Mastering Data Cleaning with Python Pandas Techniques
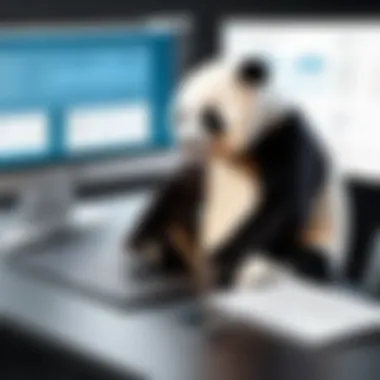
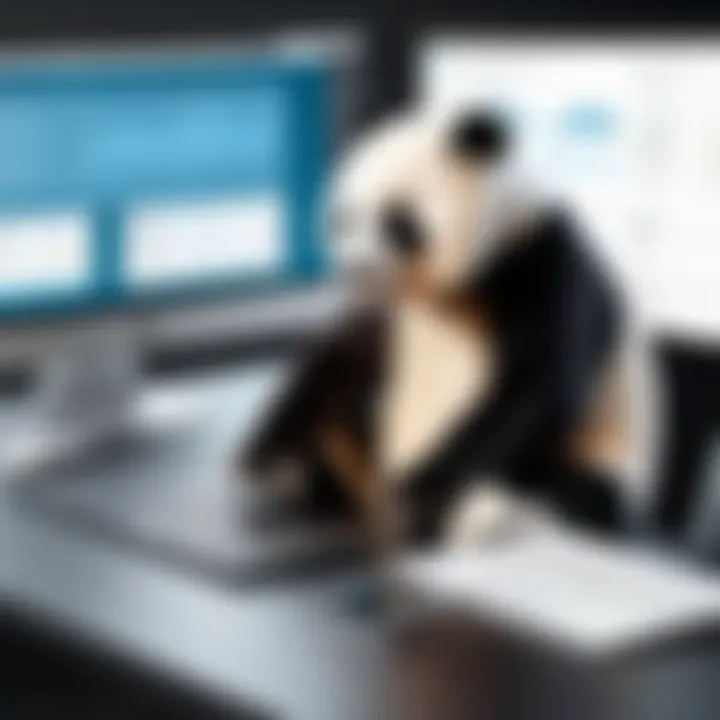
Intro
Data cleaning is a crucial step in data analysis. Inaccurate data can lead to misleading insights, making it essential to ensure the quality and integrity of datasets before analysis. Python's Pandas library stands out for its powerful capabilities in handling various data cleaning tasks. Whether you're dealing with missing values, duplicates, or incorrect data types, Pandas provides tools that streamline the process efficiently.
Python Pandas is commonly used by programmers and data scientists alike. Its functionalities allow for rapid data manipulation without requiring extensive programming experience. This article will explore a range of techniques within the Pandas library that facilitate data cleaning. We will cover basic functions and delve deeper into advanced techniques that every data professional should know.
Through practical examples, we will illustrate how to handle common data issues and provide best practices for maintaining data integrity. By the end of this article, readers should feel confident in using the Pandas library for effective data cleaning and preparation.
Prelude to Data Cleaning
Data cleaning is a foundational step in the data analysis process. It involves the identification and correction of errors and inconsistencies in datasets. Recognizing the need for data cleaning is crucial; without it, analysts may draw inaccurate conclusions. This can lead to poor decision-making and a misallocation of resources.
The importance of data cleaning cannot be overstated. First, clean data ensures the validity of the results derived from analyses. Errors in the data can skew results, leading to potentially harmful misinterpretations. Second, it enhances the efficiency of the analytic processes. When datasets are free from unnecessary clutter and inaccuracies, computational resources can be allocated more effectively. This not only saves time but also improves performance.
Moreover, in an era where data is increasingly regarded as the new oil, organizations that prioritize data quality will stand out. Companies leveraging clean and well-structured data can make more enlightened strategic decisions. Hence, understanding data cleaning is essential for anyone involved in data analysis.
In this article, we explore the widespread applications of cleaning data using Python's Pandas library. Pandas is designed to handle numerous data-related tasks with ease, making it a favored choice for many data scientists and analysts. From handling missing values to removing duplicates, Pandas offers a suite of tools and functions tailored for effective data cleaning.
The following sections will delve deeper into the specifics and practical applications of Pandas for data cleaning, equipping readers with hands-on skills that are directly applicable in real-world scenarios after understanding these fundamentals.
Setting Up Your Environment
Setting up your environment is a fundamental step when working with Python Pandas for data cleaning. This aspect determines how smoothly you can undertake the data cleaning process. An organized setup allows users to streamline their tasks, ensuring they can focus on analysis rather than dealing with technical issues.
Before diving into data cleaning, it’s crucial to have the necessary tools and libraries ready. This includes installing relevant software and managing its dependencies, which ensures compatibility and functionality. It's important to choose development environments like Jupyter Notebooks, PyCharm, or Anaconda that facilitate efficient coding and data manipulation.
Here are some specific elements and benefits of setting up your environment properly:
- Clarity: A well-organized environment reduces confusion. Each library or package has its dedicated location, making it easier to manage.
- Efficiency: Loading datasets and executing functions becomes faster with a correctly configured system. This efficiency is particularly useful when dealing with large datasets.
- Collaboration: In team settings, a standardized environment ensures all members are on the same page, reducing integration issues.
- Customization: Setting up your environment allows for personalization based on project needs. You can tailor your installed libraries and tools to fit specific applications.
Installing Pandas
To begin working with data cleaning in Python, the first step is installing the Pandas library. This library is crucial because it provides the necessary functions to handle and analyze data. Installing Pandas is quite straightforward. Here’s a standard method using pip:
For users using Anaconda, the installation can be done easily through the Anaconda Navigator or by using the following command in the terminal:
Once installed, you can confirm by importing Pandas in your IDE or script. This step reassures that the installation was successful and is essential for proceeding to data cleaning tasks.
Importing Libraries
With Pandas installed, you will need to import the library into your workspace before using it. Importing is a simple yet vital procedure. To do this, include the following line of code at the beginning of your script or notebook:
This convention, using , allows for concise coding and enhances readability as you work with various Pandas functions. Besides Pandas, consider importing other libraries that might assist in your data cleaning process:
- NumPy: Important for numerical operations; it works well with Pandas.
- Matplotlib or Seaborn: Useful for data visualization, which often follows cleaning.
Additional Recommendation
Before starting, ensure your Python version is compatible with the libraries. It's recommended to use Python 3.6 or above for optimal performance with Pandas. Using a virtual environment can also help in managing dependencies and versions without conflicts.
"A robust environment setup underpins successful data analysis. Ensure all tools and libraries are in place and verified before commencing any project."
Following these steps will give you a solid foundation for utilizing Pandas effectively in your data cleaning tasks, facilitating a smoother and more productive working experience.
Loading Data into Pandas
Loading data into Pandas is a critical step for any data analysis workflow. Before any cleaning or manipulation can even begin, one must first access the relevant datasets. This process is not merely about importing data; it serves as the foundation for any future operations. A reliable data source provides the confidence needed to perform accurate analysis and achieve valid insights.
When we talk about loading data, we are looking at different formats and storage options available. Understanding how to effectively load data from CSV files and databases enhances flexibility, allowing integration from various sources.
Additionally, knowing the nuances of these methods ensures that the dataset retains important characteristics such as data types and structures, which is vital for further processing and analysis. Overall, the ability to accurately load data into Pandas is a cornerstone skill for any data analyst or programmer.
Reading CSV Files
CSV files, or Comma-Separated Values files, are among the most commonly encountered formats in data analytics. Their simplicity and widespread use in data exchange make them essential for any data cleaning process. When working with Pandas, the function is the primary method to load these files efficiently.
The syntax is straightforward:
One important feature of the function is its flexibility in handling various parameters. For example, you can specify delimiters, encoding, and whether to include headers. This adaptability ensures that data is imported correctly, maintaining its integrity.
Moreover, loading CSV data not only brings the information into Pandas but also sets the stage for effective preprocessing. Once the data is loaded, one can easily check for inconsistencies or missing values, which is crucial for accurate cleaning.
Connecting to Databases
Data is not always stored in flat files like CSV. Sometimes, it resides in relational databases. Pandas provides powerful tools to connect directly to databases using SQL queries. This feature is particularly useful for larger datasets or when data needs to be accessed dynamically.
To connect to a database, one typically uses libraries like . An example of how to establish a connection and load data could be seen in the following snippet:
This method allows complete SQL queries to be executed, paving the way for loading subsets of data or customizing queries to fetch only the necessary information.
Advantages of connecting to a database include real-time access to up-to-date datasets and leveraging the processing capabilities of database management systems. This results in a more efficient workflow, especially for larger datasets.
"Loading data correctly is crucial as it forms the foundation of any data analysis, influencing the results significantly."
By mastering these methods of loading data into Pandas, users are equipped to handle an essential aspect of data cleaning and preparation.
Understanding Data Structures in Pandas
In the realm of data analysis and cleaning, an understanding of data structures is paramount. The foundation of using the Python Pandas library hinges on two primary structures: Series and DataFrame. Grasping how these structures operate not only streamlines data manipulation but also enhances the efficiency of data processing tasks.
Pandas is designed to handle complex data operations efficiently, and its structures are integral to this capability. Series can be considered as a one-dimensional labeled array that holds data of any type, including integers, floats, or strings. In contrast, a DataFrame represents a two-dimensional size-mutable tabular data structure, similar to a spreadsheet. This distinction is crucial because they serve different purposes in data manipulation and analysis.
Series vs DataFrame
Understanding the differences between Series and DataFrames is crucial for effective data management in Pandas.
- A Series is designed for one-dimensional data, which means it is suitable for list-like structures. Each element in a Series is indexed, facilitating quick access to individual elements or subsets of data.
- A DataFrame, however, is structured to hold multiple Series. It comprises rows and columns, making it similar to a SQL table or a data frame in R. This allows for a more organized representation of data where relationships between various pieces of information can be readily assessed.
When deciding between these two structures, one must take into account the nature of the data and the analysis needs. For instance, if you are handling a single list of numbers or strings, a Series may suffice. Yet, when you have dually related datasets—like customer information and their transactions—a DataFrame offers a more intuitive way to manage that complexity.
Exploring DataFrame Methods
DataFrames come equipped with an array of methods that significantly enhance data manipulation capabilities. Many of these methods cater to common tasks like filtering, aggregation, and data transformation. Having a good grasp of these methods makes it easier to implement data cleaning strategies effectively.
Some essential methods include:
- : Displays the first few rows of the DataFrame, allowing for a quick peek at the data, essential for initial assessments.
- : Provides a concise summary of the DataFrame, including the data types of each column and the existence of null entries.
- : Summarizes the statistics of numerical columns, offering insights into central tendencies and variability.
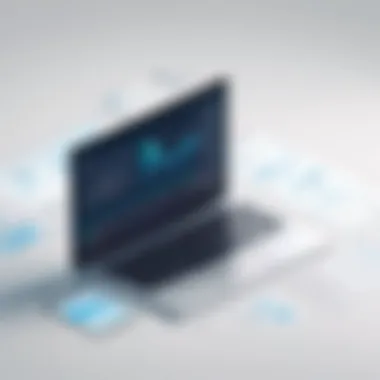
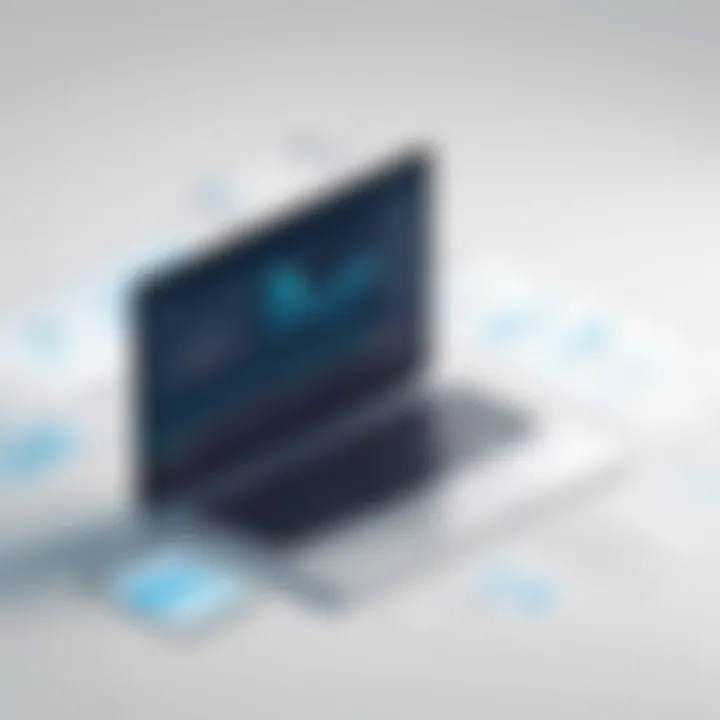
In using these methods, one can gain valuable insights that guide the next steps in data cleaning efforts. For instance, discovering the presence of null values or data types can dictate how one approaches filling missing data or converting data types, thereby enhancing the overall quality of the dataset.
Understanding these structures and their methods not only aids in efficient data handling but also lays the groundwork for more advanced data manipulation techniques, making it a critical cornerstone of using Pandas for data cleaning.
Handling Missing Data
Handling missing data is a critical aspect of data cleaning, as it significantly impacts the quality and accuracy of data analyses. Missing data can arise from various sources such as survey non-responses, errors in data entry, or system failures. When not properly addressed, these gaps can lead to skewed results and unreliable conclusions in research and data analytics projects.
The importance of meticulous handling of missing data lies in the potential consequences it can have. Datasets with missing values can distort analyses, resulting in biased estimates or erroneous inferences. Thus, it is imperative to understand how to identify and impute missing values effectively.
Furthermore, the choice of approach for managing these missing values can influence predictive models' performance. By employing appropriate methods, one can retain a more complete dataset that enhances subsequent analyses, ensuring they are both robust and reflective of the actual patterns in the data.
Identifying Missing Values
The first step in addressing missing data is to identify where these values occur within a dataset. In Pandas, this can be achieved using the method, which returns a boolean DataFrame indicating the presence of null values. Alternatively, the function provides an overview of the dataset, including the count of non-null entries per column. This initial identification is crucial because it lays the groundwork for further strategies such as imputation or removal of the missing values.
Here are some methods to identify missing values:
- Using to get the total count of missing values per column.
- Employing to obtain the percentage of missing values per column.
Strategies for Imputation
Imputation is essential in filling in missing data points, and several strategies can be employed. Depending on the distribution of the data and the assumptions one can make, different methods can yield varied results.
Mean Imputation
Mean imputation involves replacing missing values with the average of the existing values in a column. This method is straightforward and computationally inexpensive. The key characteristic of mean imputation is its ability to maintain the overall dataset size without loss of data points. It is a popular choice when the dataset follows a normal distribution. However, it has drawbacks. It does not account for the variance in the data, making it potentially misleading in datasets with outliers.
Median Imputation
Median imputation replaces missing values with the median of the data. This approach is particularly effective in datasets that may contain outliers, as it is less sensitive to extremes compared to mean imputation. Its primary advantage is that it preserves the central tendency of the data while eliminating influential outlier effects. The trade-off, however, is that it may not accurately reflect the average value, which could be essential in certain analyses.
Mode Imputation
Mode imputation is used primarily for categorical data. It entails substituting missing values with the most frequently occurring value in the dataset. This method retains the distribution of categories, making it suitable for maintaining the integrity of categorical features. While mode imputation can be beneficial, it can also lead to a loss of variability within the dataset, especially if there is a dominant category.
Removing Missing Values
In some situations, it may be more appropriate to remove missing values rather than attempting to impute them. The method in Pandas can eliminate any row or column that contains null values, allowing for a complete dataset for analysis. However, it's crucial to consider the trade-off here: while removing missing values can simplify analyses, it also may reduce the dataset size significantly, which can impact the validity of the results obtained. Therefore, a careful analysis of the amount and impact of missing data should guide the decision on whether to impute, remove, or retain missing values.
Data Type Conversions
Data type conversions play a crucial role in data cleaning and preparation for analysis. Every dataset can include various data types such as integers, floats, strings, and dates. These types can affect how data is processed by functions and algorithms in Python, particularly with the Pandas library. If data types are not set correctly, analysis could yield inaccurate results or even cause errors in execution. Hence, understanding how to identify and convert data types is essential for any data scientist or programmer.
Identifying Data Types
The first step in data type conversions involves identifying the data types in your DataFrame. Pandas offers a straightforward way to check the data types of each column using the attribute. Knowing the type of each column allows you to determine if any conversions are necessary. For instance, if you have a column that should represent dates but is stored as strings, this can lead to issues when attempting to perform time series analysis. Using the method can also provide insights into how many non-null entries exist per column, which is helpful to spot discrepancies.
Converting Data Types
Changing DataFrame Columns
Changing DataFrame columns is a common practice when dealing with data cleaning. It involves altering the data type of an entire column, which can significantly impact how you analyze that data. For example, if you convert a numerical column that is mistakenly classified as a string into an integer, you can start performing numeric operations on it. The flexibility of Pandas allows you to change data types easily with the method. This method not only enhances data processing but also optimizes memory usage when dealing with large datasets.
Using astype() Method
The method is a powerful tool for converting data types in Pandas. This method allows you to specify the desired type and can be applied to any column of a DataFrame. Its primary benefit is that it provides a clear and efficient way to ensure your data is in the right format. One of its unique features is the ability to convert columns to different numeric types, such as converting a float to an integer, which removes the decimal portion. However, caution is needed when using this feature, as it may truncate valuable data by eliminating decimal places. Thus, while is beneficial, you should always double-check your data after conversions to maintain its integrity.
Removing Duplicates
In the realm of data processing, the presence of duplicate records can significantly distort analysis results. This section will delve into why removing duplicates is a critical step in the data cleaning process. Handling duplicates ensures data integrity and leads to more accurate insights.
Duplicates can arise from various sources, such as repeated data entry, merging datasets, or importing data from different sources. If not addressed, these duplicates can lead to skewed statistics, inflated averages, and ultimately misguided conclusions. Therefore, it is important to develop an understanding of methods used in identifying and removing duplicates efficiently.
Identifying and rectifying these issues not only enhances the quality of information but also fosters trust in the analysis outcome. By ensuring a clean dataset void of duplicates, analysts can provide more reliable reports, which is essential for informed decision-making.
Identifying Duplicates
To start the process of removing duplicates, one must first identify them within the dataset. In Python Pandas, this is accomplished through the use of the method. This powerful function examines each record row by row, determining which entries are exact copies of others.
To identify duplicates, consider the following points:
- Customization: The method allows users to specify whether to consider all columns or just a subset. This flexibility helps in cases where only certain fields matter for identifying duplicates.
- Keep Parameter: Users can define which duplicates to keep. This can be the first occurrence, the last, or none at all. Setting marks all duplicates, facilitating a clearer view of redundancy in the dataset.
For example, consider the following code snippet:
This will identify the rows that are duplicates, allowing for an easy correction process. When your data is well-prepared and duplicates have been flagged, you're ready for the next step.
Dropping Duplicates
Once duplicates are identified, it is necessary to drop them from the dataset to ensure data correctness. The method in Pandas facilitates this with ease. By calling this function, the DataFrame is filtered to retain only the unique entries.
Key considerations when dropping duplicates include:
- Specify Subset: Similar to , can also target specific columns. This is beneficial when only parts of a dataset require duplicate removal.
- In-place Operation: This method allows for an option to modify the original DataFrame without creating a new one, by setting the parameter to .
Here is an example of the syntax:
This drops duplicate entries based on the 'id' column while keeping the first occurrence of each unique value. After executing this process, the resulting DataFrame will be devoid of duplicates, qualifying it for further analysis.
"Data integrity is paramount in analysis. Removing duplicates is a cornerstone for ensuring high-quality data."
Data Normalization Techniques
Data normalization techniques are crucial in the realm of data cleaning and preparation. Normalization allows datasets to be more consistent and comparable, particularly when working with variables that have different scales. This is vital when applying any analytical methods, such as machine learning algorithms, that can be sensitive to the ranges of the input data. Common benefits of normalization include improving the convergence rates of optimization algorithms and enhancing overall model performance. It is essential to understand the context of the data, selecting strategies that best suit the analysis requirements. As data scientists, we must be judicious in implementing these techniques to avoid misinterpretation of results due to improperly scaled features.
Min-Max Scaling
Min-Max scaling is a widely used normalization technique that transforms features to a common scale, typically [0, 1]. The formula for Min-Max scaling is:
Here, (X_min) and (X_max) are the minimum and maximum values of the feature, respectively. By applying Min-Max scaling, one can ensure that all features contribute equally to the distance computations involved in methods like k-nearest neighbors and clustering.
Example of Min-Max Scaling in Python:
In this example, the values from the DataFrame are scaled to the range [0, 1]. This is useful for algorithms that require feature scaling to function properly. However, care should be taken—if new data comes in that has values beyond the original range, it may lead to unexpected results unless additional steps are taken.
Z-Score Normalization
Another prevalent normalization approach is Z-score normalization. Unlike Min-Max scaling, Z-score normalization transforms features based on their mean and standard deviation. The formula for Z-score normalization is:
[ Z = \fracX - \mu\sigma ]
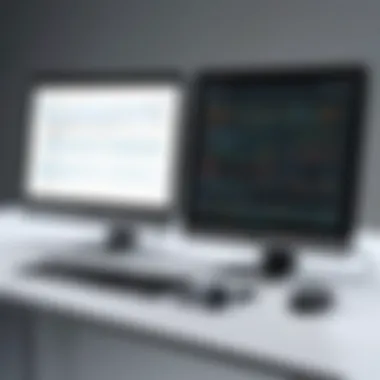
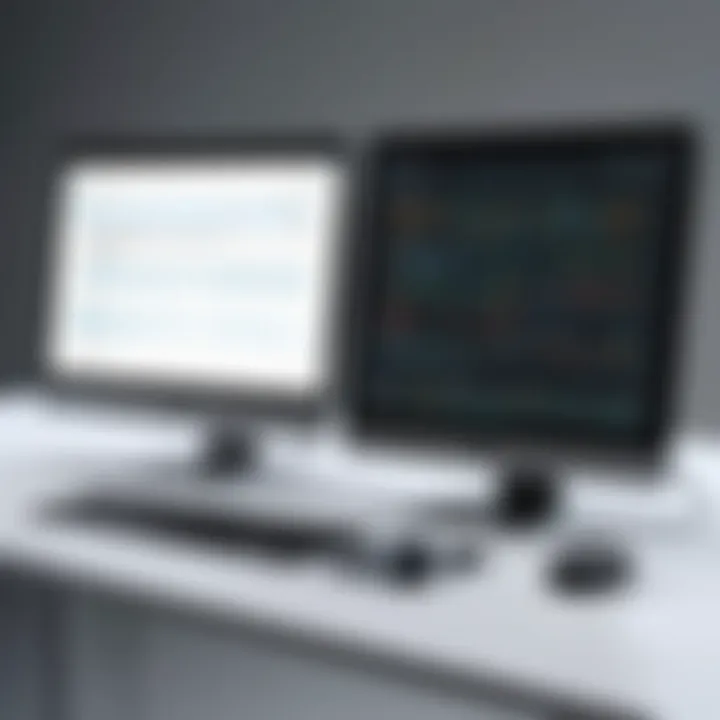
Here, (\mu) is the mean and (\sigma) is the standard deviation of the feature. By using Z-scores, each feature is centered around zero and has a standard deviation of one. This means that the transformed features represent how many standard deviations away a specific data point is from the mean.
Example of Z-Score Normalization in Python:
Z-score normalization is particularly effective when dealing with features that are normally distributed. However, it is less useful if the features are not Gaussian, as the transformation may not be meaningful in these cases.
Important Note: When applying either normalization technique, it is crucial to fit the scaler only on the training set. This prevents data leakage and ensures that model performance evaluation remains valid.
Outlier Detection and Treatment
Outlier detection and treatment is a pivotal part of data cleaning. Outliers can skew results and lead to inaccurate conclusions. In data analysis, understanding and addressing these anomalies is essential for deriving meaningful insights. Ignoring outliers can distort statistics, leading to misinformed decisions. Thus, methods to detect and treat outliers need careful consideration. Evaluating outliers can enhance the reliability of models and improve overall data integrity. Pandas provides various tools for both identifying and addressing these outliers, making it an effective choice for data practitioners.
Identifying Outliers
Identifying outliers involves recognizing data points that significantly differ from the rest of the dataset. A common method is using the Interquartile Range (IQR). This statistical process involves calculating the first quartile (Q1) and the third quartile (Q3). The IQR is defined as Q3 - Q1. Any point that lies below Q1 - 1.5 * IQR or above Q3 + 1.5 * IQR is considered an outlier. Visualization methods such as box plots also aid in spotting these values quickly.
Another approach for identifying outliers is through Z-scores. This method computes how many standard deviations a data point is from the mean. If the Z-score exceeds a certain threshold, often set to 3, the point is viewed as an outlier.
Handling Outliers
Removing Outliers
Removing outliers involves excising these anomalous data points from the dataset. This method is often viewed as a straightforward approach to enhancing data quality. By eliminating these points, analysts can achieve a more accurate representation of the data. A key characteristic of removing outliers is that it simplifies analysis by reducing noise in the dataset. This can lead to better performance for machine learning models. However, one must be cautious. Removing too many points can result in loss of valuable information. A thorough evaluation of reasons behind outliers is crucial before taking this step.
Transforming Data
Transforming data is another effective technique for handling outliers without losing data points. This process involves applying functions such as log transformation or square root transformation. These mathematical operations can reduce the influence of extreme values. A key characteristic of transforming data is that it retains all data points, allowing for a more comprehensive analysis without skewing results. However, users must understand the context of their data. Transformations may complicate the interpretability of results. Assuring that the transformation aligns with analysis goals is vital for successful data cleaning.
To ensure robust analysis, it is important to employ a balanced approach to outlier detection and treatment—both removing and transforming data have unique benefits and potential drawbacks.
In summary, outlier detection and treatment can dramatically influence data analysis outcomes. Effective strategies like identifying, removing, or transforming values are fundamental for ensuring data accuracy and integrity. Understanding the context of data and applying methods cautiously will lead to better insights.
Data Formatting and Transformation
Data formatting and transformation is a crucial aspect of data cleaning in Python Pandas. This process involves adjusting data into a suitable format for analysis. Proper formatting ensures data consistency, which is vital for generating accurate insights. Effective data formatting can provide clarity and make further analysis straightforward. Without this step, analysts may face challenges when attempting to interpret the data.
Data is often received in various formats, including text, numbers, and dates. This diversity often leads to complications when computing or visualization is needed. For instance, numerical values may be improperly stored as strings, hindering mathematical operations. Therefore, transformation processes aim to convert data into the required format, addressing inconsistencies and ensuring uniformity across datasets.
Moreover, well-structured data improves usability for various applications, such as statistical analysis and machine learning. By applying formatting directly within Pandas, one can utilize its powerful functionalities to streamline this phase of data preparation.
String Formatting
String formatting involves adjusting string data for readability and analysis. Pandas provides various methods to manipulate strings within DataFrames. For instance, it can remove excess whitespace, convert text to lowercase, or format text consistently across a dataset. These operations enhance the dataset’s integrity and help prevent errors in subsequent analyses, such as mismatches during joins or group operations.
Another important consideration is handling categorical variables, where inconsistent casing can lead to duplicate categories. For example, 'USA', 'usa', and 'UsA' represent the same entity but could result in separate categories. Using the method can easily unify these entries.
Additionally, string formatting can be essential for preparing data for presentation. When creating reports, ensuring that string data follows a specific format can greatly improve readability and user experience.
Here are some common string formatting techniques in Pandas:
- Using to remove leading and trailing whitespace.
- Using for cleaning unwanted characters.
- Utilizing the method to concatenate strings.
By applying these techniques, you can create clean and consistent datasets ready for analysis.
Date Formatting
Date formatting revolves around converting date strings into datetime objects recognized by Python. This transformation is essential because it allows for the utilization of various datetime functionalities that facilitate time-based analysis. For example, one can compute time differences, filter datasets by specific dates, or extract particular components such as year or month.
Pandas provides the function to convert dates in different formats into a uniform datetime format. This function recognizes various formats automatically, although specifying formats can enhance efficiency and accuracy. For instance, when dates are in the 'DD/MM/YYYY' format, understanding the exact format helps to prevent misinterpretation of day and month values.
It is essential to ensure your dates do not include any erroneous entries. For instance, a date recorded as '31/02/2022' is invalid. Detecting these inaccuracies is a vital part of the cleaning process before performing analysis.
Furthermore, date formatting can impact analyses based on time-series data. For instance, maintaining consistent time intervals is critical when analyzing trends over time. One way to address this is by resampling time-series data to a desired frequency using the method.
Indexing and Selecting Data
Data indexing and selection is fundamental when working with datasets in Python's Pandas library. With data often comprising thousands or even millions of rows, effective indexing allows programmers to efficiently retrieve, modify, and analyze data subsets without unnecessary computational overhead. The ability to pinpoint specific data points can significantly streamline the data cleaning process, facilitating a more focused approach during analysis.
Indexing enhances performance by providing a structured way to access relevant data. It is essential for tasks such as applying functions, filtering data, and visualizing key insights. However, improper indexing can lead to sluggish performance and confusion, making it crucial to understand the various methods available in Pandas.
Here, we will explore two primary methods for indexing and selecting data: using and , and filtering DataFrames.
Using .loc and .iloc
Both and are powerful tools in Pandas for selecting data, but they serve different purposes. The indexer allows you to access rows and columns by labels. This means you can specify the exact row or column names you wish to work with. For instance, if you have a DataFrame with columns named "Age" and "Salary", you can easily retrieve all ages as follows:
On the other hand, is used for positional indexing. It allows you to access rows and columns by their integer location. For instance, if you want to obtain the first row in your DataFrame, you can do so with:
The importance of knowing when to use versus cannot be overstated. Using labels can make your code more readable and maintainable, while positional indexing can facilitate operations where data layout is more relevant than specific labels.
Filtering DataFrames
Filtering is another essential technique that complements indexing. It allows you to create new DataFrames based on specific conditions. For example, if you want to extract rows where the salary exceeds 50,000, you can employ the following approach:
This method yields a new DataFrame containing only those rows that meet the specified condition. Filtering is advantageous for narrowing down your dataset to the most relevant observations, a vital step in data cleaning and analysis.
When filtering, it is also essential to consider the implications of your conditions. Poorly structured filters may exclude valuable data or include irrelevant records, potentially skewing your analysis.
Remember: Effective filtering leads to clearer insights and helps maintain the integrity of your data analysis.
Understanding indexing and filtering mechanisms in Pandas is a crucial step towards mastering data cleaning. By effectively utilizing , , and DataFrame filtering, you can enhance the accuracy and efficiency of your data preparation tasks.
Merging and Joining DataFrames
Merging and joining DataFrames is a crucial aspect of data cleaning and manipulation in Python using the Pandas library. This functionality allows for efficient integration of multiple datasets, resulting in a comprehensive dataset suitable for analysis. The importance of this topic lies in its application; combining datasets can enhance insights and improve decision-making in various domains such as finance, healthcare, and marketing. Key considerations include understanding the structure of the data as well as knowing how to handle duplicates and mismatched indices, which can arise during the merging process.
When working with real-world data, it's common to have related information scattered across different tables or files. For example, a company might have customer information in one DataFrame and the corresponding sales data in another. By merging these DataFrames, one can create a unified perspective that offers deeper insights, leading to more informed actions.
Concatenating DataFrames
Concatenation refers to the process of stacking DataFrames either vertically or horizontally. This is particularly useful when combining datasets that have the same structure (i.e., similar columns) or when appending rows from multiple datasets. The function enables this action seamlessly.
Here’s how the basic syntax looks:
When concatenating, it is essential to keep in mind that index values may overlap unless adjusted. Also, concatenation might introduce NaN values in places where columns are not aligned. Thus, always check the output to ensure it meets expectations.
Joining on Keys
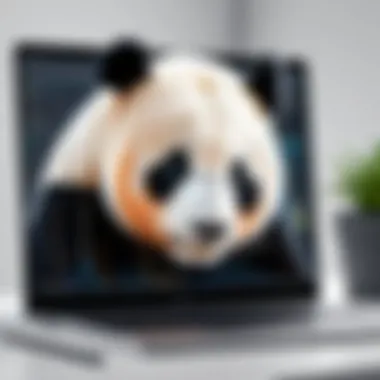
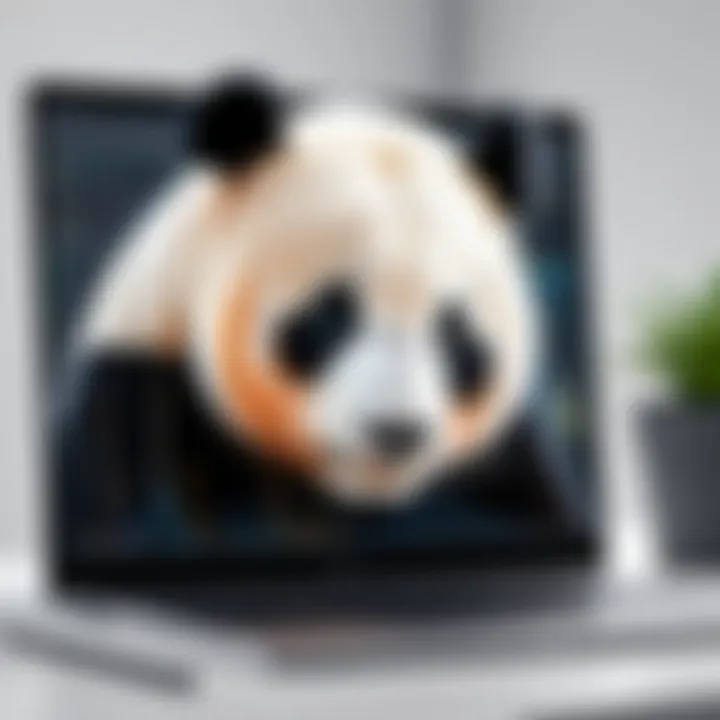
Joining is another method to merge DataFrames, wherein rows from two DataFrames are combined based on the values in specified columns known as keys. This is similar to SQL join operations and allows for more complex data integrations. In Pandas, this can be accomplished using the function.
The basic syntax for joining on keys is as follows:
Different types of joins available include inner join, outer join, left join, and right join. Each type serves a different purpose:
- Inner join: Only includes rows with keys present in both DataFrames.
- Outer join: Combines data from both DataFrames, filling missing values with NaN.
- Left join: All rows from the left DataFrame are included, and matching rows from the right DataFrame.
- Right join: All rows from the right DataFrame are included with corresponding matching rows from the left DataFrame.
Merging and joining DataFrames effectively allows for enhanced data analysis and preprocessing, ensuring that data scientists and analysts can work with comprehensive datasets that reflect the complexity of their data environments.
Practical Applications of Data Cleaning
Data cleaning is essential in various fields where accurate and reliable data is needed for decision making. The practical applications of data cleaning using Python's Pandas library highlight its importance not only in preparing data for analysis, but also in enhancing the quality and integrity of datasets. Whether for research, business intelligence, or data-driven decision making, clean data provides a foundation for effective insights. In this section, we explore the significance of practical applications of data cleaning through case studies, focusing on surveys and financial data which are common in many industries.
Case Study: Data from Surveys
Surveys are a popular method for gathering information across diverse fields such as market research, social science, and health studies. However, raw survey data often contains errors due to various factors like respondent bias, incomplete responses, and data entry mistakes. These inaccuracies can lead to misleading conclusions if not properly addressed.
In this case study, we examine a dataset from a consumer feedback survey. The dataset includes several columns, including respondent age, satisfaction ratings, and comments. Here are some steps taken in cleaning this survey data using Pandas:
- Identifying Missing Values: The first step involves checking for missing responses. Pandas provides the function to easily identify these gaps.
- Handling Outliers: Outliers in the data can skew results. For instance, an unusually high satisfaction rating might indicate a mistake. We can use methods like the Z-score to identify these anomalies and decide whether to exclude or investigate them further.
- Standardizing Responses: Survey answers might have differing formats (e.g., 'good' vs 'Good'). Using Pandas' string methods, we can standardize responses to ensure uniformity.
By the end of the cleaning process, statistical analyses would yield results that genuinely reflect consumer opinions, thus providing valuable insights for marketers and product developers.
Case Study: Financial Data
In the finance sector, decisions are heavily reliant on accurate data. Datasets might include stock prices, transaction records, or customer details. Any inconsistency in this data can lead to significant financial losses or miscalculations.
Consider a financial dataset containing historical stock prices along with transaction volumes. The first step in cleaning this dataset often involves:
- Removing Duplicates: Financial data can sometimes have duplicate entries which can distort analysis. Using Pandas' method helps in eliminating these duplicates effectively.
- Type Conversion: Ensuring numerical fields like transaction volumes are in the correct data type is crucial for calculations. Using allows for restructuring columns that may have been incorrectly formatted.
- Filling Missing Values: In financial datasets, missing values can occur due to unrecorded transactions. Strategies like forward filling () can be practices to fill these gaps based on adjacent values.
The result of effective data cleaning in finance not only improves reporting accuracy but also enhances investor confidence and strategic planning.
"Data that is not cleaned can lead to misinterpretation of financial reports, which undermines strategic decisions."
In summary, practical applications of data cleaning show how essential clean data is across domains. The process, particularly with tools like Python's Pandas, allows practitioners to convert raw data into actionable insights, driving informed decision-making.
Common Pitfalls in Data Cleaning
Data cleaning is a critical phase in the data analysis process. However, several pitfalls can hinder effective cleaning. Understanding these common issues is vital. When data is improperly managed, it may lead to incorrect insights or flawed decisions.
Here, we will discuss the two primary pitfalls: over-cleaning datasets and ignoring data quality. These topics will shed light on the risks involved in the data cleaning process.
Over-Cleaning Datasets
Over-cleaning, as the term suggests, refers to the practice of excessively modifying data to the point that its original context and meaning are lost. This represents a serious risk in data cleaning.
The allure of achieving perfectly clean datasets can lead one to exclude valid but irregular data points. For instance, removing outliers from a financial dataset might inadvertently eliminate crucial information about performance spikes or dips. This creates datasets that may look pristine but do not reflect the real-world complexities.
Benefits of Caution:
- Maintains the integrity of data.
- Ensures usable data that retains context.
- Supports richer analyses by retaining diversity.
Instead of impulsively cleaning data, it is crucial to assess the necessity of modifications. The goal should be to balance cleanliness with authenticity.
"Data that is too clean may not be reflective of reality, leading to poor insights."
Ignoring Data Quality
Ignoring data quality can be just as damaging as over-cleaning. Poor-quality data undermines the purpose of cleaning efforts. When data quality is mishandled, it can lead to errors in analysis and subsequently flawed conclusions.
Key considerations include:
- Source Validation: Ensuring that the source of data is credible. Relying on poor sources introduces many potential errors.
- Consistency Checks: Validating that data follows a standard format or consistency rule can help identify data that might propagate issues.
- Reviewing Data Regularly: Regular checks of data quality help to observe changes over time, leading to better foresight in management.
By being aware of the tendency to over-clean and the critical nature of data quality, practitioners can guard against these pitfalls effectively.
Best Practices for Data Cleaning
Data cleaning is a crucial aspect of data analysis. Best practices in this domain can significantly enhance the quality and usability of datasets. Following well-defined processes improves the integrity of your data, ensuring that the subsequent analysis is accurate. When you invest time in learning and applying best practices for data cleaning, you build a solid foundation for any data-driven project.
Documenting Your Process
Documenting your data cleaning process is essential. It not only keeps track of what has been done but also provides clarity for future reference. Documentation can include details about data sources, cleaning methods applied, and decisions made along the way. This can be very beneficial when you revisit the project or share your findings with others. It helps in ensuring reproducibility of your process and allows other team members to understand your approach.
Here are some key points to consider while documenting:
- Title and Date: Always begin with a clear title and the date of documentation.
- Data Source: State the origin of your data and any transformations that have been made.
- Cleaning Methods: List out all methods used, such as imputation strategies or methods to handle duplicates.
- Rationale: Explain why you made specific decisions during the cleaning process, as this insight can be invaluable.
- Version Control: Keep track of different versions of your dataset. Using version control systems like Git can be helpful.
"Documentation is a bridge to the past and a roadmap for the future."
Iterative Approach to Cleaning
An iterative approach to data cleaning means that the process is not a one-time task. As you analyze your data, you will likely find new issues that require cleaning. Recognizing that data cleaning is a cyclical process allows for adjustments and improvements based on ongoing insights. This flexibility can lead to a more refined dataset over time.
To adopt an iterative approach effectively:
- Initial Cleaning: Start with a broad cleaning strategy. Identify obvious issues like missing values or irregular formats.
- Analyze Results: Conduct initial analyses to understand the impact of your cleaning methods.
- Refine and Repeat: Based on insights gained, refine your cleaning methods. You may need to re-address certain areas multiple times.
- Engagement with Data: Maintain a close interaction with your dataset through visualizations and queries to ensure you understand its nuances.
In summary, best practices in data cleaning require documenting your process and adopting an iterative approach. These strategies ensure your datasets remain clean, relevant, and ready for analysis.
Ending and Future Perspectives
The process of data cleaning is an ongoing journey in the field of data science. Concluding this article brings to light not only the techniques covered but also the future trends that will continue to shape how data is managed and interpreted. The effectiveness of data cleaning directly impacts the quality of analytical insights. High-quality data serves as the foundation for sound decision-making in various sectors including business, healthcare, and technology.
As technology advances, the methods for data cleaning will also evolve. Automation plays a key role in future data cleaning processes. Regular updates to tools such as Python and the Pandas library will provide users with enhanced functionalities. This means programmers must remain adaptable and willing to learn. Being up to date with new features can streamline the cleansing process, reducing the time spent on repetitive tasks.
Additionally, as data security grows in importance, data cleaning workflows must integrate best practices in data protection. Handling sensitive data responsibly will become essential. A blend of legal knowledge and technical skills will ensure compliance with regulations such as GDPR or HIPAA.
The growth of machine learning and artificial intelligence is another consideration. These technologies hold the promise of identifying patterns in messy datasets that were previously difficult to discern. Machine learning algorithms can learn from data cleaning practices, thereby improving over time.
Looking ahead, it is crucial for aspiring and experienced programmers as well as IT professionals to stay informed about these emerging trends. Opening a dialog on platforms such as Reddit and Facebook can facilitate knowledge sharing on innovative data cleaning techniques.
In summary, data cleaning is not just a checklist activity but a fundamental step in the broader context of data science. The intersection of data cleaning with emerging technologies presents an exciting frontier for technology enthusiasts.
Summarizing Key Techniques
As we conclude this exploration of data cleaning using Python's Pandas, several key techniques stand out as fundamental to effective practices. They include:
- Handling Missing Data: Recognizing when data is missing and applying imputation strategies or removing incomplete records is crucial.
- Removing Duplicates: Identifying and eliminating duplicate entries ensures data consistency.
- Data Type Conversions: Making sure that each column in a DataFrame has the appropriate data type is necessary for accurate analysis.
- Normalizing Data: Ensuring that data is standardized can lead to more reliable analytical results, especially in applications like machine learning.
- Outlier Detection: Recognizing anomalies in data can inform decisions about how to treat them, whether it's through removal or transformation.
These techniques are not exhaustive but provide a solid framework for programmers and analysts to build upon in their work.
The Evolving Landscape of Data Cleaning
The data cleaning landscape is evolving at an unprecedented rate. As data continues to proliferate, traditional data cleaning methods must adapt. The future signals a shift towards more automated solutions, leveraging powerful algorithms to contend with increasing data complexity.
Key factors influencing this evolution include:
- Big Data: The rise of big data necessitates more sophisticated tools for cleaning vast arrays of information.
- Cloud Computing: Accessibility to cloud environments facilitates collaboration and scalability, altering how data is cleaned and shared.
- Integration of AI Tools: AI-enhanced data cleaning methods will likely emerge, enabling faster identification of flaws and patterns.
- Interactive Data Tools: User interfaces that simplify the data cleaning process will empower more individuals, reducing reliance on highly specialized skills.
In summary, the future of data cleaning is not just about preserving data integrity but also about adapting to advancements in technology. Practitioners must stay agile, continuously updating their skills and methodologies to cope with the evolving demands of data analysis.