Mastering Fast For Loops in Python for Optimal Performance
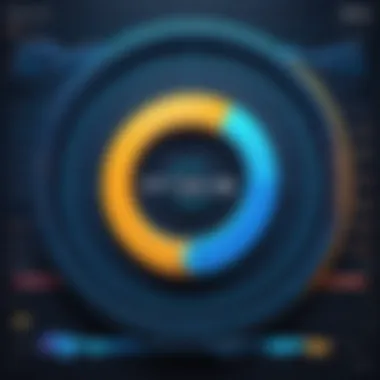
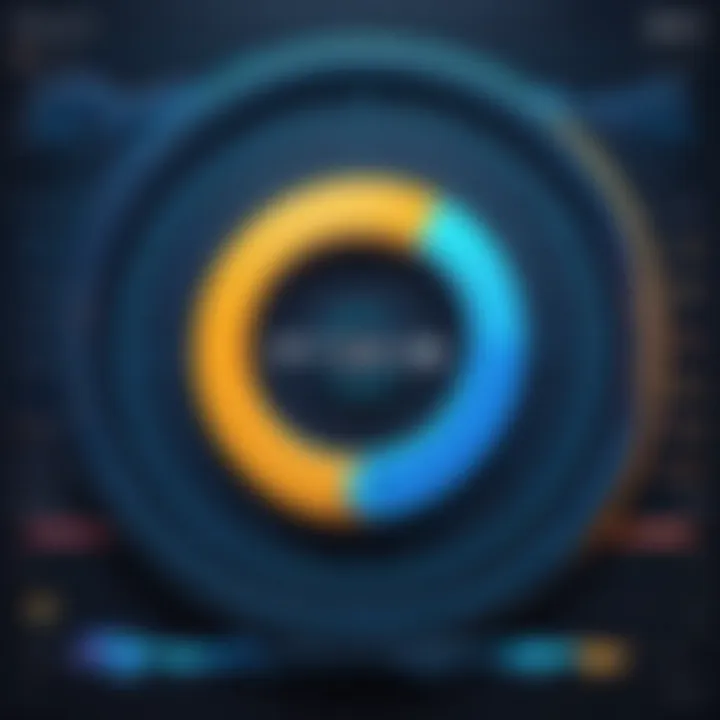
Intro
In the world of Python programming, efficiency is a topic that never grows old. With each passing day, developers are pressed to enhance the performance of their code. One valuable aspect of this is optimizing loops. Loops are fundamental to any programming endeavor, serving as the backbone for iterating through data. However, the traditional for loop can at times be a bottleneck if not handled properly. This article explores the often overlooked yet significant aspects of fast for loops, offering insights and techniques aimed at boosting the speed and efficiency of your Python code.
Coding Challenges
When it comes to honing your coding skills and assessing your grasp of loop optimization, coding challenges provide a golden opportunity. Tackling these challenges involves not just writing functioning code but doing so with performance in mind. Below is what a well-crafted coding task could include:
Weekly Coding Challenges
Consider engaging in weekly coding challenges that focus specifically on using loops in various scenarios. These might include:
- Analyzing the efficiency of different looping methods
- Tasking you with implementing a solution in as few lines as possible
- Asking you to refactor a sluggish loop into a faster variant
The idea isnât merely to solve the problem, but to do it swiftly and effectively.
Problem Solutions and Explanations
After working through problems, comparing solutions with your peers is essential. Understand every nuance of your approach and how it stacks against more optimal solutions. This collaborative aspect can lead to deeper insights into constructs that might not be immediately apparent.
Tips and Strategies for Coding Challenges
Optimizing for performance can be daunting without a roadmap. Here are a few strategic tips:
- Identify areas where a loop can be simplified, such as merging loops that can work in tandem.
- Avoid unnecessary calculations within the loop body. Instead, try pre-computing values where it makes sense.
- Consider leveraging Python's built-in functions, which are often more efficient than manually coded loops.
Community Participation Highlights
Becoming part of the coding community can significantly enhance your skills. Participating in forums like Redditâs r/learnpython can provide diverse perspectives on loop optimization challenges. By discussing various solutions and strategies, you build a richer understandingâsomething that can be crucial for both novice and seasoned developers.
Culmination
Loop optimization is more than just a skill; itâs an art form that reveals the beauty of programming. By exploring fast for loops and participating in coding challenges, you can not only sharpen your technical skills but also increase your appreciation for Pythonâs flexibility and efficiency. As you continue your journey into optimizing loops, remember that every bit of knowledge contributes to writing better, faster, and more efficient code.
Foreword to For Loops in Python
In todayâs programming landscape, for loops have emerged as a cornerstone of coding efficiency, particularly in Python. Their flexibility allows developers to iterate over various data structures, including lists, tuples, and dictionaries. A nuanced understanding of for loops goes beyond mere comfort with syntax; it's about mastering their potential to enhance performance and reduce execution time.
For loops enable a clear structure in traversing sequences. They not only streamline code but also elevate readability, granting less experienced programmers the ability to grasp complex processes with ease. At the same time, seasoned coders recognize that optimizing these loops can be critical in demanding applications, where every millisecond counts.
Importance of For Loops
For loops are significant because they encapsulate repetitive tasks within a compact framework. This can transform convoluted code into a series of elegant, understandable steps. Moreover, the capacity to iterate through large datasets opens the door to sophisticated data manipulations.
Consider the common scenario of data analysis, where the ability to loop through thousands of records and perform operations can shape results. Without for loops, this would involve tedious, error-prone code.
Benefits and Considerations
- Efficiency: For loops allow quick iterations over collections. By understanding their mechanics, one can identify and mitigate performance bottlenecks effectively.
- Readability: Properly formatted for loops can make code much easier to read than alternatives, such as while loops.
- Control: The built-in features of Python's for loops, such as the function, provide further control over iterations.
Staying mindful of how loops function can also minimize the chances of performance dips, especially when peaks in processing need to be managed. Thus, diving into optimizing loops is not just beneficial but essential for any serious programmer aiming to hone their craft.
"In Python, understanding for loops is like having a compass in the wilderness of code; it guides the way through data and operations."
Performance Concerns with For Loops
When coding in Python, one cannot simply glance over the performance of for loops. For many applications, particularly those handling large datasets or computational-heavy tasks, the efficiency of loop constructs can mean the difference between a trampoline jump and a cliff dive in execution time. Understanding this section is crucial. It's not just about accomplishing tasksâit's about doing so in a manner that respects the user's time and resources.
One might wonder why performance matters so much with basic looping. The simple truth is, as the saying goes, time is money. Developers are often faced with tight deadlines, and inefficient code can lead to frustrating delays. In high-stakes environments, where split-second decisions can affect outcomes, subpar loop performance can spell disaster. Thus, grasping the nuances of performance concerns with for loops is about enhancing productivity while minimizing risk.
Identifying Bottlenecks in Loop Performance
To truly optimize for loops, itâs essential first to pinpoint where things might be slowing down. A bottleneck isnât just a theoretical concept; itâs a real challenge that can lurk in various places within a loop. For example, if youâre iterating through a large list and making multiple database calls within that loop, the overhead can accumulate dramatically, making your code crawl like a tortoise in molasses.
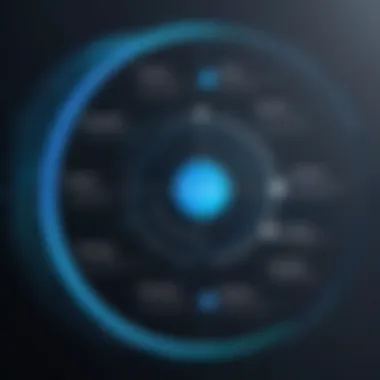
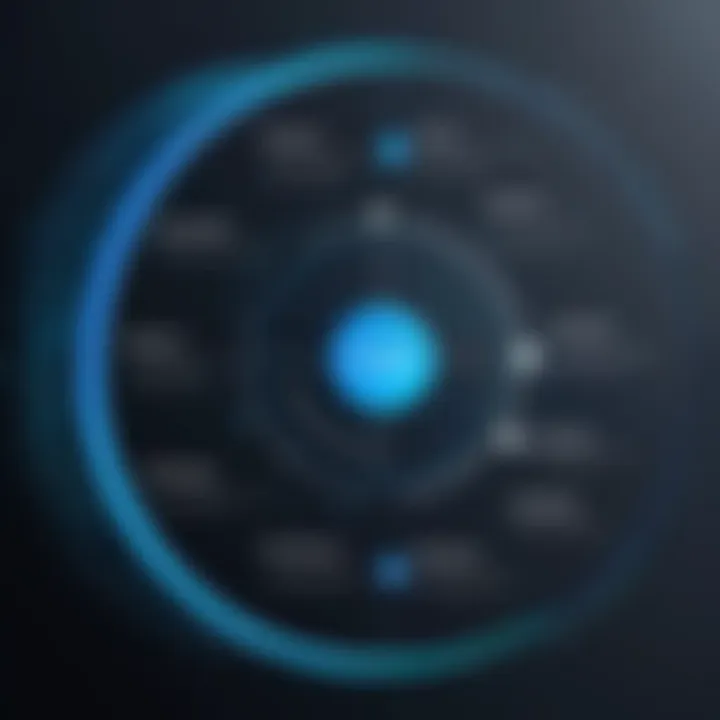
You can start identifying these bottlenecks by applying certain practices:
- Code Profiling: Tools like provide insights into which parts of your loops are causing performance hits. Profiling can help shine a light on what functions are called most frequently or are taking the longest time to execute.
- Avoid Redundant Computation: If there's a calculation that doesn't change within the loop, hoist it outside the loop. This simple refactoring can provide significant performance boosts.
- Minimize Function Calls: Pay attention to any functions called within loops; sometimes, reducing the number of calls can save more time than expected.
The aim is to keep your loops clean, efficient, and as fast as a hareâafter all, nobody wants to find their code reminded of a tortoise race.
Time Complexity in Loop Execution
Loop performance crashes through the walls of efficiency when we start discussing time complexity. Thisâput simplyâmeasures the time it takes to run an algorithm as a function of the size of the input. When you're dealing with loops, getting a grip on time complexity can be a game-changer.
Letâs say you have nested for loops. If the outer loop runs times, and the inner loop also runs times, youâre looking at a complexity of O(n²). That can get out of hand fast. But it doesnât end there.
Consider this:
- O(1): This is constant time. Whether you have one element or a thousand, the operation takes the same amount of time.
- O(n): This signifies that the time taken grows linearly with the increase in data size. Thatâs a one-to-one correlationâwhich is manageable.
- O(log n): Great for search algorithms. This is logarithmic, meaning fewer comparisons as data grows.
- O(n²): This can be a headache, especially with nested iterations. You have to be cautious; otherwise, itâs slow as syrup.
"Understanding the time complexity of your loops can keep your programs running smoothly like a well-oiled machine."
Incorporating strategies to assess and manage time complexity is pivotal in writing efficient code. The bottom line? Knowing how loops interact with data size can save you a mountain of frustration and help keep your code on the fast track.
Techniques for Fast For Loops
In the quest for efficiency in programming, especially in Python, the ability to optimize for loops stands as a vital skill. Fast for loops not only enhance the performance of your code but also streamline processes that can otherwise bog down applications. When dealing with large datasets or time-sensitive applications, employing the right techniques can lead to significant benefits. This section delves into strategies that can be employed to elevate loop performance, discussing their specific benefits and what considerations should be made.
Using List Comprehensions
List comprehensions are one of the cornerstones of Python programming, making it both cleaner and more efficient. They allow for the creation of lists in a more compact and readable manner compared to traditional for loops. Instead of using multiple lines of code to append items to a list, a list comprehension can condense this into a single line. For example, if one wants to double each number in a list:
Using list comprehensions not only reduces the amount of code but also speeds up execution since they are optimized in the background. However, while they enhance efficiency, readability should still be maintained. If the expression becomes too complex, it may be worth breaking it down into a more conventional loop for clarity.
Memory Efficiency with Generators
Generators are a powerful feature in Python that provide a way to create iterators in a memory-efficient manner. Unlike lists, which store all items in memory, a generator creates items on-the-fly. This can be crucial when dealing with large datasets, significantly lowering the memory footprint of an application. Think of a generator as a factory that produces items only when asked, rather than a warehouse storing everything at once.
To illustrate, consider the following generator function:
When invoking , it will generate numbers from 5 down to 1, each on demand. This eliminates the need to store the entire sequence in memory at once. With respect to fast loop execution, this is a game-changer as you can iterate through data efficiently without overwhelming system resources.
Optimizing with NumPy Arrays
NumPy, a powerful library for numerical computing in Python, offers significant performance enhancements over standard Python list operations when it comes to loops. NumPy arrays can perform operations on entire arrays of data in a single step, leveraging low-level optimizations that aren't available with traditional lists. This can drastically speed up computations, especially with large datasets.
For example, you can replace a nested loop operation like this:
With a NumPy operation:
Using NumPy enables vectorized operations, which leads to significant performance gains. However, one must be cautious when weighing the benefits against the learning curve that comes with understanding NumPy's principles and functionalities.
By mastering these techniques â list comprehensions, generators, and leveraging NumPy arrays â Python developers can effectively optimize their loops for better performance, facilitating faster computations and more efficient memory usage.
Alternative Loop Constructs
When discussing how to optimize loops in Python, itâs essential to explore alternative constructs available in the language. While the loop is often favored for its straightforward syntax and versatility, other looping mechanisms such as loops and recursion offer their own advantages and can be beneficial when applied appropriately. Understanding these alternatives allows developers to choose the most suitable strategy based on the specific problem at hand.
Exploring While Loops
While loops in Python give a programmer the ability to execute a block of code as long as a specified condition holds true. Unlike loops, which iterate over a sequence or collection, loops can continue indefinitely, provided the termination condition remains unmet. This quality can be particularly advantageous in scenarios where the number of iterations is not predetermined.
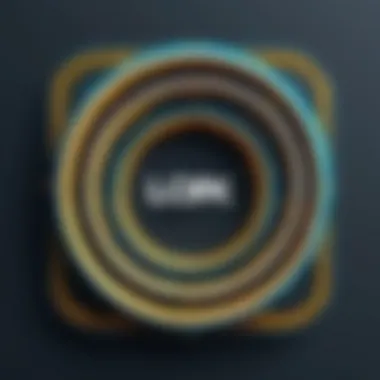
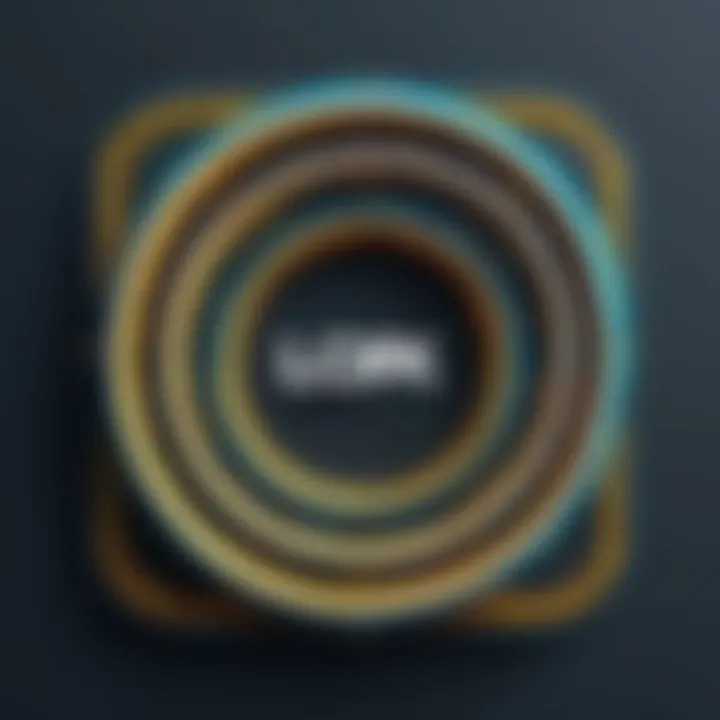
For example, consider a scenario where a program continuously processes user input until a sentinel value is reached. A loop can elegantly loop through user interactions by simply checking the input condition:
In this code, the loop will run continuously until the user types "exit." Such flexibility illustrates why loops can be a strong choice in specific applications. However, caution must be exercised to avoid infinite loops which can hinder program execution and lead to excessive resource consumption.
When to Use Recursion Instead
Recursion is another powerful construct where a function calls itself to solve smaller instances of the same problem. This method provides a clean and often elegant means of handling problems that can be broken down into subproblems, such as tree traversal or calculating factorial values.
The benefits of recursion include:
- Conciseness: Recursive solutions can often be more succinct than iterative ones, reducing code complexity and improving readability.
- Problem Decomposition: Recursion allows focusing on the current problem, deferring the handling of subproblems to subsequent function calls. This can make the logic of the solution easier to follow.
- Stack Usage: Python handles recursion through the call stack, which can lead to cleaner memory management under certain conditions.
However, itâs important to note that recursion has its downsides. Each recursive call carries a cost, both in terms of performance and memory usage. Itâs crucial to identify problems that fit the recursive model well and are not excessively deep to avoid hitting Pythonâs recursion limit.
In summary, selecting between loops and recursion depends on the problem being solved. While loops often shine through their straightforward approach to iteration, recognizing when to shift to alternatives like loops and recursion can lead to more efficient and readable code. The goal is always to match the looping construct to the taskâs needs, considering factors like readability, performance, and resource management.
Frameworks and Libraries for Enhanced Performance
When discussing the optimization of for loops in Python, the conversation often turns toward frameworks and libraries that can significantly amplify the speed and efficiency of loop execution. More than just tools in a developer's kit, these frameworks serve as force multipliers, transforming the way programmers approach performance-heavy tasks.
Using specialized libraries can reduce the need for low-level optimizations, giving developers more time to focus on logic and maintainability, rather than getting lost in the weeds of performance tuning. The bottom line is, when youâre looping through vast datasets or executing complex calculations, leveraging these frameworks can lead to tangible improvements both in speed and resource utilization.
Leveraging Cython for Speed
Cython acts as a superset of Python, blending Python syntax with C-like performance. By compiling your Python code, Cython can lead to significant speedups, especially in scenarios heavy with loops and mathematical computations. The beauty of Cython lies in its simplicity; you don't have to abandon your usual coding style. Instead, you just sprinkle a few type declarations in your code.
For instance, if you have a computationally heavy loop, you might convert it as follows:
The example illustrates how introducing Cython can lead to a more efficient loop. Notably, Cython also allows direct interfacing with C libraries, which can be a boon for high-performance applications needing a lower-level touch without completely abandoning Python.
Utilizing Multiprocessing for Parallel Execution
As data sizes burgeon and computational demands skyrocket, a single-threaded approach may fall short. This is where multiprocessing steps in as a game changer; it enables developers to allocate different processes to different cores of a CPU. This parallel execution can transform how loops are managed, allowing tasks that would typically take a single core hours to execute in a fraction of that time.
Consider the scenario where you're processing a large list of items in a for loop. Instead of running sequentially, breaking it down through multiprocessing can be as simple as this:
By utilizing a multiprocessing pool, each item can be processed in parallel, drastically reducing overall execution time. However, one must tread carefully; context switches and inter-process communication can introduce overhead, and balancing this overhead against the performance gain is crucial.
As with any optimization, the key is to measure before and after; not all tasks will benefit equally from parallelization.
Practical Examples and Case Studies
When delving into the optimization of for loops in Python, it's vital to ground theories in practical examples and case studies. Abstract concepts can sometimes feel too distant from real-world applications, which is why revealing actual cases can illuminate the depths of how loop performance can be improved. By closely examining specific scenarios, programmers can better grasp the implications of different techniques on loop optimization.
Analyzing Loop Performance in Real Scenarios
To truly understand the inner workings of loop performance, we ought to look at real-world examples. Take, for instance, a situation where a company is processing a massive dataset to generate monthly sales reports. If the existing for loops go through each data entry sequentially, the processing time can blow through the roof, resulting in delays that affect other business operations.
In one case, a data analyst realized that the nested for loops were creating a bottleneck, making the data analysis sluggish. By switching to list comprehensions, the performance saw a significant boost. Instead of iterating through the entire dataset multiple times, he managed to condense the multiple iterations into a single, more efficient list comprehension. The difference was notable â tasks that used to take several hours were reduced to mere minutes.
"Efficiency is doing the thing right. Effectiveness is doing the right thing."
â Peter Drucker
This example highlights not only the importance of optimizing for loops but also how small changes in logic can lead to considerable improvements in performance. By analyzing and assessing real scenarios like this, programmers can incorporate best practices into their codebase, ultimately leading to smarter, faster applications.
Comparative Studies of Techniques
When faced with optimizing loop performance, comparisons between various techniques can yield valuable insights. In a comparative study, two teams of developers were tasked with sorting the same dataset using traditional for loops and Python's built-in sorted function, respectively. The results were instructive.
- Traditional For Loops: The team using traditional loops took about 25 seconds to sort a dataset comprising 1 million entries, showcasing a linear relationship between size and time taken. As the dataset increased, so did the time taken by the oft-used sorting algorithm, revealing limitations in using basic for loops for large data.
- Built-in Sorting: Conversely, the other team, utilizing the built-in sorted function, achieved the same sort in under 4 seconds. This stark difference underscored the efficacy and speed of Pythonâs built-in capabilities, laying bare the power of choosing the right tools.
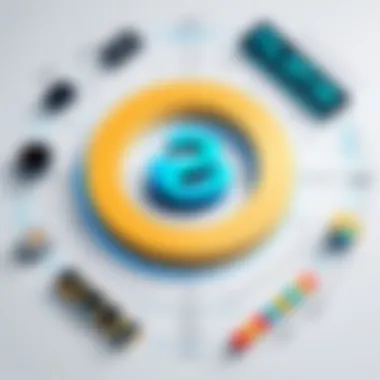
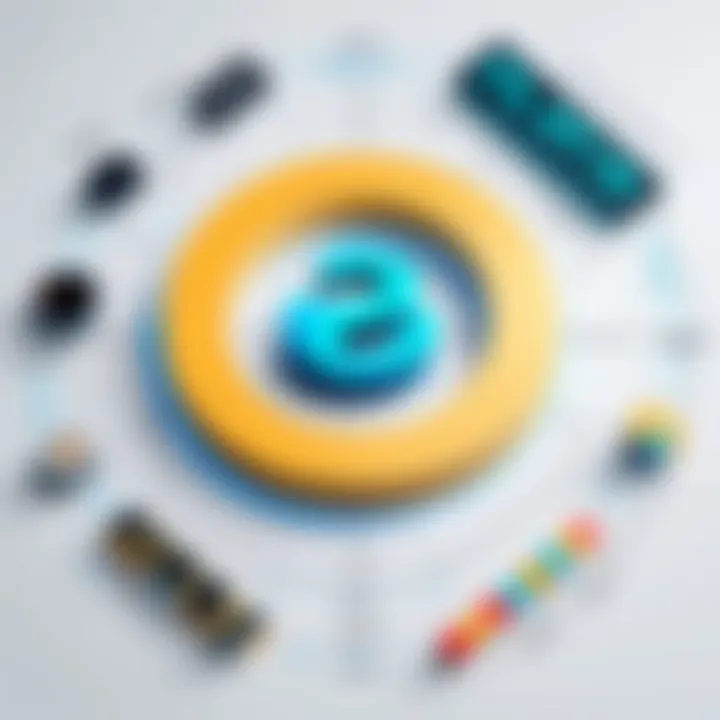
The study did not conclude here. By expanding the scope to the impact of optimizations through NumPy arrays, another round of tests illustrated further enhancements. Using NumPy, which handles array operations in a more efficient manner than standard Python lists, resulted in even faster sorting times, revealing how adopting libraries designed for performance can be a game changer.
Common Pitfalls in Loop Optimization
Optimizing loops might seem like a no-brainer for developers looking to enhance their code's performance, but there are pitfalls lurking around every corner. Understanding these common pitfalls is crucial to ensure that the efforts put into optimizing do not backfire, leading to issues down the line. Rushing into optimizations can introduce complexity that might outweigh the performance benefits, affecting the overall quality of the code.
One major consideration is the concept of over-optimization. Many developers find themselves caught in the trap of trying to squeeze every last bit of performance out of their code, which can lead to sacrifices in readability and maintainability. For example, while a clever trick using a one-liner might seem appealing, it may confuse someone unfamiliar with your thought process. Maintaining a balance between clarity and efficiency should always be a priority.
Over-Optimization and its Consequences
In today's fast-paced tech environment, itâs easy to see how over-optimization could happen. Developers often look to make their code faster by employing complex optimizations that can make the code more difficult to read and maintain. A classic example is the decision to replace simple constructs with intricate algorithms that, while they might work slightly faster, create a jigsaw puzzle for future developers.
- Trade-offs in Readability: If the code becomes so convoluted that itâs hard to follow, those supposed speed gains can be eclipsed by the time needed for debugging and maintenance.
- Performance Gains Not Worth the Cost: In some cases, the improvements may be marginalâperhaps a reduction from 0.001 seconds to 0.0005 seconds. Is that really worth the additional complexity? Most of the time, performance gains in loops should be obvious, but hidden trade-offs can easily derail the projectâs success.
Itâs also important to test the optimizations thoroughly. Relying on theoretical advantages can lead to disappointing results when put to the test. Developers should focus on areas of real concern and measure the actual impact of their optimizations rather than just guessing.
Understanding Readability versus Performance Trade-offs
This leads us to the next pitfall: the often uneasy tension between readability and performance. Every developer faces this struggle at some point in their careers. Should you write clear and straightforward for loops, or go for the more complex route that boasts higher performance?
- Clarity is Key: Writing simple loops that are easy to read often means less time is spent in documentation and onboarding new team members. A readable codebase tends to be more maintainable in the long run.
- Performance Priorities: While itâs essential to care about performance, it might not always be the chief concern. For instance, if youâre only running a loop once or twice, the speed difference wonât matter much, compared to if that loop runs thousands of times in a critical path.
Key takeaway: Balance between performance and readability is essential to avoid over-complicating your code and introducing potential bugs.
Best Practices in Writing Efficient Loops
Writing efficient loops is like crafting the perfect dish; you need the right ingredients and techniques to ensure it tastes just right. In programming, loops are fundamental components, allowing developers to execute code repeatedly until a certain condition is met. But with great power comes great responsibility. If not handled properly, loops can turn into a performance drain that bogs down your entire codebase.
Understanding the best practices in writing efficient loops can lead to code that is both performant and maintainable. This section dives into specific elements that can profoundly impact loop efficiency, including code structure, memory management, and readability. Developers, be it beginners or veterans, can benefit from these insights by refining their approach to loop optimization. Here are some core considerations to keep in mind:
- Choose the Right Loop Construct: Depending on the task at hand, different loops serve different purposes. For instance, loops excel in iterating over collections, while loops are superb for indefinite iterations. Understanding these nuances can help streamline your code.
- Avoiding Excessive Nesting: Deeply nested loops can be performance killers. If you find your loops layered like a well-frosted cake, consider ways to flatten them or simplify the logic.
- Minimize Work Inside the Loop: Each operation inside a loop takes time. Aim to move any unnecessary calculations or function calls outside. Picture it like a runner determining the most efficient pathâevery extra step counts.
- Use Built-in Functions and Libraries: Pythonâs built-in functions, such as and , are often more efficient than hand-written loops. They leverage optimized C underpinnings and can provide performance benefits.
Incorporating these best practices not only enhances performance but also leads to clearer, more maintainable code, paving the way for smoother future adaptations and enhancements.
Code Refactoring Techniques
Code refactoring techniques are essential tools in a programmer's toolkit. They allow developers to improve code structure without altering its external behavior. This is particularly valuable when working with loops, as refactoring can lead to more efficient execution and clearer code. One common approach is using list comprehensions instead of traditional loops, effectively collapsing multiple lines of looping logic into a single line while maintaining clarityâand often improving performance.
When aiming for optimization, consider the following refactoring techniques:
- Extract Method: If you find yourself repeating the same chunk of code within a loop, extract that code into a separate function. This encourages reusability and enhances readability.
- Use Early Exit Strategies: Instead of checking the conditions late in the loop, check them early. This can save processing time by skipping unnecessary iterations.
Ultimately, the goal of refactoring is not just performance but also clarity. Well-structured code makes life easier for you and others who will read it later.
Evaluating Performance with Timeit Module
To fine-tune your loops and ensure they perform efficiently, the module is an invaluable ally. This built-in module evaluates the execution time of snippets, making it straightforward to discern which approach is faster.
Using is as simple as pie. The following code illustrates how to benchmark two different loop implementations:
By leveraging the module, developers can assess not just which loop is faster but also how different refactoring techniques stack up against each other. This quantifiable approach to performance evaluation empowers programmers to make informed decisions about which methods to employ.
"Good code is its own best documentation." â This applies particularly in the context of optimizing loops. By adhering to best practices, your loops can become a delicate dance of efficiency, readability, and performance.
End and Future Directions
The discussion surrounding fast for loops holds significant weight, especially in a programming landscape where performance often dictates the success of applications. The culmination of this article invites the reader to appreciate not only the methods for optimizing loops but to also understand their broader implications on software development. As we wrap up our exploration of for loops in Python, itâs important to note that efficiency is not just about speed; it's equally about clarity, maintainability, and sustainability of code.
The benefits gained from efficient loop usage extend beyond the micro-level. When developers harness these techniques, they contribute to a larger codebase that's easier to understand and manage. This fosters collaboration among team members and allows for quicker iterations and debugging processes. Moreover, with the growing complexity of applications, future developments in Python may introduce even more tools and methodologies to enhance loop performance, ensuring that programmers are always equipped to tackle new challenges.
Recap of Key Insights
- Essential Techniques: We have discussed an array of strategies for enhancing loop efficiency, including the implementation of list comprehensions, memory-efficient generators, and the powerful capabilities of NumPy arrays. Each method offers unique strengths that can be leveraged depending on the specific needs of a project.
- Performance Analysis: Understanding the time complexity associated with different looping constructs cannot be overstated. Recognizing how these factors interplay helps in identifying bottlenecks and finding optimal solutions in real-world scenarios.
- Balance Between Readability and Performance: A critical takeaway is the notion that while performance optimization is crucial, it should never come at the cost of code readability. Striking a balance between these two aspects assists developers in maintaining a high standard of quality in their programming practices.
- Future-Oriented Approach: Looking forward, innovations such as advancements in libraries and frameworks, along with trends in parallel execution, promise to further evolve the capabilities of Python's looping constructs.
Innovations on the Horizon
The horizon for optimizing loops in Python is abuzz with potential advancements. As programming paradigms continuously shift, both the language itself and the underlying technology are evolving.
- Dynamic Libraries: Future iterations of Python may see richer APIs focused on performance tuning, making it easier for developers to write highly efficient loops without delving too deep into complex optimization techniques.
- Machine Learning Integration: With the rise of AI and machine learning, thereâs a trend toward developing algorithms that favor speed and efficiency. Developers will likely see tools that automatically suggest or implement optimizations based on usage patterns and performance metrics.
- Enhanced Parallel Processing: As computing power increases, techniques such as multiprocessing and distributed computing may become even more integrated into Python, granting developers the ability to manage loops across multiple cores and machines with minimal friction.
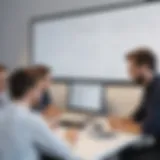
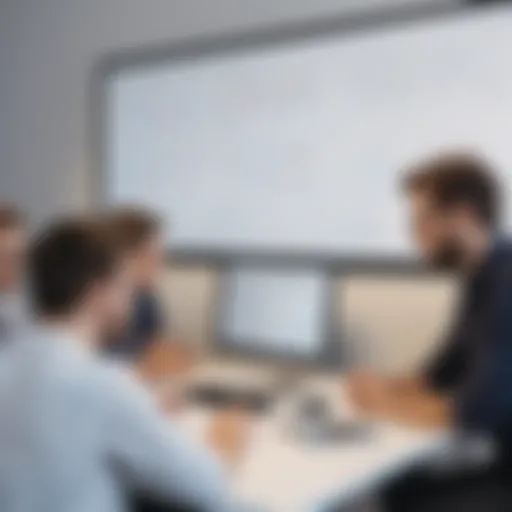