Key Java Coding Interview Questions to Master
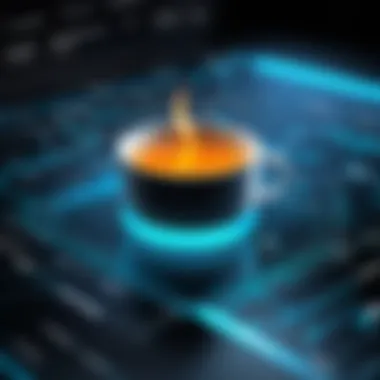
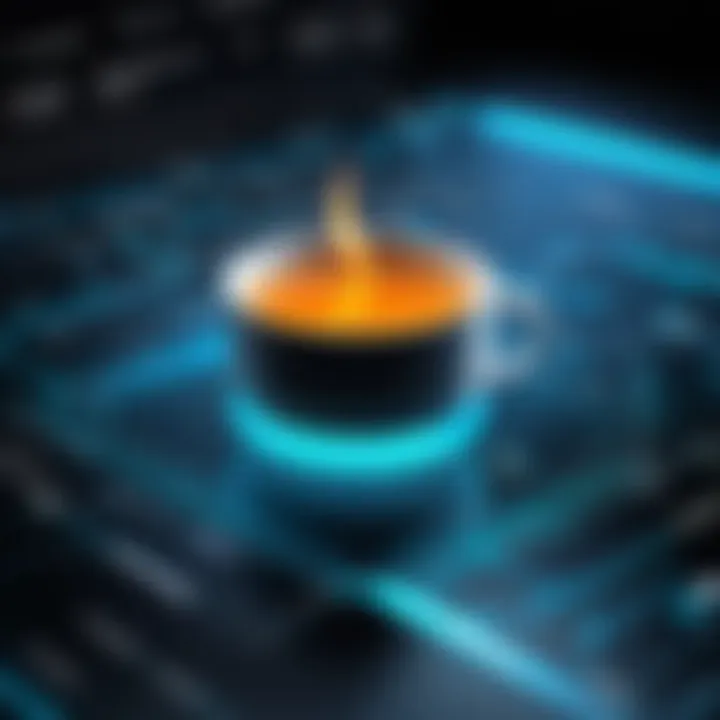
Intro
As you prepare for your next interview, it’s not just about knowing your way around Java syntax; it’s about understanding the why and how behind what you’re doing. The ability to solve problems effectively and articulate your thought process clearly can make or break your chances. Hence, we’ll walk through topics ranging from basic coding challenges to complex data structures, equipping you with insights and strategies that extend beyond rote memorization.
Let’s dive into the first section where we’ll tackle coding challenges. Prepare yourself for a blend of practical scenarios and expert tips designed to sharpen your skills as you gear up for that all-important interview.
Intro to Java Coding Interviews
In today’s competitive job market, Java skills are highly sought after. This section serves as a launchpad into the vital topic of Java coding interviews. It's not just about knowing how to code; it's about understanding why coding interviews are structured the way they are. Knowing the nuances can make a world of difference for candidates, whether they are just embarking on their careers or pivoting into new roles.
Understanding the Importance of Java in Interviews
Java remains one of the most widely-used programming languages in the industry. Its broad applicability ranges from web applications to complex enterprise systems, ensuring Java's place as a staple in many tech stacks.
- There are several reasons why Java knowledge is crucial in interviews:*
- Job Market Demand: Companies often lean toward Java for building scalable, high-performance applications. As such, Java developers are continuously in demand.
- Foundational Language: Many computer science curricula emphasize Java, positioning it as a key learning experience for students. Knowledge of Java signifies a competent grasp of programming basics and principles.
- Interview Standardization: Java is commonly used in coding challenges during interviews. Familiarity with its syntax and intricacies can ease the coding process during assessments.
Moreover, mastering Java enhances a candidate's problem-solving skills and logical thinking abilities.
By grasping Java's fundamental concepts and its applications, candidates can better prepare themselves for rigorous interview scenarios. More importantly, a solid foundation allows them to tackle complex problems with confidence.
An Overview of Common Interview Formats
The interview process for tech positions can vary widely, but several common formats persist across the board. Understanding what to expect can provide candidates with a substantial advantage. Here’s a look at some prevalent interview formats within Java coding interviews:
- Technical Interviews: These often focus on coding challenges and algorithm-related questions. Candidates are expected to demonstrate proficiency in Java while solving real-time problems.
- Behavioral Interviews: Companies seek to gauge a candidate's soft skills and cultural fit. Questions often revolve around past experiences and how candidates handle specific situations.
- Whiteboard Coding Sessions: Candidates may be asked to code by hand on a whiteboard. This format tests not only coding skills but also communication and thought processes.
- Pair Programming: In this scenario, candidates collaborate with an interviewer. This emphasizes teamwork and the ability to articulate thoughts clearly.
"Preparation is key. Knowing which interview format will be used can help tailor your practice accordingly."
By familiarizing themselves with these different formats, candidates can develop targeted strategies to showcase their skills effectively. Emphasis on clarity and logic during coding challenges in interviews can often be as crucial as the solution itself. Adjusting to such formats allows candidates to navigate the interview landscape with a greater sense of assurance.
Core Java Concepts
Core Java Concepts are the backbone of any programming interview focused on Java. Understanding these concepts is not only crucial for acing an interview but also fundamental for everyday work as a developer. They encompass the basic building blocks of the language, which can significantly influence how a programmer approaches problems. Mastery of these concepts will stand you in good stead, making it easier to navigate complex scenarios and engage with more advanced topics later on.
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a paradigm that uses "objects" to model real-world phenomena. In Java, which is inherently object-oriented, this approach brings several benefits. Here’s a closer look at key elements:
- Encapsulation: This principle restricts direct access to some of an object’s components and can prevent the unintended interference and misuse of methods and data. It promotes modularity and maintains a clear separation between an object’s interface and its implementation.
- Inheritance: By allowing a class to inherit methods and fields from another class, Java enables code reusability. This can simplify code management and extend the functionality of existing classes without altering their structure.
- Polymorphism: It allows methods to do different things based on the object it is acting upon. In practice, you can write a single piece of code that works on different types of objects.
- Abstraction: Abstraction helps simplify complex systems by breaking them into manageable pieces. It helps in hiding the implementation details and showing only the essential features of an object to the user.
Mastering these concepts can be a game changer when tackling coding interview questions, especially those that assess your analytical skills and your ability to apply OOP principles effectively.
Key Java Data Types and Variables
In Java, understanding data types and variables is essential because they dictate how data is stored, manipulated, and interacted with. Here are some points to consider:
- Primitive Data Types: Java has eight primitive data types: byte, short, int, long, float, double, char, and boolean. Knowing when to use each type will optimize memory usage and performance.
- Reference Data Types: Unlike primitives, reference data types refer to objects in memory. When handling complex structures like arrays and classes, understanding how reference types work is crucial.
- Variables: In Java, variables are containers for storing data values. Using proper naming conventions and declaring variables with appropriate types is important for readability and debugging.
To illustrate:
In summary, a solid grasp of Core Java Concepts—including OOP principles and data types—establishes a programmer’s foundation for growth. These are the lenses through which technical interviews often evaluate candidates, so take them seriously and prepare accordingly.
Common Coding Challenges
Common coding challenges serve as a cornerstone in the world of Java interviews. They test not only a candidate's proficiency in the language but also their problem-solving and critical-thinking abilities. Employers often present these challenges to gauge how a programmer approaches problems, not just the final solution. These challenges can illuminate a candidate's thought process, creativity, and technical skills. Hence, preparing for them is crucial for anyone eyeing a successful Java programming career.
When faced with coding challenges, candidates encounter various elements that can influence their performance:
- Real-Time Problem Solving: Coding challenges typically reflect scenarios you might face in real-world applications. This relevance makes the challenges not only practical but vital in evaluating how candidates would handle actual coding tasks.
- Understanding Complexity: Challenges often measure both time and space complexity, prompting candidates to consider efficient algorithms.
- Edge Cases: Candidates must also think about handling unexpected inputs or unusual cases, showcasing their completeness in coding logic.
The following sections will explore specific common coding challenges encountered in Java interviews, providing illustrative examples along with insights into their underlying principles.
Reversing a String
Reversing a string is a straightforward yet effective exercise that can test familiarity with Java's string manipulation features. At its essence, reversing a string involves taking the characters of the string and presenting them in the opposite order. This challenge can be approached in various ways:
- Using a Loop: One classic method involves iterating through the string backwards using a loop and building a new string.
- Using Built-in Methods: Java provides a simple solution through its class, which has a built-in method to reverse strings.
Example Code:
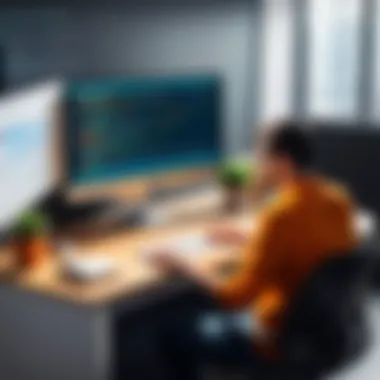
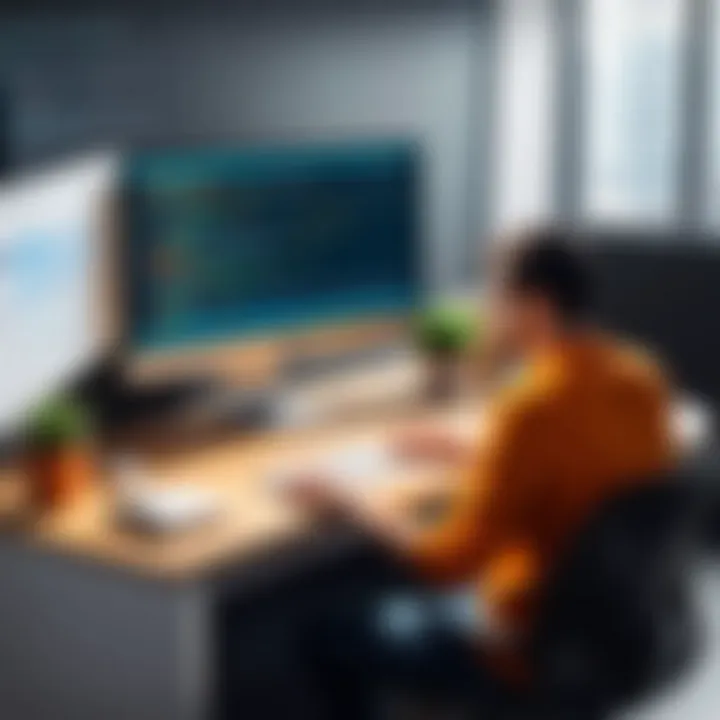
This approach keeps things tidy and leverages Java's standard library effectively.
Finding the Factorial of a Number
Calculating the factorial of a number is another popular problem that showcases an understanding of recursion versus iterative techniques. The factorial of a number , denoted as , represents the product of all positive integers up to . This is often a first step into understanding recursion, as the solution can be elegantly expressed with recursive calls:
- Recursive Approach: Factorial can be defined recursively: . This captures the essence of breaking down the problem into smaller, manageable sub-problems.
- Iterative Approach: Alternatively, a simple loop can be used to achieve the same result without the overhead of recursive calls.
Example Code:
Both methods have their merits, depending on the context in which they're applied.
Checking for Palindromes
The palindrome check is another engaging challenge that tests familiarity with string properties and conditions. A palindrome is a string that reads the same forwards and backwards, such as "radar" or "level". Recognizing whether a string is a palindrome requires a keen eye for detail.
- Direct Comparison: One can compare the characters from the beginning and end of the string moving towards the center.
- Using String Functions: Java's string manipulation functions can simplify the task considerably.
Example Code:
This problem not only requires logical reasoning but also attention to symmetry in characters, cementing valuable skills for a programmer.
By mastering these common coding challenges, candidates not only prepare for interviews but also sharpen their overall coding skills.
Utilizing Collections Framework
The Collections Framework in Java is a vital component for any programmer looking to handle groups of objects effectively. As you prepare for coding interviews, understanding how to utilize this framework can set you apart. The Collections Framework provides various data structures like lists, sets, and maps, which are essential for storing and manipulating data efficiently. In interviews, candidates are often tasked with problems that require a good grasp of these collections, making it imperative to be proficient in this area.
Working with Lists and Sets
Lists and sets serve different purposes in programming, and knowing when to use each is key to writing optimal code.
Lists are ordered collections that allow duplicates. They are useful when the sequence of elements matters, such as when you want to maintain the order of items. Commonly used implementations include ArrayList and LinkedList. The choice between these two often hinges on the specific use-case in mind:
- ArrayList: Great for fast random access and iteration.
- LinkedList: More efficient for frequent insertions and deletions.
On the other hand, sets are collections that inherently do not allow duplicates, making them effective for filtering out repeated entries. The HashSet and TreeSet are popular implementations:
- HashSet: Offers average-time complexity of O(1) for basic operations like add, remove, and contains.
- TreeSet: Maintains a sorted order, which is useful when you need to traverse elements in a specific sequence, though with slower performance at O(log n).
Understanding the differences, and the scenarios to utilize each, will show your depth of knowledge in interviews. A candidate might be asked how to store unique product IDs or maintain an ordered list of student names, giving an opportunity to discuss both data structures.
Understanding Maps and their Applications
Maps are perhaps one of the most powerful components of the Collections Framework, allowing for the association of keys to values. This structure is best exemplified through the HashMap and TreeMap.
- HashMap: This is widely used for storing key-value pairs where quick access is essential. Its average time complexity for get and put operations is O(1). It works based on a hashing algorithm, but doesn't maintain any order.
- TreeMap: Unlike HashMap, TreeMap maintains a natural ordering of keys, which can be beneficial when sorting is required. However, it trades off speed for this order, with average time complexity of O(log n).
Maps can solve various coding problems, such as counting the occurrences of words in a sentence or tracking the frequency of items in a dataset. This understanding can demonstrate problem-solving insights during interviews as interviewers often seek candidates who can think critically about their choices in data handling.
"Having a solid grasp of the Collections Framework not only enhances your coding skills but also prepares you for tackling common interview questions efficiently."
In summary, familiarity with lists, sets, and maps within the Collections Framework is invaluable for Java programmers. In a world where efficiency matters, being able to choose the right collection can drastically improve the performance of your applications and your chances of succeeding in coding interviews.
Algorithm Analysis
When preparing for Java coding interviews, algorithm analysis is a cornerstone that cannot be overlooked. This area not only helps in gauging the efficiency of your code but also in understanding how well it performs under different conditions. More than just a topic, algorithm analysis lays the groundwork for problem-solving by offering insights into how to optimize solutions. Understanding algorithmic principles gives candidates a sharper edge, making them more attractive to employers.
The significance of algorithm analysis goes beyond mere aesthetics; it's about understanding complexity—how algorithms behave as input size scales. In interviews, being able to articulate the efficiency of your approach can set you apart from the crowd. As a programmer or computer science student, familiarizing yourself with concerns related to efficiency and complexity is pivotal not just for interviews but for real-world application as well.
Time Complexity and Space Complexity
Time complexity and space complexity are two sides of the same coin, providing a comprehensive picture of an algorithm’s performance. Time complexity measures how the run time increases as the size of the input increases. It is essential for evaluating whether your solution can handle large datasets efficiently. You might often hear programmers refer to this in terms of Big O notation, which simplifies this analysis into categories like O(1) for constant time, O(n) for linear time, and O(n^2) for quadratic time.
On the other hand, space complexity evaluates how much memory an algorithm uses in relation to the input size. It’s crucial to account for both types of complexity, as an algorithm with a lower time complexity may utilize significantly more memory, which can lead to operational inefficiencies.
For instance, consider a search operation. If you use an array to search for an element, the time complexity can be O(n). However, if you implement a more sophisticated data structure such as a hash set, you could potentially lower it to O(1) while being mindful of how the space changes with the number of elements stored.
Key Points:
- Time complexity measures the run-time of an algorithm as input size grows.
- Space complexity helps assess how much memory an algorithm uses in relation to the size of the input.
Big O Notation: A Closer Look
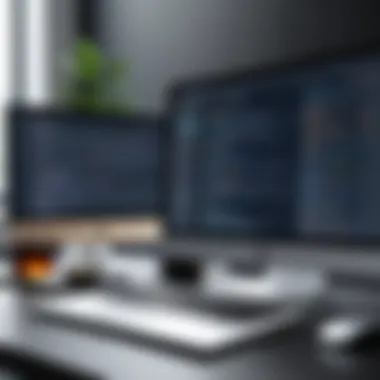
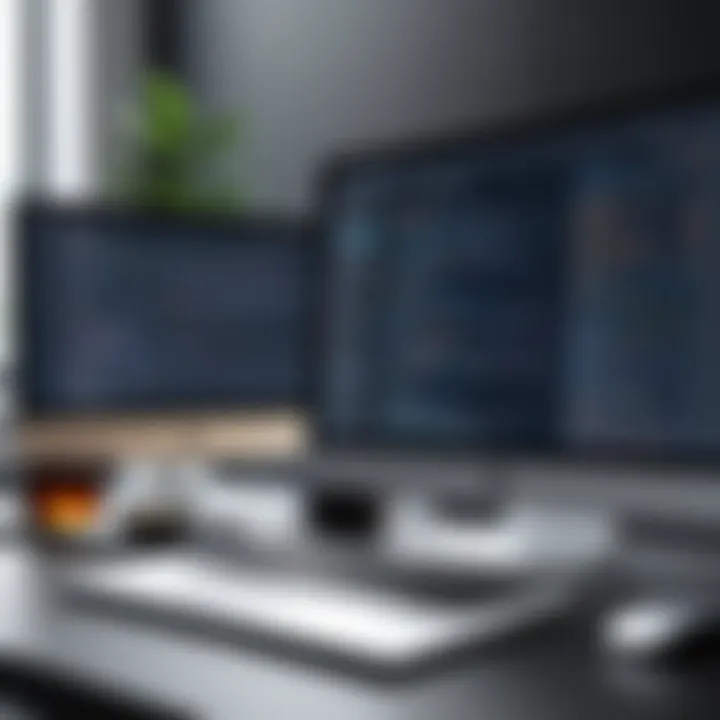
Big O notation is not just a fancy term; it’s a systematic way to describe the upper limit of an algorithm’s running time. This notation helps in expressing the worst-case scenario, providing a reliable way for programmers to estimate performance. It can be a bit esoteric for newcomers, but once grasped, it becomes a powerful tool in the coder's kit.
There are a few common classes of complexity in Big O notation:
- O(1): Constant time - the execution time does not change with the input size.
- O(log n): Logarithmic time - increases slowly as the input size grows; typical with search algorithms.
- O(n): Linear time - performance grows linearly with the input size; common in simple loops.
- O(n log n): Log-linear time - often found in efficient sorting algorithms like mergesort and heapsort.
- O(n^2): Quadratic time - seen in less efficient algorithms like bubble sort where nested iterations occur.
By understanding Big O notation, you can communicate the resource efficiency of your algorithms clearly.
"Algorithm analysis isn't about academic exercises but practical insights that build robust applications."
Mastering the principles of time complexity and space complexity, along with Big O notation, not only prepares you for interviews but equips you with evaluation strategies crucial for building more efficient software solutions.
Multi-threading in Java
In the contemporary landscape of software development, multi-threading emerges as a pivotal topic. It allows programs to operate efficiently by concurrently executing multiple threads. Understanding multi-threading is essential for developers, particularly those entrenched in Java, as it directly affects application performance and responsiveness. In many ways, it mirrors real-life scenarios — much like how a restaurant splits tasks between waiters and cooks, multi-threading divides work within applications to boost productivity.
Understanding Threads and Runnable Interface
A thread can be thought of as a lightweight process. Each thread runs within the context of a program and can execute independently while sharing resources with other threads. The Runnable interface is a key component in the Java concurrency framework. By implementing Runnable, developers create a thread's task without needing to subclass from the Thread class itself. This decoupling enriches code reusability and enhances flexibility. For instance:
In this simple example, a new thread is created which executes the run method from MyRunnable. The benefit here is clear: we can switch the Runnable's behavior without messing with the underlying thread mechanics.
Thread Synchronization Techniques
With multiple threads running, situations can arise where they attempt to access shared resources simultaneously. Such scenarios may lead to inconsistent data or application crashes. That's where thread synchronization swoops in like a guardian angel. Synchronization ensures that only one thread can access a resource at a time, preventing race conditions.
There are several techniques for synchronizing threads in Java:
- Synchronized Methods: Using the synchronized keyword, you can ensure that a method can only be accessed by one thread at any moment.
- Synchronized Blocks: More fine-grained control is enabled by synchronizing blocks of code, which can minimize the scope of the lock.
- Locks: Java has explicit locking mechanisms in the package, providing advanced capabilities beyond simple synchronization.
For example, here's how synchronized methods work:
Implementing synchronization is not without its cautions. Overusing it can lead to bottlenecks, making your application sluggish. Thus, understanding when and how to apply synchronization is crucial. As the saying goes, too much of a good thing can be bad.
In multithreaded environments, effective synchronization is the key to consistency, while balance remains essential to maintain performance.
Overall, mastering multi-threading in Java isn't just about learning the syntax; it’s about developing an instinct for when to use these powerful techniques to keep applications not just functioning, but thriving.
Error Handling and Exceptions
Error handling and exceptions form the backbone of robust and resilient Java applications. In the world of software development, even the most well-written code can encounter unexpected hurdles. Whether due to user input errors, system resource issues, or network anomalies, the ability to manage these exceptions is critical. This topic is essential not just for passing the hurdle of coding interviews, but for building trustworthy applications in real-world scenarios.
One of the primary benefits of handling exceptions properly is to enhance the user experience. When mistakes occur, having a system that can gracefully handle them without crashing is crucial. Furthermore, effective error management improves code maintainability. By systematically catching exceptions and implementing corrective actions, developers can create code that is easier to understand and troubleshoot. This all boils down to being proactive rather than reactive—anticipating potential failures before they snowball into bigger issues.
When preparing for interviews, it’s important to understand not only how to implement error handling but also when to use it. Java provides a structured way of dealing with exceptions through its built-in classes. This can also link to vital concepts such as checked versus unchecked exceptions, which are frequently discussed during interviews. Knowledge of these nuances displays a deeper understanding of Java that can impress interviewers.
"In programming, an error is not an obstacle; it’s an opportunity to enhance your code for the better."
Ultimately, mastering error handling and exceptions can serve as a strong indicator of one’s coding maturity as it reflects a developer's capability to foresee problems and resolve them adeptly. With that, let's move on to the practical implementations of error handling in Java.
How to Handle Exceptions in Java
In Java, handling exceptions is often done through the use of try-catch blocks. The typical structure goes like this: you wrap the code that may throw an exception in a block, and then use one or more blocks to handle specific types of exceptions that may arise.
Here’s a standard pattern to follow:
The block is optional, but it’s useful for releasing resources such as closing file streams or database connections, ensuring that certain actions are taken regardless of whether an exception was thrown. This contributes to writing clean, efficient code.
Additionally, remember that Java uses a hierarchy of exception classes, which means catching a super class can handle all its subclasses. However, one should aim to catch specific exceptions where possible to write more precise and user-friendly responses to errors.
Creating Custom Exception Classes
In some situations, built-in exception classes may not provide the granularity required for proper error reporting in your application. Here’s where creating custom exception classes comes in handy. Custom exceptions allow developers to define specific error situations relevant to their application’s domain.
To create a custom exception class, simply extend the Exception class (or one of its descendants) and implement a constructor that suits your needs. Here's an example:
With this setup, you can throw your exception as follows:
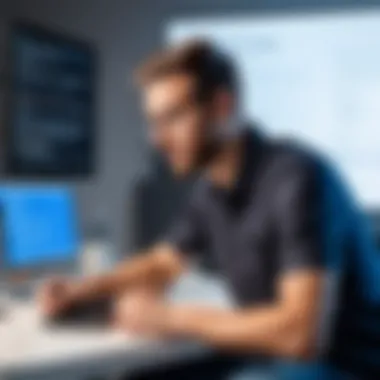
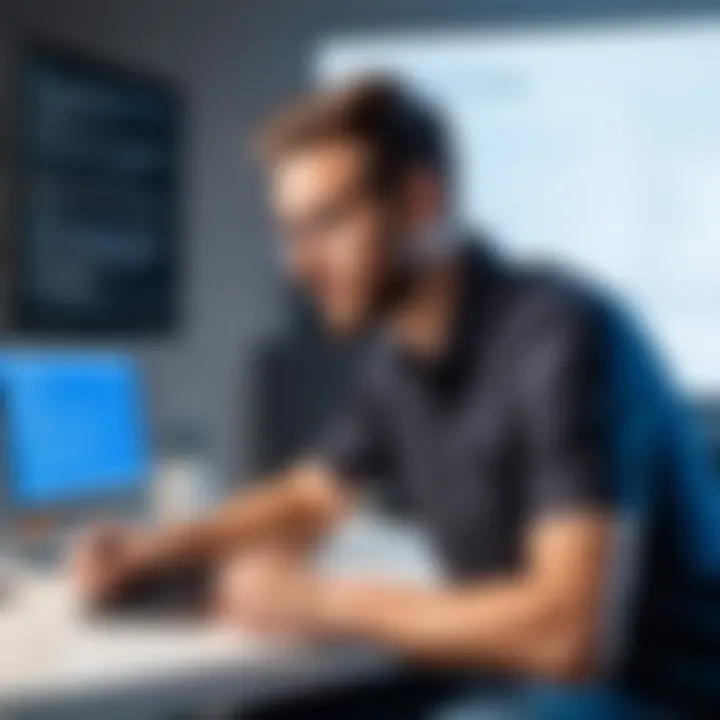
Creating custom exception classes not only helps categorize errors better but it also makes your error-handling strategy clear and maintainable. It can be particularly helpful when dealing with layered architectures where certain exceptions must be separated from more generic ones.
In summary, mastering error handling and creating custom exceptions can significantly elevate your programming skills. This knowledge is not just useful in tackling interview questions but is also a fundamental concept for producing reliable Java applications.
Practical Coding Approaches
In the realm of coding interviews, the significance of practical coding approaches cannot be overstated. This aspect goes beyond merely knowing theoretical concepts; it’s about translating that knowledge into practical solutions. Interviewers often seek candidates who can demonstrate not just problem-solving abilities but also a robust coding methodology. A solid grasp of practical coding can set one apart from other applicants. By tackling real-world problems with the right mindset, developers can showcase both their technical skills and their analytical thinking.
Effective Coding Practices
Effective coding practices form the backbone of any programmer’s success. These practices encompass a range of techniques that enhance code quality and ensure maintainability. Here are some important considerations:
- Simplicity is Key: Always aim to write code that’s straightforward. Complexity adds confusion and can lead to errors. Keeping your functions small and focused can significantly improve readability.
- Consistent Naming Conventions: Choose meaningful names for variables and functions. Avoid using vague names. If it's clear what a function does just by reading its name, it enhances collaboration when other developers skim through your code.
- Commenting Wisely: Comments are essential, but over-commenting can clutter the code. Explain why you’ve implemented something in a particular way, which aids others in understanding your logic without getting bogged down in too much text.
- Version Control: Familiarity with tools like Git allows for better code management and collaborative efforts. Tracking changes and understanding the history behind each revision can facilitate easier debugging and refinements.
"Code that is easy to read is easier to correct and adapt."
This principle highlights the importance of writing code with the understanding that future modifications are likely, be it for enhancements or bug fixes.
Debugging Common Errors
Debugging is an unavoidable aspect of programming. Insightful debugging skills can distinguish an average developer from an exceptional one. Common errors can range from simple syntax mistakes to complex logic errors. Here are some effective debugging strategies:
- Read Error Messages Denefully: Error messages are the best hints you can get. Understand your errors instead of glossing over them. For example, a indicates that your code tried to access an object that hasn’t been initialized.
- Break It Down: If you’re facing a big problem, try breaking it down into smaller pieces. Isolate segments of your code to locate where things may be going awry. Using a methodical approach can often reveal hidden issues.
- Print Statements: Although this may seem outdated, inserting print statements can be a quick way to inspect variable states throughout execution. It can help clarify the values of problematic variables at different points in the code.
- Unit Testing: Incorporate unit tests to verify each piece of the code works as intended. Testing as you go can save a lot of time and headaches down the line.
- Ask for Help: Don’t hesitate to consult peers or online communities like Reddit or Stack Overflow when you're stuck. A fresh pair of eyes might spot something you missed.
By honing effective coding practices and mastering debugging techniques, developers can confidently approach their coding interviews. They will not only present concepts clearly but also demonstrate a capacity for real-world problem-solving.
Mock Interview Scenarios
Mock interviews serve as a vital stepping stone in the journey of preparing for Java coding interviews. They provide a safe space where aspiring candidates can practice and hone their skills before facing real-world interview panels. The importance of this topic cannot be overstated, as it encompasses various elements that significantly enhance an individual's readiness.
One of the most notable benefits is the opportunity to simulate the pressure and dynamics of actual interviews. This simulation helps candidates to become accustomed to articulating their thought processes under scrutiny. In a real interview, things can get tense; you might have probing questions thrown at you with limited time to respond. Practicing in a mock setting can boost confidence and mitigate anxiety, allowing candidates to perform better when the stakes are high.
Moreover, mock interviews allow for an exploration of varied question types, from theoretical inquiries to hands-on coding problems. Participants should strive to create a realistic experience by incorporating elements such as:
- Time constraints: Set strict limits to reflect real interview conditions.
- Peer interviewing: Partnering with peers helps in gaining diverse perspectives on questioning styles.
- Feedback loops: Encourage constructive criticism that can guide candidates on areas needing improvement.
In sum, mock interview scenarios are essential tools in an interview preparation toolkit. They not only refine technical abilities but also enhance interpersonal skills, such as communication and problem-solving under pressure.
Simulating Real Interview Questions
When it comes to simulating real interview questions, having a structured approach is crucial. Candidates should focus on the types of questions they might face in a Java coding interview. These can range from general knowledge of Java concepts, such as Object-Oriented Programming, to specific coding challenges that might require writing algorithms or manipulating data structures.
Considerations for simulating these questions include:
- Source of Questions: Utilize platforms like reddit.com where many users share their recent interview experiences and questions.
- Diverse Question Pool: Ensure to cover a range of topics, from syntax-related questions to more complex algorithmic challenges.
- Adaptability: Questions should not only be fixed but should allow room for flexibility based on the interviewer's style.
Here’s a small example of a question to simulate:
Practicing such coding problems helps candidates become familiar with Java syntax and logic while providing experience in algorithm thinking.
Evaluating Responses and Providing Feedback
The evaluation of responses in mock interviews cannot be overlooked. Feedback is the cornerstone of improvement; it’s what transforms a good candidate into a great one. Effective feedback should focus on various factors, including clarity of thought, approach to problem-solving, and coding style.
Here are some effective strategies for giving constructive feedback:
- Specificity: Instead of vague comments, pinpoint exact areas of strengths and weaknesses.
- Balanced Feedback: Start with what went well, followed by areas for improvement. This helps maintain morale and encourages a growth mindset.
- Encouraging Self-Reflection: Prompt candidates to analyze their performance and thoughts on their handling of the questions. This step can often lead to deeper insights than direct feedback alone.
"Feedback is the breakfast of champions." – This saying highlights the necessity of constructive criticism in achieving excellence.
Culmination and Key Takeaways
Reflecting on the Interview Process
When stepping into an interview room, whether it's virtual or face-to-face, the pressure can be quite intense. It’s not merely about demonstrating what you know; it’s also about your approach to problem-solving, your thought process, and how you convey your answers. Taking a moment to reflect on past interviews can unveil areas for improvement.
- Preparation is Key: Familiarize yourself with common Java interview questions. This includes understanding how to articulate answers clearly and efficiently.
- Hands-On Practice: Simulated coding exercises can prepare you for the spontaneous nature of technical interviews. Practice coding on platforms like HackerRank or LeetCode to improve your speed and confidence.
- Feedback Matters: After mock interviews or even real ones, seek feedback. It's invaluable for recognizing your strengths and areas needing enhancement.
Reflection isn’t just a one-time activity; continuously seeking ways to refine your approach can lead to significant improvement over time. It promotes a mindset of growth, which is vital in the ever-evolving field of technology.
Continued Learning and Resource Recommendations
In the world of programming, stagnant knowledge is a dangerous place to be. Continuous learning should be at the forefront of any aspiring or experienced programmer's mindset. Here are some resources and strategies to keep your skills sharp and your mind engaged:
- Online Platforms: Websites like Coursera and edX offer a plethora of courses on Java and general programming. Explore topics that pique your interest or cover gaps in your knowledge.
- Books: Consider reading "Effective Java" by Joshua Bloch or "Java Concurrency in Practice" by Brian Goetz. These books are celebrated among Java developers and provide insights that are both practical and theoretical.
- Communities: Engaging in forums such as Reddit or Stack Overflow can keep you abreast of the latest trends and issues in Java programming. These platforms allow for real-time discussion and resource sharing.
- Practice Projects: Build personal projects that challenge your Java skills. This not only reinforces what you’ve learned but also demonstrates your capabilities to potential employers.
Continued learning is about fostering curiosity and dedication. With the right mindset and resources, the sky’s the limit in your programming journey!
"Learning never exhausts the mind." – Leonardo Da Vinci
By following these insights, you set yourself up not just for success in interviews but for a lifetime of growth in the field of programming.