Complete Guide to Building a Basic React App from Scratch
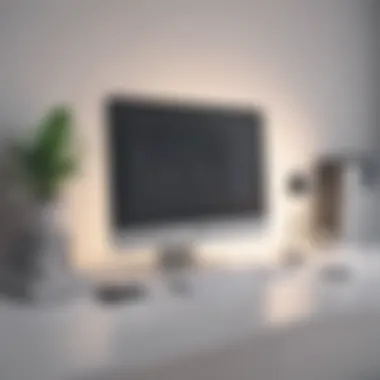
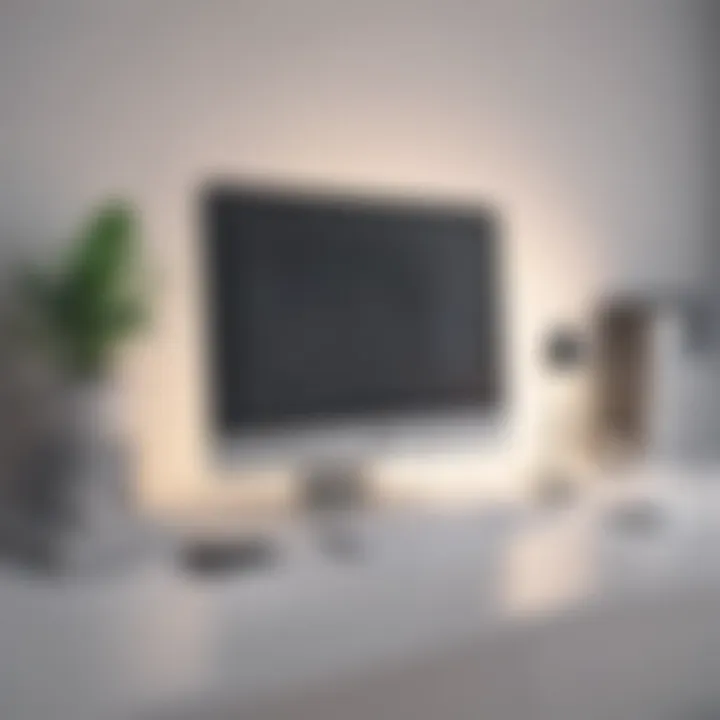
Coding Challenges
When delving into the realm of creating a simple React app, it's crucial to understand the coding challenges that may arise. One aspect to consider is the weekly coding challenges that can sharpen your problem-solving skills. By participating in these challenges, you expose yourself to diverse coding scenarios, enabling you to think critically and innovatively. Moreover, the solutions and explanations provided in these challenges serve as valuable learning tools, giving you insights into efficient coding practices. To excel in coding challenges, adopting tips and strategies tailored for overcoming common hurdles is imperative. These strategies can range from time management techniques to debugging approaches. Community participation also plays a vital role in enhancing your coding proficiency. By engaging with fellow developers, sharing solutions, and discussing coding concepts, you foster a collaborative learning environment that can significantly boost your programming abilities. Technology Trends In the ever-evolving landscape of technology, staying updated on the latest trends is essential for creating a successful React application. From the newest technological innovations driving the industry forward to emerging technologies poised to shape the future, being aware of these trends offers valuable insights for your development journey. Understanding the impact of technology on society provides a broader perspective on the implications of your coding choices. Expert opinions and analysis on current tech trends can offer guidance on leveraging advancements in your React projects. By aligning your development approach with cutting-edge trends, you position yourself to create innovative and impactful applications. Coding Resources Navigating the vast array of coding resources is integral to mastering the art of building a simple React app. Delving into programming language guides equips you with the knowledge needed to write efficient and clean code. Exploring various tools and software can streamline your development process, enhancing productivity and code quality. Tutorials and how-to articles serve as educational assets, guiding you through complex React concepts and best practices. Additionally, comparing online learning platforms allows you to choose the most effective resources for enhancing your React skills. By leveraging diverse coding resources, you can elevate your proficiency and broaden your understanding of React development. Computer Science Concepts Understanding fundamental computer science concepts is key to excelling in React app creation. Immersing yourself in algorithms and data structures primers hones your problem-solving abilities and enhances the efficiency of your code. Exploring artificial intelligence and machine learning basics opens up possibilities for integrating advanced functionalities into your React projects. Moreover, grasping networking and security fundamentals ensures the robustness and reliability of your applications. Looking ahead to future technologies like quantum computing provides insights into potential advancements that can revolutionize the field. By delving into various computer science concepts, you solidify your foundation in React development and pave the way for innovative and cutting-edge applications.
Introduction to React
In this section, we will embark on a journey through the foundational aspects of React, a popular JavaScript library for building user interfaces. Understanding React is crucial as it forms the basis of our exploration into creating a simple React app. By grasping the core principles and concepts of React, such as the Virtual DOM and Component-Based Architecture, developers can effectively leverage React's capabilities to enhance their applications.
Understanding React
Key Concepts of React
Delving into the Key Concepts of React sheds light on the fundamental principles that underpin this powerful library. React emphasizes the concept of reusable components, encapsulating both structure and logic within individual pieces that can be easily composed and reused throughout an application. This component-centric approach streamlines development, promotes code reusability, and enhances maintainability. Additionally, React's Virtual DOM implementation enables efficient rendering by minimizing actual DOM manipulations.
Virtual DOM
The Virtual DOM mechanism stands out as a hallmark feature of React, distinguishing it from traditional DOM-manipulating libraries. By maintaining a virtual representation of the actual DOM in memory, React optimizes updates by calculating the difference between the virtual and real DOM, leading to minimal re-renders and improved performance. This innovative approach boosts application efficiency and responsiveness, especially in complex UIs with dynamic data updates.
Component-Based Architecture
React's Component-Based Architecture promotes the construction of applications as a tree of modular, self-contained components. Each component encapsulates specific functionality and can be easily composed and nested within other components, fostering a modular and organized codebase. This approach enhances code maintainability, facilitates collaboration among developers, and supports scalability by allowing the incremental addition of new features without affecting existing functionality.
Benefits of Using React
Efficient Performance
The efficient performance of React stems from its Virtual DOM reconciliation process, which minimizes DOM updates to only what is necessary. By selectively rendering components based on state changes, React ensures optimal performance by reducing redundant operations, resulting in snappy and responsive user interfaces. This streamlined approach to rendering makes React a preferred choice for developing high-performance web applications.
Reusable Components
React's emphasis on reusable components revolutionizes the way developers conceptualize and build interfaces. By breaking down user interfaces into self-contained building blocks, developers can create versatile, easily maintainable components that can be reused across multiple parts of an application. This reusability simplifies development workflows, accelerates project timelines, and fosters a consistent design language throughout the application.
Declarative Syntax
React's declarative syntax streamlines the process of defining and updating UI components by abstracting away the manual DOM manipulation common in traditional frontend development. By describing how the UI should look based on underlying data changes, developers can focus on the application's logic and let React efficiently handle the rendering process. This declarative approach enhances code readability, reduces programming errors, and promotes a more intuitive development experience.
Setting Up Your Development Environment
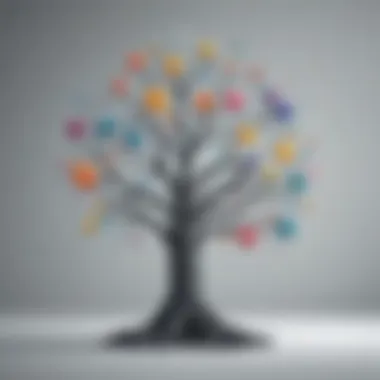
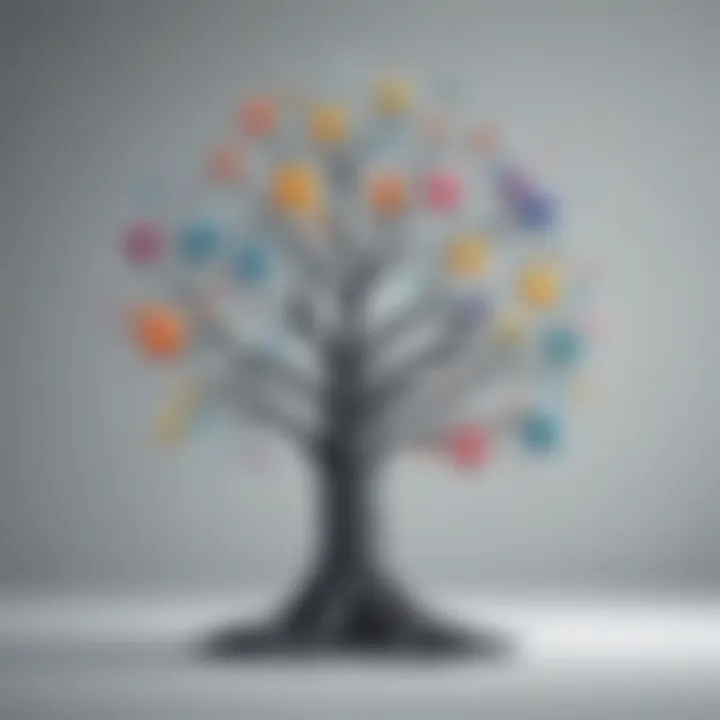
Setting up your development environment is a crucial step in the process of creating a simple React app. It lays the foundation for smooth and efficient app development, ensuring that all necessary tools and dependencies are in place. Understanding the importance of this topic is key to the success of your React project. By setting up a conducive development environment, you set yourself up for streamlined coding, testing, and deployment processes.
Installing Node.js and NPM
Node.js Installation
Installing Node.js is a fundamental aspect of setting up your development environment for React. Node.js provides a runtime environment to execute JavaScript code outside a web browser. This installation is vital as it allows you to leverage npm, the package manager for Node.js, to install various packages and dependencies required for your React project. Node.js offers high performance and scalability, making it a popular choice among developers. Its ability to manage asynchronous operations efficiently enhances the overall development experience. However, some may find the steep learning curve of Node.js challenging at first.
NPM Installation
NPM installation complements Node.js by serving as a central repository for JavaScript packages. It simplifies package installation, version management, and dependency resolution for your React project. NPM's modular approach allows you to reuse code easily, enhancing the efficiency of your development process. By utilizing NPM, you can access a vast ecosystem of open-source packages, streamlining the integration of external functionalities into your React application. Despite its advantages, NPM's reliance on network connectivity for package retrieval may pose challenges in offline environments.
Creating a New React Project
Using Create-React-App
Creating a new React project using Create-React-App offers a quick and convenient way to set up a React environment with minimal configuration. This tool automates the setup process, simplifying the initial steps involved in creating a React application. Its out-of-the-box configurations eliminate the need for manual setup, allowing you to focus on coding and development tasks promptly. Create-React-App's intuitive interface and seamless integration with popular build tools enhance the developer's productivity. However, customization options may be limited, requiring additional configurations for specific project requirements.
Project Structure Overview
Understanding the project structure is essential for maintaining a well-organized React application. A clear project structure facilitates code navigation, debugging, and collaboration among team members. By providing an overview of the project's layout, developers can identify key directories, files, and modules consistently. This structured approach fosters code clarity and maintainability, simplifying future updates and enhancements. Despite its benefits, an overly complex project structure may increase cognitive load, affecting development efficiency.
Building Your First React Component
In this pivotal section of the article, we delve into the fundamental aspects of crafting your initial React component. Understanding the process of building a React component is crucial for every developer venturing into React development. By comprehensively explaining the steps involved in creating a React component, this section serves as the bedrock for mastering React application building.
Creating Functional Components
JSX Syntax
An essential element within React development is JSX Syntax. JSX, a JavaScript extension, enables developers to write HTML elements within JavaScript code effortlessly. Its efficient integration of HTML-like syntax within JavaScript streamlines the process of building React components, enhancing code readability and maintainability. The simplicity and familiarity of JSX Syntax make it a preferred choice for React developers, offering a seamless transition from traditional web development. However, the opinion is divided on JSX Syntax, with some developers citing concerns over mixing HTML and JavaScript, which could potentially lead to bloated code.
Props and State Management
Props and State Management play a pivotal role in React development by facilitating the exchange of data between components and maintaining component state. Props enable the passing of data from parent components to child components, fostering component reusability and modular design. On the other hand, state management empowers components to manage their internal state efficiently, ensuring dynamic content rendering and responsive user interfaces. The structured approach offered by Props and State Management enhances code organization and overall application performance. Nonetheless, complexities may arise in managing state across multiple components, necessitating careful consideration of component hierarchy and data flow.
Implementing Class Components
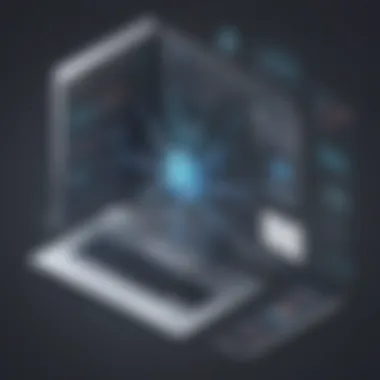
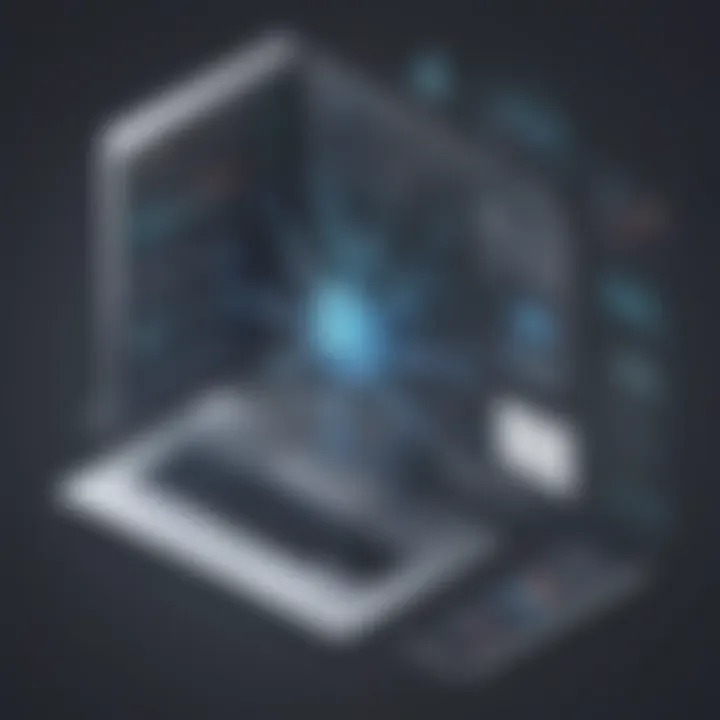
Lifecycle Methods
The implementation of Lifecycle Methods within React Class Components is vital for controlling the behavior of components throughout their lifecycle. These methods, such as componentDidMount and componentWillUnmount, allow developers to execute code at specific stages of a component's life, enabling tasks like data fetching and DOM manipulation. Lifecycle Methods offer a structured approach to managing component behavior, ensuring optimal performance and resource utilization. However, the reliance on Class Components and Lifecycle Methods may introduce complexities in code maintenance and readability, prompting the industry shift towards functional components and hooks.
State Management in Class Components
Effective State Management within Class Components is essential for creating interactive and dynamic user interfaces in React applications. By leveraging component state, developers can trigger re-renders, update component appearance based on user interactions, and maintain state consistency across multiple components. The encapsulation of state within class components promotes data integrity and enables the creation of sophisticated user experiences. Nonetheless, the class-based approach to state management may result in verbose code and nested component structures, potentially hindering codebase scalability and maintenance.
Styling Your React App
Styling plays a crucial role in enhancing the visual appeal and user experience of a React application. In this section, we will focus on the various aspects of styling within a React project, exploring different techniques to make your app visually compelling and user-friendly. Effective styling not only makes your app aesthetically pleasing but also contributes to better user engagement and overall satisfaction. By understanding and implementing proper styling practices, you can elevate the quality of your React app and create a polished final product that stands out.
Utilizing CSS Modules
CSS Modules offer a structured approach to styling in React, allowing you to encapsulate styles within individual components. This prevents style conflicts and makes your code more modular and maintainable. Scoped Styles, a key feature of CSS Modules, enable you to apply styling only to specific components without affecting other parts of the application. Scoped Styles help in creating truly reusable and isolated styles for each component, reducing the chances of unintended style overrides or leaks. This aspect of CSS Modules enhances code organization and promotes a cleaner development process.
Scoped Styles
Scoped Styles provide a scoped environment for CSS classes, ensuring that styles defined within a component stay local to that component. This prevents global style pollution and eliminates the need for verbose class naming conventions. With Scoped Styles, you can write CSS without worrying about naming collisions or interfering with other parts of your application. This localized approach to styling enhances code readability, simplifies maintenance, and fosters better collaboration among team members working on different components. Additionally, Scoped Styles facilitate better encapsulation of styles, promoting reusability and component-specific styling without external dependencies.
Class Composition
Class Composition involves combining multiple CSS classes to style a single element, offering flexibility and reusability in styling components. By composing classes, you can create complex styling patterns without increasing specificity or crowding your code with redundant styles. This approach promotes a modular and scalable styling architecture, allowing you to mix and match style rules to achieve the desired visual presentation. Class Composition enhances code reusability, simplifies style modifications, and encourages a more efficient styling workflow. Incorporating Class Composition in your React app enables you to create versatile and customizable styles that cater to diverse design requirements.
Exploring CSS-in-JS Libraries
CSS-in-JS libraries bring a different paradigm to styling in React, offering solutions that embed styles within JavaScript. This approach provides the benefits of dynamic styling, component-based encapsulation, and enhanced developer experience. By leveraging CSS-in-JS libraries like Styled Components and Emotion, you can seamlessly integrate styling logic with your component logic, resulting in more cohesive and maintainable codebases. These libraries offer unique features that simplify styling management and empower developers to create visually stunning and performant React applications.
Styled Components
Styled Components revolutionize the way styles are applied in React, introducing a component-based styling approach where styles are defined directly within the component file. This allows for better component encapsulation, improved readability, and logical organization of styles based on component hierarchy. The ability to use standard CSS alongside JavaScript conventions makes Styled Components a versatile choice for developers seeking a more structured and reusable styling solution. With Styled Components, you can effortlessly create and maintain consistent styles across your application, enhancing code maintainability and promoting a more streamlined styling process.
Emotion
Emotion offers another powerful CSS-in-JS solution for styling React components, emphasizing performance, flexibility, and developer experience. Emotion provides advanced features like CSS prop, theming, and server-side rendering support, enhancing the capabilities of styling in React applications. By adopting Emotion, developers can leverage its efficient CSS rendering, concise syntax, and built-in optimizations to deliver high-quality user interfaces with minimal effort. The unique feature set of Emotion empowers developers to streamline the styling workflow, ensure robust design consistency, and achieve optimal rendering performance in their React projects.
Adding Interactivity with React
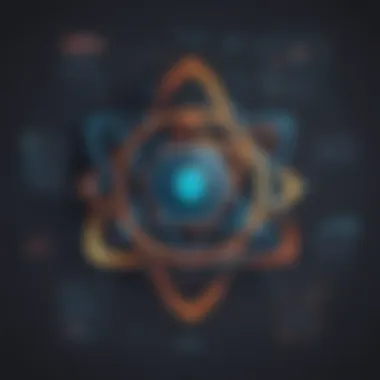
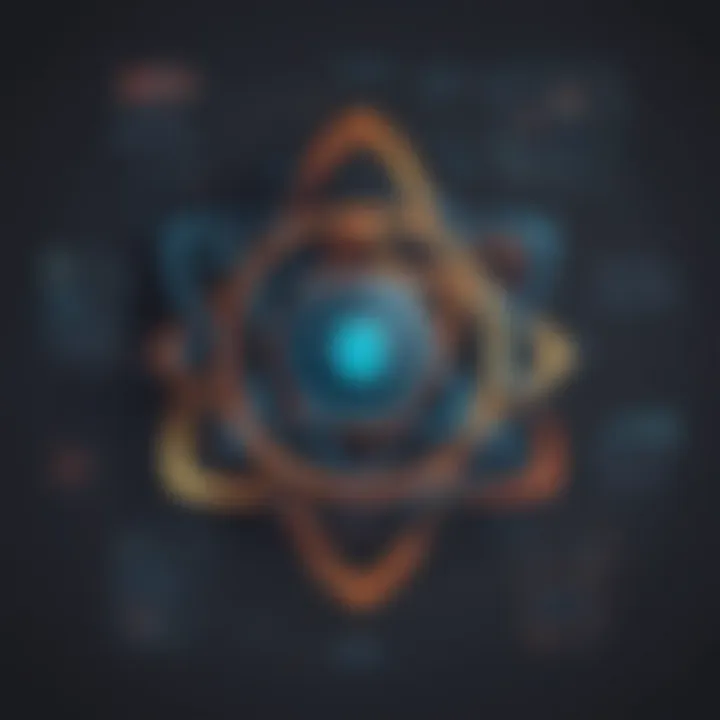
In the intricate realm of React development, adding interactivity lies at the core of creating engaging and dynamic user interfaces. This section elucidates the critical role of incorporating interactive elements using React, amplifying the user experience and functionality of web applications. By enabling users to interact seamlessly with the application, React's interactivity functions as a cornerstone for crafting intuitive and responsive interfaces. Understanding the nuances of handling events and managing state within a React application is imperative for achieving a streamlined and user-centric design.
Handling Events
Event Binding
Event binding in React signifies the process of linking user actions to corresponding event handlers, facilitating the execution of specific functions upon user interaction. Event binding is fundamental for establishing the interactivity of components, enabling developers to respond efficiently to user input. The efficiency of event binding translates to enhanced user experience and dynamic functionality, contributing significantly to the overall fluidity of the application. A notable characteristic of event binding is its ability to encapsulate user actions within defined event handlers, streamlining the code structure and enhancing code readability. While event binding streamlines the process of managing user interactions, careful consideration of event delegation and performance optimization is essential to ensure seamless functionality.
Event Handlers
Event handlers in React serve as the bridge between user actions and application behavior, dictating the response triggered by specific events. Event handlers play a pivotal role in orchestrating the interactivity of components, offering developers precise control over the behavior of the application in response to user input. The key characteristic of event handlers lies in their versatility and adaptability, providing developers with the flexibility to define custom behaviors for diverse user interactions. Leveraging event handlers empowers developers to create dynamic and responsive interfaces, elevating the user experience to a more interactive and engaging level. Although event handlers enhance the functionality of React applications, meticulous attention to event propagation and event delegation is imperative to maintain the integrity and performance of the application.
State Management in React
useState Hook
The useState hook in React revolutionizes state management by introducing a simplistic yet robust mechanism for handling component-level state within functional components. This fundamental aspect of React enables components to maintain internal state effortlessly, facilitating dynamic updates and re-rendering based on state changes. The crux of the useState hook's appeal lies in its intuitive syntax and seamless integration, empowering developers to manage state effectively without the complexities associated with class components. An advantageous aspect of the useState hook is its lightweight nature, minimizing boilerplate code while enhancing the readability and maintainability of the codebase. However, prudent utilization of the useState hook is essential to prevent state cluttering and optimize performance.
Context API
The Context API in React offers a sophisticated solution for state management across multiple components, streamlining the propagation of state data without prop drilling. This advanced feature of React allows developers to create a centralized state container that can be accessed by nested components, obviating the need to pass props down the component hierarchy. The key characteristic of the Context API lies in its capacity to enhance component reusability and maintain clean component architecture, fostering a more structured and scalable codebase. Leveraging the Context API empowers developers to optimize state management in complex applications, mitigating the challenges associated with prop drilling and state synchronization. However, strategic implementation of the Context API is crucial to maintain code clarity and ensure efficient state propagation throughout the application.
Optimizing and Deploying Your React App
In the realm of React development, Optimizing and Deploying Your React App stands as a pivotal phase, essential for delivering a seamless and efficient user experience. Optimizing a React app involves fine-tuning its performance to ensure swift loading times and responsiveness, crucial aspects in today's fast-paced digital landscape. Deploying the React application is equally significant as it involves making the app accessible to users worldwide through hosting on servers or platforms.
Performance Optimization Techniques
Code Splitting
Delving into the intricacies of Code Splitting, one uncovers a powerful technique that enhances the optimization of React applications. Code Splitting entails breaking down the application's codebase into smaller chunks, allowing for selective loading based on user interactions. This method significantly reduces initial load times, as only essential code segments are fetched initially, enhancing the app's overall performance. Code Splitting is a popular choice for developers looking to streamline their apps and improve user experience, especially in scenarios where large libraries or components are involved. Despite its advantages, developers need to carefully implement Code Splitting to avoid fragmentation issues and ensure a coherent user experience.
Lazy Loading
In the landscape of React optimization, Lazy Loading emerges as a valuable strategy to boost performance and minimize initial loading times. Lazy Loading involves deferring the loading of non-critical resources or components until they are required, thereby optimizing the app's speed and resource efficiency. By implementing Lazy Loading, developers can provide users with a faster initial load time, promoting a seamless browsing experience. This approach is particularly beneficial for applications with vast content or a multitude of features, as it ensures that only necessary resources are loaded initially, reducing unnecessary delays and enhancing user satisfaction.
Deployment Strategies
Hosting Options
When considering Hosting Options for your React app, the decision plays a crucial role in determining the application's accessibility, speed, and reliability. Choosing the right hosting service is vital to ensure optimal performance and user experience. Different hosting providers offer varied features such as scalability, security, and support, influencing the app's overall efficiency. Selecting a hosting option that aligns with the app's requirements and expected traffic volume is essential to guarantee smooth operation and responsiveness.
Deploying to Various Platforms
The process of Deploying to Various Platforms extends the reach of a React application, enabling it to be accessed across multiple devices and environments. By deploying the app to diverse platforms, developers can cater to a broader user base and enhance the app's visibility and impact. Deploying to various platforms demands consideration of compatibility, scalability, and performance optimization to ensure a consistent user experience across different devices and browsers. This approach enhances the app's versatility and accessibility, empowering users to engage with the application seamlessly, regardless of their chosen platform or device.