Mastering JavaScript Execution: Techniques and Tools

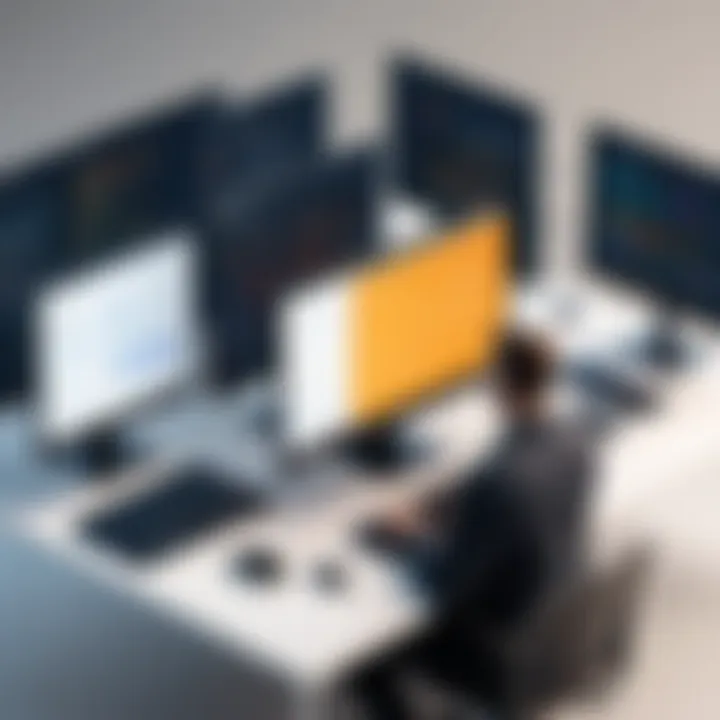
Intro
JavaScript stands as a cornerstone of modern web development, permeating various aspects of creating interactive applications. To successfully harness its full potential involves understanding how to run JavaScript in different contexts and use suitable tools. This guide encompasses not just the basic implementations but delves deeper into the nuances of execution environments, debugging strategies, and advanced techniques.
Coding Challenges
Engaging in coding challenges offers a practical way to sharpen JavaScript skills. These challenges can vary from simple exercises to complex algorithm puzzles, giving both novice and expert programmers an avenue for growth.
Weekly Coding Challenges
Participating in weekly coding challenges can enhance problem-solving abilities significantly. Websites like Codewars and HackerRank present tailored challenges in varying difficulty levels. Regular practice will build one's confidence and improve coding fluency in JavaScript.
Problem Solutions and Explanations
Each coding challenge often includes an explanation of the solution. Such insights are crucial for understanding best practices and refining coding techniques. Examine example solutions that not only work but also follow industry-standard conventions. Learning from diverse approaches can solidify concepts and encourage original thinking.
Tips and Strategies for Coding Challenges
To efficiently tackle these challenges, consider the following strategies:
- Understand the Problem: Spend time comprehending the requirements before diving in.
- Break Down Solutions: Decompose large problems into smaller, manageable tasks.
- Test as You Go: Regular testing can isolate issues early in the process.
Community Participation Highlights
Engagement with the programming community can occasionally shift perspectives. Platforms such as Reddit forums or Facebook groups can provide insights or collaborative encouragement. Community-driven contributions can often illuminate different paths towards a solution, addressing common pitfalls along the journey.
Technology Trends
Keeping abreast of technology trends related to JavaScript also serves in making informed coding decisions.
Latest Technological Innovations
Technological innovations such as frameworks and libraries are constantly emerging. Understanding tools like React and Node.js can be vital as these change paradigms in JavaScript development and server-side rendering strategies.
Emerging Technologies to Watch
Stay informed about the emerging technologies in the ecosystem. For example, advancements in WebAssembly and progressive web apps can transform performance metrics in web applications and JavaScript's role therein.
Expert Opinions and Analysis
Listening to expert opinions through platforms such astacles descriptions provide insights into effective coding strategies and best practices which enhance development efficiency. Analyze changes by reading industry updates or authoritative sources on critical shifts.
Coding Resources
Diving deep into coding resources provides structural support to your developmental journey.
Programming Language Guides
For those new to JavaScript, comprehensive guides serve as essential bellwether resources. Documentation accessible on websites like Mozilla Developer Network provides clear paths for knowledge accumulation.
Tools and Software Reviews
Choosing the right tools gears smoother workflow experiences. Software like Visual Studio Code or ESLint facilitates effective coding practices with in-built error checking features.
Tutorials and How-To Articles
In addition, curated tutorials and a rich pool of how-to articles supplements skills. Follow BlockChain websites for new JavaScript functionalities, or engage with popular blogs.
Online Learning Platforms Comparison
There is considerable divergence in online learning platforms. Comparing resources such as freeCodeCamp, Codecademy, and Udemy can spotlight strengths and weaknesses of various materials.
Computer Science Concepts
Lastly, reinforcing fundamental computer science concepts aids in mastering JavaScript beyond mere syntax.
Algorithms and Data Structures Primers
Understanding algorithms and data structures can resonate effectively with coding practices within JavaScript. Prepare to implement standard sorting algorithms such as quicksort or understand data structures like arrays, linked lists and trees which are essential knowledge.
Understanding algorithms and data structures can provide insight into JavaScript's more intricate functionalities.
Artificial Intelligence and Machine Learning Basics
Additionally, familiarity with artificial intelligence and machine learning can advance your capabilities in JavaScript too as frameworks like TensorFlow.js enable AI functionalities in web applications.
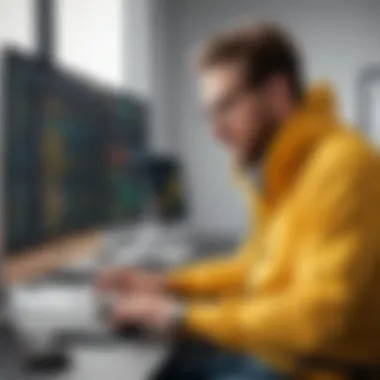
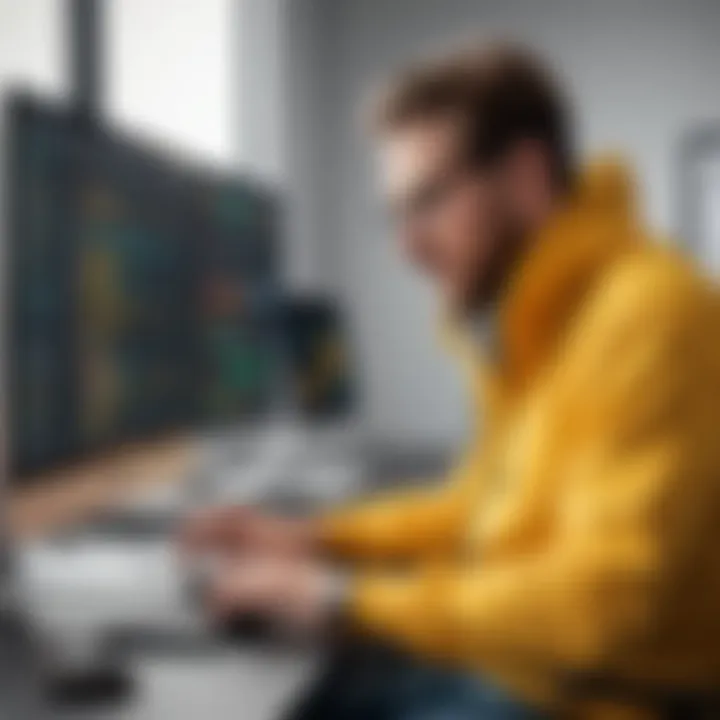
Networking and Security Fundamentals
Knowledge about networking and security fundamentals could also play a critical role in JavaScript applications. Maintain best practices for security testing as you develop programs.
Quantum Computing and Future Technologies
Even concepts concerning quantum computing slowly find relevance in mainstream computer science discussions. Keeping an eye on future technologies allows hopeful trends to integrate smoothly into one’s coding framework.
With this foundational understanding, readers should feel empowered to embark on their Journey. Learning to run JavaScript effectively requires continual growth. Agora, equipped with the knowledge of resources, strategies and technology trends, programmers can develop their unique coding hacks and excel in a landscape that constantly evolves.
Understanding JavaScript Execution
JavaScript is a powerful programming language that plays a central role in web development. Understanding how JavaScript executes is crucial for both aspiring and seasoned developers. It shapes how the code runs and interacts with web pages. Knowledge of execution mechanisms can improve code efficiency and performance. Moreover, knowing how JavaScript communicates with other components aids in building rich web applications.
What is JavaScript?
JavaScript is a high-level, dynamic programming language. It is primarily known for enabling interactive elements on web pages. Beyond this, it supports various programming styles, including functional and imperative. JavaScript runs in the browser, but it has also extended its reach to server-side environments with Node.js.
Its versatility makes JavaScript vital for modern web development. Applications range from front-end frameworks to server management. Understanding its fundamental role allows programmers to build dynamic, responsive applications.
The Role of the JavaScript Engine
Every web browser comes equipped with a JavaScript engine that interprets and runs JavaScript code. Each engine has design principles that impact performance, speed, and capabilities. Popular engines like Google’s V8, Mozilla’s SpiderMonkey, and Microsoft’s Chakra excel at these tasks.
Here are some key functions of a JavaScript engine:
- Parsing: The engine compiles the source code into machine code. This process turns the written code into a form the machine can execute.
- Execution: After parsing, the machine code is executed. It runs commands and interacts with the Document Object Model (DOM) of the web page.
- Garbage Collection: Engines manage memory practically. They identify and retrieve objects that are no longer in use, ensuring that memory is available for new processes.
Understanding the JavaScript engine's role is vital for optimizing performance and debugging the code effectively. As you learn about these engines, you can tailor your coding practices to utilize their capabilities fully.
Executing JavaScript in Browsers
Executing JavaScript within browsers is foundational for web development. It allows developers to create interactive web pages, making them dynamic and responsive to user actions. Browsers serve as environments where JavaScript code runs efficiently. Understanding this execution process helps developers leverage JavaScript’s full potential in delivering superior user experiences.
When JavaScript is executed in browsers, it interacts tightly with HTML and CSS. This integration is crucial because it ensures that the user interface responds seamlessly to both input events and data binding. By managing these elements effectively, JavaScript enhances the speed and functionality of websites. Therefore, recognizing how to execute JavaScript correctly within browsers is pivotal for any developer keen on crafting high-quality web applications.
Using the Browser Console
The browser console is a powerful tool that provides an interface to run and debug JavaScript in real time. It offers immediate feedback when executing code snippets, making it ideal for testing functions or debugging issues. Accessible in most modern browsers such as Google Chrome and Firefox, the console improves coding efficiency because it allows developers to see output, analyze errors, and quickly iterate on their code.
Using the console, one can easily log messages, inspect variables, and run scripts directly without needing a separate development environment. However, it's important to remember that the console is designed primarily for development use. Misuse or reliance on it for production code could introduce security vulnerabilities or performance issues.
Embedding JavaScript in HTML
Embedding JavaScript within HTML can be carried out through two primary methods: inline scripting and external JavaScript files. Each technique carries its own strengths and considerations. Selecting the most suitable approach is essential for effective coding practices.
Inline Scripting
Inline scripting refers to placing JavaScript code directly within an HTML file's tags. This approach allows for quick coding and easy testing of small snippets. Its greatest advantage is the immediate linkage between HTML elements and the JavaScript code, making it easy for beginners to grasp dynamic interactions.
However, this method also leads to downsides. It's not practical for larger projects or scalable applications because it can bloat the HTML file. Moreover, this tight coupling of code can reduce maintainability. Fine-tuning an operation generally requires modifications spread across various positions in HTML, which could become cumbersome as a project grows.
External JavaScript Files
Using external JavaScript files involves placing code in separate files and linking to them in HTML. This practice separates content from functionality and contributes significantly to streamlined maintenance and organized code structure.
A key characteristic of external files is reusability. A single JavaScript file can support multiple web pages, saving loading times and making updates easier. You only need to change one file, and all pages pulling from it will update instantly.
Nevertheless, relying on external scripts may introduce latency since the browser must load an additional file. It’s critical to note that while maintaining high coherence and organization in code, external file management requires careful structuring to keep track of dependencies efficiently.
Working with JavaScript Files
Working with JavaScript files extends beyond merely executing scripts; it involves understanding how these files interact within projects. A logical file structure and naming conventions, combined with version control, can enhance coding efficiency. Proper setup minimizes conflicts, and better methods amplify the collaborative nature of coding.
Crafting, optimizing, and utilizing JavaScript files proficiently not only improves workflow but also boosts application performance. Balancing these dynamics ultimately leads to smoother and more stable user experiences on the web.
Node.
js and Server-Side JavaScript
Node.js plays a significant role in the modern JavaScript landscape due to its capability to run JavaScript on the server side. Before Node.js emerged, JavaScript was confined to the browser; it was primarily used for client-side scripting. The introduction of Node.js has revolutionized how developers approach web applications, making it possible to use JavaScript throughout the entire application stack.
Incorporating Node.js in development offers numerous benefits, which include non-blocking I/O operations for enhanced performance, an extensive package ecosystem via npm, and the ability to handle many simultaneous connections with increased efficiency. Such features are particularly valuable for building scalable applications and services that require fast input and processing capabilities.
What is Node.
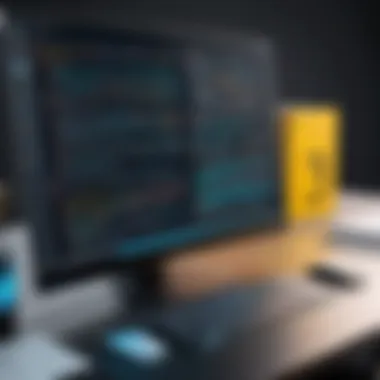
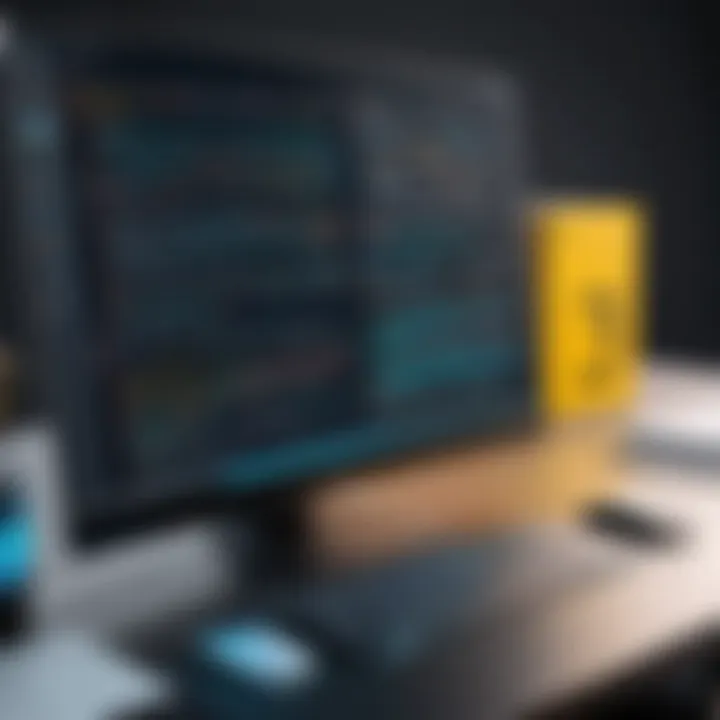
js?
Node.js is an open-source runtime environment that allows developers to execute JavaScript code outside a web browser. It utilizes the V8 JavaScript engine, which was originally developed for Google Chrome, to provide a fast and efficient runtime for executing JavaScript. Node.js is designed to be event-driven and asynchronous, traits that make it a suitable choice for data-intensive, real-time applications.
What differentiates Node.js from traditional server-side technologies is its single-threaded, non-blocking architecture. This model enables developers to create applications that can handle extensive network operations seamlessly, without being held up by input/output processes. Hence, this can lead to responsive applications which are capable of scaling out with minimal resource consumption.
Setting Up Node.
js Environment
Setting up a Node.js environment is straightforward. Below are the general steps to follow:
- Download Node.js: Visit the official website at nodejs.org and select the version that matches your operating system. Node.js provides both Long Term Support (LTS) and Current versions, the former is usually more stable.
- Install Node.js: Run the installation file. This will also install npm (Node Package Manager), which is integral to program dependencies.
- Verify Installation: Open a terminal or command prompt. Type and to verify that both Node.js and npm are installed correctly. You should see the version number in response.
- IDE or Code Editor: It’s advisable to use a suitable code editor if you are working with Node.js projects. Good options include Visual Studio Code, Atom, or Sublime Text as they provide integrated tools to simplify coding, along with useful extensions for Node.js.
Running JavaScript Files with Node.
js
To run JavaScript code with Node.js, follow these steps:
- Create a JavaScript file: Open your code editor and create a file named .
- Write your JavaScript code: In , you can write simple code, for example:
- Run the JavaScript file: Open a terminal or command prompt in the directory that contains . Execute the command:You should see the output in the terminal.
- Enter interactive mode: You can also start the Node.js REPL (Read-Eval-Print Loop) by just typing in your terminal, allowing you to test small chunks of JavaScript code interactively.
Node.js enables you to harness the power of JavaScript beyond browsers, enhancing the versatility of your applications.
Setting up and utilizing Node.js broadens programming capabilities significantly. This environment presents an excellent opportunity for developers aiming to create fast, scalable network applications while maintaining an agile approach to coding. Additionally, managing packages with npm provides access to an expansive library of community-supported modules which can significantly reduce development time.
JavaScript Development Tools
JavaScript development tools play crucial role in efficiency and productivity of developers. By leveraging these tools, programmers can optimize their coding experience, debug issues easily, and streamline workflows. Executing JavaScript efficiently requires awareness and skill in selecting, and using appropriate tools. These tools can be broadly categorized into code editors, integrated development environments (IDEs), and debugging facilities.
Code Editors and IDEs
Code editors and IDEs are among the first stops for JavaScript developers. A code editor provides simple functionalities allowing users to write, edit, and quickly access their code. Popular code editors such as Visual Studio Code and Sublime Text have features like syntax highlighting, snippets, and extensions that can enhance coding speed.
On the other hand, IDEs offer a more comprehensive solution. Tools like WebStorm combine powerful features including code completion, built-in debuggers, and version control. IDEs usually offer greater functionality for large-scale projects. Although setup may be more complex, advantages like integrated tools can outweigh these initial challenges.
Considerations: Depending on project size, a developer might choose between simple editors or complex IDEs. Assess the depth of the project, team collaboration capabilities, and personal comfort levels.
Debugging JavaScript
Debugging JavaScript is essential for maintaining and elevating code quality. Detecting and resolving errors contributes significantly to software performance. There are various methods for debugging, among which using debugging tools is notably popular.
Using Debugging Tools
Utilizing debugging tools allows developers to track down bugs efficiently while running JavaScript code within different environments. Tools like Chrome Developer Tools simplify this process with various features such as breakpoints, call stack tracking, and executing code step-by-step.
The key characteristic of using debugging tools is interactivity. It supports developers by highlighting variable values and application performance metrics dynamically. As for unique features, the ability to inspect and modify the Document Object Model (DOM) in real-time is particularly helpful for Front-End development.
However, the initial learning curve associated with this tool cannot be ignored. Navigating through various functionalities may confuse new users, potentially elongating debugging time on arrival.
Best Practices for Running JavaScript
Best practices for running JavaScript are essential for developers who want to achieve efficiency, maintainability, and scalability in their code. Applying these practices ensures that your JavaScript applications run smoothly, improve performance, and are easier to debug. Adopting such practices helps minimize common errors and promote a clean coding environment.
Minifying JavaScript Files
Minifying JavaScript files means stripping out unnecessary characters from the source code, such as white spaces, line breaks, and comments. This process greatly reduces the file size, which in turn decreases load times on web pages. For web applications, faster performance is critical; users tend to abandon sites that take too long to load. Therefore, overly logn loading times can dramatically affect user experience and engagement.
Here are some important points about minifying JavaScript files:
- Improved Load Times: Smaller file sizes directly translate to quicker load times.
- Reduced Bandwidth Usage: Less data transferred helps save users' bandwidth costs.
- Enhanced Performance: Speed improvements lead to better overall application performance.
To minify a JavaScript file, consider using tools like UglifyJS or Terser. These tools automatically perform minification while also maintaining functionality. It is vital, however, to keep an unminified version available for development and debugging purposes.
Using Modern JavaScript Features
Utilizing modern JavaScript features can significantly enhance both the approach you take to coding and your code's performance. Modern features help make code cleaner, reduce errors, and improve readability, making it easier for development teams.
ES6 Features
ECMAScript 6 (often abbreviated as ES6) introduced a suite of new features that expand the language’s capabilities. Significant aspects of ES6 include:
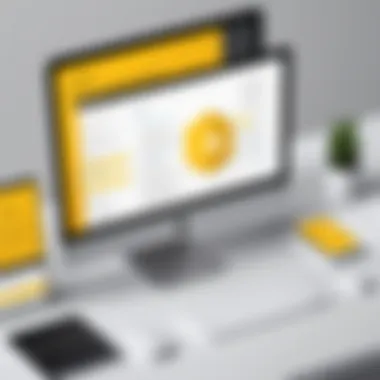
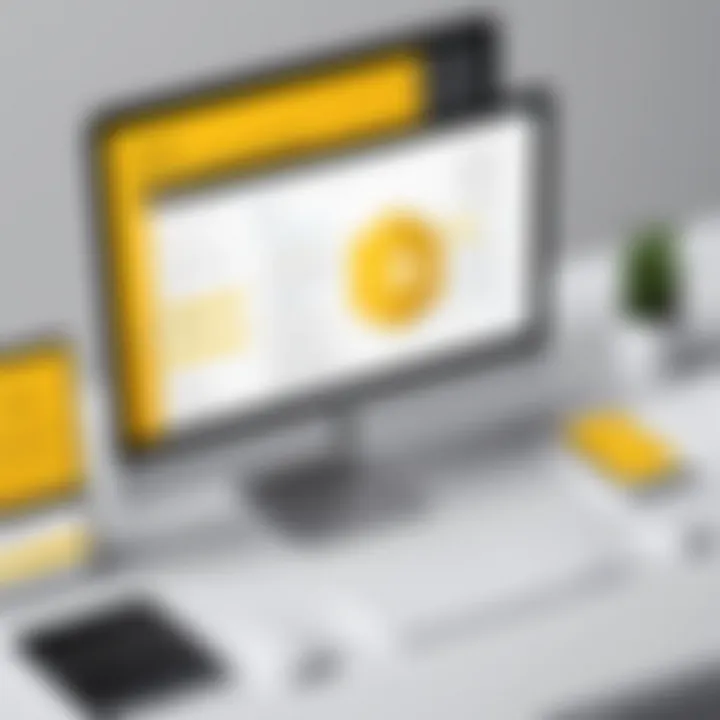
- Arrow Functions: Offer a concise syntax for writing functions, which can also enhance lexical scoping.
- Template Literals: Allow embedded expressions, making string creation simpler and clearer.
- Destructuring Assignment: Enables unpacking values from arrays or properties from objects more intuitively.
Despite these advantages, ES6 can pose compatibility challenges since older browsers may not support it natively. Developers and teams should consider tools like Babel to transpile ES6 code for older environments, avoiding browser compatibility issues.
Asynchronous Programming
Asynchronous programming is another modern JavaScript aspect that improves how developers handle operations that take an uncertain amount of time to complete, like network requests or file handling. Key benefits of asynchronous programming include:
- Non-blocking operations: Ensures that APIs and web pages remain responsive to user input while performing operations.
- Manageability: Promotes better organization of code, reducing the complex nesting problems that often come with callbacks.
One popular method to manage asynchronous operations is using the syntax, which leads to a more readable and maintainable code structure. Nonetheless, as you embrace asynchronous programming, this approach can introduce difficulties in error handling if not executed thoughtfully. Policies and practices should be defined to handle these errors effectively, ensuring that the robustness of the application is not compromised.
Key takeaway: Embracing modern features minimizes common reprimanding pitfalls and drives developers toward a more efficient coding practice. Balancing new techniques and maintaining compatibility with older systems is critical in this ever-evolving technological landscape.
Exploring JavaScript Frameworks and Libraries
JavaScript frameworks and libraries play a significant role in modern web development. With the growing complexity of applications, these tools can streamline the process and simplify many common tasks. They offer built-in functions that enable developers to focus on their code instead of dealing with repetitive and mundane problems. Overall, using frameworks and libraries helps enhance productivity and code quality.
Preamble to Frameworks
Frameworks in JavaScript provide a structured approach to building applications. They act as groundwork upon which developers can build and manage their projects more effectively. Typically, a framework sets certain standards on how an application should be organized and how different components should interact with each other.
The presence of a framework fosters collaboration between team members, making it easier to pick up a project and continue development. This aspect accelerates development cycles, making it firmer to maintain the code over its lifecycle. In addition, many frameworks come with comprehensive documentation and thriving community support which are essential for quick issue resolutions and learning resources.
Popular JavaScript Libraries
JavaScript libraries enhance the basic functionality of JavaScript and provide pre-written code to help achieve common objectives effectively. These libraries cover a broad array of tasks from DOM manipulation to data visualization. Here are some prominent libraries that are widely used across the programming community.
React
React is developed by Facebook and is known for its ease of use when creating user interfaces, particularly single-page applications. It allows developers to build reusable UI components, promoting better organization and management of your code.
A key characteristic of React is its virtual DOM representation, which optimizes rendering and boosts application performance. This feature cuts down on direct interactions with the actual DOM, resulting in a smoother user experience. Though React shines in application speed, its steep learning curve with JSX syntax can present challenges for newcomers. Still, for many developers, the benefits outweigh the initial struggles.
Vue.
js
Vue.js has quickly gained reputation for both its simplicity and flexibility, making it well-suited for integrating into existing projects. It features a progressive framework approach, which means that you can adopt it incrementally depending on your specific requirements. It provides the developer with choices, rather than mandating specific structures.
Vue.js’s key feature is its reactive two-way data binding, allowing real-time synchronization between the model and view components. This behavior enhances user interactivity greatly. While Vue.js's community and ecosystem have steadily grown, it still cannot completely match the extensive resources afforded by larger libraries like React or Angular, which may make staying up-to-date somewhat challenging for developers.
Angular
Angular is a comprehensive framework supported by Google, designed for building complex, enterprise-level applications. With Angular, developers can create scalable apps efficiently thanks to its modular architecture.
One outstanding feature of Angular is its strong support for TypeScript, which adds type-checking and object-oriented features to JavaScript. This addition helps catch errors during development and results in more reliable code. Still, Angular's complexity and array of concepts can be overwhelming for newcomers; however, once understood, it can greatly facilitate ongoing application enhancement and scalability.
Future Trends in JavaScript
The realm of JavaScript is not static; it evolves constantly. Understanding the future trends not only adds depth to one's programming skill set but also keeps one competitive in a rapidly changing tech environment. Developers and organizations are keen on trends that might affect their existing code and the future of their applications. Embracing these shifts can lead to enhanced performance and better practices in project development.
Upcoming Features and Standards
The evolution of JavaScript is closely tied to the updates in the ECMAScript standard. Each new version often comes packed with features that revolutionise code practices. For instance, the inclusion of (?.) and (??) has sharpened how programmers write concise and error-resistant code. The establishment of TypeScript has further reinvigorated discussions about type safety and its advantages. Keeping abreast of such features is key while allotting time to refactor existing codebases to apply these new standards effectively.
A few noteworthy features in the pipeline include:
- Private class fields
- Top-level await
- Logical assignment operators
These features stand to automate workflows more and allow for cleaner code. Eventually, keeping updated with these standards not only ensures developer proficiency but also improves maintainability and efficiency.
JavaScript in Emerging Technologies
JavaScript’s versatility lends it a critical role in several contemporary technologies, notably Artificial Intelligence and the Internet of Things. Each domain offers unique challenges and capabilities that tech developers must exploit effectively.
Artificial Intelligence
Artificial Intelligence (AI) leverages algorithms to perform tasks that typically require human intelligence. JavaScript, particularly with frameworks like TensorFlow.js, enables the construction and deployment of AI models directly within web browsers. This aspect appeals to many developers due to the ability to work within a familiar environment.
The key characteristic of AI that is beneficial is its capacity for deep learning models and data analysis directly in the frontend applications. The contribution it makes is notable in enhancing user experience through personalized content and intelligent user interfaces.
A unique feature of implementing AI with JavaScript is the easy interaction between UI elements and AI processing, vastly simplifying real-time predictions and analytics. However, one should consider potential downsides; complexities of AI models could contribute to performance issues on weaker devices.- This is where client-side and server-side processing would need to be carefully structured.
Internet of Things
Internet of Things (IoT) represents a network of interconnected devices that communicate and exchange data. The beauty of JavaScript shines in IoT platforms because of its ability to handle asynchronous events so efficiently.
This interconnectedness interprets the data from various devices and platforms seamlessly, which proves essential for creating efficient IoT applications. Moreover, server-side frameworks like Johnny-Five enable developers to work with hardware effortlessly. The major appeal lies in JavaScript's wide availability across browser environments rather than traditional static languages like C++ for IoT compatibility.
When examining the unique features of IoT, security stands out as a significant concern when enabling device interconnectivity. Codes should always emphasize robust defenses against potential vulnerabilities to ensure data privacy and device integrity. Balancing interactivity with security measures becomes essential in keeping applications reliable.
Keeping an eye on both AI and IoT trends is crucial for futurustic projects. Adapting JavaScript into these domains promises sustainable growth in technology.