Comprehensive Guide to the Cast Function in Programming

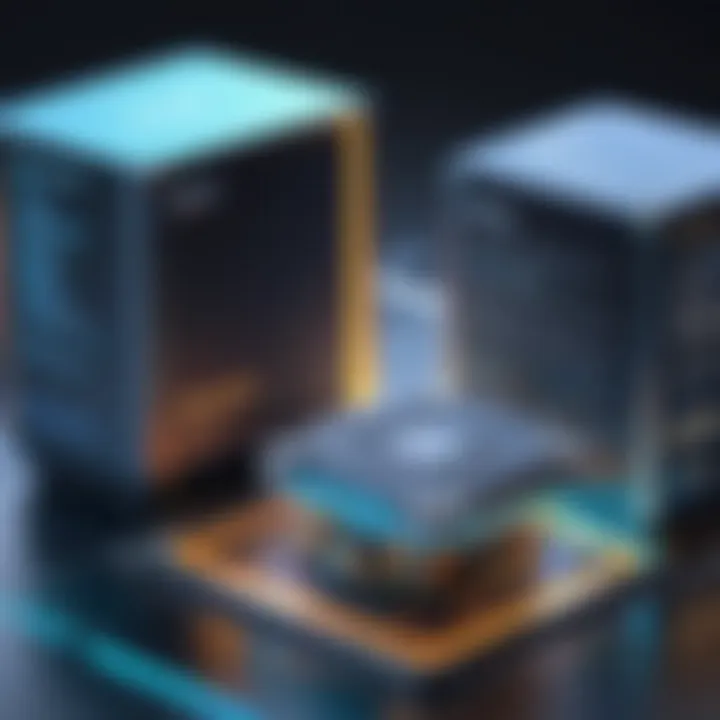
Intro
In programming, one fundamental concept that can trip up even seasoned developers is type casting. At first glance, it might seem simple enoughâafter all, we're just telling our code to treat data as a different type. Yet, dig a bit deeper, and you'll unearth a wealth of nuances that can make or break a project. This section sets the stage by providing a look at the cast function's pivotal role in various programming languages, laying the groundwork for a comprehensive understanding of its significance in coding and beyond.
Coding isnât merely about making things work; itâs about making them work efficiently and effectively. Whether you're dealing with Python's dynamic typing or stricter languages like C++, understanding the cast function is essential for any programmer trying to navigate the murky waters of data handling.
Coding Challenges
Weekly Coding Challenges
Tackling coding challenges is one way to sharpen your skills, and type casting often plays a key role. From converting integers to strings to handling user inputs efficiently, you can expect to encounter casting dilemmas in unexpected places. Consider, for instance, a challenge where you need to manipulate dates. Without a solid grasp of how to cast types appropriately, you risk running into errors that can derail your solution.
Problem Solutions and Explanations
Take, for example, a common situation in Java where you might need to cast a double to an integer. The challenge here isnât just the act of casting itself, but understanding the implications of that conversion. When you do so, you're not just changing the representation of a number; you're also potentially losing precision.
Here's a quick code snippet that may illustrate the point:
This cast effectively truncates the decimal portion of the number, which may be acceptable in some contexts but inappropriate in others. Recognizing these subtleties is what helps to distinguish a novice from an expert coder.
Tips and Strategies for Coding Challenges
To succeed in coding challenges that involve casting:
- Always check the type: Knowing what type you're starting with greatly influences how you approach casting.
- Be wary of implicit casting: Just because a language allows it doesnât mean it's a good idea. Implicit casts can sometimes lead to unexpected results.
- Test edge cases: Always think about what could go wrong with your cast. What happens when you try to convert a large number to a smaller type?
Community Participation Highlights
Engaging with the programming community can help to shed light on best practices and common mistakes. Platforms such as Reddit have dedicated threads where developers share their experiences and solutions regarding type casting. Engaging in these communities can provide perspectives that you might never stumble upon in textbooks. Remember, learning is often a collective endeavor.
Preface to Casting
Casting is a fundamental concept in programming which plays a crucial role in data manipulation and type management. This section sets the stage for understanding how and why casting is used, emphasizing its significance in handling data types across various programming languages. A lack of clarity on casting can lead to confusion in code behavior, ultimately affecting performance and functionality. Thus, grasping the nuances of casting is essential for programmers, whether novice or seasoned.
Definition of Casting
In programming, casting refers to the process of converting a variable from one data type to another. The operation might seem straightforward, but its implications can be vast. For instance, one might convert an integer to a float or change a string that represents a number into an actual numeric type. Casting can be implicit, where the compiler automatically handles the conversion, or explicit, requiring the programmer to specify how the change should take place. Consider this simple example:
The transformation is clear, and the need for such conversions frequently arises in mathematical calculations or when interfacing with APIs.
Importance in Programming
Casting is indispensable in programming for several reasons:
- Type Safety: It ensures that operations performed on data are appropriate, reducing the risk of runtime errors.
- Memory Optimization: It could also help optimize memory use, as certain operations require specific data types for optimum performance.
- Interoperability: Many programs need to interface with external systems. Casting allows different modules or services to communicate seamlessly by ensuring that the data types match across boundaries.
- Flexibility: Developers often face circumstances where data must be represented in varying formats, and casting provides the flexibility to adapt to these needs.
"Mastering casting is like learning to dance â once you understand the steps, you can gracefully navigate programming challenges with ease."
The upside of casting significantly outweighs its pitfalls, but being aware of the potential trapsâsuch as data loss or runtime exceptionsâ is essential for every developer. This highlights the need for a thorough understanding of casting, as we'll explore in more detail in the following sections.
Types of Casting
Casting serves as a crucial pillar in programming, allowing developers to manipulate data types seamlessly to meet specific requirements. Understanding the various types of casting can help programmers avoid errors, manage resources effectively, and optimize performance in their applications. In this section, we will delve into the two primary types of casting: implicit and explicit casting. Each type has its unique features, advantages, and potential drawbacks, making them vital for effective coding across different programming languages.
Implicit Casting
Definition and Examples
Implicit casting, often referred to as automatic or type promotion, occurs when a data type is converted to another type without any explicit instruction from the programmer. This process tends to happen when the conversion is considered safe, meaning there is little or no chance of losing valuable data in the process. A predominant characteristic of implicit casting is its seamless nature, allowing developers to focus on higher-level logic rather than constant type checks.
For example, in a language like C++, consider the following code:
In this scenario, the integer variable is automatically converted to a double type. The benefit? The code stays clean, and the programmer can work without worrying about potential type mismatches at this point.
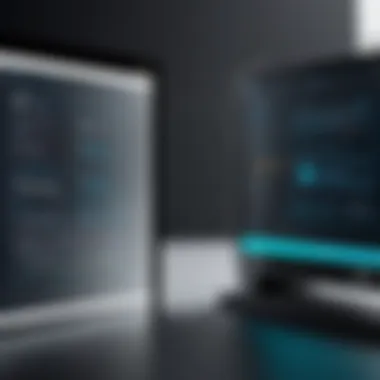
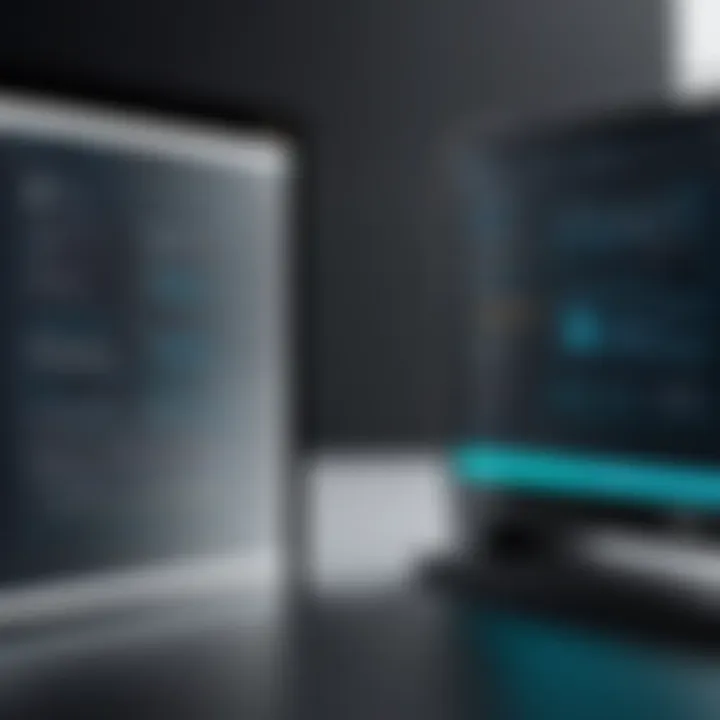
Use Cases and Limitations
The use cases for implicit casting primarily revolve around mathematical operations or when variables are assigned to broader data types. A prime example is when you perform arithmetic calculations with mixed data types like integers and floats. Implicit casting handles the conversion and takes care of any necessary adjustments.
However, it's important to be wary of certain limitations. Implicit casting can lead to unintentional data loss when converting from a larger to a smaller type. For instance, further narrowing down from a long to an int without checking its range can yield unpredictable results:
Explicit Casting
Definition and Examples
Unlike implicit casting, explicit casting requires the programmer to specify the conversion explicitly. Also known as type casting or manual casting, this method gives developers control over how data types are converted, ensuring that any potential data loss or precision loss is acknowledged and handled.
Consider the following code snippet in Python:
In this case, converting a float to an integer is explicit, thus making the developer aware of the inherent data loss that arises from dropping the decimal portion of the number.
Use Cases and Limitations
Explicit casting shines in scenarios where precision is not just appreciated but required. Developers often opt for explicit casting during operations that involve complex data structures, ensuring that they retain complete control over the data transformation. For instance, when interfacing with APIs or databases, knowing the exact data type of the variable can help prevent unexpected issues down the line.
Nevertheless, this approach does not come without its own limitations. Explicit casting can occasionally lead to overly verbose code, requiring the programmer to constantly check casting conditions, which detracts from readability. This leads to a trade-off between safety and clarity:
Ultimately, understanding the mechanics of implicit and explicit casting enables developers to write cleaner, more efficient code while minimizing mistakes and errors.
Mechanics of the Cast Function
Understanding the mechanics of the cast function is crucial for any programmer looking to harness the full power of their chosen programming language. Casting is not just a convenience; it plays a vital role in ensuring that data types interact correctly, and it lays the groundwork for advanced programming practices. The cast function bridges the gap between different data types, allowing developers to manipulate and control the flow of information seamlessly. This can enhance code readability, promote better performance, and minimize errors arising from type mismatches.
Syntax Across Different Languages
Casting syntax can vary significantly from one programming language to another. Knowing this syntax is fundamental since it dictates how developers perform casting operations.
Java
In Java, casting is straightforward. The syntax looks like this:
Here, the number 3.14 is explicitly cast to an integer, dropping the decimal values. The key characteristic of Java in this context is its strong, statically typed nature, meaning type errors are caught at compile time rather than runtime. This feature can be advantageous for developers, as it encourages them to be precise with their data types from the get-go. However, it can also lead to unexpected behaviors if one is not careful, especially when dealing with operations that may lead to data loss.
++
C++ employs a more nuanced approach with several casting options, such as , , and . A typical example using may look like this:
C++'s flexibility in casting is a double-edged sword. On one hand, it allows for fine-grained control over the casting process. On the other hand, new developers may find the range of options overwhelming and potentially lead to misuse. Therefore, understanding the specific contexts where each cast is appropriate becomes essential.
Python
Python takes a different route; it emphasizes simplicity. The casting function looks like this:
In this case, the float is converted to an integer seamlessly. Pythonâs dynamically typed nature allows for this kind of flexibility, making it popular among beginners. However, the trade-off is that it may lead to runtime exceptions if assumptions about data types are incorrect. Developers need to remain vigilant to ensure type safety, especially when working on larger projects with more complex data interactions.
Casting in Object-Oriented Programming
Casting in the realm of object-oriented programming (OOP) is a different ball game altogether. It plays a crucial role in inheritance hierarchies and polymorphism, which are central to OOP principles.
Upcasting and Downcasting
Upcasting refers to casting a subclass to a superclass. This helps in treating a more specific type as a more general type. For example:

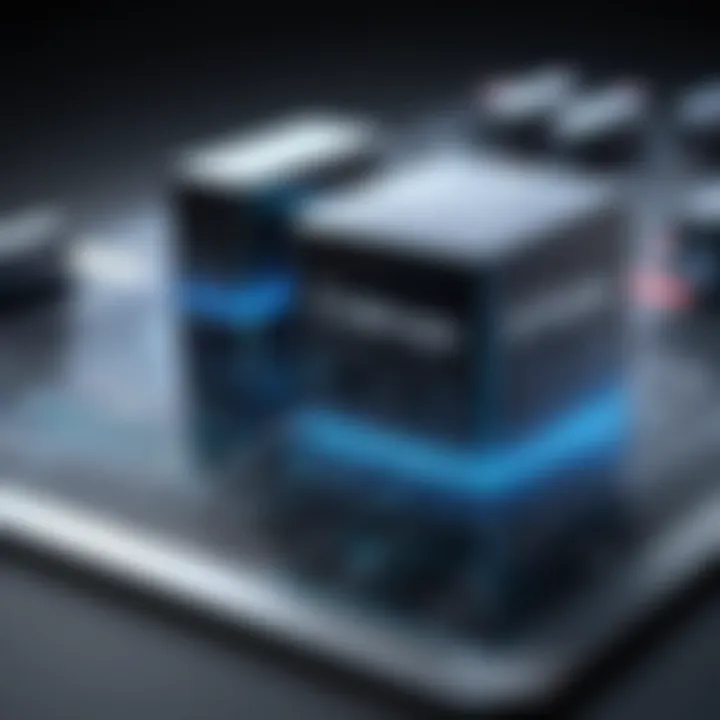
This approach is beneficial because it maintains polymorphic behavior. However, downcasting involves casting a superclass object back to a subclass, which should be approached with caution. Missteps here can lead to runtime exceptions, as the object's original type may not support the subclass methods. Therefore, understanding when to employ upcasting and downcasting is key to effective coding in OOP.
Interfaces and Abstract Classes
Casting also plays a pivotal role when dealing with interfaces and abstract classes. An interface in programming essentially defines a contract that implementing classes must adhere to, but without defining how that contract is executed. For example:
In this scenario, any class that implements the interface can be treated as an instance of . This abstraction provides a clear path for flexibility in design, though it also necessitates careful attention to casting when working with collections or lists of interface types. Misalignment between expected and actual types can lead to hard-to-trace bugs that frustrate even the most seasoned developers.
Proper casting can turn potential pitfalls into opportunities for cleaner and more efficient code.
By grasping the mechanics of casting, developers can make informed decisions that ultimately lead to more robust programs.
Common Pitfalls in Type Casting
Type casting is a vital concept in programming, enabling developers to convert one data type into another. However, this seemingly straightforward process can be fraught with pitfalls that could lead to unforeseen issues, making a deep dive into the common problems associated with type casting essential. Understanding these pitfalls not only prepares programmers to navigate challenges more effectively but also enhances their coding proficiency and reduces the likelihood of runtime failures. Let's explore two primary concerns: data loss issues and runtime exceptions.
Data Loss Issues
One of the most significant risks associated with type casting is data loss. This often occurs when converting from a larger data type to a smaller one. For example, when casting a to an , the fractional part is discarded, resulting in a potential loss of information. Consider the situation:
In this code snippet, while assigning the value of to , the decimal portion is lost. Depending on the application, this might not be just a minor slip. If the value is utilized in further calculations or decisions, it could yield incorrect results. It's crucial to assess whether the loss of information will significantly affect your application's functionality.
Tips to avoid data loss:
- Always be aware of the data types you are working with. Know the range and precision of each type to avoid scenarios where information can get truncated.
- If data loss is unavoidable, consider implementing validation checks or warnings to alert developers of potential issues during compile time.
- Document type casting intentions in the codebase as a reference for future developers or yourself.
Runtime Exceptions
Runtime exceptions can be another tricky aspect of type casting. These exceptions usually occur when the program attempts to cast an object to a type to which it doesn't belong. For instance, trying to cast a base class object to a derived class that it isn't associated with can lead to in Java.
An example to illustrate this:
The above scenario will throw an exception at runtime. The key takeaway here is to always ensure that the object you're working with is truly of the type you're casting it to. One way to safeguard against this is to use the operator in languages like Java:
By employing such checks, you can prevent your application from crashing unexpectedly.
In summary, while type casting is an uncomplicated concept at first glance, it carries with it the potential for significant pitfalls. By being aware of issues such as data loss and runtime exceptions, programmers can make informed decisions and write more robust code that minimizes risk. The coding journey truly lies in understanding the subtleties of the craft, and recognizing these hurdles marks a step toward proficient programming.
Performance Implications of Casting
In programming, the performance implications of casting are a critical yet often overlooked aspect. Understanding the costs associated with casting can enhance both the efficiency and reliability of software development. When an application performs type casting, it can impact memory usage, execution times, and overall responsiveness.
Overhead in Performance
Casting introduces some additional overhead. This overhead varies depending on the language and context of the casting operation. For example, in languages like Java or C#, converting between certain types may trigger additional checks and conversions. These checks can slow down execution, especially in loops or frequently called methods.
- Memory Usage: When casting types, particularly in large datasets or complex data structures, additional memory may be allocated. This is especially true for objects in object-oriented programming where downcasting may require creating a new instance.
- Execution Time: The time taken for casting operations can accumulate significantly if they are being called repeatedly in hot paths of execution.
- Garbage Collection: In managed languages with garbage collection, excessive or unnecessary casting can lead to more frequent garbage collection cycles, further impacting performance.
"While type casting is sometimes necessary, it can often be done more efficiently, if we are conscious of how often and where we are casting in our code."
To mitigate performance costs, developers must consider the placement and frequency of type casting operations. Optimizing these areas can lead to smoother, more efficient applications.
Best Practices for Performance Optimization
When it comes to minimizing the performance impact of casting, a few best practices can go a long way:
- Limit Casting Operations: Try to minimize the number of cast operations. If possible, design your data types to eliminate the need for casting altogether.
- Prefer Static Typing: Statically-typed languages tend to handle casting with less overhead than dynamically-typed languages. Use the strengths of your language to your advantage.
- Use Generics: Where applicable, leverage generics to manage type safety without necessitating casts.
- Profile and Test: Always profile your code to identify bottlenecks. Pay special attention to any casting that occurs inside performance-sensitive code to determine if it can be avoided or refactored.
- Educate on Type Safety: Understanding type safety and how to work within those parameters can reduce the need for casting. Build a strong foundation in the data types and structures of the programming language you are using.
By being mindful of how casting affects performance, developers can maintain cleaner, more efficient codebases. In turn, this contributes to a better user experience and reduces resource consumption.
Testing and Debugging Casting Issues
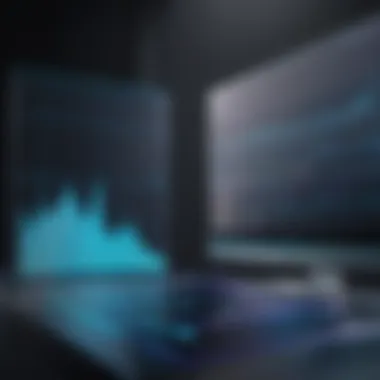
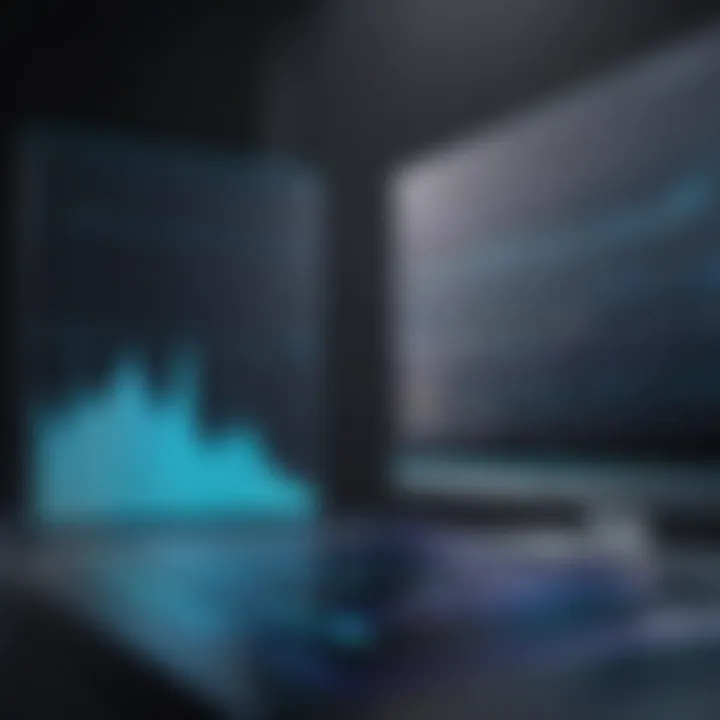
When it comes to programming, testing and debugging casting issues can't be emphasized enough. The cast function, while a powerful tool for manipulating data types, can easily lead developers astray if not handled properly. As types accumulate, the risk of errors increasesâespecially in large codebases where various data types and formats intermingle. Therefore, knowing how to test and debug casting effectively becomes a necessity rather than an option.
Importance of Testing and Debugging Casting Issues
Understanding how to troubleshoot casting issues is crucial for several reasons:
- Data Integrity: Casting errors can lead to data corruption. If a float is cast to an integer without proper checks, the decimal part might vanish silently, leading to unintended consequences.
- Improved Code Quality: By focusing on testing casting scenarios, you ensure that the code adheres to type safety. This act not only catches issues in development but also enhances maintainability.
- Efficiency: Detecting casting issues early reduces the risk of runtime failures, which can be much costlier in terms of both time and resources. Itâs like fixing a leaky faucet before it floods the entire kitchen.
Navigating through casting tests efficiently can empower developers to write more than mere functional code; it enhances reliability as well.
Tools and Techniques
To tackle casting issues, a variety of tools and methodologies are at a programmerâs disposal. Some popular options include:
- Integrated Development Environment (IDE): Many modern IDEs like Visual Studio or JetBrains provide built-in tools to check for type mismatches during development, giving you instant feedback.
- Static Analysis Tools: Tools like SonarQube can perform static code analysis. They help in identifying potential casting issues and enforce coding standards.
- Debuggers: Using debuggers like GDB or Visual Studio Debugger can help step through code line-by-line. Observing the flow of data can clarify how and when casting may cause problems.
- Unit Testing Frameworks: Frameworks available in languages like JUnit for Java or pytest for Python allow you to write specific tests aimed at ensuring type safety. This fits well into a continuous integration pipeline and is vital for ongoing software performance and reliability.
"An ounce of prevention is worth a pound of cure."
Through these tools, developers can systematically approach casting issues, allowing for a more streamlined coding experience.
Unit Testing for Type Safety
Diving into unit tests can significantly bolster the reliability of casting operations. Unit tests can effectively check if your types are what you expect before they hit production, thereby catching errors early. Key considerations include:
- Granular Tests: Each cast should be tested in isolation to ascertain its correctness. This includes scenarios of both implicit and explicit casting, covering edge cases where data types behave unpredictably.
- Use Assertions: Employ assertions to validate that your cast has resulted in the expected type. For instance, checking that a cast from a floating-point to an integer results in an integer.
- Test Failure Handling: Anticipating and testing how your code reacts to failures of type casting can provide insights into potential pitfalls. If a cast fails, how gracefully does your code adapt or react?
- Consider Edge Cases: Float to int isnât just straightforward. What happens with maximum values, zero, and negative numbers? Covering these will save a lot of headaches down the line.
By embedding these practices into your development workflow, you reinforce type safety and enhance overall robustness. This approach functions as a safeguard, allowing you to continue development with greater confidence, knowing that potential casting issues have been carefully vetted.
Case Studies of Effective Casting
Casting is not merely a technical piece of programming; itâs a skill that can significantly affect the robustness and efficiency of an application. By examining tangible case studies, one can dissect successful implementations of casting and distill valuable lessons from mistakes encountered along the way. In this section, we will explore real-world examples where casting played a crucial role in the functionality and performance of various software projects.
Successful Implementations
In real-world applications, developers often find themselves weaving casting into their code to bridge datatype gaps or facilitate interactions between different software components. Consider a scenario involving a large-scale financial application. Here, developers encountered numerous data types when processing transaction data.
When different currencies were represented as types that required precision, explicit casting became vital. Specifically, a developer involved in the project noted that without properly converting these currencies to a common datatype for calculationsâlet's say from a to a âit could lead to erroneous outputs.
The implementation adopted was straightforward. By using explicit casting, the team ensured type safety, which preserved data integrity during financial computations. Hereâs how they did it:
Without such a strategy, the risk of runtime exceptions due to type mismatches would have been high, leading to potential financial inaccuracies.
Another noteworthy instance came from a video game development project. The developers utilized polymorphism to handle different character classes that shared a common interface. Upcasting and downcasting were regularly employed to treat subclasses as their parent class, which allowed them to simplify methods that required varied character abilities. This avoided code duplication and made the game highly flexible.
Lessons Learned from Mistakes
The road to effective casting is often littered with missteps. One particularly illustrative case involved a software company that was building a customer relationship management (CRM) system. They made a mistake by relying heavily on implicit casting when pulling user data from a database.
During the initial phases, the developers thought that relying on automatic type conversion would save time. However, when it came to aggregating user data, they discovered that implicit casting led to unexpected outcomes. For example, user IDs were implicitly cast to strings but were later misinterpreted, leading to duplicate user entries during merge operations. This resulted in considerable confusion and operational issues that took a significant amount of time to address.
To rectify this, the team pivoted to explicitly defining data types at every stage of data handling. They developed a standard practice where every database retrieval was accompanied by a clear casting statement. A system where clarity prevailed helped them avoid the pitfalls from their earlier approach, making their data handling far more predictable and safer.
Learning from this, developers should always remain skeptical about implicit casting, especially when working with critical data. Adopting a methodical approach to type conversion is key in avoiding similar issues.
In summary, case studies reveal that whether casting is utilized effectively or not can distinctly influence the success of a development project. Successful implementations highlight the importance of deliberate, clear, and type-safe coding practices. At the same time, missteps bring to the forefront the risks of complacency and the necessity for vigilance in type management.
Finale and Final Thoughts
As we reach the end of our exploration into the cast function, it's vital to grasp just how essential casting is in the realm of programming. The act of changing one data type to another is not just a technical necessity; it's a fundamental skill every programmer must hone to craft effective and efficient code. Throughout the article, weâve discussed the mechanics, pitfalls, and nuances that make casting a topic worthy of meticulous consideration.
In summary, casting allows compatibility between different types, streamlining processes that could otherwise lead to chaos in the code. This brings several benefits: it enhances type safety, allows for sophisticated manipulation of data structures, and facilitates more readable and maintainable code. However, as weâve seen, itâs also fraught with potential issues like data loss and runtime exceptions. Hence, understanding these concerns is crucial for any developer.
Going forward, those in programming must be proactive in their approach to casting. Embracing best practices not only mitigates risks but also sets a solid foundation for robust applications. The importance of thorough testing and debugging cannot be understated here, nor can the value of learning from both effective implementations and mistakes made by others.
Summary of Key Points
- Key Moments in Casting: We explored what casting entails, the types available, and the roles they play in various programming languages.
- Pitfalls to Avoid: The exploration of common pitfalls offered insight into how easily one can fall into traps like data loss or exceptions, underscoring the necessity for awareness.
- Performance Matters: We discussed how casting affects performance and the importance of optimizing these instances to maintain application efficiency.
- Effective Debugging: Leveraging appropriate tools and creating a strong testing strategy can save developers a lot of headaches in the long run.
Future of Casting Functions in Programming
Looking forward, the future of casting functions in programming appears to be on a promising path. It's increasingly clear that as programming paradigms evolve and languages become progressively more robust, the nature of casting will adapt as well. Here are some facets to consider:
- Automated Type Conversion: Expect to see languages incorporating smarter casting mechanisms, potentially reducing manual oversight required in the casting process.
- Enhanced Type Inference: As machine learning and AI models become more integrated into programming tools, we could see a rise in environments where type inference plays a key role in casting. This could lead to new standards and practices.
- Broader Language Support: More programming languages are prioritizing flexibility in types, which may blur the lines between implicit and explicit casting further than ever before.
- Community and Educational Tools: With greater emphasis on education within coding bootcamps and online resources, the proliferation of knowledge around casting will be key to empowering new developers.
In a nutshell, casting is not merely a technicality but an integral part of code composition and structure. As tools and languages progress, understanding every nuance of casting will remain critical for all who wish to refine their craft.