Mastering the JavaScript Debugger Console
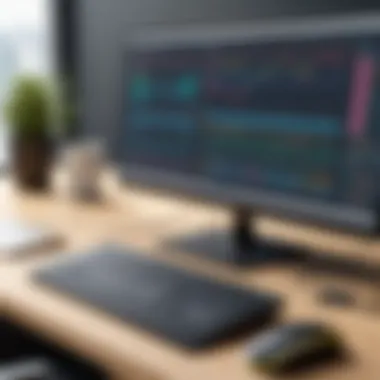
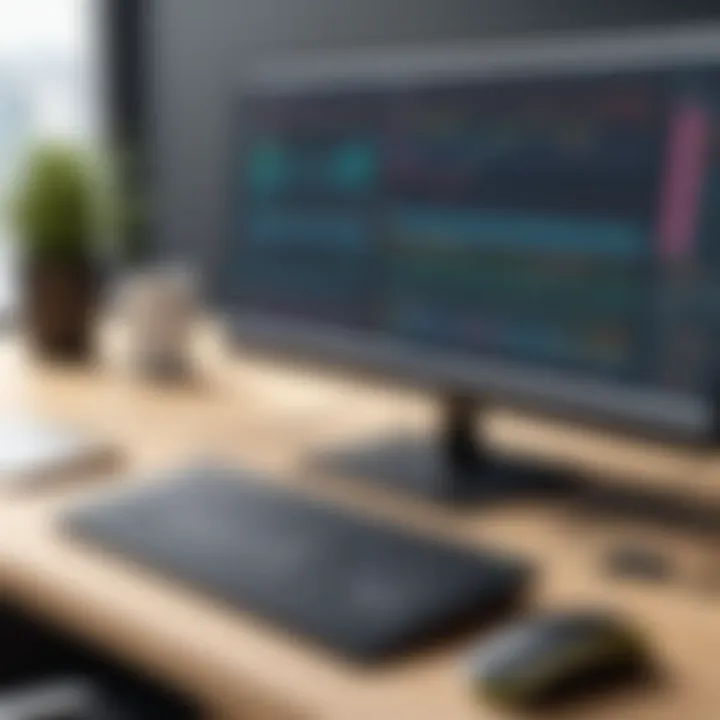
Intro
In software development, debugging is a crucial process. The ability to identify and resolve errors can drastically enhance the performance of applications. The JavaScript debugger console plays a key role in this process. It offers features that allow developers to examine their code, understand its behavior, and ultimately fix issues that may arise.
This article aims to explore the JavaScript debugger console in detail. We will dissect its capabilities, examine how to use it effectively, and discuss best practices for debugging. This comprehensive guide will cater to both novices and experienced programmers seeking to refine their skill set.
Through this exploration, readers will discover how to maximize their efficiency in coding. Understanding the debugger console not only simplifies the debugging process but also fosters a deeper comprehension of JavaScript itself, enabling programmers to produce more robust applications. Our goal is to provide insightful information that can enhance coding proficiency and problem-solving capabilities.
Each section of this article discusses different elements of the JavaScript debugger console, including its features, common usage patterns, and strategies that can be employed during debugging sessions. Let's begin our journey into the enriching world of the JavaScript debugger console.
Foreword to the JavaScript Debugger Console
In the realm of web development, the JavaScript Debugger Console holds paramount importance. It acts as a vital tool that assists developers in diagnosing and resolving errors within their code. This section elucidates its significance, enabling programmers to appreciate its role in effective debugging and enhancing programming proficiency.
Defining the JavaScript Debugger Console
The JavaScript Debugger Console is an interface usually integrated into web browsers that enables developers to execute JavaScript code snippets, view output, and diagnose issues in real-time. It serves as a space where commands can be input, enabling immediate feedback and insights on the current state of the web application.
Notably, it offers functionalities like logging messages, inspecting objects, and interacting with the Document Object Model (DOM). The console's capabilities allow for a more profound understanding of how the code behaves, especially during execution. This immediate access to the application’s state empowers developers to amend code more efficiently. Without the console, debugging becomes a much more labor-intensive task.
Historical Context of Debugging in JavaScript
Debugging in JavaScript has evolved significantly since the language's inception in the mid-1990s. Initially, developers had limited tools at their disposal. They often relied on alert boxes or cumbersome print statements to troubleshoot code, which could be inefficient and disruptive to the user experience.
Over time, as JavaScript grew in popularity and complexity, the need for more sophisticated debugging tools became evident. Browser vendors began to listen to developer feedback, leading to the birth of the console as part of the developer tools suite.
Today, tools such as Chrome DevTools, Firefox Developer Edition, and Edge Developer Tools have integrated advanced console features, allowing for more comprehensive debugging sessions. These developments have fundamentally altered how developers interact with and refine their code, moving from ad-hoc solutions to a structured debugging process.
"The evolution of the JavaScript Debugger Console is a testament to the growth in web development practices and the demand for better development tools."
In summary, the JavaScript Debugger Console not only aids in quickly identifying problems within code but also provides context for how JavaScript operates within the browser environment. As technology progresses, understanding this console remains crucial for both aspiring and seasoned developers.
Key Features of the JavaScript Debugger Console
The JavaScript Debugger Console is not just a simple interface but a powerful tool that plays a significant role in the development and debugging process. Understanding its key features allows developers to harness its full potential. This section will delve into essential elements such as basic console commands, advanced debugging techniques, and integration with browser developer tools.
Basic Console Commands
The JavaScript Debugger Console offers a set of basic commands that form the foundation of effective debugging. These commands allow developers to interact with their code in real-time. Here are some fundamental commands:
- : This is perhaps the most commonly used function. It prints messages to the console, helping developers visualize variable states and track code execution.
- : This command outputs warning messages. It is useful for highlighting potential issues without stopping script execution.
- : This is critical for error reporting. It displays error messages in red, making them easily identifiable.
- : This function presents array data or objects in a tabular format, enhancing data readability.
Using these commands facilitates a streamlined debugging process by providing immediate feedback on code execution. Each command serves a specific purpose and contributes to understanding code behavior clearly.
Advanced Debugging Techniques
While basic commands provide essential functionality, advanced debugging techniques unlock deeper insights into code execution. These techniques allow developers to tackle intricate problems effectively:
- Using breakpoints: Breakpoints pause code execution at specified lines. This enables developers to inspect variable states and control flow at critical moments.
- Watch expressions: Developers can set watch expressions for particular variables. The debugger will notify changes in these variables as the code runs, simplifying the tracking of state changes.
- Call stack inspection: This technique allows developers to view the current state of the function call stack. It provides context on how the application reached a particular state, aiding in understanding complex behaviors.
Implementing these advanced techniques reduces debugging time significantly. They equip developers to locate bugs more efficiently and understand complex interactions within their code.
Integration with Browser Developer Tools
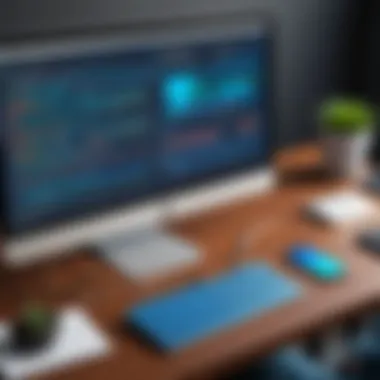
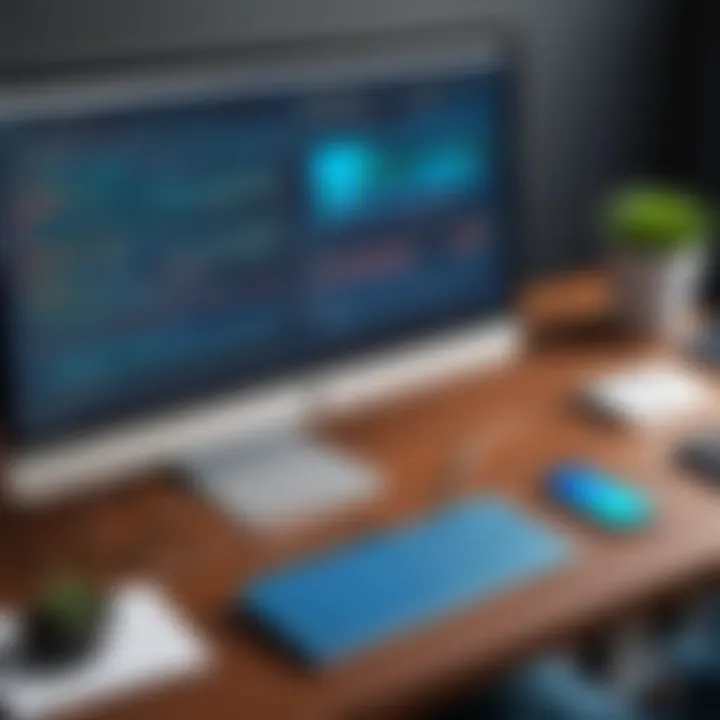
The JavaScript Debugger Console is integrated within the broader framework of browser developer tools. This integration enriches the debugging experience by combining various functionalities:
- Element inspection: Developers can directly inspect HTML and CSS elements alongside JavaScript. This ensures an all-in-one view of how front-end and back-end code interact.
- Network request monitoring: The console allows tracking of network requests and their responses. Understanding the data flow between server and client is crucial for debugging issues related to data retrieval.
- Performance profiling: Browser dev tools provide options for performance profiling, allowing developers to benchmark their code. This feature helps identify performance bottlenecks, ensuring the application runs smoothly.
This integration means developers can work efficiently without switching tools. By using the browser's developer tools in conjunction with the JavaScript debugger console, they can create a more holistic debugging environment that enhances productivity.
Using the Console for Effective Debugging
Using the JavaScript debugger console is vital for developers aiming to create bug-free and efficient code. This tool allows developers to interact with JavaScript environments directly within their browsers. Through the console, they can execute commands, inspect variables, and identify issues in real time. The ability to diagnose problems quickly enhances productivity and leads to faster learning of coding concepts.
When using the console effectively, programmers can pinpoint where their code is breaking or misbehaving, saving time in testing and development. Mastering the console's capabilities can also lead to a deeper understanding of JavaScript itself, making debugging a valuable skill in a programmer's toolkit.
Setting Breakpoints
Setting breakpoints is a fundamental technique in debugging with the console. A breakpoint allows developers to pause the execution of their code at a specific line. By doing this, they can examine the current state of the application, including variable values and execution context.
To set a breakpoint, you typically click on the line number in the Sources panel of your browser's developer tools. This action halts code execution when that line is reached, enabling the developer to gather insights on how the application behaves at that moment. Here’s why setting breakpoints is beneficial:
- It allows for focused analysis of critical points in code.
- Breakpoints aid in understanding control flow, especially in complex functions.
- It provides a precise moment to inspect the stack trace.
Stepping Through Code Execution
After setting breakpoints, the next step is often stepping through code execution. This process involves moving through the code line by line, which helps to understand the sequence and flow of execution.
Most modern browsers provide controls for stepping into functions, stepping over lines of code, or stepping out of a current function. Using these controls lets developers:
- Gain clarity on how functions are invoked and how parameters are passed.
- Identify unexpected results or behavior promptly.
- Trace the state of the application as it evolves during execution.
The combination of breakpoints and stepping through code creates a powerful debugging workflow that can significantly reduce the time spent finding bugs.
Inspecting Variables and Objects
Now that the code execution is paused, inspecting variables and objects is crucial for understanding the application state. The console enables developers to view the current values of variables at the breakpoint and evaluate the structure of objects.
Here are some techniques for effective inspection:
- Use the console.log() function to output variable values and track changes in real time.
- Leverage the built-in inspection capabilities, such as viewing object properties in a hierarchical format.
- Evaluate expressions directly in the console to check the outputs and reactions of code snippets.
By understanding the state of variables and objects, developers can make informed decisions on how to fix issues or optimize their code further.
"Effective debugging simplifies the complex, enhancing code reliability and user experience."
Common Debugging Scenarios
Understanding common debugging scenarios is crucial for any developer working with JavaScript. These scenarios often arise during development and can lead to various coding challenges. Knowing how to identify and resolve these issues directly affects code quality and efficiency. Moreover, tackling these scenarios enhances problem-solving skills and contributes to a developer's overall proficiency.
Debugging Syntax Errors
Syntax errors are one of the most elementary yet frequent problems encountered in JavaScript. These errors occur when the JavaScript engine cannot interpret the code due to incorrect syntax. Common examples include missing brackets, misplaced commas, or the use of invalid identifiers. Such errors lead to the immediate failure of script execution, making it vital to resolve them swiftly.
To detect syntax errors effectively, utilize the debugger console. The console will often point out the line number where the issue resides. Here is an example of a syntax error:
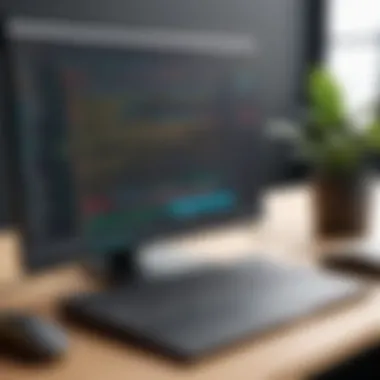
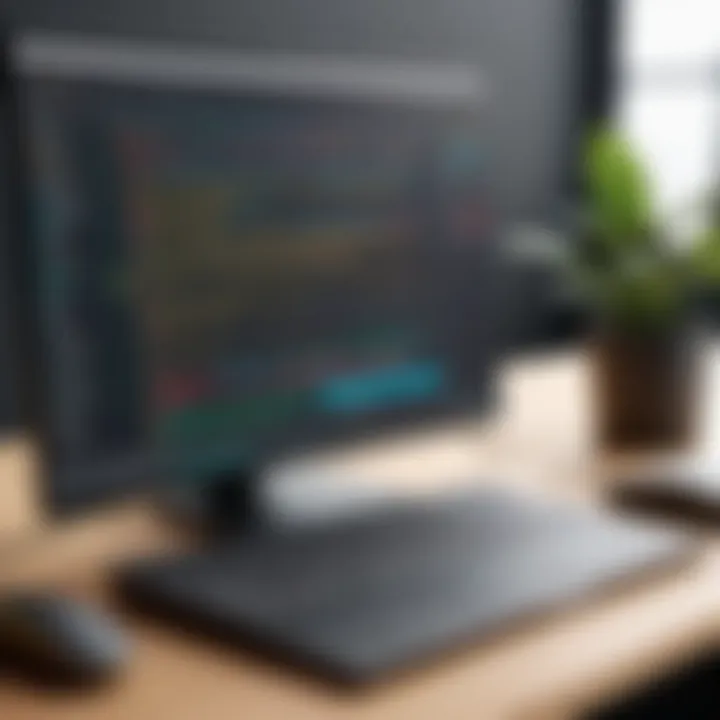
In this example, the missing semicolon will raise a syntax error, which the console highlights. Developers should ensure careful review of their code structure and be mindful of punctuation and syntax rules.
Resolving Logical Errors
Logical errors can be more challenging to identify than syntax errors, as the code is syntactically correct yet does not produce the expected output. These errors typically stem from flawed algorithms, incorrect assumptions, or oversight in the logic flow.
For instance, consider the following code snippet:
In this scenario, the developer inadvertently used subtraction instead of addition. To debug logical errors, employ techniques such as console logging intermediate values, setting breakpoints, and analyzing the flow of execution. This methodical approach allows for tracing the logic and pinpointing where the deviation from expected behavior occurs.
Handling Asynchronous Code
In modern JavaScript, asynchronous code is prevalent, especially with the use of Promises and asynchronous functions. Handling asynchronous behavior can lead to unique challenges, particularly when code execution order does not align with logical expectations. Common pitfalls include race conditions or failing to manage asynchronous callbacks properly.
Consider the following example:
Here, executes asynchronously, thus will be when logged immediately after calling the function. To manage asynchronous operations effectively, developers should harness tools like and utilize promises correctly. Utilizing the console for logging the state of variables before and after asynchronous execution helps clarify the flow of data.
By mastering these common debugging scenarios, developers can avoid many pitfalls that detract from productivity. Each type of error requires distinct approaches and methods to address effectively. Beyond merely fixing errors, understanding these challenges contributes to building robust code and enhances programming skills overall.
Best Practices for Using the Console
Using the JavaScript debugger console effectively can have a significant impact on debugging efficiency. Developers at every skill level can benefit from following best practices that streamline their debugging process and enhance code readability. These practices not only improve the organization of console output but also provide clarity when communicating errors and warnings. Adopting these strategies can lead to quicker problem resolution, reducing the time spent tracking down issues.
Organizing Console Messages
Organizing console messages is essential for effective debugging. A cluttered console can overwhelm any developer, making it difficult to identify and address issues. Here are some ways to organize messages:
- Categories: Use categories for different types of messages. For example, use for informational messages, for warnings, and for errors. This categorization allows you to quickly filter messages by importance.
- Labels: Adding labels to messages can provide context on what part of the code the message corresponds to. For instance, gives immediate insight into what the following output represents.
- Group Messages: Utilize and to create logical groups of related console messages. This method minimizes clutter and makes it easier to read through long outputs. By following these strategies, developers can maintain a clearer overview of their console outputs, improving focus during debugging sessions.
Using Console Warnings and Errors Wisely
Console warnings and errors are tools that aid in catching issues before they escalate. Using them wisely can serve multiple purposes:
- Prioritization: By correctly utilizing and , developers can prioritize issues based on severity. This enables devs to tackle critical issues first while keeping track of less pressing warnings.
- Clarity: A well-structured error message can pinpoint the line and nature of the issue within the code. For example, can efficiently lead to the root cause of the problem.
- Consistency: Maintain a consistent approach when using warnings and errors. For instance, if some resources are deprecated, regularly provide warnings. This consistent approach aids in maintaining a clean codebase and helps developers adapt to potential future changes.
Documentation and Logging Techniques
Clear documentation and robust logging techniques enhance the utility of the JavaScript console:
- Commenting Code: Use comments in combination with logging. A good practice is to describe complex logic while logging its output, creating clarity when reviewing later. For example:
- Structured Logging: Consider structured logging formats such as JSON. This makes it easier to parse console messages when the project scales.
- External Logging: For critical applications, consider integrating with external logging solutions like Loggly or Sentry. These services can capture logs from your application and provide insights into issues over time.
Using such techniques can help distribute knowledge about the code's behavior among teammates and assist in future debugging efforts.
"Good debugging practice is not just about fixing issues; it is also about understanding the nature of the code itself."
Limitations of the JavaScript Debugger Console
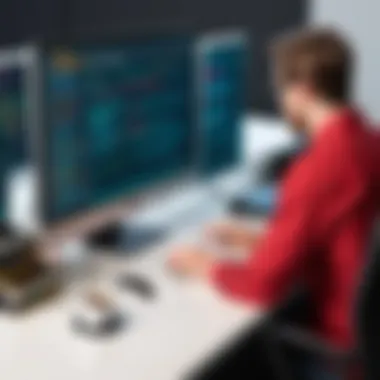
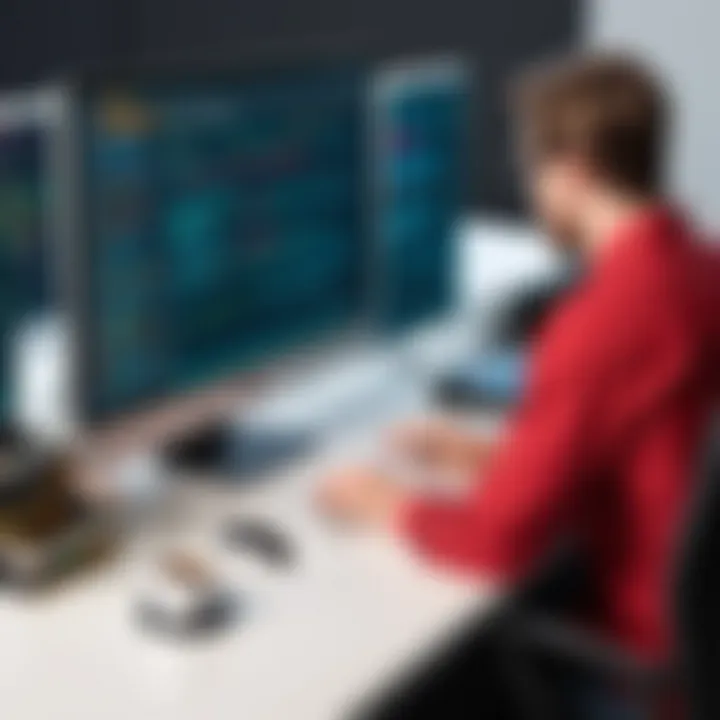
The JavaScript Debugger Console, while an invaluable tool for developers, does have its limitations. Understanding these constraints is essential for maximizing its potential and using complementary tools where necessary. This section discusses the specific challenges relating to scalability and performance that developers may encounter when using the console.
Scalability Issues
One primary limitation of the JavaScript Debugger Console is its scalability. As applications grow in complexity, the number of logged messages increases significantly. This can lead to a cluttered output that is challenging to navigate. In larger projects, developers may find it difficult to isolate relevant console messages from an overwhelming amount of information. This is particularly problematic during debugging sessions where important error messages may get lost in a sea of standard logs.
Additionally, many developers work in teams where multiple individuals may be logging messages simultaneously. Without a structured approach to logging, this could hinder efficiency and lead to a disorganized debugging process. It is beneficial to implement strategies for managing console output effectively, such as filtering messages based on severity or using unique prefixes for different team members or modules.
Performance Impacts
The performance of applications can also be negatively affected by excessive use of the JavaScript Debugger Console. Frequent logging, especially in loops or during high-frequency events, can introduce significant delays. This can create scenarios where the performance of the application may degrade, leading to incorrect assumptions regarding the code's functionality.
Moreover, the console itself can struggle to keep up with rapid logging in real-time applications. This can result in performance hits and might even lead to application crashes in extreme cases. Developers need to be mindful of how often they call logging functions in their code.
To maintain optimal performance, use logging judiciously, especially in production environments where efficiency is crucial.
Lastly, while modern browsers provide powerful debuggers, relying solely on the JavaScript console for extensive debugging practices may not yield the best results. Developers should consider utilizing other debugging tools and techniques to supplement the JavaScript Debugger Console's limitations.
Future of JavaScript Debugging Tools
The future of JavaScript debugging tools is a critical subject in software development. As applications become more complex, the need for efficient and effective debugging solutions increases. Developers seek tools that not only help identify issues more swiftly but also provide insights into code behavior, contributing to better software quality. In this section, we'll explore key emerging technologies that could reshape debugging practices and the integration of artificial intelligence, which promises to enhance productivity in debugging tasks.
Emerging Technologies in Debugging
New technologies are continually evolving within the realm of debugging. These advancements can significantly impact how developers approach issues in their code. A few notable emerging technologies include:
- Automated Code Review Tools: Tools like SonarQube and CodeClimate analyze code for potential errors and suggest fixes, thus streamlining the debugging process.
- Real-time Collaboration Tools: Platforms such as Visual Studio Code Live Share allow multiple developers to work on the same code simultaneously. This real-time interaction can facilitate quicker problem-solving.
- Visual Debugging Interfaces: Enhanced visual debugging tools, like Chrome DevTools, provide an intuitive graphical interface to track the flow of execution. This can make it easier for developers to spot issues without delving deeply into the code.
As these technologies develop, they aim to make debugging more accessible and efficient. The incorporation of visual cues and automated suggestions nurtures an environment where both beginners and experienced developers can manage debugging more competently.
Integrating AI into Debugging Processes
Artificial intelligence is making substantial inroads into the debugging landscape. Its integration offers several transformative benefits:
- Predictive Analysis: AI-driven tools can analyze historical bug data and predict where future issues are likely to occur. This proactive approach allows developers to focus on potential problem areas before they escalate into significant bugs.
- Natural Language Processing (NLP): AI tools utilizing NLP can help in interpreting developer comments and documentation. This enables automatic extraction of vital context, which may illuminate the cause of certain bugs.
- Automated Remediation: AI can aid in suggesting code fixes based on common patterns it identifies in bugs. Solutions like Facebook's Aroma can recommend code snippets that resolve errors detected during a review.
Integrating these AI capabilities into debugging processes can optimize workflow, reduce the burden on developers, and enhance accuracy in identifying issues. This ultimately leads to faster resolution of bugs and a more streamlined development process.
"The advancements in debugging tools represent not just enhancements in capability but a transformation in how developers interact with their code."
End
The conclusion of this article encapsulates the crucial aspects of the JavaScript debugger console, tying together the various discussions on its functionality, usage, and implications for developers. This tool is not merely an accessory; it's a foundational component of the programming landscape that significantly enhances a developer's capability to identify and address issues in code. By fostering a robust understanding of the console, programmers can advance their debugging skills to a professional level.
Recap of Key Points
Throughout this article, we explored several essential elements:
- Definition and Historical Context: We started with understanding what the JavaScript debugger console is and its evolution over the years, which informs its current functionalities.
- Key Features and Commands: The discussion on basic and advanced commands illustrated how the console operates as a powerful tool in both simple and complex debugging scenarios.
- Effective Debugging Practices: We delved into practical techniques such as setting breakpoints and inspecting variables, showcasing how these features lead to more efficient problem-solving.
- Common Scenarios and Limitations: Highlighting common debugging scenarios provided context on when and how to apply console features effectively, while recognizing its limitations helped in setting realistic expectations for developers.
- Future Directions in Debugging Tools: Finally, the exploration of emerging technologies and the integration of AI served to highlight the ever-evolving nature of debugging tools.
"A strong command of debugging tools like the JavaScript console is what separates proficient developers from novices."
Encouraging Continued Learning and Adaptation
The landscape of software development is in constant flux. As programming languages evolve and new technologies arise, the methods for debugging also change. Aspiring and established developers alike should see the value in continuous learning.
- Stay Updated: Following development communities on platforms like Reddit and participating in forums can provide insights into recent changes and best practices.
- Practice Regularly: Regular application of debugging techniques in personal or professional projects solidifies understanding and keeps skills sharp.
- Explore Resources: Engaging with resources such as documentation, tutorials, and tech blogs nurtures a proactive approach to learning.
Adapting to new tools and methods is not only beneficial; it is necessary. By embracing a mindset of continuous improvement, developers can enhance their capabilities and maintain a competitive edge in the industry.