Integrating MongoDB with Spring Framework: A Comprehensive Guide
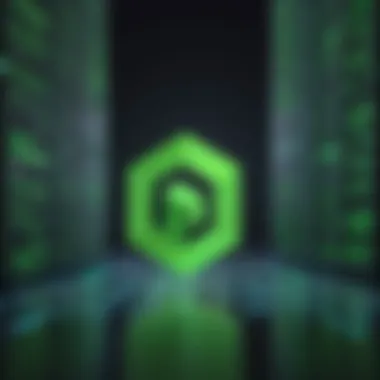
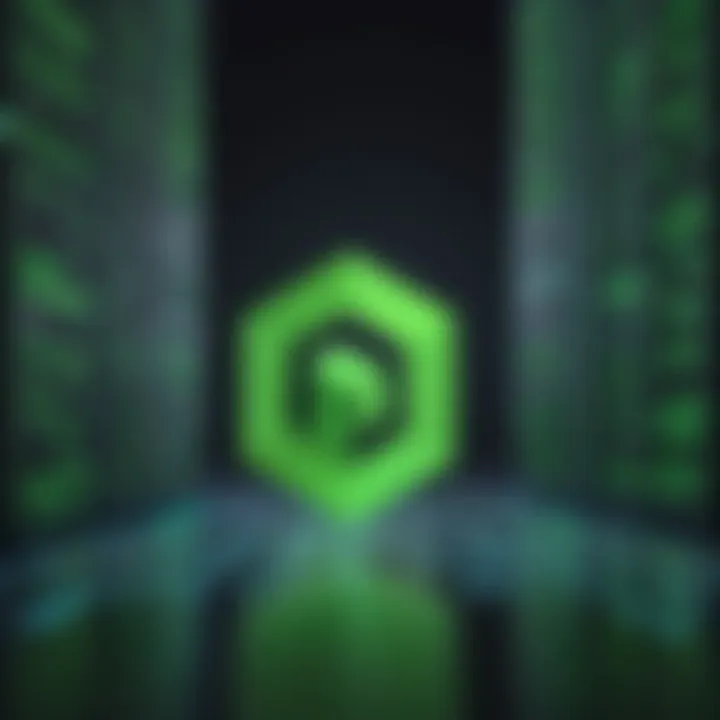
Intro
Integrating MongoDB with the Spring Framework is a relevant topic today. As software development evolves, understanding how NoSQL databases like MongoDB function within frameworks like Spring is essential. This guide aims to break down the integration process, providing details and relevant strategies that cater to both aspiring and experienced developers.
MongoDB offers a schema-less data model, making it ideal for handling unstructured data. On the other hand, Spring Framework streamlines the development process for Java applications. Combining these two technologies allows developers to build agile and efficient applications.
In this article, we will cover the basics of MongoDB and Spring Framework, their individual strengths, and how they complement each other. Additionally, we will delve into real-world application scenarios, tips for effective implementation, and performance considerations.
Coding Challenges
Integrating MongoDB with Spring Framework presents various challenges that developers may encounter. These challenges range from configuring dependencies to managing data effectively. Below are some coding challenges that developers might face:
- Dependency Management: Ensuring that the correct MongoDB driver and Spring Data MongoDB dependencies are included in the project is crucial. Using Maven or Gradle for managing these dependencies can simplify the process.
- Data Mapping Issues: Understanding how to map MongoDB documents to Java objects is key. Utilizing Spring Data's repository support can alleviate this issue but requires knowledge of annotations.
Weekly Coding Challenges
Developers are encouraged to engage in weekly coding challenges to sharpen their skills. These challenges may involve designing schemas or writing queries in MongoDB, then integrating that with Spring to retrieve or store data. Tackling these tasks can build confidence and proficiency.
Problem Solutions and Explanations
For each identified coding challenge, itâs important to provide solutions that illuminate the path toward successful integration. For example, when facing data mapping issues, developers can utilize Spring's annotation to specify the collection and fields.
Tips and Strategies for Coding Challenges
- Always start with a clear understanding of the data model in MongoDB.
- Break down tasks into smaller, manageable components.
- Utilize online forums like Reddit for community support and advice.
"By learning through challenges, developers not only build their knowledge but also maintain a lasting connection with the community."
Community Participation Highlights
Community participation can significantly enhance learning. Engaging with others can provide new perspectives and solutions to coding challenges. Platforms such as Stack Overflow and GitHub allow developers to share their experiences and seek guidance.
Technology Trends
The landscape of technology is continually shifting, and understanding current trends is vital for developers. Here, we look at some emerging technologies that affect the integration of MongoDB and Spring Framework.
Latest Technological Innovations
- The rise of microservices architecture pushes the need for flexible databases like MongoDB.
- Increased use of cloud platforms allows efficient deployment of applications using Spring and MongoDB.
Emerging Technologies to Watch
- Serverless Architecture: This provides scalability and performance improvements, making it a strong choice for applications that require fast responses.
- Graph Databases: While MongoDB is a NoSQL database, the introduction of graph features can further enhance its capabilities in certain applications.
Coding Resources
Coding resources are essential for developers wishing to bolster their skills in integrating MongoDB with the Spring Framework.
Programming Language Guides
Understanding Java is fundamental. Resources like official Java documentation can provide comprehensive guidance on Java programming.
Tools and Software Reviews
Familiarize yourself with tools like MongoDB Compass that can aid in visualizing and managing your database effectively. Spring Tool Suite is another excellent option for developing Spring applications efficiently.
Tutorials and How-To Articles
Numerous online tutorials exist that focus specifically on MongoDB and Spring integration. Websites like Medium often feature user-contributed content with fresh perspectives.
Online Learning Platforms Comparison
Platforms like Coursera and Udacity offer courses specifically in Spring and MongoDB. These can be beneficial for hands-on practice.
Computer Science Concepts
Developers should also possess a solid understanding of fundamental computer science concepts relevant to application development.
Algorithms and Data Structures Primers
Knowing how to effectively use data structures is essential in software development. Familiarize yourself with MongoDB's indexing strategies for efficient data retrieval.
Artificial Intelligence and Machine Learning Basics
Integrating MongoDB with machine learning frameworks can harness the power of data analytics and improve application performance.
Networking and Security Fundamentals
Understanding security best practices within both MongoDB and the Spring Framework is vital for any development project.
Quantum Computing and Future Technologies
As the technology landscape evolves, so too does the potential for integrating advanced computational models into Spring applications that utilize MongoDB.
By understanding coding challenges, current technology trends, and essential coding resources, developers can effectively harness the power of MongoDB and the Spring Framework. The integration of these technologies creates a robust foundation for developing modern applications.
Intro to MongoDB and Spring Framework
The integration of MongoDB and the Spring Framework represents a significant evolution in application development, particularly for those who aim to harness the power of NoSQL databases. This section establishes the context by reviewing the key elements, benefits, and considerations associated with both technologies.
Overview of MongoDB
MongoDB is a document-oriented NoSQL database designed for high availability and scalability. Unlike traditional relational databases that use tables, MongoDB utilizes collections and documents. This flexibility allows developers to store data in a more natural way, reflecting how the application itself thinks about data. The JSON-like format of documents enables easy integration with web applications, which often exchange data in similar structures. MongoDB is particularly well-suited for applications that need to handle large volumes of unstructured data or require rapid iteration and deployment.
Purpose of the Spring Framework
The Spring Framework serves as a comprehensive framework that simplifies Java application development. Its key purpose is to provide a wide array of systems for various needs such as dependency injection, transaction management, and aspect-oriented programming, which enhances modularity and maintainability. Specifically, Spring Boot, an extension of the Spring Framework, enables developers to create standalone applications with minimal configuration. This allows for accelerated development cycles and easier integration with microservices and cloud platforms. Coupled with MongoDB, Spring effectively grounds NoSQL strategies within structured application environments.
Importance of NoSQL in Modern Applications
In recent years, the shift towards NoSQL databases like MongoDB has been driven by the increasing need for scalability and flexibility in data management. Modern applications often generate large amounts of data across distributed systems, necessitating architectures that can adapt and grow without the bulkiness of traditional SQL databases. NoSQL, particularly document-oriented solutions, enables rapid data retrieval and manipulation. This aspect is crucial for real-time applications and analytics, where insights need to be derived immediately. Consequently, understanding NoSQL's place in application architecture becomes essential for developers aiming to create efficient, growth-oriented solutions.
"MongoDB addresses the limitations of traditional relational databases, making it an excellent choice for modern applications that demand high performance and scalability."
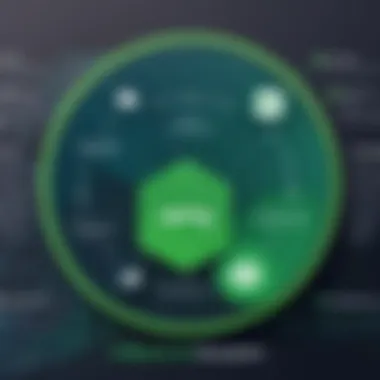
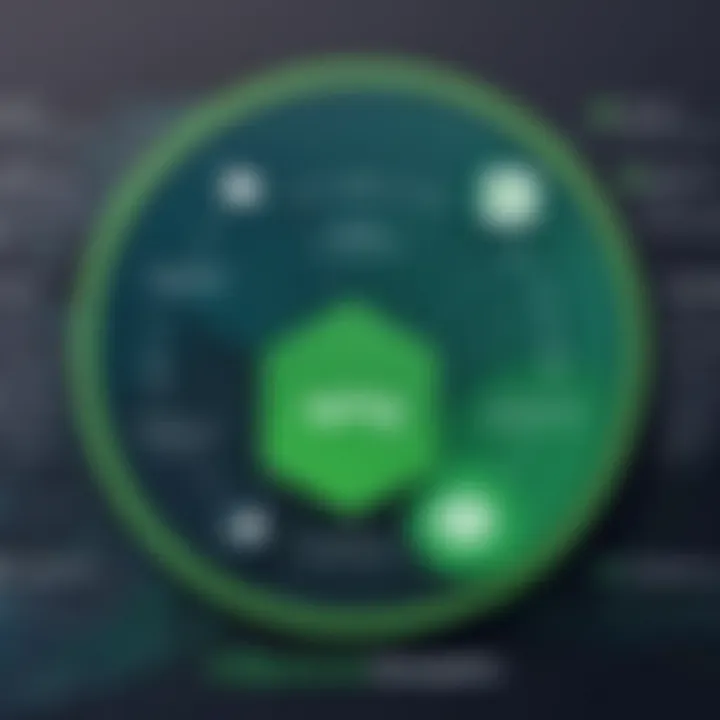
The points highlighted in this section lay the foundation for effectively integrating MongoDB with the Spring Framework. The combination not only enhances the capabilities of individual technologies but also opens new avenues for developing robust applications that are capable of meeting contemporary challenges in data management and application scalability.
Setting Up Your Development Environment
Setting up your development environment is a critical first step when integrating MongoDB with the Spring Framework. This stage ensures that all necessary tools and configurations are in place for smooth development and testing. A well-configured environment can enhance productivity and minimize issues during the integration process. Neglecting this aspect could lead to frustration and lost time due to misconfiguration or compatibility issues.
Installing MongoDB
To start, you need to install MongoDB, the NoSQL database that will store your application data. Installing MongoDB can vary slightly based on your operating system. Here are general steps to follow for a successful installation:
- Download MongoDB: Visit the official MongoDB website and download the Community Edition package.
- Install on Windows: Run the downloaded file. During the installation, you can choose either a Complete or Custom setup. A Complete installation will include all the necessary components.
- Install on macOS: Use Homebrew, a popular package manager. Execute the command: followed by . This command installs the latest version of MongoDB.
- Install on Linux: Use your distribution's package manager. For example, on Ubuntu, you can follow the guides specific to your version found on the MongoDB documentation.
After completing the installation, ensure the MongoDB service is running using the command . This will start the server backend, which is necessary to interact with the database.
Configuring Spring Framework
After MongoDB is correctly installed, the next step involves configuring the Spring Framework. Spring can manage your application's architecture efficiently, integrating well with MongoDB. First, you need to set up the Spring dependencies. This is typically done using Maven or Gradle. For Maven, add the following dependency to your :
This dependency includes all needed libraries to work with MongoDB in Spring applications. Make sure to set the Spring Boot version compatible with your project requirements. You may also configure your file to define the MongoDB connection string, specifying database, host, and port.
Using Spring Initializr for Project Setup
Spring Initializr simplifies the project setup process. It provides a web-based interface to generate a template for Spring applications. Hereâs how to effectively utilize it:
- Navigate to Spring Initializr: Open the website at start.spring.io.
- Project Metadata: Fill in your project's details such as group, artifact, and name. Choose your desired packaging method.
- Select Dependencies: Search for and select it along with other relevant dependencies needed for your project.
- Generate Project: Click the Generate button, which will create a file containing the project structure for you. Unzip the file at your desired location.
By utilizing Spring Initializr, you can save considerable time while ensuring proper structure and best practices are followed. It allows you to quickly kickstart your integration without dealing with complex setup.
"Setting up your development environment correctly is fundamental to ensure effective integration. Taking shortcuts here might result in costly mistakes down the line."
Core Concepts of MongoDB
Understanding core concepts of MongoDB is vital when integrating it with the Spring Framework. MongoDB, as a NoSQL database, operates on a different paradigm compared to traditional relational databases. This section will discuss three fundamental concepts: documents and collections, CRUD operations, and indexing and querying. Each of these components plays a critical role in how data is structured, accessed, and manipulated within a MongoDB environment, and thus directly influences effective integration with Spring.
Documents and Collections
Documents are the primary unit of data in MongoDB. They are essentially JSON-like structures that store data in key-value pairs. This format allows for flexibility in how data is stored, meaning that each document can have its own unique structure. This characteristic is especially useful in applications where data can be unstructured or schema changes are frequent.
Collections, on the other hand, are groups of documents. By default, MongoDB creates a collection automatically when a document is inserted. Unlike tables in SQL databases, collections do not enforce a schema, allowing for varied documents within the same collection. This flexibility isnât just a convenience; it enhances productivity by simplifying data modeling and retrieval processes.
Key Benefits
- Flexibility in Data Storage: With documents and collections, you can easily evolve your data model without downtime.
- Schema-less Nature: Changes to the data structure can be made seamlessly, reflecting real-world conditions.
CRUD Operations in MongoDB
CRUD stands for Create, Read, Update, and Delete. These operations are the foundational processes used to manage data within MongoDB.
- Create: Inserting data into a collection is straightforward. Using the or methods facilitates adding single or multiple documents.
- Read: Querying data is a crucial part of any application. MongoDB offers powerful query capabilities allowing users to retrieve specific documents based on field values using the method.
- Update: Updating documents allows applications to maintain accurate and current data. The , , and methods support various update strategies, ensuring efficiency.
- Delete: Removing documents is as simple as adding them. The and methods allow for clean-up based on specific criteria.
These operations are integral when integrating MongoDB with Spring, as they form the backbone of any interaction with the database.
Benefits of CRUD Operations
- Simplicity: The straightforward nature of these operations makes it easy for developers to manage data efficiently.
- Powerful Querying: Allows dynamic data retrieval, enhancing user experience in applications.
Indexing and Querying
Indexing is crucial for performance in MongoDB. Without indices, queries would require scanning every document in a collection. This can significantly slow down response times, especially with large datasets. Creating an index on a field enhances retrieval speed by optimizing query performance.
MongoDB supports various types of indexes, including single field, compound, and geospatial indexes. Choosing the right type of index is essential for achieving optimal performance, especially when scaling applications.
Querying data effectively in MongoDB often involves operators, allowing for precise control over what data is returned. Operators enable filtering, sorting, and controlling documents returned in query results.
Key Points on Indexing and Querying
- Performance Enhancement: Indexes can reduce query time dramatically, which is vital in high-traffic applications.
- Flexibility in Queries: The range of operators available facilitates complex querying mechanisms, powering enhanced data retrieval logic.
"Indexing is the most important step in optimizing performance when working with large datasets in MongoDB."
In summary, grasping these core conceptsâdocuments and collections, CRUD operations, and indexing and queryingâis essential for a streamlined integration of MongoDB with the Spring Framework. Each concept contributes significantly to how applications interact with data, improving both performance and ease of use.
Spring Data MongoDB
Spring Data MongoDB plays a crucial role in integrating MongoDB with the Spring Framework. It simplifies the development process and encourages best practices in data management. This section dives into its significance, emphasizing how it helps bridge the gap between the rich feature set of MongoDB and the familiar paradigms of Spring.
Prolusion to Spring Data MongoDB
Spring Data MongoDB provides an abstraction layer over MongoDBâs native functionality. This integration allows developers to use familiar Spring programming patterns while benefiting from MongoDB's flexibility. It enables easy mapping of Java objects to MongoDB documents. This facilitates rich data manipulations without extensive boilerplate code. Developers can conduct operations on MongoDB collections using simple method calls, which enhances productivity.
The library also supports powerful features like the repository pattern. Developers can create interfaces for their data repositories without needing to implement any methods. This promotes cleaner architecture and decouples data access layers from business logic. Moreover, the configuration is straightforward, allowing developers to focus on writing their application code.
Creating MongoDB Repositories
Creating repositories in Spring Data MongoDB is seamless due to its convenience. The base repository provides common methods for data access, and custom behavior can easily be added. Developers simply need to extend the interface. Here's an example:
With the repository in place, you can access MongoDB operations without writing complex queries. The method names used in your repository interface will translate to MongoDB queries, thanks to Spring Data's query derivation feature. This significantly reduces the amount of code required and minimizes potential errors.
Since these repositories are Spring-managed beans, it integrates smoothly into the Spring application context.
Query Methods in Spring Data
Spring Data MongoDB supports a variety of query methods for flexible data access. Developers can execute queries based on method naming conventions or use the annotation for more complex queries. For example, one can extract specific data by simply defining the method:
Using the annotation allows for even more customization. This provides a way to define precise MongoDB queries as follows:
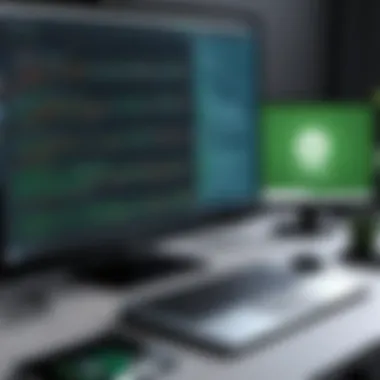
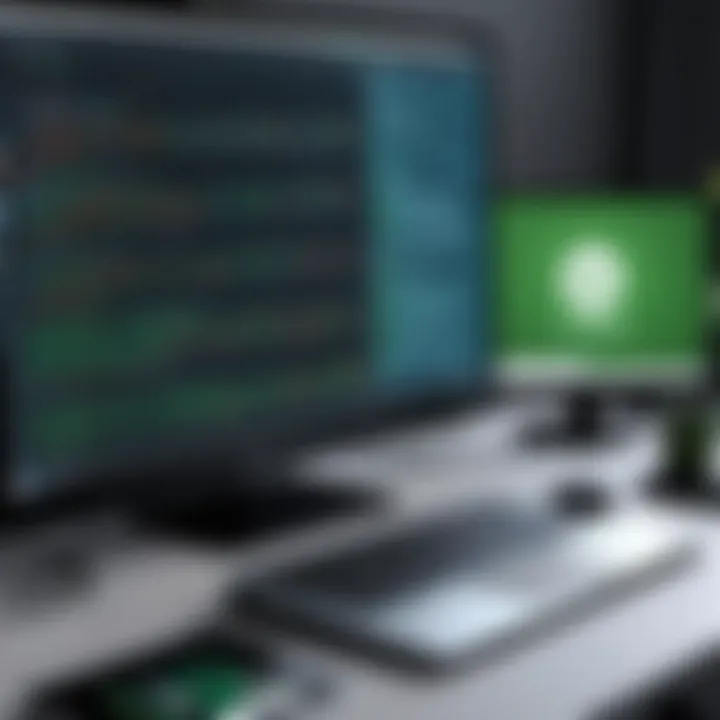
Incorporating query methods simplifies the process of fetching data. This also allows for seamless transitions between simple and complex queries, making it a valuable feature for developers.
Utilizing Spring Data MongoDB effectively increases maintainability and reduces the chance of operational errors.
The ability to easily implement complex queries enhances the power of MongoDB while adhering to the standards and practices of Spring. As a result, developers must leverage these capabilities for building effective applications.
Configuring MongoDB with Spring Boot
Configuring MongoDB with Spring Boot is crucial for any project that aims to utilize the capabilities of both technologies effectively. Spring Boot simplifies the setup process by providing default configurations and settings that save time and effort. This leads to faster development cycles, especially when working with NoSQL databases like MongoDB. Developers benefit from cleaner code due to the ease of integrating and managing dependencies, allowing for a focus on building application features rather than boilerplate configuration.
When using Spring Boot with MongoDB, one needs to pay attention to several key elements. Proper configuration ensures that the application can connect reliably to the MongoDB instance. It involves setting parameters for the MongoDB connection, understanding how to utilize data operations effectively, and implementing bean configurations to streamline application management.
Application Properties for MongoDB Connection
In a Spring Boot application, connection to MongoDB is typically managed via application properties. This is defined in the or file. The essential properties include the MongoDB connection string, database name, and other parameters such as username and password when applicable. For example, a typical configuration will look like this:
In this example, the configuration specifies the URI used to connect to the MongoDB server. It is significant to ensure that the URI is correct, as any mistakes can result in connection failures. Additionally, specifying a connection timeout can also improve the handling of network issues.
Here are some helpful tips for setting up these properties:
- Use to specify the database name.
- Enable a connection pool using for better performance.
- Implement error handling to manage potential connection issues gracefully.
Using MongoTemplate for Data Operations
Once the connection is established, MongoTemplate comes into play for performing data operations within Spring applications. This component simplifies interactions with the MongoDB database. It provides numerous methods for CRUD operations, such as saving documents, querying collections, and deleting records, all while following Spring's principles of simplicity and convention over configuration.
Here is an example of how to use MongoTemplate:
In this code snippet, MongoTemplate is used to save a object to the database and retrieve all users. Utilizing MongoTemplate not only enhances readability but also incorporates error handling and transaction management, which are essential in a production environment.
Configuring MongoClient Bean
Configuring the MongoClient bean is another vital part of integrating MongoDB with Spring Boot. The MongoClient is the main entry point for interacting with the database, managing the connections and ensuring efficient operations. In most cases, Spring Boot automatically configures the MongoClient based on the application properties set earlier, but there may be instances where custom configuration is necessary.
To manually configure the MongoClient, you can define it as a bean in your Spring configuration class:
This method gives you finer control over the MongoClient settings such as connection pooling, SSL configurations, and more. Knowing how to configure your MongoClient properly is fundamental for performance optimization and ensuring your application scales well with increasing demands.
Proper configuration of MongoDB with Spring Boot not only enables better performance but also ensures a smooth development process, allowing developers to focus on functionality over setup.
Handling Data Transactions
Handling data transactions is a crucial topic when integrating MongoDB with the Spring Framework. Transactions ensure data consistency and integrity, especially when multiple operations need to be completed together. Modern applications frequently require strong consistency, and understanding how to properly implement transactions can aid developers in designing robust systems that can handle complex business logic.
Understanding MongoDB Transactions
MongoDB transactions allow developers to group multiple operations into a single unit of work. This ensures that either all operations complete successfully, or none at all. This atomicity is critical in scenarios where partial updates can lead to data corruption or inconsistencies. MongoDB provides support for multi-document transactions starting from version 4.0, which means you can operate on multiple collections while maintaining data integrity.
Transactions in MongoDB function in a way that all involved documents are temporarily locked while the transaction is in progress, preventing other operations from interrupting the process. The transaction is either committed if all operations succeed or rolled back if any operation fails.
Some important benefits of using transactions in MongoDB include:
- Data Integrity: Ensures that data remains accurate under concurrent conditions.
- Atomic Operations: Guarantees operations execute completely or not at all.
- Simplified Error Handling: Developers can isolate failures more easily.
Implementing Transactions in Spring
In the Spring Framework, implementing MongoDB transactions involves a few key steps. Spring Data MongoDB provides an abstraction over MongoDBâs native transactional capabilities, making it easier to manage transactions in your application.
To start, you need to configure your application to use transactions. This includes setting up the , which manages the transaction lifecycle. Hereâs a basic outline:
- Configure MongoDB for Transactions:
- Set Up Transaction Manager:
- Use @Transactional Annotation:
- Ensure you are using a replica set or sharded cluster since transactions are supported only in these configurations.
- Create a bean in your Spring configuration. Here is an example:
- Annotate your service methods with . This tells Spring to handle transactions for the annotated methods.
Utilizing these steps to manage transactions allows developers to maintain a clean and efficient codebase while ensuring data consistency throughout their applications.
Best Practices for Integration
When integrating MongoDB with the Spring Framework, following best practices can streamline development and improve application performance. Effective integration not only ensures efficient data handling but also aligns with development standards that enhance maintainability and scalability.
Optimizing MongoDB Queries
MongoDB queries can greatly affect the performance of your application. To optimize queries, it is crucial to consider the following strategies:
- Indexing: Creating the right indexes accelerates query times. Use compound indexes for queries that filter by multiple fields.
- Projection: Fetch only the necessary fields instead of entire documents to reduce data transfer load.
- Aggregation Framework: Use the aggregation pipeline to perform operations on data efficiently without needing multiple queries.
- Limitations: Always apply limits to queries when retrieving large datasets to prevent overwhelming the application.
By applying these optimizations, queries will run faster, resulting in a more responsive application.
Data Modeling Strategies
Effective data modeling is key to harnessing the full potential of MongoDB within a Spring application. When modeling your data, consider:
- Schema Design: Leverage MongoDBâs flexible schema while maintaining logical relationships. A nested schema may reduce the need for complex joins.
- Normalization vs. Denormalization: Understand the trade-offs. Normalized structures may reduce duplication but could slow down retrieval if they involve many lookups.
- Document Size: Keep an eye on document size limits. Design your documents to ensure they remain efficient under the maximum size of 16 MB.
- Relationships: Use references for large datasets that do not need to be retrieved together. Consider using embedded documents for tightly related data.
Good data modeling enhances performance and keeps your application manageable as it grows.
Testing and Debugging with Spring Data MongoDB
Testing and debugging are vital in maintaining code quality. To effectively test and debug applications using Spring Data MongoDB:
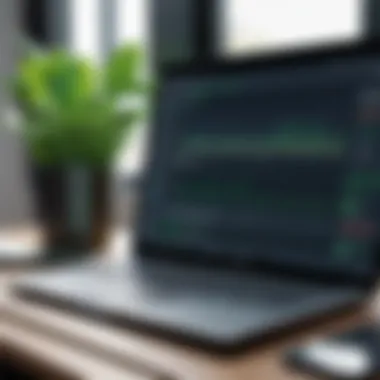
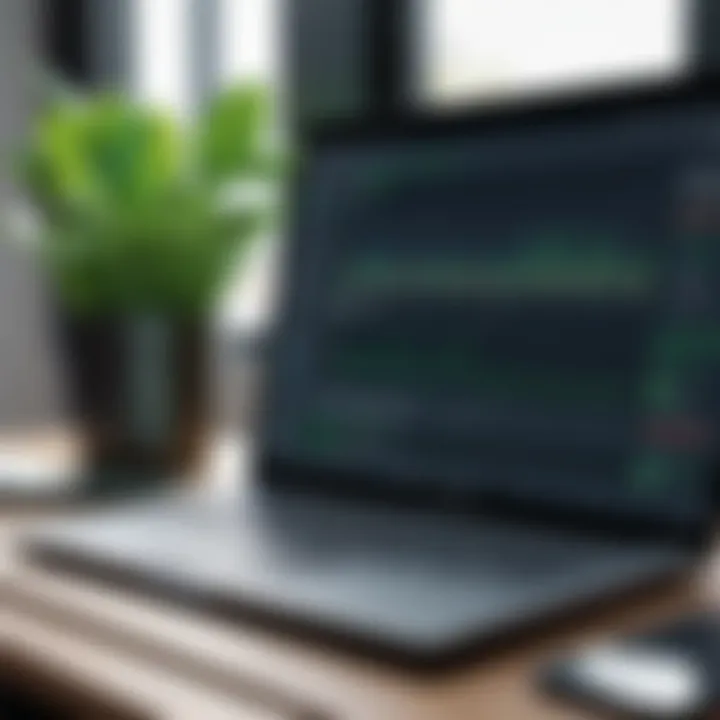
- Unit Tests: Write unit tests for your repositories. It checks the basic functionality of data access methods.
- Integration Tests: These tests should verify how well components interact with MongoDB. Use embedded databases for fast testing.
- Logging: Implement logging mechanisms to catch errors early. Proper logs can help trace issues that arise during CRUD operations.
- Error Handling: Build comprehensive error handling in your data access layer. This practice ensures better resilience during failures.
By prioritizing these practices, developers can achieve robust applications that are easy to maintain and debug.
Remember: Effective integration of MongoDB with the Spring Framework leads to powerful, responsive applications.
Monitoring and Performance Tuning
Monitoring and performance tuning are crucial aspects of maintaining an effective integration between MongoDB and the Spring Framework. These practices ensure that applications run efficiently while adapting to growing data demands. Performance issues can significantly impact user experience, making it necessary to adopt a proactive approach.
Effective monitoring provides insights into how the application is performing in real-time. It allows developers to detect potential issues before they escalate. Additionally, performance tuning optimizes the application by adjusting settings, queries, and architecture based on real usage metrics. Both elements contribute to achieving high availability and responsiveness in applications that rely on MongoDB.
Key Performance Metrics in MongoDB
Understanding key performance metrics is essential for anyone working with MongoDB. Such metrics provide a snapshot of how the database is handling requests and if resources are being utilized effectively. Here are some important metrics to track:
- Operation Counts: This metric tracks the number of reads and writes occurring. High operation counts can indicate heavy usage, which should be analyzed further.
- Latency: Monitoring request latency helps identify any slow queries or performance bottlenecks. An increase in latency could suggest the need for query optimization or index improvements.
- Memory Usage: Observing how much memory MongoDB is consuming can help prevent out-of-memory errors. MongoDB uses RAM for caching data, influencing the performance of read operations.
- Disk I/O: Disk input/output rates reveal how well the storage subsystem performs. High disk usage can lead to slow query response times, indicating a need for disk optimization.
- Connections: Tracking the number of active connections to the database enables you to understand the load on your MongoDB server. Too many connections can exhaust server resources and affect performance.
By keeping an eye on these metrics and evaluating them regularly, developers can make informed decisions about necessary adjustments or optimizations.
Utilizing MongoDB Atlas for Monitoring
MongoDB Atlas offers built-in monitoring tools that simplify the task of tracking database performance. This fully managed cloud service provides insights to help developers maintain optimal performance without extensive manual monitoring.
Atlas provides a comprehensive dashboard displaying key metrics, such as:
- Real-Time Performance Metrics: This includes throughput, latency, and operation counts, allowing for immediate insights into database health.
- Alerts and Notifications: Users can set up alerts for specific thresholds. This ensures that significant performance drops or anomalies trigger notifications, ensuring problems are addressed swiftly.
- Performance Advisor: Atlas includes a feature that suggests indexing improvements based on ongoing queries, promoting optimal database performance.
By integrating MongoDB Atlas into the development workflow, developers can focus their efforts on building features rather than on manual monitoring tasks. The insights provided by Atlas play an important role in maintaining a responsive application.
Implementing effective monitoring and performance tuning strategies will lead to improved responsiveness and a more efficient integration of MongoDB with Spring Framework.
Incorporating these practices will not only enhance current application performance but also prepare the system for future growth.
Scalability Considerations
Scalability is crucial in application development, especially when dealing with large datasets and high traffic. As businesses grow, so does the need for efficient data management. MongoDB's NoSQL architecture excels in this area, providing flexibility and scalability that traditional relational databases often lack. This section explores the significance of scalability when integrating MongoDB with the Spring Framework, looking specifically at sharding and caching strategies.
Sharding in MongoDB
Sharding is a method that MongoDB uses to distribute data across multiple servers or clusters. This is important for handling large volumes of data efficiently. When an application grows, a single server may not be able to manage all the requests or store all the data. Sharding solves this issue by splitting the dataset into manageable chunks called "shards."
Each shard is a separate database, which allows MongoDB to spread the load. This distribution results in better read and write performance since multiple operations can occur simultaneously across the shards. Additionally, adding more shards as your data grows remains a straightforward process, making MongoDB highly adaptable to changing application needs.
Key benefits of sharding include:
- Increased Throughput: Several servers working together can handle more requests than a single server.
- Load Balancing: Distributing data helps prevent any single point of failure, enhancing overall system resilience.
- Scalability: Adding hardware is simple, allowing for growth without performance loss.
Overall, sharding allows developers to design applications with high data volumes and user loads in mind.
Caching Strategies in Spring Applications
Caching is another essential aspect of scalability in Spring applications. The performance of applications can significantly improve when frequently accessed data is stored temporarily. Spring Framework provides several caching options that can be integrated with MongoDB.
Common strategies include:
- In-Memory Caching: Using tools like Ehcache or Caffeine keeps data in memory, providing rapid access and reducing database load.
- Distributed Caching: Systems like Redis or Hazelcast let multiple instances of an application share cache information. This is especially useful in cloud environments where applications may run on multiple nodes.
- HTTP Caching: For web applications, using HTTP caching can help minimize server loads and enhance user experience by serving cached content quick.
Effective caching can provide a seamless experience for users and relieves the underlying database from excessive load, maintaining performance levels even as the user base grows.
Caching is essential for improving application performance, enhancing user experience while allowing for scalability as data and access needs grow.
By focusing on these scalability considerations, we can ensure that MongoDB and Spring Framework applications are prepared to handle future demands while maintaining performance.
Troubleshooting Common Issues
Troubleshooting common issues is an essential part of working with MongoDB and the Spring Framework. As developers integrate these technologies, they may encounter various problems related to connectivity, data handling, or unexpected behavior. Addressing these issues promptly and effectively can enhance productivity and improve the overall workflow. Understanding the root causes of common problems allows developers to avoid potential pitfalls and create a more robust application. This section will delve into two primary areas of concern: Connection Problems and Data Retrieval Issues.
Connection Problems
Connection problems can manifest as various symptoms, such as timeouts, failure to connect, or authentication errors. These issues can be frustrating and can halt development unexpectedly. To effectively troubleshoot connection problems, developers must first ensure that the MongoDB server is running and accessible.
A common cause for connection failures is misconfigured connection strings. An incorrect hostname, port number, or username can prevent a successful connection. Review your applicationâs properties file to verify that the details match those of your MongoDB instance.
Additionally, network issues can impact connectivity. Firewalls or security groups might block traffic between the application server and the MongoDB server. Check the network configuration, ensuring that both services can communicate over the designated port, typically 27017 for MongoDB.
Other potential factors to consider include:
- Compatibility of MongoDB versions with the Spring Data module.
- MongoDB server limits, such as maximum connections.
- Driver dependencies that might be outdated.
In summary, resolving connection problems involves validating configuration settings, checking network policies, and ensuring compatible versions of the involved components. Regular maintenance and monitoring can proactively avert these issues.
Data Retrieval Issues
Data retrieval issues can arise from poor queries, unexpected data formats, or mismatched data types. Effective data retrieval is crucial for the functionality of applications, and any disruptions here can lead to significant setbacks.
When developers face challenges retrieving data, the first step is to analyze the queries being used. MongoDBâs flexible query structure requires a deep understanding of how to construct effective queries. Common mistakes include using incorrect field names, missing filters, or applying unsupported query operators. Misjudgment in query formulation can lead to empty results or performance bottlenecks.
Another factor that can lead to data retrieval issues is the schema design. Unlike relational databases, MongoDB does not enforce a strict schema, which can lead to inconsistent data storage. It is vital for developers to implement appropriate validation at the application level to ensure data integrity. When documents deviate from expected formats, retrieval operations may fail or return incomplete data.
Moreover, considering query performance is also necessary. For instance, if a query lacks proper indexing, it may cause slow retrieval times, leading to a poor user experience. Use MongoDBâs indexing features effectively to optimize your queries.
Final Thoughts
As we conclude our exploration of integrating MongoDB with the Spring Framework, it is essential to reflect on the critical elements discussed throughout this guide. This integration serves not only as a tool but also as a strategy for developers aiming to enhance application performance and scalability. The marriage of NoSQL capabilities with the Spring environment opens new avenues in application design, allowing for more innovative solutions.
We have examined the advantages of using MongoDB, including its flexibility, high performance, and ease of scalability. These elements become particularly valuable in todayâs fast-evolving technological landscape. When combined with the Spring Framework, developers can enjoy a potent environment for building robust applications that can adapt to varying demands and complexities. The seamlessness of this integration contributes to a more efficient development process, enabling teams to deliver high-quality products within tighter timeframes.
The Future of MongoDB and Spring Integration
Looking ahead, the future of MongoDB and Spring integration appears promising. As both technologies evolve, the synergy between them is likely to grow stronger. Features like cloud-native databases and advanced data management techniques are becoming increasingly integral to development. Understanding how to leverage these advancements will be paramount for developers.
The growing popularity of microservices architecture further supports this future. Developers are continuously seeking ways to build light-weight, decoupled, and scalable services. MongoDB complements this architecture perfectly, thanks to its document-oriented structure, which aligns with the dynamic nature of modern applications. Moreover, Spring Boot continues to simplify and enhance the development experience, making it easier to connect and manage MongoDB instances.
Continuing Education and Resources
To keep pace with advancements in both MongoDB and the Spring Framework, ongoing education is crucial. Developers should consider the following resources to stay informed:
- Official Documentation: Both the MongoDB Documentation and the Spring Framework Documentation provide comprehensive and up-to-date information.
- Online Courses: Websites like Coursera, Udemy, and Pluralsight offer specialized courses tailored for integrating MongoDB with Spring. These can deepen your knowledge and provide hands-on experience.
- Community Forums and Discussion Groups: Platforms such as reddit.com and Stack Overflow host vibrant discussions and can be invaluable for troubleshooting and gaining insights from peers.
- Books and Publications: Titles such as "MongoDB: The Definitive Guide" by Kristina Chodorow and "Spring in Action" by Craig Walls offer in-depth knowledge and practical examples.
By actively pursuing education and staying updated with industry best practices, developers can not only enhance their skills but also contribute to the broader community of technology enthusiasts. The combination of MongoDB and Spring Framework presents significant opportunities, and equipping oneself with knowledge is vital for meaningful engagement in these domains.