Exploring the Depths of React Tree Table Functionality
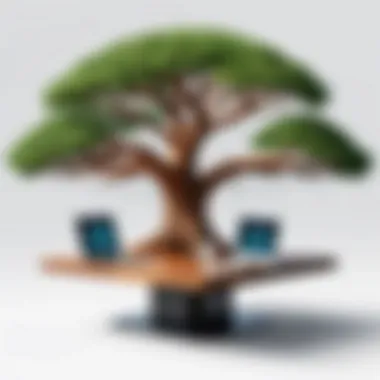
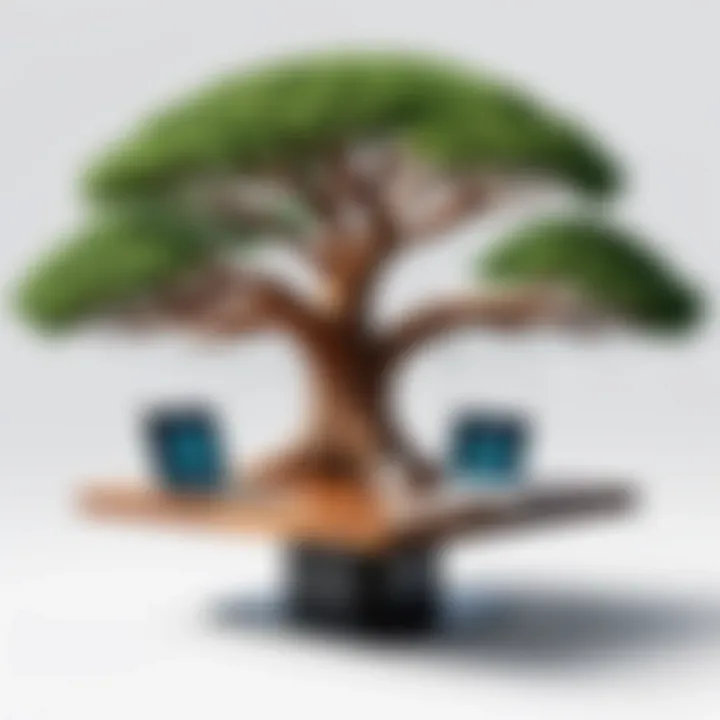
Intro
React Tree Tables represent an innovative solution for displaying hierarchical data in a structured and visually coherent manner. This article offers a deep dive into their architecture, implementation, and functionality. By understanding these elements, developers can leverage React Tree Tables to improve user experience and data management in their applications.
The significance of React Tree Tables lies in their ability to present complex relationships between data points rather than just linear lists. This capability allows users to gain insights more quickly and effectively. Moreover, a well-implemented tree table can enhance interactivity by allowing users to expand or collapse data nodes, streamlining navigation through large datasets.
In the following sections, we will explore various aspects of React Tree Tables, covering everything from architecture and implementation techniques to performance optimization and state management. This comprehensive approach provides readers with both theoretical frameworks and practical guidance, suited for developers at all levels.
Coding Challenges
Engaging with coding challenges can sharpen a developer's skills in implementing React Tree Tables. While creating tree tables seems straightforward, developers may encounter several challenges, ranging from handling state effectively to managing dynamic data.
Weekly Coding Challenges
Regular coding challenges focused on React Tree Tables can foster growth and understanding. Examples include creating a dynamic tree table from an API, optimizing rendering processes, or implementing unique sorting algorithms for nested data.
Problem Solutions and Explanations
For programmers facing difficulties, it is essential to dissect problems logically. This includes understanding the data structure used and ensuring efficient state management. Troubles can often arise when attempting to visualize large datasets or when trying to manage nested components within React. By breaking down these challenges, solutions can be built systematically.
Tips and Strategies for Coding Challenges
Efficient coding requires a mixture of knowledge and strategy. Here are some helpful tips:
- Understand the core principles of React.
- Familiarize yourself with React's state management tools, like Redux.
- Investigate existing libraries suited for tree tables, such as react-table or react-virtualized, which can significantly cut down development time.
- Utilize comprehensive testing to validate behavior across various data structures.
Community Participation Highlights
Joining developer communities is crucial. Websites like Reddit have threads where developers showcase their tree table implementations or pose questions about their challenges. Engaging in these forums can provide insights and innovative solutions.
Implementation Techniques
When implementing React Tree Tables, understanding their foundational components is vital. Developers must grasp how to design tree structures effectively and render them properly within React. Building an efficient Tree Table involves two main components: data architecture and user interface design.
Data Structure Design
A well-structured data format acts as the backbone of any tree table. JSON format is frequently used due to its simplicity and versatility. It is crucial to use objects that contain arrays, allowing for a clear parent-child relationship, enabling seamless data traversal.
User Interface Components
React allows for highly customizable UI components. Libraries like Material-UI or Ant Design provide ready-made components that can be adjusted for tree table functionality. Additionally, developers must pay attention to accessibility standards to ensure a broad user base can interact with the applications.
Performance Optimization
Performance optimization is a key concern when developing applications. Slow loading times and unresponsive interfaces diminish user experience. To enhance performance in React Tree Tables:
- Adopt lazy loading techniques to reduce initial loading times.
- Implement memoization to avoid unnecessary re-renders.
- Explore virtualization techniques to render only visible elements in a long list, efficiently managing memory usage.
Accessibility Best Practices
Accessibility should not be an afterthought. Ensuring that tree tables are navigable by all users enhances inclusivity. Adapting keyboard navigation and implementing ARIA roles augments user experience for those who rely on assistive technologies.
An accessible tree table not only helps people with disabilities but also improves overall usability.
Closure
Foreword to React Tree Table
React Tree Table is a specialized version of traditional data tables that provides a hierarchical structure for data presentation. This article focuses on why React Tree Tables are important in modern web applications and how they enhance user experience when handling complex datasets. The introduction of this subject sets the stage for understanding not only the architectural framework but also practical aspects of implementation and functionality.
Having a clear grasp of React Tree Tables is crucial for developers aiming to build intuitive user interfaces. They allow developers to display structured data more efficiently, especially for categories that require nested or hierarchical organization. This type of presentation improves accessibility and usability, boosting overall user satisfaction.
Definition and Purpose
A React Tree Table is essentially a combination of a tree view and a table, designed to manage and display data that has a parent-child relationship. It is particularly useful when dealing with datasets that include categories and subcategories – for example, filesystem hierarchies or organizational structures. The purpose of employing a React Tree Table lies in its ability to condense and manage large amounts of data in a way that maintains clarity and promotes ease of navigation.
The primary goal is to enable users to interact with the data more intuitively. By allowing expansion and collapse of nodes, users can focus on relevant information without visual clutter. As a result, developers need to consider how best to implement these features to optimize user interaction while ensuring that performance remains intact.
Significance in Data Presentation
The significance of React Tree Tables cannot be overstated, especially in fields where data is inherently hierarchical. This approach to data presentation provides numerous benefits:
- Efficient Data Organization: Tree Tables assist in logically organizing data, making it easier to understand complex relationships.
- Enhanced User Experience: The interactive aspects, such as expanding and contracting entries, allow users to customize their view, leading to improved satisfaction and engagement.
- Reduced Information Overload: By structuring data hierarchically, users are shielded from overwhelming amounts of information presented all at once.
- Improved Accessibility: React Tree Tables facilitate better screen reader compatibility and keyboard navigation, ensuring that information is accessible to all users.
"Presenting data effectively is not just about aesthetics; it's about clarity and usability. React Tree Tables bridge that gap."
In summary, the Introduction to React Tree Tables outlines their significance as effective tools for data representation. It positions developers to utilize a structure that not only displays data but also enhances user engagement and accessibility.
Fundamental Concepts
Understanding the fundamental concepts of React Tree Tables is essential for developers who aim to leverage them effectively. This section provides a detailed analysis of these foundational elements, focusing on their significance in creating dynamic and user-friendly data representations. The integration of tree structures within tables allows for a more organized presentation of hierarchical data. This methodology not only enhances visual clarity but also improves user navigation, ultimately leading to a better user experience.
Understanding Tree Structures
Tree structures are a crucial part of React Tree Tables. They represent data in a hierarchical format, allowing for parent-child relationships within the dataset. This organization is especially helpful when working with complex data sets that contain nested information. Here are several benefits of using tree structures:
- Logical Grouping: By organizing data hierarchically, users can understand relationships and dependencies between data points more clearly.
- Interactive Data Exploration: Tree structures allow users to expand and collapse nodes, making it easier to manage large datasets without overwhelming the user interface.
- Enhanced Data Retrieval: Developers can implement efficient search algorithms that utilize the tree format, enabling quicker data access and manipulation.
In React, the implementation of tree structures relies on state management and event handling to enable dynamic updates. This interactivity helps maintain data integrity while providing a responsive user interface.
Components of a Table
The components of a table in a React Tree Table are essential for its functionality and aesthetics. Each table element must work together seamlessly to present data effectively. Key components include:
- Header: The table header defines the columns and allows users to understand the type of data contained within each cell. Custom sorting and filtering options can also be integrated at this level.
- Rows and Cells: Each row represents a single entry in the dataset, while cells contain specific attributes of that entry. In tree tables, cells can hold unique content types such as text, icons, or even nested tables.
- Interactive Elements: Features like buttons for editing or deleting data, as well as controls for expanding or collapsing tree nodes, greatly enhance user engagement and functionality.
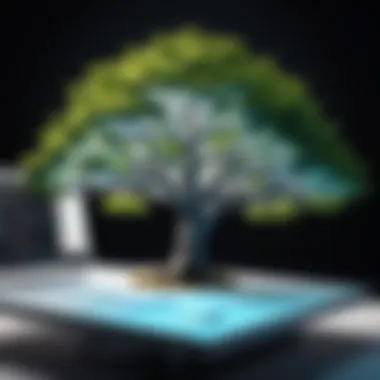
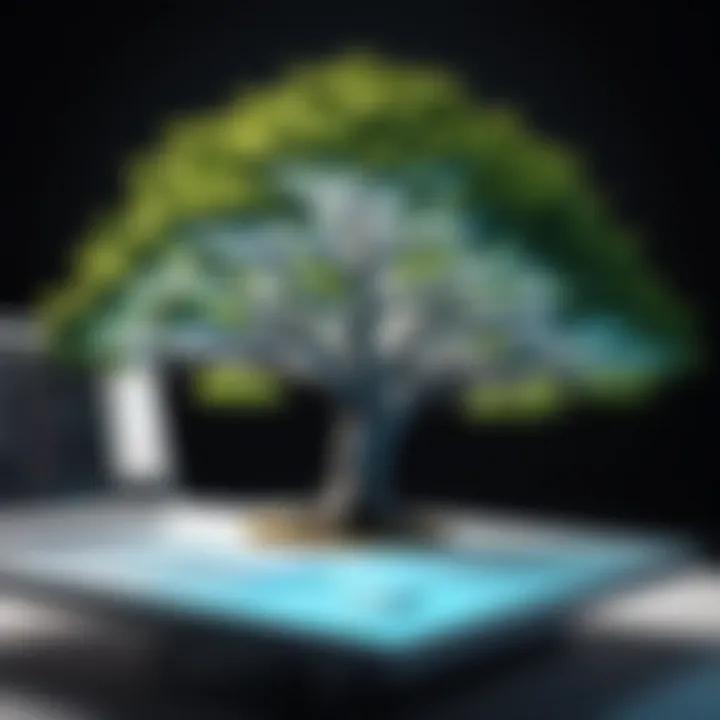
To illustrate how these components come together, consider the following simple structure in a React component:
Using this structure, developers can explore the combination of tree node representation with standard table components to create a versatile data presentation layer suitable for a wide variety of applications. Ultimately, understanding these components is vital for aspiring and experienced programmers looking to build sophisticated data visualizations in React.
Architectural Components
In the context of React Tree Tables, understanding architectural components plays a vital role in developing robust applications. These components dictate how the tree table structure is organized, how data flows through the application, and how users interact with the data displayed. The architecture of a React Tree Table determines functionality, usability, and performance, making it an essential focus for any developer tackling this challenge.
A well-defined architecture provides several benefits:
- Enhanced Maintainability: By constructing clear components, the codebase becomes easier to maintain and update. Each component can be tested and modified independently, reducing the risk of errors that affect the entire application.
- Reusability: Core components can be reused across different applications or sections within an application. This saves time and promotes consistency in design and functionality.
- Scalability: As data grows or requirements change, a solid architecture allows developers to scale the application without significant redevelopment.
The architectural components consist of various aspects, including the core components and the representation of tree nodes. Understanding these components requires a close examination of their roles within the application.
Core React Components
Core React components form the backbone of a React Tree Table. These components handle the main functions, including data retrieval, display, and user interaction.
- Table Component: This is the principal component that structures the overall table. It manages rows and columns, ensuring correct data representation.
- Row Component: Each tree node or data entry typically corresponds to a row in the table. The Row component is responsible for rendering data specific to that node. It can also handle events such as click actions to expand or collapse tree nodes.
- Header Component: This component defines the column headers, providing clarity on the type of data being presented. It can also be interactive, allowing sorting or filtering based on user action.
- Cell Component: Each piece of data within a row belongs to a cell. The Cell component can handle various data types, including images, text, and icons, ensuring a flexible presentation.
- Context Providers: Often in React applications, context providers are adopted for global state management. These serve to centralize data logic and provide state to all components without the need for deep prop drilling.
Tree Node Representation
Tree nodes represent data in a hierarchical structure, allowing for a more organized view of complex datasets. Each node can have multiple child nodes, creating a parent-child relationship. This representation is pivotal for displaying structured information, such as organizational charts or folder structures.
Key considerations when implementing tree node representation include:
- Identification: Each node should have a unique identifier. This ensures that operations such as expand, collapse, and selection can be performed accurately.
- State Management: Maintaining the state of each node, such as whether it is expanded or collapsed, is crucial for a smooth user experience. This can be achieved through local component state or global state management tools, like Context API or Redux.
- Data Loading: Depending on the dataset's size, loading data asynchronously can enhance performance. Implementing lazy loading for child nodes can prevent the initial load time from becoming overwhelming.
A tree node's visual representation needs to be intuitive. Users should easily navigate through the tree structure, understanding which nodes are expandable and which contain data. This clarity fosters a better user experience and engagement.
Understanding the core architectural components of React Tree Tables is essential for building efficient, user-friendly applications that handle complex datasets effectively.
Building a React Tree Table
Building a React Tree Table is essential for developers aiming to create rich and interactive user interfaces. A proper implementation provides clarity and structure, allowing users to navigate complex datasets effortlessly. This section will outline the critical components involved in constructing such a table, highlighting benefits and considerations.
Setting Up the Environment
Before diving into development, it is important to set up the correct environment. The foundation of any React project lies in the configuration of the necessary tools and libraries. Begin by ensuring that Node.js and npm (Node Package Manager) are installed on your system. This ensures smoother project management and dependency installation.
Once the tools are ready, create a new React application by using the command:
Installing required packages can further enhance functionality. Options include:
- react-table: Provides a set of hooks and methods for table manipulation.
- axios: An elegant way to fetch data from external APIs.
To install these dependencies, navigate to your project directory and enter:
By completing these steps, the environment will be set for building a robust React Tree Table.
Creating the Tree Table Component
The next stage involves crafting the core component. A React Tree Table component integrates both tree structures and tabular data representation. In this phase, focus on breaking down the component into manageable parts for clarity and efficiency.
Develop a functional component called . It will render the tree structure along with necessary properties. The following structure can be used:
Inside the component, set up a mechanism to handle the tree nodes, displaying them in a table format. Make sure the component receives data as props to dynamically populate content. The iterative rendering process is crucial. Consider using methods like to loop through the data array and generate rows for the table.
Implementing Data Fetching
Incorporating data fetching is a vital step. This can enhance the Tree Table by populating it with real-time data. Use Axios for retrieving data from an API endpoint. Import Axios at the start of the component with:
Then, leverage the hook to fetch data when the component mounts:
This effective fetching method ensures user interaction remains dynamic. Consider also implementing loading states and error handling to improve user experience.
"Enhancing user interaction requires thoughtful data management techniques and robust error handling."
In sum, Building a React Tree Table requires careful planning and execution. Each step, from setting up the environment to creating components and fetching data, contributes to the overall efficacy of the application.
User Interaction
User interaction is a critical component when creating effective web applications. In the context of React Tree Tables, it features prominently, enhancing the user experience through intuitive design. Effective user interaction can make complex datasets more manageable and accessible. Engaging with data through a tree structure allows users to organize information in a hierarchical manner, making it easier to understand relationships and navigate vast amounts of data.
Click and Expand Functionality
The click and expand functionality is central to React Tree Tables. This feature allows users to delve deeper into the data structure by expanding or collapsing nodes. When a user clicks on a tree node, the corresponding child nodes are displayed.
This capability is not just about visual appeal; it is practical, providing several benefits:
- Enhanced Navigation: Users can focus on specific parts of the data, reducing cognitive overload.
- Dynamic Interaction: Rather than presenting all data at once, this method engages users to interact dynamically.
- Efficiency: Users can quickly access information without scrolling through extensive lists.
To implement this functionality, developers can utilize state management to track the expanded or collapsed status of individual nodes. Here's a simplified example of how one might structure this feature using React:
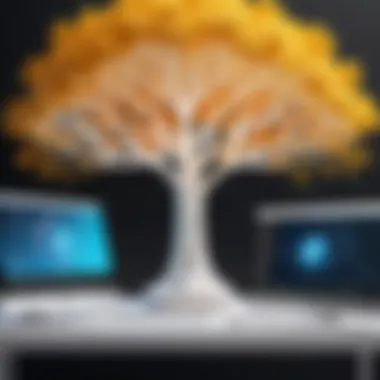
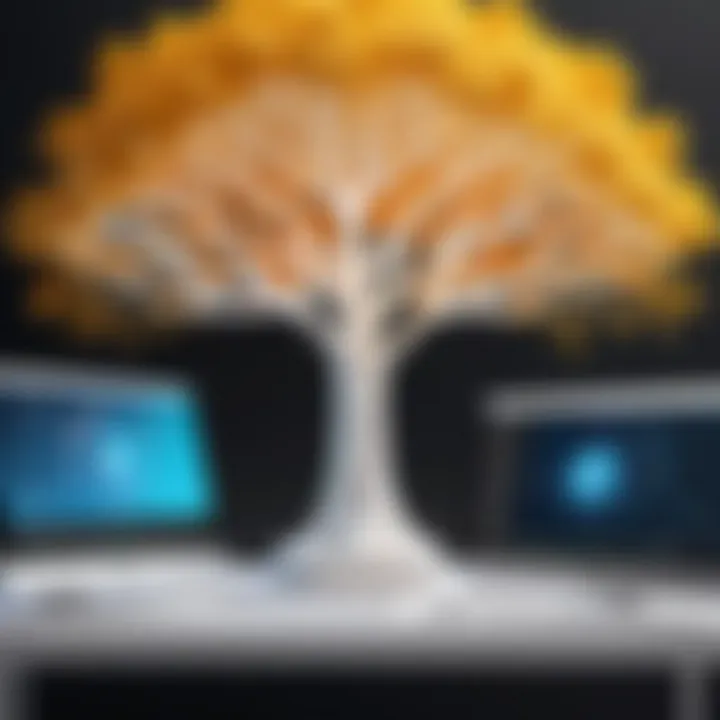
This straightforward implementation highlights user engagement by enabling a clean and organized way to navigate tree structures.
Keyboard Navigation and Accessibility
Accessibility is crucial in modern web development. Keyboard navigation ensures that all users, including those with disabilities, can interact with the React Tree Table effectively.
Implementing keyboard accessibility requires careful attention to event handling. Users should be able to navigate items in the tree using arrow keys. Enter or space should expand or collapse nodes. This approach guarantees that user interaction is smooth and inclusive.
Here are several considerations for effective keyboard navigation:
- Focus Management: Ensure focus moves logically through table elements. It should be clear where the focus is at all times.
- Keyboard Shortcuts: Providing keyboard shortcuts can facilitate faster navigation for power users.
- Screen Reader Compatibility: Use appropriate aria-labels and roles to support assistive technologies.
Maintaining these accessibility standards not only complies with regulations but also enhances user satisfaction and engagement.
Investing in user interaction leads to a more rewarding experience for all users and promotes better utilization of the tool.
Styling and Presentation
The styling and presentation of a React Tree Table play a vital role in enhancing user experience and ensuring effective data comprehension. A tree table structures data hierarchically while maintaining the familiar table format, and this need for visual clarity makes styling crucial. Developers should carefully consider aspects like color schemes, spacing, and typography. These elements contribute to how users interact with the information presented.
Good styling helps to avoid confusion, particularly in complex datasets. Users should be able to quickly grasp the relationships between data points without overexerting their cognitive load. This is especially important for businesses that rely on data-driven decisions. Additionally, presenting data well can reinforce brand identity and improve overall aesthetics.
While this section focuses on the details of styling and presentation, it directly contributes to the functionality of the tree table. A polished look promotes usability. The following subsections explore specific approaches to achieving visually appealing and functional designs for React Tree Tables.
CSS Frameworks and Libraries
CSS frameworks and libraries offer pre-defined styles which can speed up the development process for React Tree Tables. Frameworks like Bootstrap, Material-UI, and Bulma provide a wide range of components that can be utilized for styling. For instance, Material-UI includes built-in themes and styles that align with the Material Design principles. Using such frameworks can lead to quicker implementation and a more consistent user interface.
Moreover, these frameworks often come equipped with responsive layouts. This allows React Tree Tables to perform well across different devices without additional effort. It is important to consider the theme and customization options offered by these frameworks, as they might influence brand alignment and overall aesthetic.
However, developers should be mindful of potential limitations. Relying too heavily on a framework can limit creativity and lead to a monotonous design. Sometimes, further customization or even complete custom styles become necessary to truly reflect the purpose of the application.
Custom Styling Approaches
Custom styling allows developers to tailor the appearance of React Tree Tables according to specific project needs. This approach often results in unique and inventive designs. Custom styling can be achieved using plain CSS, CSS-in-JS libraries, or preprocessors like Sass and LESS.
Utilizing CSS-in-JS libraries, such as styled-components or emotion, can be particularly beneficial in React projects. These libraries let developers write CSS directly in their JavaScript files, promoting a component-based architecture. It brings styling and logic closer together. Depending on the complexity and scale of the project, this type of styling can enhance maintainability.
Some considerations for custom styling include:
- Theme Consistency: Ensure that custom styles align with the overall look and feel of the application.
- User Preferences: Consider how different users might prefer visual settings. Implementation of dark mode or adjustability can lead to higher satisfaction.
- Performance Impact: Custom styles may impact performance differently. Using appropriate CSS selectors and minimizing unnecessary styles helps maintain efficiency.
Overall, whether using frameworks or custom styles, the goal remains the same. The objective is to enhance both the usability and appeal of React Tree Tables, ultimately leading to a more engaging user experience.
Optimization Techniques
Optimization techniques are critical when developing a React Tree Table. These strategies enhance application performance and ensure a smooth user experience. As data sets grow larger, the importance of effective optimizations becomes clear. Implementing a well-optimized tree table can reduce load times and improve responsiveness, leading to higher user satisfaction.
Performance Considerations
Performance optimization directly affects how efficiently a React Tree Table functions. Several factors come into play:
- Rendering Efficiency: React’s rendering process can become sluggish with extensive data. Leveraging techniques like memoization through the function helps prevent unnecessary re-renders, making the application faster.
- Virtualization: For large datasets, using libraries like can create a virtual scrolling effect. It loads only the portion of data visible to the user, which significantly reduces memory consumption and improves performance.
- Batching State Updates: React automatically batches updates in event handlers. Yet, ensuring that multiple state updates occur within a single event handler, or using a reducer for complex state management, minimizes the number of renders triggered.
Implementing these techniques not only improves performance but also provides a more responsive user interface. When users interact with a tree table, they expect fast feedback, which is crucial for data-heavy applications.
Memory Management
Efficient memory management is vital for a high-performing React Tree Table. Managing memory effectively involves strategies that minimize memory usage and prevent leaks or wasted resources. Key practices include:
- Use of Functional Components: Functional components often consume less memory than class components. They simplify the implementation of lifecycle methods and allow for easier optimizations.
- Avoiding Excessive State: Keeping component states minimal helps maintain memory performance. If data doesn’t need to be stored in state, consider deriving it from props or using calculations instead.
- Cleaning Up Resources: Implementing proper cleanup in hooks can prevent memory leaks. When components unmount, remove event listeners and clear timers accordingly.
Effective memory management directly correlates with application stability and performance. Failing to manage memory may result in a sluggish application, impacting user engagement.
"Optimizing performance and managing memory are essential to the longevity and usability of applications, particularly in data-intensive environments."
Developers must adhere to these techniques to ensure that their React Tree Tables perform well under varying user loads while maintaining an optimal memory footprint.
State Management
State management plays a crucial role in the development of React Tree Tables. It concerns how data is shared among various components, how changes in data are tracked and handled, and how user interactions can alter the present state. Achieving effective state management enhances the performance and usability of applications, making it particularly significant in scenarios involving complex user interactions and multiple levels of data hierarchy.
In a React Tree Table, managing state well ensures that users have a responsive interface. For instance, when a user expands or collapses a tree node, it is essential that the application reflects these changes immediately. Without proper state management, inconsistencies can occur, leading to frustration and confusion.
Using Context API
The Context API serves as an elegant solution to state management in React applications. It allows developers to create global states that can be accessed by any component within a tree, eliminating the need for prop drilling. In a Tree Table, this can help to manage expandable nodes and other shared states effectively.
One of the main advantages of using the Context API is that it simplifies the management of application state, especially for components that are deeply nested. For instance, instead of passing props down through multiple levels, you can store data in a context provider and access it directly where needed. This speeds up the process of building and maintaining the tree structure.
For example, here is how you can establish a simple Context for managing the state of a Tree Table:
This code snippet demonstrates establishing a context and a custom hook to use the tree data state. By utilizing this structure, components within the Tree Table can access and manipulate the state seamlessly.
Integration with Redux
Redux is another powerful tool for state management within React applications. It offers a more centralized approach to handling states, making it suitable for large applications like React Tree Tables. The main concepts in Redux are actions, reducers, and the store, which all work together to keep application state consistent and predictable.
When integrating Redux with React Tree Tables, you can manage complex state logic while keeping your components clean and intuitive. For instance, consider a Tree Table application where multiple users may manipulate data simultaneously. Redux can help in managing user actions, ensuring that any changes in state are dispatched and managed in a uniform manner across the application.
Additionally, Redux's middleware capabilities allow for asynchronous operations, such as fetching data from an API. Here’s a basic example of how you might set up Redux to manage state in a Tree Table.
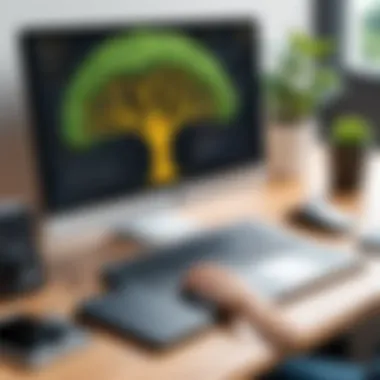
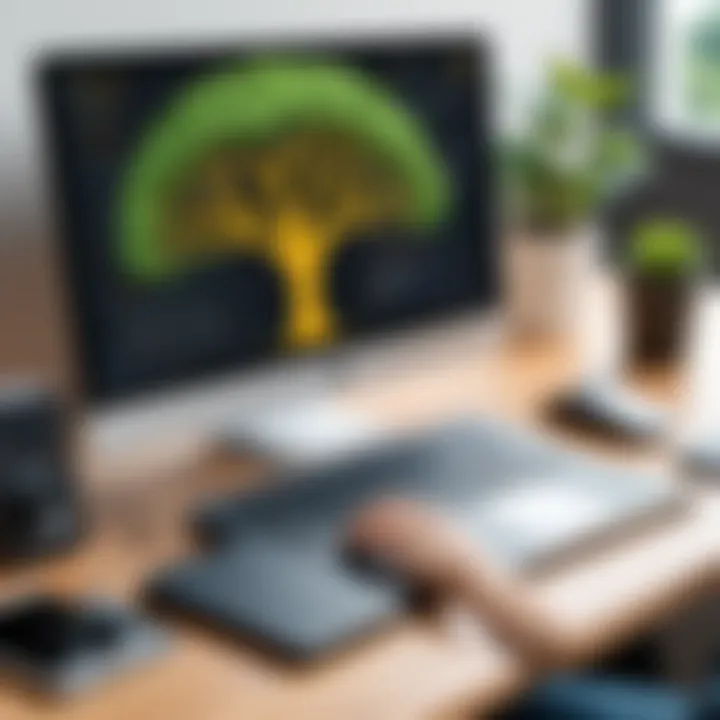
In this example, a reducer is created to handle setting the Tree Table data. The Redux store provides a centralized place for storing and accessing tree data, facilitating easier updates and modifications.
Effective state management is crucial for responsive user experiences, particularly in dynamic applications such as React Tree Tables.
Understanding and applying these concepts will greatly enhance the overall functionality and performance of your Tree Table application.
Real-World Applications
In today's data-driven environment, React Tree Tables play a crucial role in how information is presented and managed. Their importance extends beyond mere aesthetics, reaching deep into their functionality which optimizes user interaction. React Tree Tables are especially leveraged in various real-world scenarios where data complexity necessitates a clear hierarchical presentation. This article examines two significant applications: Data Management Tools and Reporting and Analytics Dashboards.
Data Management Tools
Data management tools are essential for efficiently handling vast amounts of information. React Tree Tables provide an intuitive way to display complex, nested data structures. This structure can represent any data that requires hierarchical relationships, such as file directories, organizational charts, or product categories. The ability to expand and collapse nodes allows users to navigate large datasets easily without feeling overwhelmed.
Benefits of using React Tree Tables in data management tools include:
- Clarity: Information is organized logically, making it easier to digest.
- Scalability: As data grows, tree tables can accommodate more nodes without losing performance.
- User Control: End users can interact with the data, exploring relevant details at their own pace.
However, considerations must be made regarding performance as datasets grow. Developers should implement effective lazy loading techniques to load data only when necessary. This keeps the application responsive and enhances user experience.
Reporting and Analytics Dashboards
Reporting and analytics dashboards benefit greatly from the functionalities that React Tree Tables offer. These tables allow for a clearer presentation of analytical data through a structured view. Insights derived from complex reports can be shown in a way that emphasizes relationships and hierarchies, leading to more informed decision-making.
Key advantages include:
- Enhanced Visualization: Users can see trends and patterns through hierarchical data representation.
- Interactive Elements: Users can drill down into specific data points without losing context, which fosters a deeper exploration of the data.
- Compiler for Insights: Reporting tools can compound various data types, so presenting them in a structured manner provides coherence to analytical summaries.
Developers must also consider user accessibility when building these dashboards. Incorporating features like keyboard navigation and assistive technologies ensures everyone can benefit from the insights presented. Providing clear labels and logical navigation paths within the tree table can significantly improve usability.
React Tree Tables are not just about presenting data; they shape how users interact with vast data sets effectively, making them essential in today's analytics-driven world.
Challenges in Implementation
The implementation of React Tree Tables poses several challenges that developers must navigate to ensure effective functionality and user experience. This section examines these challenges in depth, focusing on handling large datasets and managing complex user interactions. Recognizing and addressing these elements is essential for achieving optimal performance and a seamless user experience, which directly influences the success of applications utilizing React Tree Tables.
Handling Large Datasets
Managing large datasets is one of the most pressing challenges in implementing React Tree Tables. As data scales, the performance of the tree table can suffer significantly. Users typically expect quick access to data, which can become problematic if the dataset grows large. Key consideration areas include:
- Performance Issues: Rendering a significant number of nodes can lead to slower performance and longer load times. This can detract from the user experience.
- Virtualization: Implementing techniques like virtualization can greatly enhance performance. This involves only rendering nodes that are visible in the viewport, helping to minimize the workload on the browser.
- Data Fetching: Dynamic loading of nodes can also help manage large datasets. Instead of loading the entire dataset at once, it may be beneficial to fetch data as needed, such as through lazy loading or pagination.
- Search and Filter Capabilities: Integrating effective search and filter mechanisms is crucial. Users need to quickly locate specific data points within large sets.
Effective strategies for handling large datasets in React Tree Tables not only maintain performance but also enhance user satisfaction. Developers should consider each of these elements while planning their implementation strategy.
Complex User Interactions
User interactions with React Tree Tables can become complex, particularly in applications that require rich interactions. Managing these interactions effectively is crucial for a positive user experience. Challenges in this area include:
- Click and Expand Dynamics: The tree structure typically offers expand and collapse functionalities. Implementing this properly ensures users can easily navigate through data. If poorly designed, users may experience frustration when attempting to expand or collapse nested items.
- Keyboard Navigation: Accessibility remains a fundamental component of user interactions. Implementing keyboard navigation is vital for users who rely on keyboard shortcuts. If not handled correctly, accessibility can diminish, alienating a portion of the user base.
- Real-time Updates: Applications might require real-time interactivity, where data changes require immediate reflection in the tree table. This adds another layer of complexity in ensuring state synchronization across all components.
Managing complex user interactions in a React Tree Table not only enhances usability but also improves overall user satisfaction, which is a driving factor in user retention.
Addressing these challenges requires thoughtful design and rigorous testing. Developers must continually monitor and refine interaction models to meet evolving user expectations. By focusing on these critical aspects, applications can leverage the capabilities of React Tree Tables to their fullest potential.
Future of React Tree Tables
The future of React Tree Tables is promising, particularly as developers increasingly seek more efficient methods for data presentation. This section explores emerging technologies and potential enhancements that could shape how React Tree Tables function and serve user needs.
Emerging Technologies
As web technologies progress, new tools and libraries are increasingly integrated with React to improve the capabilities of Tree Tables. Among these, advancements in data visualization frameworks like D3.js and Chart.js stand out. These tools facilitate not only complex visual representations but also deeper insights into hierarchical data structures.
The rise of artificial intelligence and machine learning also plays a significant role. For instance, integrating AI can help in predictive data loading and user interaction customization. This adaptability can lead to a more seamless user experience, where data presents itself in the most relevant manner possible based on user interaction history.
In addition, the expansion of web components is noteworthy. Such components, built on standards for encapsulation, can improve the modularity of Tree Tables. This means developers will spend less time on integration and more time on optimization and functionality. Frameworks like Stencil and LitElement provide valuable resources in this domain, promising to enhance the versatility of React applications.
Potential Enhancements
The potential for enhancements in React Tree Tables is vast, offering multiple avenues for exploration. One significant aspect is the improvement of user interactivity through gesture controls and touch-enabled interfaces. This is critical as more users access applications via mobile devices. Enhancing touch feedback can lead to more intuitive user experiences.
Another area ripe for enhancement is the integration of real-time data updates. Utilizing websockets, for instance, would allow users to see dynamically changing information without needing to refresh their interfaces. This could be particularly useful in collaborative settings or dashboards that require constant updates, such as for stock prices or system analytics.
Performance management is equally crucial. Introducing techniques like virtualization can allow React Tree Tables to handle larger datasets more efficiently. Libraries such as react-window and react-virtualized enable developers to only render visible portions of the data, significantly enhancing performance.
"The adaptability of React Tree Tables will define their longevity and relevance in the fast-evolving tech landscape."
The End
The conclusion section of this article highlights the essential elements surrounding the use of React Tree Tables. It reinforces the significance of understanding their architecture, implementation techniques, and functional benefits that appeal to both experienced developers and newcomers. React Tree Tables offer a powerful solution for presenting hierarchical data effectively within web applications. The clarity of their structure combined with the flexibility of React allows developers to craft user experiences that are both efficient and intuitive.
Recap of Key Insights
In summary, several key points emerge from our exploration of React Tree Tables:
- Hierarchy Representation: React Tree Tables enable structured presentation of complex data sets, giving users an organized view.
- Interactive Features: Built-in functionalities like expand/collapse enhance user engagement and data navigation.
- Performance Optimization: Best practices for data management ensure that applications remain responsive even with large datasets.
- State Management: Effective integration of Context API and Redux facilitates smooth data flow and management across components.
- Accessibility Considerations: Attention to keyboard interactions ensures that applications meet diverse user needs.
These insights serve as pillars for any future development in applications involving React Tree Tables.
Final Thoughts on Development
As developers navigate the complexities of creating React Tree Tables, it is imperative to stay abreast of emerging practices and technologies that can enhance functionality. Future developments may encompass an expansion of accessibility features, improvements in data handling, and integration with evolving front-end frameworks. Experimentation and feedback loops with the end-users can yield valuable insights that refine the user experience. Overall, the breadth of knowledge and practical guidance provided makes tackling React Tree Tables a worthwhile endeavor for creating visually appealing and functionally superior data presentation tools.
Citing Sources and Further Reading
Citations within this article will guide the reader toward essential resources which can enrich their comprehension of React Tree Tables. It is vital for any developer to not only consume content but to also investigate the origins of the information. Here are some types of resources to consider:
- Technical Documentation: Official documentation like the React documentation website is invaluable for understanding the framework's updates and capabilities.
- Tutorials and Guides: Websites offering deep-dives into specific features, tutorials can provide hands-on experience.
- Research Papers: Seeking academic papers related to data presentation and UI components can offer insights into best practices and innovative methods.
- Community Forums: Platforms like Reddit can provide real-world perspectives and troubleshooting tips shared by developers.
- Books: Authors who specialize in React can provide comprehensive discussions and analysis in structured formats.
By incorporating diverse sources, readers can gain a multi-faceted view of the topic and its applications, ensuring they are well-equipped to use React Tree Tables effectively.