Mastering Object-Oriented Programming Concepts
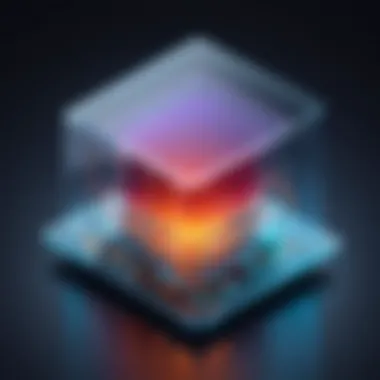
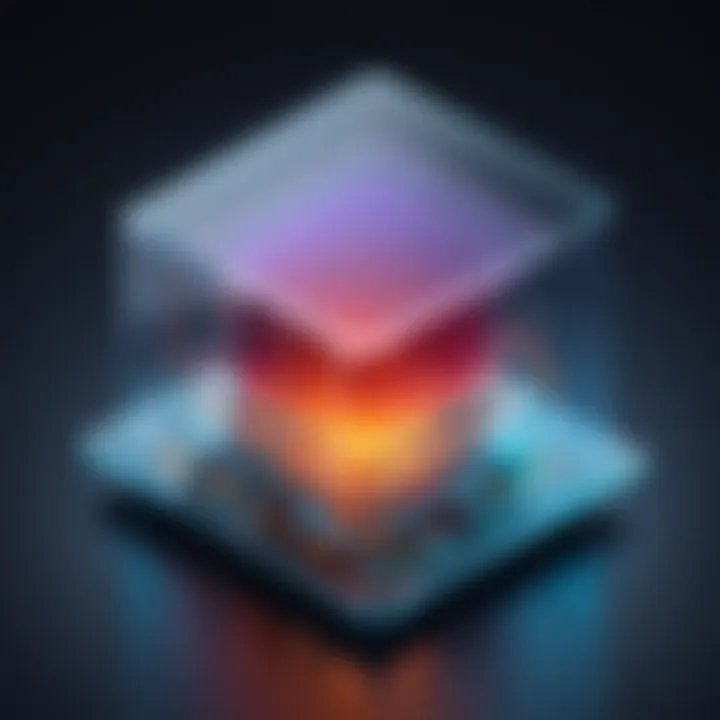
Intro
Object-oriented programming (OOP) has emerged as a key paradigm in the realm of software development, shaping the way we conceive, design, and implement applications today. It's not merely about writing code—it's about organizing it in a way that mirrors real-world interactions and entities. When you think of programs in terms of objects that possess attributes and behaviors, suddenly the lines of code transform into living entities.
Why Understanding OOP Matters
Grasping the core principles of OOP is crucial for aspiring coders and seasoned developers alike. In a world dominated by complex software systems, knowing how to effectively utilize OOP concepts like encapsulation, inheritance, and polymorphism can provide significant advantages. These principles foster a clean, reusable, and maintainable codebase. Moreover, many modern programming languages, such as Java, Python, and C#, are built around these OOP principles, making them indispensable skills for anyone keen on excelling in technology.
Key Concepts
Each tenet of OOP contributes prominently to the overall efficiency and organization of code. Let’s illuminate some of these foundational ideas:
- Encapsulation: Bundling the data and methods that operate on that data within one unit, or class, protects the object's integrity. This means internal states are shielded from unintended interference.
- Inheritance: This allows one class to derive properties and behaviors from another, promoting code reusability and extending existing functionalities with minimal changes.
- Polymorphism: Through this concept, a single interface can represent different underlying forms, enabling flexibility in method functionality.
By understanding how these principles intertwine, developers can craft robust applications that are not only functional but also scalable and easy to manage.
Where to Go From Here
In this exploration of OOP, we will delve deeper into these principles, their applications and advantages. Whether you are coding a small project or large enterprise software, the insights in this article will equip you with the knowledge to navigate the complexities of OOP effectively.
Preamble to Object-Oriented Programming
The landscape of programming has undergone a significant transformation over the past several decades. As software becomes increasingly complex, the need for a more structured approach to coding has emerged, bringing object-oriented programming (OOP) to the forefront. This section aims to unpack the importance of OOP in software development, shedding light on its elements, benefits, and considerations.
Object-oriented programming lays the groundwork for designing scalable and maintainable code. At its heart, OOP organizes software design around data, or objects, rather than functions and logic. This shift allows developers to model real-world entities more intuitively. By encapsulating data and behavior within objects, programmers can build systems that mirror the complexities of their domains, making the coding process more relatable and understandable.
Historical Background
The advent of programming paradigms
The world of programming didn't always revolve around OOP. Just as humanity moved from primitive tools to more sophisticated machinery, programming evolved through various paradigms. In the early days, procedural programming dominated, focusing on sequential tasks and functions. As complexity grew, so did the need for better structuring techniques, paving the way for OOP's advent.
The main contribution of these paradigms was a more organized way of thinking about code. With OOP, developers could build modular, reusable components that significantly reduce redundancy. One of the key characteristics of this shift is its emphasis on abstraction, allowing programmers to hide complex details and expose simpler interfaces. Because of this, OOP became a popular choice for large applications, as it helps manage the inevitable complexity.
However, OOP is not without its challenges. One unique feature is its tendency to lead to over-engineered solutions, where developers can sometimes create hierarchies that are larger than necessary. This downside stresses the importance of consciously applying OOP principles to avoid losing sight of simplicity.
Evolution of OOP concepts
As programming languages have matured, so too have the concepts surrounding OOP. The transition from monolithic procedural code to OOP has been driven by the need to create more efficient and maintainable applications. This evolution has led to the refinement of core concepts like encapsulation, inheritance, and polymorphism.
The main contribution of this evolution lies in its capacity to drive innovation. OOP concepts allow developers to create flexible systems that can easily adapt to changing requirements. A key highlight of these concepts is their focus on reusability. Once a class is defined, it can be reused across different parts of a program or even different projects altogether, saving time and effort.
Despite its benefits, the evolution of OOP also presents some unique challenges. For example, a developer may struggle with deciding appropriate levels of abstraction, leading to potential inefficiencies in code management. Balancing abstraction with practicality is essential in leveraging the advantages of OOP.
Key figures in OOP development
The establishment of OOP is attributed to several influential figures, one of which is Alan Kay. His vision of computational objects paved the way for the development of languages like Smalltalk, which encapsulated many of OOP's core principles.
The contributions of pioneers like Kay are crucial in understanding OOP's underpinnings. These individuals provided the theoretical frameworks that allow developers to embrace OOP effectively. The characteristic of their work is a blend of creativity and practicality, encouraging programming as a dynamic form of problem-solving.
One unique feature of these developments is the philosophical undercurrents that challenge traditional views of programming. By viewing objects as the primary units of computation, these thinkers opened up new avenues for understanding problems, allowing for a more holistic approach to software design.
Core Definitions
Defining objects and classes
At the core of object-oriented programming are objects and classes. Objects represent instances of classes, embodying both data and the methods that operate on that data. This relationship between classes and objects is fundamental in understanding how OOP functions.
The contribution of clearly defining these concepts cannot be overstated. It lays the groundwork for everything that follows in OOP methodology. One crucial characteristic is that classes serve as templates for creating objects, essentially allowing multiple objects to share similar functionality while retaining individual states.
A unique feature of this definition is the creation of hierarchies, which can sometimes lead to confusion about relationships among objects. It can introduce complications when trying to determine the state of an object. However, this complexity also allows for more sophisticated designs.
Understanding attributes and methods
Attributes and methods are essential components of objects in OOP. Attributes hold data, while methods define the behaviors that can be performed on that data. Understanding this relationship is key to effectively utilizing OOP.
Attributes provide a way to describe the properties of an object, allowing developers to categorize and modify them easily. One noteworthy characteristic of attributes is that they facilitate modular programming by allowing its dynamic manipulation. Conversely, methods not only encapsulate functionality but also lead to a more natural understanding of how objects interact.
One drawback is that poorly defined attributes or methods can lead to confusion and a lack of clarity in object behavior, resulting in code that is challenging to maintain.
Distinguishing between procedural and object-oriented programming
It's fundamental to grasp the differences between procedural and object-oriented programming as each caters to different needs. Procedural programming organizes code into functions, focusing on the sequence of actions, while OOP revolves around objects that combine data with methods.
The contribution of recognizing these differences helps software architects choose the right paradigm for their projects. The key characteristic of OOP is its flexibility, offering a contrasting approach to the fixed structure of procedural programming.
A unique feature of this distinction is its implications for debugging and testing, which can often be simplicity in procedural models but more complex in OOP due to interdependent objects. This complexity can be a double-edged sword, offering richer capabilities at the potential cost of readability.
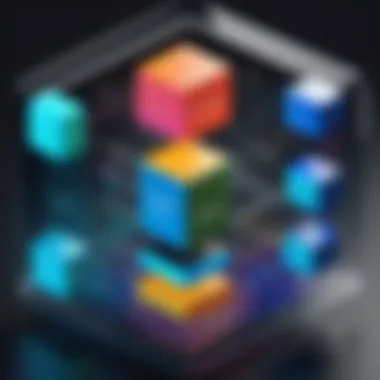
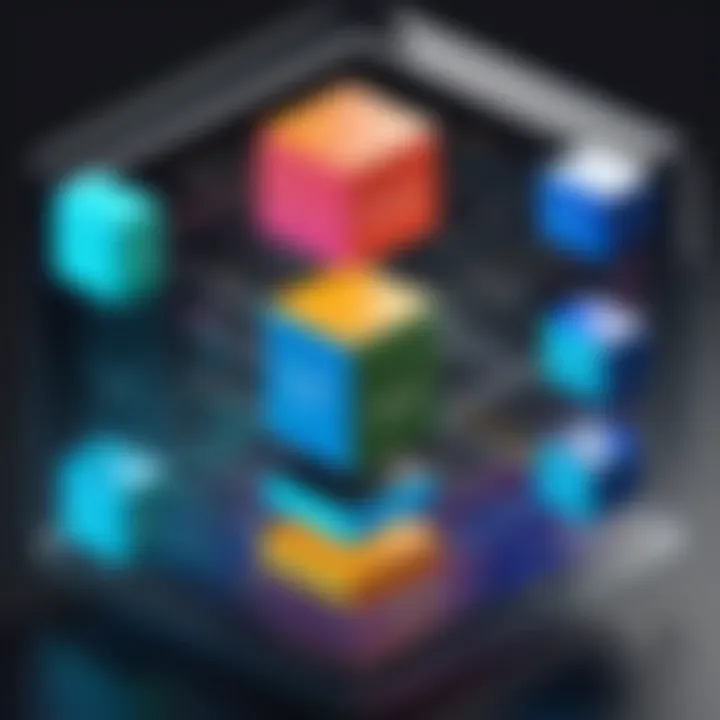
Understanding these foundational concepts of OOP provides a lens through which programmers can grasp more advanced techniques and principles, ultimately enhancing their skill set.
Fundamental Principles of OOP
Understanding the fundamental principles of Object-Oriented Programming (OOP) is crucial for developers aiming to create robust and flexible software applications. These principles—encapsulation, inheritance, and polymorphism—serve as the bedrock for creating maintainable and scalable code. They enable programmers to model complex systems by grouping related data and behaviors, promoting code reusability and enhancing the clarity of their designs.
Encapsulation
Protecting data integrity
Encapsulation stands as a guardian of data integrity in OOP by restricting access to the inner workings of objects. This idea is like placing a lock on a treasure chest—only those with the key can access what’s inside. By safeguarding an object's state, it minimizes unintended interactions that might corrupt data. For instance, if a car object possesses a speed attribute, affecting this directly could lead to errors if the values become inconsistent with the car's operational rules. Encapsulation ensures that speed can only be modified through carefully crafted methods that maintain the necessary conditions.
Not only does encapsulation protect data, but it also simplifies the interface through which other parts of the program interact with an object. It’s like having a clear interface to a vending machine—pressing a button to receive a drink is straightforward, you need not understand the complex mechanisms inside.
Access modifiers explained
Access modifiers are the keys that determine who can interact with an object’s methods and attributes. In languages like Java and C++, these modifiers often take the forms of , , and . A modifier may prevent external classes from accessing a variable, much like keeping certain secrets close to the vest. This level of control fosters better security and helps prevent misuse of the data, which is a valuable aspect of OOP.
The downside? Access modifiers can introduce complexity. Overusing them might lead to a situation where necessary functionalities become too buried, making it hard for developers to work efficiently. Striking a balance is key.
Real-world analogies
Using real-world analogies makes the principles of encapsulation more relatable. Imagine a bank’s ATM. The ATM displays only the functions needed by the user—withdraw money, check balance, and deposit funds. It cleverly hides complex processes like transaction security and account management, presenting a neat interface for customers. This analogy highlights how encapsulation leads to user-friendly interfaces, while behind the scenes, a multitude of sophisticated interactions are occurring without the end user needing to grasp the complexities.
Inheritance
Extending functionality
Inheritance offers a powerful way to extend the functionality of existing classes. Think of it like passing down traits from parent to child. If a class exists, you can create a class that inherits all properties and methods from , then adds its own unique features. This reduces redundancy by allowing the reuse of code. It’s a handy mechanism, especially in larger projects where multiple entities share common traits.
However, the downside of inheritance can be seen in situations where a deep inheritance hierarchy complicates the design. If changes are required at the base class, unintended consequences might ripple through all derived classes, potentially leading to a nightmare in debugging.
Types of inheritance
When we talk about types of inheritance, it's interesting to note how different programming languages handle this concept. Common types include single inheritance, where a class derives from one parent class, and multiple inheritance, where a class can inherit from more than one.
For example, C++ supports multiple inheritance, enhancing flexibility but also introducing complexity and potential ambiguity, known as the diamond problem. On the other hand, languages like Java restrict it to single inheritance, promoting simplicity but sometimes at the expense of substituting interfaces for multiple inheritances.
Deciding on which type to use depends on the specific needs of the project and its design goals.
Composition vs. inheritance
There’s an ongoing debate in software design regarding composition versus inheritance. Composition involves building complex objects from simpler ones—kind of like constructing a meal with different ingredients. For instance, an can be a component of a class. It’s flexible and allows for easier modifications without drastic changes throughout the codebase.
Contrasting this, inheritance creates rigid relationships between classes. A change in the parent class can lead to cascading changes in all derived classes. Consequently, while inheritance can ease code reuse, composition's flexibility usually leads to a cleaner and more maintainable code structure. Neither approach is inherently better than the other; it's about the right tool for the task.
Polymorphism
Method overriding and overloading
Polymorphism brings magic into OOP by allowing objects to be treated as instances of their parent class, enhancing flexibility. Method overriding is when a subclass provides a specific implementation for a method already defined in its parent class. For example, if you have a method in a class, different animals can override this method to provide their unique sounds. This encourages the use of the same interface while allowing specialized behavior.
Method overloading, on the other hand, is about having several methods in the same class with the same name but different parameters. It helps maintain intuitiveness in method usage. Think of it as a light switch that can operate in different ways based on how many fingers you press on it.
While both methods provide great utility, overloading can sometimes confuse readers if overloaded methods are not sufficiently distinguished by their parameters.
Runtime vs. compile-time polymorphism
Runtime polymorphism occurs when the method that’s going to be invoked is determined at runtime, usually through late binding. This is often accomplished through method overriding. Compile-time polymorphism, however, is established during the compilation of the program using variations of method signatures—this is where method overloading comes into play.
Both forms have their own advantages. Runtime polymorphism allows for greater flexibility and reduces the coupling of code, but it can lead to performance overhead due to the additional work done at runtime. Compile-time polymorphism, while faster, can lead to less flexible designs as they cannot easily adapt to new requirements.
Benefits of polymorphism in software design
By promoting polymorphism, developers allow for more adaptable and extensible code. This characteristic leads to easier updates and modifications over time, as new classes can easily plug into existing systems without jeopardizing the integrity of current functionality.
Moreover, it enhances code readability and maintainability. For example, when there’s a uniform interface for different objects, new functionalities can be executed with fewer changes in the codebase. The downside, however, is that it could introduce complexity for those trying to trace through a codebase that heavily uses polymorphism, especially if the implementations are not well documented.
Designing OOP Applications
Designing applications with object-oriented programming (OOP) principles is pivotal for creating maintainable, scalable, and efficient software. OOP encourages a systematic approach, leading to better organized code that reflects real-world scenarios. Understanding how to design OOP applications not only enhances a programmer's capabilities but also optimizes the entire development process, making it necessary for modern software engineering.
Applying OOP Principles
Identifying class structures
A cornerstone of effective OOP design is the identification of class structures. This involves determining how objects are related and what their responsibilities are within a software application. By creating a well-thought-out class structure, developers can promote code reusability and ease of maintenance. A key characteristic of identifying class structures is its ability to model complex entities in a simplified manner, making it a popular choice for this article.
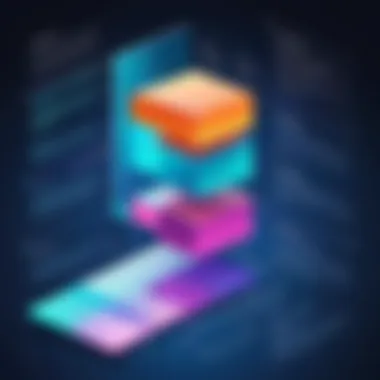
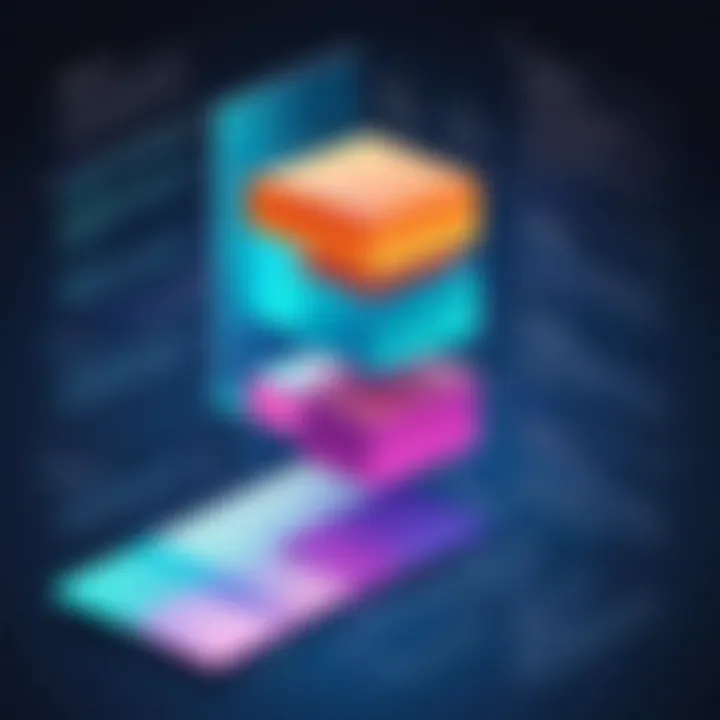
Classes act as blueprints for objects, encapsulating data and behaviors in a single unit. One unique advantage here is that once a class is defined, new instances can be created quickly and efficiently. Nonetheless, it requires careful consideration and can lead to over-complicated designs if not executed properly.
Behavior modeling with interfaces
Behavior modeling through interfaces forms a bridge between disparate components of an application, allowing them to communicate without tight coupling. This contributes to the overall topic by illustrating how interfaces can promote flexibility and adaptability in software designs. The key characteristic here is that interfaces define methods without implementing them, ensuring that various classes can interact seamlessly while hiding the complexities of their implementation.
A notable advantage of using interfaces is that they permit different classes to be used interchangeably, fostering polymorphic behavior. However, a disadvantage could come from overly relying on interfaces, leading to confusion if not documented well. The challenge lies in striking the right balance between abstraction and concrete implementation.
Practical design patterns
Practical design patterns are recurring solutions to common software design problems. They provide a proven framework that helps developers implement OOP principles effectively. One key characteristic of design patterns is that they are categorized into creational, structural, and behavioral types, each addressing different design scenarios. This makes them a valuable asset in this article.
For instance, using the Singleton pattern can ensure a class has only one instance, while the Observer pattern allows classes to be notified of state changes in others. The unique feature here lies in the time-tested nature of these patterns, which can greatly reduce development time and effort. However, one downside is that overusing patterns may lead to unnecessary complexity and ambiguity in the code, so developers must apply them judiciously.
Common OOP Languages
Comparative analysis of ++, Java, and Python
When discussing OOP languages, a comparative analysis of C++, Java, and Python stands out. These languages represent a spectrum of OOP capabilities and complexities, and understanding their differences is essential for effective application design. Each language has its unique characteristics that cater to various development needs.
C++ offers deep control over memory management with its class mechanisms and pointers, while Java emphasizes platform independence and robust standard libraries. On the other hand, Python is appreciated for its simplicity and readability, making it a fantastic choice for rapid development. However, with C++'s complexity comes a steeper learning curve, whereas Java and Python might lack some lower level control. This spectrum informs the decision-making process when selecting the right language for a given project.
Language-specific features
Language-specific features greatly influence how developers implement OOP principles. For instance, Java’s strict enforcement of encapsulation through access modifiers is a compelling feature, providing a clear structure for data hiding. In contrast, Python favors flexibility with less strict access controls, allowing for greater developer freedom at the cost of strict encapsulation principles.
Understanding these features informs programmers about the strengths and limitations of each language when designing OOP applications. However, the risk lies in selecting a language based solely on features without considering the project’s requirements, leading to potential pitfalls down the road.
Choosing the right language for a project
Choosing the right programming language for a specific project cannot be understated. The decision hinges on factors like project scope, team expertise, and performance requirements. Each language discussed offers distinct advantages—C++ in high-performance applications, Java in large enterprise solutions, and Python in rapid prototyping and data analysis.
A unique feature when making this choice is weighing long-term maintainability against immediate productivity gains. For instance, while Python allows for quicker development cycles, its performance may not match that of C++. It's crucial to align the language choice with the goals and constraints of the project for optimal outcomes.
Best Practices in OOP Programming
When venturing into the intricate realm of Object-Oriented Programming, developing a solid understanding of best practices can make all the difference. The world of programming is riddled with potential pitfalls, and following established best practices can not only prevent these issues but also enhance the maintainability and scalability of software. By adhering to proven methodologies, programmers ensure that their code not only functions correctly but is also easier to read, understand, and modify in the future.
Avoiding Anti-Patterns
Recognizing common pitfalls
Recognizing common pitfalls is paramount in addressing detrimental programming habits that can lead to bloated, inefficient code. It serves as a critical part of the software development process. One of the key characteristics here is the ability to identify these anti-patterns early in the game. For example, the God Object anti-pattern can significantly hamper project integrity, as this quirky pattern allows for excessive control over many class interactions, which muddles future modifications.
Understanding these pitfalls is a beneficial choice for this discussion. By grasping what leads a team down an inefficient path, programmers are more equipped to prevent future missteps. A common unique feature of recognizing these missteps is that it encourages ongoing learning and discussion among teams, leading to a more robust codebase.
Refactoring strategies
Diving into refactoring strategies provides programmers tools to mend existing code without altering its external behavior. It’s about cleaning up the mess without throwing the baby out with the bathwater. One pivotal aspect of these strategies is the technique of simplifying complex functions into smaller, more manageable pieces.
This is a popular choice because it enhances readability and fosters easier debugging. It allows teams to tackle issues systematically, thus improving collaboration. The unique feature of refactoring lies in its capacity for continuous improvement; however, excessive refactoring can occasionally lead to what some call "analysis paralysis"—where developers get bogged down trying to make every detail perfect.
Coding standards for maintainability
When it comes to coding standards for maintainability, standardization of code style and structure emerges as a prominent player. This ensures that all developers on a team can seamlessly navigate the code. A key characteristic here is the establishment of guidelines that dictate variable naming conventions, code formatting, and file organization.
Such standards help foster a cohesive working environment, making this approach beneficial not just for individual projects but for long-term team collaboration. The unique advantage of adhering to coding standards lies in the ability to onboard new members easily into projects, allowing them to comprehend the structure without extensive guidance. On the other hand, rigidity in coding standards can stifle creativity if not approached with some flexibility.
Testing and Debugging OOP Code
Moving into testing and debugging, having robust practices in place is just like having a safety net beneath the tightrope walker. It allows developers to pinpoint issues and ensure reliability in their codebase. This is where the necessity of systematic approaches comes to light.
Unit testing in OOP environments
Unit testing is a core component in OOP environments, allowing programmers to assess individual components in isolation. With its primary goal being to validate that each piece functions as intended, unit testing often leads to a greater overall code quality. One key characteristic of unit testing is its focus on small testable segments of code, promoting modularity.
This method is a popular choice because it permits early detection of faults, significantly reducing the timeframe for fixing bugs later in the development cycle. Its unique feature is that it fosters a culture of testing from the ground up. However, this process can sometimes require excessive time and resources if the initial code is cumbersome.
Utilizing test-driven development (TDD)
Test-Driven Development (TDD) flips the traditional development cycle on its head by putting the test creation in the driver’s seat. Here, programmers write tests before they even write the code. This approach guides the development process more efficiently by ensuring that new features fit within predefined expectations. A major characteristic of TDD is the iterative cycle it promotes, encouraging continuous testing and improvement.
Utilizing TDD is beneficial as it produces clearer and bug-free code from the get-go. The unique feature here is the emphasis on defining behavior before implementation, though, on the flip side, it can seem daunting as it requires upfront commitment to a testing framework.
Debugging techniques for complex systems
Debugging techniques for complex systems often resemble detective work. Addressing bugs within a sprawling codebase can seem like finding a needle in a haystack. A critical aspect of this is systematic approach, such as using logging, breakpoints, and interactive debuggers to trace issues.

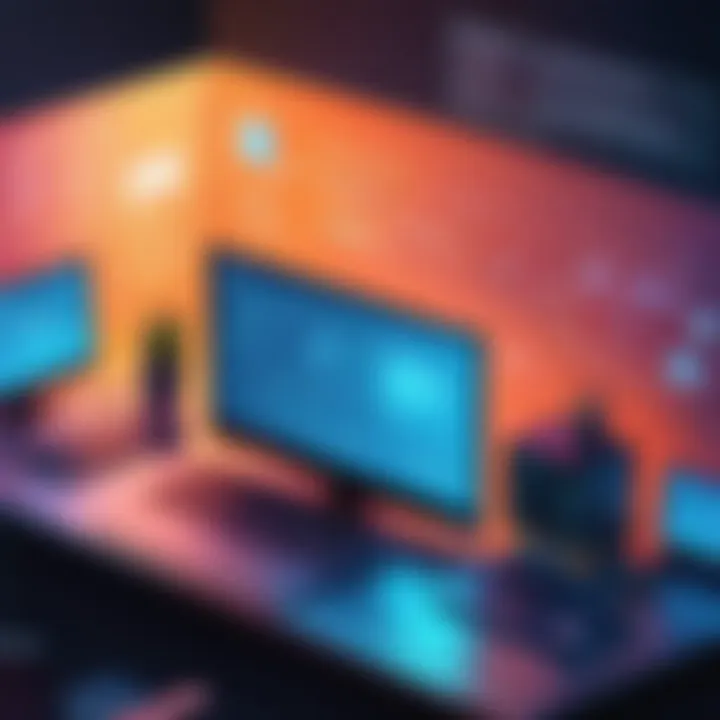
This structured approach offers a clear path toward resolving problems, enhancing understanding of the system's inner workings. Its unique feature is the potential for real-time application monitoring, which can identify faults before they impact users. However, overly complex debugging methods can overwhelm programmers, necessitating a balance between thoroughness and simplicity.
"In software development, the road to success is paved with best practices. Ignoring them is like driving blindfolded; you might get lucky or you might end up in a ditch."
In summary, adopting best practices in OOP programming not only mitigates risks but also contributes to a more harmonious coding experience.
Emerging Trends in Object-Oriented Development
In the rapidly evolving world of software development, staying ahead of the curve is paramount. Emerging trends in object-oriented programming not only guide current practices but also set the stage for future developments. This section will delve into the intricate relationship between object-oriented programming (OOP) and newer architectural paradigms, particularly focusing on microservices and the integration with functional programming. These discussions will elucidate their benefits, challenges, and practical implementation in the modern coding landscape.
Microservices and OOP
Transitioning to microservices architecture
As organizations push toward more scalable and flexible applications, the transition to a microservices architecture has gained traction. This architectural style breaks down a monolithic system into smaller, independently deployable services. One key characteristic of this transition is its emphasis on scalability and resilience. By allowing teams to build, test, and deploy services independently, microservices facilitate faster delivery and a more dynamic response to user needs.
However, while microservices facilitate agility, they also introduce complexity. Each microservice can be seen almost as an object in OOP, encapsulating specific functionalities and utilizing methods to interact with other services. The beneficial aspect of this approach is that it enables continuous deployment—teams can roll out updates or new features without taking down the entire system. On the flip side, the unique feature of this architecture involves managing multiple services, which can lead to increased overhead and the necessity of robust orchestration strategies.
Role of OOP in distributed systems
Object-oriented programming plays a crucial role in the development of distributed systems. By leveraging the principles of OOP, developers can create services that are self-contained and modular, mirroring how objects interact within a single application. This modularity is a popular choice when building distributed systems as it supports different services communicating through well-defined interfaces.
The real advantage of OOP here lies in its ability to encapsulate state and behavior, making it easier to manage data consistency across distributed components. However, a challenge arises when attempting to maintain the integrity and performance of these interactions, especially as the number of objects—or services—grows. Here, the elegant design of OOP can become a double-edged sword, offering both clarity in structure and potential complexity in integration.
Challenges and solutions
Transitioning to new paradigms in OOP often brings its own set of hurdles. The challenges of adopting microservices and other innovative practices include the difficulty in managing service orchestration, the need for effective data management strategies, and the complexities inherent in ensuring cross-service communication.
Despite these obstacles, it is essential to explore solutions that promote success. Implementing tools such as service meshes can significantly ease the pain of managing service communications. Additionally, adopting practices like API-first design ensures that interactions between services are clear and maintained consistently, ultimately leading to a more resilient and adaptable system.
Integration with Functional Programming
Harmonizing OOP with functional paradigms
As functional programming concepts gain traction, the harmony between OOP and functional paradigms presents fascinating opportunities. The integration of these two styles allows developers to leverage the readability and reusability principles of OOP alongside the declarative programming style of functional programming.
One unique aspect of this blend is the adoption of immutability, a central tenet of functional programming. This can add a layer of reliability to object states, capturing the advantages of both styles. The trade-off here often lies in the learning curve involved. Developers must adapt to new methodologies while maintaining the structure provided by OOP, creating potential friction in team dynamics.
Key benefits and trade-offs
Choosing to harmonize OOP with functional programming can lead to several key benefits, such as improved code quality, easier testing, and greater flexibility. Additionally, it fosters a programming environment where developers can choose the best approach for a given problem.
However, this merging brings its own trade-offs, specifically regarding performance. Functional programming, while elegant, sometimes carries overhead in execution and memory usage compared to traditional OOP. Balancing these elements is vital when crafting applications designed for high-performance environments.
Adapting existing codebases
Integrating functional concepts into legacy codebases requires a thoughtful approach. Start by identifying areas within your OOP structure where functional programming can enhance maintainability and performance. It might also involve revisiting foundational components and refactoring them to incorporate immutability and first-class functions effectively.
Besides improving modularity, adapting existing code to include functional capabilities can lead to a more robust application overall. Nevertheless, juggling existing systems with new paradigms can introduce challenges such as compatibility and increased complexity during transitions.
In summary, emerging trends in object-oriented development—from microservices to the marriage of functional programming—represent a vital evolution of programming practices. These developments not only offer exciting pathways for enhancing modularity and usability but also demand a new level of expertise and adaptability from developers. Navigating these trends will position programmers to meet future demands in a constantly changing digital landscape.
Epilogue and Future Directions
The landscape of Object-Oriented Programming is continually evolving, making a grasp of its principles crucial for both budding developers and seasoned professionals. The conclusion section serves not only as a summary but also as a springboard into the future of OOP practices. With technology racing ahead at breakneck speed, understanding the potential developments within object-oriented paradigms is paramount. Emphasis on adaptation, innovation, and a commitment to learning will arm developers for the challenges that lie ahead.
Summarizing Key Takeaways
Recap of OOP principles
The key principles of OOP – encapsulation, inheritance, and polymorphism – form the backbone of stress-free programming. These ideas enable developers to create organized, reusable code. Encapsulation protects data by allowing you to control accessibility, which promotes better data integrity. Inheritance helps in building a robust code base through a hierarchical relationship that reuses code without redundant effort. Polymorphism introduces flexibility, allowing for different implementations of methods across various classes. These characteristics contribute towards smoother workflows and elevate coding practices, making them well-suited for modern software development. This article pays special attention to these fundamentals as they lay the groundwork for effective coding.
The significance of continued learning
In the fast-paced world of tech, the significance of lifelong learning can't be overstated. As new challenges rise, continuing education equips professionals with the tools to tackle complex problems confidently. Embracing continuous learning not only broadens one's skill set but also enhances adaptability. This article pinpoints the need for accessibility to new resources and knowledge. A commitment to learning creates a culture of growth, which is vital for both individuals and companies striving to stay on top in an ever-changing environment.
Anticipating future developments
Anticipating future trends in OOP is as critical as learning its foundational principles. With the integration of new technologies, such as artificial intelligence and machine learning, the paradigms of programming are set to shift significantly. Tools and languages will evolve, bringing new features and libraries that can streamline existing processes. Recognizing where the field is headed allows programmers not only to prepare but also to innovate. This foresight can lead to optimizations that align with industry trends and user needs, which can ultimately enhance productivity and software excellence.
Encouraging Lifelong Learning in Programming
Resources for enhancing OOP knowledge
The pursuit of enhancing one's OOP knowledge is enriched by a plethora of resources available today. Online platforms, video tutorials, and websites like Wikipedia or Britannica.com provide extensive information that developers can leverage. Bootcamps and coding challenges can also sharpen skills in a hands-on manner. A well-rounded approach to education encourages a deeper understanding of programming concepts, fostering a community of knowledgeable practitioners. The variety of available resources means there's something out there for every learning style.
Joining programming communities
Engaging in programming communities, such as forums on Reddit or Facebook groups, can immensely benefit both new and established programmers. These platforms provide a space to share experiences, ask for advice, and collaborate on projects. Exposure to different perspectives can spark creativity and innovation. Being part of a community means gaining access to the collective knowledge and resources shared among members. The reciprocal nature of community learning can significantly enhance personal growth and skill development.
Staying abreast of technology trends
Keeping up with technology trends is another crucial aspect of developing and maintaining OOP skills. Subscribing to newsletters, attending webinars, and following reputable tech blogs allows programmers to remain informed of shifts in the industry. Being current not only helps in honing existing skills but fosters anticipation for new tools and methods that can improve workflows. Staying updated prevents becoming out of touch, ensuring that both foundational knowledge and evolving practices are balanced for optimum performance.