Mastering Error Handling in PostgreSQL with Try-Catch
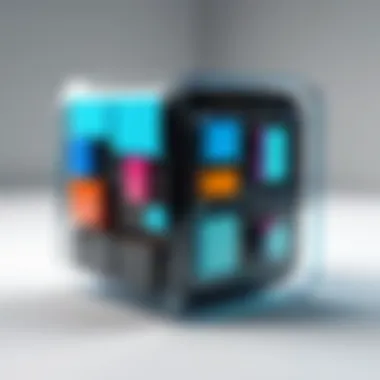
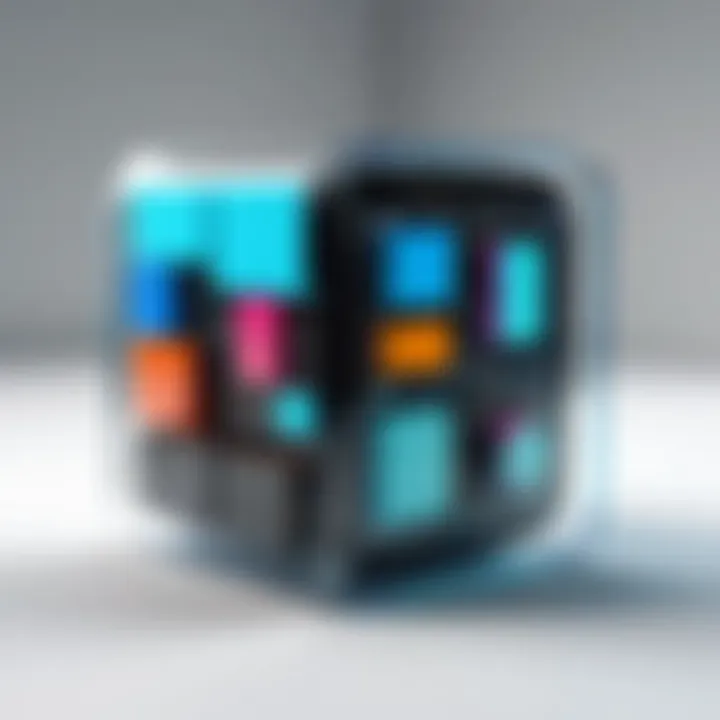
Intro
In the world of databases, handling errors gracefully can often mean the difference between a smooth user experience or a cascade of issues that leave users frustrated. PostgreSQL, a very popular relational database, offers robust techniques for error management, particularly with its try-catch constructs. By mastering how to effectively handle exceptions in PostgreSQL, developers can ensure that their applications are resilient and maintain a high level of integrity, even amidst errors.
This article aims to explore the various layers of error handling, focusing on how to implement try-catch blocks to manage exceptions effectively. We will dive into the mechanics of PostgreSQL's error handling framework, best practices for implementation, and practical examples that illustrate common scenarios and pitfalls.
Whether you're a seasoned database administrator or just starting on your SQL journey, understanding these concepts will bolster your capabilities and equip you to handle exceptions with grace. Let’s embark on this deep dive together.
Understanding Error Handling in PostgreSQL
Error handling in PostgreSQL plays a crucial role in maintaining the integrity and reliability of database operations. Without proper error management, even minor hiccups can snowball into severe issues, leading to data corruption, lost transactions, or even application crashes. As databases are at the heart of numerous applications and systems, understanding how to handle errors effectively is paramount for developers and administrators alike.
The Importance of Error Handling
Error handling isn't just a technical requirement; it's a best practice that aligns closely with principles of reliability and user satisfaction. When developers ignore errors or handle them poorly, they set themselves up for a world of hurt. Here are a few reasons why error handling should sit at the top of your database management checklist:
- Data Integrity: Robust error handling ensures that the data remains accurate and consistent. Any failed transactions can be rolled back, preventing partial updates and maintaining a stable state.
- User Experience: Errors can happen, that's a given, but how an application responds to those errors can make all the difference. Instead of tedious system failures, users can be presented with graceful error messages, allowing them to understand the issue without unnecessary confusion.
- Debugging Made Easier: Knowing where and why errors occur allows developers to iterate quickly without getting lost in the weeds. Effective logging and exception handling tools mean that developers can focus on resolving issues instead of sifting through mountains of data.
In short, effective error handling can save time, reduce frustration, and maintain trust—all essential for keeping your applications running smoothly in a production environment.
Overview of Exception Management
Understanding exception management involves recognizing how PostgreSQL categorizes and processes errors. In many ways, exceptions can be viewed as a safety net, catching problems that arise during database operations.
In PostgreSQL, exceptions are classified into two broad categories:
- User-defined exceptions: These are custom errors defined by developers. They can be tailored to specific scenarios, making them versatile for complex applications.
- System-defined exceptions: These arise from the database system itself, ranging from constraint violations to deadlocks. Understanding their nuances helps developers know what to expect during runtime.
The error messages generated convey crucial information, such as the nature of the error and the SQL command that triggered it. By systematically handling exceptions, developers can not only address issues as they occur but also prevent them from disrupting user workflows.
"Effective error handling is not just about catching the problem; it's about understanding the context and building a resilient system that can adapt and recover."
Implementing a solid framework for handling these exceptions enriches your overall database strategy. As applications grow in complexity, adapting how you manage exceptions will become increasingly important.
Prologue to Try-Catch in PostgreSQL
In the world of database management, the ability to handle errors gracefully can make or break the integrity of your applications. When working with PostgreSQL, understanding the try-catch constructs becomes crucial for ensuring that unexpected incidents do not disrupt your workflows. Try-catch not only mitigates errors but also enhances the reliability of your database operations. This section outlines what try-catch is, along with its significance in your PostgreSQL programming.
Definition of Try-Catch Constructs
Try-catch constructs in PostgreSQL are essential tools for error handling within PL/pgSQL, the procedural language used to write custom functions and trigger procedures in PostgreSQL. Essentially, a try-catch block allows you to attempt a specific operation and catch any exceptions that might occur during its execution.
In simpler terms, the try block encapsulates the code you want to execute, while the catch block is the safety net—triggered only if something goes awry. Here’s a basic outline:
This high-level structure gives you control over your error management strategy, ensuring that potential mishaps do not cause catastrophic failures in your application. You can handle specific exceptions or general errors, tailoring your response based on the situation at hand, making your applications more robust.
Differences Between Try-Catch and Traditional Error Handling
When you compare try-catch with more conventional error handling methods often employed in programming, several critical distinctions arise. Traditional error handling might simply involve checking return codes or using flags to determine if an operation succeeded or failed. This method can easily become messy, leading to cluttered code and a higher likelihood of bugs.
In contrast, try-catch constructs offer a more elegant solution:
- Clarity: The separation of error handling from regular logic enhances code readability. With traditional methods, you often mix success and failure logic, making it harder to follow.
- Specificity: You can precisely define the type of exceptions you want to handle. This granularity enables tailored error responses—for instance, distinguishing between a connection failure and a data integrity issue.
- Isolation: By isolating sections of code that may fail, you reduce the risk of subsequent operations being impacted in the event of an error. This isolation leads to more stable applications overall.
One important point to note is that while try-catch offers numerous benefits, it's not without its considerations. Overuse can lead to performance overhead since exception handling isn't as lightweight as managing control flow using return codes or inline checks. Therefore, it's judicious to employ these constructs where they matter most—ensuring that your database logic remains efficient and effective.
"Effective error handling is not about avoiding errors, but about managing them wisely."
In summary, the introduction to try-catch in PostgreSQL opens the door to a more organized and effective way to handle exceptions, setting the stage for better database management practices.
Framework of Try-Catch Blocks
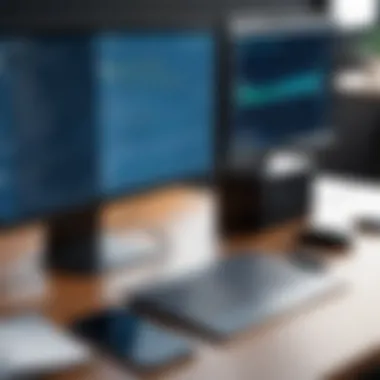
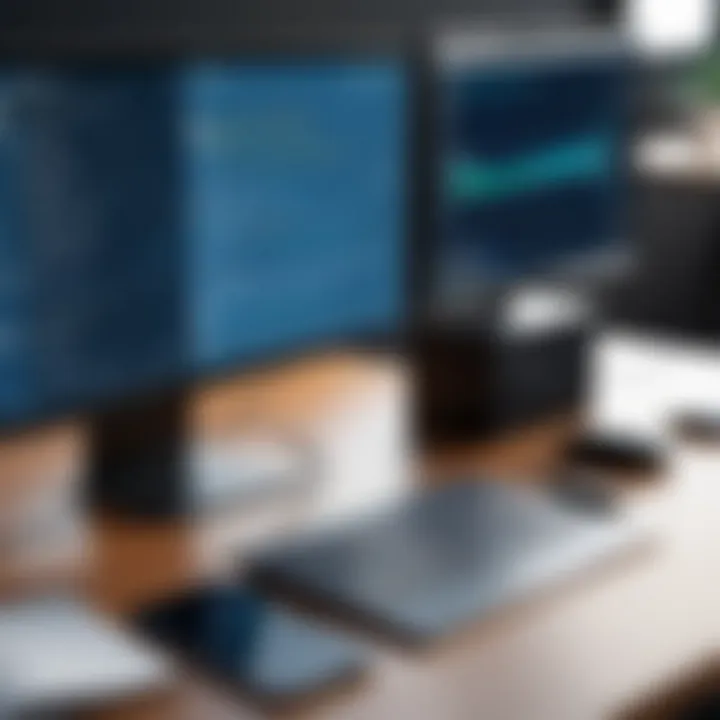
Error handling is a fundamental aspect of any robust database system, and PostgreSQL is no exception. Within this context, the framework of try-catch blocks emerges as an essential tool that permits efficient management of exceptions. Utilizing this construct, developers can catch errors before they escalate into larger problems, maintaining the integrity of their databases and ensuring smooth execution of scripts. The importance of this framework cannot be overstated, as it not only aids in debugging during development but also protects data during runtime in production environments.
Syntax of Try-Catch Statements
The syntax for try-catch statements in PostgreSQL can feel a bit like navigating through a maze at first, but once understood, it becomes second nature. A typical structure looks like this:
This basic layout allows the programmer to place SQL code that might trigger an error inside the BEGIN section. If an error arises, control shifts to the EXCEPTION block, where the programmer can define how to respond. For instance, if a unique constraint violation occurs, one might simply log the error or notify the user accordingly. Each possible error can be caught with a WHEN clause, allowing for targeted handling of various exceptions.
Context in Which to Use Try-Catch
Understanding when to employ try-catch constructs is as vital as mastering the syntax itself. Typically, you want to use these blocks in scenarios where a certain danger of failure exists. This could include:
- Database Transactions: Use try-catch to ensure that if a problem arises during a transaction, changes can be rolled back to preserve data integrity.
- Data Insertion or Update Operations: Errors during these operations, like constraint violations, are common, making try-catch particularly useful.
- Dynamic SQL Execution: When executing dynamic queries, the chances of runtime errors increase. A try-catch block can help manage these uncertainties gracefully.
Ultimately, implementing these constructs in the right contexts not only improves the reliability of your database operations but also enhances the user experience by providing clearer error messages rather than leaving users in the dark when issues occur.
Implementing Try-Catch in PL/pgSQL
Understanding how to implement try-catch constructs in PL/pgSQL is key to mastering error handling for developers and database administrators. It allows for better control over how errors are managed, paving the way for more stable database applications. Using try-catch helps in isolating the sections of code that may throw exceptions, meaning that one error won’t bring down the entire database transaction. This proactive management is especially crucial in multi-user environments where several transactions may occur simultaneously.
The benefits of implementing try-catch in your PL/pgSQL code cannot be overstated. By anticipating potential errors, developers can design code that not only operates efficiently under normal conditions but also gracefully manages unexpected situations. It's like preparing for a rainy day; you'd pack an umbrella rather than get soaked when the storm hits.
But what should you consider?
- Performance: While try-catch constructs enhance error handling, an overuse can lead to performance lags if not managed properly. Each error must be a calculated risk.
- Complexity: Adding layers of error handling can sometimes convolute code. Thus, it's vital to keep an eye on clarity for future maintainability.
- Error Types: Distinguishing between anticipated and unanticipated errors will equip programmers with the knowledge to build more robust error handling mechanisms.
In a nutshell, implementing try-catch is not just about catching errors; it’s about refining your approach to database management.
Basic Example of Try-Catch in Action
Let’s take a look at a simple example illustrating how to implement a try-catch block in PL/pgSQL. This example attempts to divide two numbers, handling division by zero errors deftly:
In this snippet, the command allows us to execute a block of PL/pgSQL code. The attempt to divide by zero will trigger an exception, which is caught, and a notice is raised instead.
Advanced Use Cases and Best Practices
Diving deeper into the world of try-catch implementations, there are advanced scenarios worth exploring. Here are a few common use cases:
- Transaction Control: When multiple data modifications are involved, it’s wise to use try-catch to rollback if an error occurs rather than partially applying changes.
- Logging: Errors can be logged to an error table for later analysis, making it easier to diagnose issues post-factum.
- Nested Blocks: Sometimes, it's essential to have try-catch blocks within other try-catch blocks, especially when dealing with complex procedures. Each layer needs its own handling logic.
Here are some best practices to comply with:
- Limit Catch Blocks: Keep the number of catch blocks to a minimum, focusing only on errors needed for that context.
- Descriptive Error Messages: Returned error messages should be clear and descriptive to aid debugging and enhance user experience.
- Consistent Logging: Use a standardized format for error logs, so tracking issues over time becomes more manageable.
Ultimately, employing try-catch constructs effectively can significantly bolster your code's resilience while keeping databases running smoothly.
Common Errors and Exceptions
Understanding common errors and exceptions in PostgreSQL is crucial for anyone involved in database management and programming. Errors can undermine the integrity of data, hinder application functionality, and lead to unwanted application crashes. By recognizing and managing these common issues through proper error handling techniques, developers can not only enhance the robustness of their applications but also maintain data reliability and optimize user experience.
When working with databases, it’s not if an error will occur, but when. Having a solid grasp of common error types allows developers to anticipate issues before they arise. This anticipation prepares them to respond effectively, minimizing downtime and maintaining smooth operational flow.
Identifying Common PostgreSQL Errors
PostgreSQL, while robust, is not immune to errors. Here are several common types of errors one might encounter:
- Syntax Errors: These occur when the SQL statement is not correctly formatted. It’s akin to a typo in a text—an errant letter can change the whole meaning.
- Database Connection Failures: Whether it’s due to configuration issues, network problems, or credentials, failing to connect can halt any data operations.
- Constraint Violations: This happens when data operations violate database constraints—such as unique constraints or foreign key constraints—leading to interference in data integrity.
- Data Type Mismatches: Trying to insert or manipulate data of an incorrect type can throw errors and complicate data handling.
By familiarizing oneself with these errors, developers can create more robust applications. Recognizing patterns in errors can simplify troubleshooting, as one begins to understand the conditions that lead to them.
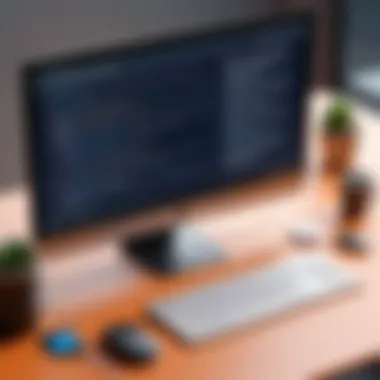
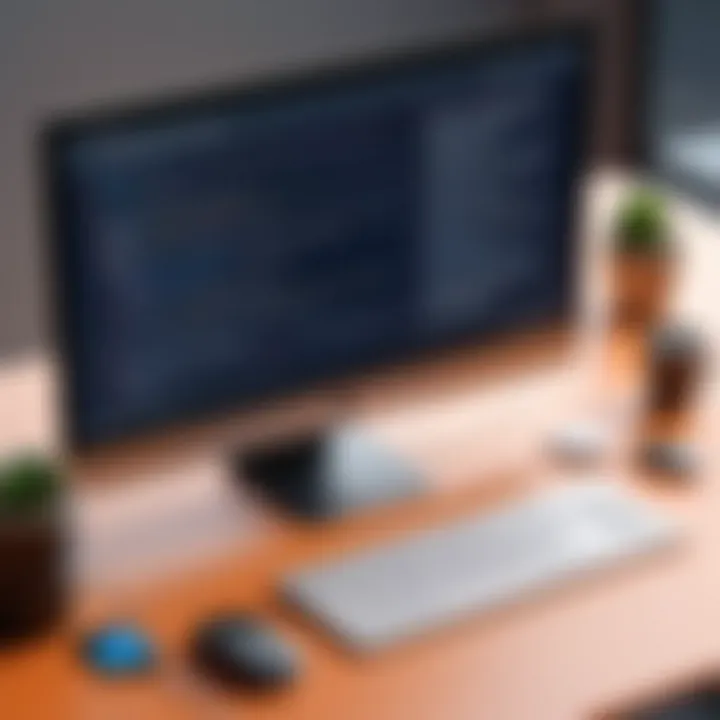
Handling Specific Exception Types
Handling specific exception types effectively is where the try-catch constructs come into play. Here’s how different exceptions can be approached:
- : This is raised when a query references a table that does not exist. A potential solution can be checking table names before running queries, ensuring that schema updates do not lead to missing elements.
- : When attempting to insert a duplicate value violating a unique constraint, capturing this error lets developers provide meaningful feedback to users, perhaps suggesting an alternative input instead.
- : This exception occurs during calculations. Implementing a check before executing such operations can mitigate these occurrences.
By leveraging the try-catch constructs, programmers can implement tailored strategies that not only capture these specific exceptions but also handle them gracefully. Implementing robust error handling minimizes user frustration and contributes to a more pleasant interaction with applications.
"A wise person learns more from the mistakes of others than a fool learns from his own mistakes." - Marcus Tullius Cicero
Debugging with Try-Catch
Debugging in any programming or database environment is a crucial step for ensuring efficiency and reliability. When it comes to PostgreSQL, leveraging Try-Catch blocks for debugging elevates error handling from mere acknowledgment of issues to robust resolution strategies. This section underscores how Try-Catch constructs streamline diagnosing problems within SQL functions and procedures. By wrapping critical code in these blocks, developers can capture exceptions as they occur without crashing the entire application. Thus, they not only provide a safety net but also foster a more graceful degradation of system behavior.
Using Try-Catch for Effective Debugging
Engaging with Try-Catch blocks in PL/pgSQL transforms the way developers approach debugging. Rather than allowing an error to propagate unchecked, these constructs help pinpoint issues while the rest of the function continues to operate. Here’s how:
- Specific Error Handling: By defining specific actions in response to different exceptions, users can tailor their strategies for varying situations, as opposed to a one-size-fits-all approach.
- Maintaining Control: Even when an error occurs, using a Try-Catch allows for conditional responses that keep applications running smoothly.
- Iterative Testing: As developers adjust logic based on errors logged in the catch segment, they can experiment with different corrections or solutions without having to rewrite larger segments of code.
For instance, suppose you have a function that fetches data from a possibly unavailable table. Wrapping this with Try-Catch allows you to not only handle the exception but also log a customized error report for further investigation without halting the whole process.
Logging Errors for Future Reference
An instrumental aspect of using Try-Catch blocks is logging errors. This provides a historical record of issues that can inform future development and troubleshooting. A few considerations include:
- Error Context: Capture valuable context information when logging, such as the date, time, and specific function in which the error occurred, leading to easier identification of recurring issues.
- Easy Retrospective: With a detailed log, developers can easily look back at past errors, understanding not just how often an issue occurred, but also the circumstances surrounding it.
- Postmortem Analysis: Detailed logging facilitates a broader, postmortem analysis of incidents, guiding future error handling strategies and code optimization.
In summary, the ability to log errors within Try-Catch constructs does not just benefit immediate debugging; it also enriches the long-term understanding of system robustness. Developers not only find and fix problems more effectively but they also cultivate a learning environment where mistakes become stepping stones for enhancement. Thus, mastering this facet of PostgreSQL framework allows programmers to systematically refine their applications and handle unexpected scenarios with confidence.
Performance Considerations
Performance considerations play a pivotal role in the realm of error handling, particularly when it comes to employing try-catch constructs in PostgreSQL. Understanding how these constructs can influence the efficiency of your database operations is crucial for both developers and database administrators. When exceptions occur, the impact on performance generally manifests in two primary ways: execution speed and resource allocation.
First off, it’s essential to acknowledge that try-catch blocks, while invaluable for error management, can introduce overhead. Each time a try-catch block is executed, the database needs to track the exception state. This tracking involves maintaining additional context that can lead to a performance penalty. Moreover, frequent errors can cause a bottleneck; if your code is resorting to exception handling as a way to manage flow, you’re likely to experience slower performance over time.
"In error handling, the cost of the exception thrown can often overshadow the cost of the operation itself, leading to deeper considerations in performance evaluation."
Therefore, striking a balance between comprehensive error handling and maintaining optimal performance is paramount. By acknowledging the implications of using try-catch blocks, developers can make more informed decisions in their coding practices. Here we delve into the detailed aspects of performance impacts and ways to mitigate them.
Impact of Try-Catch on Performance
The impact of try-catch on performance can vary greatly based on usage patterns. It's not just about adding these constructs to your code; it’s about how they interact with your overall workflow. When a try block executes successfully, its impact on performance is relatively minimal. Issues arise, however, when an exception triggers a catch block. In these scenarios, significant processing resources can be consumed:
- Error Logging: Detailed error messages and logs can take time to generate and write to your system, adding to the slowdown.
- Recovering State: If your application requires state restoration after an error, this process can become resource-intensive and slow down operations further.
- Repeated Exceptions: A recurring error that prompts the same catch block can create an unnecessary cycle of error handling, further exacerbating performance issues.
Understanding these pain points is essential. Metrics and monitoring tools like pg_stat_statements can help track the performance of SQL statements and identify if exception handling adversely affects your system's speed. Over time, gathered insights can guide revisions to the codebase to optimize performance.
Optimizing Error Handling for Speed
Optimizing error handling mechanisms requires a comprehensive understanding of your application’s flow and potential failure points. The goal is to leverage try-catch blocks without allowing them to hinder speed. Here are several strategies to improve error handling performance:
- Anticipate Exceptions: Design your workflows to account for potential exceptions before they occur. Utilize condition checks and validations to prevent errors from happening in the first place.
- Minimize Exception Handling Scope: Limit the amount of code contained within your try blocks. The longer the execution scope, the more potential for issues arising. Surround only the calls that are most likely to throw exceptions.
- Batch Operations: When dealing with multiple operations, try to consider batch processing. This can reduce the number of transitions between successful execution and catch handling, preserving overhead.
- Error Types: Distinguish between critical and non-critical errors. Handle critical exceptions with appropriate urgency while employing less resource-intensive practices for non-critical cases. For instance, logging a warning instead of an error, when feasible.
These techniques support maintaining or even enhancing the speed of your PostgreSQL operations while ensuring a robust approach to error management. As every database system faces unique challenges, aligning these strategies to your specific needs is vital. By continuously assessing performance metrics in conjunction with robust error handling practices, it’s possible to create a more efficient PostgreSQL environment.
Case Studies
The examination of case studies in the realm of PostgreSQL error handling provides a wealth of practical knowledge that is often absent from theoretical discussions. When developers encounter real-world scenarios, they see the challenges that arise during implementation in a way that abstract concepts simply can’t convey. Through these case studies, we delve into successful implementations of try-catch constructs, highlighting the specific methods and strategies employed by organizations to handle errors efficiently and maintain database integrity.
Moreover, understanding case studies facilitates a broader appreciation for the nuances of error management. It helps identify pitfalls that previous implementations faced, illuminating how such mistakes can be avoided in the future. These real-life examples work as a guiding light, showing that learning from others’ experiences can save time and resources when tackling similar problems.
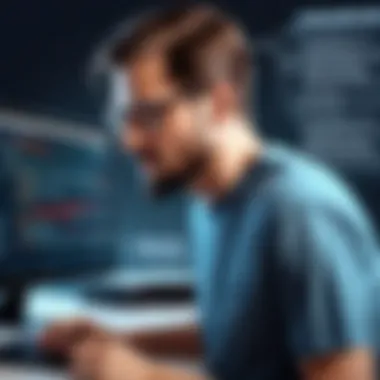
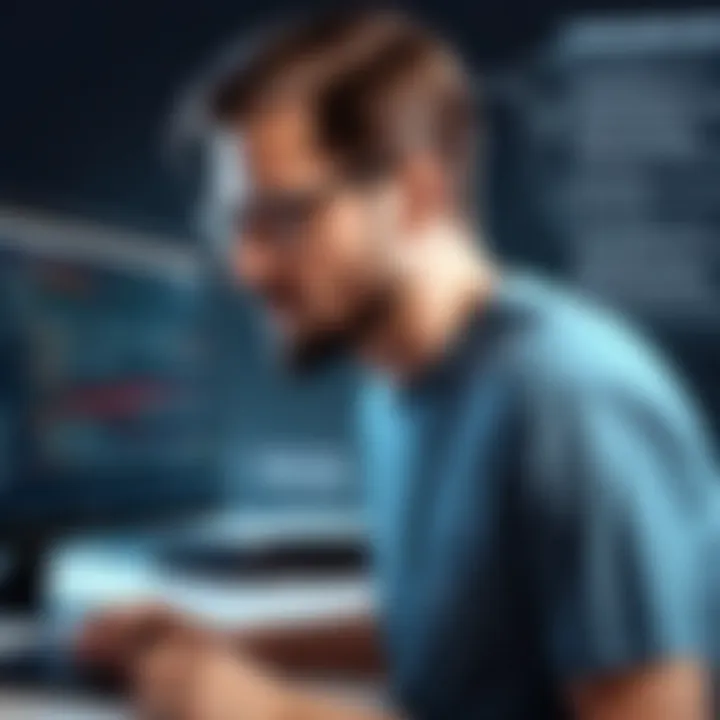
The benefits of studying case studies in error handling include:
- Enhanced Insight: Understanding the intricacies of error management through actual implementations.
- Avoidance of Common Mistakes: Learners can sidestep errors witnessed in past cases.
- Inspiration for Best Practices: The methods that proved effective can be applied to new projects.
- Adaptation of Strategies: Insights gained can be tailored to fit different environments, expanding their applicability.
In a rapidly evolving tech landscape, staying informed about real-user experiences and testing strategies can provide a competitive edge. By looking across diverse use cases, one can pinpoint innovative approaches that help refine error handling dynamics.
Real-World Implementations of Try-Catch
In various organizations, the application of try-catch blocks has demonstrated its effectiveness in mitigating risks associated with database transactions. For instance, a major online retailer employed try-catch in its PostgreSQL database to manage errors during critical sale events. During peak traffic periods, the database can experience unexpected surges in requests, leading to potential failures.
By embracing try-catch, they implemented a fail-safe mechanism. When an error occurred, the system would catch the exception and log detailed information for later troubleshooting, all while ensuring that customer transactions proceeded smoothly. This not only improved user experience but also minimized data loss during periods deemed critical for business operations.
Another notable implementation occurred at a financial services company, which integrated try-catch constructs to uphold compliance standards. Given the stringent regulations in the finance industry, any error could lead to significant complications. By capturing exceptions effectively, the team ensured that all anomalies were logged in a detailed manner, allowing for comprehensive audits which kept the company on the right side of regulatory requirements. This use of try-catch directly contributed to increased operational efficiency and risk management safeguarding.
Lessons Learned from Executing Try-Catch
Reflecting on the execution of try-catch blocks in PostgreSQL has yielded invaluable lessons for developers and database administrators alike. First, constant monitoring is necessary. No system can guarantee the absence of errors, thus, developers learned to accompany the implementation of try-catch with proactive monitoring tools. This enables them to track performance metrics and internally assess the effectiveness of their error handling in real time.
Additionally, the necessity for clear error messaging emerged as a prevalent theme. Users often encountered generic error reports, which failed to provide sufficient context. The organizations that succeeded created informative logs that not only captured error codes but also produced messages rich in detail. This clarity in communication allowed for more streamlined debugging processes.
Moreover, balancing complexity was crucial. A common pitfall was the overcomplication of error handling logic, which led to scenarios where errors could go unnoticed amidst a barrage of code. This taught many that, at times, the simplest solution is the most effective. As a result, frameworks were adjusted to create responsive yet straightforward error handling structures.
Ultimately, the insights drawn from these successful implementations fostered a clearer understanding of how to integrate try-catch in day-to-day development practices. They underscored the importance of continually refining error handling strategies and emphasized an iterative approach towards improvement.
Comparison with Other Database Systems
In the rapidly evolving landscape of database management systems, understanding how different systems handle errors is crucial. This knowledge not only enriches the user's experience but also improves efficiency and robustness of the applications being developed. PostgreSQL, with its prominent capabilities, stands as an exemplary case, particularly when compared to MySQL and SQL Server.
The comparison of error handling practices reveals fundamental differences and advantages that PostgreSQL possesses, showcasing its strengths while also identifying areas where it might lag behind its counterparts. This examination can help database administrators and developers alike make informed decisions on error handling strategies based on specific requirements and use cases.
How PostgreSQL Stands Against MySQL Error Handling
When we delve into the differences between PostgreSQL and MySQL regarding error handling, it becomes clear that both systems take different approaches. In PostgreSQL, the try-catch constructs are prominent, providing developers with a robust mechanism to manage exceptions. MySQL, on the other hand, leans towards a preventive approach with its error-handling features largely reliant on the SQL standards but offering limited flexibility in certain contexts.
For example, while PostgreSQL lets you handle multiple exceptions in a single block, creating a more fluid error management strategy, MySQL tends to segregate errors into a more basic error code structure. Users often find this leads to less comprehensive error-handling solutions. Furthermore, PostgreSQL’s strong type-checking improves the context in which errors are handled, enabling more precise diagnostics. The benefits include:
- More comprehensive exception handling.
- Enhanced flexibility in defining how each exception is managed.
- Detailed logging options that assist in debugging.
This flexibility means developers can implement custom error messages or handle exceptions in a way that's best for their application.
Evaluating PostgreSQL Error Handling in the Context of SQL Server
Shifting the focus from MySQL to SQL Server, we find yet another layer of complexity. SQL Server utilizes its own error handling practices via TRYCATCH blocks similar to PostgreSQL, yet with various differences in implementation and functionality. For instance, SQL Server supports a wide range of error functions that allow developers to access detailed information about each error encountered, such as and . This level of transparency is something PostgreSQL also strives for, but there are instances where SQL Server offers more straightforward access to error data.
Additionally, SQL Server has a more direct way of managing runtime errors, often halting the current batch and rolling back any involved transactions more manageably compared to PostgreSQL. However, PostgreSQL provides greater robustness in its error handling routines through plpgsql features, allowing for a range of actions in reaction to an error.
Both systems have their merits:
- PostgreSQL: More comprehensive handling through custom exception blocks and better performance under certain conditions due to its focus on type consistency.
- SQL Server: Streamlined access to error information, making it easier to report and rectify issues as they arise.
Future of Error Handling in PostgreSQL
As technology evolves, so does the landscape of database management systems, prompting a reevaluation of how we handle errors effectively. The future of error handling in PostgreSQL signifies a pivotal element of database robustness, directly impacting both user experience and system performance. This aspect becomes critical in the face of increasing data volumes and complexity in applications. Understanding what lies ahead is not merely an optional skill for database administrators but a necessity for anyone involved in maintaining a PostgreSQL environment.
The significance of this topic lies in the collaborative growth of error management techniques and PostgreSQL itself. As new paradigms and methodologies emerge, there is potential for improved efficiency in troubleshooting and error resolution. Innovations are on the horizon, including the integration of artificial intelligence and machine learning algorithms designed to anticipate errors before they manifest into significant issues. This construct can radically minimize service downtime and enhance program stability.
Emerging Trends in Database Error Management
The realm of database error management is witnessing some compelling developments that could reshape how we think about and address errors in PostgreSQL:
- AI-Driven Monitoring: The rise of artificial intelligence tools is setting a standard for proactive error detection. These tools analyze patterns, predict failures, and notify developers about potential issues, allowing for timely intervention. By utilizing machine learning algorithms, systems can learn from historical data to identify deviations and flag unusual behavior.
- Self-Healing Systems: Imagine a world where errors correct themselves without human intervention. Systems are being developed that can automatically apply fixes based on predefined rules, drastically reducing the manual load on IT teams. This trend could lead to a paradigm shift in how database systems maintain performance and availability.
- Dynamic Error Reporting: Traditional error reporting often entails manual checks and lengthy processes. New techniques will allow for real-time reporting and dashboard views, providing instant insights into errors as they happen. This goes beyond simple notifications to providing actionable insights that developers can use immediately.
Staying on top of these trends not only arms developers with critical tools but also enhances their ability to create a more reliable PostgreSQL environment.
Potential Enhancements in PostgreSQL Error Handling Mechanisms
The engine of PostgreSQL is continually fine-tuned, and several promising enhancements could redefine how error handling is approached:
- Granular Exception Handling: The future may offer more sophisticated ways to handle exceptions that enable categorization based on severity and context. Enhanced granularity would allow developers to create specialized responses based on the error type, improving overall handling strategies.
- Custom Error Codes: Standardizing a more uniform method of coding errors will facilitate better communication and resolution across various applications. By providing developers with a set of customizable error codes, they will be able to quickly pinpoint issues and their origins.
- Integration with Development Tools: As PostgreSQL integrates with more advanced development environments, the synergy between error handling functionalities and development tools can provide developers with a holistic view of any issue. A seamless connection between coding, debugging, and the database will ensure high levels of efficiency and effectiveness in troubleshooting.
The future of error handling in PostgreSQL is ripe with potential. Embracing these emerging trends and enhancements is not just about keeping pace with the latest in technology; it’s about setting the stage for a more efficient and resilient database environment.