Mastering Haskell Programming: A Comprehensive Guide
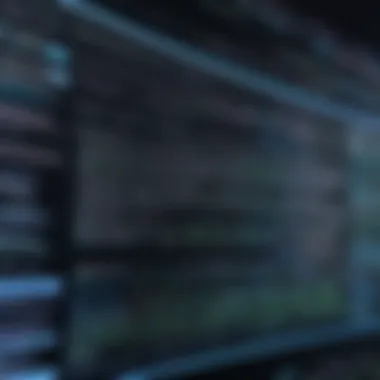
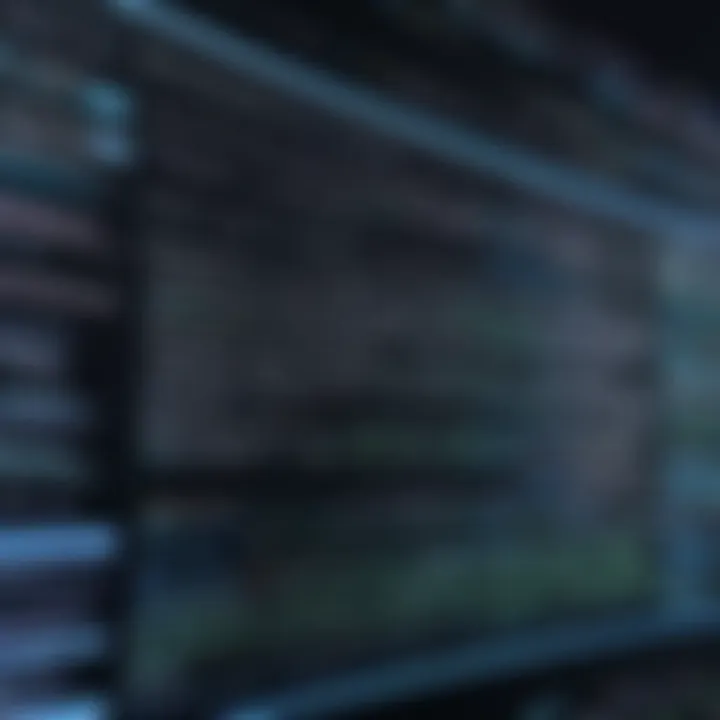
Intro
Haskell is a language that stands out in the realm of programming. It embraces functional programming principles, offering both elegance and efficiency. As coding continues to evolve, Haskell's unique features provide a robust framework for solving complex problems. This guide aims to explore various aspects of Haskell programming courses.
We will discuss key elements including coding challenges, resources, and essential computer science concepts. This comprehensive approach ensures that both aspiring and experienced programmers can refine their skills effectively. By delving into Haskell, learners can uncover practical applications and deepen their understanding of functional programming. Understanding these aspects will also prepare learners to tackle real-world challenges.
Equipping oneself with the right knowledge in Haskell can offer an edge in software development and problem solving. Letās now explore how coding challenges can play a vital role in mastering Haskell.
Prelims to Haskell Programming
Haskell is a powerful, statically typed functional programming language. Its unique features make it stand out in the programming landscape. For both aspiring and seasoned programmers, learning Haskell offers numerous benefits. In this section, we explore the significance of Haskell programming and why it warrants attention.
Firstly, Haskell's design promotes a style of programming that encourages clarity and precision. This contrasts with languages that allow for imperative programming, which can lead to ambiguous solutions. Through Haskell, developers can write code that is not only functional but also more maintainable. The use of pure functions means that functions will consistently produce the same outcome, given the same inputs. This predictability is extremely valuable, especially in large, complex systems.
Secondly, learning Haskell equips programmers with a deeper understanding of functional programming principles. These principles have become increasingly relevant in modern software development. Many contemporary languages, including Python and JavaScript, have adopted functional elements. By mastering Haskell, programmers grasp these concepts at a foundational level.
It's also important to note Haskell's role in academia and industry. The language is often used in teaching computer science concepts due to its strong typing system and focus on functions. This academic background lends itself well to research and projects that require rigorous mathematical reasoning. On the other hand, companies appreciate Haskell's ability to create robust applications, especially those in financial sectors or data science.
Haskell's community is another consideration. The Haskell community is known for being supportive and collaborative. Developers can find numerous resources ranging from online forums to workshops and meetups. This access to community support enhances the learning experience and provides opportunities for networking.
In summary, the introduction to Haskell programming provides insights into its structure and the ecosystem surrounding it. The language is not just a tool for software development; it embodies a philosophy that can change how one thinks about problem-solving. Engaging in Haskell programming is an investment into a versatile and forward-thinking approach to coding.
"Programming in Haskell not only makes you a better programmer, but it also changes the way you think about programming as a whole."
By understanding the foundation of Haskell programming, you are better prepared to explore its features, applications, and the resources available to guide your learning.
The Importance of Learning Haskell
Haskell is a language that stands out in the landscape of programming. Its importance lies not only in its syntactical elegance but also in the distinct paradigms it introduces. Learning Haskell has significant benefits for programmers at all levels, from beginners to seasoned developers.
One major reason to learn Haskell is its focus on functional programming. In contrast to imperative languages, Haskell encourages a different way of thinking about coding. This mindset promotes immutability and pure functions, which often leads to more predictable and bug-free code. As developers adopt these principles, they can enhance their problem-solving skills and create more robust applications.
Another advantage of Haskell is its powerful type system. With static typing and type inference, Haskell helps catch errors at compile time rather than run time. This feature reduces the time spent on debugging and increases confidence in the software you build. Understanding type theory through Haskell can provide invaluable insights for programmers using other languages as well.
Haskell also teaches abstraction. The ability to create higher-order functions and abstract data types allows programmers to write more general solutions to problems. This is especially useful in complex software projects, where reusability and modularity are critical. The lessons learned in Haskell can be applied to various domains including web programming and data analysis.
From a career perspective, familiarity with Haskell can set you apart from other developers. Many companies are now recognizing the benefits of functional programming. Proficiency in Haskell can enhance job prospects in specialized fields like data science, machine learning, and enterprise software development. Furthermore, many startups integrating functional languages are looking for developers with Haskell experience.
To summarize, learning Haskell is not merely about mastering a programming language; it is about adopting an effective way of thinking. Its unique features offer specific advantages that can significantly impact how developers approach programming problems. As the tech world continues to evolve, the skills gained from learning Haskell may prove essential for future growth and complexity in software development.
"Learning Haskell not only equips you with a language but also enhances your programming paradigm."
By engaging with Haskell, you position yourself at the forefront of programming methodologies, gaining a fresh perspective that can elevate your approach to coding and software design.
Overview of Haskell Language Features
Haskell is a key player in the functional programming landscape. Understanding its language features is essential for any programmer looking to harness the language's power. Haskell's design emphasizes clarity and correctness in software development. Moreover, features such as pure functions, lazy evaluation, static typing, and type inference elevate the language above many others. Each of these attributes contributes significantly to Haskell's reputation for building robust and maintainable applications. Diving into these aspects enables learners to appreciate Haskell's unique approach to coding.
Pure Functions
Pure functions are a cornerstone of Haskell programming. They produce the same output for the same input without causing any side effects. This consistency simplifies testing and debugging. By avoiding state changes or variable modifications, pure functions to create predictable and isolated solutions. When a programmer examines the function's behavior, it becomes easier to reason about the code. In addition, pure functions facilitate easier parallelism in programs, leading to potential performance gains.
Lazy Evaluation
Lazy evaluation is another defining feature of Haskell. This mechanism delays computation until the result is needed. It allows programs to efficiently handle infinite data structures and minimizes unnecessary calculations. Lazy evaluation can lead to reduced memory usage and faster application performance in some cases. Programmers can compose functions without worrying about immediate execution, which simplifies the development of complex systems. Understanding this feature is crucial for making the most of Haskellās strengths.
Static Typing
Haskell employs static typing, which ensures that type checking is performed at compile time. This approach reduces the likelihood of runtime errors, which enhances software reliability. Static typing also enables developers to catch errors early in the coding process. This significantly improves the development workflow. Furthermore, static typing provides robust documentation and a clearer understanding of function contracts. As a result, collaborating on larger projects becomes more manageable.
Type Inference
Type inference in Haskell allows the compiler to deduce the type of expressions automatically. This feature minimizes the need for explicit type annotations while ensuring type safety. By streamlining the coding process, type inference allows programmers to write cleaner code while maintaining a strong type system. It simplifies the learning curve for beginners, enabling them to focus on logic and algorithms rather than intricate type declarations. This balance between safety and convenience is one of Haskell's appealing qualities.
Understanding Haskellās key language features is fundamental for maximizing its potential in software development.
When programmers grasp these concepts, they unlock the ability to write efficient, maintainable, and scalable applications. Each feature contributes to both the robustness of Haskell and the overall programming experience. By getting familiar with these elements, one prepares to dive into more advanced topics in Haskell development.
Currying and Higher-Order Functions
Currying and higher-order functions are fundamental concepts in Haskell, shaping the way programmers approach problems and utilize the language's capabilities. Each of these topics has a unique importance, influencing how functional programming promotes code reusability and flexibility.
Understanding Currying
Currying refers to the transformation of a function that accepts multiple arguments into a series of functions, each taking a single argument. In Haskell, this is a natural characteristic due to its function-first design. By currying, a function like can be transformed as follows:
In this example, can be called with one argument, returning a new function waiting for the second argument, which greatly simplifies function application. The primary benefit of currying is its ability to create specialized functions effortlessly. For instance, using will yield a function that adds 5 to any subsequent integer.
Higher-Order Functions
Higher-order functions go hand in hand with currying. These are functions that can take other functions as arguments or return them as results. This capability promotes a clear separation of concerns and allows for advanced abstractions within code. For instance, a higher-order function could be used to apply a function to a list of numbers:
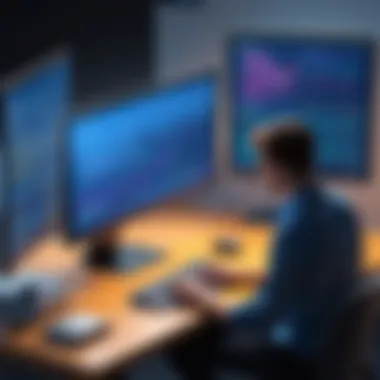
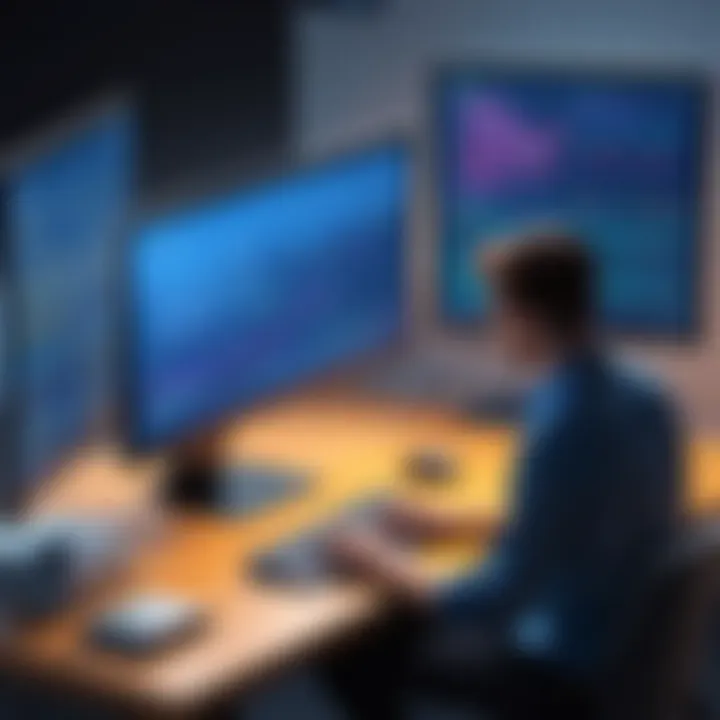
In this code snippet, takes a function and a list , applying to each element of the list, thus producing a new list. The usage of higher-order functions contributes significantly to code conciseness and expressiveness.
Benefits of Using Currying and Higher-Order Functions
- Code Reusability: By allowing functions to be composed and reused, these concepts help prevent repetition and promote cleaner code.
- Flexibility: Currying enables partial application, meaning that functions can be constructed incrementally, allowing for more adaptable coding patterns.
- Improved Readability: Higher-order functions can make code more declarative, focusing on what the code does rather than how it does it. This is ideal for complex operations that can be simplified with clear, concise expressions.
Considerations When Implementing These Concepts
While currying and higher-order functions are powerful, they require careful implementation. Some considerations include:
- Performance: Overusing higher-order functions or excessive currying can lead to performance hits due to overhead from function calls.
- Readability: For those not familiar with these concepts, code can become confusing. Clear documentation and comments are necessary to make the code more maintainable.
"The essence of functional programming is to avoid changing state and mutable data, which makes currying and higher-order functions indispensable tools in achieving pure functional design."
Exploring Haskell's Ecosystem
In any programming language, the associated ecosystem plays a vital role in defining the scope and effectiveness of its application. When it comes to Haskell, a language celebrated for its abstraction and capability in handling complex problems, its ecosystem is rich and diverse. Understanding Haskell's ecosystem allows learners to navigate useful resources, tools, and communities that will support their programming development.
Exploring Haskell's ecosystem can help programmers, both novices and seasoned experts, to leverage its full potential. This ecosystem encompasses libraries, frameworks, and development tools that enhance coding efficiency and project outcomes. By tapping into these resources, learners are better equipped to face challenges and explore innovative solutions.
Another essential aspect is the community support. Engaging with a community can provide not only motivation but also valuable insights and experiences shared by others. This network serves as a support system, fostering an environment where developers can ask questions and share their struggles.
Libraries and Frameworks
Haskell's libraries and frameworks are crucial components that widen its usage across various applications. They facilitate more efficient coding practices and enable developers to utilize pre-built functions, thus saving time and effort.
Some notable libraries include:
- QuickCheck for property-based testing, which can significantly enhance the reliability of code.
- Yesod for web development, offering robust features that simplify the creation of web applications.
- Lens for functional manipulation of data structures, making complex transformations straightforward.
By using these libraries, programmers can focus more on the logic of their applications instead of getting bogged down by low-level details. Additionally, these frameworks reflect best practices and proven methodologies, making them ideal for those looking to standardize their development approach.
Development Tools
Development tools are another pillar of Haskell's ecosystem. These tools aid in writing, testing, and managing Haskell projects. Integrated development environments (IDEs) like Stack and GHCi are essential for streamlining the workflow.
- Stack: This tool allows developers to manage project dependencies effectively, ensuring that all required libraries are included and compatible with the Haskell version.
- GHCi: The interactive environment for Haskell enables quick experimentation and testing of snippets of code. This immediacy can accelerate the learning process, as programmers can see results in real time.
- PostgreSQL: Although not a Haskell-specific tool, it works seamlessly with Haskell applications for database management and can be leveraged within Haskell frameworks.
Overall, these tools enhance the Haskell programming experience, making it more efficient and user-friendly. By exploring the ecosystem and familiarizing yourself with these libraries and tools, you are better positioned to undertake complex projects and solve challenging problems with Haskell.
Finding Quality Haskell Programming Courses
Identifying suitable Haskell programming courses is crucial for anyone looking to deepen their understanding and proficiency in this functional programming language. Quality courses can provide a framework that not only covers the foundational aspects of Haskell but also delves into more complex concepts. Furthermore, an effective learning pathway can lead to significant improvements in coding skills and problem-solving capabilities. Whether online or in a traditional university setting, the right course structure will influence the depth and effectiveness of your learning experience.
When assessing the quality of Haskell courses, students should consider several factors, including the course structure, teaching methods, and community support. Understanding these elements can guide students to make informed decisions about their educational investments.
Online Platforms
Online platforms for Haskell courses provide flexibility and accessibility, making them an attractive option for diverse learners. Many well-known online learning platforms offer structured courses that cater to different skill levels. Some of the more notable platforms include Coursera, Udemy, and edX, all of which host a variety of courses designed to improve Haskell programming skills.
Each of these platforms typically offers several advantages:
- Flexible Scheduling: Learners can access materials at their own pace, allowing them to manage their studies alongside work or other commitments.
- Diverse Learning Materials: Online courses often include video lectures, coding exercises, and quizzes that cater to various learning styles.
- Community Interaction: Many platforms come with forums or discussion groups, enabling interaction with instructors and peers.
As Haskell is still a niche language, find courses with strong reviews or recommendations from the community to ensure the quality of the content. Checking platforms like Reddit can help gauge current opinions and trends concerning specific courses.
"Finding an online course that suits your learning style can make a significant difference in your educational journey and your grasp of Haskell."
University Offerings
University offerings represent another avenue for acquiring knowledge in Haskell programming. Many academic institutions recognize the importance of functional programming and integrate Haskell into their computer science curricula. These courses often provide a more in-depth understanding of theoretical concepts alongside practical applications.
Benefits of university courses may include:
- Structured Learning Path: University courses often follow a clear curriculum that builds upon each section, ensuring a comprehensive grasp of concepts.
- Access to Experienced Instructors: Students can benefit from direct interaction with knowledgeable faculty who can provide insights and personalized feedback on assignments.
- Networking Opportunities: Engaging in a university environment allows students to connect with peers and industry professionals, possibly leading to job opportunities.
When considering university offerings, it is advantageous to review the course outlines and specific outcomes of each program. Look for programs that continue to evolve and stay current with industry trends, thus ensuring relevance in a fast-paced technological landscape.
Suggested Course Structures
Creating a structured approach to learning Haskell is critical for both novice and experienced programmers. Organizing course content not only facilitates the learning process but also enables educators to deliver complex material in an understandable manner. A well-planned course structure allows learners to gradually build their understanding, gaining necessary skills at each stage while keeping them engaged with the material.
When designing Haskell courses, it is essential to consider the target audience. Different learners will benefit from varying structures. Here, we will explore three key levels: beginner, intermediate, and advanced. Each level will include specific goals, topics, and projects that correspond to the learner's aptitude.
Beginner's Course Outline
A beginner's course should focus on the foundational aspects of Haskell. The objective is to make students comfortable with the basic syntax and core concepts. Key topics might include:
- Introduction to Haskell's syntax and tools
- Understanding data types and data structures
- Writing simple functions and exploring pure functions
- Exploring the concept of lists and tuples
- Introduction to recursion
- Basic input and output
Hands-on projects could involve creating simple applications, such as a calculator, that reinforce these concepts. This solid groundwork prepares learners for more complex topics ahead.
Intermediate-Level Course Elements
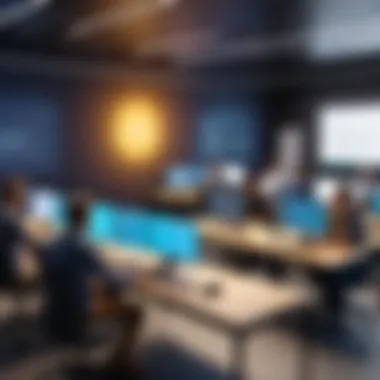
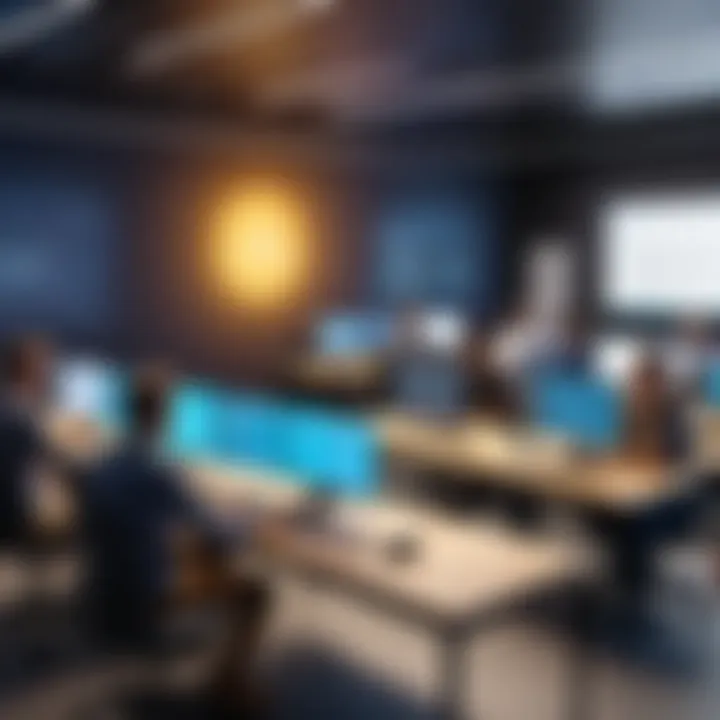
Once a solid foundation is established, learners can progress to intermediate content. This level introduces more advanced concepts, enhancing their understanding and practical application of the language. Important topics for an intermediate course may involve:
- Higher-order functions and their applications
- Understanding lazy evaluation and its implications
- Utilizing modules and libraries
- Writing type-safe code with type classes
- Error handling and debugging techniques
Intermediate projects could include developing a small web application or a basic data processing tool. Such projects will help bridge the gap between theory and real-world application, making learning more relevant.
Advanced Course Topics
For those who have mastered the intermediate level, an advanced course should cater to those looking to deepen their expertise. This would cover complex ideas, trends, and developments in Haskell. Topics to explore might include:
- Advanced type system features, such as GADTs
- Exploring functional reactive programming
- Performance tuning and optimization techniques
- Interfacing with C and other languages
- Real-world case studies of Haskell in production
Capstone projects at this level should challenge learners to tackle more significant or unstructured problems, potentially involving collaboration with peers. Such collaborative efforts mimic real-world programming environments and foster teamwork skills.
"Structured learning is essential for mastery. The way knowledge is organized impacts how effectively it is absorbed."
In summary, each course structure serves to create a comprehensive roadmap for learning Haskell. This structure not only maintains a logical progression but also enables a focus on real-world application, making the learning experience duly relevant.
Common Challenges in Learning Haskell
Learning Haskell can present several challenges, even for those with programming backgrounds. Understanding these challenges is crucial for both learners and instructors. Acknowledging potential hurdles can lead to improved study habits and course designs. Haskell's unique features often contribute to its steep learning curve, which necessitates a solid foundation in functional programming concepts. Recognizing these issues may help learners avoid frustration and better appreciate the language's benefits.
Understanding Abstract Concepts
Haskell relies heavily on abstract concepts. This can be daunting for many beginners. Functions are first-class citizens in Haskell, meaning they can be passed around like data. However, grasping this idea alongside other concepts, such as higher-order functions, can be troublesome. For instance, when learners try to wrap their heads around the idea of functions that accept other functions as arguments, confusion often ensues.
- Recommendation: Take time to study basic functional programming before jumping into Haskell.
- Practice: Coding exercises focused on pure functions can help.
Focusing on practical examples can aid in appreciating how abstract concepts are applied. It helps to gradually build a mental model by engaging with real-world examples. For instance, think of a simple function that maps over a list. This type of interaction with functions can clarify their roles and usages.
Debugging and Error Handling
Debugging in Haskell is distinct from many imperative languages. This can be a shock for programmers accustomed to languages like C++ or Java. Haskell's static type system aims to catch many errors at compile time. While this reduces runtime errors, it may confuse beginners when they encounter compile-time errors.
- Common Issues: Type mismatches and missing function arguments are common stumbling blocks.
- Tip: Review error messages carefully; they can be informative when understood correctly.
Another challenge is the concept of monads, often seen as a necessary evil. Many beginners find it unintuitive. However, mastering monads is crucial for effective Haskell programming, especially for managing side effects. The earlier one embraces this concept, the smoother their Haskell journey will be.
"A good understanding of debugging and error handling can turn initial frustrations into learning opportunities."
Practical Applications of Haskell
Understanding the practical applications of Haskell is vital for those looking to harness the power of functional programming. Haskell offers a range of benefits in various domains, making it a competitive language in specific tasks such as web development, data analysis, and even artificial intelligence. By exploring these applications, learners can appreciate the significance of Haskell, both in academic settings and industry contexts.
Web Development
Haskell's role in web development highlights its suitability for building robust applications. The language's emphasis on purity and type safety leads to fewer runtime errors and contributes to more maintainable code. Many developers select Haskell for backend development due to frameworks like Yesod and Servant, which simplify the process of creating web applications.
Benefits of using Haskell in web development include:
- Type Safety: Haskell's strong static typing helps to catch bugs at compile time, which reduces the risk of crashes in production.
- Concurrency: Haskell has built-in support for lightweight threads and asynchronous programming, making it efficient for handling multiple connections.
- Functional Reactive Programming: Haskell's functional nature allows developers to approach web applications in a more declarative fashion, improving code organization.
However, there are considerations to keep in mind. The learning curve may be steep for those not familiar with functional programming concepts. Moreover, the ecosystem may not be as vast as other languages like JavaScript, which can lead to challenges in finding libraries or tools for certain tasks.
Data Analysis and Processing
Haskell is an excellent choice for data analysis and processing. Its ability to handle large datasets and perform complex operations efficiently makes it appealing for statisticians and analysts. The language offers libraries such as HMatrix and Statistics that simplify numerical computations.
Key advantages of Haskell in this domain include:
- Lazy Evaluation: Haskell's lazy evaluation allows for processing of large datasets without consuming memory resources unnecessarily. This is particularly useful in scenarios where not all data needs to be loaded at once.
- Expressive Syntax: Haskellās syntax facilitates clear and concise representation of algorithms, making it easier for practitioners to implement and understand complex analysis.
- Immutable Data: Immutability ensures that data cannot be altered inadvertently, promoting safer manipulation of data during analysis processes.
Despite these benefits, potential users should be cautious. The abstractions that Haskell offers can sometimes lead to performance issues if not properly managed. Additionally, those moving from more imperative paradigms may find some approaches counterintuitive until they adapt to Haskellās way of doing things.
Haskell's distinct advantages in both web development and data analysis underline its potential for developers and data scientists alike, facilitating the creation of reliable and efficient applications.
Building a Haskell Portfolio
Building a Haskell portfolio is crucial in showcasing your skills and proficiency in this functional programming language. A well-structured portfolio not only demonstrates your understanding of Haskell but also highlights your ability to apply its concepts in real-world scenarios. In a competitive job market, presenting your work effectively can set you apart from others.
Importance of a Haskell Portfolio
Creating a portfolio centered around Haskell projects displays your commitment to mastering the language. It reflects your problem-solving aptitude and willingness to tackle complex programming challenges. A strong portfolio can open doors to opportunities in various software development fields, especially those emphasizing functional programming.
Key Elements to Include
Here are some critical elements to consider when building your Haskell portfolio:
- Projects: Showcase a diverse range of projects that demonstrate different aspects of Haskell. These could include web applications, data analysis tools, or even contributions to open-source projects.
- Code Samples: Include well-commented code snippets that exhibit your coding style and understanding of Haskell's unique features such as lazy evaluation and type inference. Ensure that the code is clean and easy to read.
- Documentation: Provide thorough documentation for each project. This should include an overview, functionalities, setup instructions, and any challenges faced during development. Clear documentation shows professionalism.
- Technical Blog/Articles: If you enjoy writing, consider maintaining a blog where you discuss Haskell topics, tutorials, or project experiences. This is a great way to share knowledge and establish yourself as an authority in the Haskell community.
- Community Engagement: Highlight any participation in Haskell forums, workshops, or conferences. Speaks volumes about your commitment to learning and growing within the community.
Benefits of a Well-Constructed Portfolio
- Job Readiness: A robust portfolio can make a significant impact during job interviews, as it serves as a practical illustration of your capabilities.
- Networking Opportunities: Engaging in community discussions can lead to connections with other developers who can provide valuable insights, mentorship, or job referrals.
- Skill Refinement: As you work on projects for your portfolio, you will continuously improve your Haskell skills, deepening your understanding of functional programming concepts.
A portfolio is not just a collection of work; it is a reflection of your journey and expertise in programming.
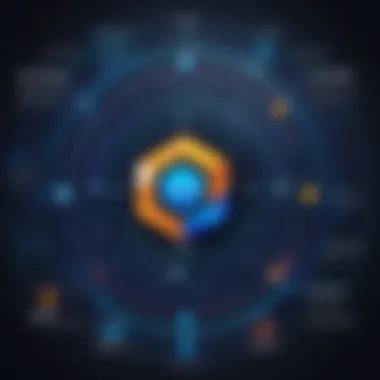
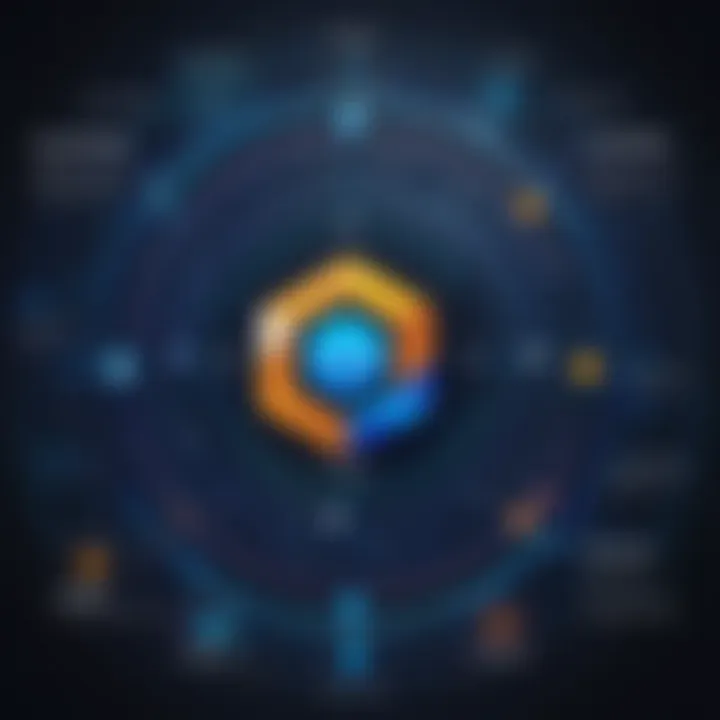
Considerations for Portfolio Development
Keep these considerations in mind while working on your Haskell portfolio:
- Updates: Regularly update your portfolio with new projects and improvements to existing ones to keep it relevant.
- Feedback: Seek feedback from peers or mentors, as they can provide valuable insights for better presentation or content.
- Personalization: Make your portfolio represent your unique style and interests within the Haskell language. This helps you stand out amongst your peers.
Community Resources and Support
Community resources and support play a crucial role in the Haskell programming journey. As learners progress through their studies, having access to a supportive community can significantly enhance their understanding and skill development. Learning a programming language can occasionally feel isolating, particularly when confronted with complex concepts inherent to Haskell, such as monads or type systems. Engaging with a community allows learners to share experiences, seek advice, and gain insights from others who have traversed similar learning paths. Furthermore, these resources can help create accountability, motivating learners to persist through challenges.
Online Forums
Online forums are a wealth of information for anyone interested in Haskell. These include platforms like Reddit's r/haskell, Stack Overflow, and dedicated Haskell forums. They facilitate exchanges of ideas and solutions to problems. In these spaces, individuals can post questions, share code snippets, and receive feedback from more experienced developers. This immediate access to a global network of Haskell enthusiasts fosters an environment of learning beyond just formal education.
Some benefits of participating in online forums include:
- Quick resolution of queries: You can gain immediate support for programming issues you encounter.
- Learning from others' experiences: Seeing how others tackle similar challenges can provide valuable learning moments.
- Building a network: Engaging in discussions can lead to connections with other programmers and professionals.
Meetups and Workshops
Meetups and workshops offer another avenue for Haskell enthusiasts to connect and learn. These events can range from small programming meetups in local areas to larger workshops hosted at technology conferences. They provide a face-to-face platform to discuss Haskell, engage in coding sessions, and even partake in hackathons.
Participating in such gatherings can be beneficial in multiple ways:
- Networking opportunities: Meeting other programmers allows for relationship building that could lead to collaborative projects or job opportunities.
- Hands-on experience: Workshops often include practical exercises that can enhance learning significantly as users apply concepts in real-time.
- Exposure to experts: Many events feature talks or presentations from seasoned Haskell developers, offering insights that are not typically found in textbooks.
Meetups and workshops are instrumental in solidifying the theoretical knowledge gained through courses by providing practical applications and community engagement.
"Communities can enhance your programming skills, connecting you with valuable resources, inspiration, and support as you learn Haskell."
By leveraging these community resources and avenues for support, learners can not only enhance their skills but also find encouragement and motivation that can help them advance their Haskell programming journey.
Assessing Your Progress in Haskell Learning
Evaluating your advancement in Haskell programming is crucial for anyone wanting to deepen their skills. By understanding how to assess your progress, you can identify strengths and weaknesses systematically. This approach helps refine your learning strategy over time.
One significant benefit of self-assessment is fostering an awareness of your competencies. It allows you to recognize which concepts, modules, or areas you grasp well and which ones require further study. This recognition is not just about passing tests or completing courses. It's about reflecting on how well you can apply Haskell in practical scenarios.
Key Considerations for Assessment
When assessing your progress, consider the following:
- Set Clear Goals: Define what you wish to achieve with Haskell. It can be working on specific projects or mastering particular concepts.
- Utilize Resources Effectively: Engage with materials such as textbooks, online courses, and coding exercises. Each resource may offer different insights.
- Practice Regularly: Learning programming languages requires consistent practice. Regular coding helps reinforce concepts.
- Seek Feedback: Engage with peers or mentors who can provide constructive criticism. Participating in coding communities on platforms like reddit.com can be beneficial.
Tools for Self-Assessment
Here are some effective tools for evaluating your knowledge:
- Quizzes and Exercises: Online quizzes can help gauge your understanding of key Haskell concepts. Websites often provide exercises tailored to various skill levels.
- Personal Projects: Building a project tests your ability to apply what you've learned. It showcases your practical skills and can highlight areas needing improvement.
- Code Reviews: Sharing your code with others allows for feedback. Their insights can help identify best practices you might overlook.
Final Thoughts
"Regular assessment is a pathway to mastery. The goal is to keep learning, iterating, and improving."
Future of Haskell and Functional Programming
The evolution of Haskell and functional programming is a topic of great importance in the broader landscape of software development. As technology continues to advance, so does the demand for languages that facilitate better problem-solving and easier maintenance of complex systems. Haskell stands out because of its strong focus on functional programming principles, offering features that can lead to more robust and error-free code.
The Continued Relevance of Haskell
Haskellās strict typing system promotes correctness at compile-time. This is increasingly valuable in large applications where bugs can be costly. As organizations recognize the benefits of rigorous type systems, there might be a rise in Haskell's adoption, particularly in sectors where safety and correctness are paramount, such as finance and aerospace. The interest in functional programming paradigms has gained momentum in recent years. More developers are appreciating concepts such as immutability, higher-order functions, and lazy evaluationāprinciples well-embedded in Haskell's design.
Emerging Trends and Technologies
With the rise of concurrent and distributed systems, Haskellās capabilities come to the forefront. The languageās model for dealing with side effects and its non-blocking I/O functions make it particularly suited for modern applications. Additionally, the integration of Haskell with other languages and technologies can expand its reach. Tools like the GHCJS compiler allow Haskell to be used for web development, which can attract a diverse group of developers looking for effective solutions in this domain.
Learning and Community Support
The Haskell community plays a key role in its future. A vibrant community not only supports learners through forums and open-source projects but also ensures the language remains relevant by continually pushing its boundaries. Many educational resources are now available, allowing new programmers to learn Haskell more easily than before. For aspiring programmers, engaging with community resources can make a significant difference.
Considerations for The Future
While Haskell offers many advantages, there are also challenges to its broader adoption. The steep learning curve can deter newcomers. As a result, it is essential to create more interactive and beginner-friendly learning environments. Increased visibility through university courses and online platforms can also help. It will be critical for Haskell to maintain its evolution while simultaneously addressing the practical needs of developers.
"The future of Haskell is closely tied to the balance between its unique academic strengths and real-world applications"
Finale
In any educational journey, the conclusion acts as a critical reflection point. This holds true for our exploration of Haskell programming courses, which encompass various aspects from learning methodologies to practical applications. In this, we can synthesize key insights that not only clarify the significance of Haskell but also underscore the benefits of a structured approach to mastering it.
Haskell programming offers unique paradigms that differ from traditional imperative programming. Understanding these paradigms can elevate your programming proficiency, especially in functional programming. Knowing Haskell means embracing concepts like pure functions, lazy evaluation, and type inference, all of which sharpen your problem-solving skills. The tailored teaching approaches found in comprehensive Haskell courses ensure that learners of all levels can engage meaningfully with these concepts.
Key Benefits to Consider:
- Logical Thinking: Learning Haskell enhances your logical reasoning, as it requires a mindset shift towards mathematical functions.
- Software Quality: Haskellās strong type system aids in reducing bugs, ultimately leading to robust software development practices.
- Career Potential: Proficiency in Haskell opens doors to opportunities in niche markets such as data science and financial modeling, where functional programming skills are highly valued.
Additionally, community resources and related courses provide essential support. Engaging with forums and attending workshops helps bridge the gap between theoretical knowledge and practical application. As the ecosystem of Haskell grows, so do the avenues for learning and experimentation.
"Knowledge of Haskell can profoundly impact a developer's ability to think abstractly and solve complex problems."
Ultimately, a well-structured Haskell programming course not only imparts valuable skills but also cultivates a deeper appreciation for functional programming as a whole. The concluding stage reaffirms the importance of continual learning in both Haskell and technology at large, suggesting pathways for further exploration beyond the initial course. With this foundation, the journey into Haskell programming can transition from novice to expert, enriching both the individual and the broader tech landscape.