Mastering Iteration Techniques and Applications in Python
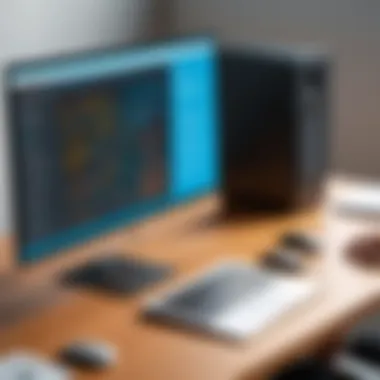
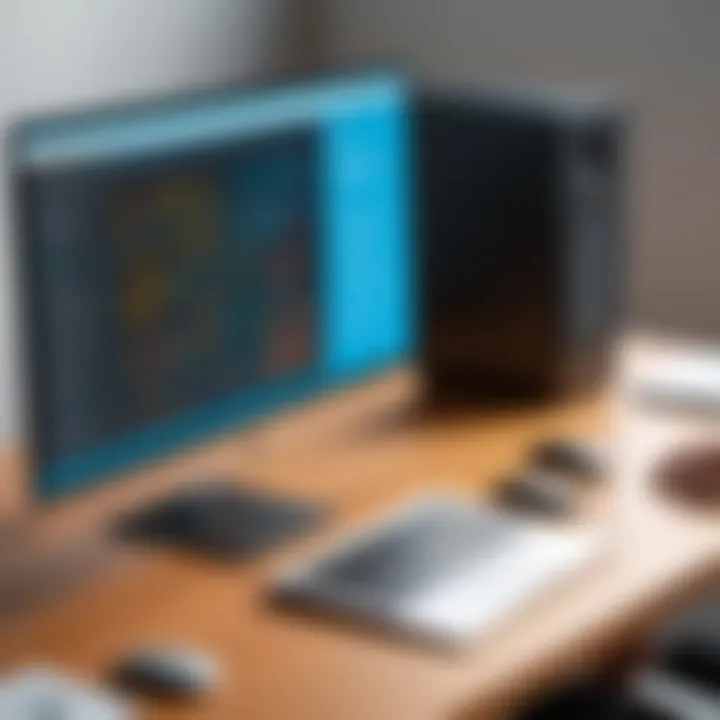
Intro
Iteration is a central concept in programming that allows developers to efficiently manage processes involving repetitive tasks. In Python, this idea is brought to life through various types of loops and iteration techniques. Understanding how to harness the power of iteration can significantly enhance code efficiency and reduce redundancy.
When we talk about loops, we often think about simple actions, like counting or traversing through a list. But there's more beneath the surface. Each loop type offers unique advantages and potential pitfalls, especially as they relate to different data structures. In this piece, we aim to unravel the complexities surrounding iteration in Python, equipping both novice and seasoned programmers with the knowledge to write cleaner, more effective code.
From foundational concepts to advanced applications such as generator functions and custom iterators, our exploration will shed light on the significant facets of iterative programming.
Moreover, we will cover common challenges and strategies helpful for solving coding problems that leverage iteration. With this comprehensive guide, you’ll deepen your understanding while refining your programming skills. Join us as we navigate the intricate landscape of iteration in Python.
Understanding Iteration
Iteration is a fundamental concept in programming, especially in Python. It allows developers to efficiently execute repetitive tasks without cluttering their code. When we speak of iteration, we refer to the process of executing a set of instructions multiple times until a specified condition is met. Understanding the ins and outs of iteration is crucial because it not only aids in writing cleaner code but also enhances performance, especially when handling large datasets or automating tasks.
In Python, iteration can be accomplished using loops, which fall into various types such as for loops and while loops. These constructs provide the means to traverse through collections, enabling programmers to manipulate data flexibly. Therefore, a solid grasp of iteration techniques lays the groundwork for more advanced programming concepts.
Definition and Importance of Iteration
Iteration refers to the process of executing a sequence of instructions repetitively. Each repetition is often referred to as an iteration itself. This programming principle can significantly reduce redundancy in code. Instead of repeatedly writing the same code blocks, iteration allows the same set of operations to be executed multiple times through concise control structures.
Its importance cannot be overstated:
- It promotes code reusability.
- It reduces potential errors associated with writing similar lines of code repeatedly.
- It simplifies complex processes by breaking them down into manageable steps.
For instance, imagine needing to calculate the sum of numbers from 1 to 100. Without iteration, you would have to manually add each number, which quickly becomes impractical. In contrast, a simple loop enables you to achieve this with just a few lines of code:
The Role of Iteration in Programming
Iteration plays a pivotal role in various programming scenarios. From data manipulation to generating dynamic content, iteration impacts nearly every aspect of programming. In data analysis, for example, iteration is invaluable when processing large datasets.
Moreover, the functionality of iteration is not limited just to lists or numerical data. You can iterate over strings, dictionaries, and more, providing a versatile approach to data handling.
Understanding how** loops interact with data structures changes the game**, allowing developers to efficiently retrieve, process, and store information without cumbersome and inefficient coding practices. In essence, iteration empowers programmers to innovate and push the boundaries of what's possible with code.
Basic Concepts of Loops
Understanding loops is essential in Python programming, as they bring efficiency and power to the way we handle repetitive tasks. Loops allow programmers to execute a block of code multiple times, depending on specific conditions or the contents of a data structure. This concept is foundational in programming as it allows for automation and simplification of code, ultimately enabling developers to write more effective scripts and applications.
When thinking about loops, it’s clear they provide significant benefits. For one, they help reduce redundancy in code, which can be a major source of errors and maintenance headaches. Imagine having to write the same line of code over and over – it’s not only tedious but also increases the chances of introducing bugs when changes are necessary. Loops eliminate much of that hassle by allowing a single set of instructions to run repeatedly.
Additionally, loops enhance the flexibility of code. They enable developers to work with different data structures seamlessly, iterating through elements without needing to know the size in advance. This adaptability becomes particularly useful when dealing with collections such as lists, dictionaries, or the like.
In essence, loops are not just a helpful tool; they are a necessity in developing robust and maintainable software. Taking the time to understand how they operate and the different types available lays the groundwork for more complex programming tasks.
What is a Loop?
A loop is a programming construct that allows for the repeated execution of a block of code. The basic idea is straightforward: under certain conditions, the same lines of code get run over and over again. Think of it like a merry-go-round; once you get it spinning, it keeps going until you choose to stop it.
In Python, there are primarily two types of loops: for loops and while loops. A for loop iterates over a sequence (like a list or a string), executing the block of code for each element. On the other hand, a while loop continues iterating as long as a specified condition remains true.
Here’s a simple illustration:
In the example above, the loop will print numbers 0 through 4. It's a clear demonstration of how looping can tackle repetitive tasks with minimal code.
Though this construct seems straightforward, at a deeper layer, the inner workings reveal an intricate dance of control flow and conditions that powerfully influences how your program executes its logic.
How Loops Work Behind the Scenes
To appreciate loops fully, it's necessary to delve deeper into how they function internally. Every loop has a lifecycle, starting with initialization. When a loop starts running, certain conditions are checked, and if they're met, the code inside the loop executes. After execution, the control returns to the condition check. If the conditions still hold true, the loop repeats; if not, execution stops.
You might think of it like a traffic light. The light turns green, allowing cars to go through; once it turns red, the cars stop. This logic keeps the flow controlled and prevents chaos.
For loops generally use an iterator, a protocol that gives access to elements within a sequence. When the iterator runs out of items, the loop halts gracefully without causing errors. Whereas while loops rely heavily on a condition that could affect the day-to-day performance of your applications; they could potentially lead to unintended infinite loops if not correctly implemented.
Important Note: Just like a door locked from one side, when a while loop's condition does not change as intended, it can lead to what's called an infinite loop, causing your program to run indefinitely.
For Loops in Python
In Python, understanding how to utilize for loops effectively is paramount for any programmer looking to harness the full potential of the language. For loops offer a structured and efficient means to iterate over various data structures and sequences. They allow you to automate repetitive tasks with ease, improving both the readability and performance of your code. By mastering for loops, developers can handle collections of data seamlessly, making it an indispensable tool in a programmer's arsenal.
Syntax and Structure
The syntax of a for loop in Python is straightforward. At its core, a for loop typically consists of a loop variable, the keyword , the name of the collection to iterate over, and a block of code that executes for each item in that collection. Here is a simple yet effective example:
This structure makes it easy to read and understand the flow of the code. The loop variable takes on each value in , executing the code block for each iteration. It’s intuitive, which is a hallmark of Python and a reason many new programmers are drawn to it.
Iterating Over Sequences
When it comes to iterating over sequences, for loops really shine. Whether you’re working with lists, tuples, strings, or even ranges, for loops provide the means to traverse these data structures efficiently. For instance, if we have a list of fruits, we can loop through them as follows:
This loop will output each fruit in the list, one by one. The simplicity and directness of this loop empower programmers to manipulate data with minimal overhead, both in terms of lines of code and cognitive load.
Using Range with For Loops
Often, you might find yourself needing to execute a for loop with a specific number of iterations. The function is a go-to option here, providing a sequence of numbers to iterate over. For example:
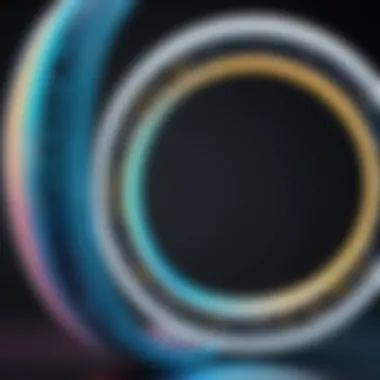
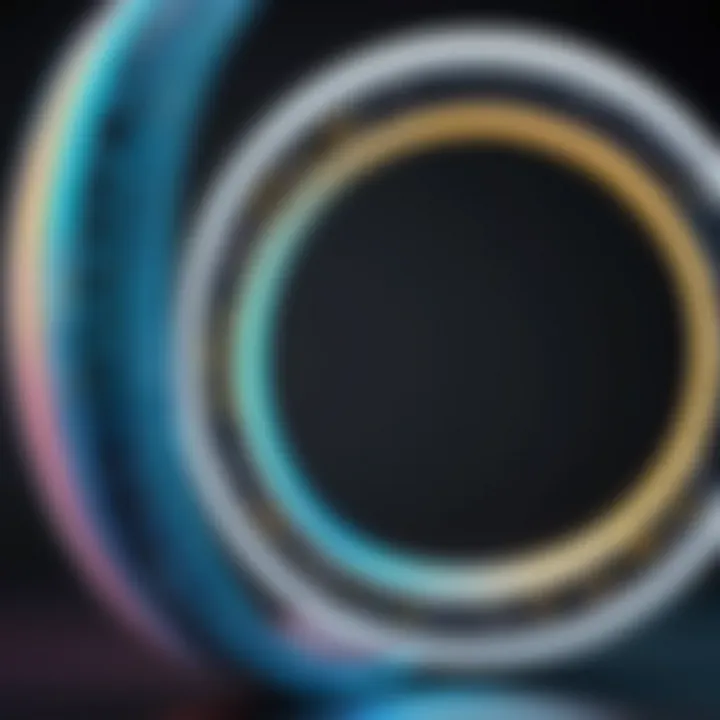
This code generates output from 0 to 4. The function is flexible, allowing you to specify start and end points, as well as an increment, making it incredibly versatile.
Remember: By combining loops with , you can loop a precise number of times, which is especially handy for scenarios like needing to manipulate lists based on their index positions.
For instance, you can create a loop that iterates through all elements of a list while also having direct access to the index — something that’s not always as straightforward with other methods.
In summary, for loops in Python are fundamental constructs that improve code clarity and efficiency. With a sound grasp of their syntax, ability to iterate over various sequences, and effective use of the function, programmers can enhance their workflows and tackle problems more systematically.
While Loops in Python
While loops play a pivotal role in Python, offering a method to repeatedly execute a block of code as long as a specified condition holds true. This flexibility proves invaluable when the number of iterations cannot be predetermined at the outset, contrasting with for loops which iterate over a predefined range or collection. While loops provide a natural way to handle ongoing processes and situations which depend on dynamic variables, making them a cornerstone of control flow in programming.
Defining While Loops
A while loop consists of a simple structure that consists of a keyword followed by a condition. Here's a general form:
In this construct, the code inside the loop continues to execute as long as the condition evaluates to true. Let’s say we want to count up from 1 to 5. We can implement it like this:
This iterative structure is beneficial when the limit is unknown until runtime. For example, perhaps you're querying a database and want to keep retrieving records until no more remain.
Creating Condition-Based Iterations
Creating condition-based iterations with while loops is a powerful practice in programming. It empowers developers to establish iterations based on runtime conditions, enhancing the interactivity of their code. Imagine a scenario where a user is prompted to input a password until they get it right.
This example shows how you can repeatedly execute a piece of code, which in this case is taking user input, until achieving the desired outcome. Clearly defining conditions allows for diverse implementations, from user-driven applications to data-crunching scripts.
Understanding Infinite Loops
One must tread carefully when utilizing while loops, as they possess a propensity to create infinite loops. An infinite loop occurs when the condition controlling the loop never resolves to false. These loops continue indefinitely, consuming system resources and potentially freezing applications.
For instance, consider the following flawed code:
In this case, without the , the loop never terminates. Detecting and preventing infinite loops requires prudence. Always ensure that your condition will eventually turn false, often by including logic that modifies the iterating variable or confirming that the loop will eventually end under any conditions that may arise.
Important tip: Always test your loop's boundary conditions to prevent infinitely looping situations, which can lead to headaches down the line.
Understanding the capabilities and limitations of while loops will enhance your ability to write effective, readable, and efficient Python code. Using these loops wisely opens up avenues for creating responsive, adaptable, and insightful programs, demonstrating the true versatility of Python as a programming language.
Nested Loops
Nested loops are an essential concept in programming, particularly in Python. They allow developers to iterate through multi-dimensional data structures, enabling more complex operations on data that isn’t just neatly arranged in a single list or sequence. Understanding nested loops is significant because they can simplify tasks that require multiple levels of iteration, though they come with their own set of considerations that programmers must learn to navigate.
What are Nested Loops?
In simple terms, nested loops refer to a loop inside another loop. The inner loop runs completely for each iteration of the outer loop. This means that the outer loop controls how many times the inner loop executes.
To paint a clearer picture, imagine this scenario:
- Outer Loop: Iterates through rows in a matrix.
- Inner Loop: Iterates through every element in each row.
The structure looks as follows:
When you deal with data structures like lists of lists, nested loops become necessary. You can't just treat these collections as flat data; instead, understanding their hierarchical nature is crucial for effective iteration.
Practical Use Cases of Nested Loops
Nested loops shine in various applications, especially when dealing with complex data. Here’s a snapshot of situations where they come into play:
- Matrix Operations: Often, a developer needs to perform calculations on all elements of a matrix. For example, adding two matrices involves iterating through rows and columns.
- Generating Combinations: When finding combinations or permutations, nested loops can be handy. If you're generating all possible pairs from two lists, nested loops will let you pull this off without a hitch.
- Creating a Grid: When drawing a grid in a graphical interface or handling pixels in an image, you require nested loops to traverse every pixel location accurately.
Nested loops are powerful, but tread carefully; they can increase computational complexity exponentially.
However, be mindful; they can lead to performance issues if not used judiciously, especially with large data sets. Understanding when and how to use nested loops optimally is key to writing efficient Python code.
Overall, nested loops are not only important for many programming scenarios, but mastering their use can greatly enhance a programmer's skillset, making seemingly insurmountable tasks manageable.
Iteration and Data Structures
When it comes to Python programming, the connection between iteration and data structures is undeniable. Understanding this interplay allows developers to effectively manipulate collections of data—be it lists, sets, or dictionaries. The efficiency and capability of a program can often hinge on how well these structures are navigated during iterative operations.
Iterating Over Lists
Lists are one of the most fundamental and versatile data structures in Python. They can hold various types of items in an ordered manner, making them easy to traverse using iterations.
When iterating over lists, Python provides a straightforward approach. The simplest method is to use a for loop as shown:
This snippet will print each element in the list in sequence. The beauty of using loops here lies in their ability to simplify operations like searching or transforming items. For instance, one could perform actions on every element, or filter results based on specific conditions without complex code.
Moreover, incorporating list comprehensions makes iteration concise and readable. Instead of using several lines of code with a loop, you might condense it:
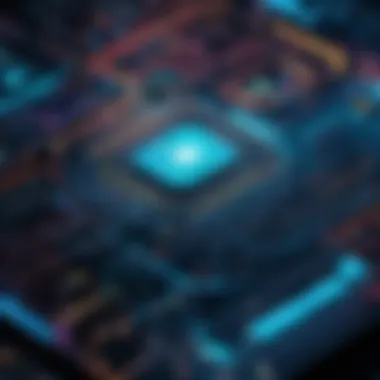
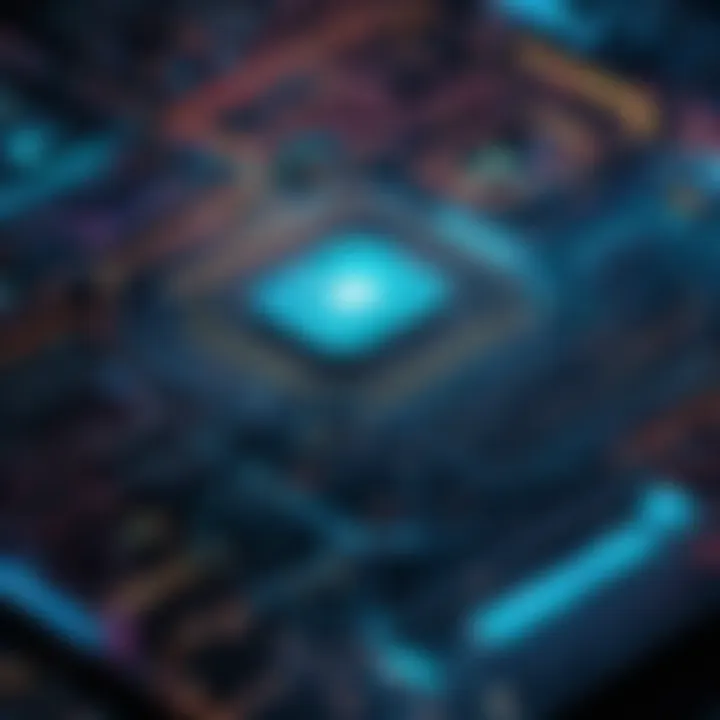
This one-liner achieves the same goal, transforming the original list into a new list of squared values. At the heart of it, utilizing lists within iterations is more than about looping through values; it allows programmers to think creatively about data manipulation and transformation.
Working with Tuples and Sets
Tuples and sets, while less frequently interacted with than lists, also play crucial roles in Python's ecosystem. Tuples, being immutable sequences, can be traversed in a manner similar to lists. Their rigidity ensures data integrity during iteration.
For example:
Meanwhile, sets, which are unordered collections of unique elements, come with their own peculiarities. They don't support indexing like lists do, but one can still iterate through them without a hitch. Here's how you’d do it:
When working with sets, it’s important to remember that the order of elements can vary with each iteration, making them ideal for scenarios where uniqueness and speed are the priority, rather than order.
Using Dictionaries with Loops
Dictionaries offer a mapping structure which combines keys and values, providing powerful ways to store and retrieve data. Iteration plays a key role here as well, as it allows you to access both keys and their associated values effortlessly.
To iterate through a dictionary:
This approach not only enables access to the keys but also their values without additional steps. Beyond that, dictionary comprehensions can further optimize the process. Here’s an example of creating a reverse mapping:
With just a single line, a new dictionary is obtained where values and keys are flipped, showcasing Python's capacity for efficiently handling intricate operations.
In summary, understanding how to elegantly iterate over various data structures opens up the landscape of Python programming. It is key to mastering data manipulation, uncovering new efficiencies, and ultimately crafting code that is both dynamic and maintainable.
Key Takeaway: Mastering iteration over different data structures will empower you to write Python code that is not only effective but also elegant and efficient.
Generating Iterators
When one thinks of iteration in Python, the concept of generating iterators often doesn't come to the forefront of the mind. However, it’s a pivotal aspect that unlocks a new dimension of programming efficiency. Generators act as a bridge between memory management and the need for streamlined data processing. They allow you to create custom iterators and yield values one at a time, thus saving memory as compared to returning a full list of items at once. In essence, they not only enhance performance but also simplify code readability.
Preface to Iterators
At its core, an iterator is an object that enables traversing through a collection of items, be it a list, tuple, or a more complex structure. The beauty of iterators lies in their laziness; they generate items on-the-fly instead of storing them all in memory simultaneously. This becomes particularly significant when dealing with large datasets.
A prime example can be seen when working with log files; imagine needing to process millions of entries efficiently. Rather than loading the entire file, which can be taxing on resources, an iterator reads and processes each line as needed. This behavior is essential in a world where data can blossom into insurmountable proportions without notice.
Python provides built-in support for creating iterators through its iterator protocol. A simple example would be an object that implements the and methods. When you call , the method is executed, returning the iterator object. The method then yields the next value, and once all values have been returned, it raises a exception.
Creating Custom Iterators
The art of creating custom iterators can lead to more elegant and manageable code. Understanding how to craft these iterators opens doors for you to tailor the iteration process according to specific requirements.
To illustrate this, consider you need to iterate over a sequence of numbers but want to filter out even ones. You can create a custom iterator accordingly:
In this example, generates odd numbers up to a specified maximum. When iterated through a loop, it yields odd numbers one by one without holding all values in memory. This is an excellent application of iterators, demonstrating how efficient and straightforward custom implementations can be.
By crafting such specialized iterators, you not only optimize performance but also encapsulate behavior and logic within the iterator class itself, leading to a cleaner separation of concerns.
In summation, generating iterators through tailored classes permits not just memory efficiency but also better maintenance capabilities, resulting in code that is not just functional, but also intuitively understandable.
"The genius of an iterator lies in its ability to provide just what you need, when you need it."
Advanced Iteration Techniques
Diving into advanced iteration techniques in Python shines a light on how efficient code can be constructed while limiting unnecessary computations. These methodologies not only streamline your coding process but also pave the way for more elegant solutions that can handle considerable data sets without a hiccup. Implementing these techniques is not just about making your code work; it’s about making it optimized for performance and clarity, which is paramount in today’s fast-paced development landscape.
Using Generators for Efficient Iteration
Generators represent a significant leap in how we handle iteration in Python. While traditional loops can consume a large amount of memory, generators produce items one by one and only when asked for them. This is like turning on the tap and getting a drink of water only when you need it—no overflow and no excessive waste. Essentially, a generator uses the keyword instead of . This allows the function to maintain its state between calls, enabling it to resume where it left off.
For example, if you’re generating a sequence of numbers, using a generator can look like this:
This snippet will create numbers from 0 to without storing them all in memory, thus saving resources.
Generators are especially handy when processing large datasets or streams of data. They can lead to better performance in applications ranging from data analysis to web scraping.
Implementing List Comprehensions
List comprehensions serve as a powerful technique for creating lists in a concise manner, replacing the traditional loop-based list construction. By combining loops and conditionals, you can generate lists in a single line of code. Beyond simplicity, they significantly enhance performance when compared to conventional methods.
A basic example to create a list of squares might look like this:
Not only does this one-liner accomplish the task efficiently, but it does so in a way that is readable and direct. When iterating through lists, comprehensions can incorporate conditional logic too:
This produces a list of even numbers and utilizes iteration beautifully, all while remaining clean and clean-cut.
The Power of Built-in Functions: Map, Filter, and Reduce
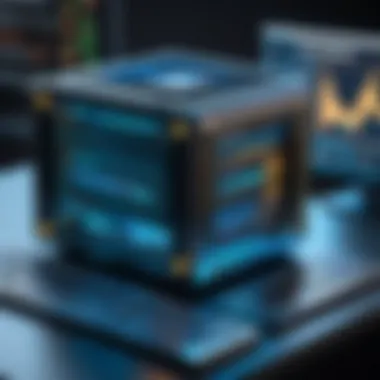
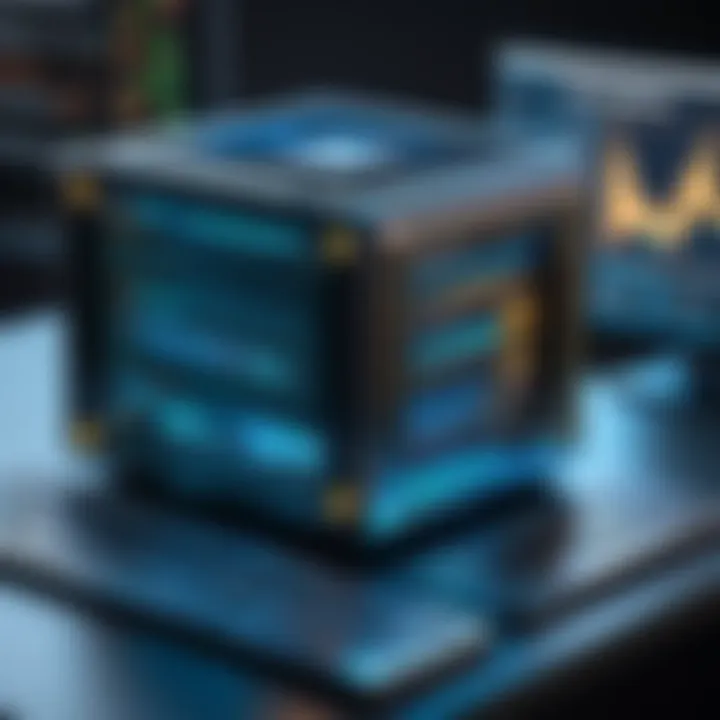
On the topic of advanced iteration techniques, you can't overlook the built-in functions: , , and . Each of these functions encapsulates an approach to processing collections of data effectively.
- Map applies a function to all the items in the input list(s) and returns a new list with the results. This technique is perfect when you need to transform each element of a list. For instance:
- Filter stands out when you want to construct a list by eliminating elements that don’t satisfy a certain condition. You might use it like this:
- Reduce, from the module, performs a rolling computation to sequentially apply a function to the items of an iterable. A typical use case might be summing a list of numbers:
These built-in functions encapsulate powerful paradigms that promote functional programming approaches and improve code efficiency.
In summary, employing advanced iteration techniques such as generators, list comprehensions, and built-in functions can transform the way you approach Python programming. These methods enable cleaner, more efficient code that’s easier to read and reduces the footprint on memory usage. By harnessing these techniques, you will not just be coding; you'll be coding smart.
Performance Considerations
In the realm of programming, particularly when working with iteration in Python, understanding performance considerations is crucial. Being mindful of how code executes can greatly influence both its speed and efficiency. It’s not just about making code work; it's about making it work well, especially when handling larger datasets or more complex algorithms. This section focuses on evaluating time complexity and optimizing iterations for efficiency, both of which are fundamental in ensuring that code runs smoothly in various scenarios.
Evaluating Time Complexity
Time complexity is a concept that quantifies the time taken by an algorithm to run as a function of the length of the input. By evaluating time complexity, programmers can make informed decisions about which algorithms to use based on the expected performance. This is particularly important when iterations are involved, as loops can exponentially increase execution time depending on their structure. In big-O notation, one might encounter terms like O(n), O(1), or O(n^2). In essence:
- O(1) indicates constant time—whether you run the loop zero times or a million times, the time taken remains unchanged.
- O(n) suggests that the execution time will grow linearly with the input size.
- O(n^2) implies that time complexity grows quadratically, which can become unmanageable with large datasets.
By recognizing these complexities, programmers can pinpoint potential bottlenecks early on. It helps in deciding if tweaking the iteration method or altogether changing it could lead to more efficient code. For instance, using a for loop with is often quicker than using a while loop that may involve additional condition checks.
Optimizing Iterations for Efficiency
To truly harness Python's capabilities, programmers need to be aware of straightforward optimization strategies during iterations. Here are some practical approaches:
- Minimize the Work Inside Loops: Reducing the workload within the loop itself can lead to significant performance gains. If certain calculations do not depend on the loop variable, calculate them beforehand.
- Use Built-in Functions: Python’s built-in functions, like and , can perform operations faster than manual loops as they utilize optimized C code under the hood.
- Employ List Comprehensions: Instead of traditional loops, using list comprehensions allows for cleaner code that generally executes faster.
Example of list comprehensions versus a traditional loop
squares = [x2 for x in range(10)]# List comprehension squares = [] for x in range(10):# Traditional loop squares.append(x2)
When looping through this list, one might mistakenly use:
This code will output all elements as expected, but if you're not cautious with your parameters, you might inadvertently use , causing an IndexError by trying to access an index that doesn’t exist.
To avoid this pitfall, it's beneficial to develop a habit of thoroughly checking your range parameters and being mindful of how Python’s zero-indexing works. It is also wise to write unit tests that can help in catching these errors early on in the development cycle.
Unintended Infinite Loops
Another classic mistake in iteration is the occurrence of unintended infinite loops. These happen when the loop's terminating condition is never met. This might not just slow down your program; it could also bring your whole system to a screeching halt in extreme cases. It often arises from a logic error in the loop, especially with a while loop, where the continuation condition is always true.
Consider the following example:
You might think this loop would run 5 times, but should you forget to increment within the loop body, it will run infinitely since remains 0.
To steer clear of such situations, it's crucial to ensure that your loop's conditional expression is appropriately modified during each iteration. Another effective strategy is to set a debug log statement inside your loop to help you track its execution, giving you insight into whether it behaves as expected.
Key Takeaway: Always handle loop conditions carefully and test your code to ensure you avoid infinite loops. A little caution goes a long way in maintaining performance.
By being aware of these common pitfalls and implementing preventative measures, you can greatly enhance the reliability and efficiency of your Python code. As you navigate through iteration techniques, keep these potential errors in mind to fortify your coding arsenal.
Best Practices for Iteration in Python
When it comes to programming, particularly in Python, adopting best practices for iteration is as vital as knowing how to use iteration itself. Following effective standards not only enhances the readability of your code, but also amplifies its maintainability and efficiency. In this section, we shall dissect critical elements that contribute to writing clean and robust iterative structures.
Writing Readable and Maintainable Code
The clarity of your code matters significantly, especially when you're working with loops to iterate through data. Writing readable code facilitates easier collaboration and onboarding for others, or even for yourself in the future.
Here are some considerations:
- Use Descriptive Variable Names: Instead of generic names like or , utilize descriptive names that convey the purpose of the variable. For example, is more informative than , especially in a loop counting users.
- Keep Loops Short and Sweet: Long loops can lead to confusion. Aim to keep loops small, ideally focusing on a single task. If a loop is doing too much, it might be time to split it into multiple smaller loops or functions.
- Comment Wisely: A well-placed comment can illuminate the path through intricate iterations. However, avoid over-commenting; it’s about providing guidance, not cluttering space with unnecessary lines.
- Utilize Comprehensions for Simplicity: Python's list comprehensions are often more readable than traditional for loops. For instance:
This one-liner succinctly conveys the intention to square numbers from 0 to 9. It’s concise and easily understood at first glance.
Testing Iterative Logic
Another critical aspect of best practices revolves around testing your iterative logic. Iteration can often introduce bugs, especially when dealing with complex data manipulations. Here’s how you can effectively test your loops:
- Unit Tests: Use unit tests to validate that your loops are producing the expected outputs. Writing tests can catch those pesky logical errors before they become bigger issues in a project.
- Check Edge Cases: Don’t just test with standard input. Think about edge cases—what happens if you iterate over an empty list? Testing under such conditions will help identify unexpected behaviors in your code.
- Use Assertions: Within loops, feel free to use assertions to validate assumptions about your data at various stages in your iteration. This can act as a safety net for catching issues.
- Print for Debugging: Sometimes, it’s helpful to print intermediate values during iteration to understand what's going on. While not the cleanest method, it can help diagnose where logic may be going astray.
Remember, iteration is powerful, but misusing it can lead to difficult-to-find errors. Pay close attention to the structure and logic of your loops.
In summary, while Python provides the framework for iteration, it is the practices you establish that will enhance your programming craft. Prioritize readability, maintainability, and thorough testing to ensure that your loops serve both your current code needs and future scalability.
Epilogue
In the landscape of programming, iteration stands as one of the foundational pillars that supports the building of complex algorithms and the efficient processing of data. This article has traversed the multiple facets of iteration in Python, offering readers a roadmap for understanding not just the mechanics of loops but their role as vital tools in a programmer's arsenal. The importance of comprehending the nuances of iteration cannot be overstated; it allows one to harness the true power of Python, enabling effective solutions to a vast array of problems.
The Future of Iteration in Python
Looking ahead, the future of iteration in Python appears robust and full of possibilities. As data continues to grow exponentially, so does the necessity for programming languages to evolve. Python's simplicity paired with its powerful iterable constructs is key to maintaining its relevance. With the increasing adoption of machine learning and data science, the demand for efficient iteration methods will likely escalate. We can expect enhancements to existing frameworks and possibly the introduction of new libraries aimed at optimizing iterative processes. As programmers, we should remain adaptable, exploring innovations that can streamline our code and improve performance.
Encouragement for Continuous Learning
To the aspiring and experienced programmers alike, continuous learning is crucial in this fast-paced technological era. The world of programming is not static; it evolves with new paradigms, libraries, and best practices regularly surfacing. Embrace the learning journey by engaging with communities on platforms such as Reddit or contributing to discussions on sites like Stack Overflow. Attending workshops and participating in coding challenges can sharpen your skills and keep you abreast of the latest trends. Don't shy away from experimenting with your insights from this article; the only way to solidify your understanding of iteration is through practice and application.