Unveiling the Power of JavaScript Regular Expressions: Exploring Efficient String Manipulation
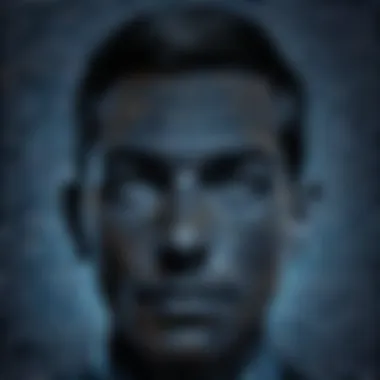
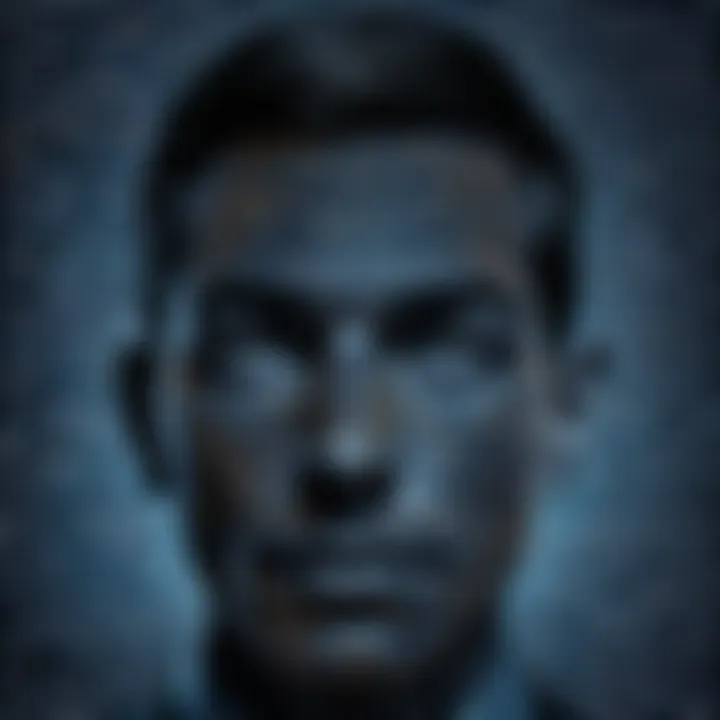
Coding Challenges
This section will delve into the realm of JavaScript regular expressions from a coding challenges perspective, offering insights into how to tackle complex problems using regular expressions. We will explore various weekly coding challenges that test your understanding and proficiency in leveraging regular expressions for efficient string manipulations and pattern matching. From problem solutions and explanations to tips and strategies for mastering coding challenges, this section aims to equip aspiring and experienced programmers with the tools and techniques needed to excel in this domain.
Technology Trends
In the rapidly evolving landscape of technology, JavaScript regular expressions play a pivotal role in shaping the future. This section will examine the latest technological innovations that leverage regular expressions, highlighting their impact on modern programming practices. From emerging technologies to watch in the realm of regular expressions to the societal implications of their widespread adoption, this section will offer expert opinions and analysis on how regular expressions are redefining the technological paradigm.
Coding Resources
A treasure trove of programming resources awaits those looking to sharpen their skills in JavaScript regular expressions. This section will serve as a comprehensive guide to programming language guides that focus on regular expressions, software reviews of tools instrumental for regular expression manipulation, tutorials offering step-by-step instructions on mastering regular expressions, and a comparison of online learning platforms tailored for enthusiasts seeking to enhance their proficiency in this domain.
Computer Science Concepts
Delving into the core principles of computer science, this section will elucidate the foundational concepts that underpin JavaScript regular expressions. From algorithms and data structures primers that showcase the practical applications of regular expressions to the basics of artificial intelligence and machine learning that leverage regular expressions for pattern recognition, this section will also touch on networking and security fundamentals where regular expressions are instrumental and provide a glimpse into the future technologies like quantum computing that stand to benefit from the versatility of regular expressions.
Foreword to JavaScript Regular Expressions
JavaScript regular expressions play a pivotal role in modern programming, offering a robust mechanism for efficient string manipulation and pattern matching. By delving into the depths of JavaScript regular expressions, programmers can unlock a plethora of functionalities that streamline coding tasks and enhance the overall quality of their programs. Understanding the basics of JavaScript regular expressions is crucial for both aspiring developers and seasoned professionals, as it forms the foundation for more advanced techniques and applications.
Understanding the Basics
Anchors and Boundaries
Anchors and boundaries in JavaScript regular expressions serve as essential markers that define where a match should occur within a string. An anchor specifies a position in the string, such as the beginning (^) or end ($) of a line, while boundaries constrain matches to specific word boundaries (\b). These elements are instrumental in enforcing precise matching criteria, ensuring that patterns are located exactly where intended. Anchors and boundaries provide a level of granularity that is indispensable in scenarios requiring pinpoint accuracy in pattern recognition.
Character Classes
Character classes in JavaScript regular expressions offer a means to match a single character from a predefined set of characters. For example, [abc] will match either 'a', 'b', or 'c'. This versatile feature simplifies the process of specifying multiple alternatives within a pattern, reducing the need for repetitive expressions. Character classes streamline pattern creation by encapsulating related characters into a concise declaration, optimizing both readability and efficiency in regular expressions.
Quantifiers
Quantifiers in JavaScript regular expressions control the number of times a character or group in a pattern can appear. These modifiers enable developers to specify ranges, such as matching a character occurring between 0 to n times (?, *, +), or exact repetitions (n). By leveraging quantifiers, programmers can define flexible patterns that accommodate varying occurrences of elements within a string. However, excessive or inefficient use of quantifiers can lead to performance issues, emphasizing the importance of mindful utilization in regex design.
Creating Regular Expressions
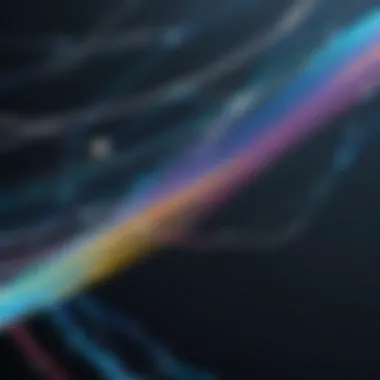
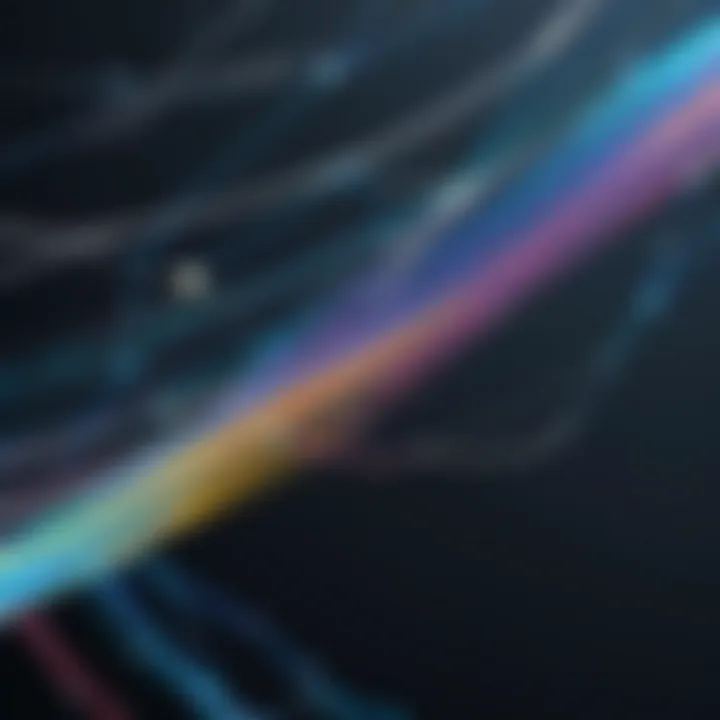
Literals vs. Constructors
In JavaScript, regular expressions can be instantiated using either literals or constructors. Using literals involves wrapping a pattern in forward slashes (//), while constructors utilize the RegExp object. The choice between literals and constructors often boils down to personal preference and specific requirements within a project. Literals offer a compact syntax ideal for straightforward patterns, whereas constructors provide flexibility for dynamic pattern generation during runtime. Understanding the nuances between literals and constructors empowers developers to select the most suitable approach based on the context of their regex tasks.
Flags in Regular Expressions
Flags in JavaScript regular expressions add additional functionality and behavior to the matching process. Common flags include 'g' (global search), 'i' (case-insensitive search), and 'm' (multiline search), altering how patterns are applied to strings. By incorporating flags, developers can fine-tune the matching behavior of their regular expressions, catering to specific requirements in different scenarios. Flags serve as powerful tools in customizing regex operations to achieve desired outcomes effectively.
Common Pitfalls
Despite the versatility of JavaScript regular expressions, several common pitfalls can impede their effectiveness when not properly addressed. Issues such as excessive greediness, overly complex patterns, and inefficient backtracking can lead to unexpected behavior or performance bottlenecks. Additionally, inadequate escaping of special characters and lack of testing can introduce vulnerabilities or inaccuracies in regex implementation. Recognizing and mitigating these common pitfalls is essential in mastering the art of regex design, ensuring optimal performance and accuracy in pattern matching tasks.
Advanced Techniques in JavaScript Regular Expressions
JavaScript regular expressions are not just about basic patterns. Embracing advanced techniques opens up a world of potent possibilities in string manipulation and pattern matching. In this section, we delve into the intricacies that set professionals apart. Mastering advanced techniques equips programmers to tackle complex scenarios efficiently, elevating code quality and performance. Understanding lookaheads and lookbehinds, grouping, and backreferences empowers developers to craft elegant solutions with precision and clarity.
Lookaheads and Lookbehinds
Positive Lookahead: Positive lookahead in JavaScript regular expressions plays a pivotal role in anticipatory matching. By asserting a condition ahead of the main pattern, positive lookahead enables selective matching based on what follows the main expression. This feature enhances the precision and flexibility of regex operations, allowing for intricate pattern validations and extractions. Embracing positive lookahead unlocks a realm of nuanced text processing capabilities, enhancing the efficiency of pattern matching processes.
Negative Lookahead: On the flip side, negative lookahead negates a match if the specified pattern is found ahead of the main expression. This selective exclusion feature is crucial in filtering out undesired matches, refining the accuracy of regex operations. Leveraging negative lookahead adds a layer of sophistication to regex usage, enabling developers to finesse pattern matching tasks effectively.
Lookbehind Assertions: Lookbehind assertions in regex empower programmers to define conditions behind the main expression for selective matching. This backward-looking feature adds depth to pattern validations, enhancing the scope of text processing tasks. By considering the context behind a pattern, lookbehind assertions enrich the expressive power of JavaScript regular expressions, catering to diverse matching requirements.
Grouping and Backreferences
Capturing Groups: Harnessing capturing groups in regex enables the extraction of specific parts of a matched pattern. This targeted extraction mechanism offers precise data retrieval functionalities, enhancing the utility of regular expressions in data processing tasks. Capturing groups streamline information extraction processes, facilitating structured data handling with ease.
Non-Capturing Groups: In contrast, non-capturing groups provide grouping without capturing the matched substring. This feature is instrumental in grouping and organizing subpatterns without affecting the extraction behavior of capturing groups. Non-capturing groups optimize regex formulations, ensuring efficient pattern grouping with a focus on pattern structure.
Backreferences: Backreferences in regex facilitate references to previously captured groups within a pattern. This referencing capability enhances data validation and transformation tasks, allowing for dynamic reuse of matched substrings within the same expression. Leveraging backreferences augments the versatility of JavaScript regular expressions, enabling fine-grained data processing with contextual referencing.
Modifying and Extracting Data
Replacing with Regular Expressions: The ability to replace substrings based on regex patterns revolutionizes data modification tasks. Replacing with regular expressions empowers developers to orchestrate complex string transformations with ease, offering a scalable approach to text manipulation. This feature-rich functionality enhances data cleansing and formatting operations, streamlining data processing workflows effectively.
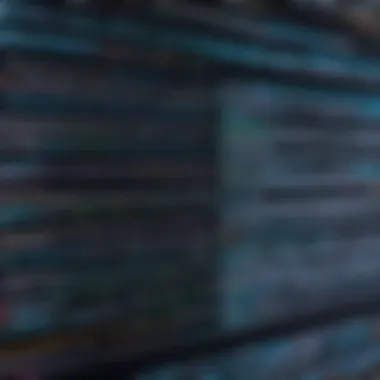
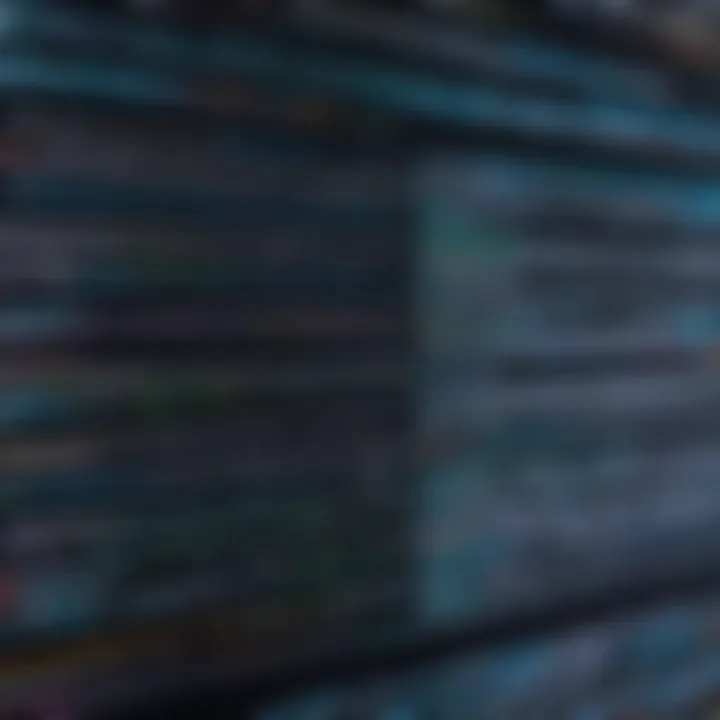
Splitting Strings: Splitting strings using regex patterns facilitates text segmentation based on defined delimiters. This versatile feature supports efficient string parsing and structuring, aiding in data partitioning for further analysis or processing. By leveraging string splitting capabilities, developers can break down text inputs into digestible components, enabling targeted data manipulation and organization.
Extracting Substrings: The extraction of substrings through regex operations allows for selective data retrieval from input texts. This data extraction mechanism empowers developers to extract specific information or segments from textual data based on predefined patterns. Extracting substrings offers a powerful tool for precise data extraction, enabling focused analysis and processing of textual information.
Best Practices and Optimization Tips
JavaScript Regular Expressions offer a wealth of possibilities, making it vital to adhere to best practices and optimize performance. By implementing efficient strategies, developers can streamline their code and enhance overall functionality. Focusing on optimization ensures that regular expressions operate effectively in diverse scenarios, promoting a seamless user experience.
Performance Optimization
Efficient Quantifiers Usage
Efficient Quantifiers Usage plays a pivotal role in enhancing the efficiency of regular expressions. By utilizing quantifiers judiciously, developers can fine-tune their patterns to precisely match the intended criteria. This approach results in optimized performance, reducing processing time and increasing the accuracy of pattern recognition. Embracing Efficient Quantifiers Usage significantly contributes to the overall effectiveness of regular expressions, elevating the quality of string manipulation tasks within JavaScript.
Lazy vs. Greedy Matching
The distinction between Lazy and Greedy Matching is crucial in determining how regular expressions interpret and process data. Lazy Matching prioritizes minimal matching, aiming to fulfill the pattern with the least input possible. In contrast, Greedy Matching seeks maximal interpretation, consuming as much input as feasible to satisfy the pattern. Understanding this disparity enables developers to choose the most suitable approach based on their specific requirements, ensuring efficient and precise pattern matching in JavaScript applications.
Caching Regular Expressions
Caching Regular Expressions empowers developers to store compiled patterns for future use, optimizing performance through swift retrieval and usage. By caching frequently utilized expressions, developers mitigate the computational overhead of recompilation, enhancing the responsiveness of pattern matching operations. This practice not only accelerates processing speed but also promotes resource efficiency, contributing to the seamless execution of regular expressions in JavaScript applications.
Security Considerations
Preventing Regex DoS Attacks
Effective prevention of Regex Denial of Service (DoS) attacks involves implementing safeguards to mitigate vulnerabilities in regular expression processing. By fortifying patterns against malicious exploitation, developers safeguard their applications from potential threat vectors, ensuring robust security measures in place. Preventing Regex DoS Attacks is essential for preserving system integrity and thwarting malicious entities from exploiting regular expressions for disruptive purposes.
Sanitizing User Inputs
Sanitizing User Inputs involves validating and cleansing data provided by users to prevent injection attacks and data manipulation. By sanitizing inputs before utilizing them in regular expressions, developers fortify their applications against potential security breaches and unauthorized access attempts. This practice ensures data integrity and confidentiality, establishing a secure environment for processing user-generated content within JavaScript applications.
Regular Expression Injection
Defending against Regular Expression Injection entails scrutinizing user inputs to detect and neutralize attempts at injecting rogue patterns into regular expressions. By implementing stringent input validation and sanitization mechanisms, developers thwart malicious actors from exploiting vulnerabilities in regular expression parsing, preserving system functionality and data integrity. Mitigating risks associated with Regular Expression Injection is imperative for upholding the security posture of JavaScript applications.
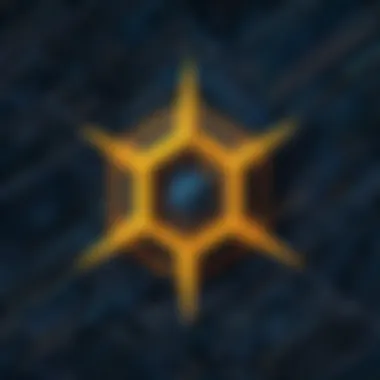
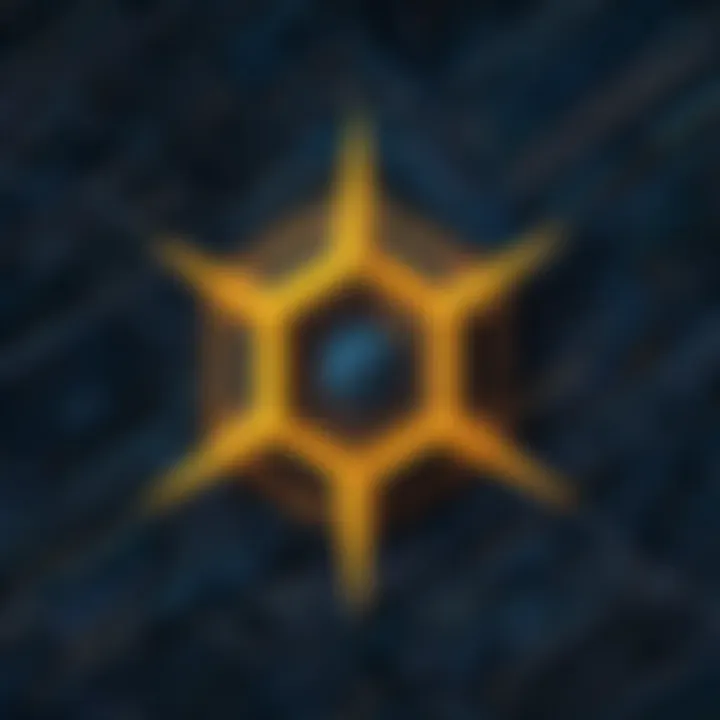
Code Refactoring Techniques
Simplifying Complex Regex
Simplifying Complex Regex enhances the readability and maintainability of regular expressions, facilitating easier comprehension and modification of intricate patterns. By breaking down convoluted expressions into simpler components, developers streamline code structure and foster code maintainability, improving collaboration and troubleshooting efforts. Simplifying Complex Regex empowers developers to cultivate more efficient and scalable regular expressions, enhancing overall code quality and functionality within JavaScript applications.
Reuse of Patterns
Leveraging the Reuse of Patterns allows developers to maximize code reusability and reduce redundancy in regular expression implementation. By defining reusable patterns and referencing them across multiple expressions, developers optimize code efficiency and ensure consistency in pattern matching throughout their applications. This practice fosters modularity and flexibility, empowering developers to build robust and adaptable regular expressions in JavaScript.
Code Readability
Prioritizing Code Readability promotes clarity and comprehensibility in regular expression implementation, facilitating easier maintenance and debugging processes. By adhering to consistent coding standards and documenting patterns effectively, developers enhance the readability of their expressions and promote transparency in code interpretation. Improved Code Readability not only simplifies program comprehension but also enhances collaboration and knowledge sharing among developers, bolstering the efficiency and cohesion of JavaScript projects.
Real-World Applications and Use Cases
In the realm of JavaScript regular expressions, understanding real-world applications and use cases holds significant importance. These practical implementations not only showcase the versatility of regular expressions but also demonstrate their crucial role in modern programming. Whether it's validating form inputs or extracting specific data from web sources, the applications are vast and varied. By exploring real-world scenarios, programmers can grasp the practical implications of utilizing regular expressions for tasks like form validation, data extraction, and text manipulation. This section provides a detailed analysis of how JavaScript regular expressions can be leveraged effectively in diverse real-world scenarios.
Form Validation
Form validation is a critical aspect of web development, ensuring that user inputs meet specified criteria before processing. Within the scope of email validation, JavaScript regular expressions offer a powerful tool for verifying the correctness of email addresses submitted through forms. By defining patterns that validate the structure of an email address, developers can enhance the accuracy of data collection processes. While email validation through regular expressions streamlines the validation process, it is essential to consider the trade-offs between strict validation criteria and user convenience.
Moving on to phone number validation, JavaScript regular expressions provide a means to validate and format phone numbers entered by users. By creating regex patterns that match various phone number formats, developers can ensure consistency in data entry and streamline communication functionalities within applications. However, the challenge lies in catering to diverse phone number formats across different regions while maintaining compatibility and usability.
Lastly, password strength checking via regular expressions enables developers to enforce secure password policies by defining complex password requirements. By incorporating regex patterns that evaluate password strength based on criteria such as length, special characters, and alphanumeric combinations, applications can enhance data security. While regex-based password strength checking enhances security measures, it is crucial to balance stringent password policies with user experience considerations.
Data Extraction
Data extraction involves retrieving specific information from various sources, ranging from websites to databases. Within the context of web scraping, JavaScript regular expressions play a vital role in extracting targeted data from web pages. By creating regex patterns that match specific content within HTML structures, developers can automate the retrieval of desired information efficiently. While web scraping with regular expressions offers a streamlined method for data extraction, it is essential to address challenges such as data consistency and website structure variations.
In the realm of information retrieval, JavaScript regular expressions facilitate the extraction of relevant data from large datasets or documents. By defining regex patterns that identify key information within textual content, developers can streamline the process of retrieving specific data points. While information retrieval through regular expressions enhances data processing efficiency, it is imperative to consider scalability and performance implications when dealing with extensive datasets.
Additionally, data parsing using regular expressions enables developers to parse and structure complex data formats for analysis or manipulation. By utilizing regex patterns to identify data elements and their relationships within strings, applications can parse data effectively for further processing. While data parsing with regular expressions simplifies data interpretation, developers need to account for variations in data formats and complexities during parsing operations.
Text Processing and Manipulation
Text processing and manipulation with JavaScript regular expressions offer versatile functionalities for modifying and formatting text content. When it comes to search and replace operations, regular expressions provide a robust mechanism for finding and replacing specific patterns within a text body. By employing regex patterns to define search queries and replacement rules, developers can automate text editing tasks effectively. However, it is essential to consider potential regex ambiguities or unintended replacements that may impact the integrity of textual content.
Furthermore, tokenization using regular expressions facilitates the segmentation of text into meaningful tokens or units for linguistic analysis or text processing. By creating regex patterns that tokenize text based on predefined criteria such as punctuation or whitespace, developers can structure textual data for various computational tasks. While tokenization enhances text analysis capabilities and linguistic processing, it is crucial to ensure the accuracy and integrity of tokenized output for downstream applications.
Lastly, formatting text with regular expressions enables developers to apply consistent styling or formatting rules to textual content dynamically. By defining regex patterns that match specific text patterns for formatting, applications can automate text styling processes and enhance visual presentation. While text formatting through regular expressions streamlines styling procedures, developers should consider potential rendering discrepancies across different platforms or display environments.