Utilizing Exit in Python: An In-Depth Exploration
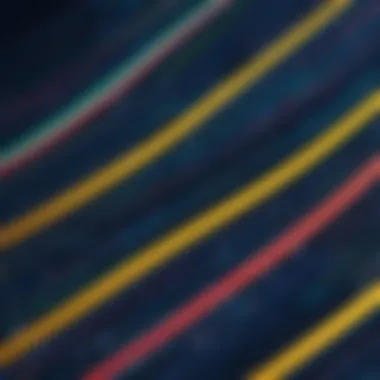
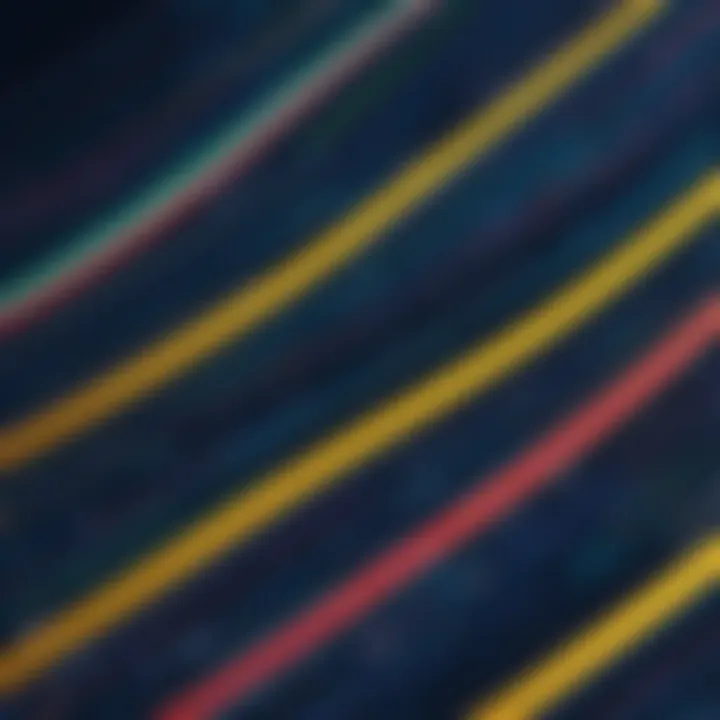
Intro
Understanding how to effectively terminate a program is a crucial skill for any programmer, regardless of their experience level. In Python, various mechanisms exist for this purpose, notably the function. This article examines the intricacies of the function and explores alternative methods for terminating code execution.
As you navigate the landscape of Python programming, it is essential to grasp not only when to terminate your programs but also the implications of such actions on overall code behavior and application lifecycle management. This topic is significant as improper termination can lead to resource leaks, incomplete transactions, or corrupted data.
In this exploration, we will delve deep into the following subtopics, ensuring a holistic understanding:
- How the function operates,
- Alternatives to the function,
- Common pitfalls associated with program termination,
- Best practices in managing program life cycles.
This guide is designed for aspiring and experienced programmers, technology enthusiasts, computer science students, and IT professionals. It will enhance your knowledge and improve your coding practices.
Let's begin by examining the programming challenges and scenarios where effective program termination plays a pivotal role.
Understanding Program Termination
In Python programming, understanding how and when a program should terminate is crucial. This topic not only involves knowing the mechanics of stopping code execution but also encompasses principles that guide effective program control flow. A well-managed termination process can make programs more reliable and easier to maintain.
Different situations require different methods of program termination. Whether due to an error condition or a natural flow of the application, how termination is handled influences the user experience and system resource management. Failing to implement proper termination could leave resources in an uncertain state, leading to memory leaks or locked files. Thus, appreciating the importance of program termination is essential for any programmer looking to write robust applications.
Defining Termination
Termination in programming refers to the process of concluding a program's execution. It can occur in various ways, such as reaching the end of the function or invoking a specific exit function. Each programming environment may define termination differently, but the essence remains similar: bringing the program to a controlled stop.
Terminating a Python program can be done explicitly or implicitly. Explicit termination involves calling functions like or . Implicit termination happens when the Python interpreter runs out of code to execute. Understanding these nuances is vital for effective program flow management.
Importance of Controlled Exit
A controlled exit is defined as stopping program execution in a predictable and safe manner. This is significant for several reasons. First, a controlled exit can ensure that all operations, such as closing file handles or freeing up memory, are conducted properly. It helps in maintaining system stability and integrity.
Moreover, a controlled exit can provide relevant exit codes. These are useful for debugging and can inform users or systems about why a program was terminated. For example, an exit code of zero typically indicates success, while non-zero codes signal various types of errors.
"Controlled exits can substantially reduce the complexity of error management in programming."
To summarize, understanding program termination is not just a technical requirement but a cornerstone of constructive software development. By mastering this area, programmers can assure that their applications operate smoothly and effectively manage resources.
Overview of Python Exit Methods
In the realm of Python programming, understanding how to effectively terminate a program is essential. This section provides a comprehensive overview of Python's exit options, including their respective functionalities and the contexts in which they are best applied. The various methods for program termination not only influence the flow of execution but also affect the overall user experience and system resource management.
Key Points to Consider:
- Flexibility and Control: Python offers multiple mechanisms for exiting a program, including built-in functions and library modules. Each method allows developers to exert specific control over how and when their programs terminate.
- Error Handling: Proper use of exit functions can improve error handling within applications. By choosing the appropriate exit method, programmers can signal specific conditions, making it easier to manage unexpected behaviors and enhance debugging.
- Portability: Some exit methods may behave differently across platforms. Recognizing these differences is critical for writing portable code that operates consistently regardless of the environment.
Overall, selecting the right exit method is crucial in ensuring that the program terminates gracefully. This provides a better experience for users and maintains system stability.
The exit() Function
The function is part of Python's built-in capabilities, providing a simple means to terminate a script. When invoked, it exits the program and can return an optional exit status. The default value is zero, signifying a successful termination.
To implement the function:
Using sys.exit()
Another well-known method for exiting a program is through . This function is generally preferred because it offers more nuanced exit options and is part of the sys module, which is always available in Python. The advantage of using lies in its ability to handle exceptions and to provide more detailed exit codes.
To use , you simply import the sys module and then call the exit function, optionally passing in an integer or string message detailing the exit reason. This is particularly useful in larger applications where multiple exit conditions might exist.
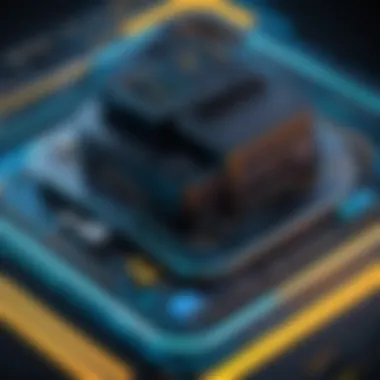
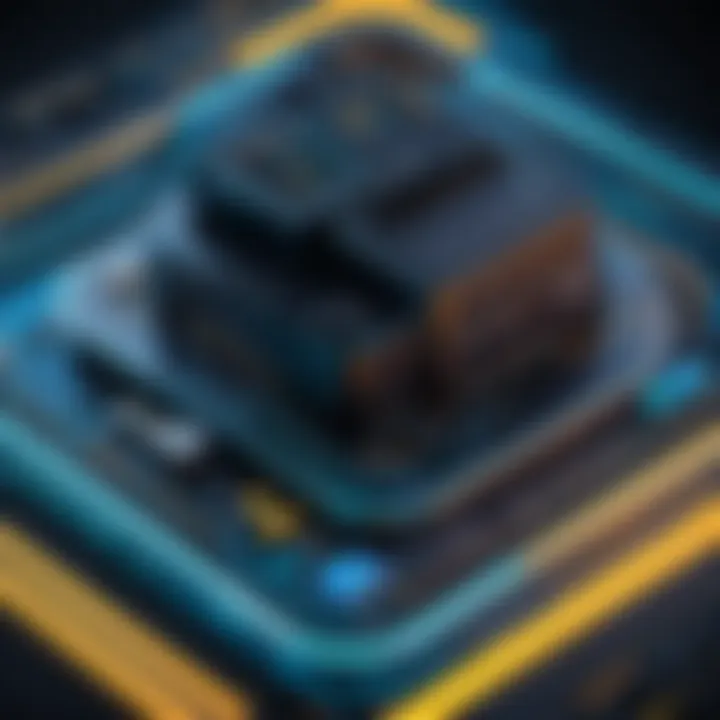
Program Termination with quit()
is another exit mechanism that can be utilized in interactive Python sessions, particularly in the REPL (Read-Eval-Print Loop) environment. While it serves a similar purpose to and , its use cases are generally more casual and often less suitable for production code.
Summary
Understanding the differences among these exit methods is crucial for leveraging Python's exit capabilities effectively. Each offers unique benefits, and selecting the right method can lead to more maintainable, clear, and controlled program terminations.
The exit() Function: An In-Depth Look
The function serves as a fundamental mechanism for halting a Python script. Understanding its implementation and the various contexts in which it can be applied is crucial for both beginner and advanced programmers. By utilizing the function, developers can effectively manage their script's termination process. This ensures that a program concludes in a predictable manner. Furthermore, using wisely can help prevent resource leaks and improve code maintainability.
When delving into the function, a few specific elements require attention. These include its syntax, how it handles different types of exit scenarios, and its relation to other methods of program termination. The decision to incorporate in responding to specific code conditions can enhance the readability and reliability of a program's control flow.
How exit() Works
The function is part of the module and is typically invoked with an optional integer argument. When called, it raises a exception behind the scenes, which causes the interpreter to stop the program's execution. If no arguments are provided, the function will exit with a status code of 0, indicating a successful termination of the program. However, when providing a specific exit code, such as a non-zero number, this typically signals an error state. This behavior aligns with traditional conventions in programming and operating systems, where exit codes are utilized to convey the status of a script or program execution.
Here is a simple demonstration of how operates:
In the above code snippet, the script executes and then calls , terminating with an exit code of 0. Conversely, should an error be detected, the argument could be changed to a non-zero value such as to indicate that an issue occurred.
Exit Codes Explained
Exit codes are a crucial aspect of program termination. They communicate the status of a script to the operating system or any calling process. By adhering to convention, exit codes can help other developers quickly interpret the outcome of a program's execution.
- Exit Code 0: This signifies successful execution without any errors.
- Exit Codes 1-127: These are typically reserved for general errors. Each code can represent specific issues, depending on the program and its context.
- Exit Codes 128 and Above: Commonly associated with various system-related issues that result in termination.
By understanding exit codes, programmers can implement robust error handling and improve debugging practices. This practice supports the overall health of code and ensures that systems are informed of their operational status.
Important Note: It is advisable to use exit codes in scripts that will be part of larger systems or frameworks, allowing for a clearer understanding of failure points and operational flow.
sys.exit(): The Preferred Method
When discussing program termination in Python, the function emerges as a vital consideration. This function allows developers to halt the execution of a script intentionally. Its significance is underscored by its integration into Python's core functionality via the module, providing not only a means to exit but also flexibility in how one can manage the exit process.
One of the primary benefits of using is the clarity it brings to code, especially when handling multiple exit scenarios. It communicates intent; when a programmer uses , it is clear that the program's flow is designed to stop under certain conditions. This can lead to more understandable and maintainable code. Moreover, can accept an exit status as an argument, which allows for more detailed signaling back to the operating system, indicating success or a specific type of error.
In addition, is better suited for larger applications, where cleanup operations and graceful termination are often required. Unlike other exit methods, can trigger user-defined cleanup actions, making it a more sophisticated choice for both small scripts and advanced applications. This versatility is essential for ensuring that resources are properly released and that the state of the system remains stable after termination.
Understanding the sys Module
The module is a fundamental part of Python, providing access to variables and functions that interact with the Python interpreter. Before utilizing , it is essential to understand its role within this module.
The module enables communication with the interpreter and includes several vital components like input/output handling, command-line arguments, and important termination functions. is included in this module, serving as a gateway for intentional exits from a script or application. It essentially provides a structured way to terminate a program, which can be particularly beneficial in complex applications where clarity and control over the exit flow are crucial.
sys.exit() Parameter Options
When employing , understanding its parameter options is critical for leveraging its ability to control termination effectively. This function accepts one optional parameter, which can be an integer or an instance of .
- Exit Code: When an integer value is passed, it serves as the exit status code. A code of 0 generally indicates a successful termination, while any non-zero value represents an error. This feature is helpful for scripts that may be called by other automated processes or scripts, as it can inform the caller about the success or failure of execution.
- Exception Instance: Additionally, if an instance of is passed, it will cause the interpreter to raise that exception. This functionality allows for high flexibility where the exit condition can be tied to exceptional cases in the program flow. Itโs a way to express termination through errors, which is often a necessary part of robust application design.
By understanding these parameter options, programmers can utilize effectively to manage their Python programs' termination properly. This understanding can lead to a more controlled and predictable operation of scripts and applications.
Alternatives to Explicit Exit
When working with Python, many developers encounter situations requiring program termination. While explicit exits using functions like or are common, alternatives exist that can provide more controlled and often cleaner termination processes. Understanding these alternatives can enhance code maintainability and readability, which are crucial in larger projects where multiple paths of execution might be present.
Adopting alternative methods to explicit exit ensures that the program can conclude gracefully. It helps in managing resources such as open files, network connections, and other critical elements. This section delves into three significant alternatives to explicit exit: exception-driven termination, conditional program exit, and using return statements.
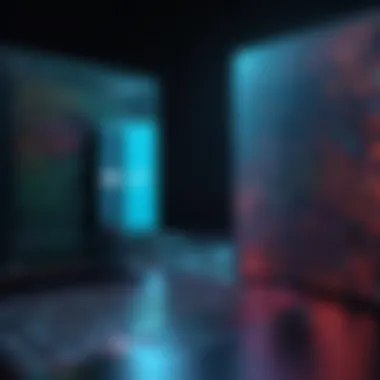
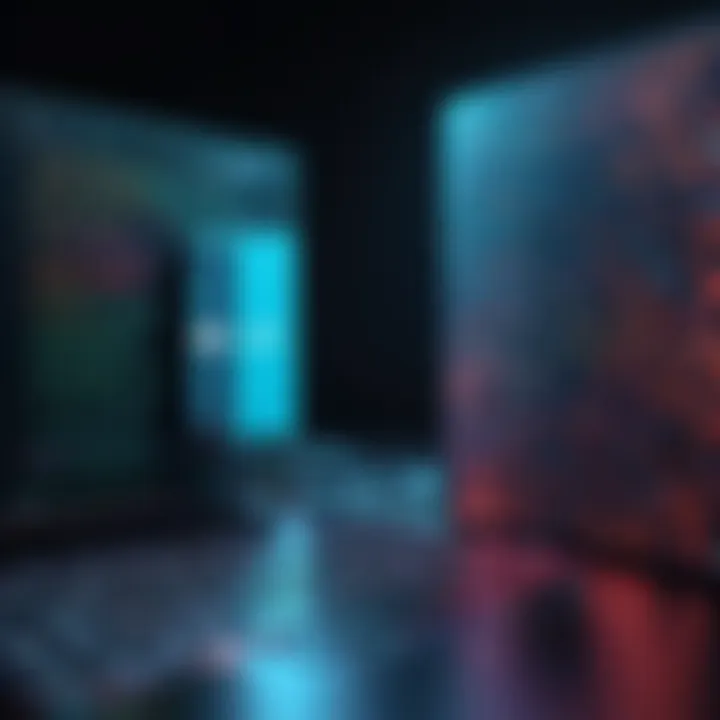
Exception-Driven Termination
Python supports a robust mechanism for handling errors through the use of exceptions. Exception-driven termination involves utilizing built-in or user-defined exceptions to signal when a program should stop executing. This method provides a way to handle unexpected situations without explicitly calling an exit function.
When an exception occurs, control is transferred to the nearest exception handler. This allows the program to terminate cleanly while also executing any cleanup code in the block, should one exist. Exception handling can also allow the program to recover from certain errors, instead of terminating immediately. A common approach is using the , , and blocks.
For instance:
In this structure, if raises an error, the program does not abruptly exit but instead executes the error handling defined in the block. This emphasizes the reusability and control offered by exception-driven termination.
Conditional Program Exit
Another effective alternative is the conditional program exit, which focuses on checking specific criteria before deciding to terminate the program. This method not only allows programmers to maintain flow control but also enhances readability. Conditions can be based on user input, state variables, or other logical constructs.
For example, you might opt to exit a loop or function based on a userโs response:
In the above code snippet, the program checks the user's input before deciding to continue execution or terminate. This method is particularly valuable in interactive applications where user experience is key.
Using return Statements
When writing functions, using statements can also serve as a method of terminating program execution. This approach is especially useful in avoiding the pitfalls of abrupt termination from an explicit exit. Instead of ending the entire program, the return statement can terminate a specific function and hand control back to the calling code. This provides a clearer flow of execution and enhances modularity.
For instance:
In this example, if is absent, the function exits by returning a message instead of halting the program entirely. This technique promotes encapsulation and allows the main program to manage various parts of execution efficiently.
Using these alternatives can reduce dependencies on explicit exit calls, leading to smoother and more maintainable code.
In summary, exploring alternatives to explicit exit methods is beneficial for both functionality and readability in Python programming. By relying on exception-driven termination, conditional program exit, and return statements, developers can ensure their programs terminate without neglecting essential operations.
Common Mistakes in Program Termination
Understanding the common mistakes that programmers make during program termination is crucial for maintaining code quality and ensuring smooth execution. This section highlights frequent pitfalls associated with program exit strategies, examining their implications and offering solutions for improvement. By identifying these mistakes, developers can enhance their coding practices and achieve better control over application behavior.
Overusing exit() in Scripts
The function is a useful tool in Python for terminating a program. However, its overuse can lead to several issues. While it seems straightforward to call whenever a condition is met, it is often better to use more controlled exit mechanisms.
The primary concern with overusing is that it can create abrupt terminations. Abrupt endings can leave some processes incomplete, especially if they involve external resources such as files or network connections. When using , the execution of the program halts immediately, not allowing the interpreter to process any remaining code or cleanup tasks. This can lead to resource leaks and corrupted data.
Here's an example of a common misuse in scripts:
In more complex applications, it is advisable to handle errors gracefully. This may involve raising exceptions or using return statements to let the caller manage the process flow. This way, the application can ensure all necessary cleanup actions are performed before termination.
Important Note: Overusing can make debugging difficult, as it can obscure the flow of execution in your program.
Ignoring Cleanup Operations
Proper cleanup is a vital aspect of program termination that should not be overlooked. Ignoring cleanup operations can lead to unintentional consequences, including memory leaks and data loss. It is essential to ensure that any resources are released properly before a program exits.
In many cases, programmers might forget to close files, release network connections, or perform other necessary cleanup tasks. This could result in increased memory usage or corrupted files. A good practice is to use context managers, which automatically manage resource cleanup. For example, instead of manually closing a file, you can use the statement:
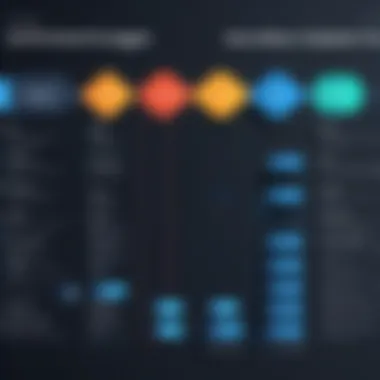
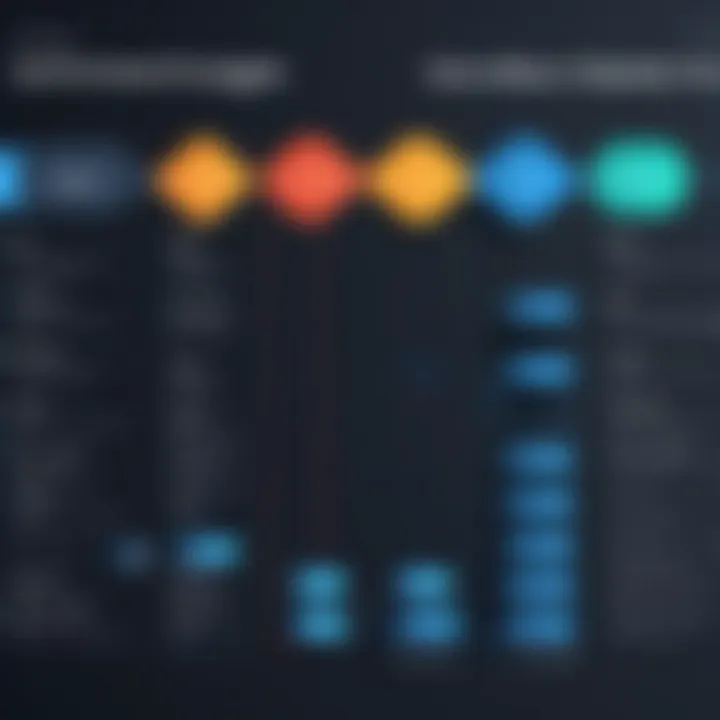
Additionally, try to wrap your exit logic in a way that ensures cleanup takes place at the end of your program. Consider using try-finally constructs to guarantee that resources are cleaned up no matter what happens during execution. Here is an example:
By adhering to meticulous cleanup procedures, one improves resource management and enhances overall program stability.
Debugging Program Exit Issues
Debugging program exit issues is a critical aspect of Python development. When a Python program does not terminate as expected, it can lead to unresolved resource utilization, incomplete data processing, and a frustrating experience for the user. Understanding the intricacies of program termination allows developers to create more robust code. This section will focus on two key elements: identifying exit points within the code and using logging techniques to diagnose exit issues.
Identifying Exit Points
Identifying exit points within a program is crucial to ensure smooth termination. Exit points are locations in the code where the program is expected to terminate, whether through normal operation or when an error occurs. Here are several strategies to identify these points effectively:
- Review the Code Path: Carefully analyze the control flow of your application. Follow conditional statements and loops to see where the logic leads to terminal points.
- Use Comments: Document potential exit points thoroughly. Comments can help clarify intentions and make it easier to spot areas that may require attention during debugging.
- Utilize IDE Features: Many Integrated Development Environments offer debugging tools to step through code. This allows you to see the exact flow and how variables change in real-time.
Understanding where exits occur helps prevent unexpected behavior during runtime. Without proper identification, a programmer may inadvertently create unwanted exit scenarios, leading to data loss, resource leaks, or hard-to-debug errors.
Using Logging for Debugging
Logging is an invaluable tool for debugging exit issues in Python programs. Effective logging can provide insights into program behavior right before it terminates. Here are key practices to implement:
- Set Up a Logging Configuration: Start by establishing a logging configuration that captures different levels of information. Include , , , and to track the execution flow.
- Log at Specific Exit Points: Place log statements just before each identified exit point. This will help clarify the execution path taken before termination.
- Log Exceptions: In case of abrupt exits due to errors, log exception details. This provides a complete picture of what went wrong and where.
- Analyze Logs: After running the program, analyze the logs systematically. Look for patterns and trigger points that may indicate unexpected exits.
Using logging not only helps in understanding why a program may not exit properly but also forms a solid foundation for improving overall code quality and maintainability. As you iterate on your code, logs serve as a reference that facilitates further refinement and error handling.
Best Practices for Program Termination
When programming in Python, how a program terminates can greatly influence its efficiency and user experience. Understanding best practices for program termination is essential. Implementing a thoughtful approach can minimize errors and enhance code readability.
Clear termination practices lead to maintainable code. Developers can quickly grasp program flow and identify potential issues. It also aids in simplifying the debugging process. As projects grow, clarity in exit strategies can significantly reduce the time spent on troubleshooting.
Prioritizing Readability
Readability is a cornerstone of effective programming. It refers to how easily another developer can read and understand your code. In the context of program termination, prioritizing readability involves using clear exit methods and well-defined error handling. Developers should avoid cryptic exit codes or overly complex exit logic. Instead, favor straightforward commands like or .
Code snippets should clearly document when and why a program exits. Maintain a consistent style throughout your code. For example, consider adding comments near exit statements:
Additionally, it is helpful to use meaningful exit codes. For instance, using 0 for successful execution and integers greater than 0 for specific error conditions enables quick identification of issues.
Ensuring Consistent Error Handling
Consistency in error handling greatly enhances a program's stability. Using systematic approaches to manage errors reduces the risks associated with unexpected termination. Error handling strategies such as try-except blocks are imperative. This ensures that the program can gracefully respond to errors rather than exit abruptly.
An organized structure for managing exceptions promotes consistency. This can look like the following example:
By clearly defining how different errors are handled, you can provide insight to the users and developers maintaining the code in the future. This practice ensures that they know what each exit code signifies.
Consistent error handling reduces the panic associated with program failures and enhances the user experience.
The End
In this article, we explored the complexities surrounding program termination in Python. The conclusion is a vital component because it consolidates all discussed elements. This section emphasizes the importance of understanding how to effectively manage program exit points. Without proper management, programs can produce unintended consequences, like resource leaks and unpredictable behavior.
Recap of Key Points
- We examined the various exit methods in Python, such as the function and .
- Detailed ways of leveraging these methods, including understanding exit codes, were discussed.
- Alternative strategies to explicitly terminating programs were highlighted.
- We also analyzed common pitfalls that many programmers encounter, such as overusing the function, which might hinder code readability and maintainability.
- The debugging strategies presented can help pinpoint issues related to program termination and manage them effectively.
Future Implications in Python Programming
As Python continues to evolve, the implications for program termination will also progress. The handling of exceptions and errors will likely become more sophisticated, providing programmers with finer control over exit points. Adopting best practices in program termination will not only enhance code quality but also improve the overall execution of applications. Understanding these concepts will be crucial for all developers who wish to create robust and maintainable code. The landscape of Python programming is constantly changing, and staying informed about these aspects can greatly influence a developer's effectiveness in the field.