Exploring Ordered Maps: Features and Applications

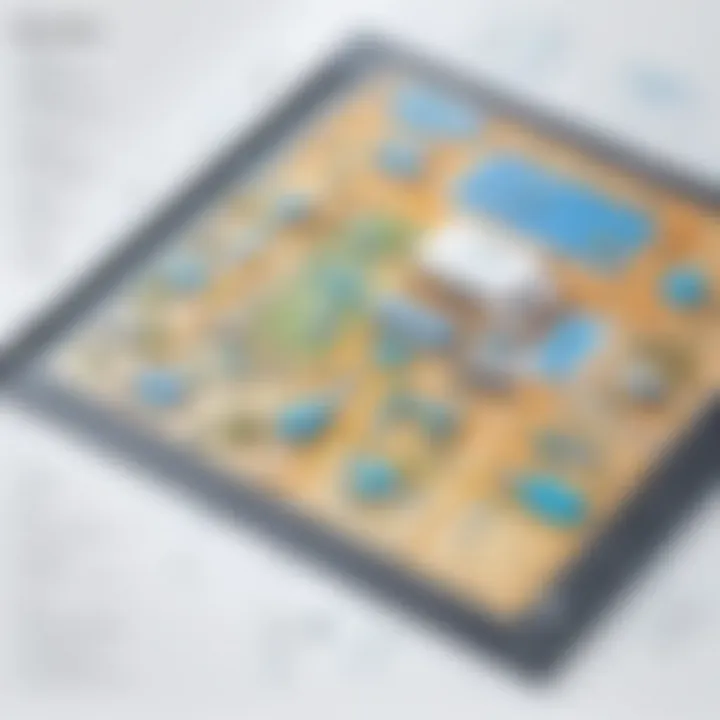
Intro
Ordered maps are a fascinating data structure that combine features of both arrays and traditional hash maps. They maintain the order of elements, allowing for predictable traversal while also offering efficient key-value pair access. This unique combination makes ordered maps particularly powerful in various programming contexts, from algorithm design to real-world application development.
In this article, we explore the intrinsic characteristics of ordered maps. We will discuss their practical applications in software development and data manipulation. As technology enthusiasts or computer science students, understanding ordered maps will provide valuable insights into optimizing your programs and enhancing data handling efficiency. This discussion will enlighten you about the advantages of using ordered maps over conventional data structures.
Key points to be discussed include:
- Defining Ordered Maps: What they are and why they are used.
- Applications in Real World: How they are utilized across various software projects.
- Comparison with Other Data Structures: Understanding their strengths and weaknesses.
- Impact on Performance: How ordered maps can enhance the efficiency of code.
Understanding these aspects will equip you for better decision-making in your coding endeavors and improve your problem-solving strategies. Let us delve deeper into the specifics by examining coding challenges related to ordered maps.
Understanding Ordered Maps
Ordered maps play a critical role in organizing data within software development. Their significance stems from the ability to maintain sequence while providing efficient access to values associated with specific keys. Understanding ordered maps enables programmers to enhance data manipulation techniques, thus improving overall system performance. Moreover, ordered maps are especially valuable when the order of entry has a functional impact on the application, such as in applications dealing with time-series data or ordered logs. This segment will delve into the nuances of ordered maps, providing insights into their structure, historical background, and evolution.
Definition of Ordered Map
An ordered map is a data structure that pairs keys with values while maintaining the sequence of keys. Unlike unordered maps, which do not preserve the order of their elements, ordered maps ensure that elements are retrieved in the same order as they were inserted. This characteristic makes ordered maps ideal for scenarios where the sequence of data entries is essential.
In programming languages such as C++ or Python, an ordered map can be implemented using classes or dictionaries that support ordering. The common implementation provides functions to insert, remove, and access key-value pairs while respecting the sequence. Therefore, the ordered map combines the benefits of both associative arrays and linked lists, offering flexible data organization.
History and Evolution
The concept of ordered maps has evolved significantly since the advent of programming. Initially, data structures were relatively simple. They focused on efficiency or order but rarely both at the same time. The development of algorithms and programming languages led to the birth of more complex structures.
The introduction of associative arrays laid the groundwork for the development of ordered maps. As programming needs grew, so did the complexity of data structures. With the emergence of languages like C++ in the early 1980s and Python in the 1990s, the need for ordered maps became clearer.
In C++, the class provides a built-in ordered map mechanism, while in Python, offers similar functionality. The ability to maintain order became a desirable feature, especially as data processing needs expanded in web applications and data analytics.
As computer science continues to advance, ordered maps remain relevant, adapting to new requirements and applications in software development. Understanding their lineage allows developers to appreciate their utility and complexity, leading to better implementation in modern programs.
Characteristics of Ordered Maps
Understanding the characteristics of ordered maps is essential in grasping their role in computing and software development. Unlike unordered maps, ordered maps maintain a defined sequence for their key-value pairs. This unique trait not only provides structure but also allows programmers to execute operations in a more predictable manner. The ordered nature of these maps is vital for applications where the order of insertion is significant or where sorted order is required in outputs.
Key Features
Ordered maps possess several key features that distinguish them from other data structures. These characteristics include:
- Maintained Order: The primary feature of ordered maps is their ability to preserve the sequence of entries as they are inserted. This characteristic is beneficial in scenarios where order impacts data processing.
- Iteration Order: When iterating through the entries in an ordered map, the order remains consistent with the insertion order. This is particularly useful for algorithms that rely on predictable data traversal.
- Performance Predictability: Insertion, deletion, and access times in ordered maps can be more predictable, which is important for performance-critical applications. The algorithms can often guarantee a certain complexity, which aids in making data-driven decisions.
- Range Queries: Some implementations of ordered maps allow for efficient range queries. This means that retrieving data within a certain range can be performed quickly.
These features imbue ordered maps with advantages in certain use cases, making them a valuable tool for developers.
Comparative Analysis with Unordered Maps
When analyzing ordered maps versus unordered maps, several distinctions arise that highlight the respective strengths and weaknesses of each structure.
- Order Preservation vs. Fast Access: Ordered maps keep data in a specific sequence based on insertion, while unordered maps prioritize fast access to the data using hash functions. This makes unordered maps potentially faster for lookups but lacking in order-sensitive operations.
- Memory Overhead: Ordered maps often require additional memory to maintain order. The structures that facilitate this ordering come with extra overhead in terms of space.
- Use Cases: Unordered maps are effective in applications where arbitrary order is acceptable, such as counting frequencies of elements. Ordered maps excel in scenarios where the order of data is important, such as maintaining the chronological order of events.
- Time Complexity Differences:
- In unordered maps, average time complexity for insertion and access is often O(1). In contrast, ordered maps usually achieve O(log n) due to their inherent ordering.
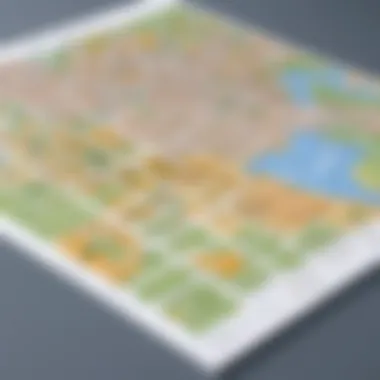
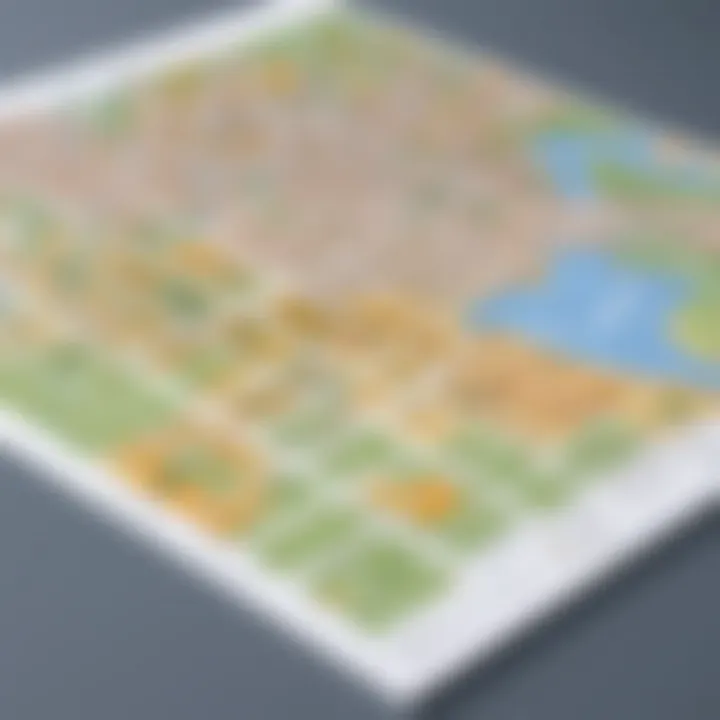
The choice between ordered and unordered maps often depends on the specific requirements of the application, including considerations such as retrieval speed, memory usage, and the importance of maintaining order.
In summation, understanding the characteristics of ordered maps provides a clearer picture of their utility and importance in software development. Their unique benefits and specific use cases mark these data structures as essential tools for both novice and experienced programmers.
Common Implementations of Ordered Maps
The use of ordered maps is becoming increasingly vital in the realm of software development. Their unique characteristics allow for organized data storage and efficient access methods. Understanding common implementations of ordered maps can significantly improve a programmer's ability to manage data effectively. Here, we explore the importance, advantages, and specific considerations of both standard libraries and custom implementations of ordered maps.
Standard Libraries and Frameworks
Standard libraries provide a ready-made solution for utilizing ordered maps in programming. For example, in C++, the and facilitate ordered and unordered collections respectively. The is crucial as it maintains its elements in a specific order based on keys.
In Python, the from the module serves a similar purpose by preserving the order of entries. This becomes valuable when the sequence of operations matters for the correct functioning of algorithms, especially in data-intensive applications.
Utilizing these libraries simplifies the development process. Developers can avoid the intricacies of implementing their own data structures and focus on higher-level problem solving. Moreover, well-established libraries are typically optimized for performance and reliability, providing better overall efficiency.
Benefits of Using Standard Libraries:
- Time Efficiency: Rapid deployment of ordered maps without extensive coding.
- Reliability: Tested and maintained by communities for bug fixes and updates.
- Ease of Use: Familiarity for programmers enhances productivity.
"Standard libraries are the backbone of modern software development, allowing programmers to implement complex data structures with minimal effort."
Custom Implementations
While standard libraries offer convenience, custom implementations of ordered maps are also prevalent. Programmers may choose to create their own ordered maps to fulfill specific requirements or optimize performance for unique use cases. This approach allows for tailored features that are not available in standard options.
Custom implementations can be engineered to handle data in a particular way that matches the application's needs. For instance, a developer might design an ordered map that integrates specific hashing functions to optimize lookups or modify balancing algorithms for quicker access times.
However, building a custom ordered map requires a strong understanding of data structure properties and nuances. Programmers must carefully consider aspects such as memory allocation, rehashing strategies, and performance trade-offs.
Considerations for Custom Implementations:
- Complexity of Design: Greater effort in planning and coding.
- Performance Optimization: Allows for tailored enhancements that can boost efficiency for specialized tasks.
- Maintenance Overhead: Custom-built solutions demand ongoing attention and updates.
In summary, whether using standard libraries or developing custom implementations, understanding ordered maps' applications is essential for programmers aiming to optimize data management in their work.
Performance Aspects of Ordered Maps
Understanding the performance of ordered maps is crucial for programmers and developers who want to make informed decisions during implementation. These data structures possess unique attributes that can significantly affect how efficiently data is stored and accessed. Performance aspects primarily encompass two critical areas: time complexity and space complexity.
Time Complexity
Time complexity defines the amount of time an operation takes relative to the size of the data set. In ordered maps, various operations—such as insertion, deletion, and lookup—behave differently compared to unordered maps. The efficient handling of these operations is essential, especially in applications where speed is critical.
For instance, accessing a value in an ordered map is done in O(log n) time when using a balanced tree structure like Red-Black or AVL trees. This is more efficient than a linear search approach, which would take O(n) time in an unordered map. Meanwhile, insertion and deletion operations in balanced trees also typically maintain this O(log n) complexity. Overall, the logarithmic time complexity makes ordered maps preferable when operations on large datasets are frequent.
Space Complexity
Space complexity is another vital performance aspect, referring to the amount of memory an algorithm uses as a function of the input size. Ordered maps generally require more memory than unordered maps because they need to maintain the order of elements. Each entry in an ordered map often includes pointers or references alongside the actual data, thus consuming a greater amount of space. For example, if you consider a Red-Black tree implementation, the overhead of storing parent pointers will add significant memory use compared to simpler structures.
However, the additional memory usage can be justified when accounting for the benefits of maintaining order, as it allows for efficient traversal and improved access patterns. For developers, carefully weighing the trade-offs between time and space complexity when working with ordered maps is crucial.
"Efficiency in use of time and space is paramount in software development, particularly in applications that demand optimal performance."
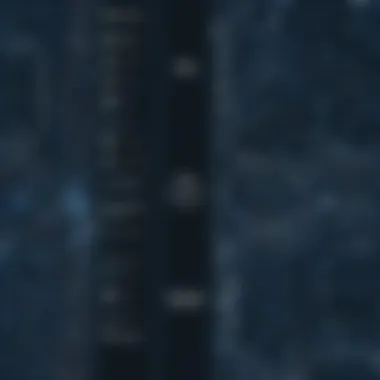
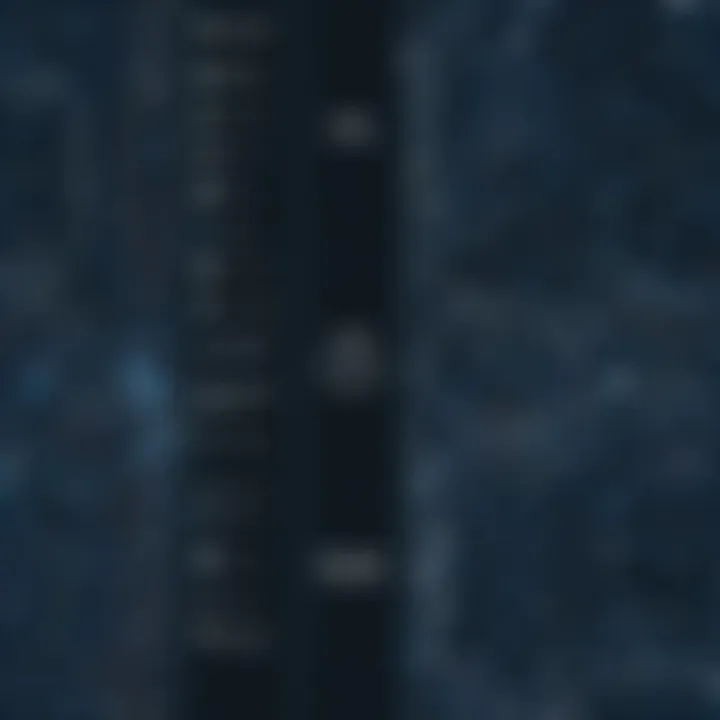
When implementing ordered maps, think about the specific needs of your application. Consider the expected operations and their frequencies. Doing so ensures that you leverage the capabilities of ordered maps efficiently while balancing both time and space complexities for improved performance in software development.
Use Cases in Software Development
Understanding the use cases of ordered maps is crucial for programmers. They offer distinct advantages over traditional data structures. In software development, ordered maps enhance efficiency when dealing with data that must remain sorted or when quick access by key is required. Additionally, their structure allows for predictable iteration order. This characteristic becomes particularly important in scenarios where the sequence of data matters.
Applications in Data Processing
Ordered maps play a significant role in data processing due to their inherent arrangement of data. One common application is in handling data streams, where maintaining order while processing incoming data is essential. For example, when dealing with time-series data, developers can leverage ordered maps to efficiently store and retrieve timestamps alongside their corresponding values. This allows for quick lookups and organized outputs, essential for analysis or reporting.
Furthermore, ordered maps can significantly improve the performance of algorithms that require sorted data. Many algorithms, like those used in search operations or range queries, benefit from the efficiency that ordered maps provide. When processing large datasets, the ability to access data in a specific order can reduce the time complexity and optimize resource usage.
In addition to time-series data, ordered maps can also be advantageous in mapping configurations, settings, or user preferences. Developers can maintain a consistent order while allowing for quick access and modifications. This structured approach leads to better code organization and improves debugging processes.
Integration in Web Development
In web development, ordered maps can enhance the management of application states and configurations. Frameworks like React or Angular often require certain structures to handle component states. By using ordered maps, developers can store states with key-value pairs, ensuring that the order of entries remains intact. This order is crucial for rendering components accurately based on user interactions or state changes.
Moreover, ordered maps can facilitate the handling of data sent to and from APIs. Many applications interact with databases or external services that return results in a specific order. Using ordered maps enables developers to maintain this order, which simplifies the processes of displaying data. Additionally, data serialization processes can become more intuitive when using ordered maps, allowing for consistent representations.
Another significant use case in web development is for URL parameters. When processing query strings, ordered maps offer a systematic way to store parameters while maintaining the order they appear in the URL. This is very useful when parameters have significance in specific sequences.
"The order of parameters can often influence how the server processes a request."
Advantages of Using Ordered Maps
Ordered maps present a distinct array of advantages that underscore their significance in programming contexts. Using an ordered map often leads to a more organized approach to handling key-value pairs compared to other data structures. This structure is crucial, especially in applications where order matters. Below are key aspects that highlight the benefits of using ordered maps:
Data Organization
An ordered map maintains the order of its elements based on the key insertion sequence. This feature allows developers to manage and retrieve data with context-aware ease. For instance, if users require data to be processed in the same sequence it was entered, an ordered map becomes an indispensable tool. This characteristic is particularly beneficial in scenarios involving configuration settings or user preferences. Using an ordered map in such cases can enhance clarity and structure.
Data organization in ordered maps is represented as follows:
- Insertion Order: Data remains accessible in the order that it was entered, ensuring a logical flow of information.
- Predictability: The retrieval of keys follows the same order, allowing developers to predict the sequence of data handling.
- Simplified Management: Grouping data points by order aids in easier navigation and management, especially in complex applications.
Ease of Iteration
Iterating over data in an ordered map is comparatively straightforward. This simplicity of iteration stems from the fact that the order is preserved, allowing developers to traverse elements predictably. In programming, iteration is often a frequent requirement, so an efficient way to loop through values is invaluable. Benefits of easy iteration include:
- Consistent Access: Developers can loop through elements with confidence that the order will remain constant, which is particularly important in algorithm-driven processes.
- Streamlined Code: Simplified iteration routines can lead to cleaner and more readable code, which aids maintainability.
- Integration with Functions: Many programming tasks involve applying functions to a dataset. Ordered maps facilitate direct application of functions to elements, enhancing functional programming practices.
"In software development, clarity and efficiency in handling data structures can significantly influence performance and maintainability. Ordered maps offer such advantages, establishing them as a favored choice among developers."
In summary, the virtues of ordered maps—ranging from data organization to ease of iteration—render them an effective tool for programmers keen on enhancing their data manipulation practices. Their particular advantages become more evident as software complexity grows, emphasizing the importance of strategically using ordered maps in various applications.
Challenges and Limitations
In the context of ordered maps, it is crucial to examine the challenges and limitations that may arise. These factors can significantly influence the decision to use ordered maps in various applications. By acknowledging these obstacles, developers and computer science professionals can better assess when and how to incorporate ordered maps in their projects.
Overhead in Memory Usage
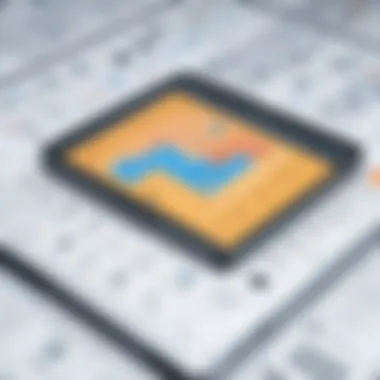
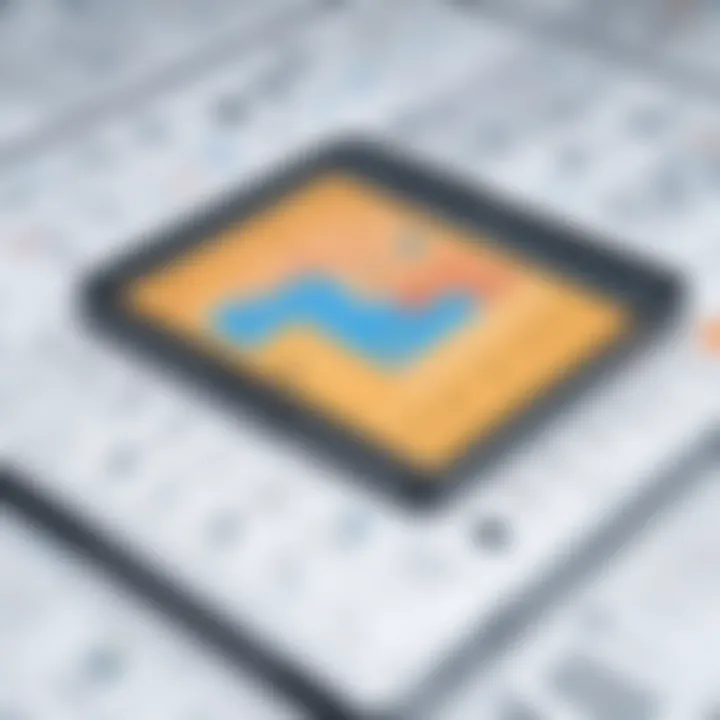
One of the primary concerns with ordered maps is their memory consumption. Unlike some simpler data structures, ordered maps maintain the order of their elements, which requires more space to store additional information.
For example, when storing key-value pairs, the map not only needs to retain the keys and values but also metadata regarding the order. This added layer of complexity can lead to inefficiencies in scenarios with limited memory resources.
Developers must consider the memory footprint of ordered maps compared to other data structures like hash tables or simple arrays. In instances where performance is critical, such as embedded systems or applications with strict memory constraints, this overhead can become an important factor.
Complexity in Implementation
The implementation of ordered maps can introduce a level of complexity that is not present in simpler data structures. For instance, various algorithms can be utilized to maintain order—these include balanced trees or linked lists. Each method carries its own set of trade-offs.
Choosing the right implementation requires a thorough understanding of how each affects both time and space complexity. Moreover, specific algorithms can introduce bugs if not implemented correctly. Developers may need to handle edge cases carefully, such as rebalancing operations or managing duplicate keys.
In general, while ordered maps offer significant advantages in terms of data organization and retrieval, the barriers related to overhead memory usage and implementation complexity must be carefully considered. This balance is essential for creating efficient, high-performing applications.
Future Trends in Ordered Maps
The exploration of ordered maps is crucial as we move towards a more data-centric technology landscape. In this digital age, where the manipulation and management of data dictate success, ordered maps stand out for their ability to maintain key-value pairs in a structured way. This section will delve into the anticipated advancements and their implications, particularly focusing on their evolving role in software development.
Advancements in Data Structures
Recent years have witnessed a significant evolution in data structures, especially ordered maps. Many languages are introducing built-in support for these structures, leading to simplified coding practices. Languages like Python, C++, and Java have enhanced their libraries to include ordered maps. This makes them more accessible to developers.
Enhancements in data structures are not only about accessibility. There is an emphasis on increasing performance efficiency. New algorithms are being developed to optimize insertion, deletion, and iteration processes in ordered maps. For instance, skip lists and balanced trees are being integrated to reduce the operational time complexity.
Moreover, the concept of persistent data structures is gaining traction. This allows ordered maps to retain previous states without being entirely duplicated. Such advancements empower developers to track changes over time more effectively, facilitating debugging and version control.
Potential Impact on Programming Paradigms
The shifts in ordered maps can have profound effects on programming paradigms. With ordered maps becoming more prevalent, their influence on functional programming can be observed. Immutable ordered maps allow for safer state management which is essential in concurrent programming scenarios.
As a result, developers may adopt more functional approaches, reducing side effects and making code easier to reason about. Data processing frameworks, like Apache Spark, leverage ordered maps to boost performance in distributed computing.
Another aspect is the impact on reactive programming paradigms. With ordered maps efficiently managing data flows, they streamline real-time data processing applications. The ability to maintain order can enhance responsiveness in applications that require immediate updates based on user interactions.
"The evolution of ordered maps will redirect how we integrate data management techniques in our programming paradigms."
In summary, the transformation and future direction of ordered maps portray a promising future. The ongoing advancements will continue to redefine how developers interact with data. There is a clear trend toward enhancing both performance and usability, which bodes well for software development and engineering.
Epilogue
In this article, we have explored the multifaceted topic of ordered maps, examining their key features, distinct applications, and implications for programming practices. The conclusion serves as a crucial element, encapsulating the essence of what ordered maps bring to the software development landscape.
The importance of ordered maps lies in their unique ability to maintain the order of key-value pairs, which can significantly enhance data handling efficiency. This feature differentiates ordered maps from unordered maps, making them a vital tool for programmers when the sequence of data is of utmost importance. Additionally, their straightforward implementation in various programming languages like C++, Java, and Python increases their accessibility to developers at all levels.
It is also important to consider performance aspects. While ordered maps provide organizational advantages, they might come with increased memory overhead compared to traditional unordered data structures. This trade-off between memory usage and the ease of data retrieval must be understood well by developers to make informed decisions on the appropriate data structure for their specific use case.
Key benefits of ordered maps include:
- Preserved order during data processing
- Enhanced ease of iteration
- Better suitability for specific applications, such as caching and data analytics
In summary, ordered maps offer significant advantages while also posing certain challenges. Their role in future programming paradigms is likely to grow as data becomes more prevalent and structured in nature. Understanding the nuances of ordered maps will aid programmers in effectively leveraging this important data structure in their projects.
Summary of Key Points
- Definition and Features: Ordered maps maintain the order of entries, enhancing the data retrieval process.
- Comparative Advantages: They are superior to unordered maps when the order of elements matters.
- Practical Implementations: Standard libraries and custom-built solutions provide various ways to utilize ordered maps.
- Performance Considerations: The trade-off between memory usage and performance is noteworthy.
- Diverse Applications: Their use spans data processing, caching mechanisms, and web development tools.
Final Thoughts on Ordered Maps
Ordered maps are essential in a variety of programming contexts. As computer science evolves, the demand for structures that efficiently manage ordered data will increase. Embracing the challenges they present while recognizing their benefits can lead to improved software design and innovation. Ordered maps are more than mere storage solutions; they facilitate strategic data manipulation, making them indispensable to contemporary programming practices. Aspiring and experienced programmers alike should prioritize understanding these structures to enhance their competency in data management.