Mastering Angular: Build Your Own Todo App Today
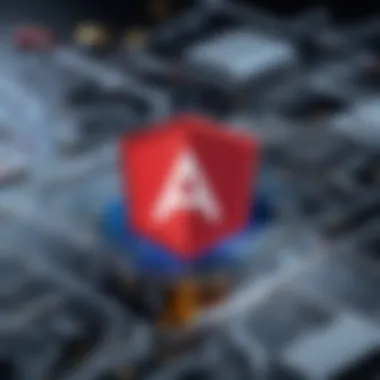
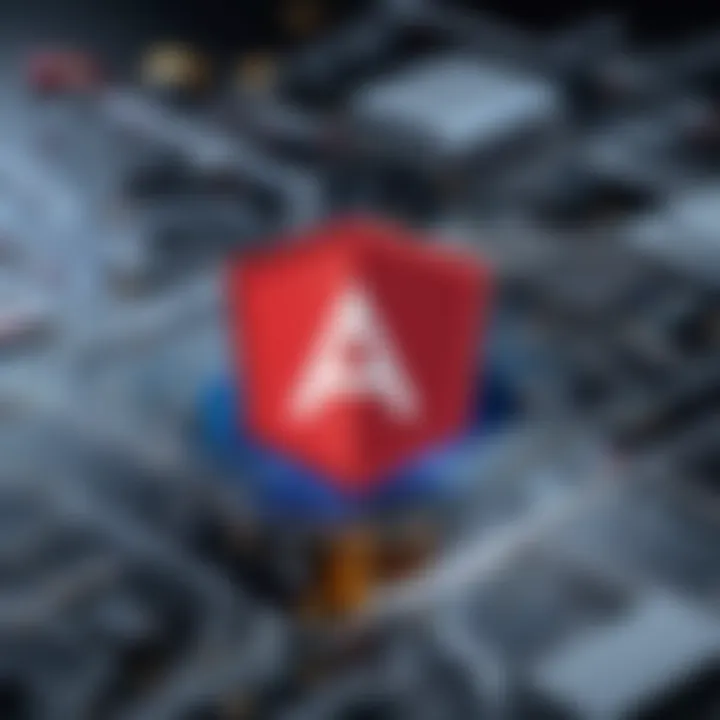
Intro
In the current digital landscape, web applications have become fundamental to how we interact with technology. Amongst a sea of frameworks available, Angular stands out class for building dynamic and responsive single-page applications. One such practical project that effectively demonstrates Angular’s capabilities is the Todo application. Though seemingly simple, a Todo app serves as an excellent foundation to understand the core concepts of Angular, such as components, services, and data binding. This guide aims to thoroughly scaffold your path as you build a feature-rich Todo application.
Understanding the significance of this endeavor extends beyond merely completing an app. It provides a significant insight into structuring smart applications and enhancing user experience. Enthusiasts embarking on this journey will learn about the pivotal elements of Angular which include modules, components, directives, and services. Beyond the coding aspects, this guide sheds light on maintaining code quality and deploying scalable applications.
Through a systematic exploration, the narrative will cover all facets of developing a Todo app, focusing on both theoretical and practical understanding. Each section will encourage readers to engage analytically with concepts which are vital for both novice and experienced programmers.
Coding Challenges
Confronting challenges is a necessity for anyone honing their programming skills. Such experiences provide seasoned developers with opportunities to innovate and newcomers with a deeper grasp of core concepts. To aid Pathfinder enthusiast in building an Angular Todo app, we will outline several coding challenges tailored to enhance critical thinking and problem-solving abilities.
Weekly Coding Challenges
Engaging in weekly coding challenges gives developers the chance to reinforce and expand their knowledge of Angular. For instance, consider creating varying user features such as filtering tasks by priority. The goal is to break down the process into smaller tasks, which aids in solidifying your understanding.
- Tasks to explore:
- Count incomplete todo items.
- Implement user authentication.
- Case-sensitive and fuzzy search features.
Problem Solutions and Explanations
Problems arise in programming but carrying out extensive ecosystems is part of the Angular grooming process. For those keen to address common errors, consider the *
Prelims to Angular
Angular is a powerful framework that enables developers to build dynamic and complex applications with ease. It is essential to grasp its foundations before undertaking projects like a Todo app. Understanding Angular not only streamlines development but also enhances maintainability and scalability of applications. Developers who are well-versed in Angular can harness its capabilities to create robust web solutions efficiently.
Overview of Angular Framework
Angular, developed by Google, is an open-source web application framework primarily designed for single-page applications. It offers a modular architecture that promotes cleaner code and better organization. Key features such as two-way data binding, dependency injection, and reusable components contribute to its effectiveness. The use of TypeScript, a superset of JavaScript, adds static typing capability, which helps in minimizing bugs during development.
Benefits of Angular
- Structured Development: Angular's modularity allows for a structured approach to coding, making it easier for multiple developers to work on the same project without conflicts.
- Reusability of Components: Components in Angular can be reused across different parts of the application, saving time and ensuring consistency.
- Community Support: With a vast ecosystem and extensive documentation, developers have access to a wealth of resources and community guidance, which is invaluable during development.
By comprehending the framework's architecture and capabilities, one can effectively navigate the complexities of web development, which lays the groundwork for constructing a functional application such as a Todo app.
Understanding TypeScript
TypeScript stands as an integral part of the Angular ecosystem. It builds upon JavaScript by introducing static types, enhancing clarity and precision in codes. This structured approach assists in catching errors at compile time rather than runtime, making projects less prone to bugs.
Key Aspects of TypeScript
- Static Typing: This feature allows for defining variable types, preventing common errors in code execution.
- Advanced Object-Oriented Programming (OOP)/JavaScript Features: TypeScript supports class-based OOP concepts, which align well with the structured nature of Angular.
- Better Tooling: Modern IDEs can provide powerful features like IntelliSense, helping developers code more efficiently with suggestions and error alerts.
In essence, understanding TypeScript not only aids in working within the Angular framework but also fortifies the development process by promoting sound coding practices.
Project Setup
Setting up your development environment correctly is a crucial first step in any project. This section will delve into the project setup required to build a Todo application with Angular. Getting off on the right foot not only saves time in the long run but also minimizes potential issues during the development phase. By managing dependencies and configuring tools effectively, developers can create a more streamlined experience. Ultimately, proper project setup lays the groundwork for coding, testing, and deploying an efficient application.
Installing Angular
The Angular Command Line Interface (CLI) is an essential tool for Angular developers. It allows for much easier creation, development, and maintenance of Angular applications.
- Consistency: The CLI creates boilerplate code that adheres to Angular's standards, which enhances project readability and maintainability.
- Automation: The CLI automates repetitive tasks such as building, linting, and testing. This saves developers from manual configurations and lets them focus on code.
- Simplified Updates: With CLI, updating Angular libraries is straightforward and manageable.
To install Angular CLI, you should have Node.js and npm installed first. You can download Node.js from nodejs.org. Run the following command in the terminal:
This command globally installs the Angular CLI on your machine, enabling you to start new projects and utilize its powerful features instantly.
Creating a New Angular Project
Once you have Angular CLI installed, it is clear to create a new project. Running a simple command can bootstrap a new Angular application within seconds. You just need to define the project name as follows:
You can add flags to customize your project setup. For example, you may include routing or choose CSS as a default style. After executing the command, Angular CLI will create all necessary files and dependencies in a folder named . It will give you a solid scaffold to begin your development process with a functional app structure.
Project Structure Overview
Navigating through your project folder and files is essential to understanding how everything fits together. Here's a brief description of the main files and directories you will encounter:
- src: Contains all source code files. This is where you'll spend most of your time.
- app: This directory typically holds your main application module. It is the core component that includes feature modules, components, services, and dependencies.
- assets: Includes images and other media assets used throughout your application.
- environments: Defines environment variables for development and production settings.
This organizational structure promotes modularity, making it easier to scale your todo application as you add more features.
Proper project setup, including understanding the project structure, ultimately leads to a more maintainable codebase. Investing time in this phase pays off during later stages of development and deployment.
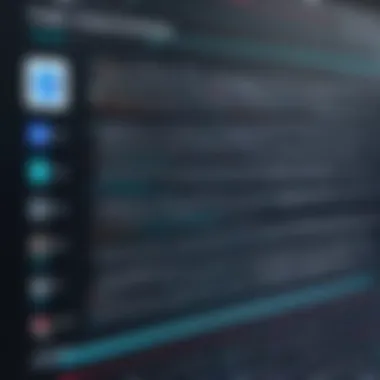
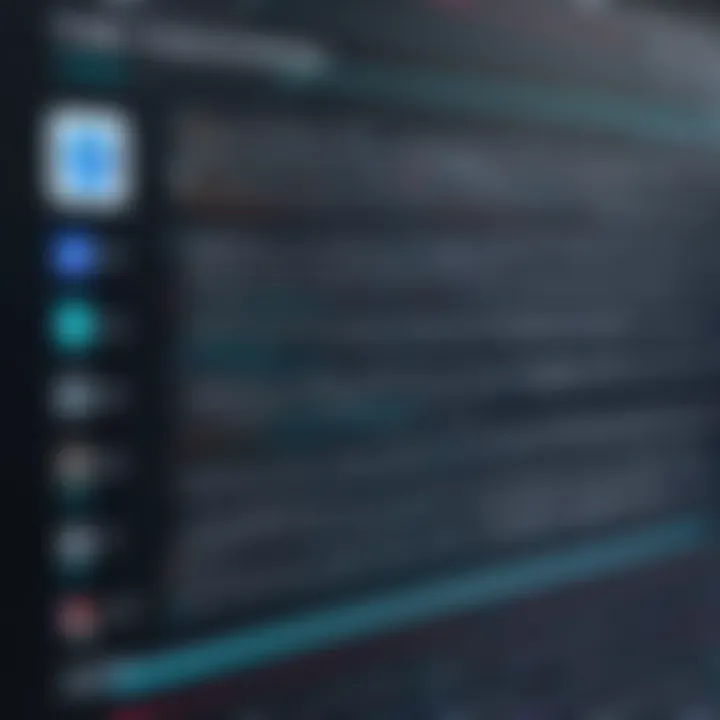
Building the Todo App
Building a Todo application serves as a practical gateway into the world of Angular. It consolidates a broad set of concepts that are pivotal for mastering this framework. Specifically, it provides clarity on component architecture, data flow, and interaction among various elements in an Angular application. This section emphasizes the significance of the Todo application in showcasing Angular's features and flexibility, enabling both new and seasoned developers to glean insights on scalable application design.
Defining the Todo Model
The Todo model establishes the foundation of the application. It identifies the core structure for managing tasks, allowing the application to function efficiently. When defining this model, one ensures that it captures essential attributes such as , , and . This attention to detail enhances consistency within the data handled by the app.
The Todo model should be straightforward, thus it might look like this:
Creating a well-defined Todo model contributes to effective data management, making the subsequent stages of development seamless. Without this critical step, the app can devolve into chaos, leading to integration and debugging woes later.
Creating Components
Components are the skeletal structure upon which Angular applications stand. They encourage modular code development and promote reusability. Each specific functionality of the Todo app will be housed in distinct components. These include the Header, Todo List, Todo Item, and Add Todo components, each serving its unique role.
Header Component
The Header Component plays a pivotal role in unifying the application’s interface. It typically contains navigation elements and application title information. A primary characteristic of this component is its simplicity and movement, providing a clutter-free user experience.
The unique feature here involves being lightweight and persistent across different views of the application, ensuring users always have immediate access to overall task management.
However, while having a Header Component helps with navigation it can also lead to issues if not managed well as it adds to overall component overhead. Thus, careful thought must go into its structure to balance functionality and performance.
Todo List Component
The Todo List Component is essential for displaying all tasks. Its primary function is to organize and render the individual todos in an array format. A significant characteristic is how it handles data through optimizations with Angular’s change detection. Utilizing structural directives such as , this component offers a dynamic visual representation of tasks.
What sets it apart is the capability to toggle visibility and organization of tasks based on completion status. Nonetheless, this can become a drawback if there's a massive data influx, which can cause performance issues, hence tuning the implementation details require attentions.
Todo Item Component
Individual tasks are handled within the Todo Item Component. This component emphasizes the specific attributes of each todo such as description and status, translating generic tasks into visually appealing items.
A key feature of this component is user interaction; it facilitates task completion, edits, and deletions directly. Its responsiveness to change is beneficial since it improves accessibility and user engagement. However, developers need to manage state accurately in relation to user actions because mishandled state updates can lead to inconsistencies.
Add Todo Component
This component handles the creation of new tasks. It includes functionality such as input boxes, submit buttons and validation errors. An essential characteristic of the Add Todo Component is simplicity, ensuring an easy interface for adding new todos.
A unique feature is input validation before adding a new todo to enhance application integrity. Stipulating rules before submission minimizes errors in the application’s workflow. However, if the requirements are overly restrictive, it can lead to a frustrating user experience.
Implementing Services
Services in Angular offer an application-wide abstraction layer, which is necessary for maintaining separation of concerns. They provide clean and efficient ways to deal with shared data across multiple components, forging a more maintainable and organized project approach.
Todo Service
The Todo Service acts as the intermediary between the components and the backend data. It is essential for fetching, adding, updating, and deleting tasks. One of its characteristic traits is its reliance on Observables to communicate changes effectively within the application.
Using a service keeps data handling modular and centralized, making it easy to update or debug. While beneficial, prime caution is needed. If services become too verbose, it can overcomplicate aspects of application logic.
Data Management
Proper data management is crucial for creating a smooth user experience. This includes handling CRUD operations (Create, Read, Update, Delete), validation, and state management. By controlling data efficiently, the overall integrity of the application is compromised.
Good data management systems inject reliability into the application by enforcing rules about data consistency and format. However, improper implementation can lead to performance pitfalls slowing the application down.
Through these components and services, developers pave a detailed path toward an effective Angular application. By ensuring each part works cohesively, a robust Todo application takes shape. The focus remains on maintaining clean, efficient code while understanding the bigger picture of application scalability.
State Management
State management is a fundamental aspect of building applications, particularly in Angular. It involves managing the application state consistently across various components, ensuring that user interactions and imported data reflect correctly throughout the application. State management plays a crucial role in maintaining integrity and flow in data presentation. Without a coherent state management strategy, applications can become challenging to manage and prone to errors.
When building a Todo application, effective state management provides numerous benefits. Dissociating state from component specific logic ensures that everything draws from the same source of truth. This confers the ability to simplify data handling, improve performance, and facilitate testing.
Preamble to State Management
State management refers to the way application data is stored, retrieved, and manipulated. In a Todo app, it drives how users interact with tasks, like adding, removing, or updating items on their list. Angular provides several built-in tools to establish and organize state management; among notable instruments are services, observable patterns, and NgRx. Understanding how to utilize these tools can significantly elevate the app's responsiveness and effectiveness.
For instance, a centralized service can be evaluated to handle updates to the task list, interacting with distinct components while every part gets notified accordingly when changes occur. This encourages cleanly written code adherent to principles of reactivity. Therefore, managing state efficiently allows developers to implement time-saving strategies while enriching user experiences.
Using Observables for State
Observables play a significant role in Angular's infrastructure. Leveraging them allows developers to react properly to state changes. Observables offer a powerful alternative to managing state updates. They provide a stream of data various components can subscribe to, making them particularly useful when asynchronous operations or multiple data sources are involved.
For example, if a Todo is added, every component receiving the observable can independently react without additional subscription setup. This decoupling creates a more maintainable and scalable structure. Observables fit seamlessly with Angular's architecture, providing significant flexibility with reactive programming. In scenarios involving extensive user interactions, such as filtering tasks by status or due dates, their use will enhance the performance of your application. The confirmatory instance is that users receive real-time updates without any perceivable delay.
Implementing observables entails using the interactive functionalities of RxJS and thus contributes appropriately towards effective state management within your Angular Todo app. Expect reliance on advanced Angular tools as a means to manage application states more effectively.
Routing in Angular
Routing is a fundamental aspect of modern web applications, enabling a seamless user experience. In this guide, we delve into the critical role that routing plays in an Angular Todo App. Routing facilitates navigation between various components of the application. This enhances user interaction by allowing them to access different parts of the application without requiring a full page reload. Therefore, understanding Angular routing is essential not only for functionality but also for improving the overall user experience.
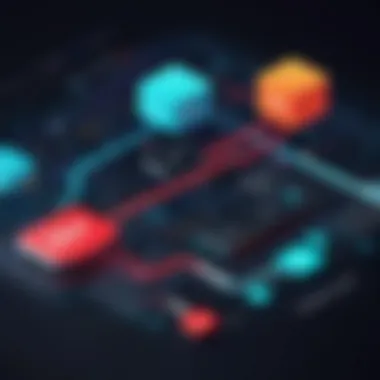
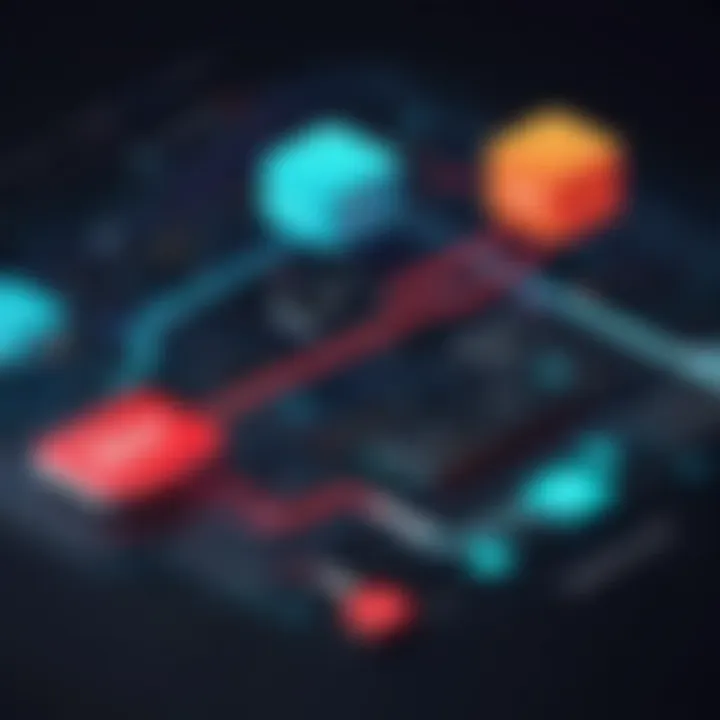
With proper routing, not only can one change the URL, but the content is dynamically updated to reflect the new state. This makes the app feel more like a native application.
Setting Up Angular Routing
To get started with Angular routing, begin by ensuring you have routed modules in your app. During project generation, you can enable routing by choosing to include the router module. If you missed it, you can imported into the main app module as seen below:
This code sets up a basic routing configuration. Here, routes lead to designated components based on the defining paths.
Navigating Between Components
Once routing is set up, navigation between components is simplified. Use the Angular router-link directive in your templates to link between routes. For instance:
This creates a clickable link that will take the user directly to the Todo List component. Additionally, @angular/router handles navigation seamlessly, preserving the app state while transitioning between views.
Important Considerations:
- Ensure Path Matching: Angular supports route parameters and wildcards for complex navigations.
- Router Guards: Use guards for protecting routes from unauthorized access.
- Lazy Loading: For optimizing performance, implement lazy loading for certain modules that are resource-intensive.
By tailoring routing functionalities effectively, Angular developers can craft responsive applications ready for dynamic user interaction.
Styling the Todo App
Styling is a critical element in web development, especially for a Todo application. When users interact with an app, the visual appearance shapes their user experience. A well-styled interface attracts users and encourages them to engage meaningfully with the features of the app. In Angular development, the styling adds an essential layer that intertwines with components, improving usability and accessibility.
By implementing effective styling practices, you can enhance your application's aesthetic appeal and functionality. Custom styling adds to an app’s identity, which is significant if you're aiming to differentiate your Todo application from a myriad of others. A thoughtfully styled app not only captivates the audience but also provides cues about its functionality.
Considering responsive design is also crucial. Users access applications on various devices, and if your Todo app is not responsive, users might face frustrations. It leads to poor user retention. Angular offers capabilities to facilitate adaptive style settings—with Angular Material and CSS giving you the tools to achieve a polished, responsive design. Know how to leverage these tools wisely.
Using Angular Material
Angular Material is a UI component library specifically designed for Angular applications. It incorporates Material Design principles that provide guidance on styling and interaction interfaces. Using Angular Material can considerably simplify the styling process.
- Pre-built Components: Angular Material comes with predefined UI components like buttons, dialogs, and lists. These components help maintain a consistent aesthetics across your application.
- Responsive Layouts: The library offers features such as grid lists and responsive layouts which are customizable, effectively preparing your Todo app for various screen sizes.
- Accessibility Features: Built-in screen reader compatibility ensures the app functions well for all users.
Integrating Angular Material involves modifying your Angular project configuration to include it. Run this command to install Angular Material:
After installation, you can start creating components with established templates, saving you time on frontend development.
Custom Styles with CSS
While Angular Material provides provided components, adding your custom styles enables uniqueness in the application’s look. Tailoring the standards provided by Angular Material while also using CSS directly empowers your technical creativity.
- Scoped Styling: Angular allows for component-level styles. This scoped styling ensures your changes won't impact other components inadvertently.
- Media Queries: With custom styling, setting specific media queries helps ensure functionality across all devices while targeting different screen widths, heights, and orientations.
Consider the user interface hierarchy when applying styles. The core functionality should remain prominence. For instance, the primary actions should be clear and intuitive.
To define styles for your component, an example CSS file might look like this:
Testing the Application
Testing an Angular application is vital for ensuring its reliability and functionality. In any software development lifecycle, testing acts as a safety net that catches issues early on. For a Todo application, effective testing guarantees that core features such as adding, editing, and deleting tasks work as intended.
Testing the application helps identify bugs, improves software quality, and fosters confidence in features before deployment. By employing proper testing strategies, developers can uncover integration issues and confirm performance criteria. There exists a strong correlation between well-tested applications and higher user satisfaction, making testing an indispensable practice in software development.
Unit Testing with Jasmine
Unit testing refers to testing individual components in isolation, verifying both their correctness and functionality. Jasmine is a popular framework for writing unit tests in Angular. Implementing unit tests helps catch errors and regressions. This is particularly important when features undergo modifications or enhancements.
Using Jasmine, basic structure for a unit test includes:
- Description of test suite.
- Implementation of test cases.
Here is an example of how to write a simple unit test using Jasmine:
As shown in this sample, developers can harness the power of assertions to validate different outcomes. Elements like and statements provide a clear outline of what is being tested, keeping code well-organized. Emphasizing accuracy during unit testing streamlines eventual integration into the larger app, minimizing complications.
End-to-End Testing with Protractor
End-to-end testing focuses on validating the entire workflow of the application, simulating real user scenarios to ensure all components function in harmony. Protractor is a testing framework specifically designed for Angular applications, allowing developers to test applications like an end-user would.
With Protractor, one writes tests that interact with the UI, performing actions such as clicking buttons, filling out forms, and navigating between pages. Key advantages include:
- Coverage of the entire application execution flow.
- Ability to catch integration errors among various components.
A simple example of an end-to-end test written using Protractor might look like:
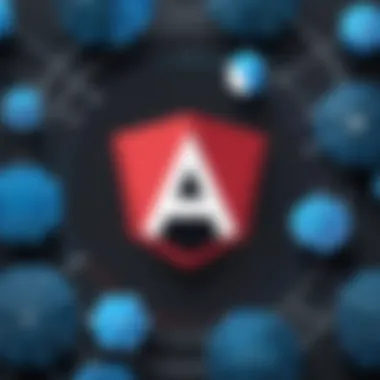
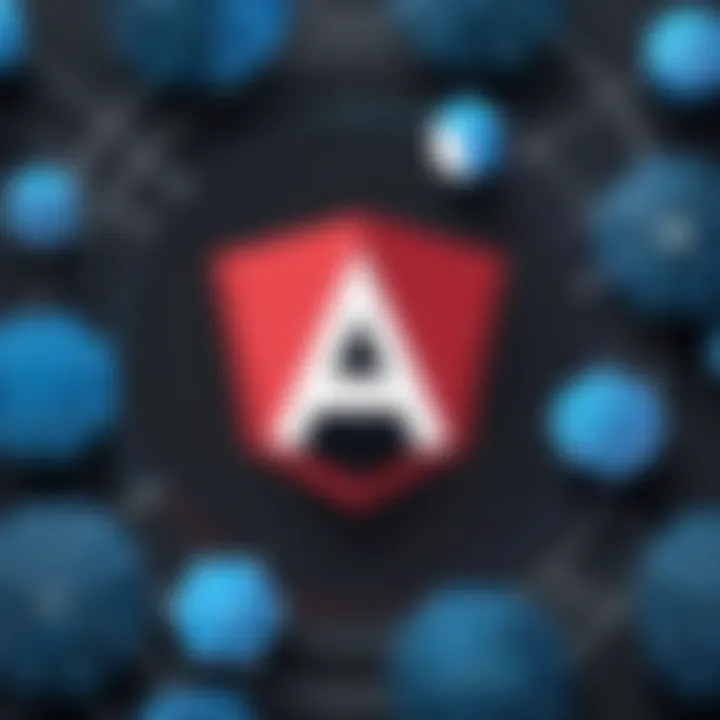
This test checks that the app correctly adds a new todo after inputting data and clicking a button, showcasing Protractor’s seamless integration with various Angular features. By conducting thorough end-to-end tests, developers ascertain that user interactions yield the desired outcomes, ensuring the application upholds quality standards.
Effective testing strategies lead to fewer incidents in production, ultimately enhancing user experience and reducing maintenance costs.
Deployment Strategies
Deployment strategies involves understanding how to effectively release and manage applications in a live environment. In the context of an Angular Todo app, this topic is vitally important. It ensures that the application is not only functional but also reliable and scalable when accessed by multiple users. Having efficient deployment strategies can minimize downtime and improve the overall user experience. Considerations such as security, popularity of hosting services, and continuous integration play a significant role in selecting the right deployment approach.
Additionally, effective deployment can enhance performance, enable rapid updates, and reduce maintenance frequencies. Tackling deployment from the onset allows teams to tailor their project needs and goals simulations that are crucial for the app's achieving good reach and performance as it scales.
Building for Production
Building for production is essential for making sure your application runs smoothly, especially under load. It involves preparing the code, optimizing it, and ensuring correctness. Optimized builds help to cut down unnecessary file sizes while ensuring the necessary features and functionalities. A poorly constructed build can resource depletion, slow response times, and an overall ineffective user experience.
To create production-ready builds with Angular, you would usually run the following command:
This commands signals Angular CLI to compile your app into an optimized package stored in the folder. The resulting output features minification and tree-shaking, reducing the total footprint your application has, while improving speed and efficiency.
Deploying with Firebase
Firebase is one popular solution for deploying Angular applications. As a cloud-based solution from Google, Firebase significantly simplifies the hosting process. It offers free-tier hosting with an easy setup process. Using Firebase ensures that your app can dynamically handle scaling requirements, maintaining good performance regardless of growing user demands.
To deploy your Angular Todo app to Firebase, follow these steps:
- Install the Firebase CLI. Use command:
- Login to firebase: Authenticate yourself:
- Initialize your project: Run in the project directory:
- Deploy: Simply deploy your changes with:
Once the deploy is complete, your app is live, and people can start using it. With Firebase's technology, various services such as real-time database, storage, and authentication can easily integrate into your app as you develop it further.
Firebase allows building and deploying at scale. Merging development process ensures the maintenance is done with speed and efficiency.
Best Practices and Optimization
In software development, particularly within frameworks like Angular, adhering to best practices and optimization techniques is essential for building robust applications. This section focuses on how thoughtful coding practices can greatly benefit the performance, maintainability, and scalability of your Todo app. By implementing these strategies, developers not only enhance the user experience but also simplify future updates and develop a clear architectural approach.
Code Optimization Techniques
Code optimization refers to the process of making code run faster or use fewer resources without sacrificing accuracy. It is an integral aspect of Angular application development, impacting both performance and overall functionality. Here are important techniques developers should consider:
- Lazy Loading: A powerful optimization that allows you to load modules only when they are needed. This reduces the initial load time and enhances application responsiveness.
- Change Detection Strategy: Angular has a default change detection method that checks the entire component tree whenever a change occurs. Implementing the strategy allows you to limit checks and improves speed.
- AOT Compilation: Ahead-of-Time (AOT) compilation helps in converting Angular HTML and TypeScript code into JavaScript during the build process. This results in faster rendering and lower runtime errors.
- Avoiding Unused Imports: Remove unused imports and components as these increase the bundle size, affecting load times negatively.
- Use Observables Efficiently: Using observables smartly helps manage asynchronous operations without blocking the main execution thread, increasing performance.
“Writing optimal code today means your application is quicker tomorrow.”
These techniques, when combined effectively, will result in a more optimized and streamlined application.
Performance Tuning the Angular Application
Performance tuning involves altering system parameters to achieve better efficiency. For Angular applications, it means ensuring that the app runs exceptionally well under different conditions. A Todo app, regardless of its complexity, can face performance issues as user data grows. Here are specific tuning methods to consider:
- NgZone: Fine-tune change detection by manipulating the NgZone class, which controls how Angular's change detection gets triggered and optimizes resource allocation.
- TrackBy in NgFor: By employing the function within directives, you can reduce superfluous DOM manipulations, which can dramatically enhance rendering times.
- Reduce bundle size: Use tree shaking and optimization techniques in the application build process to eliminate dead code that clouds initial load times.
- Optimizing Images: Use optimized images or SVGs to maintain fast loading speeds, vital for user experience.
- Server-Side Rendering (SSR): Implementing server-side rendering provides a faster loading experience as the initial HTML is sent directly from the server, improving perceived performance.
Incorporating these performance-tuning strategies will result not only in quick load times but also in a smooth user interaction, maximizing the overall effectiveness of your Angular Todo application.
The End
In this section, we finalize our discussion on developing a Todo application using Angular. This examination of the entire process—ranging from foundational concepts to deployment strategies—serves an essential purpose. It not only reiterates the importance of mastering angular but also encourages adapting these principles to various types of applications.
Reflecting on the development process is crucial. It allows developers to critically assess their choices made throughout the journey. Comprehension of pitfalls and successes guides future projects and itself fosters continuous improvement.
Reflecting on the Development Process
Reflecting on the development process is important. It creates space for self-evaluation. A detailed review can reveal insights that might be fleeting amid the hectic pace of programming. Methods used, time taken, and productivity can all come under scrutiny. Such reflection aids programmers to identify strengths and areas for improvement.
Also, documentation plays a vital role. Well-commented code captures decisions and the rationale for actions. This can be invaluable for future projects, harkening back to previous experiences can speed up development phases significantly.
- Encourage code review practices among team members.
- Organize regular retrospective meetings.
- List down possible enhancements for future implementations.
These measures create a reflective culture which is fundamental in the tech world, promoting both individual and collective growth among developers.
Future Directions for Angular Development
As we consider future directions for Angular development, we recognize signs of rapid evolution within the framework. Angular is continuously being refined for improved performance and enhanced user experience. Emerging techniques and tools will certainly play a pivotal role in shaping this evolution, ensuring that Angular remains competitive and relevant.
Continued integration with tools like server-side rendering remains beneficial. With frameworks such as Next.js gaining traction against React, the Angular ecosystem could explore similar approaches. Industrial trends toward microservices suggest that Angular could also extend its boundaries for specific-focused applications. This specialization approach fits well with the increasingly modular development practices.
Integrating machine learning is another exciting potential direction. Angular's data binding features blend nicely with ML algorithms. This can open avenues for developing intelligent applications that learn and adapt to users' needs.
As Angular continues to evolve, they may need to place emphasis on a better developer experience. Improved tooling and comprehensive documentation will always attract new users while retaining experienced developers.
In closing, developers crafting their competencies in Angular find that grasping these principles bolsters both immediate and long-term success in application development. Alongside this guide, aspiring programmers and seasoned practitioners are equipped to navigate the advanced facets of Angular. Whether through continual education, exploration of cutting-edge techniques, or consistent practice in real-world applications, the future is full of promise for those who invest their time into mastering Angular.