Mastering Data Visualization with JavaScript Techniques
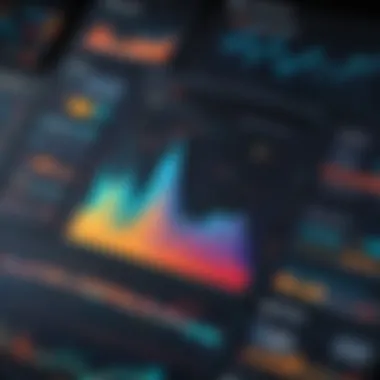
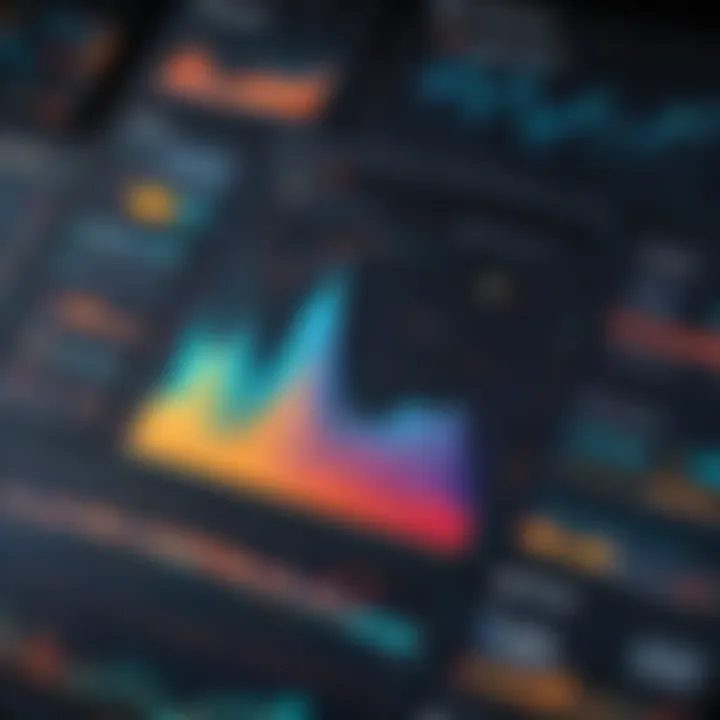
Intro
In the realm of programming, data visualization stands out as a critical component, particularly within the JavaScript ecosystem. This article serves as a guide to several techniques and tools available for visualizing data with JavaScript. Understanding data visualization is more than just aesthetics; it bridges the gap between complex data sets and meaningful insights.
As we navigate through this discussion, we will examine why effective data visualization is indispensable in our data-driven world. The explosion of information necessitates clarity in how data is presented. Popular libraries such as D3.js, Chart.js, and Highcharts will be highlighted, alongside strategies for their effective implementation. This article is crafted for a varied audience—from novices looking to grasp the basics, to seasoned developers seeking to refine their skills. The objectives are clear: to furnish readers with the essential tools and techniques for impactful visual communication in their projects.
Prologue to Data Visualization
Data visualization is a crucial aspect of data analysis and communication. In a world inundated with information, the ability to present data clearly and effectively cannot be overstated. Visualizations ease understanding of complex datasets, allowing users to identify trends and insights that may not be apparent in raw data alone. This article emphasizes the relevance of data visualization, particularly through JavaScript, a versatile language known for its capability in creating dynamic and interactive visualizations.
Data visualization serves multiple purposes. It enhances comprehension, aids in decision-making, and communicates findings to a broader audience. By representing data visually, stakeholders can grasp significant patterns and outliers, leading to more informed conclusions. In this context, tools and techniques equipped for effective visualization become invaluable.
Moreover, the importance of data visualization has grown with the exponentially increasing volume of data generated daily. In sectors like business, healthcare, and science, effective data presentation aids in drawing actionable insights. JavaScript libraries offer powerful resources that facilitate this process, making it easier for developers and analysts to create visuals without significant hurdles.
In sum, understanding data visualization is foundational for anyone who engages with data. It is the bridge between raw numbers and meaningful interpretation, crucial in today's data-driven environment.
The Importance of Data Visualization
Recognizing the significance of data visualization reveals its multifaceted benefits. First, it transforms complex data structures into accessible visuals. This simplification is especially important in environments where quick decisions are necessary. Visuals compel attention and can drive user engagement more effectively than text-heavy reports.
Here are some key points regarding its importance:
- Improved Decision Making: Visual representations of data can lead to quicker and better-informed decisions.
- Pattern Recognition: Humans are inherently visual learners. Patterns that are difficult to detect in tables can stand out in graphs and charts.
"Good visualization is a key part of the storytelling process in data."
- Facilitated Communication: Visuals help in sharing findings with others who may not possess the same technical expertise. This accessibility is vital for collaborations and presentations.
Understanding Data Types
Understanding the various types of data is essential before diving into visualization techniques. Each data type offers distinctive characteristics that influence how it should be represented visually. Broadly, data can be classified into two categories: qualitative and quantitative.
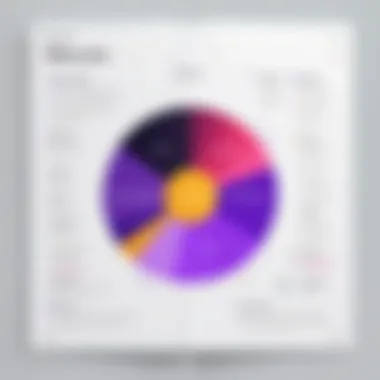
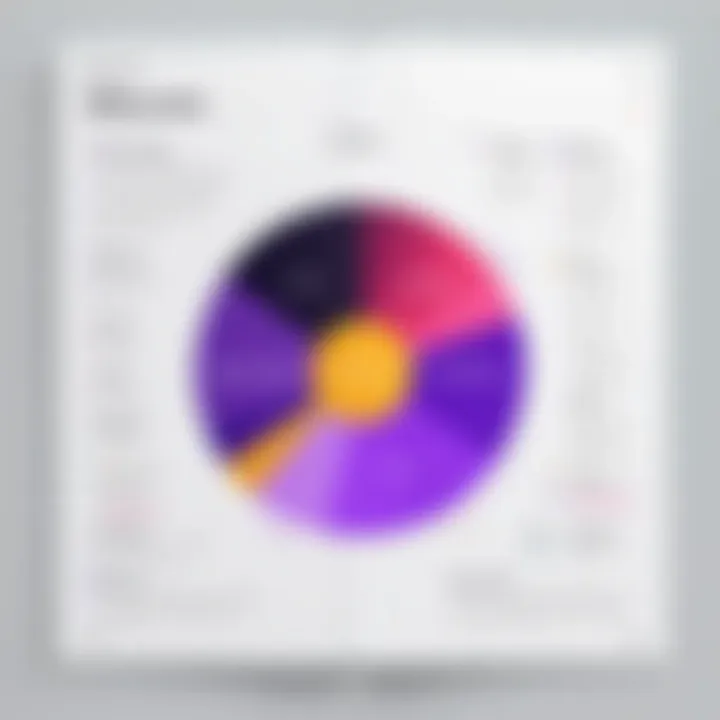
- Qualitative Data: Also known as categorical data, it represents characteristics or attributes. Examples include names, colors, and labels. Visualizing qualitative data often involves bar charts or pie charts, which can convey frequency or proportions effectively.
- Quantitative Data: This type encompasses numerical values that can be measured. It includes intervals and ratios. Visual representations such as line graphs or scatter plots are more suitable for quantitative data since they show relationships over time or differences between groups.
Understanding these distinctions is crucial for selecting the right visualization method. Using the appropriate tools can significantly enhance the clarity and impact of the communicated data. In the following sections, we will explore JavaScript fundamentals and libraries that cater to these data types.
JavaScript Basics for Visualization
JavaScript serves as a fundamental building block for data visualization in the modern digital landscape. Understanding JavaScript basics is essential for anyone looking to create effective and interactive data visualizations. This section delves into the core concepts of JavaScript and how they relate specifically to visualization tasks. A solid grasp of JavaScript allows developers to manipulate data and create visual elements dynamically, thus offering a more interactive experience.
Core Concepts of JavaScript
Core JavaScript concepts such as variables, functions, and control structures provide necessary tools for any data visualization project. Variables enable you to store data values. Functions encapsulate reusable logic. Control structures, such as loops and conditionals, let you manipulate data flow.
Here are some key elements to consider:
- Variables: Use , , or to declare variables that can hold your data.
- Functions: Write functions to perform specific operations on the data, such as filtering or aggregating.
- Objects and Arrays: Most data in JavaScript is represented as objects or arrays. Understanding how to access and manipulate these structures is critical.
By mastering these core aspects, you lay the groundwork for more sophisticated data operations. For example, using an array to hold your data sets allows for direct manipulation via JavaScript's powerful array methods like , , and . This reduces manual data handling and simplifies your code.
Working with the DOM
The Document Object Model (DOM) represents the structure of an HTML document. When visualizing data, it is important to know how to interact with the DOM to render your visuals. JavaScript enables you to manipulate DOM elements dynamically, adding or modifying them based on your data.
Some important considerations when working with the DOM include:
- Selecting Elements: Use methods like , , and to select relevant elements for your visualizations.
- Creating Elements: Utilize to generate new elements that will hold your visualization components, such as charts or graphs.
- Appending Elements: Use to place newly created elements onto the page, making your data visualizations visible to users.
- Event Listeners: Adding interactivity often requires capturing user actions. Use to respond to clicks, hover events, or other interactions that can affect the visualization.
"Mastering the DOM is crucial for creating dynamic visualizations that engage users."
Integrating these JavaScript fundamentals with DOM manipulation strategies empowers developers to create responsive and meaningful data visualizations. As you gain experience, these concepts will become second nature, allowing for fluid and effective visual interpretation of complex data sets.
Popular JavaScript Libraries for Data Visualization
Data visualization is a critical concept in the realm of data analysis. As we navigate through complex data sets, leveraging effective visualization tools becomes essential. In JavaScript, there is a rich ecosystem of libraries designed to simplify this task. Each library comes with its unique features, strengths, and ideal use cases. Understanding these libraries can significantly enhance project efficiency and improve the clarity of the presented data.
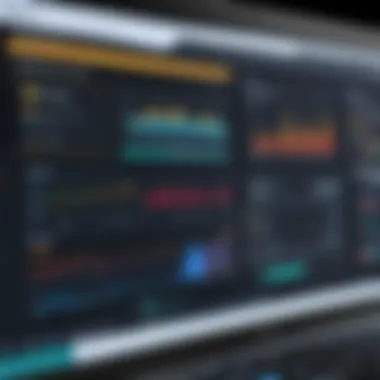
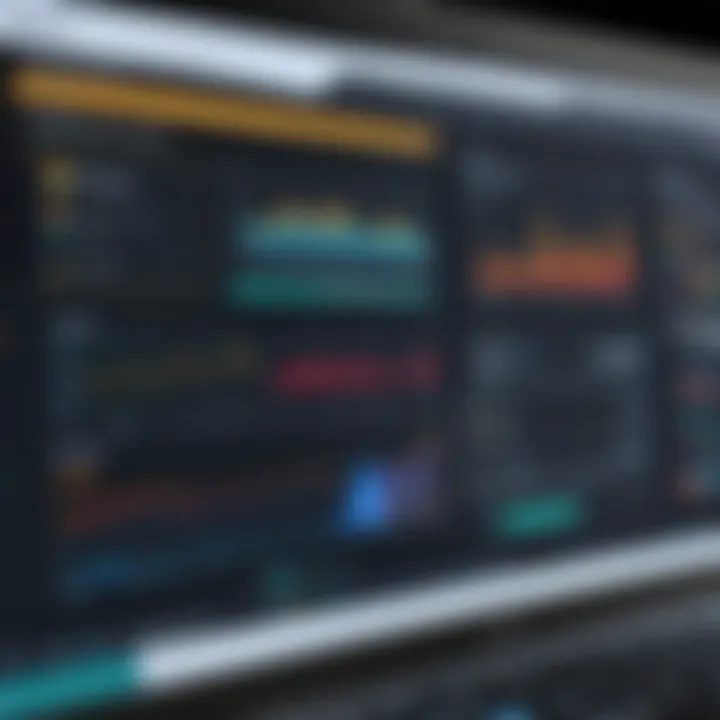
When selecting a library for data visualization, it is important to consider several factors. The complexity of the data being analyzed, the desired aesthetic appeal, and the level of interactivity required are all key elements. Using the appropriate library can lead to clearer insights and more engaging presentations of data.
D3.js: A Comprehensive Approach
D3.js stands out as one of the most powerful JavaScript libraries for data visualization. Its versatility allows developers to create a wide range of visualizations, from simple bar charts to complex interactive maps. D3.js operates by manipulating the Document Object Model (DOM) based on data, providing a reactive environment.
This library has a steep learning curve but offers unmatched flexibility in customization. It supports a variety of formats for data input which can be beneficial for adapting to many different projects. Furthermore, D3.js is particularly effective in creating dynamic visualizations that respond to user inputs or real-time data.
Chart.js: Simplicity and Ease of Use
Chart.js is another popular choice, especially for developers looking for an easier entry into data visualization. It supports eight types of charts including line, bar, and pie charts. The simplicity of Chart.js makes it easy to integrate into projects without extensive setup.
One advantage of Chart.js is its clean and responsive design. The library automatically adjusts to screen sizes which is helpful for maintaining readability across devices. Although it lacks the extensive capabilities of D3.js, Chart.js is ideal for quick, straightforward visualizations where ease of use is a priority.
Plotly.js: Interactive Graphing
Plotly.js provides a robust solution for creating interactive plots. It allows users to generate complex visualizations that can be manipulated by the end user. With support for 3D graphs and contour plots, this library is particularly well-suited for scientific and engineering applications.
One of the standout features of Plotly.js is its ability to enhance user engagement. Users can zoom, pan, and toggle data visibility which encourages exploration of data sets. This interactivity enhances the storytelling aspect of visual data, making it more impactful.
Three.js: Visualizing 3D Data
Three.js is specifically engineered for rendering 3D graphics in the browser. It leverages WebGL for high-performance rendering. This library shines in applications that require advanced visual representations of data, such as simulations or data modeling.
Utilizing Three.js, developers can create immersive and interactive visual experiences. This could be particularly useful in fields such as gaming, architecture, and scientific research. However, users must have a solid understanding of 3D space to effectively utilize this library.
"The choice of the right library for data visualization can make a significant impact on the quality and effectiveness of data presentation."
Each of these libraries offers distinct advantages that cater to various needs in the realm of data visualization. Selecting the correct tool is essential and can enhance not only clarity, but also the engagement and experience of the audience.
Implementing Data Visualization with JavaScript
Implementing data visualization using JavaScript is a crucial step for those seeking to make data more accessible and understandable. This section emphasizes the strategies and steps needed to turn raw data into visual formats that can effectively communicate insights. Proper implementation enables developers to present complex datasets in a more digestible way, aiding decision-making and enhancing user experience. The key to successful visualization lies not only in the choice of library but also in how one integrates these tools into their existing workflows.
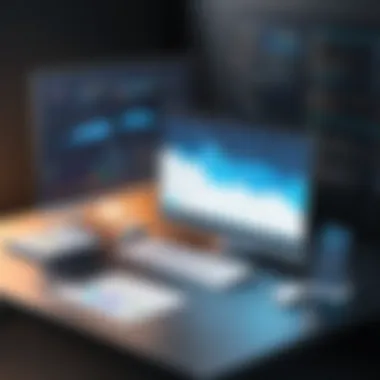
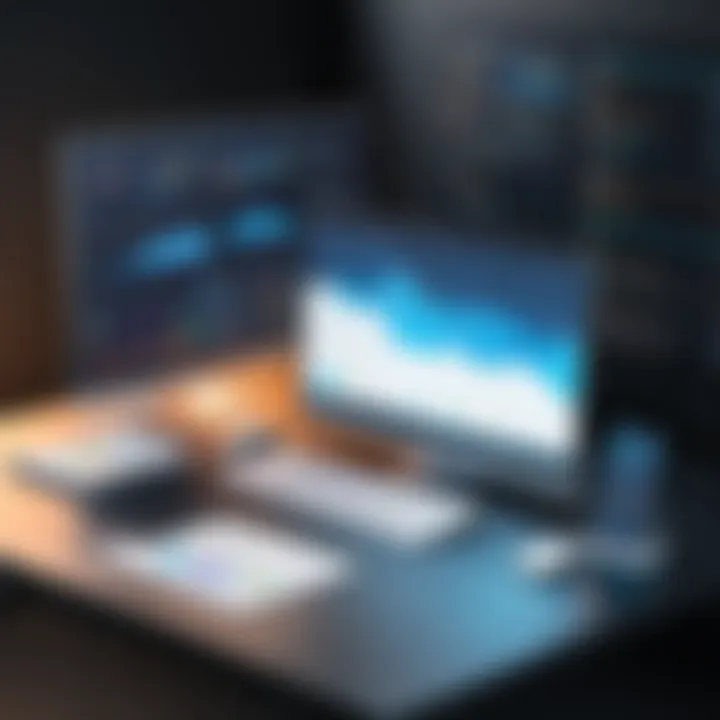
Setting Up Your Environment
Before starting any data visualization project, it's fundamental to set up your development environment appropriately. This ensures you have all the necessary tools and libraries to work efficiently. Here are important steps for establishing your environment:
- Editor: Select a code editor. Visual Studio Code is a popular choice due to its versatility and support for various extensions.
- Node.js: Install Node.js to manage libraries and packages easily. This also allows you to run JavaScript on the server side, which can be useful for data fetching and processing.
- Version Control: Using Git can be beneficial for tracking changes and collaborating with others.
After these steps, ensure your tools are up to date. Keeping software current helps maintain compatibility and security.
Integrating Libraries into Your Projects
Integrating libraries simplifies the visualization process. JavaScript offers several powerful libraries that can be included in your project. Key libraries include D3.js, Chart.js, and Plotly.js, each with distinct advantages. Here’s a brief overview of how to integrate these libraries:
- Include via CDN: For quick projects, you can link to a library directly from a CDN in your HTML file.
- Install via npm: For larger projects, install libraries using Node Package Manager (npm). For example:This method allows for better version control and dependency management.
- Execution: After adding the library, you can call its functions to create visualizations. Be sure to reference the documentation for specific libraries, as each has its syntax and capabilities.
Fetching and Preparing Data
Once the environment is set up, the next step is data handling. Visualizations require clean and relevant data. Here are some practices to keep in mind:
- Data Sources: Identify where your data comes from. It could be a local file, a web API, or a database.
- Data Format: Ensure your data is in a usable format like JSON or CSV. These formats are straightforward for JavaScript to parse.
- Data Cleaning: Implement techniques for cleaning data. This may involve removing duplicates, handling NaN values, or transforming data types.
For fetching data, you can utilize the Fetch API to retrieve data from external sources. Here’s a simple example:
This snippet fetches data asynchronously and is essential for modern web applications.
Creating Basic Visualizations
With your libraries integrated and data prepared, it’s time to create your first visualizations. Start with simple charts like bar and line graphs before moving to more complex visualizations. Here are some steps to follow:
- Choose the Visualization Type: Determine the type of visualization that best represents your data.
- Reference Documentation: Each library has specific functions for different chart types.
- Create the Visualization: Use the library's functions to render your charts. An example with Chart.js:
label: '# of Votes', data: [12, 19, 3],