A Comprehensive Guide to Creating a Basic Discord Bot
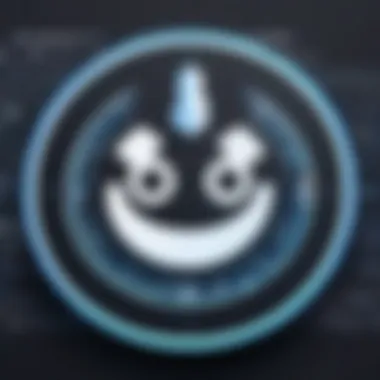
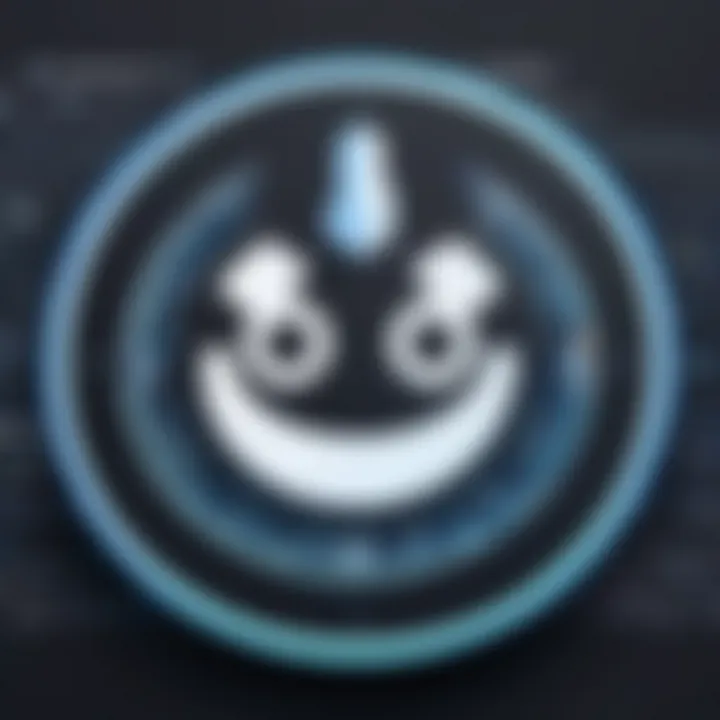
Intro
Creating a Discord bot can seem daunting at first. However, with the right tools and knowledge, it is an achievable goal for programmers at any level. This guide aims to illuminate the steps for setting up a basic bot using Discord's application programming interface (API).
Before diving into the coding and setup process, it's crucial to understand the essential components of a Discord bot. A bot interacts with users in a Discord server, reacting to messages, commands, and events. Its functionalities depend heavily on how well it is coded and configured. This introductory section lays the foundation for an in-depth exploration of Discord bot creation, covering topics ranging from prerequisites to final deployment.
Beyond establishing a bot, this article will analyze key challenges one may face in development. Furthermore, it will highlight various coding resources that aid both novices and seasoned developers. With these insights, readers will be well-prepared to craft their own Discord bots that enhance their personal experience or serve larger community needs.
Equipped with the motivation to learn and a commitment to action, aspiring programmers will find both the structure they seek and the creativity necessary for customizing their bots. This guide therefore aims to be a valuable reference that provides clarity and practical knowledge throughout the development journey.
Coding Challenges
Coding can often present challenges. Encountering difficulties is a common part of the development process. Thus far, tackling small tasks known as coding challenges can greatly improve problem-solving skills and coding proficiency.
Weekly Coding Challenges
Consider participating in weekly coding challenges that can enhance your understanding of programming logic. Many platforms host coding contests that focus on different areas of programming, vital in honing the skills needed for building Discord bots.
Problem Solutions and Explanations
When confronted with coding problems, examining existing solutions can foster new insights. Platforms like Reddit and forums allow developers to share troubleshooting techniques, offering varying approaches to the same problem. It's helpful for readers to engage not only in learning solutions but also in understanding diiferent perspectives on problem-solving.
Tips and Strategies for Coding Challenges
When tackling coding challenges, here are a few recommendations:
- Break down problems into smaller sections.
- Incrementally test each part of your solution.
- Favor clear readability in code, as simple solutions often prevail.
- Embrace failure as a step in learning to foster resilience.
Community Participation Highlights
Finally, engaging with a friendly and motivated community can make the programming journey more enjoyable. Websites such as en.wikipedia.org offer tutorials and a wealth of knowledge, while forums on sites like reddit.com allow discussions ressembling real-time coding partnerships or mentorship.
Engagement with a developmental community can widen one’s learning horizons and foster significant growth.
In summary, the area of coding challenges is foundational. Throughout the bot creation process, one may face setbacks, but with support and resources, most obstacles can be overcome. Starting gradually enables programmers to build confidence and expertise, ushering them to incidentally mastering the complexities of Discord bot functionality.
Understanding Discord Bots
Discord bots are essential tools within the expansive ecosystem of Discord, a platform extensively utilized for community engagement and communication. Grasping the nuances of Discord bots forms the backbone of developing not only functional custom solutions but also rich user interactions that enhance the experience for Discord communities.
Definition and Purpose
A Discord bot serves as a software application designed to perform automated tasks on the Discord platform. In essence, these bots facilitate various functions, ranging from moderation to entertainment. They operate on commands dispatched by users, responding in real time, which enriches user experience and eases community management.
The primary purpose of employing a Discord bot is to improve engagement. For example, bots can curate discussions, provide information, or even run games that captivate users. Additionally, they streamline repetitive tasks, helping server admins manage members and activities efficiently. By automating processes, server owners can focus on strategizing their community's growth and fostering more interactive exchanges among users.
Common Use Cases
Discord bots find application in numerous scenarios. Here are key use cases:
- Moderation: Automated bots like Dyno help manage user behavior, provide warning alerts, and even mute or ban users who violate rules, contributing to a safer environment.
- Command and Control: Through basic command integrations, users can retrieve server information, make polls, or initiate games utilizing command structures predefined by bot developers.
- Entertainment & Community Engagement: Bots like Rhythm or FredBoat have made inroads for music sharing and group listening experiences, injecting enjoyment into member interaction.
- Notifications & Updates: Bots can be set to deliver updates from various external sources, such as GitHub repositories, ensuring that server members are consistently informed about relevant changes or events.
Understanding the expansive roles bots serve extends beyond technical significance; it shapes the future evolution of community interactions on platforms like Discord. The varied implementations of bots allow for customized solutions that elevate the modular capabilities of Discord itself, promising robust limitlessness in development possibilities.
This highlights how bots have become foundational, integrating innovation and community needs seamlessly.
Prerequisites for Bot Development
Developing a Discord bot is both rewarding and educational. But before diving into coding, it's crucial to understand the foundational requirements. This section outlines the basic prerequisites for bot development. Understanding these will enhance the overall experience and the effectiveness in creating a bot.
Basic Programming Knowledge
Having a solid grounding in programming is essential for anyone looking to create a Discord bot. At a minimum, familiarity with JavaScript is expected since the most commonly used libraries, such as Discord.js, use this language. Programming concepts, such as control structures, data types, and error handling, play a pivotal role in writing efficient code.
Knowing coding practices also aid significantly in debugging and troubleshooting, common issues developers face while building their bots. It's beneficial to look into the following areas:
- Syntax and Semantics: Understanding the basic structure and rules of JavaScript.
- Control Flow: Grasping concepts like loops and conditionals.
- Functions: Learning how functions work to reduce redundancy within your code.
Without these knowledge areas, coding effectively can become fundamentally challenging.
Familiarity with Discord
Before creating a bot, you should know what Discord is and how it operates. Discord is a powerful platform for community and team communications, incorporating text channels, voice calls, and streaming features. The more you know about how users engage with Discord, the better suited you will be in creating useful functionality for your bot.
- Understand the layout of servers, channels, and roles.
- Familiarity with Discord's user interface aids in visualizing which commands your bot should have.
- Recognize the culture of interactions on Discord. This can guide you in developing features that resonate with users,
Grasping how Discord functions will ultimately influence the choices you make as you create your bot.
Understanding Bot Permissions
Another critical aspect of developing a Discord bot is understanding the permissions model. The permissions determine what your bot can and cannot do within a Discord server. This plays a significant role, not just in functionality but also in accountability.
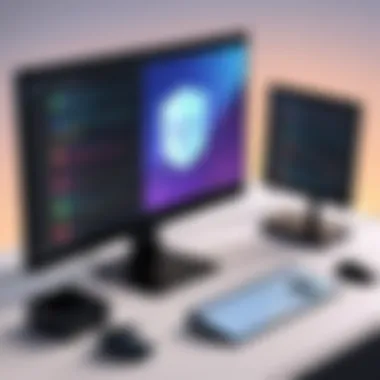
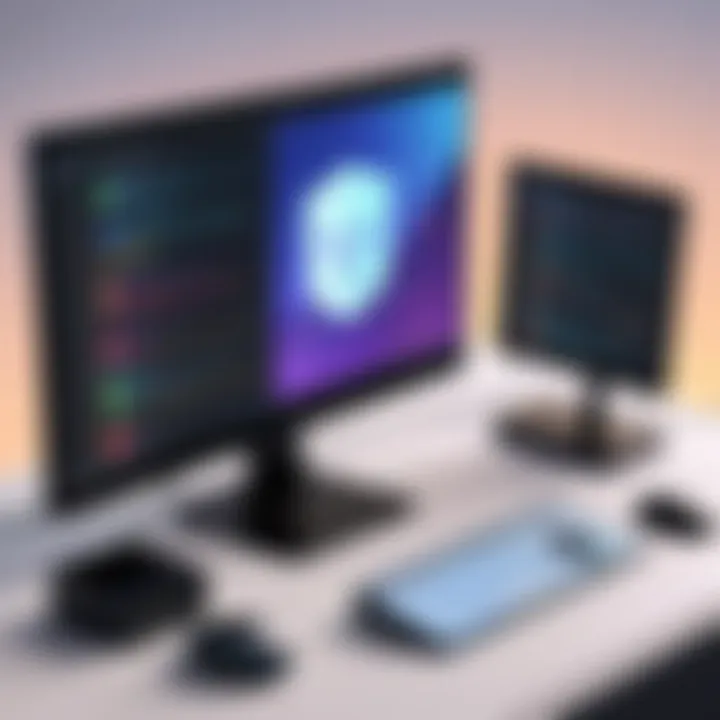
Permissions can include, but are not limited to:
- Read Messages: Required for viewing messages in text channels.
- Send Messages: Allows the bot to communicate within channels.
- Manage Roles: Grants capabilities to create, delete, and edit member roles.
To effectively create a functional bot, you must think about:
- What the bot needs access to for completing its tasks.
- The balance between functionality and security, ensuring not to grant excessive permissions.
Aligning your bot's permissions correctly can prevent both usability problems and potential security issues in your application.
Understanding these prerequisites sets the bedrock for developing a practical and functional Discord bot. The clearer your grasp on these elements, the smoother your journey will be throughout the development process.
Setting Up the Development Environment
Setting up the development environment is a foundational step in creating a basic Discord bot. This section elucidates the technical prerequisites for establishing a supportive framework that enhances your programming experience. A well-configured environment helps in mitigating challenges during coding and testing phases. Each of the components discussed here plays an essential role in simplifying the development process and streamlining the integration with Discord's API.
A robust development environment will aid both beginners and experienced programmers. It provides beneficial tools for maintaining organization in projects, debugging code, and employing necessary libraries. Ensuring that all components are correctly installed and configured can save valuable time and avoid hurdles later on.
Installing Node.
js
Node.js is a critical platform for building Discord bots due to its event-driven, non-blocking I/O model. This model makes it lightweight and, by extension, efficient. Installing Node.js can be broken into a few straightforward steps:
- Download the installer from the official Node.js website. Select the version that matches your operating system—Windows, macOS, or Linux.
- Run the installer and follow the prompts. Make sure to include the additional tools needed for a successful installation. This may help set the PATH environment variable, which is necessary for running Node.js from the command line.
- Verify the installation by opening your command line interface (CLI) and typing:This command should return the current version number of Node.js. If it does, the installation was successful.
With Node.js successfully installed, you can begin leveraging it to create your bot. The event-driven nature of Node.js makes it particularly suitable for real-time applications like those accessed through Discord.
Using Visual Studio Code
A code editor significantly enhances your development capabilities, and Visual Studio Code stands out in this regard. This editor is renowned for its features, including syntax highlighting, IntelliSense, and debugging capabilities. Setting up Visual Studio Code can enhance productivity as follows:
- Download and install Visual Studio Code from the official site. Follow the on-screen guidance to complete the installation.
- Open Visual Studio Code, and upon initial launch, you can customize key settings to your preferences. Adjusting your workspace for clarity can significantly streamline your development flows.
- Utilize extensions for Node.js development. Useful extensions facilitate the use of Git, ESLint, etc. Listing some beneficial extensions includes:
- Prettier: A code formatter that makes your code tidy.
- ESLint: Helps identify and fix problems in your JavaScript code.
Getting acquainted with Visual Studio Code will directly influence your efficiency and comfort in coding for your Discord bot. It presents an interface which supports productivity and code management.
Installing Necessary Packages
To enhance your bot's functionalities, certain packages are essential. In this case, Discord.js is a library that simplifies interactions with the Discord API. Here’s how to install the necessary packages:
- Open your terminal within Visual Studio Code. Ensure your working directory is the one where your bot project resides.
- Execute the npm command:This command retrieves the Discord.js library and installs it along with its dependencies, streamlining your coding efforts.
- In addition to Discord.js, consider incorporating additional packages based on your needs, such as:
- dotenv: For environment variable management, protecting your bot tokens.
- axios: To facilitate requests when integrating with APIs.
By setting up a thoroughly equipped development environment, you relinquish many potential pitfalls that come with bot programming. Following these structured steps puts you in a position of strength for creating a successful Discord bot.
Creating a Discord Bot
Creating a Discord bot opens up a realm of possibilities for interaction and automation on the platform. This section will discuss the key aspects involved in setting up your bot account and obtaining the necessary authentication token. It is vital to follow these steps correctly as they form the foundation of your bot's functionality.
Setting Up a Bot Account
Before coding anything, you need to set up a bot account on Discord. This account is distinct from a regular user account, meant for automated tasks.
- Visit the Discord Developer Portal: Go to the Discord Developer Portal at discord.com/developers/applications.
- Create a New Application: Click on “New Application” to begin the setup. Provide a clear name for your application, as this will be the identity of your bot.
- Create a Bot: In your application settings, locate the “Bot” tab and click “Add Bot.” Confirm the addition, and your bot will now have a unique identity.
- Set a Profile Picture and Username: It’s advisable to customize your bot by giving it an appropriate profile photo and username. While this is not strictly necessary, it helps in the identification process within your Discord community.
- Permissions and OAut Settings: To prepare for the next steps, review and set necessary permissions under the “OAut” section. Knowing what your bot can do is essential.
Setting up the bot account properly ensures that you avoid issues later in development. Remember to take it step by step to minimize errors. If you wish to make changes, you can always return to the Developer Portal.
Generating the Bot Token
The bot token is crucial. This unique string allows your bot to connect with the Discord API. Here’s how to generate your bot token securely:
- Navigate to the Bot Settings: In the same Developer Portal where you created your bot, find the “Bot” tab.
- Copy the Token: Under the “Token” section, you will see an option to reveal the token. Click on
Coding the Basic Bot
Creating a basic Discord bot has significant benefits for further developing your programming and technical skills. Proper coding techniques allow you not only to understand the Discord platform better but also to create functionalities that engage users effectively. This section covers the fundamental aspects of coding by connecting to the Discord API, implementing commands, and conducting local tests. These areas stimulate creativity, technical growth, and problem solving.
Connecting to Discord API
The Discord API is the foundational framework that allows a bot to function within servers. Understanding how to connect to this API is crucial for any developer. The process typically requires incorporating the library, which is vital for establishing this relationship with the Discord ecosystem.
To connect your bot, you start by installing via npm. The command is simple but essential:
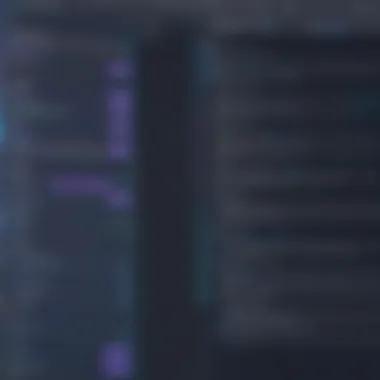
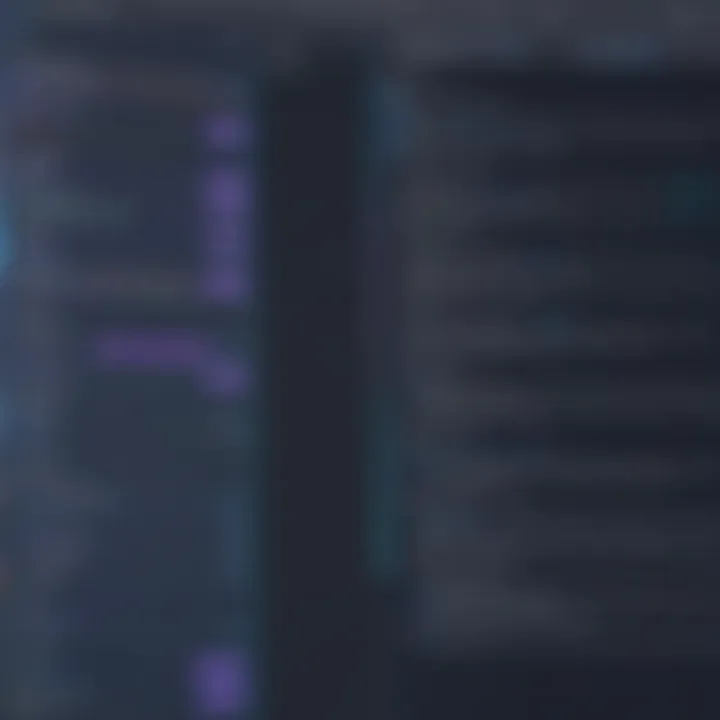
Then, in your main bot file, you will need to instantiate a Discord client. Here's a brief example:
Once the client is set, you must authenticate the bot with your token generated earlier. After that, the client can begin listening for events such as when it's ready to start working in your server. Ensure that proper error handling is in place. This will minimize issues during runtime.
Important: Always keep your bot token secure. Exposing the token can lead to account compromise.
Implementing Basic Commands
Commands give users a way to interact with your bot in an understandable manner. Essential in designing effective user experiences, implementing basic commands defines how users can engage with the functionalities you want your bot to offer.
Commands often take forms like text manipulation or remembrance features. Here is non-exhaustive list of how you can design commands:
- Messages triggered by particular words or phrases.
- Responses generated from user input, using command format like .
- Shortcuts to perform actions like welcome messages with an introductory phrase.
A simple example of creating a command might look like this:
By having distinct commands, you enable your bot to serve different functions fulfilling various user needs in the server context. Adopting well-structured command architecture simplifies future improvements and customization.
Testing the Bot Locally
Testing is an integrity check before deploying to public servers, ensuring functionalities behave as expected. Local testing allows abuse of repetitive execution cycles for fast iterations.
Often referred to as a sandbox environment, local testing commonly involves the Discord development mode. It allows you to inspect the workings of your bot without spending resources on a live server. Initiate your bot by simply executing the node command in your terminal:
Pay close attention to console outputs as those inform you about the bot's interaction and performance. Several key points to assess might include:
- Response accuracy to commands.
- Stability and performance under simulated load.
By carrying thorough local tests, you reduce issues once the bot gets deployed to a live environment, providing a smoother experience for users.
Thus, coding the basic bot encompasses several critical tasks. Engaging with the API, implementing commands, and testing locally ensures a robust and functional Discord bot. Focus on these elements will enhance both the efficiency of your bot and your skills as a developer. Recall that each step, no matter how minor, lays the groundwork for complicated future advancements.
Deploying Your Bot
Deploying your bot is a critical phase in the process of creating a Discord bot. It involves moving your locally developed code to a live environment where it can be accessed by others on Discord. The deployment process ensures that your bot can run continuously and interact with users globally. Before going live, consider various factors like uptime, scaling, and reliability to ensure your bot remains accessible. A well-deployed bot enhances user experience, encouraging engagement and robust performance.
Choosing a Hosting Platform
Choosing the right hosting platform can significantly impact your bot’s performance and availability. Some common options include cloud services like DigitalOcean, AWS, or platforms specifically designed for developers like Replit and Heroku. Each has its unique strengths, enabling different scales and features.
- Cost Efficiency: Assess the costs associated with running a bot. Some platforms offer free tiers, which can help minimize initial expenses when testing.
- Ease of Use: Select a platform with user-friendly setups if you are new to deployments.
- Support for Node.js: Most Discord bots use Node.js, so the platform should support it well.
It is vital to review these elements and select a solution that best fits your needs and potential future scaling of your bot.
Deploying with Heroku
Heroku is a popular choice for deploying Discord bots due to its straightforward deployment process and free tier availability. To deploy your bot on Heroku, follow these basic steps:
- Set Up Your Heroku Account: Create a free account on Heroku and install the Heroku Command Line Interface (CLI).
- Prepare Your Project: Ensure your project files are ready, including a which tells Heroku how to run your bot.
- Push Your Code: Use Git to commit your current code and push it to Heroku. This commands deploy your project into the Heroku environment and start your bot.
- Set Config Vars: Retrieve your Discord Bot Token and other environment variables required. Set them in the Heroku dashboard under your app settings to keep them secure.
After deployment, your bot will run as defined in your scripts and will be operational for public use.
Monitoring and Maintenance
Once your bot is deployed, monitoring it ensures it operates as intended. You can encounter issues such as downtime, bugs, or user feedback glitches. Staying proactive in maintenance is important.
- Log with Heroku Dashboard: Heroku offers real-time logging. This allows you to view and analyze logs that can help with quick diagnoses of issues.
- Regular Tests: Making regular tests necessary ensures your bot works fine with the Discord API and reacts to commands accurately.
- User Feedback: Encourage users to report any bugs or suggest improvements. Sometimes direct user experiences reveal insights that monitoring tools can miss.
Being diligent about monitoring and adjusting your bot assists in handling any issues quickly and maintaining a high-quality experience for all users. Staying committed to this practice is essential for keeping your bot performing well.
Advanced Features and Customization
Advanced features and customization in Discord bot development are crucial. They empower creators to tailor their bots to specific functionality that meets user demands. In this section, we will explore vital elements like adding commands, integrating APIs, and creating interactive features. These aspects enhance the overarching user experience and allow the bot to perform a wider range of tasks. Such versatility is not merely advantageous; it is essential for bots intending to maintain engagement over time.
Adding More Commands
Commands are the primary way users interact with a bot. Expanding the command set enriches user experience. Bot developers should consider the needs of their audience. Incorporating need-based commands will make the bot more relevant and helpful. This customization essentially transforms the bot into a tailored tool that serves its community.
To implement additional commands, developers can modify their code similar to how the initial command structures were formed. Here is a brief overview of steps:
- Define the new command in your code.
- Use suitable triggers, such as specific keywords or phrases that users might expect.
- Ensure your command does not duplicate existing functionalities, as this may confuse users.
- Test each command to confirm seamless operation.
By taking these steps, users may find customized commands such as , which lists bot functionalities, enabling ease of use.
Integrating APIs
Integrating external APIs significantly enhances a bot's utility. APIs can fetch real-time data from various platforms. This feature adds depth to your Discord bot and allows it to offer valuable information. An example could be fetching weather data or serving game stats.
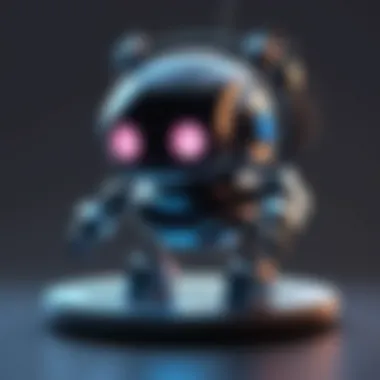
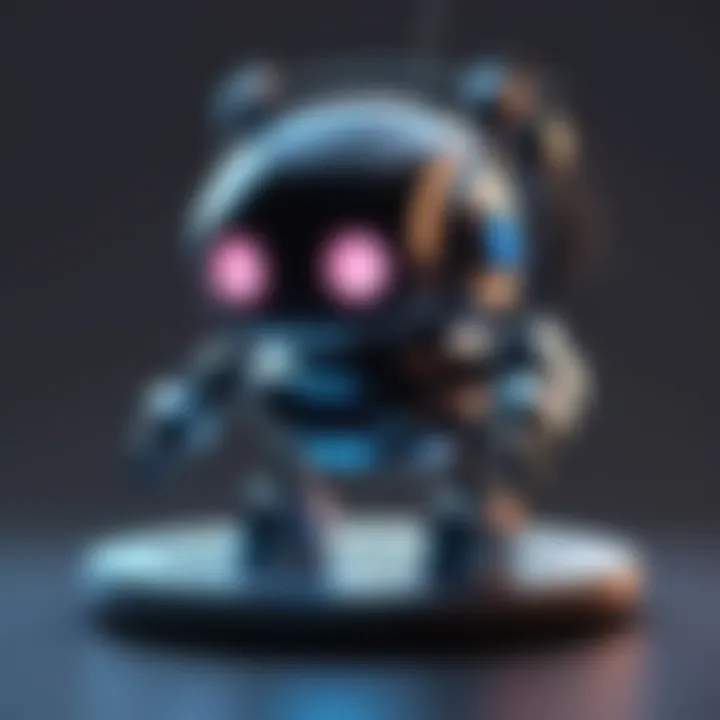
Here are the key considerations when integrating an API:
- Understanding API Documentation: Knowledge of API functions is crucial for effective implementation. Most APIs will have comprehensive documentation outlining how to make requests and handle responses.
- Data Handling: Ensure your bot can manage the data format returned by the API. Most APIs return JSON, and your bot should parse this information accurately.
- Rate Limiting: Many APIs impose limitations on requests. Consequently, your bot should handle limit errors gracefully, ensuring user expectations are managed.
By exploiting APIs, the bot becomes a bridge to external knowledge and authority on many topics.
Creating Interactive Features
Consumers increasingly seek interactive experiences. This trend applies particularly in gaming and social environments like Discord. Implementing interactive features encourages greater user engagement. Features may include game integrations, polls, or user feedback forms. Such implementations invite user feedback, improving user relations.
Consider these methods for building interactive capabilities:
- Using React Components: You can embed user choices with buttons, dropdown menus, etc. This allows users to respond without typing.
- Custom Responses: According to user input, program dynamic responses. This feature gives a lively interaction feeling.
- Incorporating Levels and Rewards: Use gamification strategies to encourage participation. Users can earn rewards by interacting with your bot frequently.
As your bot becomes more tailored through advanced features and customization, it attracts a more dedicated user base. New users find a robust and responsive Discord bot while experienced users appreciate the depth and range of functionality provided. This refinement is key in ensuring that the bot remains relevant and retains user interest over time.
Innovatively creating advanced features hinges on understanding user needs and properly integrating relevant technology. With continuous improvement and refinement, you can design an engaging bot that stands out in the crowded Discord landscape.
Troubleshooting Common Issues
Creating a Discord bot can involve encountering various issues that may hinder its performance. The ability to troubleshoot these common problems is an essential skill for both novice and seasoned developers. Troubleshooting helps pinpoint the root cause of errors, saving time and preventing further complications. Understanding these common issues equips developers with the tools to optimize their bots effectively.
Debugging Bot Errors
Debugging is a crucial part of bot development. Bot errors can manifest in several ways, including unresponsive commands or unexpected behaviors. Effective debugging can clarify what went wrong during the coding phase. Here are key strategies to debug your bot:
- Check Error Logs: Review log files for error messages that can give clues about the issue. The console output often reveals where in the code things are failing.
- Use Print Statements: Inserting print statements at various points in your code can help track the flow of execution. You can see if certain parts of the code are being reached.
- Isolate Code: Try to isolate the problematic section of code. Test it independently to discern if it works as intended.
- Utilizing Debuggers: Debugging tools available in IDEs like Visual Studio Code can make following the code much easier. Set breakpoints to halt execution and inspect variable values in real time.
- Collaborate: When in doubt, discuss the issue with other developers. Platforms like Reddit group communities possess knowledgeable members who offer assistance or ideas.
By consistently applying these strategies, the debugging process feels less daunting and more manageable.
Resolving Permission Issues
Permission issues are a common roadblock in Discord bot development. Discord's permission settings are designed to protect the community, and correctly configuring these permissions is crucial for bot functionality. Understanding permissions commonly resolves malfunctioning commands or unexpected failures in features. Here are some points to consider:
- Understanding Permission Roles: Each user can have different roles with varying permission levels. Ensure the bot has the required roles to execute relevant tasks in a server.
- Bot Permissions: When setting up a bot account, verify the permissions initializing your bot needs. Double-check the scope you gave when inviting the bot to servers to include all required permissions.
- Adjust Server Settings: Analyze the bots' roles in your Discord server settings as those can affect how the bot functions. Roles positioned higher in the hierarchy can override others.
- Testing Privileges: Conduct tests in controlled environments where you can check limits on commands as a regular user and the bot itself. Doing so will reveal if the bot operates correctly under given restrictions.
- Modify Extra Permissions if Necessary: If specific commands don’t work, retrace steps to grant the bot administration privileges or additional privileges where required.
By methodically reviewing and adjusting these permissions, functionality issues typically resolve quickly and can enhance the bot operation overall. It saves both time and maintains healthy interactions within Discord workflows.
Exploring Social Features
Exploring social features within a Discord bot is critical for facilitating user interaction and enhancing engagement. Ideally, the bot should not just serve a functional purpose but also foster community building and user satisfaction. In this section, we will examine several valuable components of social features that contribute to a streamlined user experience on Discord.
Implementing Moderation Features
Moderation features empower bots to maintain a positive environment. Issues like spam, bullying, and inappropriate content can disrupt community harmony. Bots with effective moderation tools can help manage these matters more efficiently than manual efforts. Some common moderation tasks include:
- Automatically deleting spam messages.
- Temporarily or permanently banning repeat offenders.
- Setting up filtered content.
- Logging moderation actions for transparency.
By implementing these features, you not only protect the community but also instill a sense of safety among users. This capability is increasingly relevant, especially in larger servers where human moderation becomes challenging.
Engaging with Users
Engagement should be at the forefront of any successful Discord bot. Users seek not only functionality but a connection to the bot and community. Several approaches include:
- Real-time interactions: Leveraging commands that trigger immediate responses enhances user involvement. Examples are reaction roles or polls.
- Community events: Bots can organize events, giveaways, or contests, thereby motivating participation. These functions create excitement and a sense of urgency.
- Personalization options: Allowing users to customize their experience can increase interaction rates as users feel a stronger tie to the environment.
Benefits of Engagement
Engagement extends beyond enjoyment. When users feel involved, they're more likely to contribute positively to the community. A bot that promotes fun interactions lowers the barriers for new users. Aside from ensuring the bot has an immediate role in daily communications, engagement gears towards long-term cultivation of a loyal user base.
Engaging effectively leads to a sense of belonging, something crucial for thriving online communities.
The consideration of social features and their implementation directly influences the appeal and usability of your bot, shaping the community in important ways.
Epilogue and Next Steps
In the concluding segment of this guide, it is essential to reflect on the substantial knowledge gained throughout the article. Understanding the journey of bot development—from setting up the environment to deploying your bot—serves as a foundational block for deeper exploration into the realm of programming and automation. Each step provides not just functional skills, but also instills confidence and curiosity to venture into more complex areas.
With that knowledge, users can progress further into particular facets of Discord bot creation. This may include exploring advanced functionalities, optimization techniques, or diversifying the application of their bot across different server environments.
Considerations for the next steps should focus on ongoing learning. The world of technology changes rapidly. Staying updated on programming languages, new tools, and community best practices ensures that the bots remain relevant and efficient in operation. For creating dynamic engagements in Discord, consistently enhancing your bot’s capabilities is critical. Details imparted throughout this guide enable readers to own the process of customizing and iterating their bots.
“Knowledge accumulates over time, serving as both a toolkit and playground. Engage with it continuously.”
Recap of Key Points
- Understanding the Role of a Bot: Grasp the fundamental nature and common purpose that drives the need for a Discord bot.
- Setting Up the Basics: Follow the correct procedures for initial setup, ensuring the environment is conducive to seamless development.
- Programming Concepts: Familiarity with core programming principles is centralized, as they shape the command structures within the bot.
- Deployment Processes: Recognize the critical steps in taking a bot from a local environment to a live Discord environment.
- Troubleshooting Methods: Gain insights into resolving common errors that can arise during development, a key skill for maintaining bot integrity.
Incorporating these elements significantly empowers anyone embarking on this technological venture. Each key aspect supports an interconnected understanding of the broader landscape of Discord bots.
Resources for Further Learning
Continuous learning is crucial as you advance in your bot development journey. Here are some valuable resources to enrich your knowledge:
Moreover, consider exploring documentation provided directly by Discord for insights that are frequently updated and hold significant practical implications. Various programming communities also host discussions and problem-solving threads which can foster skill growth. Engaging in these platforms can cultivate not just expertise but also connections with like-minded individuals.
Equipping yourself with these resources and knowledge will empower your evolution in the world of Discord bot creation.