Mastering Python: A Comprehensive Guide for Beginners
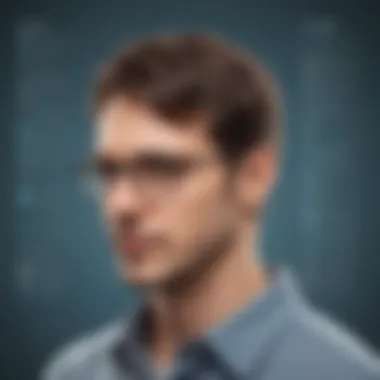
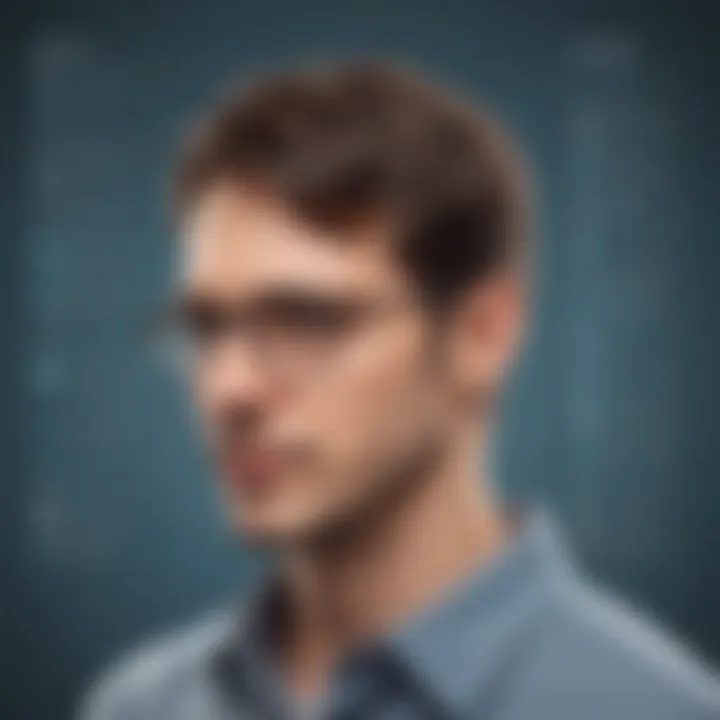
Coding Challenges
In this section, we will delve into a series of weekly coding challenges designed to test and enhance your Python skills. Each challenge will present a unique problem for solving, accompanied by detailed solutions and explanations to improve your understanding. Additionally, we will provide valuable tips and strategies to tackle coding challenges effectively, empowering you to think critically and creatively. Community participation highlights will showcase the achievements and contributions of aspiring and experienced programmers, fostering a collaborative and supportive learning environment.
Technology Trends
Exploring the latest technological innovations relevant to Python programming, this section will examine emerging technologies worth monitoring. By analyzing the impact of technology on society, we aim to provide insights into future trends and possibilities within the realm of programming. Expert opinions and analyses will offer a deeper understanding of the dynamic tech landscape, guiding aspiring and experienced programmers towards informed choices and developments in the field.
Coding Resources
Within this section, we will curate a collection of programming language guides, software reviews, tutorials, and how-to articles tailored to Python enthusiasts. Comparing online learning platforms, our aim is to equip readers with valuable resources and tools for continuous skill enhancement. By providing a comprehensive overview of coding resources, we endeavor to empower individuals with the knowledge and support necessary for navigating the vast landscape of programming effectively.
Computer Science Concepts
Delving into fundamental computer science concepts, this section will explore primers on algorithms, data structures, artificial intelligence, machine learning, networking, security fundamentals, and future technologies like quantum computing. By dissecting complex topics into digestible insights, we aim to broaden the understanding of readers on critical concepts shaping the technological landscape. Understanding these foundational principles is essential for aspiring programmers, technology enthusiasts, computer science students, and IT professionals looking to excel in their respective domains.
Introduction to Python
In the expansive world of programming languages, Python stands out as a versatile and powerful tool that is revered for its simplicity and readability. As we delve into the rudiments of Python in this detailed guide for beginners, we aim to lay a robust foundation for understanding its intricacies. Python's popularity stems from its emphasis on code readability and its vast array of applications, making it an ideal choice for beginners and seasoned developers alike. Exploring the basics of Python is crucial for grasping its core concepts, enabling individuals to embark on a journey towards mastering a valuable programming language.
Understanding the Basics
Python Installation and Setup
Python Installation and Setup is an elemental aspect essential for anyone aspiring to delve into Python programming. The significance of this process lies in providing users with a seamless way to establish the Python environment on their systems, enabling them to start coding without hindrances. The straightforward installation procedure of Python ensures that individuals can swiftly set up the programming language on various operating systems, be it Windows, macOS, or Linux. This simplicity makes Python Installation and Setup a favored choice for beginners, eliminating the complexities often associated with configuring programming environments. However, it is worth noting that while Python's installation process is user-friendly, attention to detail during setup is paramount to avoid compatibility issues later on.
Introduction to Python Syntax
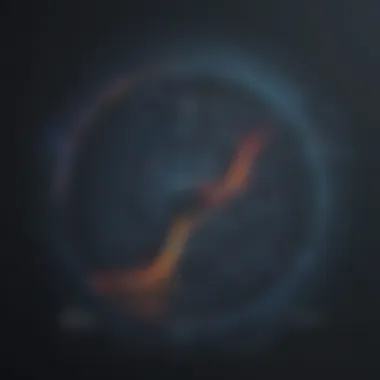
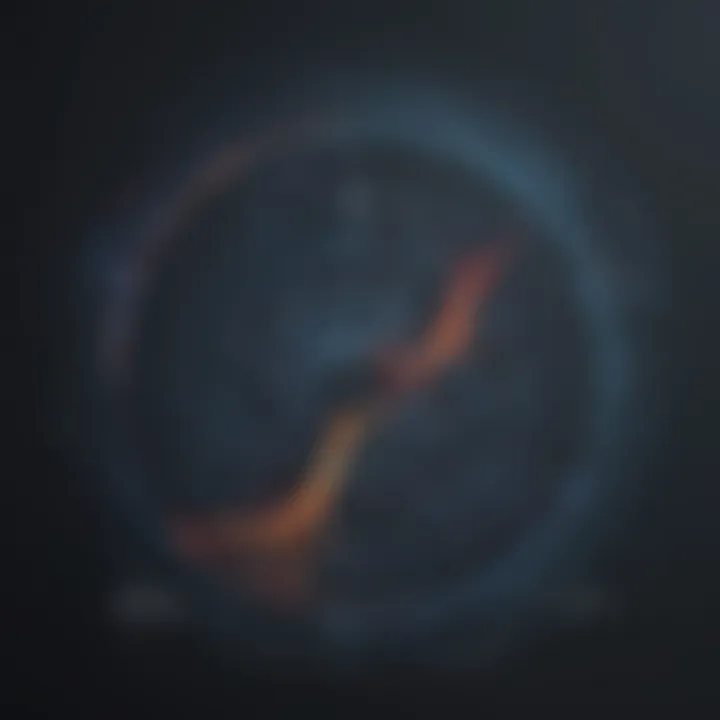
Introduction to Python Syntax serves as the gateway to comprehending the fundamental structure of Python code. This aspect plays a vital role in molding a coder's understanding of Python's syntax rules, such as proper indentation and syntax for defining variables and functions. The fundamental nature of Python Syntax makes it a popular choice among developers for its clean and expressive code style, enhancing code readability and maintainability. One unique feature of Python Syntax is its reliance on indentation to define code blocks, fostering a habit of writing organized and visually appealing code. While this feature streamlines the coding process, it may pose challenges for newcomers accustomed to other languages that use brackets or keywords to encapsulate code blocks. Understanding and adapting to Python's syntactical nuances is crucial for aspiring programmers to write efficient and error-free code.
Data Types and Variables
Numbers and Strings
The realm of Numbers and Strings in Python engulfs the handling of numerical data and textual information, constituting the cornerstone of data manipulation in programming. Mastering Numbers and Strings equips individuals with the ability to perform arithmetic operations, manipulate text, and make their code more dynamic and interactive. Python's robust support for numeric and string operations makes it a preferred choice for handling a wide range of data types, simplifying complex computations and string manipulations. A key characteristic of Numbers and Strings in Python is their immutability, ensuring that once assigned, they retain their value throughout the program's execution. Despite these benefits, individuals need to exercise caution while handling sensitive data in strings, as manipulating them improperly can lead to security vulnerabilities.
Lists, Tuples, and Dictionaries
Lists, Tuples, and Dictionaries form the crux of data structuring in Python, offering versatile ways to store and manipulate collections of data. Understanding the nuances of Lists, Tuples, and Dictionaries empowers programmers to organize data efficiently, iterate through elements, and implement complex data structures with ease. The key characteristic of these data types is their distinct capabilities - Lists facilitate mutable data storage, Tuples ensure data integrity through immutability, and Dictionaries enable key-value pair mappings for quick data retrieval. Python's support for these versatile data structures positions it as a top choice for handling diverse data organization needs, from basic lists to complex dictionary mappings. However, while these data types enhance code flexibility and readability, improper usage or manipulation can lead to runtime errors or unintended data modifications.
Control Structures in Python
Control structures in Python play a crucial role in the programming language's logic flow. They are essential elements that dictate how the code executes based on specified conditions. Understanding control structures enables programmers to create efficient and organized code. In the context of this comprehensive guide for beginners, grasping control structures in Python is fundamental for building a strong foundation in programming.
Conditional Statements
Conditional statements, including if-else statements and nested conditions, provide decision-making capabilities in Python code. When discussing if-else statements, it is essential to highlight their role in executing specific blocks of code based on whether a certain condition is deemed true or false. The key characteristic of if-else statements lies in their ability to control the program's direction, making them a popular choice for branching logic in this article. Despite their utility, if-else statements may introduce complexity in code organization due to multiple branches that need to be managed.
Nested conditions extend the functionality of if-else statements, allowing for more intricate decision-making processes. They involve embedding one if-else statement within another, expanding the range of conditions that can be evaluated. The unique feature of nested conditions is their hierarchical structure, enabling programmers to address various scenarios within the code. However, nesting conditions too deeply can lead to code readability issues and potential logic errors in this article.
Loops and Iterations
Loops and iterations are fundamental in Python for executing repetitive tasks efficiently. For loops are particularly valuable when a certain block of code needs to be repeated for a defined number of iterations. Their key characteristic lies in iterating over a sequence of elements, making them a preferred choice for iterating through lists or arrays in this article. Despite their efficiency, for loops may be less suitable for situations requiring dynamic iteration conditions.
While loops, on the other hand, excel in scenarios where code repetition is dependent on a specific condition being met. Their key characteristic lies in continuously executing a block of code until the specified condition evaluates to false. While loops are beneficial for scenarios that demand indefinite iterations or iterations based on user input. However, the risk of infinite loops poses a challenge in using while loops effectively, requiring careful handling of loop termination conditions.
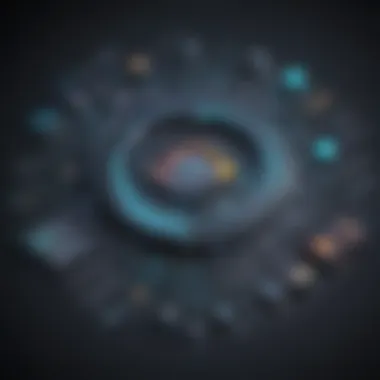
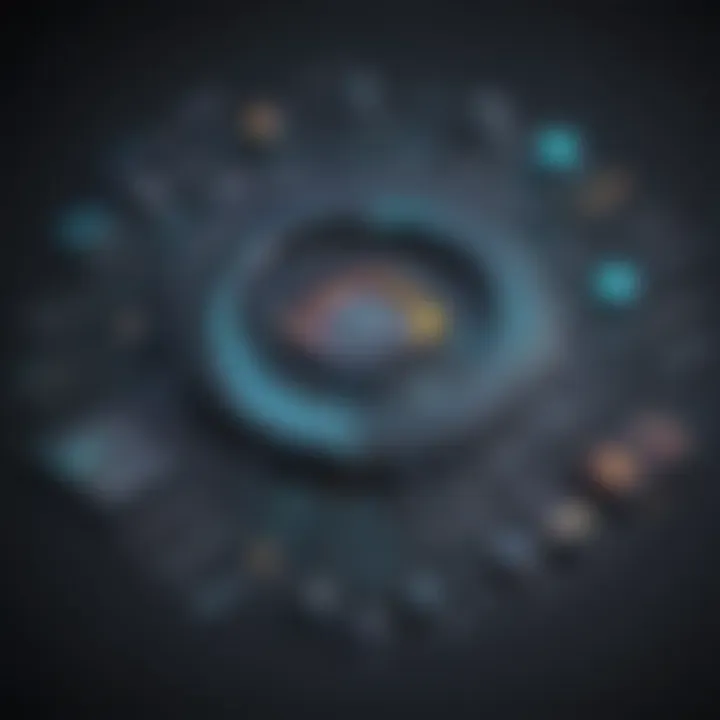
Loop control statements, like 'break' and 'continue', offer additional control within loops by altering their execution flow. These statements allow programmers to exit a loop prematurely or skip certain iterations based on specified conditions. The unique feature of loop control statements lies in their ability to fine-tune loop behavior, enhancing code efficiency and logic. While beneficial for streamlining code execution, improper use of loop control statements can lead to unintended logic flows and result in functional errors within the code.
Functions and Modules
In the realm of Python programming, Functions and Modules play a pivotal role that cannot be understated. The utilization of Functions allows for the encapsulation of code into reusable blocks, enhancing code organization and promoting efficiency. By defining Functions, programmers can break down complex tasks into manageable chunks, streamlining the coding process. Furthermore, Modules serve as containers for Functions, variables, and classes, aiding in code structuring and maintenance. In this comprehensive guide for Python beginners, the exploration of Functions and Modules brings forth a deeper understanding of code modularity and reusability, essential principles in mastering the Python language.
Defining Functions
Parameter Passing
When delving into the intricacies of Function definition, Parameter Passing emerges as a critical aspect. The method by which arguments are passed to a Function significantly impacts code functionality and flexibility. Python's versatility in Parameter Passing allows for the use of positional, keyword, and default arguments, offering programmers a spectrum of options to tailor Functions according to specific needs. Understanding the nuances of Parameter Passing lends a strategic advantage in crafting efficient and dynamic Functions, contributing to the overarching goal of code optimization in this guide.
Return Values
Another fundamental component of Function definition is the concept of Return Values. Return Values enable Functions to produce output based on the provided inputs, facilitating information flow within the code framework. By realizing the significance of Return Values, programmers can design Functions that generate specific outputs, enhancing code readability and logic flow. Exploring the realm of Return Values sheds light on the value of Function execution and output handling, enriching the learning journey of Python enthusiasts aspiring to master this versatile language.
Importing Modules
In the domain of Python development, the integration of external functionalities is achieved through Importing Modules. Standard Library Modules offer a repertoire of pre-built functionalities that cater to diverse programming requirements, saving developers time and effort in implementing common functionalities. Embracing Standard Library Modules amplifies code productivity and fosters a collaborative coding ecosystem, aligning with the ethos of efficiency and proficiency emphasized in this guide.
Creating Custom Modules
Complementing the utilization of Standard Library Modules, Creating Custom Modules empowers programmers to encapsulate specific functionalities tailored to project needs. Custom Modules afford developers the flexibility to encapsulate unique code components for reuse across projects, streamlining development workflows and promoting code reusability. Delving into the realm of Custom Modules unveils the art of modular coding and adaptive software design, equipping Python enthusiasts with the skills to create tailored solutions catered to diverse coding challenges in this comprehensive Python guide.
Advanced Concepts
In the realm of Python programming, delving into advanced concepts plays a pivotal role in expanding one's coding proficiency. Understanding these intricate topics sets the foundation for honing your skills to a higher level. The section on Advanced Concepts in this comprehensive guide is designed to unravel complex ideas and methodologies that are essential for mastering Python. By exploring advanced concepts, learners can grasp sophisticated programming techniques, enhance problem-solving abilities, and gain insights into optimizing code efficiency.
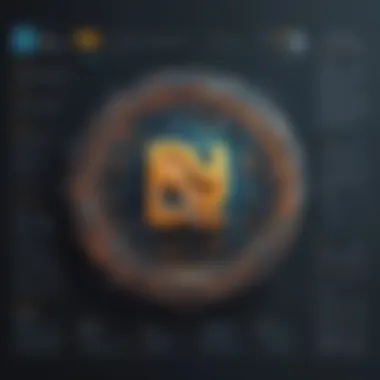
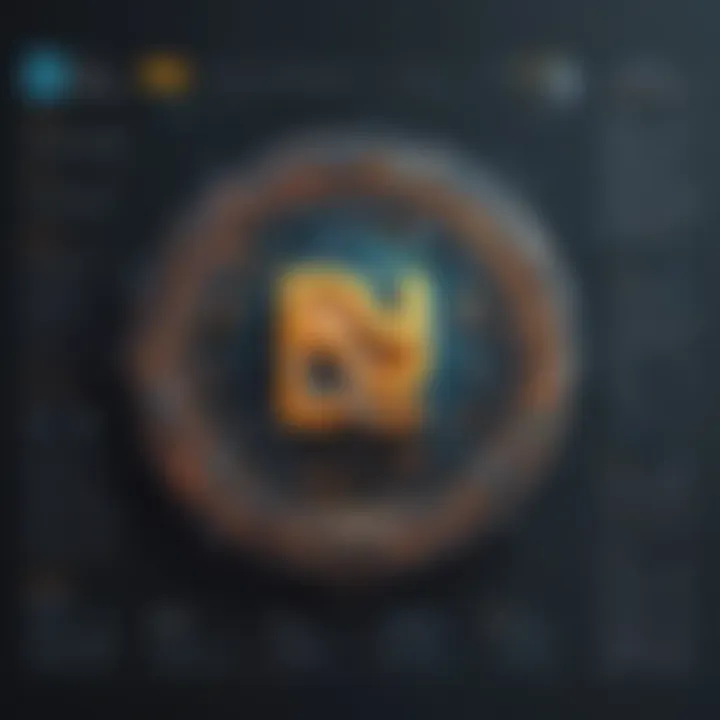
Object-Oriented Programming (OOP)
Classes and Objects
Classes and objects form the cornerstone of Object-Oriented Programming (OOP) in Python. Classes act as blueprints for creating objects, which are instances of classes holding attributes and methods. The encapsulation offered by classes enables better organization and reusability of code. Objects, on the other hand, represent tangible entities with specific behaviors and characteristics, allowing for a more structured approach to coding. The versatility of classes and objects in Python makes them indispensable in developing scalable and maintainable code structures. Despite the complexity that classes and objects may introduce, their modular nature enhances code readability and promotes effective problem-solving strategies.
Inheritance and Polymorphism
Inheritance and polymorphism are key components of OOP that further enrich Python programming. Inheritance enables the creation of new classes based on existing ones, fostering code reuse and minimizing redundancy. By inheriting attributes and methods from parent classes, developers can build upon existing functionalities and extend capabilities efficiently. Polymorphism, on the other hand, empowers objects to take on different forms based on context, promoting flexibility and simplifying complex implementations. The dynamic nature of polymorphism enhances code adaptability and extensibility, facilitating the construction of versatile and scalable applications in Python. While inheritance and polymorphism introduce robust mechanisms for code organization, they also bring forth challenges such as potential hierarchy complexities and increased maintenance efforts.
Exception Handling
Exception handling is a critical aspect of Python programming that allows developers to anticipate and manage errors effectively. By incorporating robust exception handling mechanisms, programmers can ensure the reliability and stability of their applications under diverse scenarios. The inclusion of exception handling in this guide underscores its significance in fortifying code resilience and mitigating unexpected failures.
Try-Except Blocks
Try-except blocks form the backbone of exception handling in Python, enabling programmers to differentiate normal code execution from error-handling routines. By encapsulating error-prone code within try blocks and defining corresponding exception handling in except blocks, developers can preemptively address potential issues and prevent program crashes. The structured approach offered by try-except blocks enhances code robustness, error traceability, and overall stability. However, overreliance on try-except blocks may lead to masking genuine errors and complicating debugging processes, necessitating a balanced implementation strategy.
Handling Multiple Exceptions
The ability to handle multiple exceptions exemplifies the flexibility and resilience of Python's exception handling capabilities. By specifying distinct handling mechanisms for various error scenarios, developers can tailor responses to specific exceptions, ensuring precise error management and streamlined program flow. Handling multiple exceptions fosters comprehensive error handling practices, improving code reliability and user experience. Nevertheless, managing an extensive array of exception types may introduce code verbosity and complexity, calling for meticulous design considerations and comprehensive exception hierarchies.
Practical Applications and Projects\n\nIn the realm of mastering Python, Practical Applications and Projects stand as pivotal concepts in solidifying one's understanding and skills. This segment delves into the hands-on aspect of Python programming, offering learners the opportunity to apply theoretical knowledge into real-world scenarios. By engaging with practical projects, individuals can enhance problem-solving skills, gain confidence in coding abilities, and foster a deeper understanding of Python's capabilities. The importance of Practical Applications and Projects lies in bridging the gap between theoretical concepts and practical implementation, paving the way for a comprehensive learning experience. It serves as a platform for learners to test their comprehension, experiment with different functionalities, and hone their coding prowess. \n\
Building a To-Do List Application\n\nDesign and Implementation: Within the context of this article, the Design and Implementation of a To-Do List Application showcases the structuring and integration of user-friendly interfaces, efficient data management, and seamless functionality. Emphasizing user experience and task organization, the design aspect focuses on creating an intuitive layout that enhances productivity and user interaction. Implementing various features such as task categorization, priority settings, and deadline monitoring elevates the application's utility and effectiveness. The key characteristic of this design lies in its simplicity and clarity, ensuring ease of use for individuals across different proficiency levels. While its advantage lies in its minimalist approach and straightforward navigation, a potential disadvantage could be limited customization options for advanced users in this article. \n\nAdding Functionality: Exploring the realm of Adding Functionality in the To-Do List Application illuminates the importance of dynamic task management, interactive features, and seamless integration of new functionalities. By incorporating features like task reminders, progress tracking, and collaboration options, the application's functionality is elevated to streamline task management processes effectively. The key characteristic of this functionality lies in its ability to enhance user efficiency, time management skills, and overall task tracking capabilities. Its advantageous nature stems from the simplification of task-related processes and the provision of insightful data on productivity levels. However, a likely drawback could be potential complexities arising from excessive features that may overwhelm users in this article. \n\n#### Analysis with Python\n\nData Extraction and Manipulation: Delving into Data Extraction and Manipulation in Python sheds light on the extraction of valuable insights from large datasets, data cleaning procedures, and structured data manipulation techniques. The key characteristic of this process lies in its ability to streamline data preprocessing tasks, ensure data accuracy, and facilitate seamless data transformations. Its beneficial nature is derived from its efficiency in handling vast amounts of data, automating repetitive tasks, and enhancing data quality. However, a potential downside could be the computational intensity required for processing extensive datasets and the learning curve associated with complex data manipulation methods in this article. \n\nVisualization Techniques: Exploring Visualization Techniques showcases the visual representation of data through graphs, charts, and interactive visuals to derive meaningful insights and communicate information effectively. The key characteristic of visualization lies in its ability to simplify complex data sets, identify trends, patterns, and outliers, and enhance data-driven decision-making processes. Its advantageous nature stems from its ability to present information in a digestible format, facilitate quick interpretation of data, and enhance overall data comprehension. However, a probable drawback could be the selection of inappropriate visualization types that may misrepresent data or lead to inaccurate interpretations within this article.
Conclusion
In the delineation of this detailed guide aiming at beginners to hone their Python prowess, the Conclusion segment stands as a crucial wrap-up, summarizing the key insights and learnings expounded throughout the article. It serves as the culminating point where readers can grasp the significance of the journey they undertook by closely following the comprehensive overview of Python programming. The Conclusion not only encapsulates the main takeaways but also instills a sense of accomplishment and consolidation of knowledge acquired. By revisiting the essential concepts, best practices, and practical exercises elucidated in the preceding sections, the Conclusion acts as a pivotal component in solidifying the foundational understanding required to excel in Python programming for both novices and individuals seeking to refine their coding skills.
Summary of Key Takeaways
Reinforcing Learning Concepts
Delving into the realm of Reinforcing Learning Concepts within the context of this enlightening guide, we encounter a paramount aspect that enhances the overall comprehension and retention of Python programming principles. This segment plays a pivotal role in fortifying the grasp on fundamental concepts, thereby fostering a robust foundation for further exploration and application. The inclusion of Reinforcing Learning Concepts in this article underscores its significance in consolidating theoretical knowledge into practical skills, ensuring a comprehensive understanding that transcends mere passive learning. The key characteristic of Reinforcing Learning Concepts lies in its ability to bridge the gap between theory and practice, enabling learners to internalize and apply Python programming principles effectively. This approach proves to be exceedingly beneficial for readers as it cultivates a deeper understanding of the intricacies of coding, propelling them towards mastery in Python programming. Emphasizing the unique feature of Reinforcing Learning Concepts, it emerges as a strategic tool that reinforces key ideas through hands-on exercises and targeted reinforcement, thereby heightening the efficacy and relevance of the learning experience. Despite the meticulous nature of this method, its benefits far outweigh any perceived drawbacks, as it enriches the learning process by fostering active engagement and long-term retention of Python programming concepts in the educational journey.