Exploring Top Algorithms in Computer Science
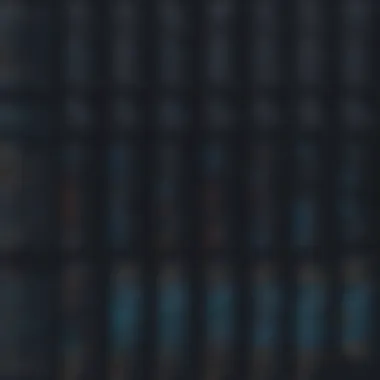
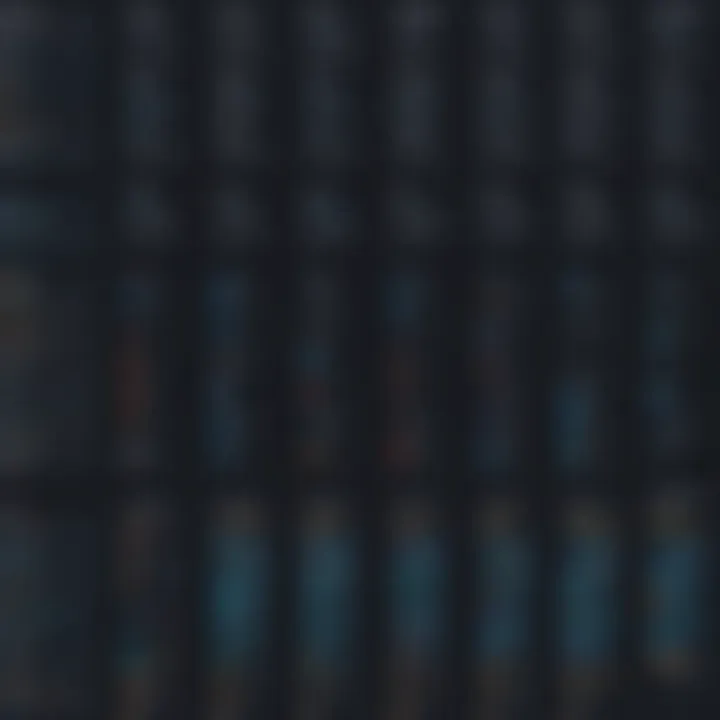
Intro
Algorithms form the backbone of computer science, influencing how data is processed, analyzed, and utilized across a multitude of applications. From simple sorting tasks to complex machine learning implementations, understanding the landscape of algorithms is crucial for anyone involved in technology. This article aims to provide a detailed exploration of the best algorithms in use today, shedding light on their applications as well as the advantages and challenges they pose.
Coding Challenges
Coding challenges often serve as a practical method to gauge an individual's programming skills. These challenges not only test knowledge but also encourage creative problem-solving. They are essential in competitive programming and technical interviews. Let's delve into some aspects of coding challenges:
Weekly Coding Challenges
Participating in weekly coding challenges can greatly enhance problem-solving skills. Websites such as HackerRank, LeetCode, and Codewars host regular challenges that cater to varying levels of expertise. Regular practice hones coding abilities and improves understanding of algorithms.
Problem Solutions and Explanations
Each coding challenge typically includes detailed explanations of solutions. Analyzing these solutions is crucial for understanding the different approaches to a problem. It provides insight into algorithmic efficiency and optimization. Moreover, many platforms allow users to discuss and share alternative solutions, offering a wider perspective.
Tips and Strategies for Coding Challenges
- Understand the Problem: Read the problem statement carefully. Misinterpretation can lead to incorrect solutions.
- Break Down the Problem: Decomposing a problem into smaller parts can simplify reasoning and lead to a more robust solution.
- Choose the Right Data Structures: Using appropriate data structures is often key to achieving optimal performance.
- Practice Regularly: Consistency is essential. Regular exposure to different types of challenges builds comfort and confidence.
Community Participation Highlights
Engaging with the programming community during challenges not only fosters learning but also opens avenues for networking. Platforms like Reddit feature communities where programmers discuss strategies and share experiences. Such interactions can facilitate a deeper understanding of both algorithms and coding cultures.
Technology Trends
Technological innovation is ever-evolving, and staying aware of trends is vital for professionals. Understanding new algorithms or improved versions of existing algorithms is essential for competitive advantage. Here are some notable trends:
Latest Technological Innovations
Solutions designed to incorporate machine learning and artificial intelligence algorithms are increasingly prominent. Technologies like TensorFlow and PyTorch are being widely adopted for developing advanced models.
Emerging Technologies to Watch
The field of quantum computing is generating excitement. Algorithms like Shor’s and Grover’s have the potential to outpace classical algorithms, changing the landscape of computing entirely.
Technology Impact on Society
The implications of these technologies impact data security, privacy, and ethics. Algorithmic transparency is now a debate point in the technology sector.
Expert Opinions and Analysis
Insights from industry leaders help decipher the ongoing changes in technological paradigms. Regularly following reports and commentary on algorithms can provide valuable foresight into future developments.
Coding Resources
For anyone aiming to deepen their understanding of algorithms, a plethora of resources exist:
Programming Language Guides
Published guides on programming languages such as Python, Java, and C++ often include sections specifically about algorithms. These can be useful for learners seeking context about algorithm implementation.
Tools and Software Reviews
Familiarity with tools like GitHub for version control, Visual Studio Code for editing, and various debuggers can streamline the coding process, thus affecting algorithm execution.
Tutorials and How-To Articles
Websites and forums present a wealth of tutorials focusing on algorithm development. These act as practical guides for understanding complex concepts through hands-on experience.
Online Learning Platforms Comparison
Platforms such as Coursera, edX, or Codecademy offer structured courses on algorithms. Comparing their course offerings based on learner reviews can assist in choosing the right learning path.
Computer Science Concepts
A grasp of fundamental concepts in computer science is essential for understanding algorithms comprehensively.
Algorithms and Data Structures Primers
Basic data structures include arrays, linked lists, and trees. Familiarity with these structures aides in applying algorithms effectively.
Artificial Intelligence and Machine Learning Basics
An introduction to AI and machine learning provides context for more advanced algorithms like neural networks and decision trees.
Networking and Security Fundamentals
Knowledge of network protocols and security basics aids in understanding algorithms related to data transmission and encryption.
Quantum Computing and Future Technologies
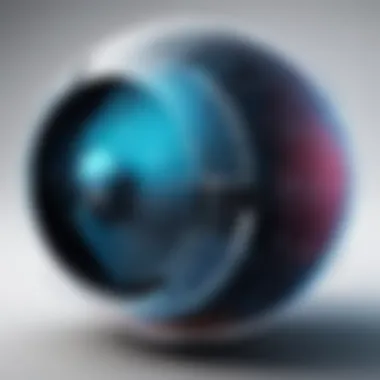
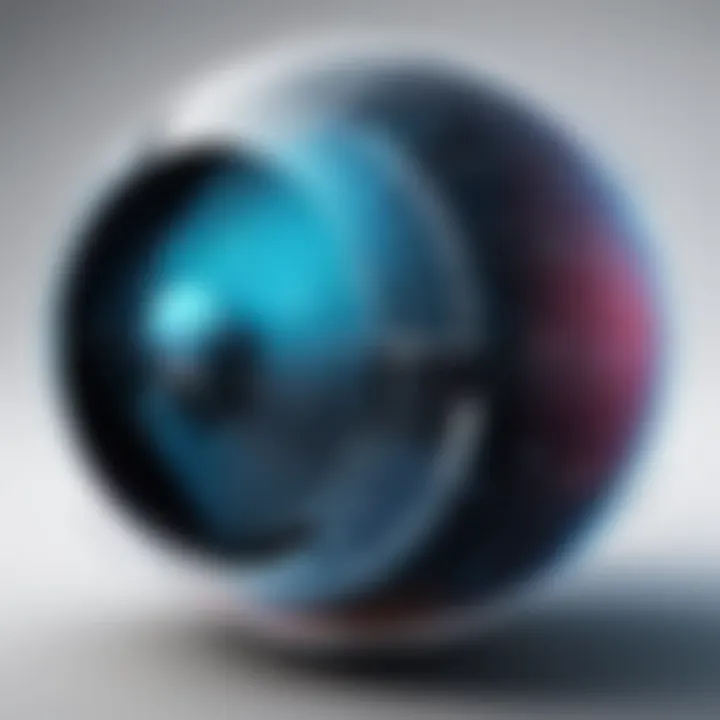
Emerging fields like quantum computing present new algorithmic techniques that challenge classical paradigms. Keeping up with these developments is essential for forward-thinking professionals.
Preamble to Algorithms
Algorithms are the backbone of computer science and technology. Their study encompasses a wide range of techniques that solve problems efficiently and effectively. Understanding algorithms provides insight into how computers process information, enabling optimizations in various practical applications, from software development to data analysis. This section focuses on several key aspects of algorithms, which will set the foundation for the following discussions in the article.
Definition and Importance
An algorithm is a step-by-step procedure for solving a given problem or performing a computation. They are crucial in defining how tasks are completed with precision. Without well-defined algorithms, tasks can become erratic and inefficient, leading to poor performance in software applications.
The importance of algorithms cannot be overstated. In an era where data is abundant, algorithms enable the processing and retrieval of information in a manageable way. They facilitate everything from simple calculations to complex data mining techniques. In short, algorithms are the guiding principles that govern the orderly execution of tasks in computer systems.
Types of Algorithms
Algorithms can be classified into several categories, each serving distinct purposes. This section will elaborate on three primary types:
- Sorting Algorithms: These algorithms arrange data in a specified order. Their key characteristic is the ability to reorder data quickly and efficiently. Popular examples include Quick Sort and Merge Sort. Sorting algorithms are beneficial because they improve the efficiency of search operations and facilitate data analysis. However, some sorting methods can be less effective on large datasets, highlighting the need for careful selection based on the context.
- Search Algorithms: These algorithms enable the retrieval of data from structured sets. Their primary feature is the capacity to locate items quickly, significantly accelerating the data findability in large datasets. Examples such as Binary Search provide efficient ways to access information. The implications of good search algorithms are profound, as they enhance user experience and decrease response time in applications. Nonetheless, they often require sorted data to perform optimally.
- Graph Algorithms: Algorithms that are tailored for navigating graphs. They optimize the search for paths and connections within network structures. Dijkstra's Algorithm is a classic example that seeks the shortest path, which is essential in network design and analysis. Graph algorithms are advantageous in situations where relationships are critical, such as social networks or transportation systems. Yet, their complexity can also introduce challenges in implementation and resource management.
Overall, understanding these types of algorithms is essential for anyone entering the field of computer science. The insights gained will help inform future discussions about best practices and real-world applications, making algorithms an intriguing topic worthy of exploration.
Best Sorting Algorithms
Sorting algorithms play a critical role in computer science as they organize data efficiently to enhance performance when processing data sets. This article examines two of the most recognized sorting algorithms: Quick Sort and Merge Sort. By understanding their mechanisms, efficiencies, and applications, readers can appreciate how these algorithms facilitate various data management tasks.
Sorting algorithms not only improve data organization but also optimize search operations. Efficiency in sorting can directly impact the overall execution time in applications, making it a focal point for developers and programmers. Moreover, with the growing volume of data, the ability of an algorithm to scale its performance becomes essential. Understanding the nuances of sorting algorithms helps in the right application of these techniques in software development.
Quick Sort
Quick Sort is widely adopted for its efficiency and performance in sorting large data sets. Its divide-and-conquer approach helps in minimizing the time complexity, making it an appealing choice for many programming tasks.
Efficiency Analysis
An important aspect of Quick Sort is its average time complexity of O(n log n). This efficiency contributes significantly to its popularity and is one of the main reasons it is frequently chosen for practical applications. The key characteristic of Quick Sort lies in its method of partitioning the data into smaller sub-arrays that are sorted independently.
However, Quick Sort can exhibit worst-case performance of O(n²), particularly when dealing with already sorted data or datasets where all elements are the same. This drastic drop in performance is a disadvantage, particularly in scenarios requiring predictable execution times. On the whole, the efficiency of Quick Sort makes it a contributor to faster computations in many scenarios, which is vital in modern application development.
Use Cases
Quick Sort finds its application in various real-world scenarios, especially in programming environments where speed is essential. File sorting and data processing tasks often implement Quick Sort due to its efficiency.
One unique feature of Quick Sort is its ability to be implemented in place, which reduces space complexity to O(log n). This effectively utilizes memory, an important consideration in environments with limited resources. However, its not-so-stable nature means that it may not preserve the relative order of equal elements, which can be a drawback in some applications where data stability is required.
Merge Sort
Merge Sort is another deeply studied sorting algorithm that stands out for its stable sorting capability. It uses a different approach than Quick Sort, focusing on merging sorted sub-lists into a single list.
Stability of Merge Sort
The stability of Merge Sort is a vital aspect, particularly in scenarios where the order of equal elements must be maintained. By preserving the input order of equivalent elements, Merge Sort proves beneficial in applications that require sorted lists where this characteristic is crucial.
The algorithm’s average and worst-case time complexity stands at O(n log n), similar to Quick Sort, but it does not suffer from the worst-case scenarios Quick Sort does. This may make Merge Sort a better choice in applications where performance predictability outweighs raw time performance.
Comparison with Other Sorts
When comparing Merge Sort with other sorting algorithms, its consistent runtime characteristics give it an edge over less efficient sorting methods. For example, Bubble Sort and Insertion Sort commonly exhibit average time complexities of O(n²), making them unsuitable for large datasets.
The unique feature of using additional memory to store temporary arrays during the sort can be viewed as a disadvantage of Merge Sort, but the benefits of stability often compensate for the increased space requirement. In environments where memory is accessible, Merge Sort can outperform its counterparts by providing better data management and efficiency.
Best Search Algorithms
Search algorithms play a crucial role in computer science. They enable the retrieval of information from data structures efficiently. Understanding various search algorithms helps in optimizing the way data is accessed and manipulated. Each algorithm has its own pros and cons, which can greatly influence the performance of applications across multiple domains. This section aims to explore two significant search algorithms: Binary Search and Depth-First Search, providing insights into their workings and applications.
Binary Search
Binary Search isthe optimal method for searching in a sorted array. Its efficiency is rooted in the principle of divide and conquer, where the algorithm repeatedly divides the search interval in half. This significantly reduces the number of comparisons needed to find a target value, making Binary Search an essential tool in scenarios where speed and efficiency are paramount.
Algorithm Steps
To perform a Binary Search, follow these steps:
- Initial Setup: Set two variables, low and high, to represent the bounds of the search. Low starts at the beginning of the array, and high starts at the end.
- Midpoint Calculation: Calculate the midpoint index by taking the average of low and high.
- Check Middle Element: Compare the middle element with the target. If they are equal, the search is successful.
- Adjust Bounds: If the target is less than the middle element, adjust high to mid - 1. If the target is greater, adjust low to mid + 1.
- Repeat: Continue the process until the target is found or low exceeds high.
The key characteristic of Binary Search is its logarithmic time complexity, O(log n), which allows it to handle large datasets efficiently. However, it requires the array to be sorted beforehand, which might be a disadvantage when faced with unsorted data.
Performance Metrics
When evaluating the performance of Binary Search, some key metrics include:
- Time Complexity: As mentioned, the average and worst-case time complexity is O(log n). This shows a remarkable efficiency gain compared to linear search, which has a complexity of O(n).
- Space Complexity: The space utilization remains O(1) in the iterative implementation, indicating minimal resource consumption.
The unique feature of these metrics is their ability to scale with data size. While Binary Search is quite efficient, its prerequisite for sorted data can be a limiting factor. If input sorting is needed, the overall complexity may climb to O(n log n), thus diminishing its advantages in some cases.
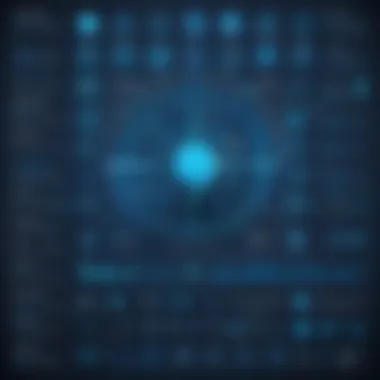
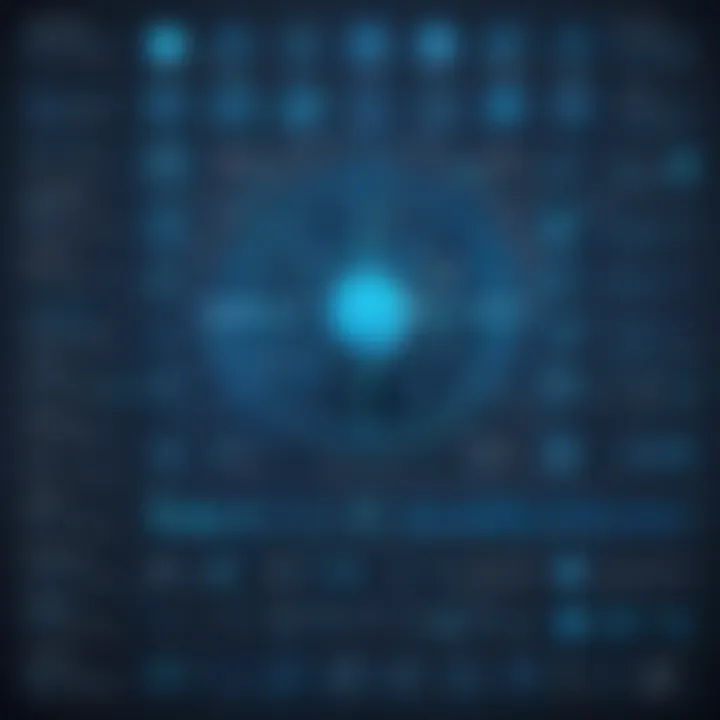
Depth-First Search
Depth-First Search (DFS) is another pivotal search algorithm, primarily used in graph traversal. It explores as far as possible along a branch before backtracking, making it suitable for certain types of problems like pathfinding in game development or solving puzzles.
Implementation Techniques
Implementing DFS can be approached in two main ways:
- Recursive Approach: This method involves using recursive function calls to implement the depth-first traversal logic. The simplicity of this approach allows for easier maintenance.
- Stack-based Approach: Using a stack data structure, the algorithm can simulate recursion, enabling traversal without deep function calls.
Both methods yield the same result but vary in their characteristics. The stack-based approach may provide better control over memory usage and handle deeper graphs better without risking stack overflow.
Use Cases in Graphs
The applicability of DFS extends to various areas, including:
- Pathfinding Algorithms: In maze-solving, where all possible routes need exploration.
- Network Analysis: To discover all connections or web crawlers, which traverse the internet data.
The key characteristic of DFS is its capability to explore all nodes efficiently. Its depth-centric approach can be beneficial for complete traversal. However, it may not always provide the shortest path in graphs, making other algorithms more suitable for that purpose.
Consideration: Each search algorithm serves specific needs. Choosing the right one depends on the kind of data structure used and the goals of the application. Understanding their strengths and weaknesses is fundamental for any development work.
Best Graph Algorithms
Graph algorithms play a crucial role in many applications across computer science and technology. Their capability to process and analyze structures that represent pairwise relationships makes them invaluable in fields such as networking, AI, and more. In this section, we will explore two of the most influential graph algorithms: Dijkstra's Algorithm and the A* Search Algorithm. Each offers unique strengths and has specific use cases that highlight their importance. Understanding these algorithms contributes significantly to the overall comprehension of algorithmic efficiency in real-world scenarios.
Dijkstra's Algorithm
Application in Networks
Dijkstra's Algorithm is primarily recognized for its role in determining the shortest paths from a single source node to all other nodes in a weighted graph. Its primary application in networks allows for the efficient routing of data packets. By leveraging the pre-defined weights, which represent costs or distances, the algorithm systematically identifies the best routes for data transmission.
One key characteristic of Dijkstra's Algorithm is its use of a priority queue to select the most promising nodes for exploration. This feature not only streamlines the process but also enhances performance.
In practical scenarios, Dijkstra's Algorithm is popular due to its ability to handle dynamic changes in the graph. For instance, if a new path is added or a cost changes, Dijkstra's can rapidly adjust without the need to re-compute the entire graph. However, it is essential to note that the algorithm struggles with negative weight edges, which can limit its application in certain contexts.
Complexity Analysis
The complexity analysis of Dijkstra's Algorithm reveals important insights regarding its performance. The algorithm runs in O(V^2) time complexity using a simple array, where V is the number of vertices. This can be improved to O(E + V log V) where E represents the number of edges. This enhancement typically employs a priority queue, which optimally manages node selection.
The uniqueness of this complexity lies in its scalability. As the graph grows, the improved version maintains feasible performance. However, while it excels in dense graphs, it may not be as efficient in sparse graphs compared to other algorithms like the Bellman-Ford method. Thus, understanding its complexity aids in making informed decisions about which algorithm to deploy based on graph characteristics.
A Search Algorithm
Why A is Effective
A* Search Algorithm is esteemed for its efficiency in pathfinding and graph traversal. It uses both the actual cost to reach a node and a heuristic estimate of the cost to reach the goal. This combination allows A* to explore promising paths and bypass less optimal routes effectively.
A key attribute of A* is its adaptability. By adjusting the heuristic function, one can tailor the algorithm for specific applications. This flexibility often leads to faster solutions, especially in problems requiring real-time responses, such as navigation systems. However, it's important to manage the heuristics carefully. An overly aggressive heuristic could lead to suboptimal solutions, negating its benefits.
Use Cases in AI
In terms of use cases in AI, the A* Search Algorithm shines in game development and robotic navigation. Developers favor A* for its balance of performance and simplicity when devising game AI behaviors or routing for autonomous vehicles. Its efficacy in these domains can significantly enhance functionality.
As it provides clear and accurate paths, A* can improve user experience by reducing the time taken to make decisions in complex environments. Nevertheless, the overhead of calculating heuristics can sometimes slow down performance in larger datasets, so careful consideration is essential.
A* Search Algorithm remains a prime tool for solving practical pathfinding problems, blending usability with efficiency.
Emerging Algorithms
Emerging algorithms signify a pivotal advancement in the landscape of computer science and technology. As we navigate through a world increasingly reliant on data and computational power, the role of novel algorithms becomes crucial. They not only tackle complex problems but also optimize existing processes. Key considerations include the adaptability of these algorithms to real-world problems, their computational efficiency, and their potential applications across various sectors. By understanding these emerging algorithms, one can appreciate their transformative effects on industries ranging from healthcare to finance.
Machine Learning Algorithms
Supervised Learning
Supervised learning stands as a cornerstone of machine learning, focusing on training algorithms using labeled datasets. This aspect allows the model to learn from input-output pairs, making it effective for predictions and classifications. The key characteristic that makes supervised learning prominent is its ability to achieve high accuracy with sufficient data. This is particularly beneficial for applications such as fraud detection or recommendation systems.
A unique feature of supervised learning is its reliance on vast amounts of labeled data, which can be a double-edged sword. While it provides an effective way to train models, gathering and labeling data can be time-consuming and often costly. Moreover, models may struggle with unseen scenarios as they heavily depend on the provided data during training.
Unsupervised Learning
Unsupervised learning, in contrast, deals with unlabeled data, allowing algorithms to identify patterns or groupings on their own. This aspect is vital for discovering hidden structures in data, making it significant in fields such as customer segmentation or anomaly detection. A key characteristic of unsupervised learning is its capacity to glean insights from vast datasets without human intervention. This autonomy makes it increasingly popular in data science.
The unique feature of unsupervised learning lies in its variability. Unlike supervised approaches, unsupervised learning does not require labeled data. However, it presents challenges such as difficulty interpreting the results. Outputs can often lack structure or might reveal patterns that are not readily actionable, which can be a disadvantage for businesses looking for direct insights.
Quantum Algorithms
Shor's Algorithm
Shor's algorithm represents a remarkable advancement in the field of quantum computing, particularly in breaking widely used encryption schemes. Its contribution to the overall topic is significant because it demonstrates the potential power of quantum algorithms in tackling problems inefficient for classical computers. The key characteristic of Shor's algorithm is its ability to factor large integers exponentially faster than the best-known classical algorithms, posing a serious challenge to current cryptographic systems.
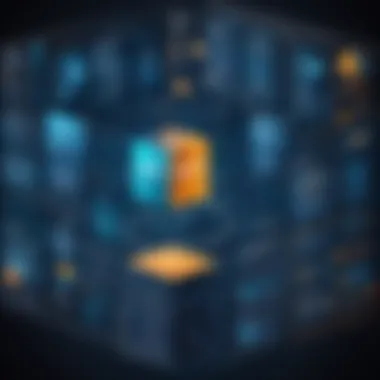
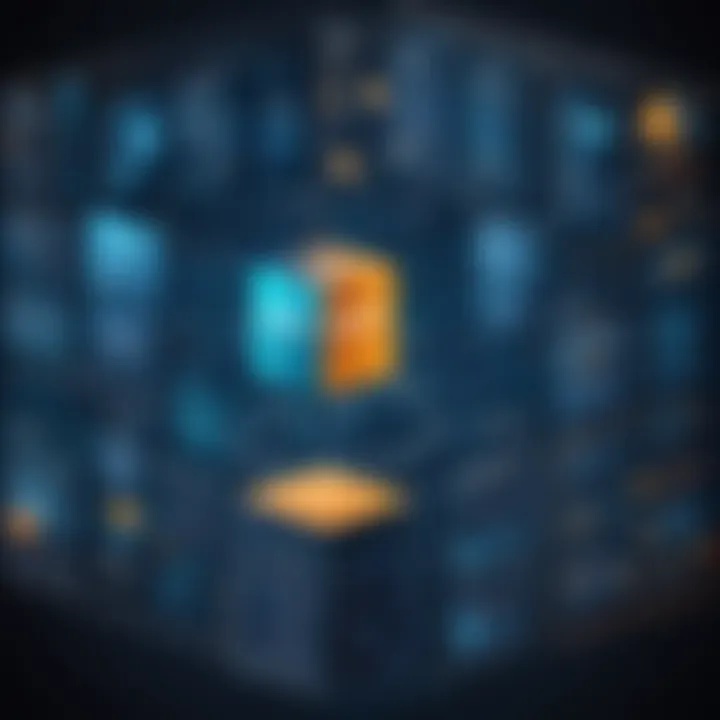
The unique feature of Shor's algorithm lies in its application to integer factorization, a problem foundational to modern cryptography. However, the requirement for a functional quantum computer remains an obstacle. The technology is still in its infancy, and the advantages of Shor's algorithm are theoretically promising, while practically implemented quantum systems remain limited and currently expensive.
Grover's Algorithm
Grover's algorithm provides another compelling glimpse into quantum computing, specifically focusing on unstructured search problems. It offers a quadratic speedup for searching through unordered databases, making it highly relevant in data retrieval contexts. The key characteristic of Grover's algorithm is its ability to reduce the time complexity of search operations significantly compared to classical databases.
A unique feature of Grover's algorithm is its versatility. It can be applied to a variety of problems, from optimizing search queries to solving complex combinatorial puzzles. However, like Shor's, it relies on the availability of quantum hardware, which is still under development. This presents a notable limitation, as the theoretical benefits are yet to be realized in practical scenarios.
Understanding emerging algorithms is essential for anyone involved in technology, as they represent the future of computational problem-solving, targeting inefficiencies and opening new avenues of inquiry in various domains.
Algorithm Optimization Techniques
Algorithm optimization techniques are crucial in the understanding of algorithms as they directly influence performance and efficiency. As algorithms can be resource-intensive, optimizing them ensures that they run in an optimal manner while handling large datasets or complex problems. This section will explore time complexity reduction and space complexity management, two key aspects of algorithm optimization, detailing their significance and practical implications.
Time Complexity Reduction
Big O Notation
Big O Notation is a foundational concept in algorithm optimization, representing the upper bound of an algorithm in terms of time complexity. It allows programmers to describe the efficiency of an algorithm by articulating the worst-case scenario. This is key because it helps in comparing algorithms under the same conditions.
The primary characteristic of Big O Notation is its focus on the growth rate of an algorithm's runtime as the size of the input increases. This simplicity is beneficial, as it provides a clear framework for evaluating performance. For instance, an algorithm with a time complexity of O(n) will perform linearly, while O(n^2) indicates that performance will degrade exponentially with input size.
One unique feature of Big O Notation is its abstraction. It disregards constant factors and lower-order terms, leading to a focus on the dominant term. While this can simplify understanding, it can also mask performance issues in certain scenarios. Therefore, while it is a popular choice in this article, complexities like constants or hidden factors can be overlooked in real-time applications.
Amortized Analysis
Amortized Analysis provides insight into the average performance of an algorithm over a sequence of operations, making it especially useful for data structures that require occasional costly operations like resizing. In contrast to worst-case time complexity, this analysis looks at the long-term performance across multiple operations.
The key characteristic of Amortized Analysis is its ability to present a more realistic scenario for evaluating an algorithm's efficiency. It treats expensive operations as infrequent occurrences. For example, when adding items to an array that requires resizing, the individual cost of resizing is spread over all operations, leading to a lower average time per operation.
This method is beneficial in practice, as it allows developers to navigate trade-offs and improve performance without over-emphasizing rare worst-case events. However, understanding its nuances can be challenging. The advantage of Amortized Analysis is clear, but improper application can lead to misjudgments regarding an algorithm's efficiency.
Space Complexity Management
Mutable vs Immutable Structures
Mutable and immutable structures play a significant role in space complexity management. Mutable structures allow changes, while immutable ones do not. The key characteristic of mutable structures is flexibility, as elements can be updated or changed without creating a new object. In contrast, immutable structures are fixed and require new objects for any changes.
Mutable structures are beneficial when changes to the data are frequent, as they minimize memory overhead. On the other hand, immutable structures can lead to safer, more predictable code, particularly in concurrent programming, where shared data undergoes modifications. However, excessive use of mutable structures can lead to issues such as memory leaks.
Memory Efficiency
Memory efficiency refers to the use of memory resources in the execution of algorithms. It is a key factor that impacts overall system performance. When discussing memory efficiency, the goal is to minimize waste while maintaining the effectiveness of the algorithm.
The key characteristic of memory efficiency is its influence on both time and space complexities. Efficient memory usage leads to reduced access times and lower overhead. This efficiency is a beneficial choice for systems with limited resources, such as embedded systems or mobile devices.
In practical aspects, poor memory efficiency can result in increased latency and slower performance. Thus, optimizing algorithms not only focuses on time but also on ensuring that memory usage is kept to a minimum while achieving desired functionality. Proper attention to memory efficiency can drastically improve overall algorithm performance and user experience.
Real-World Applications of Algorithms
Algorithms serve as the backbone of many modern technologies. They are crucial in various domains, influencing the way data is processed and utilized. In the world we live in, understanding how algorithms function can help us appreciate their role in solving practical problems. This section explores some key areas where algorithms are applied, highlighting their significance and the benefits they offer.
Algorithms in Web Development
Web development relies heavily on algorithms to create efficient and responsive applications. These algorithms facilitate various functions, from data handling to user interaction.
Data Retrieval
Data retrieval is a fundamental aspect of web development. It involves fetching data from databases or APIs to present it to users. This process is vital for creating dynamic content that reacts in real-time to user inputs.
One key characteristic of data retrieval is its speed and efficiency. When a user inputs information on a website, such as a search query, an effective data retrieval algorithm ensures that results are provided swiftly. This capability is essential for maintaining user engagement and satisfaction.
A significant advantage of data retrieval algorithms is their ability to optimize the amount of data fetched, often using caching mechanisms. However, one must consider the potential section of outdated or irrelevant data during retrieval processes. The balance between speed and data accuracy can sometimes be a challenge.
User Experience Optimization
User experience optimization focuses on improving how users interact with web applications. Algorithms play a pivotal role in analyzing user behavior to suggest improvements and create more seamless interactions.
A key aspect of user experience optimization is personalization. Algorithms can track users' preferences and patterns, thereby providing tailored experiences. This feature makes websites more appealing and encourages longer visits.
While user experience optimization brings significant benefits, such as increased engagement, it can also introduce complexity. If not managed well, excessive personalization may lead to information overload or privacy concerns. Finding an equilibrium is vital for effective user experience design.
Algorithms in Data Science
Data science has emerged as a critical field where algorithms are extensively employed. From extracting insights from data to predicting future trends, algorithms play a significant role in decision-making processes across industries.
Data Cleaning Techniques
Data cleaning techniques are necessary for preparing raw data for analysis. This process ensures the data used is accurate, consistent, and ready for further evaluation. The importance of data cleaning cannot be understated, as quality data directly impacts the reliability of outputs and conclusions drawn.
A prominent feature of data cleaning algorithms is their ability to identify and rectify inconsistencies, such as duplicate entries or missing values. These algorithms automate a tedious process, saving considerable time and resources. However, the downside includes the potential for losing valuable information during the cleaning process, which can skew results.
Predictive Modeling Algorithms
Predictive modeling algorithms aim to forecast future events based on historical data. They are widely used in various sectors, including finance, healthcare, and marketing.
The power of these algorithms lies in their ability to generate actionable insights and make informed predictions. This attribute makes them particularly appealing for organizations looking to improve their strategic decisions.
Nonetheless, predictive modeling algorithms come with challenges. The models can become overly complex, leading to difficulties in interpretation. Additionally, their accuracy heavily depends on the quality of the input data. Overall, when applied correctly, predictive modeling can significantly enhance decision-making capabilities.