties and behaviors within a unified structure. Classes define the characteristics and behaviors common to a set of objects, providing a template for creating new instances. Objects, instantiated from classes, interact by sending messages to each other, fostering a modular and scalable codebase. The versatility of classes and objects enables developers to model real-world entities accurately and efficiently, promoting a more structured approach to software development.
Inheritance and Polymorphism
Inheritance and polymorphism are key principles of OOP that facilitate code reuse and promote extensibility. Inheritance allows a class to inherit attributes and methods from another class, establishing a hierarchical relationship between classes. Polymorphism enables objects of different classes to be treated interchangeably, increasing flexibility and simplifying code maintenance. By leveraging inheritance and polymorphism, developers can design flexible and modular codebases that adapt to changing requirements with ease.
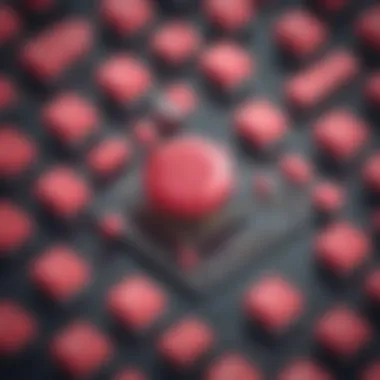
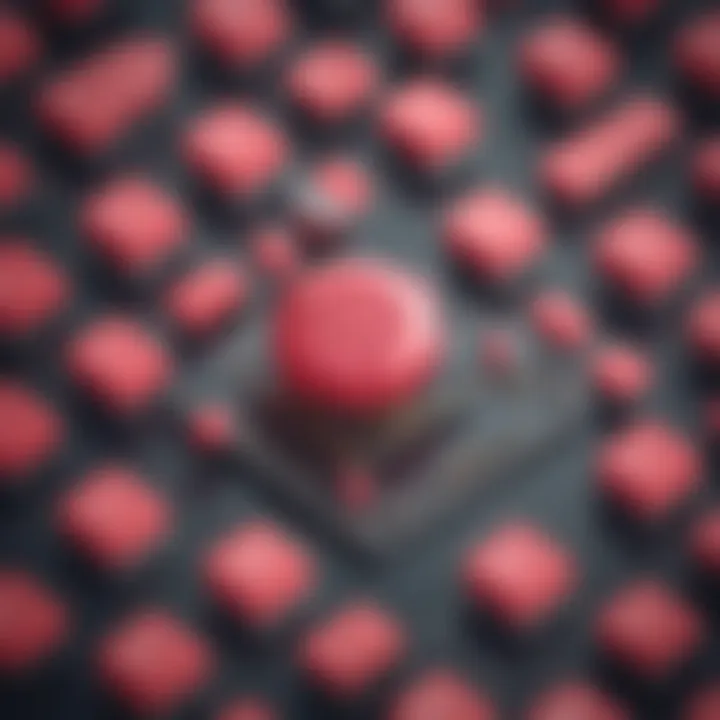
Modules and Mixins
Modules and mixins offer a versatile way to enhance code reuse and maintain a modular codebase in Ruby. Modules encapsulate methods, constants, and variables, acting as libraries of functionalities that can be mixed into classes. Mixins, achieved through including modules in classes, enable shared behavior across multiple classes without the need for inheritance. This promotes code organization, reduces duplication, and enhances the flexibility of Ruby applications.
Working with Collections
Efficient manipulation of collections is essential in Ruby programming to process data effectively and facilitate complex operations. By leveraging arrays, hashes, enumerables, iterators, and sorting techniques, developers can streamline data management and enhance the efficiency of their algorithms.
Arrays and Hashes
Arrays and hashes are fundamental data structures in Ruby that facilitate the storage and retrieval of data in an organized manner. Arrays store elements sequentially, allowing easy access through indexes, while hashes store key-value pairs for efficient data lookup. Both arrays and hashes offer versatile data storage options, catering to diverse programming needs and enabling developers to work with structured data effectively.
Enumerables and Iterators
Enumerables and iterators provide developers with powerful tools for iterating over collections and processing elements efficiently. Enumerables offer a range of methods for iterating, filtering, and transforming data, enhancing productivity and simplifying complex operations. Iterators, such as 'each' and 'map,' enable developers to traverse collections and apply operations to each element, promoting code clarity and readability.
Sorting and Filtering Data
Sorting and filtering data play a crucial role in organizing and manipulating collections effectively. Sorting allows developers to arrange data in a specified order, such as alphabetical or numerical, to facilitate searching and data retrieval. Filtering enables developers to extract specific elements from a collection based on defined criteria, enabling precise data processing and analysis.
Error Handling and Exceptions
Error handling and exception management are critical aspects of robust software development in Ruby, ensuring graceful handling of unexpected scenarios and providing mechanisms to recover from errors effectively.
Rescuing Errors
Rescuing errors involves capturing and handling exceptions that occur during program execution, preventing abrupt termination and allowing for controlled error recovery. By implementing exception handling mechanisms, developers can anticipate and manage unforeseen errors, enhancing the reliability and resilience of their applications.
Throw and Catch Mechanism
The throw and catch mechanism provides a way to jump out of nested control structures and transfer control to a designated block of code. By utilizing throw to raise an exception and catch to capture and process the exception, developers can manage flow control and execute specific handling routines based on program logic. This mechanism enhances the flexibility and control flow within Ruby programs, enabling developers to handle exceptional conditions effectively.
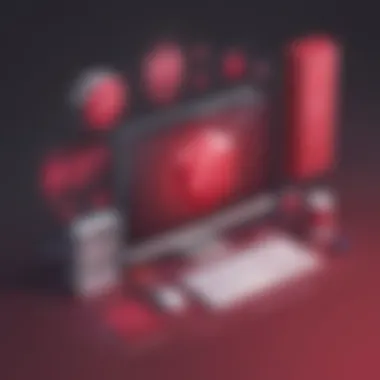
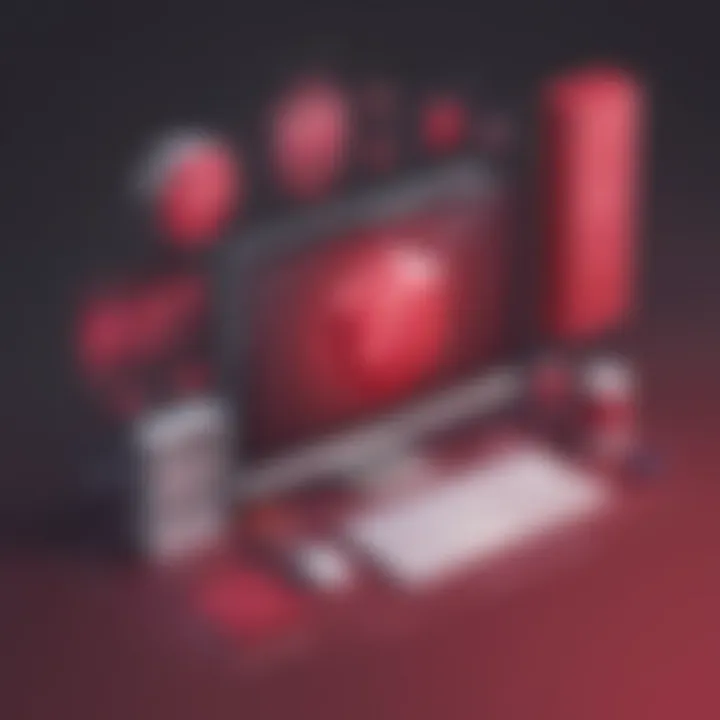
Using Custom Exceptions
Custom exceptions empower developers to define and raise specialized exceptions tailored to their specific application logic. By creating custom exception classes that inherit from Ruby's standard exception class, developers can distinguish and handle unique error scenarios distinctively. Using custom exceptions enhances code clarity, simplifies error diagnostics, and promotes precise error identification and resolution.
Ruby Programming Best Practices
Writing Clean and Readable Code
Code Formatting Guidelines
Code Formatting Guidelines play a crucial role in maintaining code consistency and readability within the Ruby programming landscape. These guidelines encompass rules for indentation, spacing, line length, and code organization. By adhering to these guidelines, developers can enhance code comprehensibility, streamline collaboration, and expedite debugging processes. The strict adherence to formatting standards promotes code uniformity and reduces ambiguity in code interpretation, ultimately leading to a more maintainable and scalable codebase.
Naming Conventions
Naming Conventions serve as the foundation for creating coherent and standardized naming patterns for variables, methods, classes, and other elements in Ruby code. By following naming conventions consistently, developers can improve code clarity, facilitate code navigation, and enforce a predictable structure across projects. The clarity introduced by meaningful and consistent names contributes to code maintainability, simplifies troubleshooting, and enhances the scalability of the application architecture.
Refactoring Techniques
Refactoring Techniques involve the process of restructuring existing code to enhance code quality, reduce complexity, and optimize performance without altering the external functionality. Through refactoring, developers can improve code maintainability, eliminate duplications, and enhance code readability. By systematically applying refactoring practices, developers can incrementally evolve the codebase, address technical debt, and ensure the long-term sustainability and extensibility of the software application.
Practical Applications of Ruby (300- words):
Practical Applications of Ruby hold immense significance in this exhaustive guide on mastering Ruby programming. By delving into the realm of real-world utilization, learners can comprehend the practicality and versatility of Ruby beyond theoretical concepts. This section sheds light on how to implement Ruby in diverse scenarios, ranging from web development to data analysis. Understanding the practical applications equips both novices and seasoned developers with the necessary skills to apply Ruby efficiently in various projects, ensuring an adept comprehension of its potential.
Web Development with Ruby on Rails (250- words):
Overview of Rails Framework:
The Overview of Rails Framework is a pivotal component of this course, offering insights into the foundational structure and architecture of the Ruby on Rails framework. It elucidates the framework's core principles, emphasizing its role in accelerating web development processes. The Rails framework's convention over configuration approach simplifies coding tasks and streamlines project organization, making it a preferred choice for aspiring and professional developers alike. Despite its advantages, familiarity with the Rails framework is essential to leverage its capabilities optimally.
Building Web Applications:
The Build Web Applications section delves into the intricacies of constructing robust and scalable web applications using Ruby. It highlights the significance of architecture design, database integration, and user interface development in creating functional web solutions. By emphasizing best practices and efficient coding methodologies, this segment equips learners with the skills to develop dynamic web applications effectively. Understanding the nuances of building web applications is imperative for mastering Ruby programming comprehensively.
RESTful APIs with Rails:
The RESTful APIs with Rails portion explores the implementation of Representational State Transfer (REST) architecture in web services using Ruby. It underscores the significance of creating APIs that adhere to REST principles, promoting interoperability and standardization in web communication. Leveraging Ruby on Rails for developing RESTful APIs provides a structured approach to interface design and data communication. However, practitioners need to grasp the nuances of RESTful API development to ensure seamless integration within projects, enhancing overall performance and functionality.