Exploring Rails Software: A Comprehensive Overview
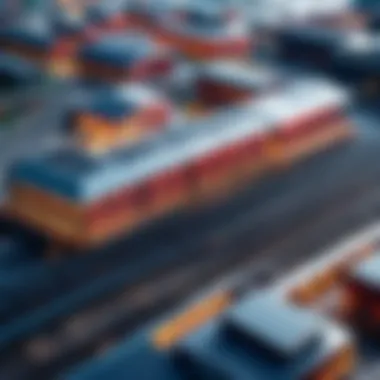
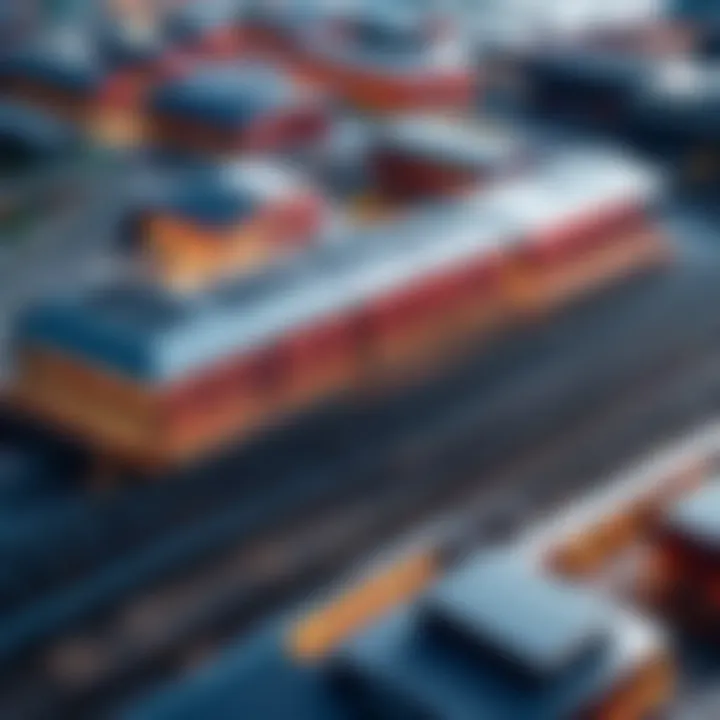
Intro
Rails software, or Ruby on Rails, is a powerful web development framework that simplifies the process of creating applications. It embodies a set of conventions and principles that aim to streamline and expedite the development cycle. Understanding Rails involves examining its architecture, features, and evolving role in the modern technology landscape.
In this article, we will explore several key aspects of Rails, from its foundational elements to more advanced topics like best practices and challenges faced by developers. This journey is designed to inform both seasoned programmers and newcomers to make the most of the framework. By dissecting its core principles and features, we aim to highlight Rails' significance and utility in today's digital world.
In the sections that follow, we will delve into coding challenges, technology trends, coding resources, and essential computer science concepts as they pertain to Ruby on Rails. Each of these areas will not only reinforce understanding of the framework but also enhance our grasp of the broader web development ecosystem.
Preface to Rails Software
The significance of Rails software cannot be overstated in the realm of web development. As a framework built on the Ruby programming language, Ruby on Rails provides a robust structure for developers to create dynamic websites and applications efficiently. The nature of its design allows programmers to focus more on functionality rather than intricacies of configuration, vastly streamlining the development process.
Definition and Overview
Rails, often abbreviated as RoR, is an open-source web application framework. It is designed to facilitate the creation of database-backed web applications. At its core, Rails adopts a Model-View-Controller (MVC) architecture, which not only separates concerns but also promotes organized and maintainable code. This framework emphasizes convention over configuration, meaning that developers can follow established patterns to reduce the amount of code they must write. The core principles of Rails include the DRY (Don't Repeat Yourself) philosophy, which encourages reducing repetition of code.
The technology stack includes features such as Active Record, an object-relational mapping system that connects the database with the Ruby objects. This connection allows for seamless data management, making it a preferred choice for developers looking to build applications quickly and efficiently.
Historical Context
Ruby on Rails was created in the early 2000s, with the initial release occurring in 2004. It was developed by David Heinemeier Hansson while he was working on a project at Basecamp, a web-based project management tool. Ruby on Rails quickly gained popularity due to its developer-friendly features and an engaging community that thrived on sharing knowledge and best practices. Its rise coincided with the burgeoning growth of web applications, paving the way for many startups and companies to adopt this framework.
In the years following its launch, Rails has undergone numerous updates and improvements, keeping pace with modern software practices and advances in computing. The introduction of gems—packages that add functionality—has expanded its ecosystem significantly, making it an attractive option for both new and experienced developers. Today, Rails remains a prominent choice in the technology landscape, powering a vast array of applications from small businesses to large enterprises.
Understanding Ruby on Rails Architecture
Understanding the architecture of Ruby on Rails is essential for grasping how this framework operates effectively in building web applications. The architecture serves as a foundation upon which developers can create complex, interactive web environments. Essentially, Rails employs a Model-View-Controller (MVC) architecture, which separates concerns, allowing for a cleaner codebase and improved maintenance. This separation significantly benefits developers, as it simplifies debugging and enhances the testing process.
The benefits of a well-structured architecture cannot be overstated. It promotes a logical flow of data and helps in organizing project files intuitively. Moreover, understanding how the various components of Ruby on Rails interact empowers developers to utilize the framework to its fullest potential, leading to more efficient and scalable applications.
Model-View-Controller Framework
The Model-View-Controller framework is a fundamental aspect of Ruby on Rails and is key to its design philosophy. In this architecture, the Model represents the application's data and business logic, while the View is responsible for the user interface and presentation of data. The Controller acts as the intermediary, processing user input, interacting with the Model, and sending data to the View.
This triadic structure brings notable advantages:
- Separation of Concerns: Each component focuses on its particular task, ensuring that data handling, business logic, and presentation do not interfere with one another. This leads to greater clarity in the codebase.
- Modular Development: Developers can work on individual components without impacting others. This modularity fosters collaboration in larger teams, as different parts of the application can be developed simultaneously.
- Easier Testing: Isolated components make it simpler to write unit tests for each part of the application, enhancing code reliability and reducing bugs.
To illustrate how this framework functions, consider this simple example:
In this snippet, the retrieves a specific post from the Model layer. This data can then be rendered in the View layer to be presented to the user.
Routing in Rails
Routing is another critical aspect of Rails architecture. It is the mechanism by which HTTP requests are directed to the appropriate controller actions. Understanding routing is vital for efficient application design as it determines how users navigate through the application.
Rails employs a RESTful routing pattern, which allows developers to map HTTP actions to CRUD operations seamlessly. The conventions in Rails promote sensible defaults, minimizing the need for extensive routing configurations.
In Rails, routing configurations reside in the file. Here are some points to consider about routing in Rails:
- Simplicity: The syntax for defining routes is straightforward, enabling quick setups. For example:
This creates all standard routes for the resource automatically.
- RESTful Design: By following RESTful principles, not only do developers create predictable URLs, but they also adhere to modern web design standards, enhancing usability.
- Nested Resources: Rails enables the definition of nested resources, allowing related data to be grouped logically. This feature is especially useful in cases where resources have a parent-child relationship, which can improve database query efficiency.
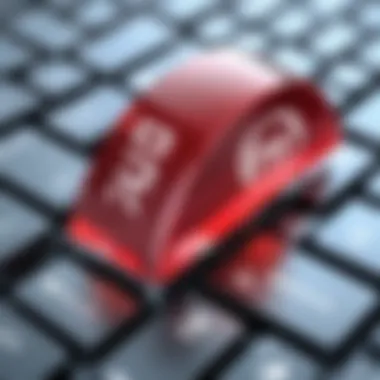
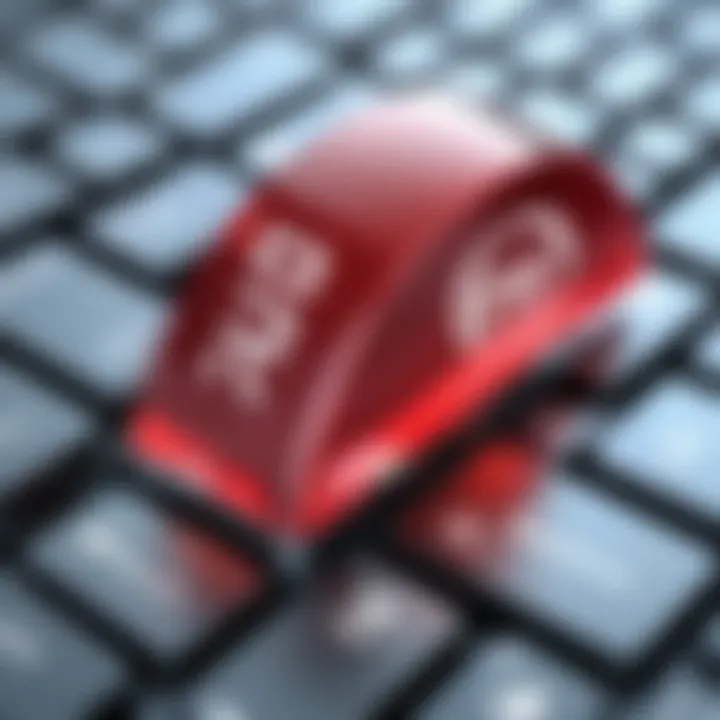
In sum, routing plays a vital role in the Rails ecosystem, helping maintain a straightforward and organized structure that aligns closely with MVC architecture. A deep understanding of both MVC and the routing system is indispensable for any developer looking to harness the full power of Ruby on Rails.
Key Features of Rails Software
Understanding the key features of Rails software is crucial, as these elements define its usability and appeal. The framework has been designed with certain principles that simplify the development process and make it more efficient. Developers should be aware of these traits when considering Rails for their projects, whether they are seasoned programmers or newcomers striving to broaden their skills. Here, we will examine three fundamental aspects of Rails: Convention over Configuration, the DRY Principle, and Active Record ORM.
Convention over Configuration
The concept of Convention over Configuration is a cornerstone of Rails software. This principle dictates that, whenever possible, developers should rely on conventions rather than extensive configuration files. By doing this, Rails reduces the number of decisions a developer must make. It speeds up the development process.
For instance, if a developer follows standard Rails naming conventions for models and controllers, the framework automatically knows how to handle them without requiring detailed configurations. This clarity enhances productivity because developers spend less time setting up and more time coding the actual application logic.
"Convention over configuration aims to decrease the mental overhead in programming."
Here are some key benefits of this principle:
- Simplicity: The framework allows for rapid application development by minimizing the need for verbose configuration.
- Consistency: Following conventions means the codebase remains uniform, making it easier for teams to collaborate.
- Focus on Development: Developers can concentrate on writing application logic instead of worrying about settings and configurations.
DRY Principle
Another critical feature is the DRY Principle, which stands for "Don't Repeat Yourself." This principle encourages developers to reduce repetition within their code. Excessive duplication can lead to increased maintenance workload, errors, and inconsistencies. By adhering to the DRY principle, Rails promotes cleaner code and improved maintainability.
In practical terms, if a certain functionality is needed in multiple parts of the application, developers should abstract it into a single method or service class rather than copying it multiple times. This makes it easier to manage changes and updates since adjustments only need to be made in one location.
The DRY principle leads to several advantages:
- Reduced Errors: Fewer copies of the same code mean fewer opportunities for bugs.
- Easier Modifications: Updates can be made in one place, simplifying the maintenance process.
- Enhanced Readability: The code stays cleaner and easier for other developers to understand.
Active Record ORM
Finally, the Active Record ORM is a powerful feature of Rails. It abstracts the database interaction and allows developers to interact with the database using high-level objects rather than writing tedious SQL commands. This enables a more intuitive and object-oriented approach to dealing with data.
With Active Record, every model class corresponds to a database table, and instances of these classes correlate to rows in the table. This structure helps in maintaining a clear relationship between the objects in the application and the data stored in the database.
Key aspects of Active Record ORM include:
- Simplified Database Operations: Developers can create, read, update, and delete records using methods instead of manual SQL queries.
- Built-in Validations: Active Record comes with built-in mechanisms for data validation, enhancing data integrity.
- Associations: The ability to establish relationships between models with ease allows for more organized data structures.
Advantages of Using Rails Software
Rails software has garnered attention in the sphere of web development for its distinct advantages. These benefits make it an attractive choice for both seasoned developers and newcomers alike. Its elegant design principles, combined with practical features, streamline the development process, reduce redundancies, and facilitate effective project management.
Rapid Development
One of the most touted advantages of Rails software is its ability to enable rapid development. This allows developers to create applications in a fraction of the time compared to other frameworks. The philosophy of "Convention over Configuration" permits developers to focus on writing code rather than spending extensive time on configurations. This simplicity can considerably speed up the development cycle. For example, integrating various functions like databases and services can be done with minimal setup, granting developers more time to concentrate on crafting unique features.
Rails' extensive library of gems also complements this rapid development advantage. Gems are pre-packaged libraries that extend the functionality of Rails. Using gems, developers can add complex features like authentication, file uploads, or payment processing quickly and without deep programming knowledge. This access to a rich ecosystem allows teams to quickly prototype and iterate on their applications.
Scalability and Performance
While speed is essential in development, scalability and performance are critical in production. Rails software has evolved to accommodate growing applications. With background processing libraries like Sidekiq and tools such as Puma for managing server load, developers can effectively handle an increase in user activity without sacrificing application performance.
Moreover, using tools like Redis for caching helps to reduce server load and enhances response times. These optimizations contribute to a more scalable architecture, allowing applications to grow seamlessly. Choosing the right plugins and configurations can further enhance performance, ensuring that applications built with Rails can service a larger user base efficiently.
Community Support
Another significant advantage of Rails software is the solid community support. The Rails community comprises a diverse group of developers, contributors, and enthusiasts who share knowledge and resources. This collaborative environment fosters innovation and continuous improvement within the framework. Resources such as forums, GitHub repositories, and blogs are readily available for anyone seeking guidance.
"The community's commitment to sharing knowledge leads to a richer understanding of best practices and project development."
Aspiring developers can benefit from various tutorials, walkthroughs, and third-party resources that simplify learning. Established developers often share their insights on platforms like Reddit and other forums, which helps create a bustling environment of shared expertise. This wealth of information can make it easier to troubleshoot issues and find solutions efficiently.
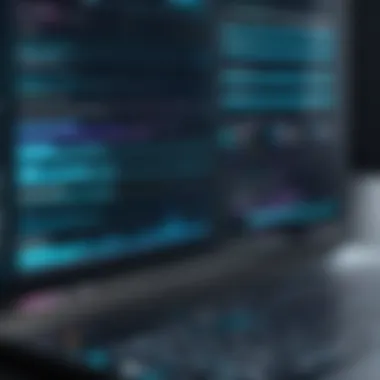
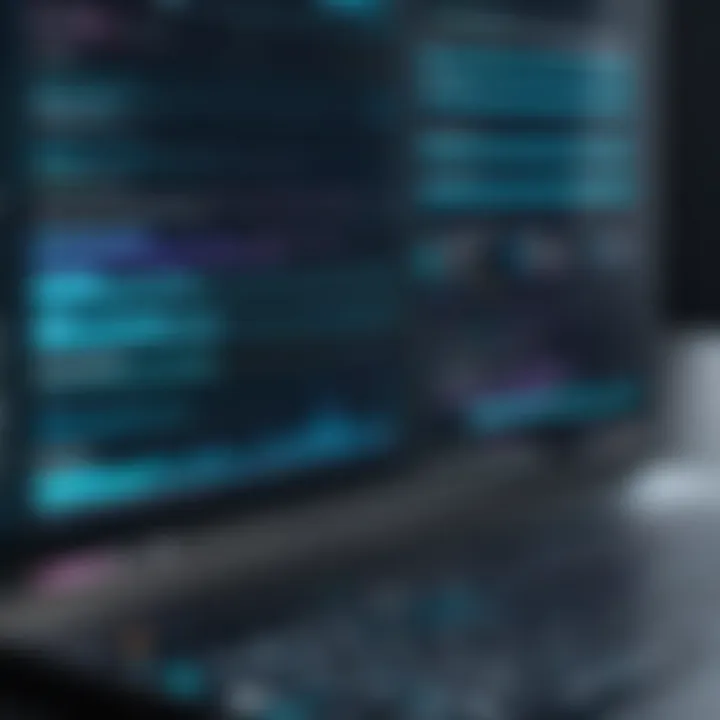
Best Practices for Implementing Rails Software
Implementing Ruby on Rails software effectively requires an adherence to best practices that enhance the development process. These practices not only streamline workflow but also assure maintainability and scalability of applications. Successful Rails projects are often distinguished by a commitment to industry standards, rigorous testing, and an efficient collaboration approach, which collectively contribute to superior software quality and user satisfaction.
Adherence to Standards
Following established standards in Rails development is critical. It ensures that the code remains consistent, readable, and easy to maintain. One of the primary benefits of this practice is that it facilitates onboarding new developers. When they encounter well-structured code, it is easier for them to understand the architecture and logic. Common standards in Rails include:
- RESTful design: Structure routes according to REST principles to promote a natural workflow for web applications. This makes APIs more intuitive.
- Use of established gems: Leverage popular gems, such as Devise for authentication and Pundit for authorization, instead of reinventing the wheel. This enhances security and saves time.
- Consistent naming conventions: Following Rails conventions for naming controllers, models, and views increases clarity and reduces confusion.
- Documentation: Regularly documenting code and using comments where necessary will help guide other developers and stakeholders in understanding decisions made.
By implementing these practices, developers not only improve collaboration but also increase the overall robustness of their projects.
Testing Strategies
In the context of Rails software, testing cannot be overlooked. An effective testing strategy is integral for identifying bugs, ensuring code quality, and simplifying future changes. Well-defined test cases encompass unit tests, integration tests, and acceptance tests, providing full coverage of the application functionality. The following strategies can improve testing efforts:
- TDD (Test-Driven Development): This approach emphasizes writing tests before writing the actual code. By focusing on the tests, developers can ensure that their code is robust and meets the required specifications from the outset.
- Utilizing RSpec and Capybara: RSpec is a prevalent testing framework for Ruby, allowing for expressive and human-readable tests. Capybara helps in simulating user interactions, making acceptance tests straightforward and reliable.
- Automated testing: Automate tests to run frequently, especially in continuous integration/continuous deployment (CI/CD) environments. This assures that new code does not break existing functionality.
- Code coverage tools: Using tools like SimpleCov helps to visualize which parts of the code are exercised by tests, enabling developers to achieve comprehensive coverage.
By systematically implementing these testing strategies, developers can maintain a high-quality standard in their Rails applications, minimizing the risk of defects and enhancing overall performance.
"Effective testing strategies not only catch problems early but also boost developer confidence in the codebase."
In summary, committing to best practices such as adherence to standards and strategic testing can significantly affect the success of a Rails project. These principles lead to better quality software and a smoother development process.
Challenges in Rails Development
Rails development, while powerful and efficient, does have its challenges. Understanding these difficulties is crucial for developers aiming to maximize their effectiveness when working with this framework. By recognizing potential issues, they can devise strategies to mitigate them. This section focuses on two main challenges: performance bottlenecks and security concerns. Addressing these is essential not only for achieving robust application performance but also for maintaining user trust and data integrity.
Performance Bottlenecks
Performance bottlenecks are one of the most pressing challenges that developers face when using Rails. These bottlenecks often stem from inefficient database queries, bloated application logic, or improper asset management. When a Rails application struggles with speed, users experience slow load times, leading to frustration and decreased user satisfaction.
Some common areas where performance issues arise include:
- Database query optimization: Rails uses Active Record, which can sometimes generate complex queries that may not be optimal. Understanding how to efficiently use joins and indexes is key.
- Asset pipeline management: Rails' asset pipeline can sometimes lead to unnecessarily large file sizes or unoptimized assets if not handled properly. This can slow down page load times significantly.
- N+1 query problems: This occurs when an application makes separate database calls for related data instead of a single query. Developers should be vigilant about eager loading to avoid this situation.
By recognizing these issues early in the development process, teams can implement performance monitoring tools, conduct regular code reviews, and refactor code when necessary. Effective performance management leads to a smoother user experience and enhances overall application responsiveness.
Security Concerns
Security concerns also pose significant challenges in Rails development. Given the highly interconnected nature of web applications, vulnerabilities can expose sensitive data and jeopardize both user trust and regulatory compliance. Common security challenges in Rails include:
- Cross-Site Scripting (XSS): Poor input validation can lead to XSS attacks, where attackers inject scripts into a web page viewed by unsuspecting users.
- SQL Injection: Rails is generally safe from SQL injection when using Active Record. However, developers must be cautious while executing raw SQL queries to avoid data breaches.
- CSRF (Cross-Site Request Forgery): Rails provides built-in mechanisms to prevent CSRF attacks, but developers need to understand and utilize these tools effectively.
To enhance security, developers must adhere to best practices such as employing strong parameter filtering, encrypting sensitive data, and regularly updating dependencies to patch vulnerabilities. Continuous security training and awareness are also vital for development teams.
In today’s digital environment, security is not just an afterthought. It needs to be integrated into every aspect of Rails development.
By confronting these challenges directly, developers can build robust, secure applications that perform well under pressure. This attention to both performance and security is essential for crafting high-quality software in the Rails ecosystem.
Comparing Rails with Other Frameworks
Comparing Ruby on Rails with other web frameworks is crucial for understanding its advantages and limitations. Each framework has unique characteristics, strengths, and weaknesses. This analysis helps developers choose the appropriate tool for their projects. By examining Rails alongside other frameworks, especially Django and Flask, readers can grasp the nuances of Rails in different contexts and applications.
Rails vs. Django
Ruby on Rails and Django are among the most popular frameworks for web development. Both offer rapid development capabilities but have different philosophies and structures. Rails follows the convention over configuration principle, allowing developers to build applications quickly by adhering to common practices. In contrast, Django emphasizes explicit configurations and comes with a powerful admin interface out of the box.
One of the notable differences lies in their programming languages. Rails is built on Ruby, known for its expressive syntax and dynamic capabilities. Django, on the other hand, uses Python, which is celebrated for its readability and simplicity. Developers familiar with Python often find Django more approachable than Rails.
Performance-wise, Django may be more efficient with complex queries and larger datasets due to its ORM capabilities and caching methods. Rails, however, has made significant improvements in performance with recent versions, employing advanced query optimization techniques.
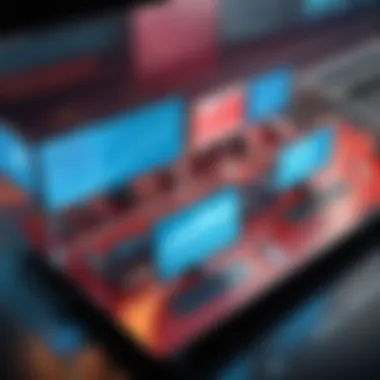
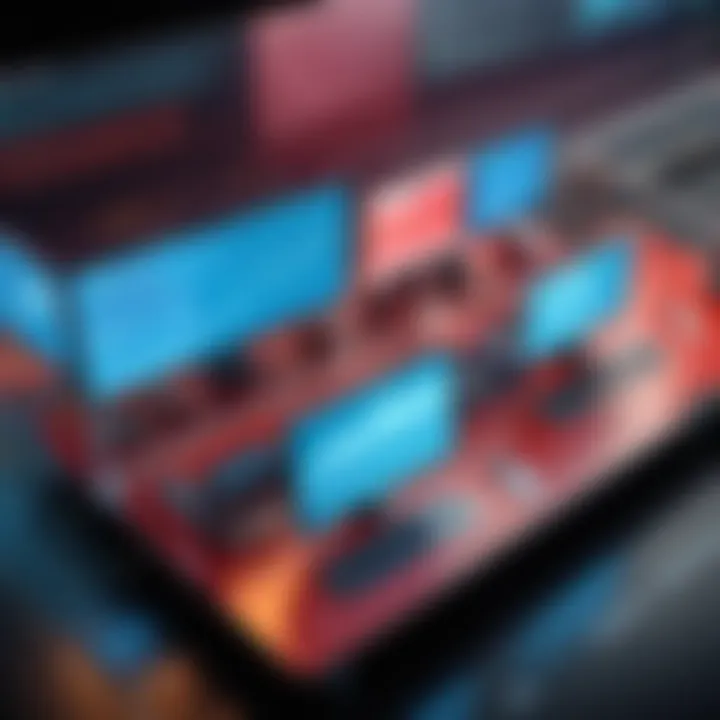
When it comes to community support, both frameworks boast vibrant communities. The Rails community is known for gems, which are reusable libraries that enhance functionality. Django also has an extensive library of packages, ensuring that any feature can be integrated into the application with relative ease.
In summary:
- Language: Ruby (Rails) vs Python (Django)
- Philosophy: Convention over configuration (Rails) vs Explicit configuration (Django)
- Performance: Both frameworks have strengths; Rails is improving steadily.
- Community Support: Both have strong ecosystems with many useful libraries.
Rails vs. Flask
Flask is another framework that often comes up in discussions about alternatives to Rails. Unlike Rails and Django, Flask is a micro-framework. This means it is lightweight and flexible, giving developers the freedom to choose how to structure their applications. Developers who prefer more control over their application architecture might find Flask appealing.
Ruby on Rails provides a more robust structure from the start, integrating numerous features into its core. This can lead to faster development times for both small and large projects, as developers can leverage Rails' built-in solutions for common tasks. However, this can sometimes be a double-edged sword, as the complexity of Rails can lead to longer onboarding times for new developers.
Flask allows the integration of various components as needed, leading to a tailored solution for unique project requirements. Because of this, it can be ideal for smaller applications or prototypes where scalability is not an immediate concern. However, scaling a Flask application often requires additional decisions around module selection and integration that are handled more straightforwardly in Rails.
Important points in comparing Flask and Rails:
- Framework Type: Full-stack (Rails) vs Micro-framework (Flask)
- Flexibility: Flask offers more customization; Rails provides out-of-the-box solutions.
- Development Speed: Rails typically allows for faster development in larger applications.
- Use Case: Flask is great for simple applications, while Rails suits complex systems.
In all, choosing between Rails, Django, and Flask depends on the specific nature of the project and the preferences of the development team. Each framework has distinct characteristics that make them suitable for different situations.
Emerging Trends in Rails Software
In a rapidly evolving technology landscape, staying updated with emerging trends in Rails software is crucial. These trends reflect how developers adapt and optimize their applications in response to changing user needs and technological advancements. The integration of microservices and the increasing adoption of GraphQL are two pivotal elements that illustrate Rails' adaptability and growth potential.
Integration with Microservices
The concept of microservices denotes an architectural style that structures an application as a collection of loosely coupled services. This allows each service to be developed, deployed, and scaled independently. Rails developers are increasingly embracing this architecture to enhance flexibility and scalability.
Integrating microservices with Rails offers several benefits:
- Scalability: By allowing independent scaling of services, applications can efficiently manage varying loads, optimizing resource use.
- Isolation: If one service encounters an issue, it does not necessarily disrupt the entire application, enhancing overall system reliability.
- Technology Diversity: Developers can use different programming languages or databases tailored for each service, driving innovation.
However, this approach poses challenges too:
- Complexity in Management: A distributed system requires thorough monitoring and management solutions, which can add operational overhead.
- Inter-service Communication: Efficient communication between services is critical. Options include REST APIs or message brokers, each with its own trade-offs.
Adoption of GraphQL
GraphQL is a query language for APIs and a runtime for executing those queries with existing data. Its adoption within Rails software presents a game-changing capability for data retrieval and manipulation. Unlike traditional RESTful APIs, GraphQL allows clients to specify exactly what data they need.
The advantages of integrating GraphQL into Rails applications include:
- Efficiency in Data Retrieval: Clients can fetch only the information required, reducing over-fetching and under-fetching issues common with REST APIs.
- Single Endpoint: GraphQL enables the use of a single endpoint for multiple queries and mutations, simplifying API management.
- Strong Typing: Developers define the data types a GraphQL API can return, enhancing clarity and reducing runtime errors.
It is essential to consider some challenges that accompany GraphQL adoption:
- Learning Curve: Developers need to familiarize themselves with its concepts, which might slow initial development.
- Caching Complexity: Unlike REST, which caches responses based on URL, caching in GraphQL can be more complex.
In summary, integrating microservices and adopting GraphQL represent significant steps forward for Rails software. These trends not only enhance the performance and scalability of applications but also align with contemporary demands for agility and user-oriented design.
Ending and Future Outlook for Rails
The conclusion of this article synthesizes the insights gathered throughout our exploration of Rails software. This section highlights the significance of acknowledging the framework's current state and potential future developments. With consistently high demand for web applications and the agility that Rails provides, understanding the trajectory of this software is vital for developers, businesses, and tech enthusiasts.
Summary of Key Points
In summarizing the key points, we reflect on several critical aspects of Rails:
- Architecture: Rails’ robust architecture allows for organized code, promoting maintainability and ease of updates.
- Features: The framework's unique characteristics, such as Convention over Configuration and the DRY principle, facilitate rapid development.
- Community Support: A vast, dedicated community provides extensive resources, including gems and plugins, enhancing development capabilities.
- Challenges: Certain limitations, like performance bottlenecks and security issues, must be addressed by developers to optimize their applications.
- Future Trends: Emerging trends such as microservices integration and the adoption of GraphQL indicate how Rails is adapting to modern demands.
Predictions for Development
Looking ahead, several predictions for development in Rails emerge:
- Increased Adaptability: As the tech landscape shifts towards microservices, Rails will likely evolve to facilitate seamless integration with these architectures. This could enhance its appeal for larger applications requiring modularity.
- Focus on Performance Enhancements: Developers will continue to prioritize performance optimization, leading to innovations within the framework that address current limitations.
- Greater Emphasis on Security: As cyber threats become more sophisticated, we can expect Rails to incorporate stronger security measures to protect web applications.
- Broader Usage of GraphQL: The increasing adoption of GraphQL may reshape how Rails interacts with data, making it more efficient for API-driven applications.
- Community Growth: With a growing number of developers engaging with Rails, the community will likely produce more resources and tools that enrich the development environment further.
Overall, the future of Rails appears promising as it continues to adapt to changing technological demands while maintaining its core strengths. Developers can expect an evolving ecosystem that supports innovative solutions in web development.