A Comprehensive Guide to Java Programming for Beginners
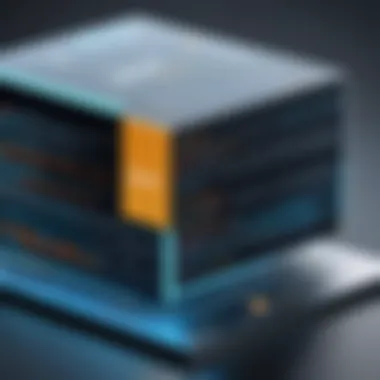
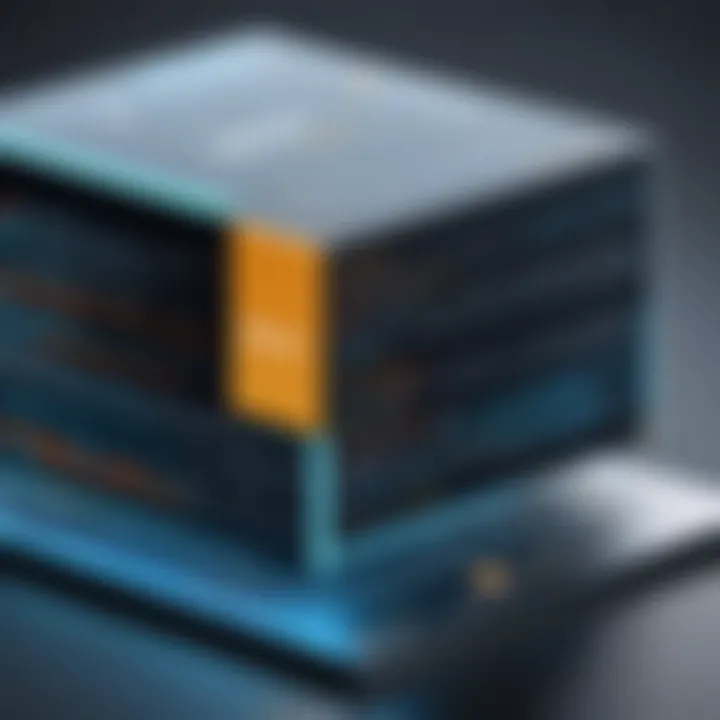
Intro
Java has long been a cornerstone in the programming world, cherished for its versatility and robust capabilities. This guide aims to cultivate foundational knowledge for beginners, equipping them with essential skills through various resources. Understanding Java is not merely an academic exercise, it is a pathway into the expansive realm of software development. The significance of Java extends beyond its syntax; it embodies principles that govern effective programming practices.
As we navigate through this guide, the focus will be on resources in PDF format, which are easily accessible and conducive for self-paced learning. A blend of theoretical knowledge and practical application will be discussed, ensuring that learners can bridge the gap between concepts and their implementation.
In the rapidly evolving technology landscape, Java remains relevant, powering applications across diverse sectors. It is important for beginners to recognize the depth of the language and the continued learning opportunities it offers. By examining various aspects of Java programming, including the structure, syntax, and best practices, this article endeavors to provide a comprehensive foundation for those embarking on their coding journey.
Intro to Java Programming
Java stands as a significant pillar in the landscape of programming languages. This article aims to demystify its core elements, emphasizing why Java is a critical skill for both budding and seasoned programmers. With a rich history dating back to its inception in the mid-1990s, Java has evolved into a versatile language widely used in diverse applications including web development, mobile applications, and large-scale enterprise systems.
Java’s unique platform independence, achieved via the Java Virtual Machine, allows developers to write code that can run on any device with the JVM installed. This feature not only increases Java's accessibility but also makes it integral for cross-platform applications. In a world increasingly driven by mobile and interconnected applications, understanding Java programming is not merely beneficial; it is essential.
Understanding Java
Java is a high-level, object-oriented programming language that focuses on simplicity and readability. Unlike low-level languages, Java abstracts many complexities, allowing programmers to write clear and maintainable code. Its syntax is influenced by C and C++, making it somewhat familiar to developers with knowledge of these languages.
The language employs a strongly typed system, where every variable must declare its type before use, enhancing code stability and minimizing errors. Java also uses automatic garbage collection, which helps in managing memory more effectively by removing unused objects from memory.
As a beginner, grasping these key features provides a solid foundation to explore advanced concepts. Java's core libraries support a wide range of functionalities, from networking to graphical user interface development, enabling developers to create comprehensive applications.
Importance of Java in Software Development
Java has established itself as a cornerstone of modern software development for several reasons. First, it is object-oriented, which means it promotes modular, reusable code. This approach not only improves productivity but also fosters a collaborative programming environment, essential for team-based projects. Additionally, the use of classes and objects simplifies complex systems into manageable components.
Moreover, the extensive ecosystem surrounding Java is crucial. The availability of robust frameworks like Spring and Hibernate allows developers to accelerate their projects while maintaining high-quality standards. Furthermore, with the proliferation of cloud computing, Java’s compatibility with cloud services strengthens its role in developing scalable applications.
In a rapidly evolving technological landscape, Java’s continued relevance is underpinned by its community. The support from forums such as Reddit, together with numerous resources on sites like Wikipedia), ensures that programmers, whether novice or expert, have access to a wealth of knowledge and community support.
"Java enables developers to create dynamic web applications that enhance user experience and engagement."
Learning Java is not just about understanding the language itself. It is about entering a broader ecosystem that enhances your programming skills, facilitates collaboration, and prepares you for a future in technology that increasingly relies on software solutions.
Getting Started with Java
Getting started with Java is an essential step for anyone aspiring to enter the world of programming. This section provides the foundation necessary for beginners to comprehend the core concepts and tools involved in Java development. Understanding how to set up an effective coding environment is crucial, as it ensures that learners can focus on writing code without being hindered by technical setbacks. Furthermore, starting off on the right foot can significantly enhance the learning experience, providing a smoother transition into more advanced programming tasks.
Installing the Java Development Kit (JDK)
The Java Development Kit, commonly known as JDK, is the first component you need to install to begin Java programming. The JDK includes several tools that are essential for developing Java applications. Among these tools are the Java compiler, which compiles the source code into bytecode, and the Java Runtime Environment (JRE), which provides the necessary libraries for running Java applications.
To install the JDK:
- Download the JDK: Visit the official Oracle website or adopt OpenJDK for a more open source version. Select the version suitable for your operating system.
- Follow Installation Instructions: Once downloaded, run the installer and follow the prompts to complete the installation. Ensure to set environment variables to make Java accessible from the command line.
- Verify Installation: After installation, open your command line interface and type . This command displays the installed version of Java, confirming that the installation was successful.
Installing the JDK is a straightforward process, but it is crucial to ensure the installation is completed correctly to avoid future issues.
Setting Up an Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) significantly enhances coding efficiency for beginners. An IDE provides a user-friendly interface, code editor, compiler, and sometimes debugging tools all in one package. Therefore, it is vital to choose and set one up to streamline the coding process.
Popular IDEs for Java include IntelliJ IDEA, Eclipse, and NetBeans. Each has unique features and benefits, catering to different coding styles and preferences.
To set up an IDE:
- Download Your Preferred IDE: Go to the official website of the IDE you choose and download the installation file.
- Install the IDE: Follow the installation prompts. Most IDEs offer a straightforward installation process.
- Configure the IDE: Once installed, you may need to configure the environment settings within the IDE. This may include pointing the IDE to the JDK installation to ensure it can compile the code correctly.
- Create a New Project: After configuration, create a new project to start coding. This will usually be facilitated through a menu option in the IDE.
Engaging with an IDE allows for more productive coding practices and offers tools that assist with code organization and debugging. This is the final step in getting started with Java, leading to a solid foundation for further exploration into programming.
Fundamental Java Concepts
In Java programming, fundamental concepts serve as the building blocks for understanding more complex topics. This section explores the essential elements of Java, offering beginners the necessary framework to begin coding. The benefits of mastering these concepts extend beyond mere functionality; they lay the groundwork for effective problem-solving, code structure, and overall programming proficiency. Knowing these fundamentals makes it easier to grasp advanced programming ideas and methodologies. The clarity provided by understanding these principles enhances both learning and practical application.
Data Types and Variables
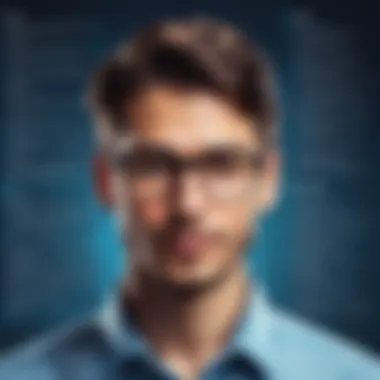
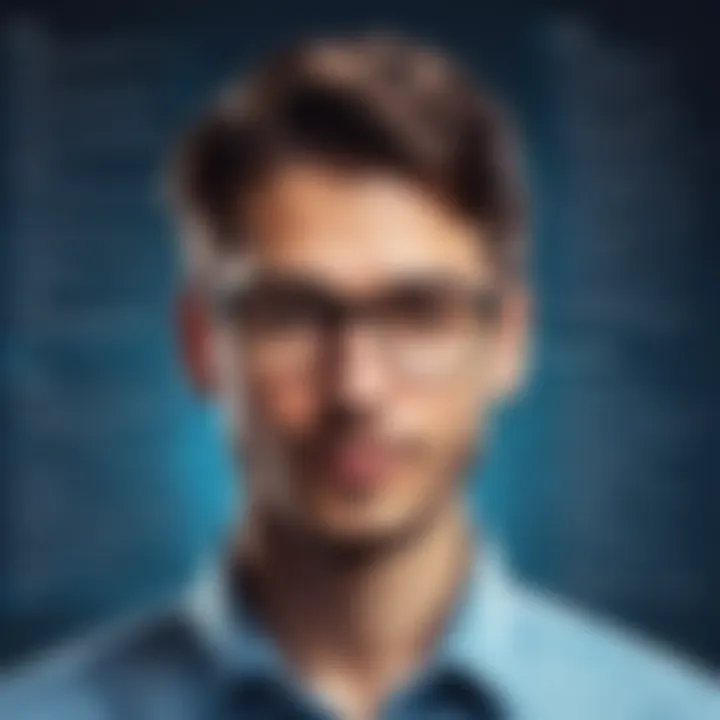
Data types in Java are crucial. They define the kind of values a variable can hold. Java is a statically typed language, meaning that variable types are declared at compile time, promoting efficient memory management and type safety. The main data types include:
- Primitive types: Such as , , , , and , which hold basic values.
- Non-primitive types: Includes , , and user-defined classes.
Here's an example of declaring variables:
Variables can store values which makes them versatile in program areas, such as mathematics or user input. Correctly applying data types and variables enables programmers to build applications that are efficient and functional.
Control Structures: Conditionals and Loops
Control structures are essential for controlling the flow of a program. In Java, conditionals help decide which blocks of code to execute based on certain conditions. The primary conditional statements are , , and . For example:
Loops, on the other hand, allow repetitive execution of code blocks. Understanding loops helps optimize code and minimize redundancy. The two main types of loops are and . For instance:
Leveraging these control structures in programming leads to more dynamic and interactive applications.
Methods and Functions
Methods in Java are blocks of code that perform specific tasks. Defining methods enables code reusability and organization. Every method is defined with a name, return type, and parameters. For example:
This method takes two integers and returns their sum. Functions also enhance code clarity and management. With encapsulation and modular programming, it’s easier for developers to troubleshoot and maintain code.
Object-Oriented Programming in Java
Object-oriented programming (OOP) is foundational to Java and is one of its most significant features. Understanding OOP concepts is critical for both beginners and more experienced programmers who wish to write efficient and maintainable code. The principles of OOP—encapsulation, inheritance, and polymorphism—help in structuring software in a modular way, enhancing reusability and scalability. As software systems grow in complexity, the importance of these concepts increases, making OOP a valuable skill in a programmer's toolkit.
Classes and Objects
In Java, everything revolves around the concept of classes and objects. A class acts as a blueprint for creating objects. It contains properties (attributes) and methods (functions) that define the behavior of an object. When you instantiate a class, you create an object that has its own data and can perform operations defined by the class.
For example, consider a class called . This class may have properties such as , , and . It may also have methods like and . When an object of the class is created, it can perform these methods independently.
Here's a simple code snippet to illustrate this:
Using classes and objects enables programmers to represent real-world phenomena in a systematic way, making code easier to understand and manage.
Inheritance and Polymorphism
Inheritance allows one class to inherit the properties and methods of another. This promotes code reuse and establishes a hierarchical relationship between classes. The class that inherits is called the derived or child class, while the class it inherits from is known as the base or parent class. By leveraging inheritance, developers can create more specific classes without rewriting common code.
For instance, if you have a class , you can create subclasses like and . These subclasses would inherit features such as and , while also having unique characteristics of their own.
Polymorphism refers to the ability of a single interface to represent different underlying forms (data types). This is achieved through method overriding in inheritance. A child class can define a method that is already provided by a parent class, allowing for different implementations based on the object making the call.
Encapsulation and Abstraction
Encapsulation is the mechanism of restricting access to certain components of an object, ensuring that the internal state of an object can only be modified through designated methods. This protects the integrity of the object's data and avoids accidental interference from outside code. For encapsulation, private variables in a class can be updated only via public setter methods.
Abstraction is closely related to encapsulation and focuses on exposing only the necessary parts of an object while hiding the complexity. It allows programmers to deal with the functional capabilities of a system without delving into implementation details.
Through encapsulation and abstraction, Java promotes cleaner, more maintainable code. Developers can focus on interactions with objects rather than implementation specifics, streamlining the development process.
"Object-oriented programming allows for a clear modular structure that aids in management of complex systems."
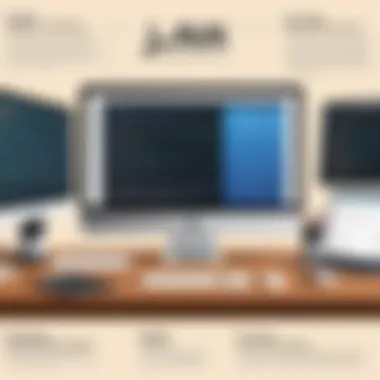
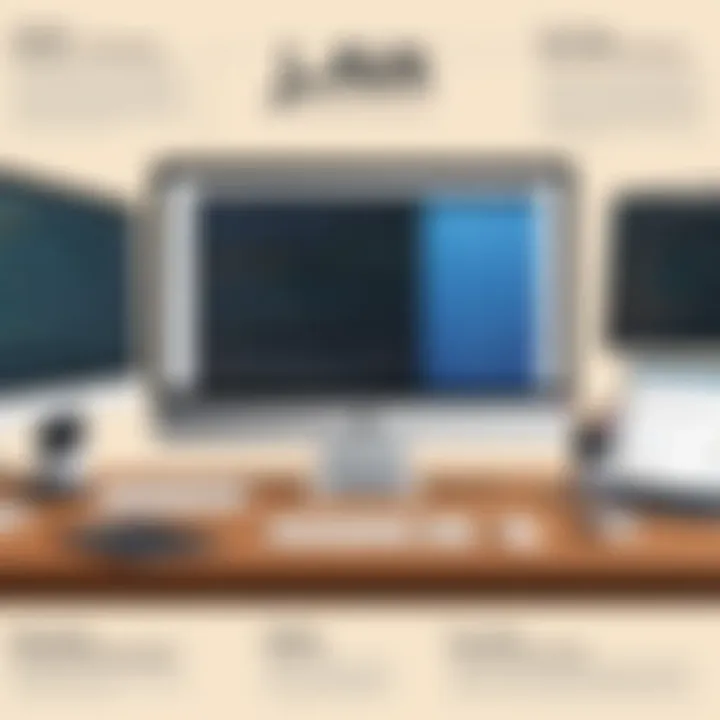
Java Programming Best Practices
In the realm of software development, especially with Java, adhering to best practices is crucial. These practices enhance the maintainability of code, improve its readability, and help prevent errors early on in the development lifecycle. A well-structured Java program is not only easier to understand but also simplifies the debugging and enhancement processes later.
Code Readability and Documentation
Code readability stands as a fundamental pillar in programming. It refers to how easily a human reader can understand the code. Several techniques can be employed to enhance readability:
- Meaningful Naming Conventions: Variables, methods, and class names should convey their purpose. For instance, using is preferable to .
- Consistent Formatting: Using a consistent style throughout the code helps in understanding its structure. Indentation and spacing should be uniform to delineate code blocks clearly.
- Comments and Documentation: Writing comments is key for clarifying complex logic, explaining variable purposes, and guiding future developers. Tools like Javadoc can be useful for generating documentation from comments, aiding in the overall understanding of the code.
Adhering to these principles aids not only the original developer in recalling their logic but also others who may join the project later. This leads to reduced onboarding time and fosters collaboration.
"Good code is its own best documentation."
Error Handling and Debugging
Error handling and debugging are vital components of the software development process. They ensure that the program behaves predictably, even when faced with unexpected input or system issues. Java provides a robust mechanism for managing errors through exceptions.
- Using Exceptions: Proper exception handling can prevent a program from crashing. For example, wrapping code that might throw exceptions in try-catch blocks allows for graceful error handling. This is essential for maintaining a good user experience.
- Logging: Implementing logging allows developers to trace issues in the application. It is advisable to use logging frameworks like Log4j or SLF4J to capture detailed logs of the application’s behavior.
- Debugging Techniques: Utilizing debugging tools integrated within IDEs like IntelliJ IDEA or Eclipse accelerates the debugging process. Setting breakpoints and watching variable values help pinpoint problematic areas in the code.
By focusing on error handling and debugging practices, developers can create more resilient applications that are adaptable and easier to maintain.
Resources for Learning Java
Java programming has gained significant popularity over the years due to its versatility and ease of use. Learning resources specific to Java can substantially enhance a beginner’s programming skills. These resources equip learners with knowledge of fundamental concepts, best practices, and effective problem-solving strategies. They help bridge the gap between theory and practical programming capabilities. Through a combination of reading materials, online courses, and community engagement, learners can ensure a comprehensive understanding of the language and its applications.
When considering Resources for Learning Java, it is important to evaluate content quality, accessibility, and suitability for different learning paces. This helps maximize the learning experience. Effective resources not only provide information but also support engagement with the Java community. This engagement fosters networking and motivates learners to apply what they have gained into real-world scenarios.
PDF Resources for Beginners
In the realm of programming, PDF resources play a distinctive role for beginners. These documents often serve as a structured approach to foundational learning. Books and e-books in PDF format provide clear explanations and examples that cater to diverse learning styles. They represent a range of topics from basic syntax to advanced Java applications.
PDF resources offer several benefits:
- Offline Access: Many beginners prefer reading materials that can be accessed without the internet. PDFs allow learners to study anywhere and at any time.
- Detailed Explanations: These resources often contain thorough explanations accompanied by examples, making complex concepts easier to grasp.
- Free or Low-Cost: Numerous beginner-friendly PDFs are available for free, making them accessible to a wider audience.
Some notable PDFs include:
- "Head First Java"
- "Java: A Beginner's Guide"
- "Thinking in Java"
Additionally, educational institutions frequently publish PDF resources for their courses. Leveraging reputable PDFs can significantly benefit one's learning journey. These documents often include exercises to help reinforce learning.
Online Courses and Tutorials
Online courses and tutorials present another essential avenue for learning Java. They offer a dynamic and interactive approach to programming education. Many individuals favor video tutorials for their visual and auditory elements. Online platforms provide a vast array of courses tailored to different skill levels. Learning from professionals in the field can provide insights and tips that are not readily available in texts.
Key considerations when choosing online courses include:
- Course Structure: Look for courses that start with basics and gradually progress into more complex topics. This scaffolding allows for a steady learning curve.
- Instructor Experience: Verify the qualifications and experience of instructors. Courses taught by industry professionals can offer practical insight.
- Reviews and Recommendations: Checking reviews or seeking recommendations can lead to the discovery of high-quality resources.
Some well-regarded platforms include Coursera, Udemy, and edX. These platforms often feature both free and paid courses. They also provide opportunities for project-based learning, which enhances a learner's ability to apply what they learn.
Engaging in hands-on projects is an effective way to solidify understanding. Tutorials that guide students through constructing simple applications can be particularly beneficial.
Investing time in quality resources and engaging content will significantly strengthen one's Java programming foundation. Learners should continuously explore various avenues for education to find what works best for them.
Practical Applications of Java
Java is a versatile and powerful programming language widely used across various sectors. Understanding its practical applications is crucial for beginners aiming to leverage their skills effectively. Java's robustness, portability, and security make it a preferred choice for many projects. This section will explore several key areas where Java excels, the benefits of using it, and considerations for each application.
Building Desktop Applications
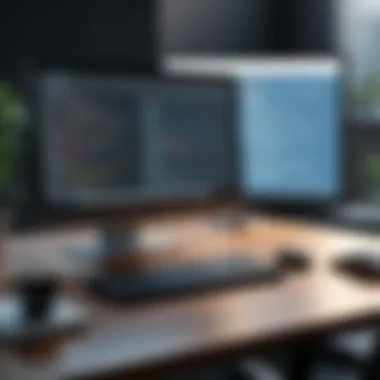
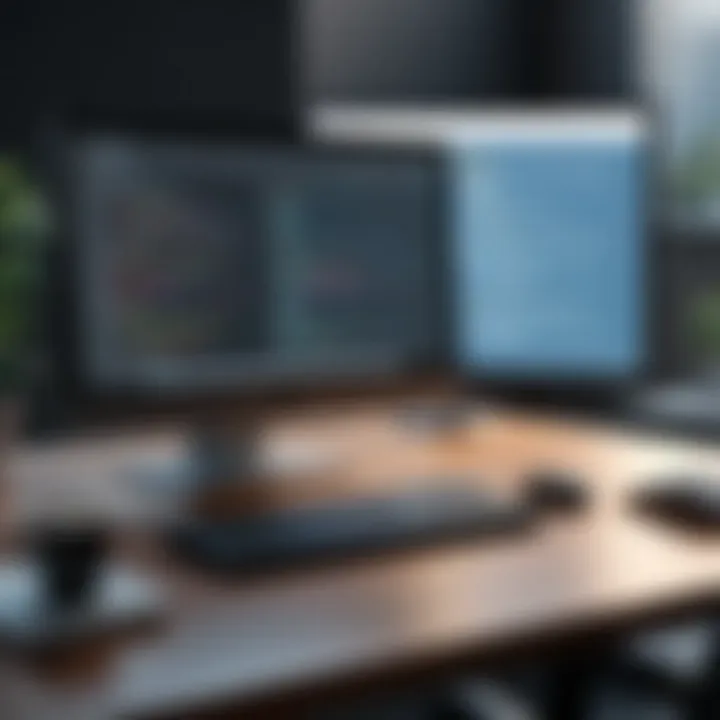
Java is adept at developing desktop applications. The Java Swing and JavaFX libraries provide the necessary framework to create rich graphical user interfaces. SWING builds aesthetically pleasing interfaces while maintaining a consistent behavior across platforms. This cross-platform capability is significant; developers can run the same Java program on Windows, Mac, or Linux, with little to no changes required in the codebase.
Key benefits of building desktop applications in Java include:
- Cross-Platform Compatibility: Write once, run anywhere due to Java's virtual machine.
- Rich User Interfaces: Utilize extensive libraries to enhance user experience.
- Strong Community Support: A vast community offers help and resources for problem-solving.
Developers should consider performance and resource management, especially for resource-intensive applications. Planning and optimization can lead to successful desktop software solutions.
Mobile App Development with Java
Java also plays a critical role in mobile app development, particularly for Android applications. Android Studio, the official Integrated Development Environment (IDE) for Android, uses Java as its primary language. The Android framework provides extensive libraries and APIs to access device features like GPS, cameras, and sensors.
Benefits of using Java for mobile development are:
- Vast Audience Reach: Android holds a large share of the mobile operating system market.
- Robust Development Tools: Android Studio and associated libraries enhance productivity.
- Performance: Java allows efficient management of resources, crucial for mobile applications.
However, developers must be aware of device fragmentation in the Android ecosystem. Ensuring compatibility with various screen sizes and Android versions is essential for maximizing app reach.
Web Development Using Java
Java's relevance extends into web development through technologies like Servlets, JSP (JavaServer Pages), and Spring Framework. These tools empower developers to create dynamic, secure, and scalable web applications. The overall structure allows for the creation of enterprise-level applications that can handle high traffic and complex transactions.
Advantages of Java in web development include:
- Scalability: Java applications can grow alongside user increases.
- Security: Built-in security features reduce vulnerabilities, making it ideal for sensitive applications.
- Mature Ecosystem: Various frameworks and libraries are available for rapid development.
When choosing Java for web development, one must consider the learning curve and the complexity of some frameworks. However, with the right approach, Java can be highly effective for modern web applications.
"Java is not just a language, it's a complete ecosystem that continues to evolve to meet modern demands, making it indispensable in various applications."
In summary, the practical applications of Java are diverse and impactful. From desktop and mobile applications to web development, Java's strengths provide ample opportunities for programmers. By understanding these applications, beginners can make informed decisions as they embark on their programming journey.
Engaging with the Java Community
Engaging with the Java community is vital for both novices and seasoned programmers. The community serves as a crucial support system, fostering an environment where knowledge, experience, and resources are shared. This engagement allows individuals to learn from others, get feedback on their work, and stay updated on the latest industry trends and technologies. Networking within the community can lead to job opportunities, collaborations, and even mentorships, which are invaluable for career growth in the tech sector.
Moreover, participating in community activities can enhance coding skills and expand one's understanding of complex topics. As programming languages and technologies evolve, being actively involved assists in keeping current. Engaging with peers can also boost motivation, providing encouragement during challenging learning phases. Below, we dive into two prominent avenues for engaging with the Java community: online forums and coding contests.
Online Forums and Discussion Groups
Online forums serve as essential hubs for knowledge sharing among programmers. Websites like Reddit and Stack Overflow host vast communities where Java enthusiasts can ask questions and receive answers from experienced developers.
Advantages of participating in these forums include:
- Access to a wealth of knowledge: Asking specific questions often yields detailed explanations and various solutions.
- Diverse perspectives: Engaging with individuals from different backgrounds promotes new ideas and approaches to programming challenges.
- Building a network: Regular interaction helps in forming connections with other programmers, which can be beneficial for future collaborations or job searches.
It is crucial to approach these platforms with respect. Being clear and polite while asking questions encourages helpful responses. Answering others' questions fosters community spirit and establishes you as a resourceful member of the Java community.
Participating in Coding Contests
Coding contests offer a practical and competitive way to enhance your programming skills. Platforms like HackerRank and Codeforces host regular contests tailored for different skill levels. Participating in these competitions not only tests your knowledge but also sharpens your problem-solving abilities under pressure.
Benefits of joining coding contests include:
- Real-world problem-solving: Challenges often mimic real programming scenarios, providing practical experience.
- Improved coding efficiency: With time constraints, you learn to write code more quickly and efficiently.
- Recognition and rewards: High performers can gain visibility in the programming community and even win prizes, which adds an element of motivation.
Culmination
The conclusion serves as a vital component of this guide to Java programming for beginners. It provides an opportunity to synthesize the information presented, highlighting the key points and reflections from the learning journey. For many aspiring programmers, this section becomes a moment of realization about the skills they have acquired and the progress they have made.
Reflecting on the learning journey emphasizes not only the understanding of Java as a language but also the development of problem-solving skills that are essential in any programming endeavor. Java, with its vast ecosystem and application in various domains, offers numerous opportunities for practical application. The ability to grasp fundamental concepts is essential in building a solid foundation.
Reflecting on the Java Learning Journey
As one concludes their journey of learning Java, it is crucial to think about the progress made. In the beginning, concepts like data types, loops, and methods may have seemed daunting. However, through practice and application, these elements become second nature. This transformation underscores the importance of persistence and the iterative nature of programming.
The connection to the Java community can also play a pivotal role in this journey. Engaging with others, whether through forums or study groups, fosters a deeper understanding and exposes learners to diverse perspectives. Being part of such a community can enhance one's skills dramatically and motivate individuals to pursue their learning actively.
Future Directions in Java Programming
Looking ahead, the future of Java programming is filled with both challenges and possibilities. As technology evolves, so do the frameworks and tools associated with Java. Keeping abreast of advancements such as Jakarta EE, modular programming with Java 9, and the increasing incorporation of cloud services much more in Java applications is essential.
Furthermore, the integration of Java with emerging technologies such as artificial intelligence and machine learning opens new avenues. These technologies rely on Java's robust performance and scalability, making knowledge of Java crucial for anyone looking to specialize in these areas.