The Role of 'else' in Python: Understanding Its Functionality
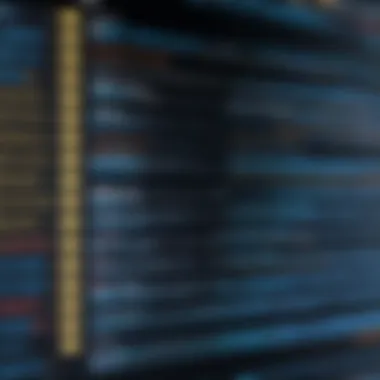
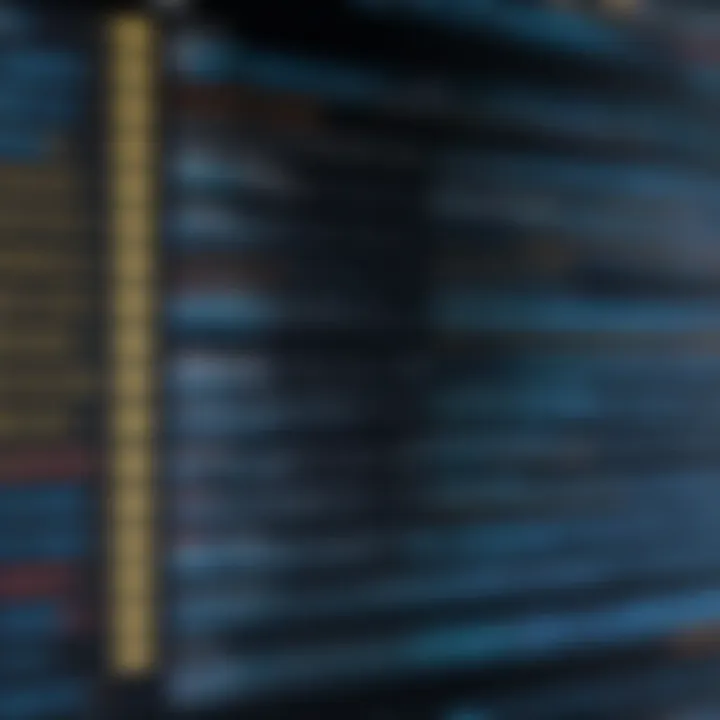
Intro
In Python, understanding the role of the 'else' statement is essential for writing clear and effective code. Often considered a secondary feature compared to the more prominent 'if' statement, its usage can significantly enhance the flow of logic in programs. By employing 'else', programmers can handle alternative conditions and define clear paths for their code execution.
This section sets the stage for a deeper exploration into its functionality and diverse use cases, shedding light on how this component contributes to better programming practices. Balancing simplicity and detail, the journey begins with examining the implications of integrating the 'else' statement into various programming scenarios.
Coding Challenges
While procuring a solid grasp of control flow statements in Python, engaging with coding challenges can be quite instructive. Issues often arise when aspiring programmers overlook the potential of 'else', leading to inefficient code.
These challenges can serve as practical exercises to reinforce core concepts, particularly focusing on control structures. Here are ways in which focusing on 'else' can structure your approach:
- Use 'else' for concise decision-making.
- Optimize flow control by incorporating 'else' in loops.
- Implement 'else' in exception handling to manage errors effectively.
Weekly Coding Challenges
Engaging with a variety of tasks can highlight common pitfalls associated with the 'else' statement. For instance, a challenge could involve coding a simple number guessing game, allowing one to explore how to implement an 'else' for incorrect guesses, improving code readability.
- Present a number for the user to guess.
- Use input capture to determine correctness using 'if' and 'else'.
- Incorporate a loop to repeat until the correct guess is made.
This exercise directly teaches the meaningful placement of the 'else' statement within logical conditions. Practicing various iterations paves way for adept proficiency.
Problem Solutions and Explanations
Post-challenge analysis can clarify common misunderstandings of 'else'. For example, programmers may struggle with nested conditions—with 'else' compounding their confusion. An effective solution is to contextualize nested 'ifelse' blocks using comments, illuminating the pathways through the code.
Analyzing why 'else' serves to provide an alternative allows for enhanced logic processing, ensuring better flow control.
Tips and Strategies for Coding Challenges
Implementing effective strategies helps coders leverage 'else' for problem-solving.
- Always brainstorm possible outcomes prior to coding.
- Visualize control flow to understand each 'if' threshold and its respective 'else'.
- Refactor code regularly to maintain clarity, especially during expansions or additions.
Consistency in practicing these strategies can help mid-level programmers refine their skills.
Community Participation Highlights
Participation in coding forums can expose one to innovative uses of the 'else' statement. Websites like Reddit and Stack Overflow are rife with discussions that dissect common scenarios in use cases. These platforms allow coders to share insights related to effective implementations of 'else', creating a richer understanding across many real-world applications.
Prelude to Control Flow in Python
Control flow is a fundamental concept in programming, particularly in Python. It determines how a program executes its statements and makes decisions based on various conditions. Understanding control flow allows programmers to manage the flow of execution effectively, which is crucial for creating logical and efficient code.
In Python, control flow is primarily handled through conditionals, loops, and exception handling mechanisms. By mastering these elements, developers can create programs that respond dynamically to user input and other factors. For this reason, the 'else' statement has notable significance within control flow structures.
Understanding Conditional Statements
Conditional statements in Python are the logical foundations on which control flow builds. These statements evaluate certain conditions and execute specific blocks of code when the conditions are met. The basic structure includes keywords such as , , and .
- The statement tests a condition. If the condition is true, it executes the corresponding block.
- The (else if) statement offers an additional condition check if the previous was false.
- The statement serves as a catch-all, executing its block when none of the prior conditions are satisfied.
By using these statements correctly, programmers can construct decision trees that follow logical paths. This not only makes code more efficient but also enhances clarity and maintainability.
Overview of Logical Branching
Logical branching refers to the routes a program can take based on conditions. It helps direct the flow of a program and contributes significantly to its functionality. Different paths in the flow allow developers to respond to varied situations and variables. Through structured logical branching, it becomes easier to identify errors or steps that require rollback, improving overall robustness.
Additionally, incorporating the 'else' statement into the branching logic comes with important advantages. There may be scenarios where a direct result is needed when all other conditions fail. For example, providing a default outcome ensures that the program doesn't experience fatal errors due to unsatisfied conditions.
Summarizing these points highlights the crucial role control flow plays in Python, making it essential for effective programming.
Key takeaway: Mastering control flow, including the use of 'else', allows for precise, efficient, and logical programming in Python.
The 'else' Statement Defined
The 'else' statement serves a crucial role in Python's control flow mechanisms. It provides an alternative path when the preceding conditions specified in 'if' or 'elif' statements evaluate to false. Without the 'else' statement, the program's logic becomes cumbersome, as it lacks a clear resolution for scenarios which do not satisfy any preceding conditions. This contributes to enhanced readability and coherence in the code, allowing programmers to handle various outcomes in a structured manner.
An essential benefit of using 'else' is that it creates a sense of completeness in decision-making constructs. When a programmer establishes various routes based on specific conditions, the 'else' statement encapsulates the unexplained cases neatly, providing a default action. Additionally, this statement is instrumental in embracing strategies for cleaner error handling, leading towards more resilient code.
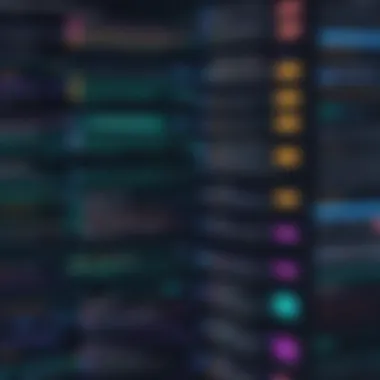
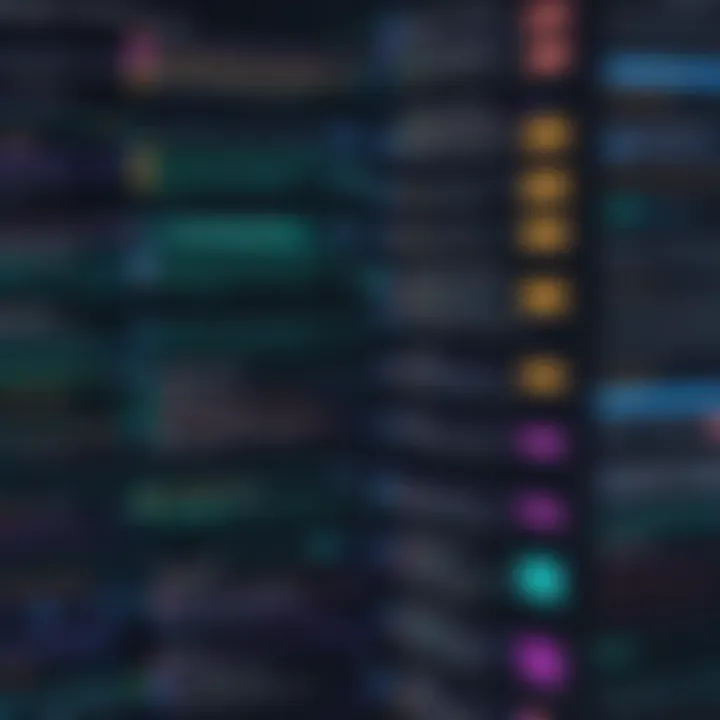
In sum, mastering the 'else' statement equips programmers to express logic precisely. It automates divergent paths within a program and aligns conditional efficiency with clarity. This skill is valuable across all experience levels, allowing both budding and professional developers to hone their craftsmanship with Python's control flow tools.
Basic Syntax of 'else'
The syntax of the 'else' statement is fairly straightforward yet powerful. To define it correctly, the statement follows after an 'if' or 'elif' block. Here is the basic syntax structure:
The 'else' statement must be indented to align with its associated 'if' statement, establishing a clear parentage in logic. This concatenation creates a linear flow across scenarios. Each statement under these control structures is only executed if its associated precondition holds true.
When structuring your code, it is essential to be conscious of placement and indentation. This ensures that there is a proper linkage recognized by Python's interpreter, minimizing logic errors that may arise from improper organization.
Combining 'else' with 'if'
The effectiveness of 'else' is most apparent when combined with 'if' statements. This combination allows programmers to dictate different outcomes based on varied conditions. Here’s how these two interact:
- Sequential Logic: When structured properly, an 'if-else' block will manage normal program flow, operating sequentially from the first true condition down to the 'else'.
- Short-Circuiting Conditions: Combining 'if' with 'else' means that once a true condition is met, the rest of the conditions, including that of 'else', are ignored. This optimizes processing and enhances performance.
- Clear Decision Trees: By effectively using 'else' alongside multiple 'if' statements, the program becomes a decision tree with divergent branches which simplifies complex condition handling.
It can be demonstrated through this illustrative code:
In this example, the output skyline enhances clarity: the response will change depending on the value assigned to age. The 'else' here captures any age situation that does not fulfill the preceding conditions specifically defined.
The 'else' statement effectively streamlines program logic, ensuring intuitive outcomes based on provided constraints.
Common Use Cases of 'else'
The 'else' statement serves several crucial functions in Python programming. Its applications are predominantly observed in conditional statements and control flow. Understanding how to use 'else' effectively can significantly improve the clarity and functionality of code. Here we discuss the salient use cases, which cover scenarios most programmers encounter regularly.
Single Condition Handling
The 'else' statement simplifies scenarios where a single condition directs the flow of the program. This consists of the statement followed naturally by . For example, if a basic check is required to determine if a user input meets certain criteria, the coding structure becomes clear after including 'else'. Here’s a succinct illustration:
In this example, if the input exceeds five, a message alerts success; otherwise, it notifies minimal input. Such clear two-way decision-making improves both the visibility and functionality of your code.
Multiple Conditions with 'elif'
When handling multiple conditions, 'else' jumps into action alongside . This allows programmers to manage a myriad of states without cluttering their code with additional nesting. The usage remains straightforward and maintainable. Consider the advanced setup below:
In this piece, three outcomes occur relative to 'user_input'. Here, 'else' collects cases where the user’s input does not meet earlier conditions, acting as a catch-all. Such constructs add significant understanding and control mechanisms to Python programs, improving their robustness during employ.
Default Case in Conditional Structures
'else' predominantly provides a default case, that comes exceptionally handy when no listed criteria are satisfied. Including this fall-back option not only enhances the logical flow but also minimizes the requirements for exhaustive conditions. Building on the previous concepts, it becomes clear how essential this aspect can be. An example may illustrate:
In this instance, if the server status code returns something odd or unanticipated, the 'else' section gracefully defaults to informing the developer of this. This usage helps in easily addressing unforeseen situations, thereby making error handling more manageable and recent. An integral understanding of the role of 'else' ensures that even edge cases in code are prudently controlled.
The ability to effectively utilize 'else' statements in Python structures not only mitigates the risk of recessive logic but also ensures functional clarity across extensive systems.
Iterative Constructs Involving 'else'
In Python, control flow statements enable efficient decision making and channel execution paths. One intriguing aspect of this is how the 'else' statement integrates with iterative constructs like loops. When the 'else' statement pairs with loops, it extends programmability and provides additional outcomes based on loop termination status. This can enhance the experience of both intermediate and advanced programmers, facilitating more elegant and readable code.
Using 'else' with Loops
When loops are employed in code, situations can arise where verification of success or failure needs clear indication. Iterative constructs in Python, such as and , allow an 'else' clause to be used after their blocks. This usage not only makes the flow of control more streamlined but it can also eliminate the need for separate flags or additional checks. In essence, an 'else' clause after a loop executes only if the loop completes without hitting a statement, thus allowing clearer logic flow.
Consider the following situation:
In this example, if the loop finishes without discovering the number 3, the clause will execute. Such behavior directly refers to the value of the clause utilized with loops, and thus makes certain iterations more straightforward to implement.
Behavior of 'else' in For Loops
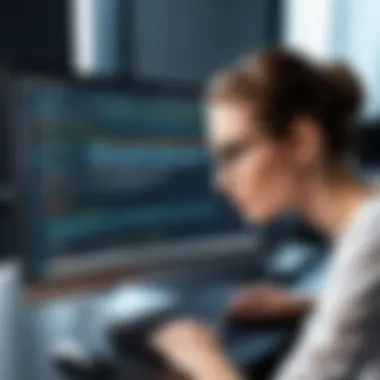
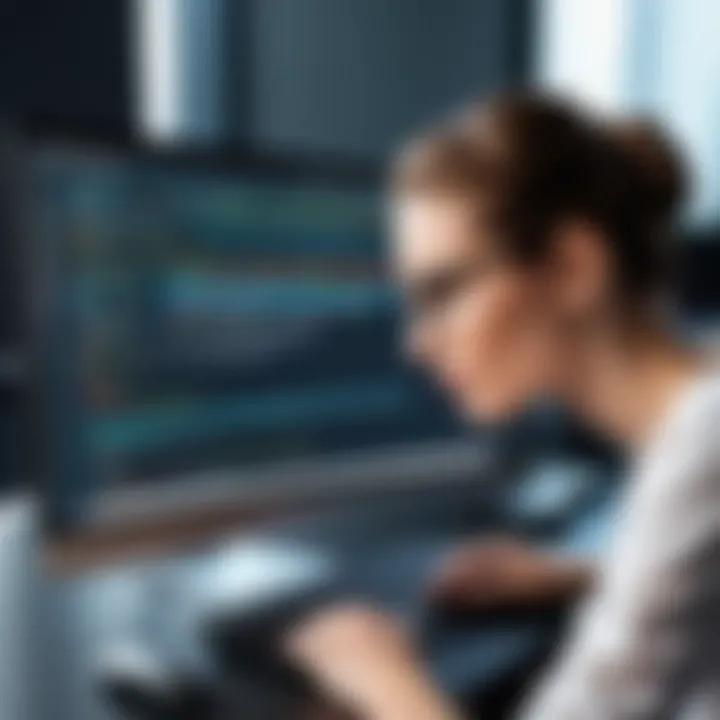
When it comes to loops, the presence of the 'else' can bring specific advantages, particularly during iterations over collections. The 'else' executes if the entire for loop runs without interruption from a statement. This approach eliminates the complex logic often needed to ascertain if a condition was ever satisfied during the loop’s execution.
Here is an example demonstrating how a loop behaves:
In this case, because there is no bird in the list, the clause indicates that no matches were met throughout each iteration, effectively feeding back important information without added complexity.
Behavior of 'else' in While Loops
The application of 'else' within loops follows a similar trajectory to that of loops although methodologies and practical utility can diverge slightly. When working with , the interaction between conditions will direct the flow. Like , the value triggers only when the loop terminates normally — that is, the condition of the evaluates as False — and is not redirected with the use of .
For instance:
Here, we've laid out a basic loop producing an increasing sequence of numbers. After 0 through 4 has been printed, the else statement indicates successful completion. The rests cogently positioned, enabling visibility into execution states.
In summary, using 'else' in both and loops allows cleaner delineation of the loop's result. It significantly aids in enhancing code readability, ultimately leading to cleaner logic flow. Programmers who master this functionality can derive numerous benefits from their iterative constructs. This is just one avenue for advanced usage of 'else', showing how, when leveraged effectively, it can enhance procedural clarity.
Error Handling with 'else'
Error handling is an essential part of programming. In Python, using the statement in conjunction with try-except blocks provides a structured way to manage exceptions. This enhances the robustness of the code by allowing specific actions to occur when a block of code runs without errors.
Integrating in your error handling workflow can improve clarity and make your intentions within the code clearer for others.
In this section, we will explore how to effectively integrate into try-except constructs, as well as the scope of related to exception handling.
Integrating 'else' in Try-Except Blocks
The presence of the clause following a try-except block can be quite beneficial. When a try block executes successfully without raising an exception, the code inside the block is run. The benefits of this are manifold:
- It promotes clear separation between normal operations and exception handling.
- Enhances readability, which is invaluable in maintaining the code.
- Reduces logic errors caused by placing regular code within the try block.
Here’s a simple code example illustrating this concept:
In this piece of code, if runs smoothly without any exception, the block will execute. This form of control flow makes intentions explicit, thus aiding future programmers in grasping the functioning without sifting through exception handling logic.
Scope of 'else' in Exception Handling
Understanding the scope of the clause is crucial when constructing error handling scenarios. The role of is limited to situations where the try block executes without error.
Some critical elements involve:
- The clause only executes if no exceptions are raised in the preceding . In any other case, it remains unexecuted.
- It reflects the exact flow of logic post the successful completion of the risky operation.
- Therefore, variables initialized or modified inside the try block are usable in the else block.
It's importan to consider that mixing exception handling code with normal operation code can lead to convoluted designs. To enforce cleaner designs, make sure the remains singularly focused on the successful resolution of the operation. Through this approach, you can cultivate a fulfill listing of operations intended for successful execution, greatly enhance comprehension of the workflow involved, and simplify future modifications of the code. As part of your overall development strategy, utilize these concepts to craft better exception-handling structures within your codebases.
Remember, structuring code this way encourages best practices while adhering to principles of clean code.
Activities revolving around error handling molds programming reliability. As we navigate through complex applications, the clarity and compartmentalization around become paramount. Through understanding and adhering to its principles within error handling constructs, we pave the path towards effective code when utilizing Python.
Best Practices for Using 'else'
Utilizing the 'else' statement correctly is crucial for ensuring maintainability and clarity of code. Adhering to best practices can greatly enhance how 'else' is use in your coding projects. The significance of this subject matter cannot be overstated. Many programmers struggle with control flow management, often overlooking 'else' or using it inappropriately. This section sheds light on the fundamental principles that guide its effective application.
Clarity and Readability Considerations
Clarity is paramount in programming. When using 'else,' consider how it impacts the readability of your code. The intent of a code section should be instantly recognizable.
- Keep Conditions Simple: The more complex a condition is, the more difficult it is to read. Strive for simplicity, ensuring that conditions inside an 'else' statement are clean and direct.
- Proper Indentation: Use consistent indentation to differentiate between conditional statements. This aids in visual segregation and hierarchy of logic.
- Meaningful Naming: When your 'else' relates to specific variables or functions, use descriptive names. For examples:
- Instead of using A clearer usage helps future programmers understand context immediately.
Being intentional about these considerations leads to fewer bugs and faster comprehension for anyone reviewing your work.
Avoiding Common Pitfalls
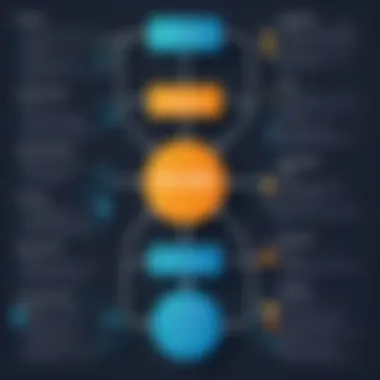
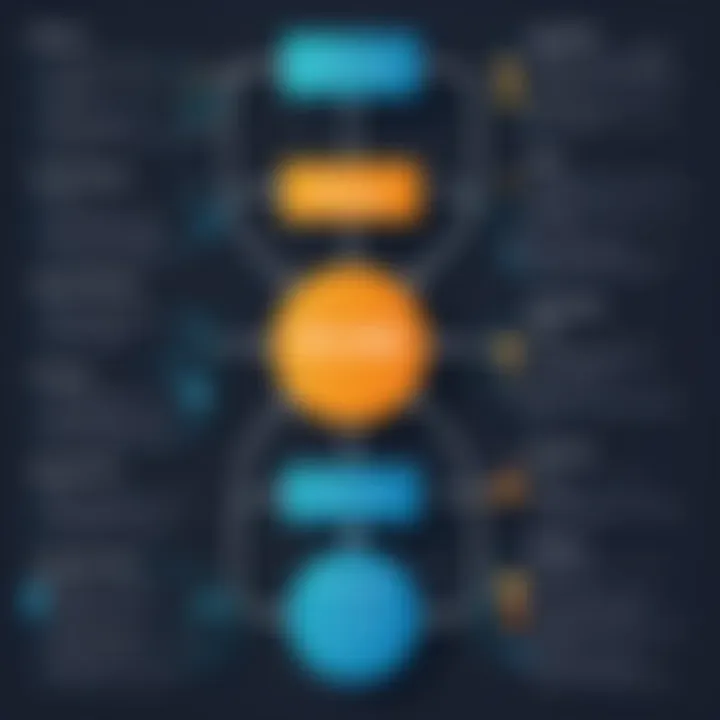
Every programmer encounters stumbling blocks. Recognizing and evading typical errors is vital for efficient programming with 'else'. Here are a few traps to avoid:
- Too Many Nested Ifs: Over nesting complicates logic flow. Instead of using multiple 'if-elif-else' structures, aim for clarity. Refactor into functions where possible.
- Using 'else' Imprudently: Sometimes skipping the 'else' statement is more logical. If your logic can stand without it, it often reduces unnecessary clauses.
- Ignoring Edge Cases: Be sure to account for scenarios that can break your logic outside of standard conditions. Not addressing edge cases results in errors that will often confuse.
- Avoid deep nesting: Consider separating conditions into smaller parts. When you find you're nesting further, your logic is likely too complex.
Employing these best practices strengthens your understanding and application of 'else' in Python, enhancing both code quality and personal growth as a programmer. As your proficiency increases, focus not only on functional code but also on elegant solutions that can withstand scrutiny while remaining accessible to readers.
In summary, effective coding goes hand-in-hand with the practice of maintaining clarity and the wisdom to bypass pitfalls. Those who apply these principles will inevitably create more robust programming solutions.
Advanced Concepts in Control Flow
Control flow is a fundamental aspect of programming that allows developers to manage the execution path of their code. In this article section, we focus on the advanced concepts that expand on basic conditional logic, particularly with the 'else' statement in Python. Understanding these concepts enhances not only how code flows but also increases efficiency and maintainability in programming.
With programming languages, especially Python, advanced control flow allows for complex decision-making processes. Techniques like nested conditional statements and how 'else' functions in function definitions do clarify patterns of logic that might not be intuitive to every developer. Being knowledgeable about these advanced constructs lets programmers create more concise and effective code.
Nested Conditional Statements
Nested conditional statements refer to the scenario where an 'if' or 'else' statement is placed within another 'if' or 'else' statement. This method allows for creating multiple layers of logic, enabling more selective reasoning. For instance, when handling scenarios with multiple criteria, nesting enhances the granularity of conditional checks.
Syntax of Nesting
In Python, nesting works seamlessly, adhering to the same principles you find with standalone conditionals. Consider the following example:
Here, we check if is above 18 first. If true, we further check if the age qualifies for 'Senior Citizen.' This structure allows developers to handle conditions with precision.
Benefits of Nesting
Nested statements offer multiple layout options when it comes to decision paths. However, several things need to be considered; for example:
- Clarity: Too many nesting can lead to confusion. Each layer must add, not complicate.
- Readability: Overly nested statements may affect maintainability. Therefore, strive for simplicity over complexity.
Using 'else' in Function Definitions
The placement of the 'else' statement in function definitions marks an essential aspect of structuring Python code. Functions can have strategic 'else' executions, which can guide the pathway after certain expected actions. Structurally, using 'else' within a function can provide fallback actions if the preliminary conditional checks do not pass.
Example of 'else' in Functions
Consider this function that assesses a number:
The function implements various return paths based upon the value of . If it bypasses the first two conditions, the 'else' statement handles outputting a neutral response. In such cases, returning appropriate outcomes is crucial for reliability in logic flow.
Key Considerations
When using 'else' in this manner, several considerations emerge:
- Ensure the last path clearly defines expected outcomes.
- Focus on definitive states within logical function execution.
Employing advanced control strategies and being adept with functions that employ 'else' can greatly enhance clarity in Python programming.
Closure
The function of in Python serves as a crucial aspect within control flow mechanisms. This article underlines its role not only in standard conditional expressions but also in iterative constructs and exception handling scenarios.
The structured understanding of the statement enhances clarity while coding. It allows programmers to plan alternative pathways through code, leading to straightforward yet effective logical progressions. This can greatly help a programmer during troubleshooting, revealing unobvious logic flaws or missed conditions. Overall, the capabilities imparted by foster improved readability and maintainability, which are essentials in programming best practices.
Recap of 'else' Utility
The statement helps simplify complex control flows by providing fallback options. By incorporating it, one can avoid considerable nesting of statements, thereby sharpening clarity. The inability to fit results into standard - code can quickly be responded to with a fallback through , whereby specific scenarios can be covered without excessive additional lines of code. This succinct method invites efficiency and better structure in program logic.
Moreover, 's utility extends beyond mere condition evaluation; it can be pivotal in maintaining smooth iterations in a variety of loops such as and statements, fundamentally ensuring logic that operates without exceptions even when conditions are unmet.
Future Directions in Python Control Flow
As Python continues its journey in evolving impactful programming paradigms, control flow enhancements present exciting possibilities. Among these, potential modifications to usability could be proposed to support combinatorial logic or more complex branching. Scope for extension might see functions adopting the behavior characteristics observe in pattern matching, a concept gaining traction.
Additionally, the exploration of leading frameworks introducing alternatives could offer deeper methodologies to rendering conditionality and exception understanding even clearer.
Fostering a streamlined, efficient control flow aids not just one programmer but entire teams. Keeping up with potential future adjustments to the language enables everyone familiar with it to remain adept and ready to capitalize on emerging features.
Thus, while serves its present purpose within Python’s syntax and usages, its future remains open to innovation, promising enhancements that cater to ever-developing programming needs.
The visualization of logic pathways aided by instance cultivation is a straight narrative of usability in varied developments - programming is about solving problems, after all.