A Comprehensive Guide to Java for Beginners
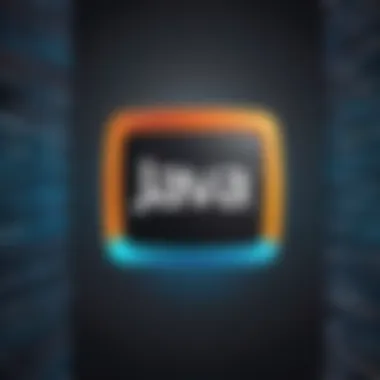
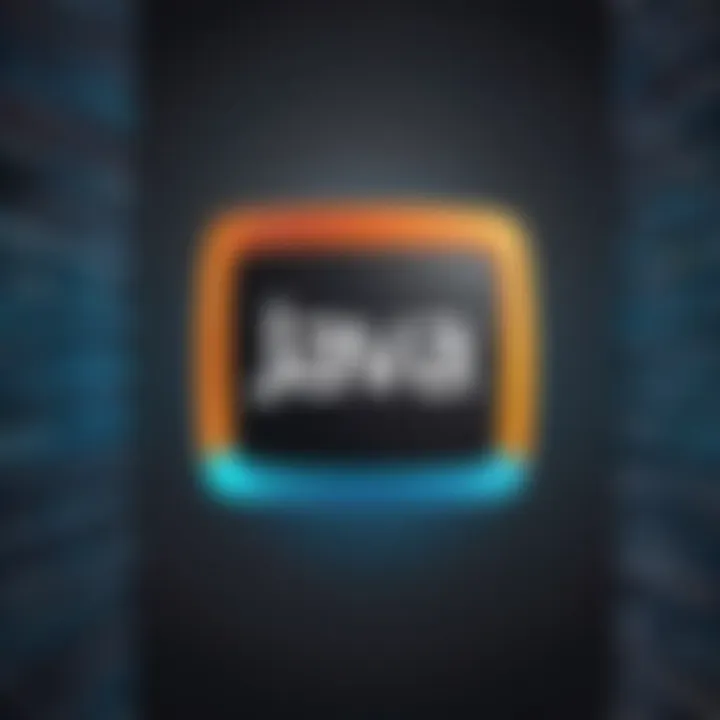
Intro
Java, a longstanding contender in the world of programming languages, remains a popular choice for many beginners looking to dip their toes into the coding waters. As the saying goes, "you have to walk before you can run," and so understanding Java is often the first step in a budding programmer's journey.
This guide aims to illuminate the path ahead, offering a detailed exploration of the Java programming language. Whether you're looking to build mobile apps, develop web-based applications, or make your mark in enterprise-level solutions, grasping Javaās core concepts is indispensable in todayās tech landscape.
In this guide, we will cover essential concepts, familiar tools, best practices, and resources. By blending theoretical knowledge with practical exercises, youāll gain a solid grounding in Java, setting you on a sustainable coding career.
"Learning Java is not just about writing code; itās about understanding how to think logically and solve problems."
As we delve deeper, you'll find intriguing insights and approaches that can foster your growth as a programmer, making it a worthwhile read for both novices and those with some programming experience.
With this as a backdrop, letās jump right into some engaging Coding Challenges.
Prolusion to Java
Java stands as one of the cornerstones of modern programming languages. For those taking their first steps into the world of code, grasping Java is not just about learning syntax; itās about familiarizing oneself with a language that powers a vast swath of applicationsāfrom enterprise systems to mobile apps. Understanding Java not only provides a solid foundation in programming principles but also introduces the learner to an ecosystem rich with tools and libraries.
What Is Java?
At its core, Java is a high-level, object-oriented programming language, originally released by Sun Microsystems in 1995. It was built with the philosophy of āwrite once, run anywhere,ā meaning that Java code can run on any device equipped with a Java Virtual Machine (JVM). This powerful feature makes Java incredibly versatile, giving rise to cross-platform capabilities that many languages struggle to achieve.
Java inherits many features from its predecessors, including C and C++, while omitting some of the complexity associated with those languages. It uses a simple syntax that promotes understanding, making it easier for beginners to pick up.
In addition, its robust API and community support render it ideal for applications ranging from web development to complex software systems. In simple terms, if youāre looking for a reliable language to start your programming journey, Java fits the bill.
Importance of Learning Java
Learning Java holds significance for various reasons. Here are some key points:
- Market Demand: Java consistently ranks as one of the most in-demand programming languages. Major companies, including Google, LinkedIn, and Amazon, rely on Java for their back-end operations. Job seekers often list Java skills prominently on their resumes, and many tech roles require a strong understanding of the language.
- Object-Oriented Paradigm: Javaās object-oriented structure promotes good programming practices like encapsulation and abstraction. Understanding these concepts can help you design more robust software, ultimately paving the way for advancement in your career.
- Rich Ecosystem: By learning Java, you're essentially gaining access to a wealth of frameworks such as Spring and Hibernate, as well as tools for build management like Maven and Gradle. This ecosystem not only simplifies development but also enhances productivity.
- Cross-Platform Compatibility: With Javaās ability to run on various devices and operating systems, youāre not locked into a specific environment. This flexibility is a significant advantage in todayās multi-device world.
"Java is to software what bread is to the meal. It's everywhere, indispensable, and can be a great start for anyone looking to get their hands dirty in programming."
Embracing Java is a step into a world of opportunities. As you navigate through this guide, take to heart that the skills and concepts you acquire will remain relevant and beneficial in a rapidly evolving tech landscape.
Setting Up the Development Environment
Setting up the development environment is a crucial first step in learning Java. This section ensures you have the necessary tools and configurations at your fingertips, which significantly aids in your journey from novice to proficient coder. An appropriate development environment can help you streamline the coding process and promote an efficient learning experience. When considering the vast world of Java programming, having the right setup can make a world of difference in how you understand and interact with the language. Here, we will explore how to choose an IDE, install the JDK, and configure your environment variables.
Choosing an IDE
Selecting the right Integrated Development Environment (IDE) forms the backbone of your Java programming experience. An IDE is essentially a software application that provides comprehensive facilities to programmers for software development. The right choice can make coding feel like second nature, while the wrong one may leave you grappling with frustration. Popular IDE options include Eclipse, IntelliJ IDEA, and NetBeans. Each has its own set of unique features that cater to different needs.
When choosing an IDE, consider the following factors:
- User-Friendly Interface: You want something that doesn't overwhelm you with options or settings right off the bat.
- Code Completion and Suggestions: This can help save time and reduce errors, especially when starting.
- Debugging Tools: These features can be invaluable for tracking down errors in your code.
- Community Support: A well-supported IDE gives you access to a wealth of resources and troubleshooting help.
In the end, the best IDE is one that feels comfortable for you to work with. Many developers suggest trying out a few different options to see which one resonates the most.
Installing the JDK
The Java Development Kit (JDK) is indispensable for anyone looking to code in Java. It includes the tools necessary to create Java applications, such as the Java Runtime Environment (JRE), the Java compiler, and various libraries.
Follow these steps to install the JDK:
- Download: Go to the official Oracle website to download the latest version of the JDK. If you prefer open-source options, you can also consider OpenJDK.
- Install: Running the installation file is straightforward. Be sure to follow the prompts and remember the installation path for later use.
- Verify Installation: Once installed, you can confirm it by opening your command line and typing . This should display the installed version of Java.
Hereās a quick command you might need to run:
"The right tools open the door to improvements and discoveries you might not have considered before."
Configuring Environment Variables
After installing the JDK, configuring the environment variables is essential for your system to recognize Java commands. It may sound technical, but itās fairly simple once you get the hang of it.
To set up environment variables, follow these steps:
- Access Environment Variables: Depending on your operating system, navigate to the System Properties. On Windows, you can find this by searching for "Environment Variables" in the Control Panel.
- Edit Path Variable: Locate the āPathā variable in the system variables section. Add the directory of the JDK installation (e.g., ). This allows you to run Java commands from any command line.
- JAVA_HOME Variable: Create a new variable named and set its value to the JDK installation path (e.g., ). This is often used by various Java applications to locate your JDK.
Setting these environment variables correctly ensures that your system is prepared to handle Java applications seamlessly, paving the way for a smoother development experience.
Core Java Concepts
Understanding Core Java Concepts is akin to grasping the very foundations upon which the edifice of Java programming is built. These core elements not only define the structure of Java but also enhance your ability to write code that is both efficient and effective. Mastery of these concepts is essential for any budding programmer seeking to thrive in the vast universe of software development. Not only does it lay a solid groundwork, but it also aids in navigating the complex layers of the Java ecosystem as one ventures into more advanced topics.
Variables and Data Types
Variables in Java act as containers that store data values. The importance of choosing the right type of variable cannot be overstated, as it directly influences memory usage and the functionality of your Java application. Each variable type has its own scope and functionality, making it crucial to understand how they work together.
For instance, consider these primary data types in Java:
- int: for integers
- double: for decimal numbers
- char: for single characters
- boolean: for true/false values
Each variable type is optimized for specific kinds of operations. For example, is ideal for counting purposes, while is essential when working with binary states.
Control Flow Statements
Control flow statements direct the processing of Java code based on certain conditions. They are critical for increasing the dynamism of your applications. Letās delve into the three primary control flow constructs.
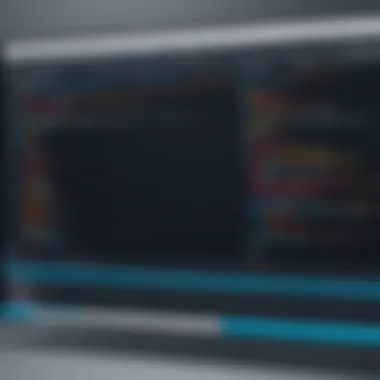
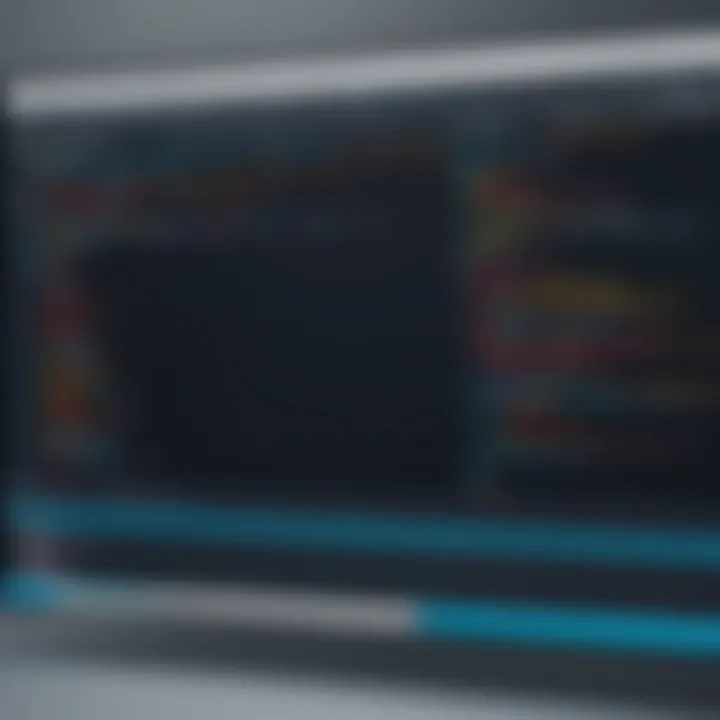
if Statements
If statements check specific conditions to determine the path of execution within your program. The hallmark of an if statement is its ability to make decisions, which is foundational in programming. This characteristic makes it an indispensable element when implementing logic in applications.
The advantage of using if statements comes from their theoretical simplicity and practical ability to encapsulate clarity. However, they can create complicated structures when excessive nesting occurs, leading to what some call "spaghetti code." Yet, with thoughtful application, they remain a powerful tool in a programmer's arsenal.
Switch Case
The switch case statement serves as a cleaner alternative to if statements when dealing with multiple potential values for a single variable. Its primary draw is simplicity when compared to a string of if statements, allowing for cleaner code and easier readability.
This structure helps reduce the risk of errors and streamlines the flow of execution. However, it is important to note that switch cases are generally less flexible than if statements since they evaluate single variables, which could limit broader conditional logic situations.
Loops
Loops, crucial in their capability to execute a block of code repeatedly based on a given condition, provide an efficient way of handling repetitive tasks. Java presents several types of loops, such as for, while, and do-while loops, catering to different use cases.
- for loop: best suited for situations with a definite number of iterations.
- while loop: ideal for choices made at runtime, letting you drive the loop based on varying conditions.
- do-while loop: ensures code execution at least once, as the condition is checked after the execution of the loop block.
The benefit of employing loops is their efficiency in manipulating large data sets or when repeated operations are required. Nonetheless, careless use can lead to infinite loops, which can crash your programāa reminder of the importance of solid condition checks.
Methods
Methods, in many ways, encapsulate the power of reusability in Java programming. They allow developers to define specific tasks under a single name, simplifying code management and execution.
Every method is characterized by its signature, which includes its name, return type, and parameters. Using methods, you can segment your code into manageable chunks, making the program easier to debug and maintain.
Object-Oriented Programming in Java
Understanding Object-Oriented Programming, or OOP for short, is akin to learning the very principles that govern not just Java, but many languages in the programming realm. OOP is key to Java's design, allowing developers to model real-world entities and boost code reusability, making life a whole lot easier when it comes to managing complex systems.
From a high-level view, OOP encourages a method of organizing software that mirrors the intricacies of real-life interactions. It's about representing concepts through objects that possess both state (attributes) and behavior (methods). This organizational strategy is not just a fancy idea; it's a practical approach that can improve the maintainability and scalability of applications significantly.
Classes and Objects
At the heart of OOP in Java lies the notion of classes and objects. A class can be thought of as a blueprint or a template. It's a definition that outlines properties and behaviors that the objects (manifestations of the class) will have. For instance, if we were to define a class , it might contain properties such as , , and , along with methods like and . Here's a quick look at how a simple class is structured:
When you create an instance of this class, you're sculpting an object based on its design, like modeling a real dog that can bark and display its characteristics.
This setup boosts code reusability because you can create multiple objects without rewriting the properties or methods. Each object can hold different data, in terms of breed, color, and so on, while sharing the same methods defined in their class.
Encapsulation, Inheritance, and Polymorphism
Diving deeper, we come across encapsulation, inheritance, and polymorphism, often dubbed the pillars of OOP.
- Encapsulation is about bundling the data (attributes) and methods that operate on the data within one unit, the class, and restricting access to some of the object's components. This is often enforced using access modifiers like , , and . For example, by declaring properties as private, direct access can be limited, which leads to safer and more secure code.
- Inheritance allows one class to inherit fields and methods from another, promoting a hierarchical classification of classes. For instance, if we have a base class , we can have subclasses like and , which inherit common features from while also introducing their own unique characteristics. This saves time and reduces redundancy.
- Polymorphism is the capability to present the same interface for differing data types. Basically, it lets us call the same method on different objects but see different behaviors based on their class. In simpler terms, itās like having a standard command that performs specific actions depending on what object itās dealing with.
Abstract Classes and Interfaces
Lastly, we must touch on abstract classes and interfacesāboth of which elevate your grasp of OOP in Java.
An abstract class canāt be directly instantiated. It typically contains one or more abstract methods which are declared without an implementation. Consider this useful when you want to provide a common template for derived classes. Hereās a brief illustration:
Any subclass of like or would have to provide an implementation for , enforcing a contract that all animals must conform to a behavior.
On the other hand, an interface is a completely abstract class that allows you to define a contract without getting into the nitty-gritty of implementation. When a class implements an interface, it agrees to perform specific behaviors.
"Interfaces are a powerful way to establish a form of contract for your classes, leading to highly flexible and maintainable code."
By utilizing both abstract classes and interfaces, you can build a robust architecture thatās not just clean but also conducive to agile development and future enhancements.
In summary, delving into Object-Oriented Programming in Java lays the foundation for strategic programming approaches that enhance the conceptual understanding of your software architecture. From optimizing your coding practices to embracing a mindset of reusability and maintainability, OOP is a key player in sharpening your Java skills.
Exception Handling
Handling exceptions in Java is akin to preparing for the unforeseen challenges that pop up in any project or journey. Just as one would anticipate potential roadblocks when setting out on a trip, Java programmers must be ready to tackle unexpected issues that arise during code execution. Proper exception management is crucial in building robust applications where errors can be gracefully caught and resolved, rather than crashing the program outright.
In the realm of programming, exceptions are like uninvited guests; they're always around, but how you deal with them significantly influences the experience and final results. Java's exception handling framework provides the necessary tools to catch these errors and respond to them appropriately. This skill not only enhances your coding capabilities but also leads to better application performance and user satisfaction.
What Is Exception Handling?
At its core, exception handling refers to the method of managing errors that occur during program execution. When the Java Virtual Machine (JVM) encounters an error it cannot handle, it throws an exception. An exception can happen due to various reasonsāsuch as trying to access a resource that doesnāt exist, performing operations that are not allowed, or running out of memory, among others.
To put it simply, if you think of a program as a tightly run business, exceptions represent the mishaps and mistakes that can lead to disruptions. A good exception handling strategy is akin to having a crisis management plan in place. It allows developers to define how to respond to different types of errors and ensure the program can either recover from the issue or at least inform the user in a user-friendly manner. By implementing such practices, you begin to foster a code environment that respects both performance and user experience.
Types of Exceptions
In Java, exceptions fall into two primary categories: checked and unchecked exceptions.
- Checked Exceptions: These types of exceptions are checked at compile time, meaning the programmer is required to handle them in the code. Common checked exceptions include , , and . If your program has the potential to throw a checked exception, you mandate that proper handling (like using a try-catch block) be in place before the code can compile.
- Unchecked Exceptions: Unlike checked exceptions, these are not checked at compile time; they occur during program execution and typically stem from coding mistakes. Common examples are , , and . To illustrate, if you try to divide a number by zero, youāll wind up with an .
"Itās not the fall that kills you, itās the sudden stop at the end." - Douglas Adams, which could well apply to exceptions in programming.
Acknowledging the differences between these types ensures you're well-prepped to handle them effectively.
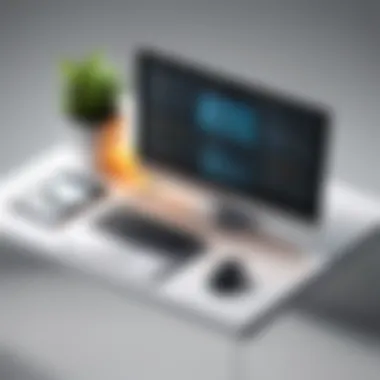
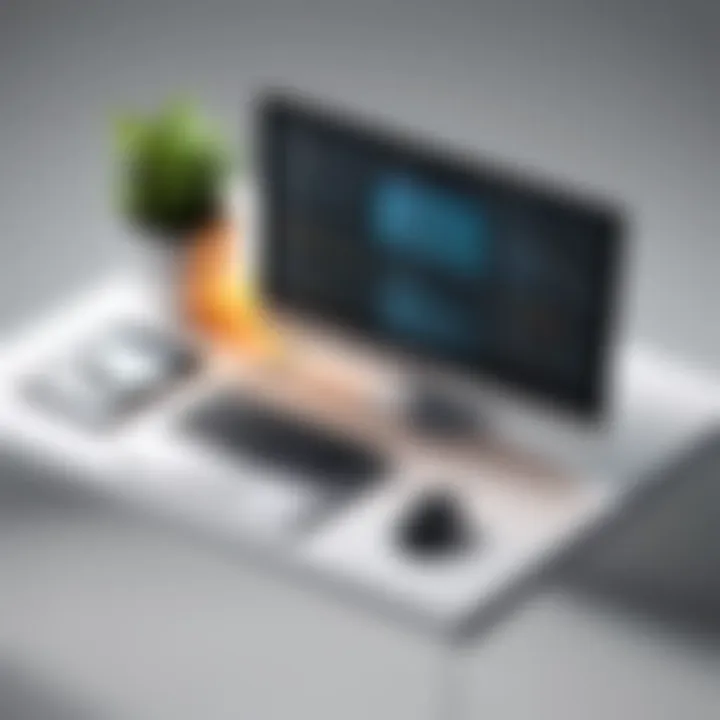
Using Try-Catch Blocks
In Java, the primary mechanism for managing exceptions is the try-catch block. This structure allows you to attempt to execute a piece of code while simultaneously keeping an eye out for exceptions. Here's how it generally works:
- The try block contains code that might throw an exception.
- The catch block follows the try, allowing a programmer to handle specific exceptions if they occur.
Hereās a simple example:
In this snippet, the program tries to access an index of the array that doesnāt exist. Because this will trigger an exception, the catch block steps in, preventing the program from crashing and instead providing a clear message informing about the error.
Using try-catch blocks proficiently can not only protect your applications from abrupt terminations but also enhance maintainability and user satisfaction. This practice ultimately helps in writing cleaner, more efficient programs that handle errors with grace.
By mastering exception handling, you set forth a frontline defense against the inevitable surprises of coding, allowing you as a developer to effectively manage the unpredictable nature of software development.
Java Collections Framework
In the realm of Java programming, the Collections Framework stands as a fundamental pillar, providing essential tools that allow developers to efficiently manage groups of objects. Understanding this framework is vital for anyone seeking to master Java. The Collections Framework offers various data structures that can be utilized to store, manipulate, and access data effectively. This is not merely about easier management; it also entails significant improvements in performance and scalability, which are crucial in todayās fast-paced tech environment.
Preamble to Collections
When we talk about collections in Java, we are referring to a set of classes and interfaces specifically designed to handle collections of objects. The major benefit of using collections is their ability to guarantee type safety at runtime while also offering dynamic sizing. Unlike traditional arrays that have a fixed size, collections can grow or shrink as the number of elements changes, making them far more flexible.
These collections run the gamut from lists and sets to maps, each type having its distinct characteristics and use cases.
For instance, if you need to maintain the order of elements, a could be your best bet. On the flip side, if uniqueness is paramount and you donāt care about order, a would serve better.
List, Set, and Map Interfaces
The Java Collections Framework breaks down into three primary interfaces: List, Set, and Map. Each of these interfaces has its purpose and is geared towards handling data in a specific way:
- List: This interface allows for an ordered collection of elements. Common implementations include and . These are excellent when you need to maintain the order of insertion and allow duplicates.
- Set: A is designed to store unique elements. This means that adding the same item multiple times wonāt have any effect. Implementations like and serve various needs based on performance and order.
- Map: Unlike the previous two, a holds key-value pairs. Keys must be unique, while values can be duplicated. This interface is crucial for scenarios where quick lookups based on keys are required. Common implementations include and .
Common Implementations
The effectiveness of the Collections Framework is largely attributed to its implementations, which are tailored for specific use cases. Here are some common implementations worth noting:
- ArrayList: Recommended when you need fast random access to elements. Itās backed by a resizable array and has a low overhead for accessing elements, though adding or removing from the middle can be slow due to shifting.
- LinkedList: Best for applications requiring frequent insertions and deletions from the middle of the list, as it uses a doubly-linked list structure that allows for quicker rearrangements.
- HashSet: Offers constant-time performance for basic operations like add, remove, and contains, making it highly efficient for storing unique items.
- TreeSet: Maintains elements in sorted order, which makes it useful for operations that require ordering, but with a slightly higher lookup time when compared to HashSet.
- HashMap: Enables you to associate values with unique keys, allowing for efficient lookups. However, it does not maintain any order, which is a characteristic feature of maps that need to keep track of sorted entries.
These various implementations exemplify how the Collections Framework can adapt to the needs of different programming scenarios.
In summary, grasping the concept of the Java Collections Framework can empower programmers to handle data proficiently. Whether itās maintaining order, ensuring uniqueness, or enhancing performance through effective data structure choices, collections play a crucial role in building effective Java applications.
Java Development Tools and Libraries
In the world of Java programming, having the right tools and libraries can be a game changer. Dealing with complex projects, you are bound to run into situations where frameworks and build tools come to your rescue. Using effective tools not only eases up the coding process but also ensures your projects are well organized and maintainable. In this section, we will discuss some of the essential development tools and libraries that every Java developer should be familiar with.
Build Tools: Maven and Gradle
Build tools are crucial for streamlining the software development process. Maven and Gradle happen to be the most widely used in the Java ecosystem. These tools automate the build process, managing dependencies, and packaging applications.
Maven follows a more conventional XML configuration method. You define your project structure and dependencies in a file, and Maven takes care of the rest. For example, if you are working on a project that requires specific librariesāsay, the Apache Commons libraryāMaven automatically downloads the required versions by simply adding a couple of lines in your .
On the other hand, Gradle offers a more flexible and powerful build automation system with a groovy-based DSL. With Gradle, developers can orchestrate builds with fewer lines of code while maintaining clarity. Many prefer Gradle for large scale projects because of its flexibility and speed. For instance, in a large enterprise application, Gradle can handle multiple modules seamlessly.
"The right build tool can save you countless hours of headache and make coding a breeze."
Testing Frameworks: JUnit and Mockito
Testing is vital in software development, and in Java, two tools stand head and shoulders above the rest: JUnit and Mockito. Each serves a special purpose that complements the other.
JUnit is the de facto standard for unit testing in Java. It helps assess the performance of your code in isolation, ensuring that each component behaves as expected. To use JUnit, you simply create test classes annotated with , encapsulating the specific functionality you want to verify. For example, verifying a methodās expected output can be done as follows:
Then, there's Mockito, which specializes in creating mock objects. It allows developers to simulate the behavior of complex dependencies without needing to rely on the real implementation, thereby focusing on unit tests. For instance, if your class relies on an external API, using Mockito helps isolate tests by mocking that API call, making your tests faster and more reliable.
Popular Libraries and Frameworks
Javaās ecosystem is brimming with Libraries and frameworks that minimize boilerplate code and enhance productivity. Some of the most popular include Spring, Hibernate, and Apache Commons.
- Spring Framework simplifies the development of enterprise applications. Leveraging inversion of control and aspect-oriented programming, it provides a structured environment for building secure and scalable applications.
- Hibernate streamlines database operations. It abstracts the complexity of direct database interactions, allowing developers to interact with databases using high-level object-oriented constructs instead of SQL queries.
- Apache Commons is a collection of reusable Java components. From utilities for string manipulation to file operations, it saves hours that one would otherwise spend coding fundamental features.
Utilizing these tools effectively can take your Java development skills to the next level. The right mix of libraries and frameworks, combined with the proper testing and build tools, creates a robust environment that can handle even the most demanding programming tasks.
Best Practices in Java Programming
When delving into Java, embracing best practices can be a game-changer. These principles not only help in crafting solid code but also enhance maintainability, readability, and collaboration among teams. Good practices lay the pathway for scalable projects, turning haphazard code into efficient, well-structured solutions that can withstand the test of time.
Code Organization
An organized codebase is like a well-maintained library; it saves time and prevents headaches. When files and folders are structured logically, developers can navigate the project effortlessly, finding what they need without feeling lost in a maze of code.
- Follow a sensible folder structure: Divide your project into packages that logically group similar functionalities. For instance, place all user-related classes in a package and all order-related classes in an package.
- Use meaningful naming conventions: This applies to classes, methods, and variables. A name like is much clearer than , allowing others to instantly grasp its function.
- Keep methods short and focused: Ideally, a method should perform one task. If it starts to creep into other functionalities, it might be time to refactor.
These practices not only streamline your workflow but also make your code easier to hand off to others or revisit after some time.
Writing Readable Code
Readable code is not just about aesthetics; itās the backbone of productive programming. Code that flows clearly is easier to test, debug, and enhance. When they say "write it easy, so the next guy can understand it," they're onto something.
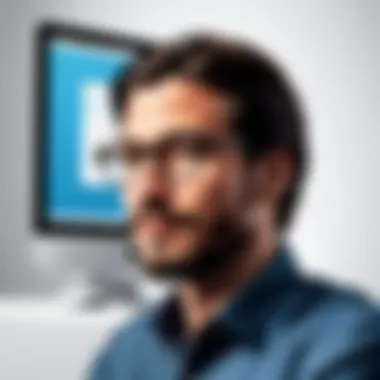
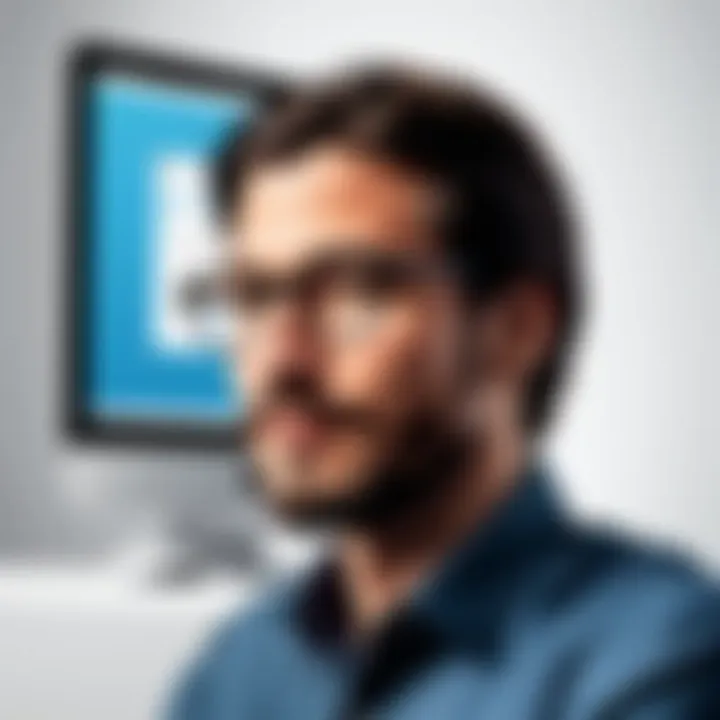
- Use comments judiciously: A well-placed comment can clarify complex logic. However, avoid redundancy; if your code is self-explanatory, thereās no need to explain it again.
- Consistent formatting: Stick to a consistent style for indentation, spacing, and brackets. Tools like Checkstyle can help enforce these styles, making the code more visually appealing.
- Avoid deep nesting: If your logic gets too deep, consider simplifying it through methods or helper classes. This leads to a cleaner structure and easier debugging.
Following these things will not just help you but also make life easier for those who step into your shoes later on.
Version Control with Git
In the realm of programming, version control has become an essential tool. As projects expand, keeping track of changes and collaborating with others can quickly turn chaotic without a system.
- Commit often and with purpose: Each commit should represent a meaningful change. A good commit message helps others understand what has been done and why.
- Branching strategy: Implementing a strategy such as Git Flow allows for effective management of features, fixes, and releases. Each branch serves a unique purpose, which keeps the master branch clean and deployable at all times.
- Code reviews: Encourage a culture of code reviews using tools like GitHub or Bitbucket. This ensures multiple eyes see the code before it gets merged into the main branch, promoting higher code quality.
Adopting these practices makes version control a powerful ally, enabling seamless collaboration and efficient project management.
Implementing best practices in Java programming leads to not just robust applications, but also a positive working culture that encourages learning and growth.
Resources for Further Learning
In the fast-evolving world of programming, especially with a language as versatile as Java, staying up-to-date is essential. The pathway to proficiency doesn't just hinge on coding; rather, it's intertwined with continual learning. The resources you choose can either be stepping stones or stumbling blocks. Hence, dedicating time to explore and utilize quality resources can significantly enhance your coding journey.
Online Courses and Tutorials
Online courses have burst onto the scene as a go-to solution for learning programming languages, and Java stands no exception. Platforms like Coursera, Udemy, and edX offer extensive tutorials that cater to all skill levels. They typically come with video lectures, hands-on projects, and quizzes to test your understanding.
- Benefits: The interactive nature of these courses keeps learners engaged. Moreover, they allow you to learn at your own paceāperfect for busy schedules.
- Key Considerations: When selecting a course, check for user reviews and the credentials of the instructors. A well-structured curriculum can make a noticeable difference in grasping concepts.
Books and E-Books
Books remain a timeless resource in learning any programming language. Several well-written texts can guide you through the intricacies of Java. Titles like "Effective Java" by Joshua Bloch and "Java: The Complete Reference" by Herbert Schildt are widely regarded as essential reads.
- Benefits: Books often provide deeper insights and methodologies that aren't as easily conveyed through video. The ability to book mark, highlight, and jot down notes in the margins can sometimes aid memory retention.
- Key Considerations: Look for recommendations from online forums or programming communities to find the most relevant texts. Before purchasing, a quick glance at reviews on sites like Amazon or Goodreads can offer clarity.
Developer Communities and Forums
Joining developer communities can be a game changer. Platforms like Reddit and Stack Overflow enable you to connect with fellow enthusiasts and seasoned professionals. There's a wealth of knowledge shared through discussions and Q&A threads that you wonāt find anywhere else.
"Itās not just about learning; itās about sharing. Teaching is the best way to solidify your understanding."
- Benefits: These forums provide support and encouragement during your learning process. They allow you to ask questions, receive feedback, and even collaborate on projects.
- Key Considerations: Ensure to engage in communities that align with your learning objectives. Avoid lurking too long; ask questions and contribute. It's a great way to build your network.
In summary, leveraging various resources can help you build a solid foundation in Java. Whether it's through online courses, books, or actively participating in forums, the choice is invaluable in shaping your programming journey.
Building Your First Java Application
Building your first Java application marks a significant milestone in your programming journey. It's the bridge between understanding the theory behind Java and applying that theory in practice. This section aims to arm you with knowledge on how to create a simple yet functional Java application, thus laying a solid foundation for your future projects. We will cover project setup, core functionality, and the process of compiling and running your application.
Project Setup and Structure
Before diving into coding, you need to set the stage properly. A well-structured project makes navigation easier and enhances maintainability. First, create a new directory for your Java project. Let's say you call it . Inside this folder, you'll have a dedicated place for your source code. You'll typically create a folder here where all your files will reside.
Here's a simple layout:
Within your folder, will contain your Java code. The naming of the file should match the main class declared inside it. This is a crucial discipline to follow, as it helps the Java compiler identify your entry point easily when executing your program.
Implementing Core Functionality
Now that the groundwork is laid, itās time to add some functionality to your application. Let's start small with a simple "Hello, World!" program. This often serves as the gateway for beginners into programming. Open your file and start typing the following code:
In the code above, we have defined a class named . The method is the entry point for any standalone Java application. Inside the method, the program prints "Hello, World!" to the console. Even though it's a small snippet, it embodies the essence of Java syntax and structure.
Compiling and Running Your Application
The final step of this journey is compiling and running your Java application. To compile your code, you will use the Java Compiler. Open your command line interface, navigate to the folder where your file resides, and execute the following command:
This command tells the compiler to look for the file and compile it. If no errors arise, it will create a file, which contains the bytecode that the Java Virtual Machine will understand. To execute your program, simply run:
If everything is set up properly, you should see "Hello, World!" printed in the console. Congratulations, you've written and run your first Java application! This process epitomizes how simple projects can serve as important exercises to grasp broader Java concepts.
Remember: The journey in software development is cumulative. The skills you cultivate with small projects will become invaluable as you tackle more complex applications. Stay persistent, and keep experimenting with your code.
In summary, building your first Java application enhances your exposure to essential programming practices and structures while cultivating a sense of accomplishment. As you grow your skills, you will discover how integral this simple process is to your overall development journey.
Ending
In this final section of the guide, we will take a moment to synthesize the knowledge we have covered, solidifying the vital information for both beginner and intermediate Java programmers. Concluding is not just about wrapping things up; it serves as a crucial reminder of the journey you've embarked upon.
Recap of Key Concepts
Throughout this guide, we've delved into various essential aspects of Java. Hereās a concise summary that encapsulates the core elements:
- Understanding Java: Recognizing Java's significance in the programming world lays the foundation for all subsequent learning.
- Core Java Concepts: Variables, data types, and control flow statements were dissected to instil a basic yet paramount understanding of Java syntax and logic.
- Object-Oriented Principles: The concept of classes and objects provided insight into Java's structure, emphasizing the principles of encapsulation, inheritance, and polymorphism.
- Exception Handling: We examined how to manage errors, an integral aspect of writing robust Java applications.
- Collections Framework: Familiarity with the various interfaces and their implementations ensures that you can handle data effectively.
- Development Tools: Tools such as Maven, Gradle, JUnit, and Mockito give programmers the necessary utilities for building, testing, and managing Java applications.
- Best Practices: Adhering to coding standards, keeping a clean project structure, and utilizing version control with Git are practices that signify professionalism in programming.
- Continuous Learning: The journey of a programmer never truly ends. Exploring additional resources will enhance your skills incrementally.
This recap serves as a springboard to deeper knowledge. A firm grasp of these concepts is critical not only for your growth as a developer but also for your credibility in the field.
The Path Forward in Java Programming
As you stand at the threshold of your programming journey, thereās a world of possibilities ahead. Here are some avenues to explore as you advance:
- Building Projects: Apply your skills by creating real-world applications. This not only solidifies your understanding but showcases your abilities to potential employers.
- Contributing to Open Source: Engage with open-source projects on platforms like GitHub. Itās an excellent way to gain experience and learn from seasoned developers.
- Networking: Join forums, social media groups, or local meetups focused on Java. Connections with like-minded individuals can lead to collaborations and mentorship.
- Expanding Your Knowledge: Dive into advanced topics like concurrency, JavaFX, Spring Framework, or microservices architecture which can open new paths in your career.
- Pursuing Certifications: Having certificates like Oracle Certified Associate can provide an edge when applying for jobs.
By following these paths, you'll be well on your way to becoming a proficient Java developer. Keep your curiosity alive, always willing to learn and grow. Java will continue evolving, and so should you.
"The best way to predict the future is to create it." - Peter Drucker