Building a GUI in Python: A Comprehensive Guide
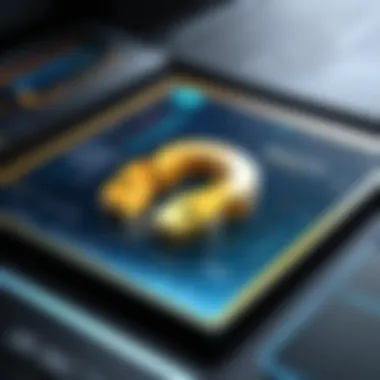
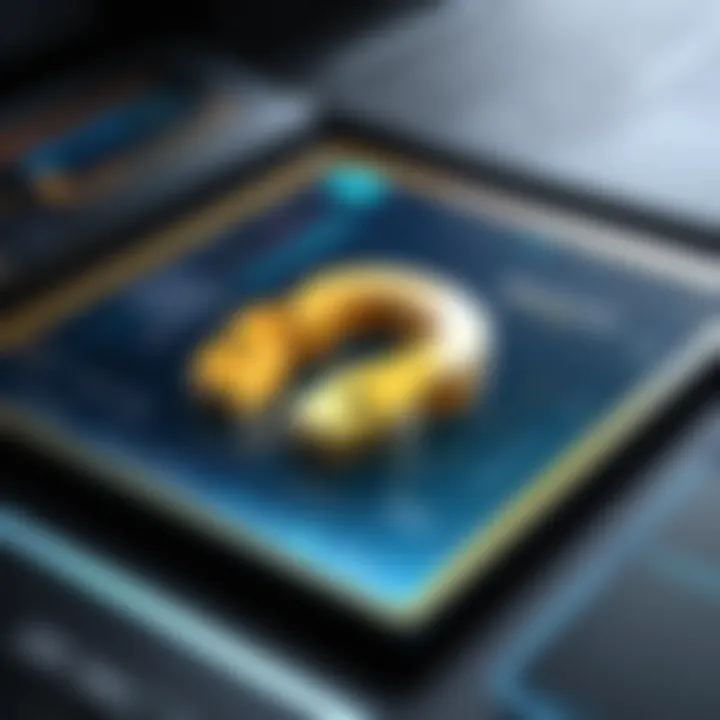
Intro
In the evolution of software development, the significance of graphical user interfaces (GUIs) cannot be understated. They serve as the bridge between complex coding and user experience, allowing non-technical individuals to interact with technology seamlessly. This article delves into the realm of building GUIs in Python, reflecting on various frameworks and best practices that can help you master this essential skill. Our exploration will not only cover the technical aspects but also highlight strategies that cater to both beginners and those seeking to deepen their understanding.
Learning Outcomes
By the end of this guide, readers will gain insights into:
- Popular frameworks for GUI development in Python
- Best practices for designing user interfaces that are both functional and visually appealing
- Practical applications that highlight the importance and versatility of GUIs
The journey into GUI development can be daunting, yet it promises rewarding experiences. Let’s begin by navigating through coding challenges that often confront developers in this space.
Understanding GUIs and Their Importance
Graphical User Interfaces, or GUIs, play a critical role in modern computing. They provide the vital link between users and software applications, translating complex commands into intuitive visual elements. The ease with which users interact with a software program directly affects its adoption and usability. Therefore, understanding GUIs is essential for anyone involved in software development, from programmers to graphic designers. This article delves into the various aspects of GUIs, outlining their definition, roles, and significance in software design.
Definition of a GUI
A Graphical User Interface is a visual way for users to interact with a computer or software application. Unlike command-line interfaces, which require text-based commands, GUIs use graphical elements such as windows, icons, buttons, and menus. This structure allows users to engage with the interface more seamlessly, as they can manipulate visual objects rather than memorizing commands. GUIs are designed to be user-friendly, aiming to minimize the learning curve associated with new software.
Role of GUIs in Software Applications
GUIs are integral to enhancing user experience. They serve multiple roles, including the following:
- Improving Interaction: Users can interact easily with applications through graphical elements, enhancing engagement.
- Facilitating Learning: A well-designed GUI reduces the time required for new users to become proficient with software.
- Promoting Functionality: GUIs can showcase the features of applications clearly, helping users understand their capabilities.
- Encouraging Consistency: Standardized graphical elements allow users to transfer their knowledge from one application to another.
Overall, GUIs not only improve user satisfaction but also increase the efficiency of software usage. As a result, understanding their design and implementation is paramount for developers aiming to create applications that meet user needs effectively.
Overview of Python for GUI Development
In this section, we explore Python's position as a language for developing graphical user interfaces (GUIs). Python has gained enormous traction in programming circles, not only for its simplicity but also for its versatility. GUI development is vital as it enhances user interaction and experience. Therefore, understanding Python's role in this domain is crucial for any developer looking to create impactful applications.
Suitability of Python for GUIs
Python's suitability for GUI development lies in its clear syntax and large community support. These aspects make it easier for both beginner and experienced programmers to create user-friendly interfaces. Frameworks like Tkinter, PyQt, and Kivy offer a diverse range of functionalities tailored for different applications.
Another key factor is Python's cross-platform capabilities. A Python-based GUI application can run on various operating systems, such as Windows, macOS, and Linux, without requiring major adjustments to the codebase. This flexibility can save developers a significant amount of time and resources.
In addition, Python's extensive libraries facilitate integration with other technologies, streamlining the development process of complex software systems. This versatility also encourages the building of prototypes quickly, enabling iterative design and testing, which are core practices of effective software development.
Key Features of Python
Python offers several features that are beneficial for GUI development:
- Readable Syntax: The clear and readable syntax makes it easy to learn and maintain code, which is essential for GUI development.
- Rich Libraries: Python has access to a vast ecosystem of libraries, such as NumPy for calculations and Matplotlib for plotting, that can enhance the functionality of GUIs.
- Event-Driven Programming: Python supports event-driven programming, which is fundamental in GUI development. Developers can easily handle user actions or events like clicks and key presses.
- Community Support: An active community means that developers can find extensive documentation, tutorials, and forums that can help resolve issues during development.
"Python is a great choice for those looking to create robust and engaging user interfaces, given its flexibility and ease of use."
By leveraging these advantages, Python developers can create GUIs that not only fulfill functionality requirements but also provide an engaging user experience. The next sections will delve deeper into the specific frameworks available in Python for GUI development.
Popular Python GUI Frameworks
When it comes to GUI development in Python, selecting the right framework is pivotal. The chosen framework can significantly influence the application's functionality, responsiveness, and user experience. Each framework offers unique features, strengths, and trade-offs. Therefore, a deep dive into popular Python GUI frameworks is essential for any programmer looking to build efficient and aesthetically pleasing user interfaces.
Tkinter: The Standard GUI Toolkit
Tkinter is often lauded as the de facto standard for GUI development in Python. Included with most Python installations, its primary strength lies in its ease of use and accessibility. For beginners, it provides a straightforward way to create windows, buttons, and other GUI elements without extensive knowledge of the underlying API. Moreover, Tkinter supports several widgets such as menus, buttons, and text fields, making it versatile for a range of applications.
One notable aspect of Tkinter is its lightweight nature. Given its smaller footprint, it consumes fewer system resources compared to other frameworks. Therefore, applications built with Tkinter often load quickly and run smoothly on various platforms. However, it should be noted that while Tkinter is capable of creating decent GUIs, its design is somewhat basic and may lack the polish that more advanced frameworks provide.
PyQt: A Comprehensive Framework
PyQt, an interface for the Qt application framework, is a robust option for developers seeking a feature-rich environment. It allows for the creation of sophisticated applications with complex interfaces. PyQt excels in providing advanced components, making it suitable for both desktop applications and data visualization tools. Its rich set of widgets, layout options, and the ability to manage multiple windows adds to its appeal.
However, PyQt comes with a steep learning curve, especially for those new to GUI programming. The extensive documentation and tutorials available can help mitigate some of these challenges. Importantly, applications built with PyQt have a professional and modern appearance. This framework is well-suited for developers aiming to create polished and visually appealing applications.
Kivy: For Multi-Touch Applications
Kivy is an open-source Python library that focuses on multi-touch user interfaces. It is particularly useful for applications that interact with touch devices such as smartphones and tablets. Kivy provides a unique layout system that accommodates touch gestures, a feature beneficial for modern applications looking to leverage mobile technology.
Along with its support for multi-touch, Kivy is designed to run on Windows, macOS, Linux, Android, and iOS. This cross-platform capability is advantageous for developers wishing to create applications that reach a wide range of users. Nonetheless, Kivy may not be the best choice for traditional desktop applications, as its design principles primarily cater to touch-based interfaces.
wxPython: A Native Look and Feel
wxPython offers an alternative for developers who desire native applications across different platforms. It wraps the native GUI components of operating systems, allowing developers to create applications that integrate seamlessly with the host platform's look and feel. This results in a more natural user experience, as it adheres to the native standards of interface design.
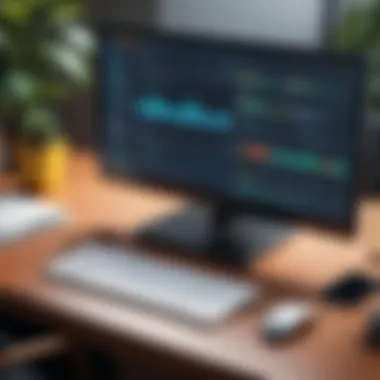
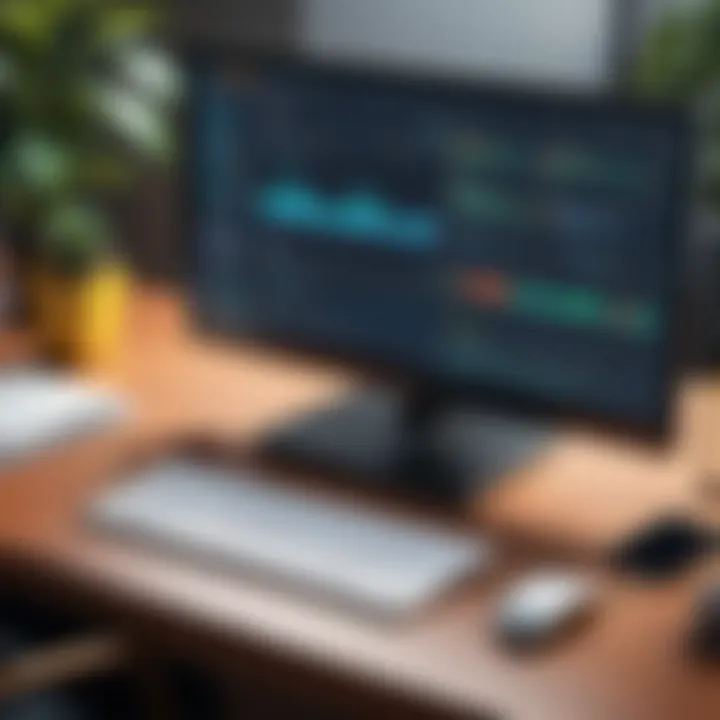
One of wxPython's strengths is its comprehensive documentation and community support. Developers can find numerous resources and examples, easing the learning process. Moreover, wxPython supports various features like print functionality, clipboard access, and more, facilitating the development of comprehensive applications. While it has a slightly more complex setup process compared to Tkinter, the benefits in terms of usability and aesthetics can be quite significant.
Initial Setup for GUI Development
The initial setup for GUI development is often overlooked, yet it lays the foundation for the effectiveness and efficiency of your projects. This step is crucial as it determines how easily you can implement and enhance your graphical user interface. Proper setup helps prevent future complications that may arise during development, ensuring that you can focus more on creating value rather than troubleshooting basic issues. This section will delve into the essential elements of setup, including library installation and environment configuration.
Installing Required Libraries
Installing the right libraries is an indispensable part of setting up your GUI development environment in Python. Various frameworks such as Tkinter, PyQt, and Kivy require specific libraries that enhance their functionality. For instance, Tkinter comes pre-installed with Python, making it quite accessible for newcomers. On the other hand, libraries like PyQt5 or Kivy need to be installed separately using package management tools such as pip.
To install these libraries, you can use pip commands in your terminal or command prompt. Here’s how you can do it for PyQt5:
Similarly, for Kivy:
Having the necessary libraries not only equips you with the tools needed but also keeps your project adaptable. You can expand your GUI with more functionalities as needed, enhancing user experience without substantial overhead.
Environment Configuration
Once you have installed the necessary libraries, the next step is to configure your development environment. A well-structured environment promotes good practices and can significantly impact productivity. This involves setting up your code editor, such as Visual Studio Code or PyCharm, to work fluidly with your libraries.
Consider configuring your project structure, too. Typically, you would want to separate your code files, resource files, and other assets into distinct directories. This organization aids in easy navigation and maintenance of your project. A simple directory structure might look like this:
- project_folder/
- main.py
- resources/
- assets/
Additionally, environment variables can streamline your development. They can store sensitive information or default configurations, making your code more secure and portable. Remember, the right setup leads to a smoother development experience, allowing you to focus on crafting intuitive user interfaces.
Proper initial setup can drastically reduce time spent on troubleshooting during later stages of development.
Fundamental Concepts in GUI Design
Understanding the fundamental concepts in GUI design is crucial for anyone involved in developing graphical user interfaces. These concepts serve as the backbone for creating applications that are not only functional but also intuitive for users. A well-designed GUI can enhance usability and improve overall user satisfaction, which is vital in friendly and efficient software applications.
Widgets and Their Types
Widgets are the building blocks of any GUI. They are the elements users interact with, such as buttons, text boxes, and sliders. Each widget serves a specific purpose and contributes to the application's interactivity. Common widget types include:
- Buttons: Trigger actions or commands when clicked.
- Labels: Display static text or images to inform the user.
- Text Entries: Allow users to input text data.
- Checkboxes and Radio Buttons: Enable users to make multiple or single selections.
- Sliders: Control values within a defined range.
Properly choosing the right widgets is essential. It ensures clarity and functionality in the interface. For instance, using a slider for volume adjustment feels more natural than a text box, which can lead users to input an incorrect value.
Event-Driven Programming
Event-driven programming is a programming paradigm that is central to GUI design. This approach is based on responding to user actions, such as clicks or key presses. Each interaction triggers an event, and the application must handle these events effectively to provide a seamless experience.
In Python, frameworks like Tkinter and PyQt leverage event-driven techniques. Developers define event handlers—functions designed to execute when a specific event occurs. For example, clicking a button might trigger a function that performs a computation or brings up a new window. This structure keeps the application responsive and allows for dynamic user interactions, which enhances the experience significantly.
User Experience Considerations
Designing a GUI is not just about making an application look good. The user experience (UX) should be at the forefront of all design decisions. Key factors to consider include:
- Consistency: The appearance and behavior of widgets should remain uniform throughout the application, reducing cognitive load on users.
- Feedback: Users should receive immediate feedback for their actions, such as visual changes when a button is pressed.
- Accessibility: Considerability for users with disabilities is critical. It is important to ensure that applications are usable for everyone, which can involve keyboard navigation and screen reader support.
Good design is not just what it looks like and feels like. Good design is how it works.
Overall, focusing on these fundamental concepts is key in constructing effective GUIs. By understanding widgets and their types, employing event-driven programming, and emphasizing user experience, developers can create applications that are not only functional but also enjoyable to use.
Creating a Simple GUI Application
Creating a simple GUI application is fundamental in understanding how user interface design works. This section lays the groundwork for implementing functional applications, illustrating the connection between theory and practice. Learning to build a basic GUI introduces concepts like user interactions, event handling, and the creation of interactive elements. For a novice programmer, it demystifies the development process, while experienced programmers can refine their skills and explore new features.
The significance of developing a simple GUI cannot be overstated. It serves as an entry point, where developers can grasp essential components without being overwhelmed. A clear understanding of the basics is vital before diving into more complex systems and applications. Additionally, this exercise enhances problem-solving skills and fosters creativity in design and functionality.
Step-by-Step Guide
To create a simple GUI application in Python, the process can be broken down into manageable steps:
- Choose a GUI Framework: Select a framework, for example, Tkinter, which is included with most Python installations. It is a good starting point due to its simplicity and ease of use.
- Setup the Environment: Ensure your environment is properly configured. This involves verifying that Python is installed. You can use Integrated Development Environments (IDEs) such as PyCharm or Anaconda for convenience.
- Create the Main Application Window: Begin by initializing the main window of your application. This forms the primary interface where widgets will be placed.
- Add Widgets: Incorporate interactive elements such as buttons, labels, and entry fields on the main window. Each widget has its own function and can be adjusted through various parameters.
- Design Layout: Use layout managers to control how widgets are arranged in the window. It is essential to consider user experience while doing this.
- Implement Logic: Attach functions to handle events triggered by user interactions, such as pressing a button or typing in a field.
- Run the Application: Finally, execute the application. The GUI should now respond to user input appropriately.
Code Walkthrough
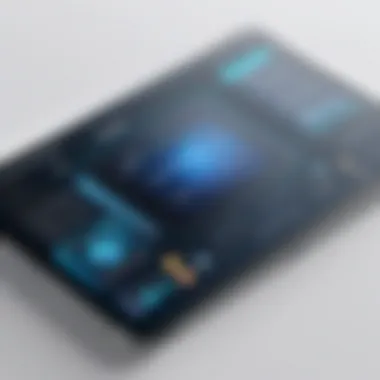
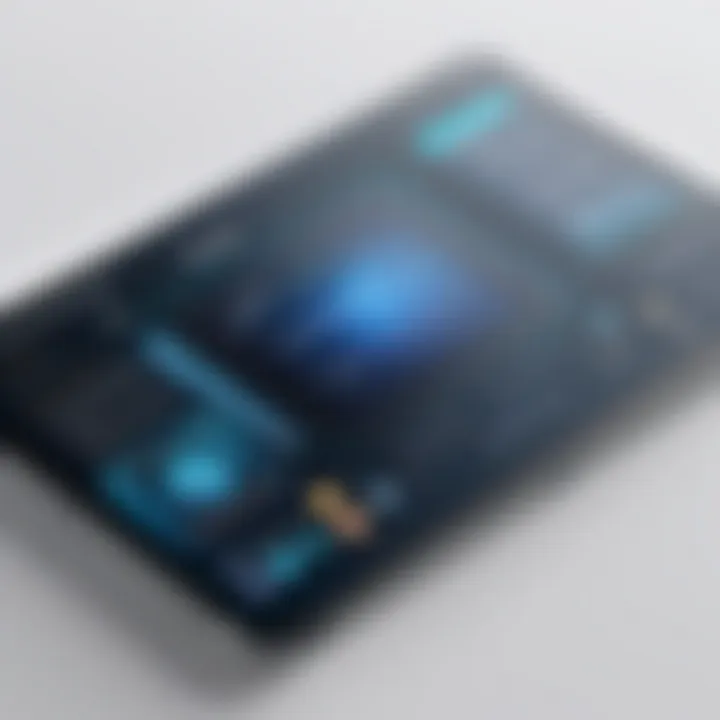
Here is a simple example of a Python GUI application using Tkinter:
This code establishes a basic GUI with a label and a button. The label displays a greeting, while the button allows users to terminate the application politely. This straightforward example illustrates the core elements of GUI programming in Python, providing a solid foundation on which to build more complex applications.
In this section, we've explored the initial steps for creating simple Python GUI applications. Understanding the structure and processes involved creates a pathway for tackling more advanced GUI projects in the future.
Enhancing Functionality with Complex Widgets
Enhancing functionality with complex widgets in a Python GUI is crucial for providing users with a more powerful and engaging interface. Complex widgets are specialized components that allow developers to implement advanced features within their applications. By integrating these elements, developers can improve usability and maintain a high level of interaction. This section explores two primary aspects of complex widgets: the usage of menus and toolbars, and the incorporation of dialogs and prompts. In essence, these elements enrich the user experience and provide the necessary tools for effective interaction with the application.
Using Menus and Toolbars
Menus and toolbars are vital for organizing functionalities and actions within a GUI application. They serve as focal points for navigation, allowing users to access various features efficiently. Properly designed menus can help in categorizing functions, making tasks simpler and more intuitive for users. Toolbars, on the other hand, offer quick access to frequently used features, increasing productivity.
Some key considerations when implementing menus and toolbars include:
- Strategy in Design: A logical structure is critical. Group related actions together. This clarity reduces user effort.
- Icons and Text: Use icons along with text for clarity. Users appreciate visual representation which supports easy recognition.
- Customization Options: Allow users to customize their menu and toolbar preferences. It promotes a personalized experience.
In practice, using libraries like Tkinter or PyQt makes it easier to create and manage menus and toolbars. For example, in Tkinter, the widget can be utilized to generate a complete menu bar, while with PyQt, the class can conveniently handle toolbars.
Proper organization and intuitive access to tools are essential for effective user interaction.
Incorporating Dialogs and Prompts
Dialogs and prompts are essential components in enhancing the user experience. They serve specific purposes, such as informing users, answering questions, or receiving input. Properly implemented dialogs ensure that the interaction is meaningful and context-sensitive.
When incorporating these components, consider the following:
- Types of Dialogs: Use modal dialogs to interrupt workflow, or non-modal dialogs for less intrusive interaction. Decide based on the scenario.
- User Feedback: Ensure dialogs provide feedback to users. It builds trust and informs them that actions are being processed.
- Input Validation: Properly validate user input in prompts. This reduces errors and improves data integrity.
Utilizing Python’s various GUI libraries simplifies the integration of dialogs and prompts. For instance, Tkinter offers the module for standard messages, while PyQt provides comprehensive dialog handling through classes like .
Implementing complex widgets effectively not only enhances functionality but also results in a more polished and professional application. By incorporating tools like menus, toolbars, dialogs, and prompts, developers can create applications that are efficient, user-friendly, and capable of managing complex functionalities with ease. This allows programmers, from novices to experts, to build impactful applications that leave a lasting impression.
Working with Layout Managers
Layout managers are crucial in the development of graphical user interfaces. They determine how widgets are arranged within the application window. In Python, the proper use of layout managers contributes significantly to the application's usability and aesthetics. A well-structured layout enhances user experience, allowing for intuitive interaction. Understanding layout managers helps developers create applications that not only function well but also appear organized and visually appealing.
Types of Layout Managers
There are several types of layout managers commonly used in Python GUI development. Each has its own unique characteristics and is suitable for different application needs. Understanding these options is vital for selecting the most appropriate manager for a specific project.
- Pack: This layout manager organizes widgets in blocks before placing them in the parent widget. It is simple to use and allows developers to control the arrangement through side, fill, and expand options.
- Grid: The grid layout manager organizes widgets in a table-like structure. Developers specify the row and column for each widget, making it easy to create complex layouts.
- Place: This layout manager allows precise control over the widget's position. By using absolute positioning, developers can place widgets exactly where they want them in the window. However, it is less flexible when the window size changes.
Best Practices for Layout Management
Utilizing layout managers effectively involves following several best practices. These practices are key to achieving a clean and functional interface.
- Choose the Right Layout Manager: Understand the strengths and weaknesses of each layout manager. Selecting one that aligns with the application's requirements will result in better usability.
- Consistent Alignment: Ensure that widgets are consistently aligned. This leads to a more professional appearance and makes it easier for users to interact with the application.
- Responsive Design: Respectively manage how widgets respond to window resizing. Use layout managers that automatically adapt to changes to maintain usability.
- Minimize Overlap: Avoid overlapping widgets. Clear and distinct placement of widgets makes the interface easier to navigate.
- Test Across Different Screen Sizes: Evaluate the layout on various screen sizes. This ensures that the application is accessible and user-friendly on all devices.
"Using the right layout manager can make the difference between a cluttered interface and an intuitive one."
In summary, effective layout management is fundamental in GUI development. By understanding the available types of layout managers and adhering to best practices, developers can enhance the user's interaction with the application.
Debugging and Testing GUI Applications
Debugging and testing GUI applications is a crucial element of software development. When developing a graphical user interface, issues may arise that disrupt user experience and application functionality. Without thorough debugging and testing, users may encounter bugs that hinder their interaction with the application. This section emphasizes the significance of testing methodologies, common problems, and practical solutions that aid developers in creating a reliable application.
Common Issues and Solutions
Developers often face various challenges when working on GUI applications. Here are some of the most frequent issues:
- Event Handling Problems: Users may experience unresponsiveness or unexpected behavior due to incorrect implementation of event handlers.
- Layout Issues: Poorly designed layouts can result in overlapping widgets, inappropriate sizing, or elements that do not adjust properly across devices.
- Performance Bottlenecks: Heavy processing tasks in the main thread can lead to sluggish interfaces.
- Inconsistent Behavior: Different platforms or versions of Python may produce varied results, leading to confusion.
To address these concerns, developers should consider the following solutions:
- Implement Logging: Utilize logging libraries to track events and data flow. This process aids in identifying where issues occur in the application.
- Use Version Control: Employ version control systems to isolate changes and more easily identify bugs related to recent updates.
- Adopt Best Practices for Code Quality: Writing clean and understandable code minimizes the likelihood of errors.
- Conduct Regular Code Reviews: Collaborating with other developers can uncover potential issues that one might overlook.
"Good debugging is as much about understanding your application as it is about writing code."
Testing Methodologies for GUIs
Testing methodologies are vital for ensuring that GUIs function as intended. Here are some approaches that can be employed:
- Unit Testing: This involves testing individual components of the application. In Python, libraries like unittest or pytest can be useful. Writing unit tests ensures that widgets behave correctly in isolation.
- Integration Testing: After unit testing, integration tests validate how well different parts of the application work together. This can be achieved using frameworks like PyQt’s QTest, which facilitates automated testing of GUI interactions.
- Manual Testing: While automation provides efficiency, manual testing allows for checking the user experience directly. Not all aspects of a GUI can be automated, so human interaction is required to truly assess usability.
- User Acceptance Testing (UAT): Final testing ensures that the application meets the requirements and expectations of end-users. This phase should include real users interacting with the application to gather feedback.
Incorporating these methodologies results in a more robust application, ultimately enhancing user satisfaction. The combination of consistent testing and diligent debugging practices forms a strong foundation for any successful GUI development.
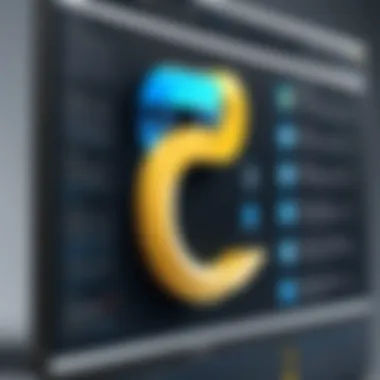
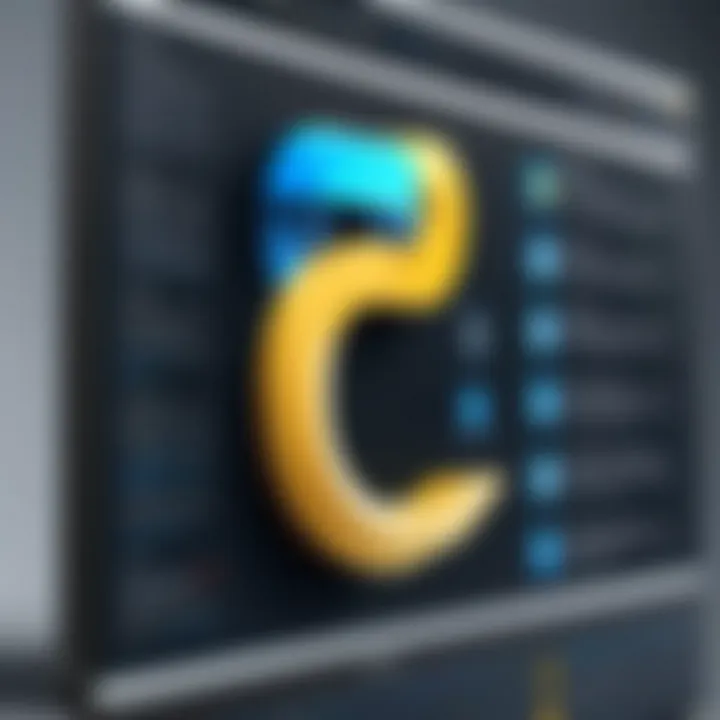
Deploying Your GUI Application
Deploying a graphical user interface (GUI) application is a critical step in the software development lifecycle. This process not only impacts how users interact with your software but also how effectively they can access its functionalities. In Python, deploying GUI applications involves several considerations, including creating executables, ensuring compatibility across different operating systems, and efficiently distributing the application to end users. A well-executed deployment can enhance user experience and satisfaction, thereby contributing to the application’s success in the competitive landscape of software development.
Creating Executables
Creating executables is the process of converting Python scripts into standalone applications. This allows users to run your application without needing to install Python or any additional dependencies. Tools like PyInstaller, cx_Freeze, and Py2exe are popular for this purpose. They can package your Python project, including its libraries and resources, into a single executable file.
The primary benefit of creating executables is accessibility. Users can quickly launch your application with a double-click. It eliminates the complexity involved in setting up a Python environment, which can be a significant barrier for users who are not technically inclined.
Steps to Create an Executable:
- Install Required Tools: Ensure that you have the necessary packaging tools installed.
- Prepare Your Code: Structure your code correctly so it can be packaged without issues, focusing on file paths and resource management.
- Run the Packaging Tool: Use commands specific to the tool you choose. For instance, with PyInstaller, you might run the command .
- Test the Executable: After creation, run the executable in various environments to check for potential errors.
It is crucial to test the generated executables before distribution. This ensures that users will have a smooth experience without troubleshooting issues.
Distributing to End Users
Distribution of your application involves making the executable available to users in a manner that is both efficient and user-friendly. There are several methods to consider for distribution, depending on your target audience and how you want them to access your application.
Methods of Distribution:
- Direct Downloads: Hosting the executable on a website for users to download directly. This is straightforward and allows you to control the distribution process.
- Package Managers: Use package managers like Homebrew for macOS or WinGet for Windows to simplify installation for users. These tools automate the download and installation process.
- Software Marketplaces: Consider platforms such as the Microsoft Store or the Mac App Store for increased visibility and credibility.
Engagement with the user community is also important. By facilitating easy access to your application, you promote further engagement, feedback, and ultimately, adoption.
"The success of a GUI application is not just in its design, but in its accessibility and ease of use."
In summary, deploying a GUI application in Python is a multi-faceted process involving the creation of executables and strategic distribution to users. By focusing on these elements, developers can ensure that their applications reach their intended audience efficiently, making an impactful presence in the market.
Case Studies: Real-World Applications
In the realm of graphical user interface (GUI) development in Python, case studies serve as practical demonstrations of concepts discussed previously. They provide tangible examples of how various frameworks and design principles are applied in real-world scenarios. By examining actual use cases, developers can grasp the transformative potential of their work. Case studies also highlight practical challenges and unique solutions, allowing both novice and experienced programmers to learn from others’ experiences.
Desktop Applications
Desktop applications remain a significant arena for Python GUI development. Many desktop applications leverage the flexibility and extensive libraries available in Python. PyQt and Tkinter are common frameworks used for these types of applications. A notable example is the application Anaconda Navigator, which uses PyQt to provide users with a seamless interface for data science tools. The ease of installation and usage makes it approachable for data scientists who may not be adept at programming.
From a development perspective, various thresholds exist when developing desktop applications like the challenge of operating system compatibility. Python's cross-platform nature mitigates this, allowing developers to design applications that function on various systems without significant modification.
Data Visualization Tools
The demand for data visualization continues to rise in many sectors, leading to the creation of numerous specialized tools. Libraries such as Matplotlib and Plotly enable the development of graphical representations of complex data sets, often resulting in interactive user experiences. For instance, Dash is a framework built on top of Flask and uses Plotly for creating analytical web applications. Users can effortlessly visualize data trends through interactive dashboards, making it a compelling solution for business intelligence.
Data visualization tools epitomize the importance of clear and engaging GUIs. When presenting complex concepts, the interface’s design either facilitates understanding or hinders it. Therefore, usability in this context is critical, as users often seek swift access to insights.
Overall, the exploration of these case studies reinforces the vast applications of Python in developing user-friendly, effective GUI solutions that address real-world needs. By studying successful projects, developers can draw inspiration and practical strategies to enhance their own work.
Future Trends in Python GUI Development
The landscape of graphical user interfaces (GUIs) is continuing to evolve rapidly, largely driven by advancements in technology and changing user expectations. This section centers on important trends within Python GUI development. Understanding these trends is critical, not just for enhancing user experiences, but also for staying relevant in an increasingly competitive field.
Integration with Web Technologies
One area of significant growth is the integration of GUI applications with web technologies. This trend is gaining traction as users expect more seamless experiences across platforms. Here are some key considerations:
- Cross-Platform Compatibility: With the rise of web applications, the expectation for GUIs to operate smoothly across different operating systems has increased. Python developers are using frameworks that support this integration, making desktop applications as accessible as web applications.
- Web Frameworks in Desktop Apps: Technologies such as Flask and Django are now being utilized to create rich, interactive user interfaces. This allows developers to leverage their existing web development skills, contributing to a smoother learning curve.
- Rich User Experiences: Technologies like WebSocket and AJAX enable real-time communication between the client and server. This creates dynamic interfaces that respond instantly to user actions, enhancing overall usability.
The ability to combine traditional desktop applications with web-based technologies offers programmers unprecedented flexibility and power.
Advancements in Frameworks
Frameworks are at the forefront of these developments. Changes in existing frameworks and the rise of new ones are impacting how GUIs are built in Python. Consider the following advancements:
- Framework Maturity: Established frameworks like Tkinter and PyQt are continuously updated to incorporate new features and respond to feedback from the user community. This evolution leads to improved performance, security, and usability, thus making them more appealing to developers.
- Emergence of New Frameworks: Frameworks like Kivy and BeeWare are significant as they focus on mobile and cross-platform application development. They provide tools that allow GUIs to reach a broader audience. Similarly, these frameworks support modern design principles, making applications more visually attractive.
- Community Support and Documentation: As the popularity of Python GUI frameworks grows, so does the community support around them. Rich documentation and a wide array of online tutorials can significantly reduce development time. Such resources are easily accessible on platforms like GitHub and Reddit.
Resources for Continued Learning
Understanding how to build GUIs effectively is just the beginning. Continued learning plays an essential role in mastering techniques and keeping pace with advancements in technology. Developers who engage in lifelong learning can adapt more easily to new development frameworks and shifting industry demands. This section outlines several important resources that can help programmers at all levels further their knowledge and enhance their skills in GUI development.
Books and Online Courses
Books and online courses are invaluable for expanding your understanding of GUI development. They offer structured content, comprehensive knowledge, and deeper insights compared to casual learning resources. A notable book is "Python GUI Programming Cookbook", which provides a hands-on approach, covering several frameworks like Tkinter and PyQt. Online platforms such as Coursera and Udemy offer courses specifically focused on Python GUI development, allowing you to learn at your own pace. Videos and interactive exercises can be especially helpful for grasping complex concepts.
Consider the following key benefits when choosing your learning materials:
- Diverse Perspectives: Different authors and instructors can provide unique insights.
- Practical Examples: Real-world projects help contextualize theory.
- Flexible Learning: Online courses allow you to manage your time effectively.
Community Forums and Networking
Engaging with community forums is a beneficial practice for any developer. Sites like reddit.com or specialized forums provide platforms for asking questions, sharing knowledge, and finding solutions to common problems. Networking with fellow programmers opens up potential collaborations on projects and might provide access to exclusive resources.
Key points regarding community engagement:
- Support and Guidance: Get advice from more experienced developers.
- Knowledge Sharing: Contribute your insights while learning from others.
- Staying Updated: Community discussions often keep you informed about the latest trends and technologies.
In summary, leveraging books, online courses, and community forums leads to a deeper understanding of GUI development. Continual education is crucial for any aspiring or experienced programmer. Keep pushing the boundaries of your knowledge.