Top Java Coding Interview Questions for Automation Testing
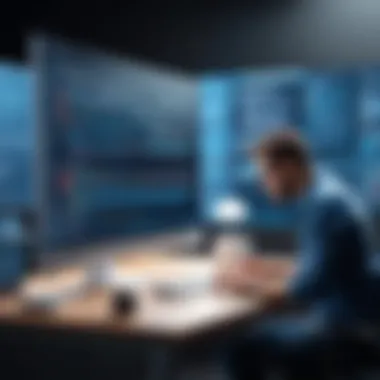
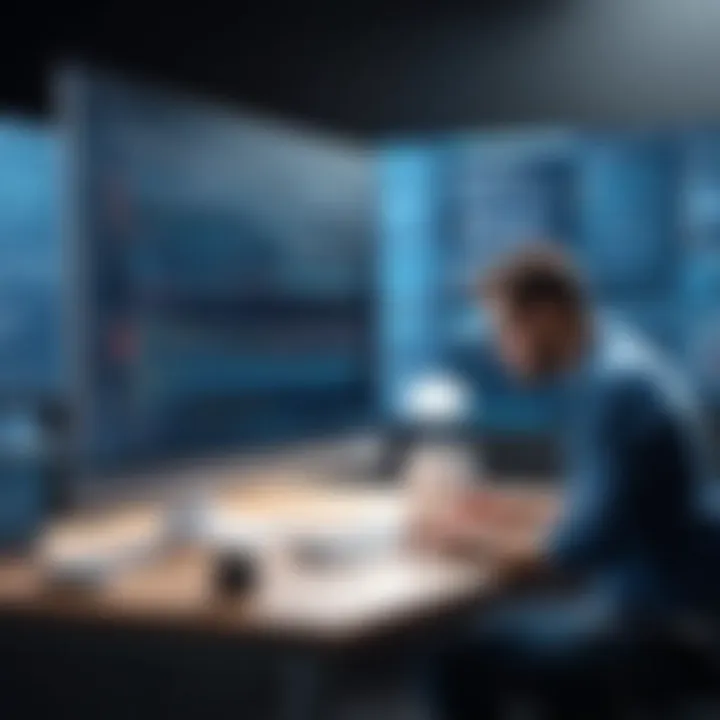
Intro
Preparing for a Java coding interview, especially in an automation testing context, can seem daunting. This process calls for not just familiarity with Java and its frameworks, but an understanding of various coding principles and methods applicable in automation testing.
In this guide, we focus on essential coding questions that address the requirements often seen in interviews. A strong foundation in Java concepts boosts confidence and allows candidates to face technical evaluations head-on. What's paramount here is not merely answering questions, but also grasping the underlying principles that drive those answers.
With real-world relevant problems, we cover principal concepts, key methodologies, and practical applications that prepare aspiring programmers and IT professionals for their future roles in automation testing.
Knowing how to tackle coding challenges is crucial, therefore our upcoming sections will specifically delve into coding challenges and the mindset necessary to approach them successfully.
Coding Challenges
In approaching coding interviews, engaging with coding challenges becomes mandatory for honing skills. These challenges simulate real job tasks. Candidates need to think critically and solve problems efficiently.
Weekly Coding Challenges
Regular practice through structured coding challenges helps maintain and enhance coding proficiency. Platforms like HackerRank, LeetCode, or Codewars offer a range of challenges, tailored specifically for automating testing problems and Java programming. Candidates may consider dedicating specific days each week to practice, boosting both speed and problem-solving skills.
Problem Solutions and Explanations
Having a repertoire of solved problems can provide candidates a strong edge. Look for solutions which explain not only the 'how' but also the 'why' of each approach. For example, consider this simple Java problem concerning string manipulation:
This example demonstrates the simplicity of string reversals, often reflecting a candidate's understanding of Java collections.
Tips and Strategies for Coding Challenges
To excel in coding challenges, the following tips may prove beneficial:
- Break the problem down into smaller components.
- Discuss potential solutions with others, exposing gaps in understanding.
- Focus on writing clean and efficient code.
- Time yourself while practicing to improve speed.
Regular review of algorithms ensures candidates stay sharp and prepared for any question's angle.
Community Participation Highlights
Engaging in coding communities can instill motivation and provide various perspectives. pursuing forums like Reddit and studying how others have tackled given challenges provides learning opportunities.
Active participation in such communities can lead to beneficial feedback and highlight common pitfalls.
Your journey through this preparation framework is iterative; consistency is key in seeking out further knowledge and refining skills relevant for automation testing roles.
Prolusion to Java in Automation Testing
Java has established itself as a fundamental language in the context of automation testing. Understanding Java is crucial because much of the industry's automation frameworks and tools rely heavily on it. When candidates appear for interviews, having a solid command of Java principles not only showcases technical skills but also indicates readiness to tackle real projects. This scrutiny into Java's role makes it clear why aspiring and experienced programmers need to prepare effectively for technical interviews.
The Role of Java in Automation
Java serves as the backbone for numerous automation frameworks, such as Selenium. The object's ability to be platform-independent through Java's Write Once, Run Anywhere (WORA) capability makes it suitable for automation testing across various environments. Java's syntax is relatively straightforward, which eases the learning curve for those new to coding. Conversely, its robust features and extensive libraries allow seasoned developers to implement complex scenarios.
Automation engineers often work closely with development teams, and Java fluency allows for seamless communication. This synergy can lead to higher quality software and fewer bugs in deployments; thus, strong proficiency therein becomes invaluable. Mastery of Java in automation testing is not just a competitive advantage but often a typical requirement in job listings within this field.
Importance of Coding Interviews
Coding interviews serve as a fundamental assessment tool for employers. They evaluate not only a candidate’s ability to solve problems but also their understanding of core concepts. 👨💻# Through coding questions focused on Java, interviewers assess a candidate's logical thinking, coding style, and efficient problem-solving methods. Moreover, problems often mirror real-world situations, providing both parties a glimpse into daily challenges that may occur in automation testing roles.
A well-prepared candidate enhances the interview process for everyone involved. In this sense, deeply knowing topics of Java can ease candidates' nerves, leading to mostrar confidence during discussions. Getting accustomed to typical Java coding interview questions helps individuals refine their thoughts and articulate solutions clearly under the pressure of timed assessments.
Core Java Concepts for Automation Testing
Core Java is essential for understanding automation testing. It comprises fundamental principles of Java programming that relate directly to writing efficient and automated test cases. Knowing these core concepts can enhance a programmer's ability to tackle complex problems in testing environments and ensure high quality in software delivery. This section will delve into the vital topics necessary for candidates preparing for technical interviews in automation testing roles.
Object-Oriented Programming Principles
Object-Oriented Programming (OOP) principles form the backbone of Java. These principles include encapsulation, inheritance, and polymorphism. Understanding OOP allows testers to create modular and extendable code, making it easier to adapt tests to changing requirements.
- Encapsulation protects data integrity by restricting direct access, hence fostering a controlled interaction with data objects. In testing, it aids in maintaining stability within test cases.
- Inheritance promotes code reuse, reducing redundancy and simplifying maintenance. When new features or components are added, testers can leverage existing code, increasing productivity.
- Polymophism allows methods to perform differently based on object type. In testing scenarios, this enables the same test to apply across multiple contexts or object types, enhancing flexibility.
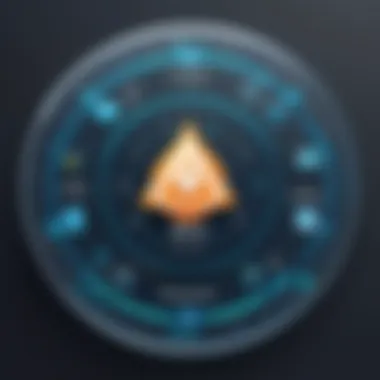
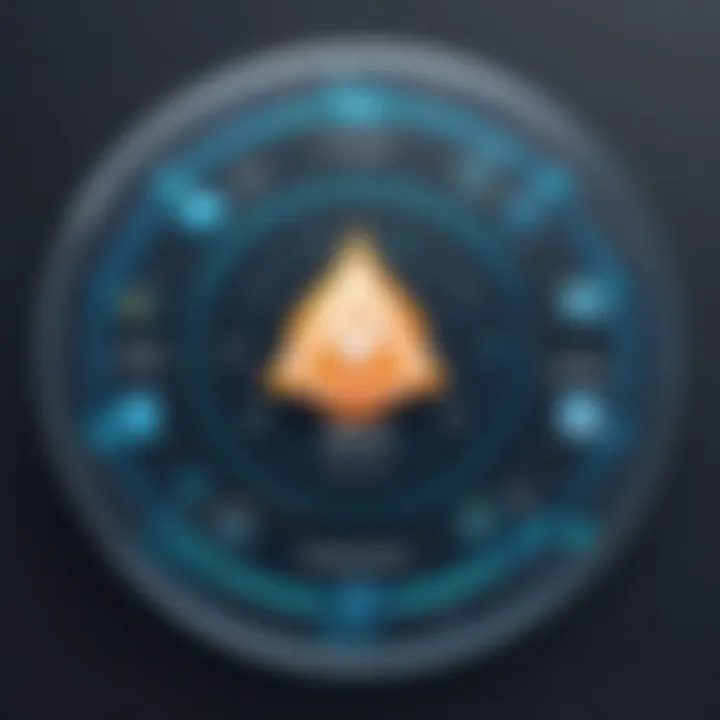
By mastering these principles, automation testing candidates can demonstrate their ability to write scalable and maintainable tests.
Java Collections Framework
The Java Collections framwork comprises a set of classes and interfaces that facilitate the handling of groups of objects. Effective utilization of collections can simplify the code structure and improve functionality. In testing, the collections framework provides various data structures that can enhance the implementation of test scenarios.
Lists
Lists in Java are an ordered collection that allows for duplicate elements. They are particularly important because they are flexible and can store heterogeneous elements. This key characteristic makes it easier to maintain data sequences in automation tests.
A unique feature of lists is their ability to grow dynamically as elements are added. This allows test scripts to handle varying test sizes effectively. An example of list usage is storing dynamic test data, which can improve test reliability. However, the unique feature also comes with a performance consideration; operations like insertions at the start of a list can become costly if not managed correctly.
Sets
Sets are used for storing unique elements, and they are unordered collections. The major advantage of sets in automation is that they prevent duplicates effectively. For example, when managing unique identifiers during testing sessions or keeping track of unique session objects, sets show great advantages.
One unique feature of sets is their capacity to optimize lookups, because they use hashing or balancing trees to manage elements. A disadvantage could be their lack of order. For instance, if the order of processing items matters in a test scenario, sets may pose challenges. Thus, decisions on using sets need careful consideration on the testing context.
Maps
Maps hold key-value pairs, making them incredibly useful for storing relationships between values. The ability to quickly access associated data gives them strategic importance in automation testing. For instance, mapping test cases to specific inputs or keeping key configurations is oftentimes preferred using maps.
The key characteristic of maps is their fast lookup capabilities due to efficient indexing. A unique aspect is how values can be retrieved without concerning the properties of entries. However, developers should be mindful of the varied implementations like HashMap or TreeMap, as the choice affects performance and ordering characteristics, which are often crucial in run-time contexts.
In summary, understanding these core concepts and collections will equip candidates with practical tools needed for passing technical interviews in automation testing roles.
Key Java Libraries for Automation Testing
In the realm of automation testing, Java libraries play a pivotal role in simplifying and expediting the development and execution of tests. Given Java's widespread adoption in the automation framework landscape, familiarity with these libraries is critical for anyone pursuing a career in automation testing. Understanding the essential libraries can make a substantial impact on the efficiency and effectiveness of test automation strategies.
JUnit and TestNG
JUnit and TestNG represent the backbone of unit testing in Java. JUnit focuses on simplicity and is widely used for test-driven development. It allows for the creation of repeatable tests and includes annotations to enhance test execution, making it easier to write and maintain tests over time. Key features include:
- Simple Annotations: Annotations like , , and offer a clear structure to tests.
- Assertions: We can check that certain conditions are true with assertion methods like or , tightening the reliability of tests.
- Tool Integration: JUnit easily integrates with other tools and IDEs, streamlining the workflow.
On the other hand, TestNG is more flexible and powerful compared to JUnit. It brings several additional capabilities as:
- Annotations: Advanced annotations like and allow for parameterized tests, adding versatility.
- Dependency Tests: TestNG enables execution of tests in dependency chains, which can be a significant advantage when order matters.
- Parallel Execution: It can run tests in parallel, aiding in speeding up test processes and efficiency.
Both frameworks are designed to endow Java developers with systematic approaches to maintain the quality of their code through predefined tests. Their compatibility with various build tools like Maven or Gradle further increases their value in continuous integration setups.
Selenium WebDriver
Selenium WebDriver is one of the most critical tools for web automation testing. It provides a programming interface for creating test scripts in Java to control a web browser. Key strengths of Selenium WebDriver include:
- Cross-Browser Testing: It supports various browsers such as Chrome, Firefox, IE, and Safari, ensuring tests can be run across multiple platforms.
- Programming Language Compatibility: Though primarily used with Java, it supports multiple languages, enabling teams to deploy it in environments that best suit their programming practices.
- Rich Set of Features: With Selenium WebDriver, testers can handle advanced scenarios, like setting up parameters, managing cookies, and taking screenshots during test execution.
Selenium WebDriver transforms how web applications undergo testing by facilitating an automated approach to navigate and interact with the UI elements effortlessly.
Used together with JUnit or TestNG, Selenium simplifies testing for dynamic web applications, enabling a robust automation framework that scales efficiently.
Apache POI for Excel Integration
Managing test data is paramount in automation testing. The Apache POI library serves as a bridge between Java applications and Excel files, enabling procedures for reading and writing Excel documents seamlessly. Its relevance lies in:
- Accessing Spreadsheet Data: POI allows for easy retrieval of information from Excel, making it ideal for storing test datasets and controlling the input/output operations.
- Writing Test Results: In automated frameworks, test outcomes can be documented back to the Excel file directly, providing a central storage point for the results.
- Flexible Format Support: Apache POI supports both and formats, making it versatile across various Excel versions affecting many teams.
As data-intensive testing grows, solutions like Apache POI provide a helping hand in dealing with large data sets ensuring tests are reliable and repeatable.
Essential Java Coding Interview Questions
The concept of essential Java coding interview questions is central to preparing candidates for roles in automation testing. Understanding specific coding skills not only demonstrates programming competency but also highlights a candidate's ability to apply these skills in real-world testing scenarios.
Knowledge in this area improves problem-solving abilities, and proficiency in Java can set candidates apart in the competitive job market. Interview questions often cover a range of topics, from basic syntax to complex data structures, giving employers insight into a candidates’ analytical and coding skills. This understanding can directly impact one's ability to automate test cases effectively and enhance the overall testing process.
String Manipulation
String manipulation is a fundamental aspect of Java programming that often comes up during interviews. Many testing environments involve string processing, especially when validating inputs, generating reports, or parsing data. Candidates should be prepared to demonstrate their ability in several areas, such as concatenating strings, parsing them, and performing transformations on the data.
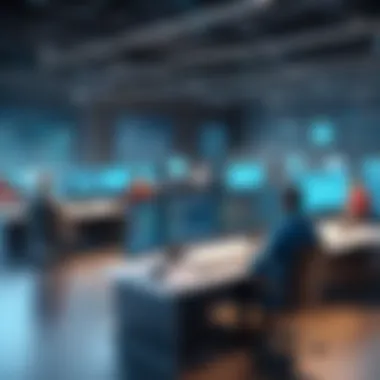
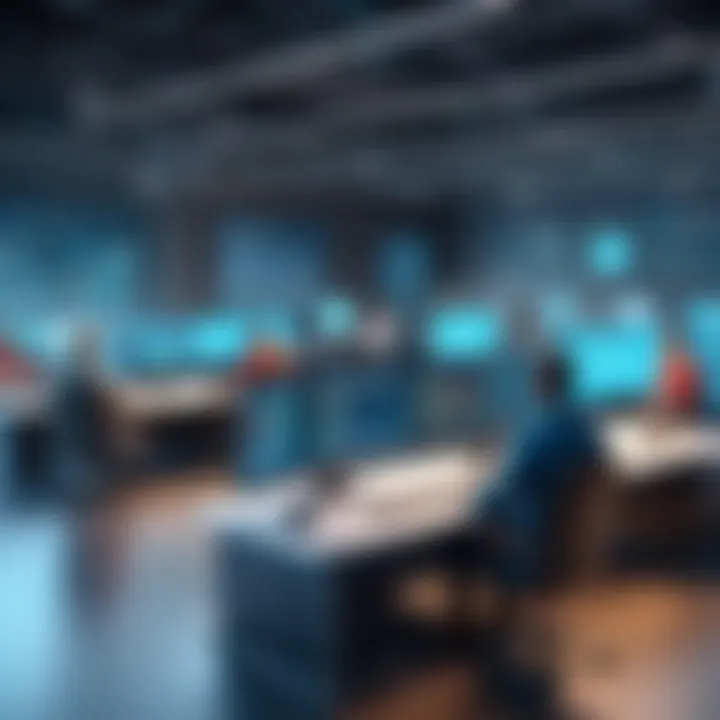
Practical examples often focus on unique challenges. Developers might be asked how to determine if a string is a palindrome or to reverse a string character by character. These tasks not only test basic Java knowledge but also assess a coder’s prowess in applying logic to solve problems efficiently.
Data Structures Implementation
The implementation of data structures in Java is another prominent subject in automation testing interviews. Java offers a variety of built-in data structures, including arrays, lists, sets, and maps. Candidates should understand when to use each type based on specific testing scenarios. A solid foundation in these structures can increase effectiveness in organizing, storing, and retrieving information, which is critical in navigating complex testing environments.
*Key characteristics of common data structures:
- Arrays: Good for fixed-size lists and fast access times.
- ArrayLists: Allow for dynamic resizing and ease of use.
- HashMap: Useful for key-value pairs, offers quick insertions and lookups.
An interview might involve implementing a certain data structure and demonstrating how to use it in the context of a test automation task. Such practical knowledge is crucial for an automated framework's efficiency.
Algorithm Questions
Code interviews often include questions focused on algorithms. These questions help evaluate candidates’ problem-solving skills and cognitive testing abilities. Automation testers should expect questions focused on sorting and searching techniques.
Sorting Algorithms
Sorting algorithms are a fundamental part of algorithmic interviews. Knowing when and how to apply different sorting techniques can be critical for real-time data management in testing. For example, a candidate may be asked to sort a list before further analysis or execution.
Some common sort techniques include:
- Quick Sort: Very efficient with an average time complexity of O(n log n).
- Merge Sort: Ideal for linked lists and has a consistent O(n log n) complexity due to its divide-and-conquer nature.
It’s beneficial to also discuss their space complexity and stability. Candidates who can express the pros and cons of these algorithms will show a deeper understanding of computational efficiency relevant in automation testing contexts.
Searching Algorithms
Searching algorithms also hold significant importance in coding interviews and automation. They relate to how quickly a piece of data can be located within a dataset. Candidates might pose challenges to implement and analyze their efficiency here as well.
- Binary Search: Offers excellent performance with an O(log n) time complexity for sorted data.
- Linear Search: Simpler but slower, runs in O(n) complexity since it checks each element one by one.
Employers will gauge if a candidate can implement solutions but also analyze the best techniques applicable in various scenarios. Understanding searching algorithms can enhance a candidate's ability to efficiently query information during automation tasks or retrieve data across a network.
Understanding these concepts aids candidates in marking their proficiency in Java, reinforcing their testing methodologies and enhancing their overall capabilities in automation. Keep practicing these challenges and concepts to stand out during technical interviews.
Testing Frameworks in Java
Testing frameworks serve as an essential backbone in Java automation testing. They provide a structured methodology to execute tests, thereby significantly enhancing the productivity and reliability of the testing process. Utilizing a testing framework allows developers to write test scripts in a standardized form, which simplifies both maintenance and execution of tests. Furthermore, employing such frameworks can lead to improved test coverage by making it easier to set up and run extensive test suites.
In Java, there are numerous prevalent testing frameworks, each catering to specific needs and offering unique features. Selecting the right framework can streamline the automation process and enable more efficient test management. Test frameworks decrease redundancies and minimize human errors in test execution. By offering tools for reporting results and managing test configurations, they make it easier for testing teams to track progress and to identify areas needing attention.
Another important element is how they facilitate integration with other tools in the CI/CD pipeline, ensuring that code changes are automatically validated. Proper utilization can ultimately lead to higher software quality, faster delivery cycles, and greater overall developer satisfaction.
As candidates explore job opportunities in automation testing, understanding testing frameworks becomes essential not just for interviews, but also for undertaking real-world projects.
Unit Testing with JUnit
JUnit is a widely adopted framework in the Java ecosystem. It is crucial for implementing unit tests, which are designed to verify the correctness of small, individual units of code, typically methods or classes. With its emphasis on simple annotations, JUnit allows for straightforward test creation and execution.
JUnit provides several benefits:
- Concise Documentation: Offers easy annotations @Test, @Before, @After that help in maintaining clarity.
- Test Results: Automatically produces meaningful results, allowing developers to quickly assess code quality.
- Integration Capabilities: It integrates seamlessly with build automation tools like Maven and Gradle, as well as CI servers like Jenkins.
Understanding JUnit is fundamental for software developers, especially those in automation testing. It serves as a foundational skill developers ought to demonstrate during technical interviews.
Behavior Driven Development (BDD)
Behavior Driven Development (BDD) improves collaboration between developers, testers, and domain experts. Cucumber emerged as a pivotal tool utilized within BDD practices. It works by allowing everyone involved to write specifications in plain language. This accessibility promotes better understanding of requirements and improves overall team alignment.
Cucumber Overview
Cucumber's standout feature is its ability to execute scenarios written in natural language, making it achievable for non-technical stakeholders to provide input on test cases. Cucumber uses Gherkin, a simple syntax for behavior expressions and is versatile enough to implement behavior across various programming languages,. Advantages of Cucumber include:
- Encouraged collaboration among team members across disciplines.
- Natural language development of test cases aids understanding and promotes clarity.
However, drawbacks may surface in its learning curve since some developers might find Gherkin syntax verbose compared to traditional test scripts.
Integrating Cucumber with Selenium
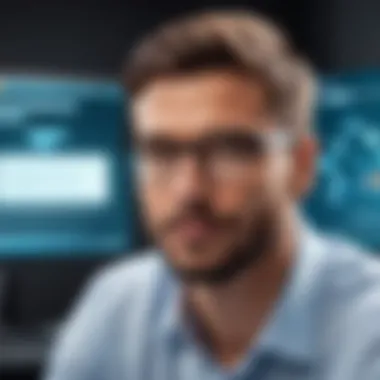
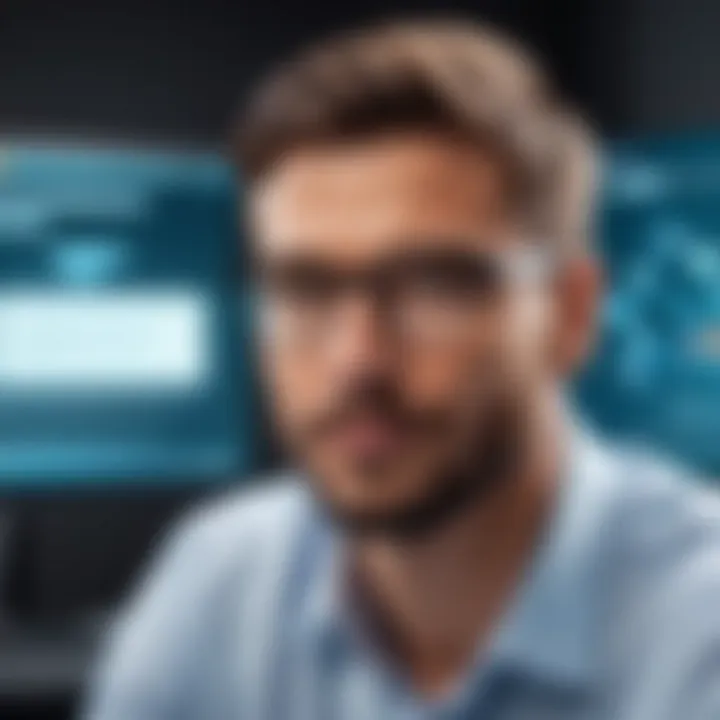
The integration of Cucumber with Selenium fortifies automation tests for web applications. Selenium excels in automating browsers, while Cucumber focuses on defining user behavior, which creates a comprehensive testing structure. Integrating these two provides a powerful platform for automated functional testing. Advantages of this integration:
- Better test coverage: By utilizing Cucumber for behavior descriptions and Selenium for executing test cases, testing can be done comprehensively.
- Promotes clear communication: Each test step in the scenario maps directly to code, making it easier for all stakeholders to participate during testing phases.
It is important to consider, however, that establishing the initial framework can require significant setup and understanding of both tools, which could be an overhead for some teams.
Common Challenges in Automation Testing
Automation testing brings many advantages, but it also has its own unique set of challenges that professionals need to understand and navigate. Recognizing these challenges allows candidates to prepare for potential topics in coding interviews. This section discusses specific challenges faced during automation testing, emphasizing the relevance of these insights in building robust automated tests.
Handling Dynamic Web Elements
Dynamic web elements can pose significant challenges in automation testing. These elements, like responsive buttons or sections that load with JavaScript, are not static. Hence, their properties, such as ID or class names, may change on different loads or user interactions. To effectively test such elements, it is essential to apply various strategies instead of relying solely on fixed attributes.
- XPath and CSS Selectors: Utilizing XPath and CSS selectors is often preferred. They allow for flexibility in locating elements, as they are less prone to breaking from simple updates in element attributes.
- Wait Mechanisms: Implement implicit and explicit waits in your test scripts. These mechanisms help manage the timing when web elements become available for interaction, reducing potential errors.
- Consistency Checks: Implement checks to ensure that a dynamic element still resides in the DOM before interacting with it. Failing to do so may lead to functions executing on elements that are not currently displayed.
Synchronization Issues
Time management plays a crucial role in the success of automation testing. Synchronization issues arise when the execution speed of test scripts does not align with the application’s response time. These inconsistencies lead to test failures or, at worst, erratic behavior of tests.
- Thread Sleep: Although you might be tempted to use fixed pauses with , this should be limited. It halts the execution for a hardcoded time, which is not efficient.
- Explicit Waits: Instead of that, employ explicit waits that allow the code to pause only until the desired condition occurs. Conditions may include an element becoming clickable or a specific element being present.
- Understanding Load Patterns: During the approach to testing, ensure you have a clear understanding of how load affects response. Recording response times during load will be beneficial in refining your scripts.
Ultimately, enabling understanding of dynamic web elements and synchronization issues prepares candidates for real-world challenges. Solving these does not just improve individual test scripts but lays a solid foundation for evolving to a mature automated testing suite.
Mock Interviews and Practical Assessments
Mock interviews and practical assessments are critical components in preparing for Java coding interviews focused on automation testing. These preparation methods provide candidates an opportunity to face real-world scenarios that simulate the dynamics of an actual interview. Through these exercises, individuals can build confidence, improve technical skills, and gain valuable experience that may positively influence their performance.
Importance of Mock Interviews: Mock interviews allow candidates to simulate a formal interview setting. They afford the chance to practice articulating thoughts and answering questions under pressure. Feedback received can illuminate narrow areas of knowledge or skill, prompting improvement before the formal interview. Such exercises remove some of the unpredictability associated with coding assessments and enhance readiness for common challenges.
Practical Assessments: Practical assessments go beyond theoretical learning and dive into application. In these evaluations, participants often need to write code, debug errors, or solve problems. These tasks mirror the day-to-day responsibilities expected of an automation tester using Java. With hands-on experience, candidates become adept at the nuances of various tools and frameworks used in the field, such as Selenium or JUnit.
Simulating Real Interview Scenarios
Simulating real interview scenarios is essential in preparation for automation testing roles. The complex nature of interviews can often lead to nervousness that impacts performance. Practicing in a low-pressure environment reduces anxiety and helps candidates determine their strengths as well as areas for growth. Role-playing with peers, mentors, or online platforms that offer interactive mock sessions helps create realistic situations.
During these simulations, focus on familiarizing oneself with common types of questions, coding styles, and approaches to problem-solving. Understanding how to think aloud during a coding challenge is also beneficial as it shows the interviewer a candidate's thought process and reasoning.
Resources for Practice
Online Coding Platforms
Online coding platforms are treasure troves for practicing coding interview questions. These websites often feature a robust library of practice problems specifically tailored for automation testing roles using Java. One key characteristic of online coding platforms is their immediate feedback mechanism. Candidates can test their code, find errors instantly, and gain insights on how to optimize their solutions.
Platforms like HackerRank or LeetCode are renowned due to their vast user community and competitive environments. This encourages participants to engage in more than just solitary practice; they can participate in contests that foster quick thinking and agility.
However, while online platforms offer multiple advantages, they sometimes do not provide the full experience of a traditional coding interview. This might lead to false confidence in reading questions straightforwardly without considering all aspects of the coding requirements.
Books and Tutorials
Books and tutorials remain invaluable in further strengthening preparation for Java automation testing interviews. Unlike online platforms, which often induce time pressure, books provide a slower-paced, deep dive into Java concepts. A common feature is structured content that leads from foundational practices to more advanced topics, which can be particularly beneficial for individuals starting from a basic level of knowledge.
Recent popular titles often focus on both programming skills and testing frameworks. They tend to include practical coding challenges with solutions explained thoroughly. The downside is that consuming literature requires more time and discipline than interactive platforms. However, the grounding in theory presented within these books often translates to a deeper understanding when candidates encounter relevant practical problems during interviews.
To summarize, mock interviews and practical assessments serve as pivotal elements of thorough preparation for Java coding interviews in automation testing. With both the flexibility of online coding platforms and the depth of books along with structured practice, candidates can significantly improve their chances at succeeding in their interviews.
End
Recapping critical concepts is vital. It reinforces what you have learned, whether you focus on string manipulation, data structures, or relevant libraries like JUnit and Selenium. During interviews, articulating your knowledge succinctly prepares you to address unforeseen questions effectively.
Consider the increasing importance of automation testing in the software development life cycle. As companies advance, they seek candidates who not only know Java and its applications but can also engage with evolving frameworks and trends in technology. Thus, keeping abreast of these advancements is essential for one's career growth.
Recap of Key Points
- Importance of Core Java: Mastery of Java fundamentals, like OOP principles and collections framework, is crucial.
- Key Libraries: Familiarity with libraries such as Selenium for web automation and TestNG for structured testing can set a candidate apart.
- Problem-Solving Skills: Java coding questions often emphasize data structures and algorithms, outlining the need for strong logic and analytical ability.
- Mock Interview Preparation: Engaging in simulated interview scenarios can uncover gaps in knowledge while increasing confidence.
Future Trends in Java Automation Testing
Automation testing is steadily evolving, with advancements anticipated in various domains:
- Artificial Intelligence Integration: Utilizing AI to enhance test automation robustness and efficiency is a growing trend that promises accuracy and flexibility.
- Low-Code Development: The rise of low-code platforms is simplifying the process of automating tests. Familiarity with these tools can be beneficial for testing professionals.
- Performance Testing Expansion: There is increased recognition of the need for integrating performance testing within the automation strategy.
Staying informed about these trends allows Java developers and automation testers to be proactive in their career paths. Continuous learning will not just make candidates favorable but also enable them to innovate in the field of automation testing.
"Understanding the evolving landscape of automation testing ensures that one remains relevant and valuable in the tech industry."