Unleashing the Power of Python Functions: A Programmer's Comprehensive Guide
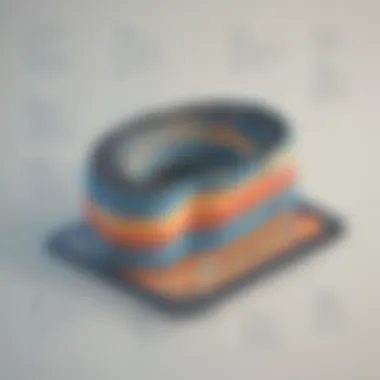
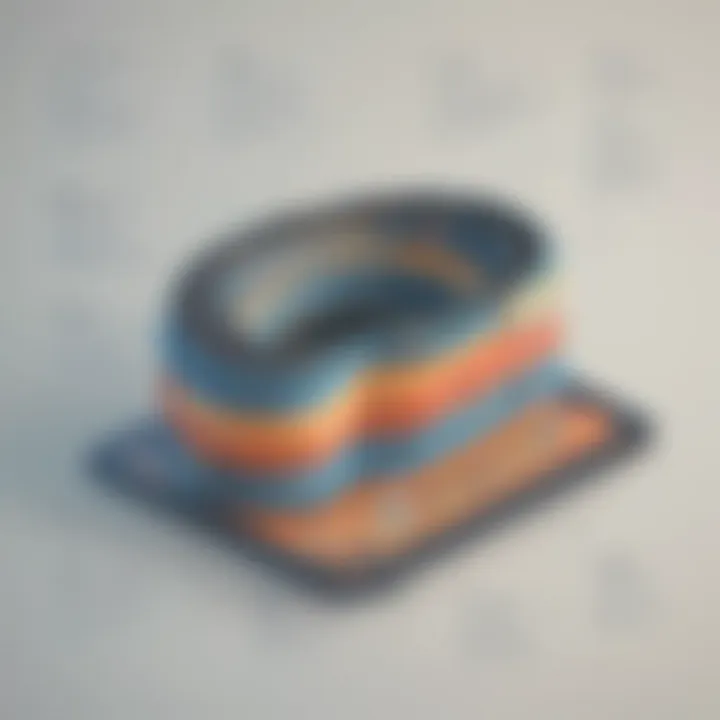
Coding Challenges
Python functions are fundamental building blocks in programming, enabling developers to encapsulate logic for reusability and maintainability. By delving into mastering Python functions, programmers can elevate their coding proficiency and problem-solving skills. Understanding the intricacies of function syntax and implementation is crucial for handling complex tasks effectively. This section will explore key concepts such as defining functions, passing arguments, and returning values, providing a solid foundation for readers to grasp and apply these principles in real-world projects.
From weekly coding challenges to problem solutions and explanations, this guide will offer practical examples and insights to sharpen your Python function skills. Tips and strategies for approaching coding challenges will be discussed to equip programmers with the knowledge and techniques needed to tackle various coding problems effectively. Additionally, community participation highlights will showcase how collaboration and knowledge-sharing among programmers can foster a supportive and enriching learning environment.
Technology Trends
In the ever-evolving landscape of technology, staying abreast of the latest trends and innovations is essential for tech enthusiasts and professionals. This section will delve into the technological trends that are shaping the industry, from emerging technologies to their impact on society. By exploring expert opinions and analysis, readers will gain valuable insights into the future direction of technology and how it influences different aspects of our lives.
As Python functions play a significant role in software development and automation, understanding how technology trends intersect with programming languages like Python is crucial. This section will examine the nuances of Python functions within the broader context of technological advancements, providing readers with a holistic perspective on how programming languages evolve in tandem with emerging technologies.
Coding Resources
To master Python functions effectively, having access to comprehensive coding resources is indispensable. This section will curate programming language guides, tools, and software reviews, as well as tutorials and how-to articles tailored to enhance readers' understanding of Python functions. By comparing online learning platforms and their offerings, aspiring and experienced programmers can identify the most suitable resources to bolster their Python skills and stay updated with the latest developments in the field.
Whether you are looking for in-depth tutorials on recursive functions or seeking recommendations for code refactoring tools, this section will provide a curated list of resources that cater to varying levels of expertise. From beginner-friendly guides to advanced programming techniques, readers can leverage these coding resources to expand their knowledge and proficiency in Python function optimization.
Computer Science Concepts
In the realm of computer science, a deep understanding of algorithms, data structures, and emerging technologies is paramount for navigating the complexities of modern software development. This section will offer primers on fundamental concepts such as algorithms and data structures, shedding light on their practical applications in Python function optimization.
Additionally, exploring artificial intelligence and machine learning basics within the context of Python functions will elucidate how these advanced technologies intersect with core programming principles. Networking and security fundamentals will also be discussed to underscore the importance of robust coding practices in safeguarding digital systems against potential threats.
As the landscape of technology continues to evolve, concepts like quantum computing and future technologies pose new challenges and opportunities for programmers. By delving into these cutting-edge topics and exploring their implications for Python function optimization, readers can gain valuable insights into the potential future directions of computing and how Python functions will adapt to meet changing computational paradigms.
Introduction to Python Functions
In this segment of the article, we will embark on a journey to unravel the significance of Python functions. Python functions serve as fundamental building blocks in programming, allowing developers to encapsulate specific functionalities for reusability and organizational purposes. Understanding the basics of Python functions is crucial for programmers at any proficiency level. By dissecting the core concepts of defining functions, handling function arguments, and utilizing return statements, individuals can enhance their coding efficiency and maintain clean, structured code.
Understanding the Fundamentals
Defining Functions
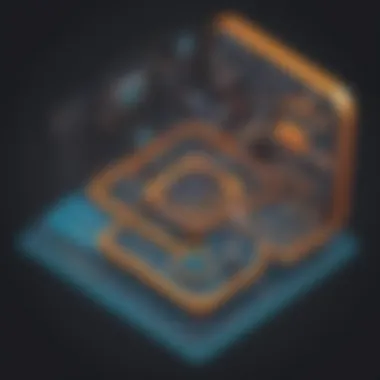
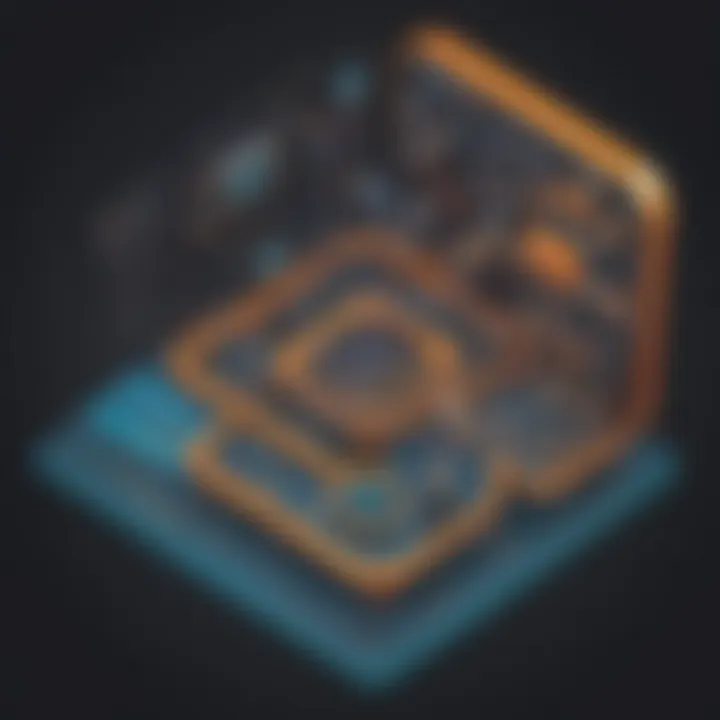
Delving into the realm of defining functions illuminates the process of declaring a named block of code responsible for carrying out a specific task. This fundamental aspect of Python function emphasizes modularity and abstraction, enabling developers to break down complex operations into manageable and coherent segments. The ability to define functions not only promotes code reusability but also enhances code organization, promoting a structured approach to software development. While defining functions offers flexibility and scalability in code implementation, it is essential to consider the trade-offs between code modularity and potential performance impacts in large-scale projects.
Function Arguments
Exploring function arguments delves into the mechanism through which inputs are passed to a function for processing. Function arguments play a pivotal role in customizing the behavior of functions based on varying input parameters. By understanding different argument types such as positional, keyword, and default arguments, developers can create versatile functions that cater to diverse use cases. Leveraging function arguments facilitates code flexibility and adaptability, allowing for dynamic function behavior based on the provided inputs. However, the misuse or overcomplication of function arguments can lead to code readability issues and potential maintenance challenges in intricate software architectures.
Return Statement
The return statement signifies the culmination of a function's execution, as it specifies the value to be returned to the function call. This critical component of Python functions enables functions to produce output or results based on the processing logic defined within the function body. By employing return statements effectively, developers can convey computed results, status indicators, or data structures back to the calling code segment. The return statement plays a pivotal role in function output management and result propagation, influencing the overall functionality and interactivity of Python applications. However, improper usage of return statements may result in unexpected behavior or logical errors, underscoring the importance of accurate result handling in function design.
Advanced Function Concepts
In the realm of Python programming, delving into advanced function concepts is paramount to expand one's knowledge and skill set. This section serves as a cornerstone in our comprehensive guide, offering a deep dive into intricate elements that elevate coding proficiency. By focusing on topics like function parameters, scope, and decorators, programmers can gain a profound understanding of Python's capabilities and nuances, enabling them to write more efficient and robust code. Mastery of advanced function concepts opens doors to complex problem-solving strategies and sets the stage for coding elegance and efficiency.
Function Parameters
Default Parameters
In the context of this article, default parameters play a pivotal role in enhancing the flexibility and usability of functions. By allowing functions to have predefined values for certain parameters, default parameters streamline the coding process and offer a level of customizability that is invaluable in practical coding scenarios. The key characteristic of default parameters lies in their ability to provide default values unless explicitly overridden, simplifying function calls and enhancing code readability. While default parameters simplify coding, it is crucial to use them judiciously to avoid potential confusion and unintended consequences.
Keyword Arguments
When exploring function parameters, keyword arguments emerge as a powerful tool in Python programming. By enabling programmers to pass arguments to functions using parameter names, rather than relying on positional order, keyword arguments enhance code clarity and maintainability. The distinguishing feature of keyword arguments lies in the ability to specify values for specific parameters, regardless of their order in the function signature. This characteristic not only enhances code readability but also simplifies debugging and maintenance, making keyword arguments a popular choice for enhancing code robustness and flexibility.
Variable-Length Arguments
Another noteworthy aspect of function parameters is the utilization of variable-length arguments, catering to situations where functions need to handle an arbitrary number of arguments. By employing variable-length arguments such as *args and **kwargs, programmers can create versatile functions that accommodate varying input sizes without the need to define a fixed number of parameters. The key characteristic of variable-length arguments is their adaptability to different data structures and sizes, offering developers a convenient way to work with dynamic inputs. While variable-length arguments provide flexibility, it is essential to use them judiciously to maintain code clarity and avoid potential complexities.
Scope and Lifetime of Variables
Global vs. Local Scope
Regarding the scope and lifetime of variables, understanding the distinction between global and local scopes is crucial for writing sound and reliable code. Global variables are accessible throughout the program, while local variables are confined to specific blocks or functions, promoting code organization and mitigating naming conflicts. The key characteristic of global vs. local scope lies in their unique visibility within different parts of the code, influencing variable accessibility and potential conflicts. While global variables offer broad accessibility, they can introduce pollution and unintended side effects, necessitating careful management to maintain code integrity.
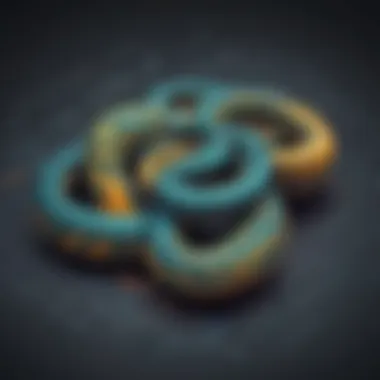
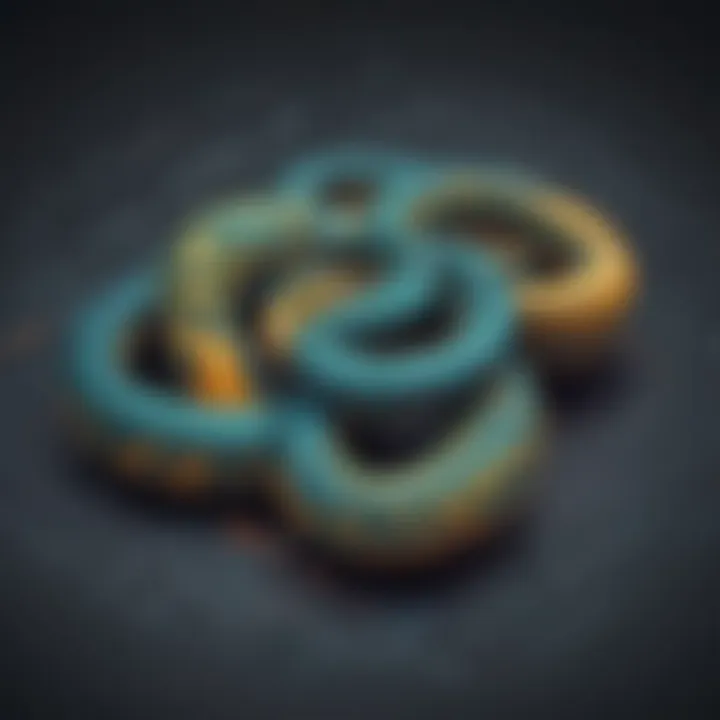
Variable Access
In the realm of variable access, the ability to retrieve and manipulate variables across different scopes plays a critical role in programming versatility and efficiency. Python's flexible scoping rules allow for both accessing variables defined in outer scopes and modifying variables at different levels of nesting. The key characteristic of variable access lies in its impact on code modularity and data flow, enabling developers to create more concise and readable code structures. By understanding and leveraging variable access effectively, programmers can enhance code reusability and maintainability, leading to more robust and adaptable software solutions.
Decorators and Closures
Using Decorators
Exploring the realm of decorators unveils a powerful mechanism in Python that enhances function behavior and modularity. Decorators enable programmers to extend or modify the behavior of functions without directly altering their code, promoting code reusability and maintainability. The key characteristic of using decorators lies in their ability to add functionality to existing functions dynamically, offering a clean and elegant solution to common programming challenges. By leveraging decorators, developers can encapsulate common functionalities and apply them consistently across multiple functions, reducing redundancy and enhancing code efficiency.
Closure Functions
In the context of Python programming, closure functions embody a unique feature that promotes encapsulation and data privacy within functions. Closures allow functions to retain references to their enclosing scope's variables, even after the scope has finished execution, enabling a form of data hiding and protection. The key characteristic of closure functions lies in their ability to create self-contained units of functionality with access to outer scope variables, enhancing code encapsulation and security. By harnessing closure functions, programmers can achieve better control over variable scope and visibility, leading to more robust and maintainable code structures.
Best Practices for Function Design
When delving into the realm of Python functions, understanding the significance of best practices for function design is paramount. It serves as the cornerstone for creating efficient and reliable code structures. By adhering to best practices, programmers can enhance code maintainability, scalability, and readability. Emphasizing clear and concise coding methods, best practices ensure that functions are structured logically, making the codebase easier to comprehend and modify. Furthermore, incorporating best practices facilitates collaboration among team members, streamlining the development process and reducing errors.
Writing Efficient Functions
Code Readability
Code readability plays a pivotal role in the overall functionality and comprehension of Python code. When code is readable, it is easier to debug, maintain, and extend, leading to enhanced productivity and code quality. The key characteristic of code readability lies in its clarity and organization, enabling programmers to quickly grasp the purpose and flow of the code. In this article, highlighting the importance of code readability underscores its role in fostering effective communication and collaboration among developers. Despite its advantages, achieving optimal code readability might entail some trade-offs, such as potential increases in code length to ensure clarity and understanding.
Modular Design
Modular design, another critical aspect of function design, focuses on breaking down complex functionalities into smaller, independent modules. This approach promotes reusability, testability, and scalability in software development. The key characteristic of modular design is its ability to compartmentalize code into distinct units, each serving a specific function or feature. In the context of this article, modular design emerges as a popular choice due to its capacity to enhance code organization and maintainability. However, while modular design simplifies code maintenance and updates, it may introduce additional complexity when defining module interactions and dependencies.
Error Handling in Functions
Exception Handling
Exception handling stands out as a fundamental aspect of writing robust and resilient Python functions. It allows programmers to gracefully manage errors and prevent application crashes, improving overall system reliability and user experience. The key characteristic of exception handling lies in its capacity to separate error-handling code from regular function logic, enhancing code clarity and fault tolerance. In the context of this article, highlighting the benefits of exception handling underscores its critical role in safeguarding program execution and mitigating unexpected failures. Despite its advantages, implementing exception handling may introduce additional code complexity and potentially obscure the main flow of functions.
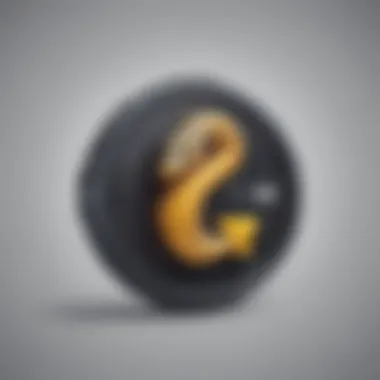
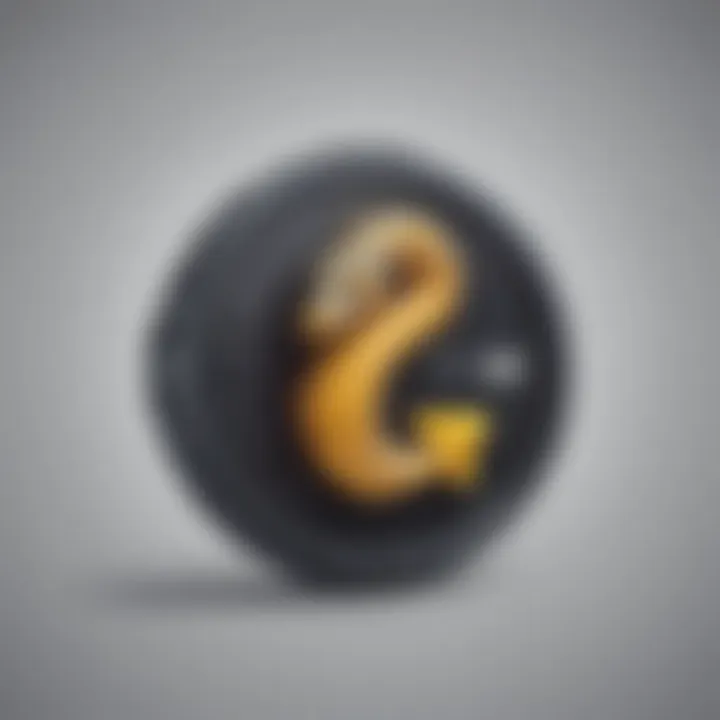
Try-Except Blocks
Try-except blocks offer a structured approach to handling exceptions in Python functions, enabling developers to anticipate and manage potential runtime errors effectively. By encapsulating risky code within a try block and defining specific error-handling procedures in except blocks, programmers can ensure smooth error recovery and fault isolation. The key characteristic of try-except blocks is their capability to identify and address errors without disrupting the program execution flow. In the scope of this article, emphasizing the utility of try-except blocks emphasizes their role in promoting code stability and fault resilience. Nonetheless, extensive use of try-except blocks may obscure underlying issues and complicate troubleshooting efforts.
Practical Applications of Python Functions
In the realm of Python programming, the section on Practical Applications of Python Functions serves as a pivotal cornerstone in this comprehensive guide. By delving into functional programming concepts, programmers can harness the power of functions to streamline their code, enhance clarity, and improve efficiency. Understanding how to implement functional programming paradigms like Map, Filter, and Reduce can revolutionize how programmers approach problem-solving. With these tools at their disposal, developers can manipulate data structures with ease, apply operations across collections, and reduce complex processes into concise, elegant solutions. Embracing practical applications empowers programmers to write cleaner, more maintainable code, ultimately leading to enhanced productivity and code quality.
Functional Programming Concepts
Map, Filter, Reduce
The exploration of Map, Filter, and Reduce within functional programming concepts is crucial in this discourse on Python functions. These higher-order functions enable a declarative style of programming, allowing developers to express operations concisely while iterating through sequences. Map transforms each element using a defined function, Filter selects elements based on specified criteria, and Reduce aggregates elements into a single value. The elegance of Map lies in its ability to apply a transformation uniformly to every element in a collection, simplifying repetitive tasks and enhancing code readability. Similarly, Filter provides a convenient mechanism for selectively extracting elements that meet prescribed conditions, offering flexibility in data manipulation. On the other hand, Reduce consolidates elements iteratively, facilitating the computation of cumulated results. Understanding the nuances of Map, Filter, and Reduce equips programmers with versatile tools for data processing, enabling scalable and efficient solutions within Python applications.
List Comprehensions
A fundamental aspect of Python programming, List Comprehensions contribute significantly to the overarching topic of practical applications. List Comprehensions offer a concise and expressive way to create lists by iterating over existing sequences and applying transformations or filtering conditions in a single line of code. This feature not only simplifies the syntax but also enhances the readability of code, making it more compact and intuitive. By leveraging List Comprehensions, programmers can generate lists efficiently, avoiding the verbosity of traditional loops and conditional statements. The unique feature of List Comprehensions lies in their ability to condense complex iterative operations into a succinct format, promoting code efficiency and maintainability. While List Comprehensions offer a streamlined approach to list generation, it is essential for programmers to strike a balance between brevity and readability, ensuring that code remains comprehensible and idiomatic.
Recursive Functions in Action
Recursive Factorial
Within the realm of recursive functions, Recursive Factorial emerges as a fundamental concept with immense value in this narrative on Python functions. By exploring Recursive Factorial, programmers can grasp the essence of recursive logic and its application in solving complex computational problems. Recursive Factorial exemplifies the power of self-reference, where a function calls itself to break down a task into simpler sub-problems until reaching a base case. This recursive approach offers an elegant solution for factorial calculations, showcasing the beauty of iterative decomposition in algorithm design. The key characteristic of Recursive Factorial lies in its ability to handle repetitive tasks efficiently, enabling the computation of factorials for large numbers with minimal code complexity. While Recursive Factorial embodies elegance and conciseness, it is essential for programmers to understand the underlying principles of recursion to avoid potential pitfalls such as infinite loops.
Binary Search
In the landscape of efficient search algorithms, Binary Search emerges as a cornerstone of algorithmic efficiency intertwined with practical applications in Python functions. The essence of Binary Search lies in its divide-and-conquer strategy, where it repeatedly divides the search interval in half until the target element is found or the interval is empty. This binary partitioning enables Binary Search to locate elements swiftly within sorted collections, showcasing logarithmic time complexity for search operations. The key characteristic of Binary Search stems from its efficiency in handling large datasets, offering a significantly faster search process compared to linear search algorithms. While Binary Search excels in speed and scalability, programmers must ensure that the underlying data structures are sorted and accessible for optimal search performance. By mastering Binary Search within the realm of Python functions, programmers can unlock the potential for rapid and reliable search operations, augmenting the efficiency and effectiveness of their code.
Optimizing Function Performance
In the realm of Python programming, optimizing function performance stands as a pivotal element that can elevate one's coding expertise. Efficiency is the cornerstone where intricate algorithms meet execution speed, ultimately defining the success of software applications. Embracing the essence of optimizing function performance not only streamlines code processes but also enhances the overall user experience. By delving into this facet, programmers gain a profound understanding of how to fine-tune their functions to deliver optimal results. Whether it's reducing time complexity or minimizing resource usage, the focus on function performance optimization underscores the commitment to excellence in software development.
Time Complexity Analysis
Big O Notation
When deciphering the intricacies of time complexity analysis, Big O Notation emerges as a beacon of clarity in quantifying algorithmic efficiency. Its fundamental role lies in providing a standardized notation to express the upper bound of an algorithm's performance in the worst-case scenario. The allure of Big O Notation stems from its ability to encapsulate complex algorithms into simplified, scalable representations, enabling programmers to compare and contrast various solutions with precision. It serves as a compass guiding developers towards efficient coding practices, fostering algorithmic design that is both elegant and effective. While its simplicity is a key strength, understanding its intricacies empowers programmers to make informed decisions when optimizing function performance.
Efficient Algorithms
Delving deeper into the realm of optimizing function performance, efficient algorithms take center stage as the driving force behind computational optimization. These algorithms epitomize elegance and speed, unraveling a realm where complexity meets simplicity in pursuit of optimal solutions. The crux of efficient algorithms lies in their ability to mitigate resource wastage and computational overhead, paving the way for streamlined code execution and enhanced program efficiency. By integrating efficient algorithms into function optimization strategies, programmers catapult their code to unprecedented levels of performance, turning intricate processes into seamless operations. The strategic implementation of efficient algorithms not only refines code execution but also fosters a mindset of continuous improvement, propelling developers towards mastery in Python function optimization.