Maximizing Java Validation Techniques: A Comprehensive Exploration
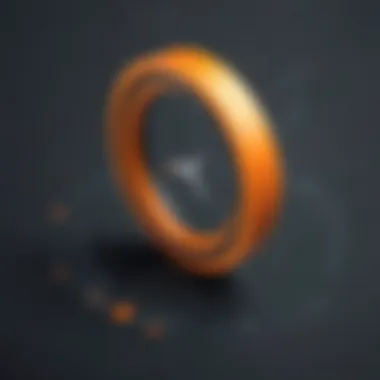
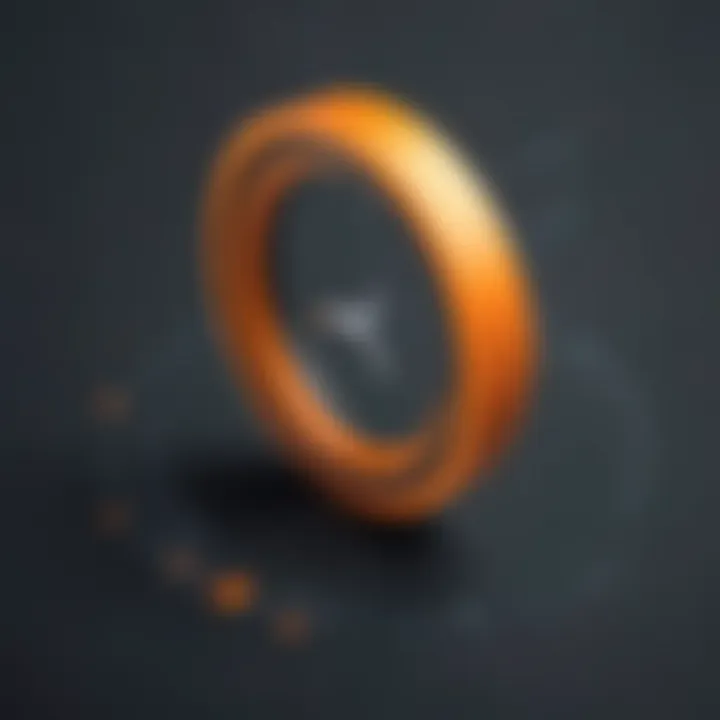
Coding Challenges
Write here about how Java validation poses unique challenges for programmers. Explore the nuances of error handling, input validation, and data sanitization within Java applications. Discuss the importance of meticulous coding practices to ensure the robustness and security of the validation process. Break down common issues that developers encounter during validation tasks and provide practical solutions to streamline the coding workflow. Highlight the significance of staying updated with industry best practices and leveraging efficient algorithms for effective validation implementations.
Technology Trends
Delve into the latest technological trends shaping the landscape of Java validation. Explore how advancements in AI, machine learning, and automation are influencing validation practices in Java development. Analyze the impact of emerging technologies like blockchain and IoT on the validation process, emphasizing the need for adaptive strategies to meet evolving industry requirements. Incorporate expert opinions and case studies to illustrate the practical implications of integrating cutting-edge technology trends into Java validation workflows.
Coding Resources
Provide a curated list of valuable resources for Java developers looking to enhance their validation skills. Present comprehensive programming guides that cover essential topics such as regex patterns, error handling techniques, and efficient data validation strategies. Review top tools and software applications designed to simplify the validation process and improve code quality. Offer in-depth tutorials and instructional articles that cater to programmers at different skill levels, equipping them with the necessary knowledge to elevate their Java validation capabilities.
Computer Science Concepts
Explore key computer science concepts that underpin Java validation methodologies. Explain the fundamental principles of algorithms and data structures relevant to efficient validation code. Introduce basic concepts of artificial intelligence and machine learning that can be leveraged to enhance validation accuracy and performance. Discuss the importance of networking and security fundamentals in ensuring data integrity during validation processes. Provide insights into emerging technologies such as quantum computing and their potential impacts on the future of Java validation practices.
Introduction to Java Validation
In the realm of Java programming, validation occupies a pivotal role in ensuring the efficiency and accuracy of code execution. Understanding the nuances of Java validation is fundamental for programmers seeking to optimize their applications and enhance data security. This section of the article delves deep into the core principles of validation, shedding light on its importance and application in Java development. By exploring various validation techniques and strategies, programmers can elevate their coding proficiency and minimize errors effectively.
Understanding the Role of Validation in Java Programming
The Concept of Data Validation
Data validation encompasses the process of verifying and ensuring that input data is accurate, reliable, and secure. In the context of Java programming, data validation plays a crucial role in safeguarding the integrity of applications by detecting and preventing erroneous or malicious data inputs. Emphasizing the validation of user inputs and system interactions, data validation mitigates the risk of data breaches and enhances the overall robustness of Java applications. Integrating comprehensive data validation mechanisms within Java code not only fortifies security measures but also optimizes performance by eliminating potential vulnerabilities.
Importance of Validation in Java Applications
The significance of validation in Java applications cannot be understated, as it serves as a cornerstone for maintaining data integrity and system reliability. By incorporating validation processes into Java programming, developers can enforce constraints, rules, and standards that ensure the consistency and accuracy of data handling. Validation acts as a gatekeeper, filtering out invalid inputs and safeguarding against data corruption or manipulation. Moreover, effective validation mechanisms bolster the overall quality of Java applications, instilling confidence in users and enhancing the software's credibility.
Common Challenges Faced in Java Validation
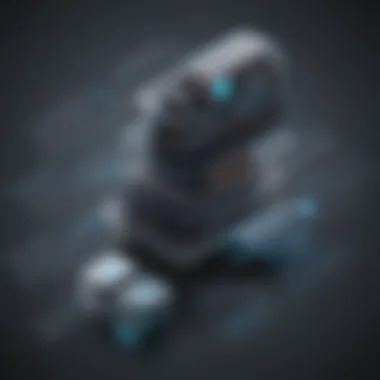
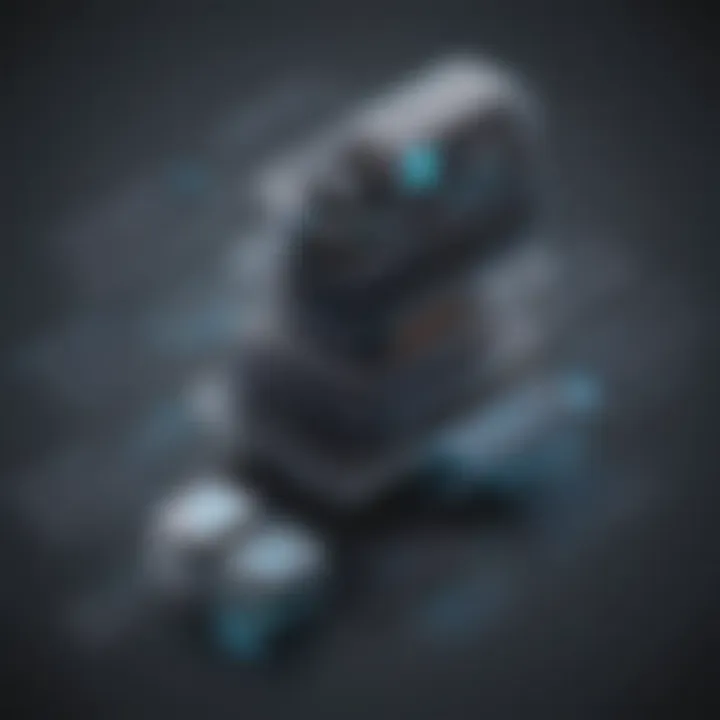
Handling Null Values
One prevalent challenge in Java validation pertains to the effective handling of null values within the codebase. Null values, which denote the absence of a meaningful data reference, can pose complications during validation processes, leading to runtime errors or unexpected behaviors. Addressing this challenge requires implementing robust null checks and validation protocols to manage and interpret null values accurately. By devising specific strategies to handle null scenarios, programmers can enhance the resilience of their validation mechanisms and ensure the seamless operation of Java applications.
Ensuring Data Integrity
Maintaining data integrity is paramount in Java programming, as it underpins the reliability and credibility of information processed within applications. Challenges related to data integrity in validation entail preserving the consistency and correctness of data throughout its lifecycle. By validating data inputs, enforcing constraints, and implementing error detection mechanisms, developers can safeguard against data manipulation, unauthorized access, and integrity violations. Striving to ensure data integrity in Java applications promotes trustworthiness, accuracy, and compliance with data protection standards.
Basic Java Validation Techniques
Using Built-in Java Validation Annotations
When it comes to Built-in Java Validation Annotations, specific annotations like @NotNull, @NotBlank, and @Size contribute significantly to the validation process outlined in this article. These annotations offer a convenient means to check for null values, ensure non-empty strings, and validate data sizes within Java applications. The @NotNull annotation, for instance, is widely used to enforce non-null values, enhancing the reliability of data inputs. Similarly, @NotBlank focuses on verifying the presence of non-empty strings, promoting data consistency. Moreover, @Size allows developers to validate the length of input values, ensuring compliance with specified constraints. Understanding the nuanced functionalities of these annotations provides programmers with powerful tools to streamline the validation process effectively.
@Min, @Max, @Email Annotations
In the context of Java validation, annotations such as @Min, @Max, and @Email offer distinct functionalities that contribute to the overarching goal of validating data comprehensively. The @Min annotation enables validation based on minimum values, setting thresholds for acceptable input ranges. Conversely, the @Max annotation establishes upper limits for data values, preventing inputs that exceed defined boundaries. Additionally, the @Email annotation serves as a specialized validator for email addresses, ensuring the proper format and structure of email inputs. By incorporating these annotations into Java validation logic, programmers can implement precise data validation rules that improve the quality and reliability of their applications.
Implementing Custom Validation Logic
Incorporating Custom Validation Logic into Java programming elevates the validation process to a more personalized and specialized level. The concept of Creating Custom Validators enables developers to tailor validation rules specific to their application requirements. By creating custom validators, programmers can address unique validation scenarios that may not be covered by standard validation annotations. This customization empowers developers to enforce complex validation criteria, validate diverse data types, and adapt the validation process to the intricacies of their software. Additionally, Defining Validation Constraints allows developers to establish precise rules and conditions for validating input data. Defining constraints encapsulates the validation criteria within the application's logic, ensuring thorough data validation and adherence to predefined standards. Implementing custom validation logic not only enhances the effectiveness of validation processes but also enables greater flexibility and customization in handling data validation tasks.
Advantages and Disadvantages of Custom Validation Logic
The advantages of implementing Custom Validation Logic include tailored validation rules, enhanced data accuracy, and the ability to address specific validation requirements effectively. By customizing validation logic, developers can fine-tune the validation process to suit the unique needs of their applications, resulting in more precise error detection and resolution. However, the customization aspect may also introduce complexity and additional development efforts, requiring meticulous planning and implementation. Moreover, defining validation constraints offers developers a structured approach to validation, ensuring consistency and reliability in data validation procedures. While custom validation logic provides unmatched flexibility and control over the validation process, it necessitates careful consideration of design choices and continuous refinement to optimize the validation workflow.
Advanced Strategies for Java Validation
In the realm of Java programming, the utilization of advanced strategies for validation holds paramount significance. Going beyond basic validation techniques, advanced strategies provide programmers with a sophisticated toolkit to ensure data accuracy and application robustness. These strategies play a pivotal role in fortifying the overall integrity of Java applications by implementing nuanced validation protocols that go beyond surface-level checks. By delving deep into advanced strategies for Java validation, programmers can elevate their coding capabilities and achieve a higher level of precision in data validation procedures. The meticulous implementation of these strategies can lead to enhanced application security, streamlined data processing, and improved user experience.
Utilizing Validation Groups
Grouping Validation Constraints
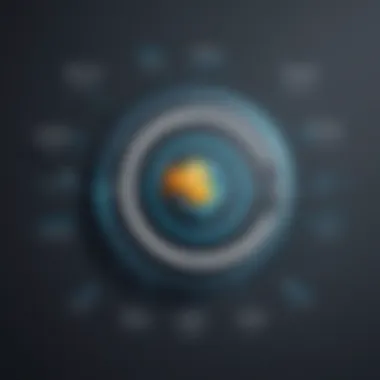
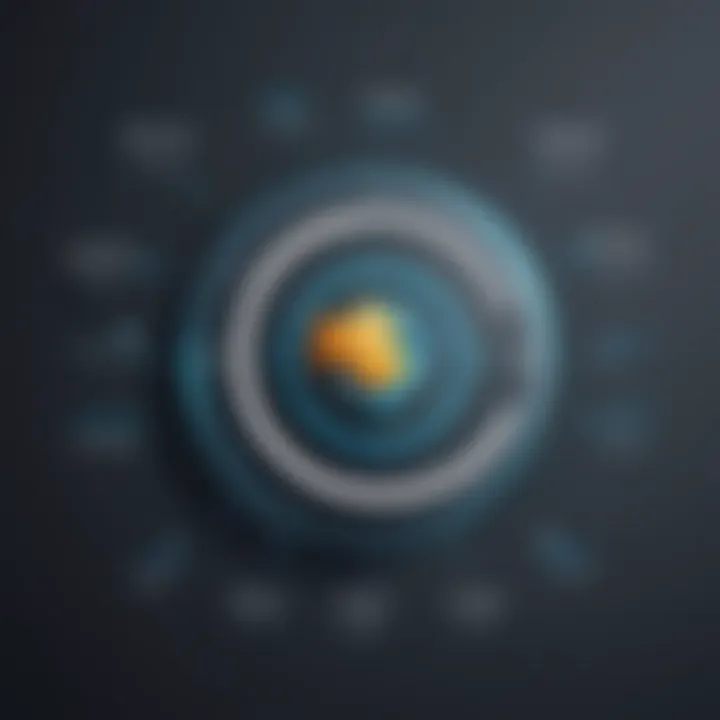
When it comes to the concept of grouping validation constraints, the overarching objective is to streamline and organize validation rules based on specific criteria. By categorizing validation constraints into logical groups, programmers can enhance code readability, maintainability, and scalability. This structured approach to validation simplifies the debugging and maintenance processes, making it easier to add or modify validation rules as needed. The key characteristic of grouping validation constraints lies in its ability to encapsulate related validation rules, allowing for efficient management and application of validation logic. This organizational method proves to be a popular choice in Java validation as it promotes code reusability, minimizes redundancy, and fosters a systematic validation framework within Java applications.
Conditional Validation
Conditional validation introduces a dynamic element to the validation process, enabling programmers to define validation rules based on specific conditions or states. This adaptive approach to validation empowers developers to tailor validation logic according to variable scenarios, enhancing the flexibility and adaptability of data validation mechanisms. The crux of conditional validation lies in its responsiveness to contextual factors, ensuring that validation rules are applied judiciously based on predetermined criteria. By incorporating conditional validation in Java applications, programmers can fine-tune validation protocols to accommodate diverse user inputs, environmental factors, or business requirements. This customization feature of conditional validation adds a layer of sophistication to Java validation procedures, enabling precise control over data validation outcomes and error handling mechanisms.
Cross-Field Validation Techniques
Dependency Validation
Dependency validation pertains to the interrelation between data fields within Java applications, where the validity of one field depends on the value or state of another. This technique allows programmers to establish validation rules that span multiple fields, enforcing data coherence and consistency across interconnected data points. The key characteristic of dependency validation lies in its ability to maintain data integrity by verifying dependencies and relationships between fields. By enforcing dependency validation, programmers can prevent data inconsistencies and ensure that linked data fields remain synchronized and logically sound. This approach proves to be beneficial in scenarios where data dependencies dictate the validation criteria, necessitating a coordinated approach to data validation within Java applications.
Applying Rules Across Multiple Fields
Applying rules across multiple fields involves the implementation of validation protocols that consider the collective state of interconnected data fields. This technique enables programmers to define comprehensive validation criteria that encompass the interaction between various data elements, enhancing the holistic validation process. By setting rules that span multiple fields, programmers can address complex data validation scenarios that require cross-referencing and contextual validation. The unique feature of applying rules across multiple fields lies in its ability to assess data cohesiveness and conformity across diverse sets of information. This comprehensive validation approach facilitates the detection of data anomalies, dependencies, and inconsistencies, ensuring a thorough validation process that takes into account the interconnected nature of data within Java applications.
Integration with Spring Validation Framework
Spring Validator Interface
The integration of Java validation with the Spring framework introduces the Spring Validator interface, a key component that facilitates the seamless execution of validation logic within the Spring ecosystem. This interface serves as a bridge between Java validation mechanisms and the Spring framework, allowing developers to leverage Spring's robust features for effective data validation. The key characteristic of the Spring Validator interface lies in its interoperability with Spring components, enabling the integration of validation rules within Spring-managed beans and workflows. By utilizing the Spring Validator interface, programmers can enhance the validation capabilities of their applications, tapping into Spring's dependency injection, aspect-oriented programming, and declarative transaction management functionalities. This seamless integration streamlines the validation process, improves code modularity, and fosters synergy between Java validation practices and the Spring framework.
Binding Error Messages
Binding error messages play a crucial role in the validation feedback loop, providing informative notifications to users regarding validation failures. These error messages are bound to specific validation conditions, offering precise details on the nature of validation errors and corrective actions to be taken. The key characteristic of binding error messages lies in their contextual relevance to validation outcomes, enhancing the user experience by offering clear and actionable feedback. This feature proves to be a popular choice for Java validation as it promotes user engagement, error resolution, and transparency in data validation processes. By incorporating binding error messages in Java applications, developers can enhance user understanding of validation requirements, mitigate user errors, and improve overall application usability.
Best Practices and Tips for Java Validation
In this section, the focus shifts towards the vital aspects of executing best practices and incorporating helpful tips for Java validation within the comprehensive guide. Emphasizing the significance of this topic, it is crucial for programmers looking to optimize their Java validation processes efficiently. By adhering to best practices and leveraging expert tips, developers can elevate the quality and security of their Java applications significantly. With a keen eye on details, meticulous execution of validation procedures ensures data integrity, minimizes errors, and fortifies the overall robustness of the application.
Ensuring Secure Input Validation
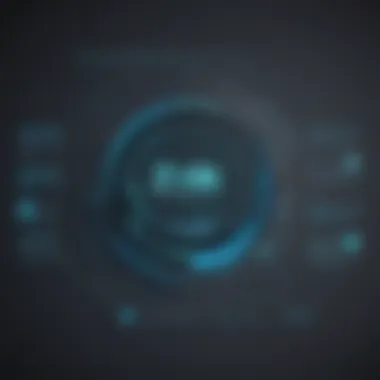
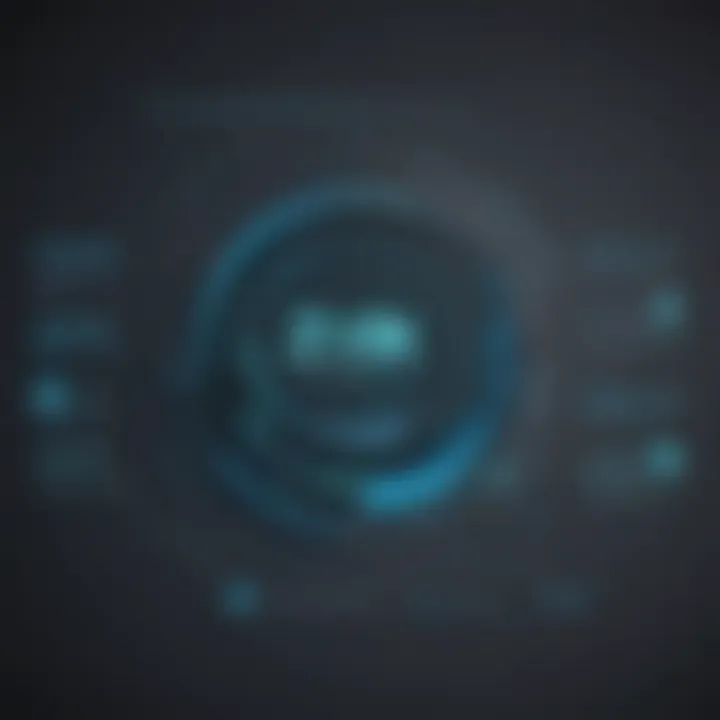
In the realm of Java validation, ensuring secure input validation is a top priority. One key element under this domain is the practice of sanitizing user input. Sanitizing user input involves cleansing and validating incoming data to mitigate the risks of potential vulnerabilities and exploits. This procedure acts as a shield against malicious attacks such as SQL injection or Cross-Site Scripting, safeguarding the application's integrity and user data. The meticulous scanning for suspicious inputs and the removal of harmful elements underscores the importance of user input sanitization within the validation process.
When it comes to avoiding injection attacks, Java programmers must adopt stringent measures to prevent unauthorized access to the system through tainted data inputs. By implementing strategies to identify and neutralize injection threats, developers can uphold the sanctity of their applications and prevent security breaches effectively. The proactive stance against injection attacks not only bolsters the application's resilience but also instills confidence in users regarding the system's trustworthiness.
Handling Validation Errors Gracefully
In the pursuit of flawless Java validation, handling validation errors in a user-friendly manner is paramount. Displaying user-friendly messages serves as a beacon of clarity for users encountering validation issues, aiding in prompt error identification and resolution. The crisp and concise error messages provide users with actionable insights, enabling them to rectify their inputs and proceed seamlessly within the application environment.
Logging and tracking validation failures offer an invaluable resource for developers striving to monitor and analyze the occurrence of errors systematically. By maintaining a log of validation failures, programmers can trace back and pinpoint the root causes of these failures, facilitating efficient troubleshooting and refining the validation logic. This systematic approach not only streamlines the error resolution process but also contributes to enhancing the overall stability and performance of the Java application.
Continuous Improvement in Validation Processes
Within the realm of Java validation, continuous improvement in validation processes emerges as a cornerstone for sustained excellence. Incorporating feedback mechanisms enables developers to gather valuable insights from users and stakeholders regarding the effectiveness of the validation mechanisms deployed. This iterative feedback loop fosters ongoing enhancements, ensuring that the validation processes align with evolving user requirements and industry standards.
Regular review and refinement play a pivotal role in the optimization of validation processes, underscoring the need for periodic evaluation and enhancement. By subjecting the validation procedures to regular scrutiny and refinement cycles, developers can fine-tune the validation logic, address emerging challenges, and adapt to the dynamic landscape of security threats proactively. This iterative refinement process fortifies the validation framework, elevates the application's quality, and safeguards against potential vulnerabilities effectively.
Conclusion
In delving deep into the complex world of Java validation code, this article has provided a comprehensive guide that equips programmers with advanced techniques to enhance their validation processes effectively. The significance of the conclusion lies in consolidating the key insights discussed throughout the article, emphasizing the critical role validation plays in optimizing Java applications for improved functionality and security. By summarizing the core takeaways and offering a forward-looking perspective, the conclusion encapsulates the essence of elevating Java validation practices to meet evolving industry standards and user expectations.
Summary of Key Points
Importance of Robust Validation:
The robust validation framework plays a pivotal role in fortifying Java applications against vulnerabilities and ensuring data integrity. By incorporating stringent validation measures, programmers can safeguard against potential security breaches and data manipulation risks, elevating the overall reliability and robustness of their applications. The meticulous validation process not only enhances the user experience but also mitigates the likelihood of errors and malfunctions, thus contributing significantly to the seamless operation of Java programs.
Enhancing Java Application Security:
The enhancement of Java application security through rigorous validation practices is paramount in today's digital landscape. By prioritizing security protocols and integrating sophisticated validation mechanisms, programmers can mitigate cybersecurity threats and bolster the resilience of their applications against malicious attacks. The stringent validation criteria not only protect sensitive data but also instill user trust and confidence in the application's ability to maintain confidentiality and integrity, establishing a solid foundation for secure digital interactions.
Looking Ahead
Future Trends in Java Validation:
As the technological landscape evolves, future trends in Java validation are poised to revolutionize the validation process, offering advanced capabilities and functionalities to streamline validation workflows. From leveraging machine learning algorithms for automated validation to incorporating blockchain technology for enhanced data security, the future holds exciting prospects for Java developers seeking to optimize their validation processes and stay ahead of industry trends.
Innovations in Validation Technologies:
Innovations in validation technologies are catalyzing a paradigm shift in the way programmers perceive and implement validation strategies. With the advent of cloud-based validation services and real-time validation frameworks, developers can harness cutting-edge technologies to enhance the precision and efficiency of their validation efforts. These innovations not only expedite the validation process but also enhance scalability and adaptability, empowering programmers to address diverse validation demands with agility and precision.