Unraveling the Intricacies of Depth-First Search (DFS) in Java: An In-Depth Guide
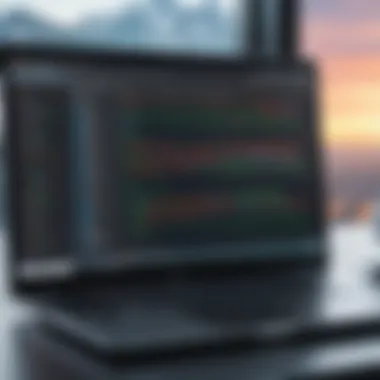
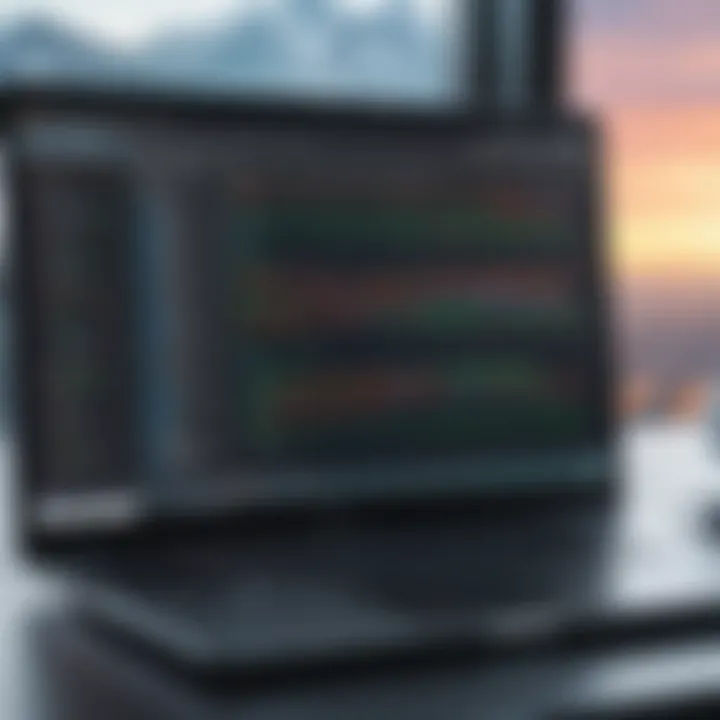
Coding Challenges
Deep-dive into the realm of Coding Challenges to enhance your proficiency with Depth-First Search (DFS) in Java. Unravel complex problems through Weekly Coding Challenges designed to push your problem-solving skills to new heights. Gain valuable insights into Problem Solutions and Explanations to deepen your understanding of DFS application. Discover essential Tips and Strategies for Coding Challenges, equipping you with the tools necessary to tackle any programming obstacle with finesse. Immerse yourself in Community Participation Highlights, where like-minded individuals come together to discuss and conquer coding challenges.
Technology Trends
Stay ahead of the curve with the latest Technological Innovations shaping the landscape of DFS implementation in Java. Explore Emerging Technologies to Watch that have the potential to revolutionize the way DFS operates in Java programming. Delve into the Technology Impact on Society, analyzing the implications of DFS advancements on various aspects of our digital world. Engage with Expert Opinions and Analysis, offering valuable perspectives on the ever-evolving field of DFS in Java.
Coding Resources
Equip yourself with a plethora of Coding Resources tailored to enhance your journey in mastering DFS in Java. Dive into comprehensive Programming Language Guides to deepen your knowledge of Java and its applications in DFS. Explore Tools and Software Reviews to streamline your development process and optimize DFS implementation. Immerse in Tutorials and How-To Articles that provide step-by-step guidance on leveraging DFS effectively. Compare Online Learning Platforms to identify the ideal resources for honing your DFS skills.
Computer Science Concepts
Unveil the foundational pillars of Computer Science Concepts underlying DFS in Java. Navigate through Algorithms and Data Structures Primers to grasp the fundamental elements driving DFS algorithms. Explore Artificial Intelligence and Machine Learning Basics to understand the interplay between DFS and cutting-edge technologies. Delve into Networking and Security Fundamentals to fortify your DFS implementations against potential threats. Peer into the future with insights on Quantum Computing and Future Technologies, envisioning the evolution of DFS in the digital landscape.
Prologue to Depth-First Search (DFS)
Depth-First Search (DFS) represents a fundamental algorithmic approach in graph theory. Its significance lies in its capability to systematically explore and traverse graphs, unraveling intricate patterns and connections that underlie complex data structures. In this comprehensive guide, the focus is on deciphering the inner workings of DFS in Java, offering a comprehensive understanding of its principles, applications, and implementation nuances. By delving into DFS, readers can enhance their problem-solving abilities and harness the power of this algorithm to navigate data structures effectively.
Understanding DFS Algorithm
The concept of backtracking
The concept of backtracking within the DFS algorithm is pivotal. This technique involves systematically exploring all possible paths from a starting point and backtracking when a dead-end is encountered. The essence of backtracking is its ability to efficiently search for solutions by exploring various options and reverting back when necessary, enabling a systematic and thorough traversal of graphs. While backtracking can be computationally intensive, its effectiveness in identifying solutions and analyzing complex data structures makes it a valuable tool in the DFS algorithm.
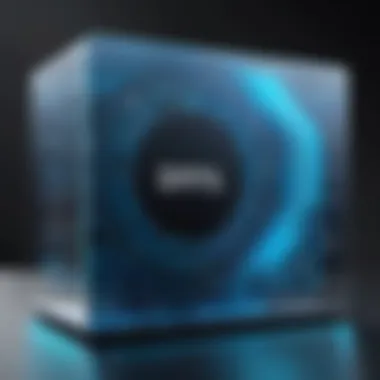
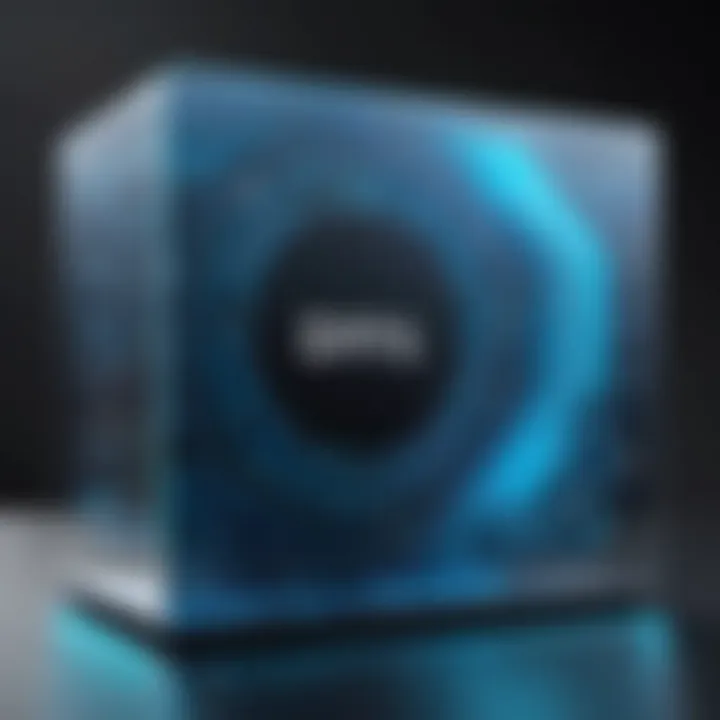
Stack-based traversal approach
The stack-based traversal approach is another critical component of the DFS algorithm. By utilizing a stack data structure to track and visit nodes, this approach enables a depth-first exploration of graphs, ensuring a systematic and efficient traversal mechanism. The key advantage of the stack-based approach is its ability to maintain the traversal path and backtrack when needed, allowing for a comprehensive exploration of connected components within graphs. Although stack-based traversal can consume additional memory due to maintaining the traversal history, its deterministic nature and ability to handle large-scale graph structures efficiently make it a favored choice for DFS implementation in Java.
Importance of DFS in Graph Theory
Detecting cycles in graphs
The ability of DFS to detect cycles in graphs is paramount in graph theory. By systematically traversing graph nodes and edges, DFS can identify cycles, loops, and interconnected components within complex graph structures. This capability is invaluable in various applications, such as determining connectivity patterns, analyzing dependencies, and identifying recurring patterns in data. Despite its computational complexity in certain scenarios, the cycle detection feature of DFS enhances the robustness and analytical depth of graph theory algorithms.
Finding connected components
DFS plays a crucial role in finding connected components within graphs, highlighting the interconnections and relationships between nodes. This functionality is essential for understanding the structural integrity of graphs, identifying disjoint sets of nodes, and analyzing the overall connectivity within graph representations. By systematically exploring connected components, DFS aids in data clustering, network analysis, and component-based optimizations. The versatility and analytical power of DFS in finding connected components contribute significantly to graph theory applications, providing valuable insights into the underlying graph structures.
DFS vs. BFS: A Comparative Analysis
Time complexity considerations
The analysis of time complexity is a key factor in comparing DFS and Breadth-First Search (BFS) algorithms. DFS, being a depth-first approach, emphasizes exploring routes sequentially, potentially leading to deep traversal paths before backtracking. This sequential nature impacts the time complexity of DFS, particularly in scenarios with deep recursive calls or long traversal paths. Understanding the time complexity implications of DFS is crucial in designing efficient algorithms and optimizing traversal processes in graph-based applications.
Space efficiency trade-offs
In contrast to BFS, which prioritizes breadth-first exploration, DFS focuses on depth-first traversal, potentially leading to deeper recursion levels and increased memory consumption. The trade-off between space efficiency in DFS and BFS lies in the nature of explorationโDFS favors depth-first search, maintaining a history of nodes visited on the traversal path, which can lead to increased memory usage. Balancing space efficiency considerations with algorithmic requirements is essential in leveraging the strengths of DFS while mitigating potential drawbacks associated with higher memory consumption. The strategic optimization of space efficiency trade-offs in DFS implementations is critical for ensuring optimal performance and scalability in graph traversal algorithms.
Implementing DFS in Java
When it comes to exploring the realm of Depth-First Search (DFS) in Java, the implementation holds a paramount position. Implementing DFS in Java paves the way for unraveling the algorithm's intricacies and applying them practically. By dissecting the code structure and delving into the recursive and iterative approaches, programmers gain a profound understanding of how DFS functions within Java frameworks. This section serves as the backbone for grasping the core concepts of DFS and sets the stage for in-depth exploration. The significance lies in the hands-on experience it offers to enthusiasts keen on mastering DFS within the Java programming landscape.
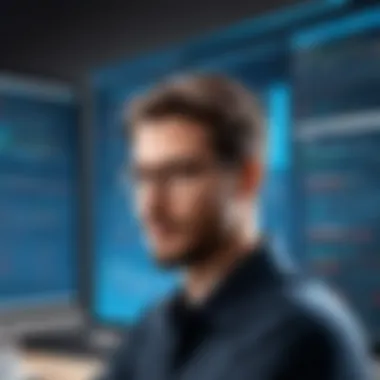
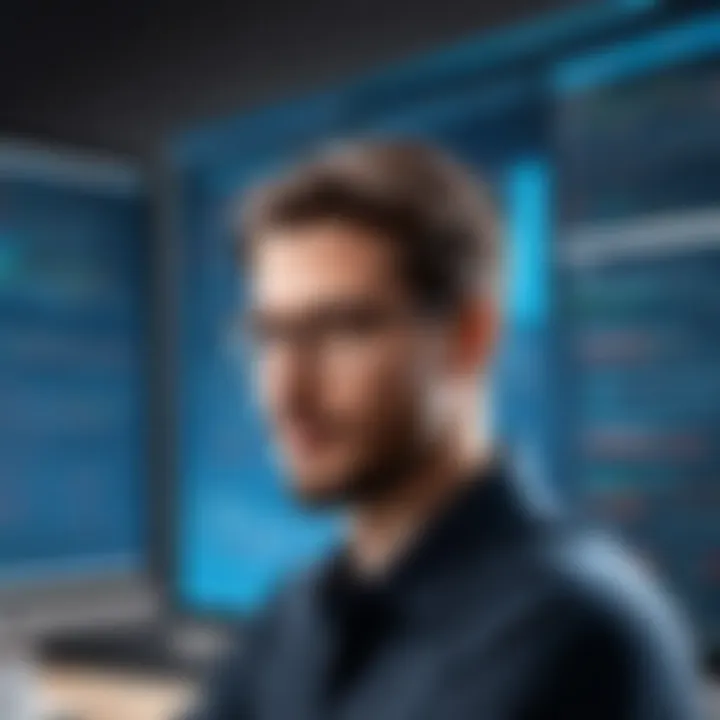
Recursive Approach
Code snippet for recursive DFS
The incorporation of a code snippet for recursive DFS stands as a cornerstone in comprehending the inner workings of DFS. This snippet encapsulates the essence of recursion, demonstrating how the algorithm traverses through nodes in a systematic manner. The recursive approach allows for a concise yet powerful implementation, simplifying the process of exploring graph structures. Its elegance and simplicity make it a favorable choice for programmers aiming to dive deep into DFS intricacies. Despite its efficiency, the recursive approach may exhibit limitations in handling extremely large datasets or deep levels of recursion, requiring careful optimization for optimal performance.
Depth-first traversal in trees
Delving into depth-first traversal in trees sheds light on the specific methodology adopted by DFS within tree structures. By prioritizing depth over breadth, this traversal technique follows a path until reaching the deepest node before backtracking. This approach proves beneficial in scenarios where deep exploration is preferred over broad analysis. The technique's simplicity and effectiveness make it a popular choice for navigating tree-based data structures using DFS. However, its linear nature may pose challenges when dealing with unbalanced trees or skewed branching, necessitating careful consideration of tree structure for efficient traversal.
Iterative Approach
Using a stack for iterative DFS
The utilization of a stack for iterative DFS introduces a different dimension to traversing graphs in a non-recursive manner. By mimicking the recursive call stack, this approach enables iterative exploration of nodes without the limitations of excessive recursion. The stack-based approach offers enhanced control over memory consumption and can handle larger datasets efficiently. Its versatility and scalability make it a preferred technique for implementing DFS in scenarios where recursion may be impractical or resource-intensive. However, managing the stack and tracking node visits require careful handling to prevent stack overflow or redundancy issues.
Impact on memory consumption
Analyzing the impact of memory consumption sheds light on the resource utilization dynamics of iterative DFS. Compared to the recursive approach, iterative DFS has a more predictable memory footprint due to stack management. By optimizing memory allocation and deallocation, programmers can streamline the traversal process and mitigate memory-related performance bottlenecks. The iterative approach's efficiency in memory usage enhances its applicability in handling large-scale graphs or complex data structures with minimal overhead. However, iterative traversal may introduce additional complexity in managing the stack structure and ensuring proper memory management to prevent memory leaks or inefficiencies.
Handling Graphs in Java
Understanding the intricacies of handling graphs in Java is crucial for efficient DFS implementation. Graphs serve as the playground for DFS algorithms, requiring robust data structures and traversal mechanisms. By adopting an adjacency list representation, programmers can efficiently store and navigate graph connections, facilitating seamless exploration of nodes. This representation excels in scenarios where sparse graphs are prevalent, offering a compact and resource-efficient storage mechanism. However, in densely connected graphs, the adjacency list may incur overhead in managing pointers and memory allocation, necessitating hybrid approaches for optimized performance.
Depth-first traversal implementation
Implementing depth-first traversal within Java bridges theory with practice, enabling programmers to translate graph theory concepts into functional code. By traversing graphs using the depth-first strategy, developers gain insights into graph connectivity and component structures. This implementation elucidates the inner workings of DFS, highlighting its role in uncovering graph properties and relationships. The depth-first traversal approach empowers programmers to analyze graph structures comprehensively, paving the way for diverse applications ranging from pathfinding algorithms to cycle detection. However, meticulous attention to edge cases and graph complexities is essential to ensure accurate traversal and optimal performance.
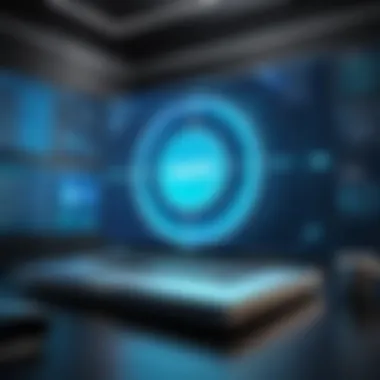
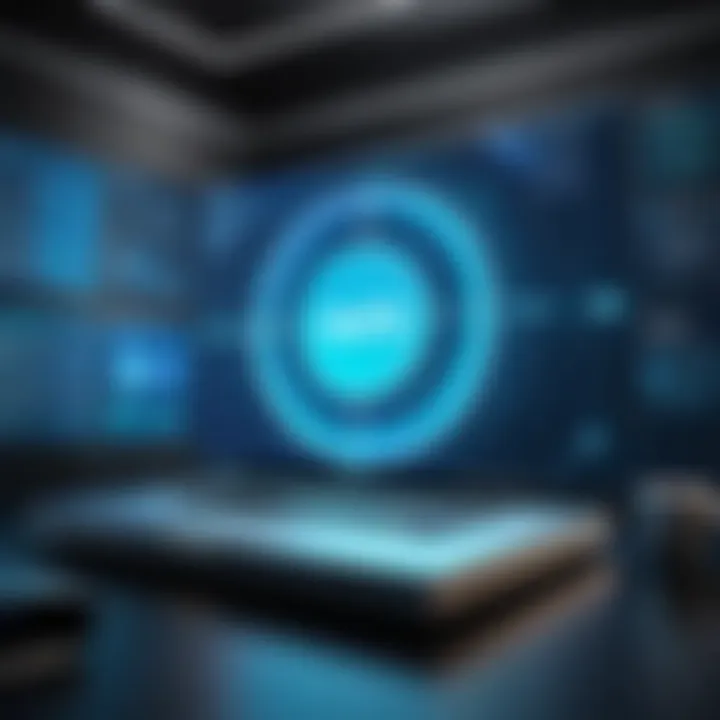
Applications of DFS in Java
The section on Applications of DFS in Java delves into the practical usage and significance of Depth-First Search in the Java programming realm. By focusing on real-world scenarios and problem-solving, this segment sheds light on how DFS can be leveraged to tackle complex challenges in various applications. From pathfinding algorithms to critical graph operations, the versatility of DFS in Java becomes evident. Understanding these applications not only broadens one's programming horizons but also enhances problem-solving skills through the utilization of an efficient algorithmic approach.
Pathfinding Algorithms
Finding shortest paths
One of the key aspects within Pathfinding Algorithms is the concept of Finding shortest paths. This fundamental technique plays a crucial role in optimizing traversal through graphs or networks, offering a solution to determine the most efficient route from one point to another. By minimizing the distance or cost involved in reaching a target node, Finding shortest paths streamlines navigation and resource allocation in various scenarios. Its intrinsic ability to enhance efficiency and streamline decision-making processes makes it a preferred choice in scenarios where optimization and resource utilization are paramount.
Exploring maze solutions
Within the domain of Pathfinding Algorithms, Exploring maze solutions stands out as an intriguing application. This particular aspect focuses on navigating complex mazes or layouts, ensuring that an optimal path is identified to reach a specific destination. By unraveling the intricacies of maze structures and simulating paths, this approach offers a practical way to solve spatial puzzles and optimize route planning. The unique feature of Exploring maze solutions lies in its ability to handle uncertain or dynamic environments, adapting pathfinding strategies to overcome obstacles and reach predefined goals efficiently.
Topological Sorting
Defining precedence relationships
Topological Sorting involves the critical task of Defining precedence relationships within a given dataset. This essential process establishes the order of tasks or elements based on dependencies, enabling a systematic arrangement that adheres to logical constraints. By defining the sequence in which activities must occur, Defining precedence relationships ensures a smooth flow of operations and enhances the overall structure of a system. Its distinctive feature is the capacity to resolve interdependencies and streamline workflows, making it an invaluable tool for organizing complex scenarios.
Sorting dependencies
Another vital aspect of Topological Sorting is Sorting dependencies, which focuses on arranging tasks based on their prerequisites and requirements. By structuring elements in a sequential manner that respects interconnections and dependencies, this approach fosters efficiency and coherence in the execution of operations. The key characteristic of Sorting dependencies lies in its ability to optimize processes by aligning activities in a logical order, thereby reducing bottlenecks and enhancing productivity. This feature makes it a preferred choice in scenarios where task prioritization and execution efficiency are paramount.
Cycle Detection
Identifying loops in graphs
Cycle Detection plays a pivotal role in identifying recurring patterns or loops within graph structures. By pinpointing cycles that may lead to inefficiencies or errors in computation, this aspect aids in ensuring the integrity and stability of algorithms and systems. The key characteristic of Identifying loops in graphs is its proactive approach to identifying potential issues before they escalate, thereby minimizing the risk of system malfunctions or inconsistencies. The advantage of this process lies in its ability to preemptively address critical issues and enhance the reliability of graph-based applications.
Preventing deadlock scenarios
Preventing deadlock scenarios involves preemptive measures to circumvent situations where system processes are unable to proceed due to resource conflicts. By implementing strategies to avoid deadlock conditions, this aspect aims to maintain operational continuity and prevent system stagnation. The unique feature of Preventing deadlock scenarios is its emphasis on proactive resolution of resource conflicts, thereby ensuring smooth workflow and uninterrupted system operation. The advantage of this approach lies in its ability to enhance system reliability and performance by mitigating the risks associated with deadlock situations.