Unraveling the Core Principles of Data Structures and Algorithms
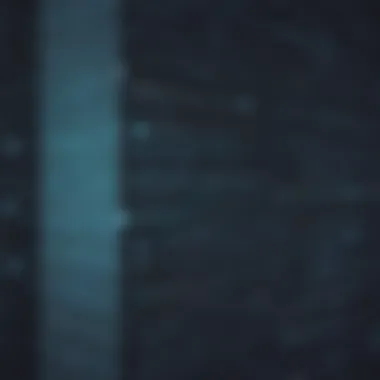
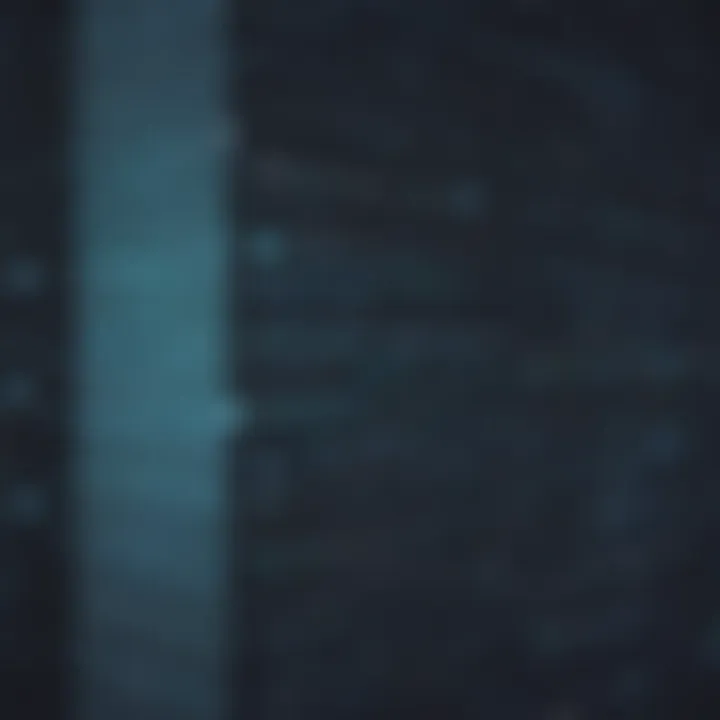
Coding Challenges
Data structures and algorithms are the bedrock of computer science, driving the efficient organization and manipulation of data. Aspiring and seasoned programmers alike understand the significance of mastering these fundamentals. To hone their skills and challenge themselves, many participate in weekly coding challenges. These challenges not only test problem-solving abilities but also provide a platform to showcase innovative solutions.
Weekly Coding Challenges
Participating in weekly coding challenges offers programmers the opportunity to engage with diverse problem sets, sharpen algorithmic thinking, and enhance code efficiency. It fosters a sense of healthy competition and camaraderie among peers, pushing individuals to explore creative solutions to complex problems.
Problem Solutions and Explanations
After each coding challenge, the community often comes together to dissect the problems, share their approaches, and discuss the efficiency of various solutions. This collaborative environment promotes a deeper understanding of different problem-solving techniques, enriching the learning experience for all participants.
Tips and Strategies for Coding Challenges
Navigating coding challenges can be daunting, especially for beginners. Experienced programmers often share invaluable tips and strategies to help newcomers approach problems systematically, manage their time effectively, and optimize their code for better performance.
Community Participation Highlights
The coding community thrives on collaboration and knowledge sharing. Highlighting community members who exhibit exceptional problem-solving skills or contribute significantly to the collective learning experience not only inspires others but also fosters a sense of community pride and appreciation.
Introduction
Data structures and algorithms form the bedrock of computer science, underpinning the very essence of efficient data organization and computational processes. In this article, we unravel the intricacies of these fundamental concepts, providing a comprehensive guide suitable for both aspiring programmers and seasoned IT professionals. By delving into the core principles of data structures and algorithms, we aim to equip our readers with a profound understanding of these crucial elements that drive innovation and problem-solving in the digital realm.
Understanding Data Structures
Diving into Arrays and Linked Lists
When we discuss the realm of data structuring, delving into the realm of arrays and linked lists becomes imperative. Arrays present a structured way to store elements of the same data type, offering efficient access and manipulation. On the other hand, linked lists provide dynamic memory allocation, facilitating seamless data insertion and deletion. The key characteristic of arrays lies in their contiguous memory allocation, enabling fast random access, while linked lists excel in flexibility and efficient memory usage. While arrays boast simplicity and constant-time access, linked lists shine in dynamic sizing and easy element removal.
Exploring Stacks and Queues
Moving further, the exploration of stacks and queues sheds light on essential data structuring paradigms. Stacks follow the Last In, First Out (LIFO) principle, ideal for function call management and backtracking scenarios. On the contrary, queues adhere to the First In, First Out (FIFO) discipline, crucial for tasks like process scheduling and breadth-first search algorithms. Stacks offer simplicity and quick operations like push and pop, while queues provide orderly processing and enqueue-dequeue functionality. Stacks aid in recursive algorithms and depth-first search, whereas queues support printer task management and level-order traversals.
Introducing Trees and Graphs
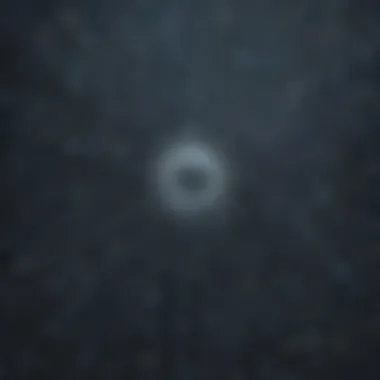
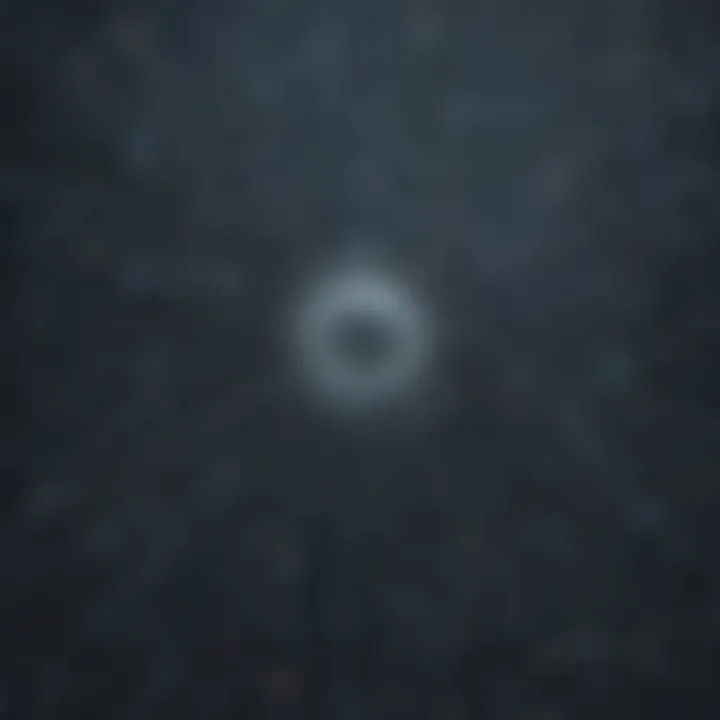
As we segue into the realm of trees and graphs, a sophisticated world of interconnected data structures unveils. Trees, with nodes and edges linked in a hierarchical manner, bring order and quick search functionality to data representation. Graphs, on the other hand, offer versatile representations of relational data, essential for problems like social network analysis and GPS routing. Trees provide efficient searching and sorting mechanisms, while graphs excel in modeling complex relationships and pathfinding algorithms. Trees present balanced and unbalanced variations affecting search efficiency, whereas graphs come in directed and undirected forms catering to diverse connectivity needs.
Basics of Algorithms
Algorithm Analysis and Complexity
The foundation of algorithms lies in robust analysis and complexity understanding, critical for evaluating computational efficiency. Algorithm analysis involves assessing time and space complexities, crucial for gauging performance and scalability. Complexity classes like O(1), O(n), and O(log n) dictate algorithmic behavior concerning input size. Understanding complexity aids in algorithm selection for optimal performance tuning, balancing time and space trade-offs. While lower complexity signifies better efficiency, higher complexities entail resource-intensive computations.
Sorting and Searching Algorithms
Sorting and searching algorithms stand as pillars of algorithmic design, enriching data manipulation and retrieval operations. Sorting algorithms like bubble sort, merge sort, and quicksort arrange data elements in ascending or descending order, optimizing search efficiency. Searching algorithms like linear search and binary search enable quick data retrieval based on key comparison operations. Sorting facilitates data organization for expedited search, while searching algorithms pinpoint specific data items swiftly. Selecting the right sorting or searching algorithm hinges on the data volume, structure, and operational requirements.
Recursion and Backtracking
The recursive nature of algorithms embodies a powerful problem-solving approach, maneuvering diverse computational challenges with ease. Recursion involves defining a problem self-referentially, breaking it into smaller subproblems until a base condition is met. Backtracking complements recursion in exploring and pruning the search space, essential for constraint satisfaction problems and combinatorial optimization. Recursion simplifies complex problems into manageable steps, promoting code readability and logical structure. Backtracking navigates solution spaces smartly, eliminating invalid paths efficiently. Leveraging recursion and backtracking optimally demands a deep grasp of problem decomposition and solution enumeration techniques.
Advanced Concepts
In the realm of data structures and algorithms, the section dedicated to Advanced Concepts stands as a pivotal component for in-depth understanding. These sophisticated concepts delve into complex problem-solving strategies and optimization techniques that elevate programming proficiency to new heights. By exploring topics like Dynamic Programming, Graph Algorithms, and Specialized Data Structures, readers can broaden their computational horizons and bolster their analytical skills. Advanced Concepts serve as the cornerstone for pushing the boundaries of traditional algorithms and opening doors to innovative solutions.
Dynamic Programming
Memoization and Tabulation
Dynamic Programming in the context of Memoization and Tabulation embodies a strategic approach to optimize computational efficiency. Memoization involves storing previously computed results to alleviate redundant calculations, while Tabulation focuses on building a table of solutions to subproblems for swift access. The essence of Memoization lies in its ability to reduce time complexity by memorizing intermediate solutions, contributing to faster algorithm execution. Conversely, Tabulation proffers a bottom-up solution strategy that promotes systematic problem-solving through organized data structures. This dynamic duo of Memoization and Tabulation empowers programmers to tackle complex problems with elegance and precision, making them indispensable tools for honing algorithmic prowess in this comprehensive guide.
Optimization Techniques
Optimization Techniques encapsulate a range of strategies aimed at enhancing algorithm performance and streamlining computational processes. By fine-tuning code structures, reducing redundant operations, and optimizing resource utilization, programmers can significantly boost program efficiency and functionality. The crux of Optimization Techniques lies in their capacity to identify bottlenecks, rectify inefficiencies, and transform algorithms into sleek, high-performing routines. This section delves into the nuances of optimization, elucidating how savvy programming practices can revolutionize algorithmic efficiency and elevate software development standards.
Graph Algorithms
Shortest Path Algorithms
Navigating through the intricate web of Graph Algorithms unveils the significance of Shortest Path Algorithms in optimizing traversal and route planning. These algorithms prioritize finding the most efficient path between nodes, minimizing distance or cost to expedite navigation processes. Their innate ability to compute optimal routes swiftly and accurately makes them indispensable in a variety of applications, ranging from GPS systems to network optimization. By delving into the mechanics of Shortest Path Algorithms, programmers can unveil a world of algorithmic optimization that reshapes computational paradigms.
Minimum Spanning Tree
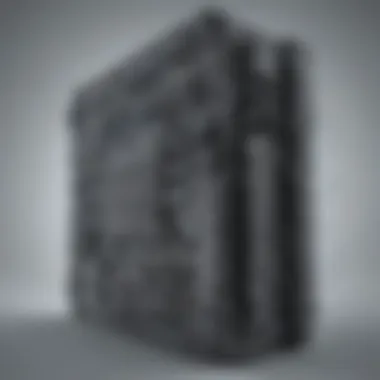
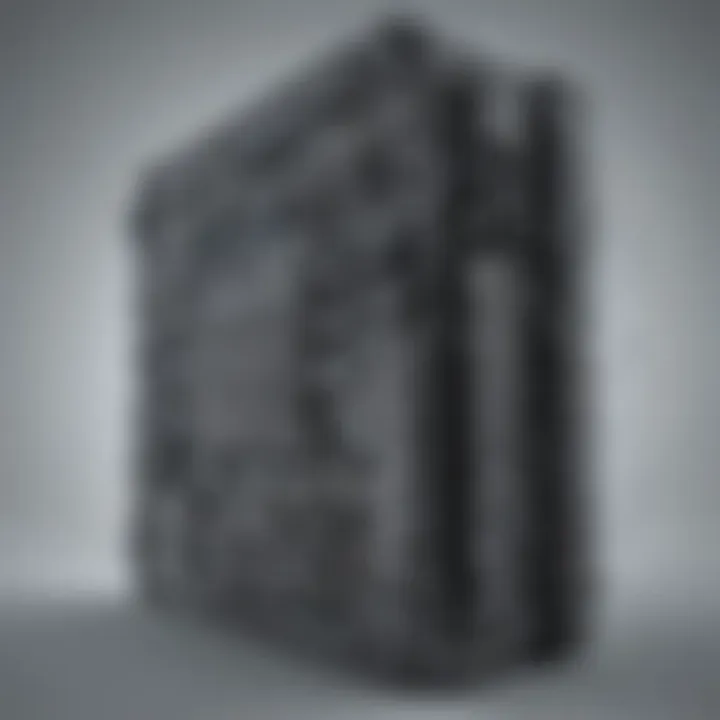
The concept of Minimum Spanning Trees represents a fundamental aspect of Graph Algorithms, emphasizing the importance of establishing efficient connections within a network. By identifying the most cost-effective method to link nodes without forming cycles, Minimum Spanning Trees facilitate streamlined data transmission and network connectivity. Their role in optimizing network design and resource allocation underscores their relevance in modern computing landscapes. Exploring the foundations of Minimum Spanning Trees illuminates the intricate balance between connectivity and efficiency, providing insights into strategic network optimization strategies.
Specialized Data Structures
Heaps and Priority Queues
Heaps and Priority Queues epitomize the essence of efficient data organization and priority-based operations. Featuring key characteristics such as logarithmic search complexity and optimal element retrieval, Heaps and Priority Queues offer swift data access and manipulation capabilities. Their distinctive feature lies in maintaining the highest or lowest priority element at the forefront, ensuring rapid processing of critical information. By integrating Heaps and Priority Queues into algorithmic frameworks, programmers can enhance sorting, scheduling, and prioritization tasks with unparalleled efficiency, augmenting the performance of data-intensive applications.
Disjoint-Set Data Structure
The Disjoint-Set Data Structure serves as a foundational construct for managing dynamic connectivity relationships in various algorithmic scenarios. By categorizing elements into disjoint sets and facilitating efficient union and find operations, this structure enables cohesive data management and expedited connectivity queries. Its unique feature lies in its ability to represent connectivity components while offering swift access to critical connectivity information. Leveraging the Disjoint-Set Data Structure in algorithmic implementations enhances data organization and streamlines connectivity determinations, making it a valuable asset in optimizing computational workflows.
Applications in Real-World Scenarios
In the realm of computer science, the significance of real-world applications cannot be overstated. This section delves into how the theoretical constructs of data structures and algorithms manifest in practical settings, shaping various industries and technological advancements. Understanding the practical utility of these fundamentals is crucial for aspiring programmers and seasoned professionals alike. By exploring real-world scenarios, readers gain insights into the pragmatic implementations of abstract concepts, enabling them to bridge the gap between theory and practice.
Database Management Systems
Indexing and Search Optimization
Within the domain of database management systems, Indexing and Search Optimization emerges as a pivotal component. This subsection focuses on the intricate process of indexing data to expedite search operations, enhancing database efficiency and performance. The key characteristic of Indexing and Search Optimization lies in its ability to streamline data retrieval by creating structured indices, facilitating swift query executions. Despite its advantages in accelerating search processes, Indexing and Search Optimization may entail drawbacks such as increased storage requirements and overhead costs. Understanding the nuances of this approach is vital for optimizing database operations and refining data accessibility.
Query Processing
Moreover, Query Processing plays a crucial role in database management systems, enabling users to retrieve and manipulate data effectively. This segment delves into the complexities of query optimization, where queries are parsed and executed to yield precise results while minimizing resource consumption. The essence of Query Processing lies in its capacity to interpret user commands and transform them into actionable database operations, contributing to streamlined data retrieval and manipulation. Although Query Processing enhances query efficiency, it may also introduce computational overhead, necessitating careful consideration in balancing performance and resource utilization.
Network Routing Algorithms
Dijkstra's Algorithm
When discussing network routing algorithms, Dijkstra's Algorithm emerges as a fundamental tool for finding the shortest path between nodes in a graph. Its key characteristic lies in its optimality, guaranteeing the shortest path in weighted graphs, making it a preferred choice for routing applications. Dijkstra's Algorithm's unique feature lies in its ability to iteratively explore and update the shortest path to each node, ensuring efficiency and accuracy in path determination. While celebrated for its effectiveness in pathfinding, Dijkstra's Algorithm may encounter limitations in cases of negative edge weights, necessitating alternative algorithms for certain scenarios.
Bellman-Ford Algorithm
Conversely, the Bellman-Ford Algorithm serves as another essential network routing algorithm, capable of addressing scenarios with negative edge weights. Its key characteristic includes the ability to handle negative weight cycles, providing a versatile solution for diverse graph structures. The unique feature of the Bellman-Ford Algorithm lies in its iterative relaxation process, which detects and mitigates negative cycles, ensuring robust path computation even in complex network topologies. While offering flexibility in handling negative edge weights, the Bellman-Ford Algorithm may exhibit higher computational complexity in comparison to other pathfinding algorithms.
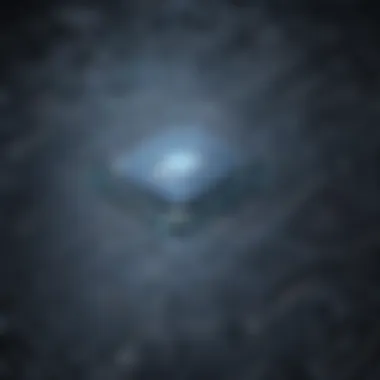
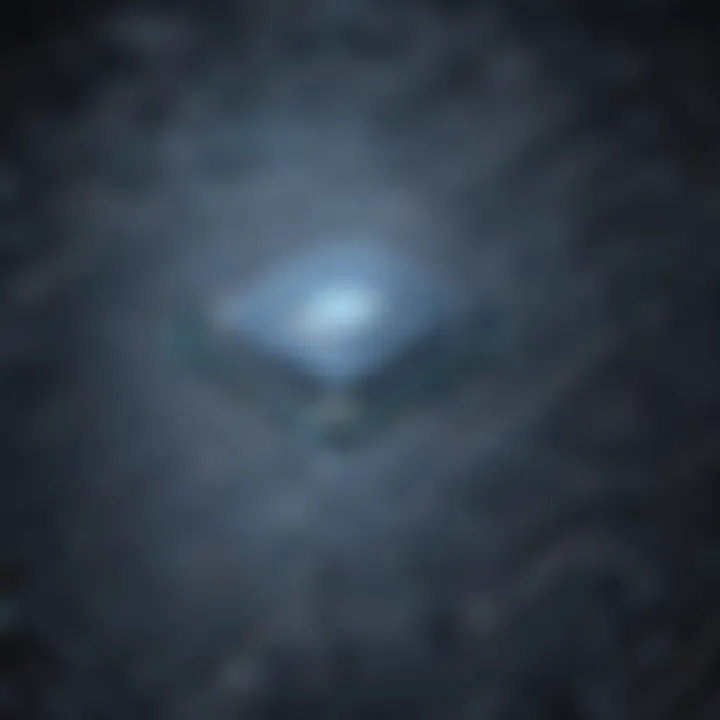
Machine Learning and AI
Neural Network Training
In the realm of machine learning and artificial intelligence, Neural Network Training stands out as a core process for model development and optimization. This subsection explores the training paradigm that empowers neural networks to learn complex patterns and relationships within data. The key characteristic of Neural Network Training is its iterative adjustment of model weights based on error minimization, facilitating convergence towards accurate predictions. The unique feature of this process lies in its adaptability to diverse datasets and problem domains, showcasing its versatility in tackling a myriad of machine learning tasks. While Neural Network Training excels in capturing intricate patterns, it may encounter challenges related to overfitting and computational resource demands, underscoring the importance of model regularization and optimization techniques.
Genetic Algorithms
Additionally, Genetic Algorithms represent a powerful optimization approach inspired by biological evolution processes. This segment delves into the evolutionary principles underpinning Genetic Algorithms, where candidate solutions evolve over generations towards optimal outcomes. The key characteristic of Genetic Algorithms lies in their population-based search methodology, exploring solution spaces through crossover and mutation operations to discover robust solutions. The unique feature of Genetic Algorithms lies in their ability to handle complex, multimodal optimization problems that traditional algorithms may struggle to address. While offering robust optimization capabilities, Genetic Algorithms may require fine-tuning of parameters and control mechanisms to balance exploration and exploitation, ensuring effective convergence towards high-quality solutions.
Challenges and Best Practices
In the realm of data structures and algorithms, delving into challenges and best practices is paramount for honing one's skills and fostering a deep understanding of computational processes. By scrutinizing the obstacles that may arise during algorithmic design and implementation, individuals can fortify their problem-solving abilities and enhance the efficiency of their solutions. Moreover, embracing best practices not only streamlines the development process but also cultivates a mindset of optimization and continuous improvement. Consequently, by exploring the nuances of challenges and best practices, learners and professionals can navigate the intricate landscape of data structures and algorithms with acumen and finesse.
Optimizing Algorithm Performance
Space and Time Complexity
Grasping the intricacies of space and time complexity within algorithm analysis is instrumental in assessing the efficiency and scalability of computational solutions. Space complexity pertains to the amount of memory an algorithm requires to solve a problem, while time complexity focuses on the computational resources needed for algorithm execution. Understanding these metrics enables developers to gauge the performance of their algorithms across varying input sizes and optimize codebase for enhanced efficiency. By evaluating space and time complexity, programmers can fine-tune their solutions to achieve optimal performance and resource utilization, aligning with the overarching goal of efficient data manipulation and processing.
Code Optimization Techniques
Code optimization techniques play a pivotal role in refining algorithmic implementations to operate at peak performance levels. By employing strategies such as loop unrolling, algorithmic restructuring, and memory management, developers can enhance the efficiency and speed of their code execution. These techniques not only eliminate redundant operations and improve code readability but also contribute to the scalability and maintainability of software systems. Embracing code optimization practices empowers programmers to fine-tune their algorithms for superior performance, elevating the quality and efficacy of their computational solutions.
Maintaining Scalability
Load Balancing Strategies
In the context of data processing and system design, load balancing strategies are essential for equitable resource allocation and optimal performance distribution. By evenly distributing workloads across multiple servers or computing nodes, load balancing mitigates server overload and enhances system resilience to fluctuations in user traffic. With load balancing mechanisms in place, organizations can ensure efficient utilization of resources, expedite task execution, and deliver seamless user experiences. By implementing robust load balancing strategies, enterprises can achieve scalability and reliability in data processing and computational tasks.
Scaling Data Structures
Scaling data structures involves optimizing data organization and storage mechanisms to accommodate growing datasets and evolving computational requirements. By selecting appropriate data structures such as dynamic arrays, hash tables, or trees, developers can effectively manage data volume and facilitate quick retrieval and manipulation operations. Scalable data structures foster efficient data processing and retrieval, ensuring optimal performance and responsiveness in complex computational environments. Embracing scalable data structure implementations equips programmers with the tools to address increasing data demands and sustain high-performance levels across diverse applications.
Debugging and Testing
Unit Testing Algorithms
Unit testing algorithms is a crucial step in validating the correctness and functionality of individual algorithmic components. By isolating and testing specific units of code, developers can detect and rectify errors or bugs early in the development cycle, ensuring the robustness and reliability of their algorithms. Unit testing promotes code stability, fosters code reusability, and facilitates seamless integration of algorithmic modules within larger software systems. Embracing unit testing practices empowers programmers to identify and address algorithmic flaws promptly, enhancing the overall quality and maintainability of their computational solutions.
Identifying Edge Cases
Identifying edge cases involves scrutinizing the extreme or atypical scenarios that may impact algorithm performance and output accuracy. By evaluating boundary conditions, exceptional inputs, and outlier scenarios, developers can fortify their algorithms against unexpected behaviors or inaccuracies, enhancing the robustness and reliability of their solutions. Addressing edge cases proactively ensures algorithmic functionality across diverse input domains and usage scenarios, bolstering the resilience and effectiveness of computational processes. By adeptly identifying and handling edge cases, programmers can fortify their algorithms for a comprehensive range of computational challenges and complexities.