Mastering Single Quote Escaping in PostgreSQL Queries
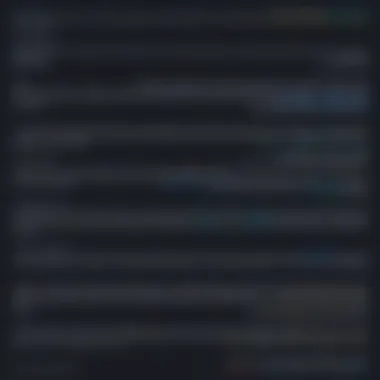
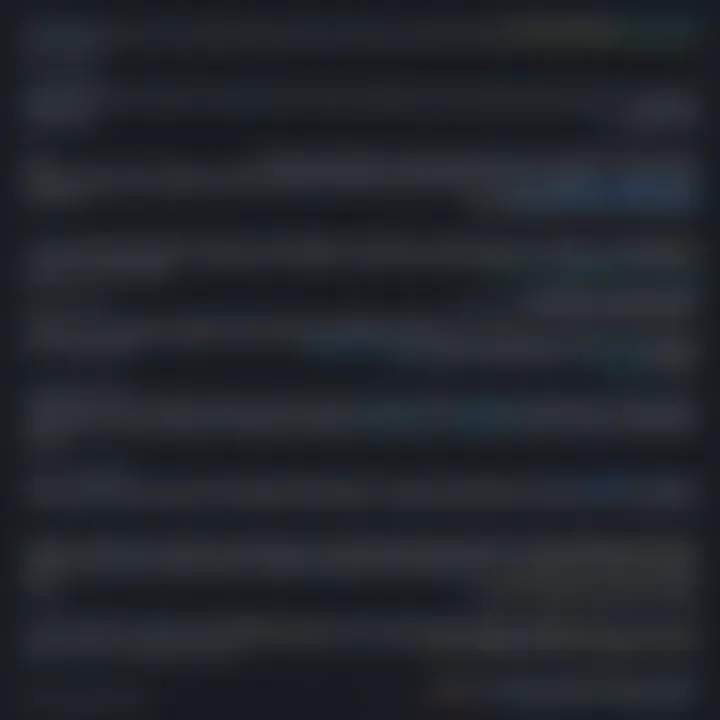
Intro
Dealing with single quotes in PostgreSQL can often be a source of confusion for developers and database administrators. Single quotes are essential for defining string literals but can also lead to complications like syntax errors and injection vulnerabilities if not handled correctly. This section aims to lay the foundation for understanding how to escape single quotes and offers an insight into why it is crucial for writing secure SQL queries.
When constructing SQL queries, special characters like single quotes can interfere with the database's ability to parse commands properly. An unescaped quote can lead to incomplete statements, causing queries to fail. Moreover, if user input is not sanitized correctly, an attacker can leverage this punctuation to inject malicious SQL code. This underscores the need for careful consideration of how single quotes are treated in your SQL statements.
In the following sections, we will explore various techniques available in PostgreSQL for escaping single quotes. Programers will find both native functions and best practices to efficiently handle these characters, while database administrators can sharpen their security protocols. Along the way, we will review common examples and pitfalls, ensuring that readers come away with a comprehensive understanding.
Coding Challenges
Single quotes present distinct challenges during Query Construction. Understanding these hurdles can enhance code quality and system reliability.
- Weekly Coding Challenges: Practicing SQL formats regularly can streamline handling unexpected input in production code.
- Problem Solutions and Explanations: A typical problem with single quotes arises from concatenating user input into queries. Here’s a simple solution: escape single quote with another single quote. You will turn a ' into a two-times \'.
- Tips and Strategies for Coding Challenges: Adopt consistent methods for escaping single quotes as well as minimize reliance on dynamic SQL.
A good practice is to rely on parameterized queries instead of constructing SQL commands from strings.
- Community Participation Highlights: Engaging in forums like Reddit can provide real-world knowledge to improve coding practices.
The discourse surrounding the most effective ways to escape single quotes in PostgreSQL reveals immense depth and complexity but opens the door for engaging discussions on secure database management.
To build knowledge, let's seek further into technology trends alongside best practices in code construction.
Prelude to PostgreSQL and Single Quotes
Overview of PostgreSQL
PostgreSQL is a powerful, open-source object-relational database system featuring a robust set of advanced database functionalities. It supports a variety of programming languages and allows for the complex handling of transactions and large amounts of data. One of PostgreSQL’s key strengths lies in its standards compliance. It effectively implements SQL standards alongside unique features that benefit application developers and database administrators. Understanding PostgreSQL is therefore essential for anyone working with data and ensuring that queries function properly.
In essence, PostgreSQL provides flexibility and reliability necessary for developing modern applications. Its architecture supports both simple electronic web applications and massive data warehouses, establishing a solid groundwork for a diverse range of use cases. Nevertheless, using PostgreSQL often involves difficulties, such as dealing with special characters. This includes single quotes. That brings us to the core of our topic.
Importance of Single Quotes in SQL
Single quotes play a significant role in SQL, particularly in PostgreSQL, as they denote string literals. Every time a programmer creates or executes a SQL statement, the presence of data structures in that context, influenced by single quotes, comes into play. Recognizing this significance is crucial because incorrect handling of single quotes can lead to syntax errors and, potentially, security vulnerabilities.
Improperly managed single quotes often disrupt queries and can expose a system to SQL injection attacks, impersonating grave dangers, especially in web environments. Therefore, it is paramount for developers to learn methods of escaping single quotes where necessary, to maintain integrity in their queries.
“Single quotes require particular attention when programming in SQL, as their incorrect usage can wreak havoc on data integrity and security.”
The need for proficiency in escaping single quotes leads to better practices in craftsmanship of SQL statements, which in turn enhances application stability.
The Mechanics of String Literal Handling
Understanding string literals is fundamental for constructing accurate queries in PostgreSQL. This section discusses the nuances of string handling, including its significance, advantages, and intricate considerations that programmers and database administrators should be aware of. Improper handling of strings often leads to errors and potential vulnerabilities in SQL statements, leading to security risks. This overview serves as a segue into exploring how single quotes are essential constituents of string literals, calling attention to common pitfalls and effective handling strategies.
Understanding String Literals
String literals are sequences of characters enclosed in single quotes, forming the backbone for text representations in SQL queries. When integrated into a SELECT statement or a WHERE clause, these literals define constant values. Recognizing their structure is critical because:
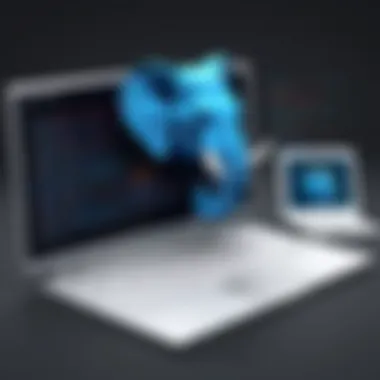
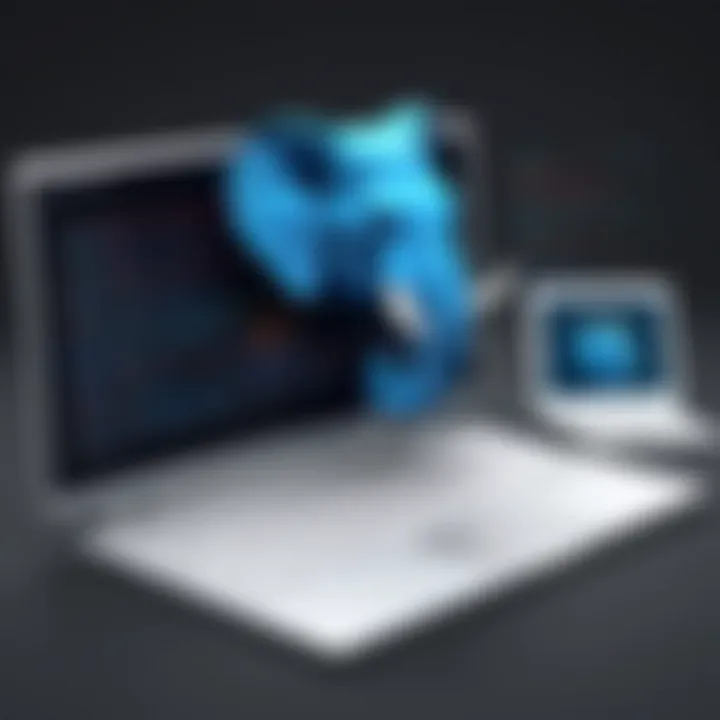
- They pertain to data types like varchar, char, and text.
- Correct syntax prevents runtime errors.
- They shape the expression of textual data in queries.
To declare string literals correctly, one must be mindful of the placement and encapsulation of single quotes. Inserting or avoiding extra quotes has severe implications, leading potentially to syntax errors or misleading data retrieval.
Role of Single Quotes in Queries
Single quotes serve a paramount role in encapsulating string literals. Here are important aspects worth noting:
- Delimitation: They mark the beginning and end of a string, defining the scope of data being referenced. For example, in the query , ‘Alice’ is clearly distinguished as a string.
- Security Considerations: Improper handling of single quotes can open doors for SQL injection attacks. Security revolved around escaping them, as they dictate the flow of query interpretation.
Additionally, violating string syntax due to incorrect usage can render an entire SQL command invalid, halting operations in production environments. Awareness of these roles enhances the ability to write reliable queries, safeguarding not just the developer's functionality but also the integrity of the database.
Avoiding pitfalls in string literal handling is critical for secure and effective database interactions.
Escaping Single Quotes in PostgreSQL
Escaping single quotes in PostgreSQL is a critical skill for anyone working with SQL queries. An understanding of how to properly manage these characters ensures that queries function as intended, without interruptions or errors. This section articulates various methods used for escaping single quotes while considering security, syntax correctness, and broader implications for database management. This knowledge is valuable for developing robust applications and avoiding common errors associated with improper quote handling.
Using Backslashes for Escaping
One method of escaping single quotes in PostgreSQL is by using backslashes. When a developer needs to include a single quote within a string literal, placing a backslash immediately before the quote will effectively escape it. For instance, the practical implementation of this can be seen in the following example:
This query results in the output: It’s a sunny day. It is direct and easy to remember. However, it's important to note that some database configurations may not allow backslash escaping by default, potentially leading to confusion. Always confirm how your database handles escape sequences before relying heavily on this method.
Doubling Single Quotes
Another common technique involves doubling single quotes within a text string. This approach is widespread and favored for its clarity and simplicity. To represent a single quote in a string, simply type two single quotes in succession. For example:
Again, the result will be suitably processed as It’s a sunny day. Most SQL programmers utilize this method, especially since it aligns with standard SQL syntax.*
This method works uniformly across PostgreSQL approaches, eliminating the ambiguity around escape preferences, creating considerable advantage in producing more reliable code.
The E'' Syntax
The third technique to escape single quotes employs the E'' syntax, which stands for
Practical Examples of Escaping Single Quotes
Understanding how to escape single quotes effectively is paramount for anyone working with PostgreSQL. The way strings are handled can determine if a query succeeds or fails. Properly managing single quotes also eliminates risks associated with SQL injection. This section provides practical examples that illustrate escape techniques. They aim to help programmers overcome potential pitfalls in query construction.
Basic Queries Demonstrating Escape Techniques
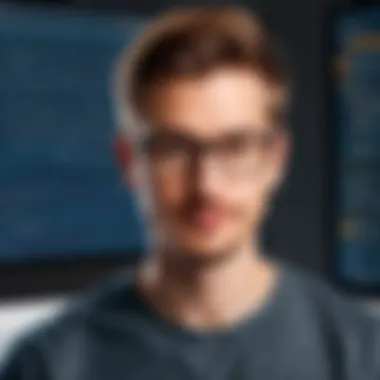
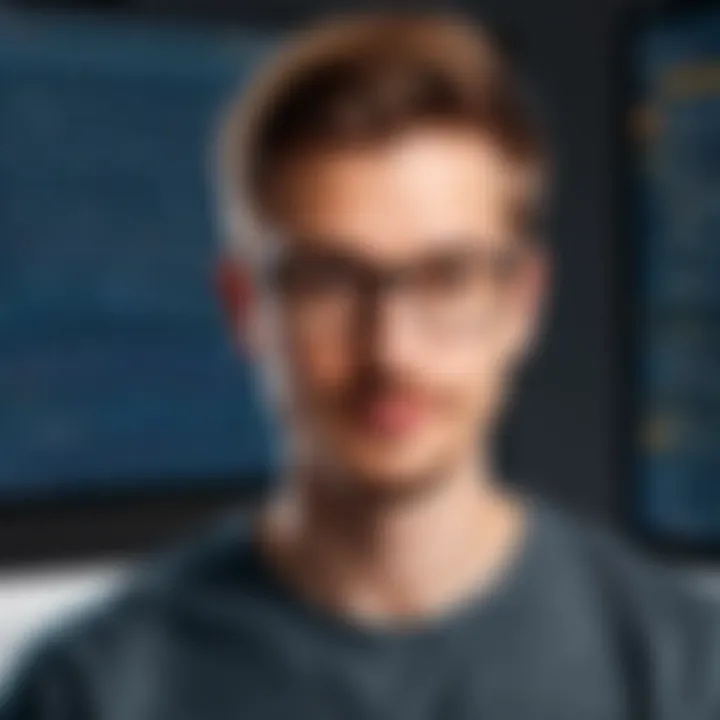
In this subsection, we step through some essential queries aimed at escaping single quotes. For instance, the most common technique is doubling single quotes. This method is particularly straightforward and widely accepted. Let's look at a simple example where we want to include a single quote in a string.
In this query, we insert the phrase It's a nice day. by doubling the single quote. Such syntax ensures PostgreSQL understands we are referring to an apostrophe instead of the closing quote. The output will return: It's a nice day.
Another technique employs the backslash to escape single quotes. PostgreSQL primarily recognizes backslash escaping within the context of certain configurations. The statement below demonstrates it:
Using the backslash to escape the single quote effectively signals to PostgreSQL that the primary intention is to insert the text string, not terminate it. Understanding queries such as these is crucial for aspiring and seasoned programmers. Solid command over these techniques translates directly into more reliable applications.
Advanced Use Cases in More Complex Queries
As codes grow more intricate, using escape methods similarly demands a deeper understanding. Queries that rely on user inputs often lead to needing resourceful escape techniques.
This example illustrates a more complex scenario involving a table called comments where users might leave a response that includes single quotes:
In this instance, we again double the single quotes within the comment text to safely insert it into the database. Ignoring this method in a more intricate operation can derail data integrity, increasing the threat of SQL injection.
Another noteworthy scenario is using the E'' syntax. This allows you to take advantage of special escape characters, like in the following example:
The use of signals to PostgreSQL that the text contains escape sequences. Learning to use escape methods, especially in complex scenarios, encourages better safety and functionality in database applications. Considering common tasks as outlined can refine one's SQL agility.
Remember: Always test your queries before deploying them in a production environment. Proper knowledge of escaping techniques along with practical application can significantly mitigate risks.
Best Practices for Query Construction
When dealing with PostgreSQL, the construction of secure and efficient queries is paramount. Best Practices for Query Construction encompass strategies that not only maintain the integrity of your SQL commands but also shield them from attacks and bugs. This section aims to outline these essential techniques with a focus on avoiding vulnerabilities and enhancing reliability in the handling of single quotes.
Avoiding SQL Injection Risks
SQL injection is one of the most common threats faced by database applications. It occurs when an attacker manipulates SQL statements via insecure input feeds, potentially accessing or corrupting data. To prevent such risks while handling single quotes, it is essential to consider various approaches.
- Input Validation: Before executing any SQL commands, particularly those containing user inputs, it's needed to validate inputs for accepted formats.
- Escaping Inputs: Ensure proper escaping of single quotes to prevent direct insertion into SQL commands. This can be achieved using the doubling method or using the E'' prefix for strings.
- Implement Proper Access Controls: Users should be granted the least privilege necessary for database interactions. This limits exposure to SQL injection vulnerabilities.
By implementing these measures, database professionals can significantly mitigate the risks presented by SQL attacks, ensuring a more secure application.
The cost of inadequate security is far greater than investing time into proper safety measures and best practices.
Utilizing Parameterized Queries
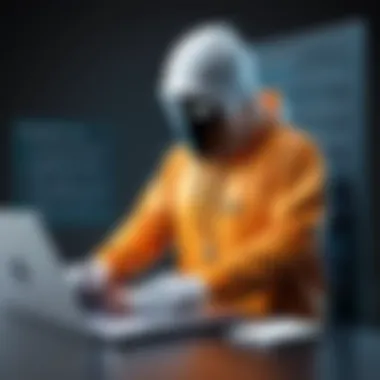
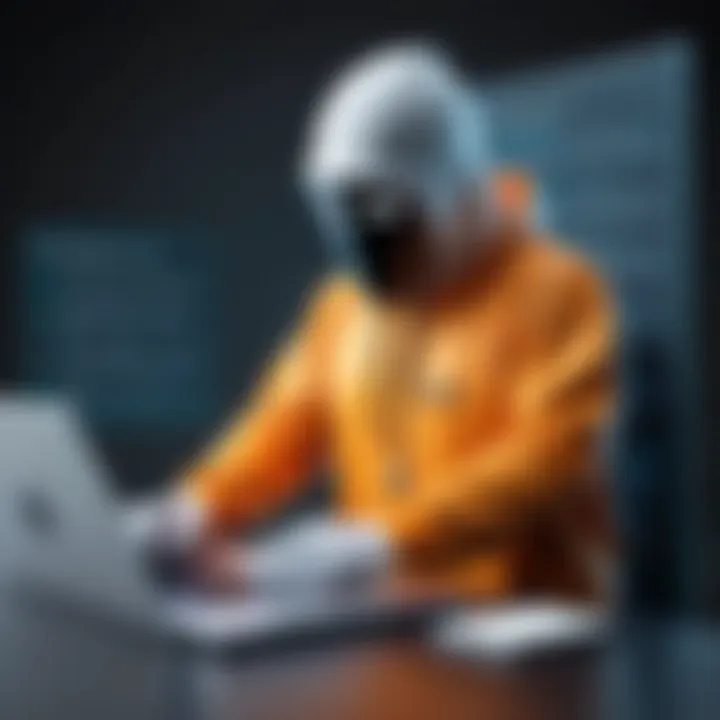
One of the most effective methods for construction secure SQL commands is by making use of parameterized queries. These statements allow the separation of SQL code from data inputs, meaning user inputs never directly interact with SQL commands. Below are reasons why this approach is beneficial:
- Security Enhancement: Accessing the database through parameterized queries effectively prevents injection attacks by treating input as data rather than executable code.
- Performance Improvements: PostgreSQL can cache the execution plan for parameterized queries, which can enhance performance by reducing planning time on subsequent executions.
- Code Clarity: Using parameterized queries tends to produce cleaner code. The intention of the query is clearer, which can improve maintainability.
Sample syntax of parameterized queries in PostgreSQL:
Using these methods of query construction, programmers and database administrators can safeguard their applications from typical pitfalls and improve overall database performance.
Common Errors and Troubleshooting
Understanding common errors and effective troubleshooting techniques related to single quotes in PostgreSQL is crucial for maintaining reliable database interactions. Errors often arise when single quotes are not properly escaped, leading to unintended syntax errors or, even worse, SQL injection vulnerabilities. This section aims to provide strategies to identify these issues and suggest appropriate resolutions.
Identifying Syntax Errors Related to Single Quotes
Syntax errors primarily happen during SQL execution when single quotes are not used correctly. A runaway single quote in your query may result in various error messages, which indicate the syntax is malformed. Below are some key points to consider:
- Error Messages: PostgreSQL provides specific error messages. For example, it may give a warning like which indicates that a single quote may not be closed properly.
- Common Patterns: When strings that include single quotes are not escaped correctly, errors become evident. For instance:This statement results in a syntax error due to the unescaped single quote after “O.”
- Tools: Use SQL tools and environments such as PgAdmin to identify and debug such issues more efficiently.
By recognizing these patterns and utilizing PostgreSQL’s built-in error messages, you can quickly pinpoint where escape characters need to be applied.
Resolving Data Entry Issues
Data entry issues can also stem from non-escaped single quotes. Such issues often arise during the collection of user input. Here are several considerations:
- User Input: When collecting data that may contain single quotes from forms or APIs, proper validation is crucial. If relevant characters are not handled properly before reaching the SQL layer, escaping becomes necessary.
- Escape Functions: Programmatically, utilize functions like or employ libraries that automatically handle escaping string literals. For instance:
- Consistent Protocols: Establish procedures for incoming data processing. All entries in parameters should first sanitize to remove or escape problematic characters before they interact directly with the database.
Overall, handling escaping effectively requires awareness and action at various stages of query processing. By methodically implementing validation and escaping protocols, you can significantly reduce data entry errors related to single quotes. Maintaining these best practices in your development cycle enhances the robustness and security of your PostgreSQL interactions.
Culmination and Key Takeaways
Summary of Escaping Methods
In summary, there are key methods to escape single quotes in PostgreSQL:
- Using Backslashes: Applying a backslash before a single quote allows the database to recognize it as part of the string, not as a terminator.
- Doubling Single Quotes: By writing a pair of single quotes, PostgreSQL interprets it correctly, allowing insertion of single quotes within string literals.
- The E'' Syntax: This syntax is especially useful for inserting special characters, and enables string literals to be defined explicitly.
These methods not only serve to write effective queries but also to foster better programming practices among developers and database administrators.
The Future of SQL Handling in PostgreSQL
The evolving landscape of data management in PostgreSQL necessitates a clear understanding of how characters, such as single quotes, are handled within the queries. Ongoing development in PostgreSQL emphasizes improving the syntax and providing better tools for querying. The focus will likely blend usability with performance, driving innovations around handling various data inputs.
It is reasonable to expect PostgreSQL will continue enhancing its mitigating strategies against common vulnerabilities associated with string handling. This may manifest through improved documentation, built-in safety mechanisms, and possibly further standardization of guidelines for escaping characters.
Properly managing string literals now may save programmers from painstaking errors and security issues in the future.