Unveiling the Art of Crafting Effective Unit Tests in Python
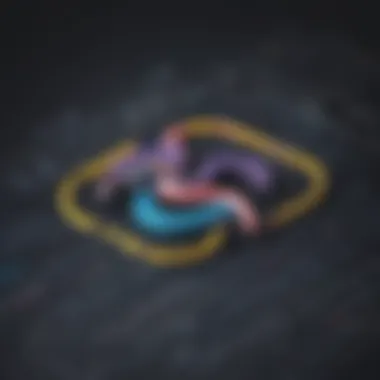
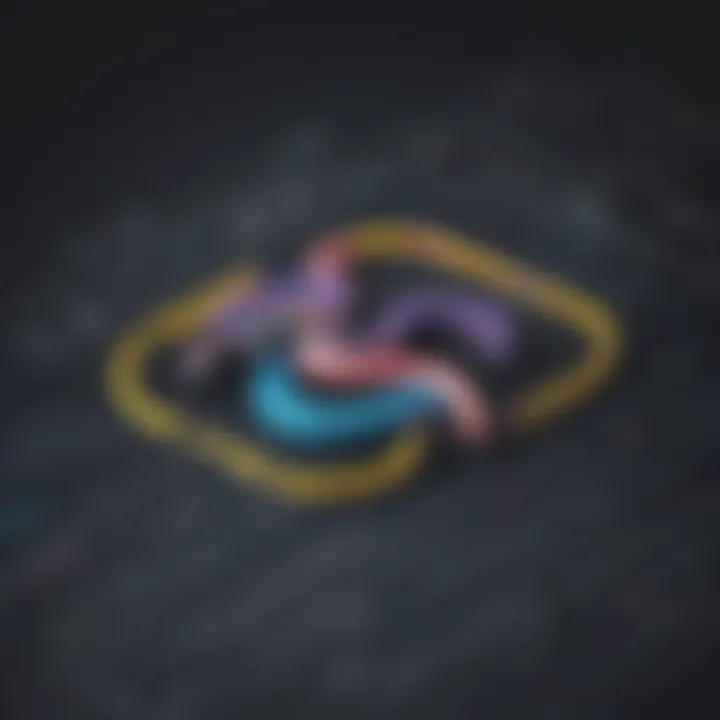
Coding Challenges
To truly master unit test writing in Python, one must embrace the world of coding challenges. These challenges serve as invaluable practice grounds, refining programming skills and problem-solving abilities. Engaging in weekly coding challenges sharpens the mind, presenting unique and diverse problems to tackle. By delving into various problem solutions and explanations, individuals can dissect different approaches, enhancing their understanding of efficient and elegant coding practices. Moreover, discovering tips and strategies specific to coding challenges provides a competitive edge, allowing enthusiasts to excel in their coding endeavors. Community participation highlights the collaborative nature of coding challenges, fostering a sense of camaraderie and shared learning among like-minded individuals.
Technology Trends
Understanding the latest technology trends is paramount for staying ahead in the ever-evolving realm of software development. By exploring the landscape of technological innovations, individuals gain insight into emerging technologies that are shaping the industry. Keeping a pulse on these advancements not only informs one's current practices but also paves the way for future exploration. Delving into how technology impacts society offers a holistic perspective, showcasing the far-reaching effects of technological integration. Expert opinions and analyses provide nuanced viewpoints, guiding professionals in navigating the intersection of technology and society.
Coding Resources
Computer Science Concepts
A solid foundation in computer science concepts bolsters one's proficiency in unit test writing in Python. Exploring algorithms and data structures primes individuals with vital problem-solving frameworks, essential for designing effective test cases. Delving into artificial intelligence and machine learning basics expands horizons, showcasing the intersection of cutting-edge technologies with testing practices. Understanding networking and security fundamentals is paramount for safeguarding testing environments and ensuring robustness. Exploring futuristic domains like quantum computing offers a glimpse into potential testing methodologies, anticipating future technologies and their impact on software testing.
Introduction
Understanding the fundamental concepts of unit testing is paramount before delving into its intricacies further. It serves as the first line of defense against potential bugs and defects, thus improving code quality and reliability. Embracing unit testing in Python empowers developers to streamline the debugging process and verify the correctness of their functions systematically.
Furthermore, delving into the benefits of unit testing sheds light on its significance within the programming domain. By accentuating the importance of code stability and dependability, unit tests promote a culture of accountability and precision in software development. Developers are compelled to adopt best practices, thereby fostering a conducive environment for collaboration and innovation.
The introduction sets the stage for an in-depth exploration of unit testing in Python, signifying its pivotal role in enhancing software quality and accelerating the development lifecycle. By elucidating key concepts and principles, this article aims to equip aspiring programmers with the necessary tools to elevate their testing proficiency. Mastering unit test writing transcends mere technical competence; it cultivates a mindset of meticulousness and foresight, essential in navigating the complexities of modern software engineering.
Understanding Unit Testing
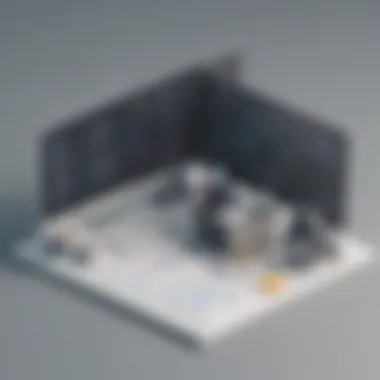
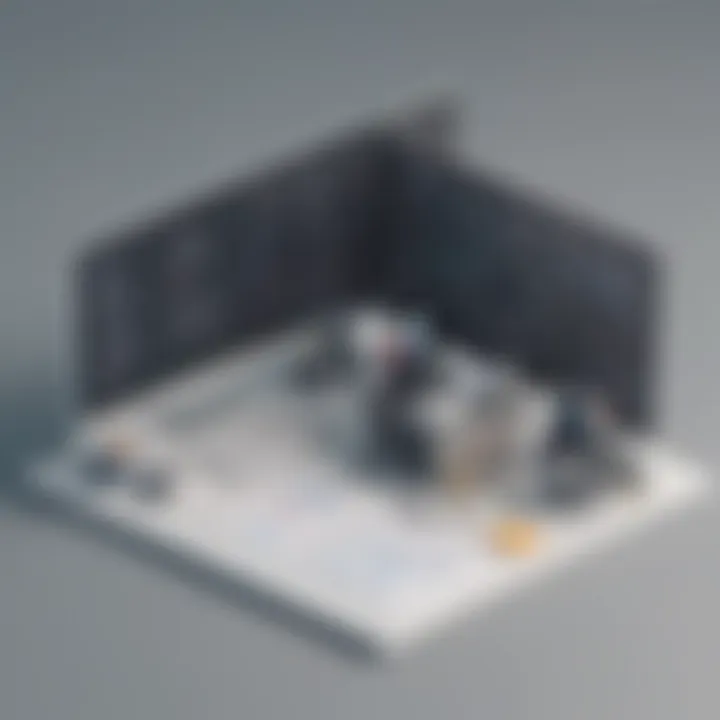
In the realm of software development, understanding unit testing holds paramount significance. It serves as the foundation upon which robust and reliable code stands. By dissecting the essence of unit testing, programmers can unravel the intricacies of their codebase, ensuring each component functions as intended. This section delves into the core principles that underpin unit testing, emphasizing the necessity of this practice in fostering quality software. Through a meticulous exploration of unit testing concepts, developers can hone their testing acumen, elevating the overall integrity of their programs.
What is Unit Testing?
Unit testing, at its core, involves the practice of isolating individual units or components of code and subjecting them to a series of tests to validate their functionality. This granular approach allows developers to detect errors or bugs within specific segments of code, facilitating targeted debugging and problem resolution. By encapsulating code functionality within discrete units, programmers can verify its correctness independent of other program elements, paving the way for streamlined troubleshooting and enhanced code maintainability.
Benefits of Unit Testing
The merits of unit testing resonate across the software development landscape, offering a multitude of advantages to discerning programmers. Firstly, unit tests serve as a protective shield, fortifying code against regressions and unintended side effects. Through comprehensive test coverage, developers can confidently refactor code, knowing that existing functionality remains intact. Additionally, unit testing promotes code reliability by identifying issues early in the development cycle, reducing the likelihood of critical bugs surfacing in production. Furthermore, these tests enhance code documentation, providing living examples that elucidate intended program behavior and usage.
Common Unit Testing Frameworks in Python
Python, renowned for its simplicity and versatility, boasts a rich ecosystem of unit testing frameworks that cater to varying developer preferences. Among the popular frameworks are unittest, pytest, and doctest, each offering unique features to streamline the testing process. Unittest, Python's built-in framework, provides an xUnit-style testing experience, fostering the creation of well-structured test suites. On the other hand, pytest embraces simplicity and extensibility, enabling developers to write expressive tests with minimal boilerplate code. Notably, doctest diverges from traditional testing paradigms, leveraging docstrings to execute test cases directly from code examples. By exploring these frameworks, developers can harness the power of Python's testing ecosystem to optimize their unit testing endeavors.
Getting Started with Unit Testing in Python
Unit testing in Python is a fundamental aspect of software development, ensuring the stability and functionality of code. Getting started with unit testing sets the foundation for robust programs. It involves creating small, targeted tests for specific components or units of code. Implementing unit testing at the beginning of a project can detect bugs early, making them easier and less costly to fix. By automating the testing process, developers can verify their code's correctness continuously. This section explores the essential steps to kickstart your unit testing journey in Python, emphasizing its significance in enhancing code quality and minimizing errors.
Setting Up Your Environment
Before writing unit tests in Python, it's crucial to set up the testing environment. This includes installing a unit testing framework like 'unittest' or 'pytest,' which provides tools for test discovery, organization, and execution. Additionally, configuring a virtual environment for testing ensures that tests are isolated from the main project environment, preventing interference between dependencies. Establishing a robust testing environment enhances productivity, allowing developers to run tests seamlessly and effectively track their project's test coverage.
Writing Your First Unit Test
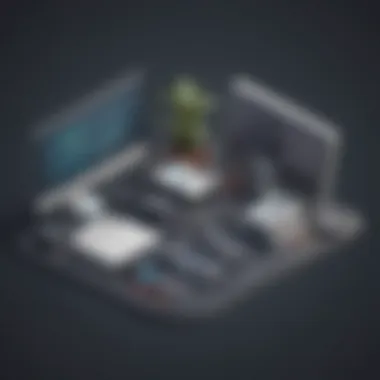
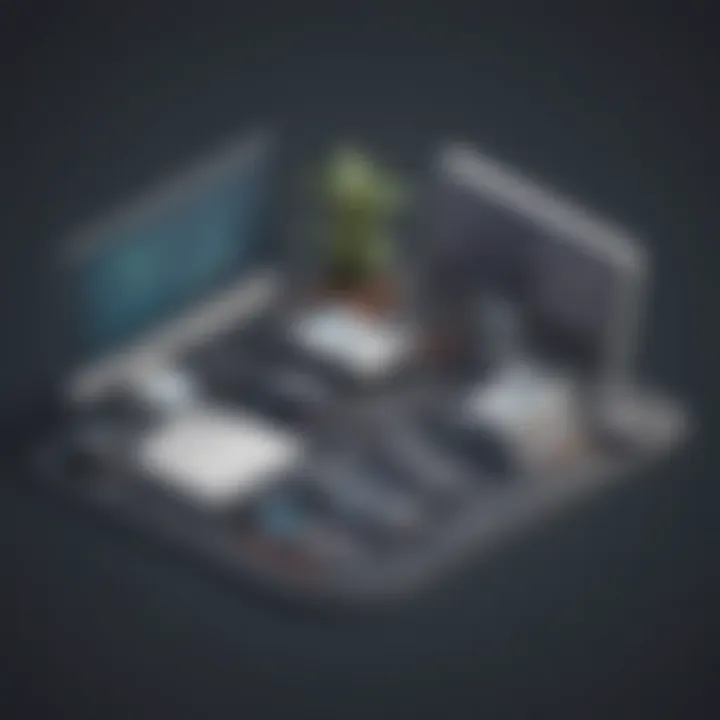
Crafting your first unit test in Python involves creating a test case to verify the functionality of a specific code unit. A test case typically comprises of setup, execution, and assertion phases to confirm expected outcomes. When writing unit tests, developers must focus on testing individual functions or methods in isolation, ensuring that each test targets a distinct functionality. Incorporating descriptive test method names and clear assertions enhances test readability and aids in quick issue identification. Writing effective unit tests from the outset cultivates a testing culture within the development process, promoting code quality and reliability.
Running Unit Tests
Once unit tests are written, running them is essential to validate code behavior and detect inconsistencies. Python offers tools like test runners that execute all tests within a designated directory or module. Running unit tests triggers assertions, indicating whether the code passed or failed the test cases. Continuous testing integration tools can automatically run unit tests upon code changes, facilitating early bug detection and swift issue resolution. By regularly running unit tests, developers can maintain code integrity and ensure that new modifications do not inadvertently break existing functionalities.
Best Practices for Writing Effective Unit Tests
In the realm of software development, mastering the art of writing effective unit tests is crucial for ensuring the reliability and robustness of code. Best practices play a pivotal role in this domain as they set the standards for test creation, execution, and maintenance. By adhering to best practices, developers can streamline the testing process, identify defects early, and improve the overall quality of software products. Emphasizing best practices for writing unit tests underscores the significance of consistency, clarity, and efficiency in testing procedures. It involves establishing clear guidelines for test design, execution, and documentation, ensuring that tests remain accurate, reliable, and easy to interpret.
Isolation and Independence
One of the fundamental principles in unit testing is the concept of isolation and independence. This principle underscores the importance of testing individual components in isolation without relying on external dependencies. By isolating components, developers can evaluate their functionality independently, making it easier to pinpoint defects and ensure that each unit functions as intended. Independence, on the other hand, refers to the ability of tests to run autonomously without being influenced by other tests or external factors. By adhering to the principles of isolation and independence, developers can create test suites that are self-contained, predictable, and straightforward to maintain.
Test Readability and Maintainability
Test readability and maintainability are essential aspects of effective unit testing. Readable tests are easy to understand and interpret, making it simpler for developers to identify test scenarios and expected outcomes. Maintaining clear and concise tests improves code comprehensibility and accelerates debugging processes. Additionally, readable tests contribute to overall software quality by ensuring that testing logic is coherent and logical. On the other hand, maintainable tests are easy to update and modify as code evolves. By prioritizing test readability and maintainability, developers can enhance the efficiency and effectiveness of their testing practices.
Mocking and Patching
Mocking and patching are vital techniques in unit testing that enable developers to simulate the behavior of external dependencies and focus on testing individual units in isolation. Mocking involves creating mock objects that mimic the behavior of real objects, allowing developers to control the responses of external components during testing. Patching, on the other hand, involves modifying attributes or behaviors of objects during test execution to isolate units for testing purposes. By leveraging mocking and patching techniques, developers can create comprehensive test suites that accurately evaluate the functionality of individual units while minimizing dependencies on external systems.
Advanced Unit Testing Techniques
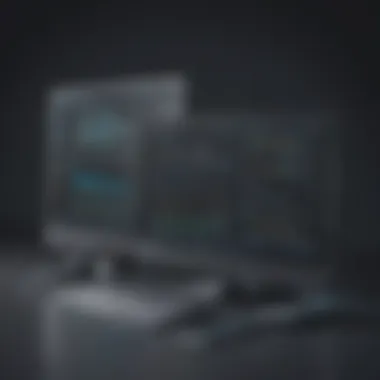
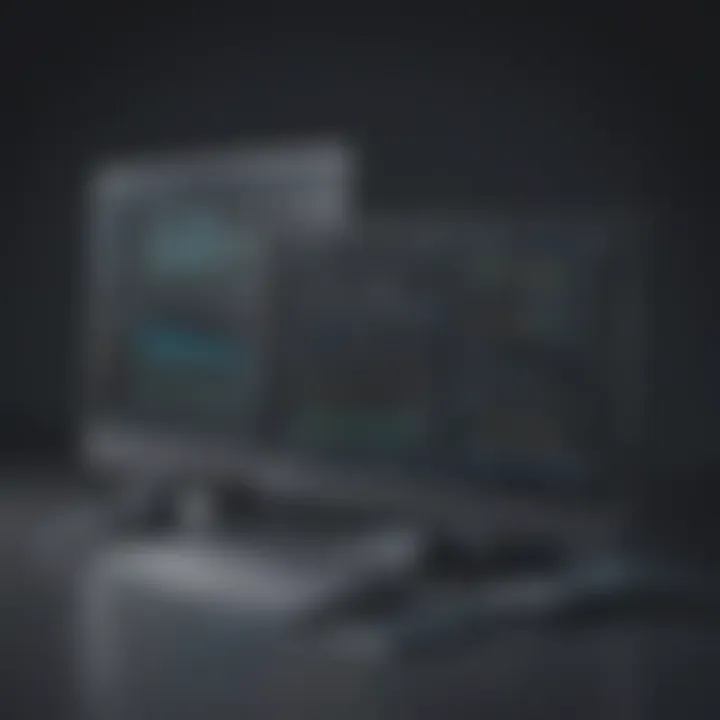
In the realm of software development, mastering advanced unit testing techniques stands as a pivotal skill set for programmers looking to elevate their testing practices in Python. Embracing advanced techniques goes beyond the conventional boundaries of unit testing, delving deeper into comprehensive methodologies that enhance the resilience and effectiveness of test suites. Understanding the intricacies of parameterized tests, test fixtures, and test doubles (mocks, stubs, and spies) equips developers with the tools to create more robust and adaptable unit tests.
Parameterized Tests
Parameterized tests offer a strategic approach to test programming logic with multiple inputs. By dynamically generating test cases based on parameters, programmers can efficiently assess the behavior of functions under various conditions, enhancing test coverage and accuracy. This technique proves valuable in scenarios where validating different inputs against expected outcomes is required, streamlining the testing process and promoting code efficiency.
Test Fixtures
Test fixtures play a fundamental role in establishing a consistent and reliable testing environment. By setting up preconditions and postconditions for test cases, developers ensure a standardized testing context, reducing the likelihood of variable influences on test outcomes. Test fixtures aid in simplifying test case setup, teardown, and cleanup procedures, fostering a structured approach to unit testing that enhances repeatability and clarity in test results.
Test Doubles: Mocks, Stubs, and Spies
Test doubles, including mocks, stubs, and spies, offer versatile mechanisms for emulating external dependencies within unit tests. These components enable developers to isolate the unit under test by simulating the behavior of collaborating modules, database interactions, or network requests. Mocks provide pre-defined responses, stubs return specific outputs, while spies enable monitoring of interactions between the unit test and external components. Leveraging test doubles enhances test isolation, reduces dependencies on external resources, and facilitates focused unit testing geared towards comprehensive code coverage and robustness.
Common Pitfalls and How to Avoid Them
In the realm of unit testing in Python, understanding common pitfalls and how to circumvent them is paramount to the efficacy of the testing process. Identifying and addressing these pitfalls can significantly enhance the reliability and robustness of your test suite. One prevalent pitfall is Testing Implementation Details. Falling into this trap involves testing the internal workings of the code rather than focusing on the expected behavior of the units. This can lead to fragile tests that break easily with any minor code change, hampering the maintenance of the codebase. By shifting the focus to testing outcomes rather than implementation specifics, one can create more resilient and valuable unit tests.
Another common pitfall to navigate is Writing Unreliable Tests. Such tests introduce uncertainties and inconsistencies, rendering the testing results unreliable. Factors like excessive dependencies, lack of clear test objectives, or inadequate coverage can contribute to the unreliability of tests. To mitigate this issue, it is crucial to establish clear testing goals, minimize dependencies by using mocks or stubs where necessary, and ensure comprehensive test coverage to boost test reliability.
Lastly, Neglecting Edge Cases poses a significant risk in unit testing. Overlooking edge cases, such as boundary conditions or error scenarios, can result in undetected bugs that may surface in production environments. Vigilance in considering all possible scenarios, including edge cases, ensures thorough test coverage and, subsequently, robust software performance. Prioritizing the identification and incorporation of edge cases into your unit tests fortifies the quality and effectiveness of the testing process.
Conclusion
Unit testing, despite its apparent simplicity at the surface, plays a pivotal role in the software development lifecycle. It serves as the bedrock for integrating quality assurance practices seamlessly within the development process. By meticulously validating the smallest units of code in isolation, developers can confidently ensure their functionality within the broader system architecture.
Moreover, the essence of unit testing extends beyond mere error detection. It fosters a proactive approach to code quality by instilling confidence in the codebase's correctness, enabling rapid iteration and refactoring without the fear of unintended consequences. Additionally, comprehensive unit test suites act as living documentation, offering insights into the expected behavior of functions and modules, facilitating code maintenance and collaboration among team members.
Embracing unit testing is akin to cultivating a culture of quality within the development team. It encourages precision, attention to detail, and a mindset oriented towards building resilient software solutions. Through thorough unit testing practices, developers can mitigate the ripple effects of changes, streamline debugging efforts, and ultimately deliver superior software products that meet stringent quality benchmarks.
In essence, mastering unit test writing transcends the realm of technical proficiency; it embodies a philosophical shift towards fostering software quality through meticulous validation and continuous improvement. By internalizing the principles and best practices outlined in this guide, aspiring programmers and seasoned developers alike can elevate their craft, fortifying their codebases against the pitfalls of bugs and regressions, ultimately paving the way for sustainable software systems that stand the test of time.