Integrating MySQL with Python: A Comprehensive Guide
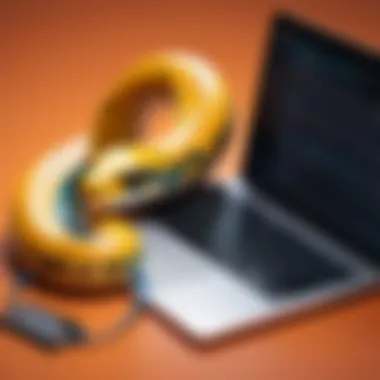
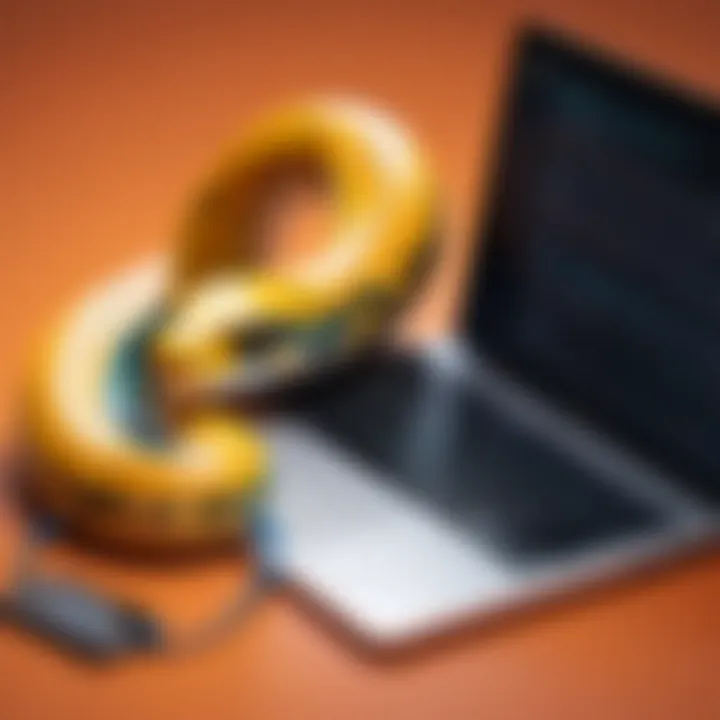
Intro
In today’s data-driven world, the need to manage and manipulate databases effectively cannot be overstated. Relying on MySQL, one of the most popular relational database management systems, alongside Python, a powerhouse for data processing and analysis, opens doors to countless possibilities. This combination is more than just a partnership; it is a pivotal skill set that can enhance your programming toolkit and professional repertoire.
Whether you are an aspiring programmer, an experienced developer, or a computer science student, grasping how to weave Python with MySQL can define your approach towards effective database management. Imagine being able to seamlessly fetch data, run complex queries, or even build your own applications to manipulate data just as a maestro conducts an orchestra. With robust libraries like and , you can effortlessly interact with MySQL databases, opening the floodgates to creativity and efficiency.
In this guide, we delve into the nitty-gritty of using Python with MySQL. From installation to advanced techniques, we will walk you through fundamental CRUD operations (Create, Read, Update, Delete) and provide insights into best coding practices. We'll also touch on performance considerations, which are imperative for developing efficient systems.
Relevance of This Integration
Merely knowing Python and MySQL in isolation won't cut it in a competitive landscape. Their integration equips you to handle data more effectively, which can lead to insightful decision-making in businesses. In the age of AI and big data, the ability to interface these two technologies is not just beneficial but essential. From backend development to data analytics, the skills you will acquire in this guide will serve you well, whether it's for a startup project or a corporate environment.
This read promises to blend practical coding challenges with theoretical insights, ensuring you come away knowledgeable and ready to tackle real-world projects in your journey of mastering MySQL and Python together.
Understanding MySQL and Python
Understanding MySQL and Python is crucial in today’s data-driven world. Both tools are powerful on their own, but their integration opens up a wealth of possibilities for developers and data enthusiasts alike. MySQL, as a widely used relational database management system, allows for efficient data storage, querying, and manipulation. Python, famed for its readability and simplicity, offers a flexible programming environment that can be adapted for a variety of tasks.
When programmers leverage these two technologies together, they can create robust applications that handle immense datasets with ease. The synergy between MySQL and Python comes from Python's extensive libraries and frameworks designed for seamless database interaction. This article aims to unravel how to utilize this connection effectively.
Preface to MySQL
MySQL, developed in the mid-90s, has stood the test of time and continued to evolve. It operates on a client-server model, where the server manages the databases while the clients send queries to retrieve or manipulate data. Its popularity is attributed to its open-source nature and compatibility with numerous platforms and programming languages.
For anyone delving into the world of databases, MySQL serves as an accessible starting point. With an array of data types and powerful SQL (Structured Query Language) commands, users can perform operations ranging from simple selections to complex JOINs involving multiple tables. In essence, mastering MySQL is about understanding how to efficiently structure data and perform meaningful queries.
Overview of Python
In the realm of programming languages, Python often edges out the competition due to its simplicity and versatility. Introduced in the early 90s, Python features a sleek syntax that prioritizes code readability. Its extensive standard library includes modules for various tasks, making it advantageous for both beginners and experienced developers.
Python's utility across different domains — web development, automation, data analysis, machine learning — makes it particularly attractive. Libraries such as Pandas and NumPy empower users to process and manipulate data efficiently. By incorporating MySQL into the Python mix, developers can harness the best of both worlds, enabling quick data handling alongside powerful programming structures.
Importance of Database Management in Programming
In programming, database management is not merely an auxiliary task; it’s a cornerstone of application development. Understanding how to manage databases effectively can greatly enhance the performance, reliability, and scalability of applications.
When dealing with data, one must consider how it will be stored, retrieved, and manipulated. An efficient database management system like MySQL allows developers to handle large datasets logically and systematically. Proper database practices can also lead to:
- Improved Data Integrity: Establishing relationships and constraints ensures data remains consistent and accurate.
- Enhanced Performance: Efficient indexing and query optimization techniques reduce the time it takes to access or modify data.
- Scalability: Understanding how to design databases for growth is crucial in today’s fast-paced environment.
The successful integration of MySQL with Python exemplifies how effective database management can streamline programming efforts. Ultimately, proficiency in this area positions developers to build applications that not only meet user demands but also stand the test of time.
Setting Up the Environment
Setting up the environment is a crucial step in integrating MySQL with Python. This stage involves installing the necessary software and configuring it correctly to establish a successful connection between your Python code and the MySQL database. Without a properly set up environment, even the best-designed code can lead to unending errors and frustrations. Here, we will cover important elements, benefits, and considerations surrounding this setup.
When you set up the environment, you ensure that all required components—such as MySQL server and Python libraries—are available and configured correctly. Achieving this can save a great deal of time and effort in the long run. Misconfigurations could lead to errors that are cumbersome to troubleshoot, making it imperative to address setup properly from the outset. An efficient setup grants you a solid foundation upon which to build and test your applications.
Installing MySQL Server
To kick things off, installing MySQL server is the first step. This software is fundamental as it acts as the backbone of your database operations. Depending on your operating system, installation methods can vary. For instance, on Windows, you might download the installer from the official MySQL website. Meanwhile, Linux systems often facilitate installation using package managers like apt or yum.
The key characteristic of installing MySQL server is its impact on your ability to manage and interact with data effectively. Once MySQL is installed, you can create databases, run queries, and perform various data operations essential for your applications. Remember, a functional MySQL server is not just a requirement; it’s a powerful tool that allows you to harness data’s full potential.
Installing Python and Necessary Libraries
Now, let’s move on to installing Python and the required libraries. Without Python, you won’t be able to write the scripts that will communicate with your MySQL database. Python’s simplicity and versatility are what make it a go-to choice for many developers.
Using pip for Installation
Using pip for installation is an efficient way to handle Python packages, including libraries necessary for MySQL integration. The beauty of pip lies in its simplicity. It allows users to easily install and manage packages with just a command your terminal. For example, using the command will quickly install the MySQL connector.
This method is popular because it streamlines the installation process. Instead of hunting for individual package files, pip fetches and installs dependencies automatically. Just keep in mind that relying solely on pip means you should make sure you have a stable internet connection.
Installing MySQL Connector
The MySQL Connector is an essential library that bridges Python with MySQL databases. Its main characteristic is its ease of use, allowing for straightforward interaction between your code and the database. This library supports multiple connection options and allows you to execute queries seamlessly.
One unique feature of the MySQL Connector is being actively maintained by Oracle. This means you can expect updates and support, which can be beneficial as features or security improvements are made. On the downside, if you’re working in a very particular setup, sometimes version compatibility might raise issues, so keeping your libraries updated is a good practice.
Setting Up Environment Variables
Setting up environment variables is one of those behind-the-scenes tasks that can make or break your integration efforts. Environment variables are used to store configuration settings needed by your applications. For instance, you might need to specify the path to the MySQL connector or other environmental settings that your application will rely on.
The unique feature of environment variables is that they allow code to adapt to different environments without changing the code itself. This is especially handy if you’re working in various stages like development, testing, and production. However, incorrect settings can lead to miscommunication between your Python code and MySQL server, resulting in frustration. So, always verify these variables after you set them up.
Configuring MySQL for Remote Access
Once you've installed MySQL and configured your Python environment, the next step is to ensure that MySQL can support remote connections. This is particularly important if you are deploying your application or working with a team. Out of the box, MySQL may be secure by only allowing local connections. To allow remote access, you'll need to adjust some configurations in the MySQL setting files.
Check the MySQL documentation for specific configuration options, and ensure that user permissions are correctly set. With the proper configurations, you'll be able to access your database from different locations effortlessly, enabling more fluid collaboration and application testing.
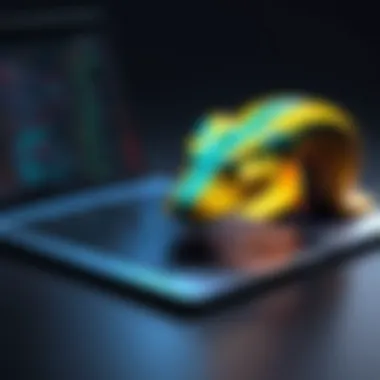
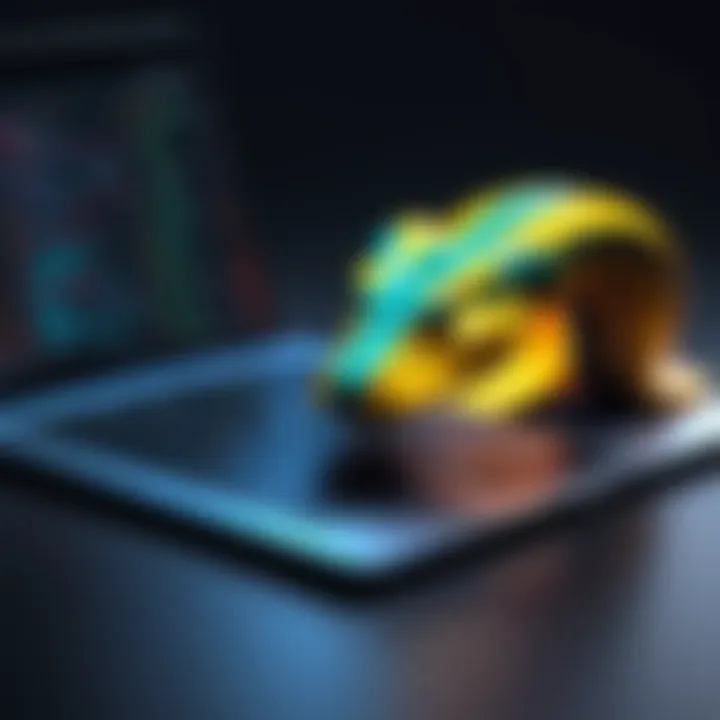
Remember, proper configuration and setup not only streamline your development process but also serve as a safety net against future complications that could arise.
Establishing Database Connections
Establishing a connection between Python and a MySQL database is the backbone of any database interaction. Without a proper connection, all the sophisticated queries and data manipulations become moot. This section will break down essential elements and considerations that revolve around making these connections, ensuring you have a firm grip on the process.
Connecting to MySQL Database in Python
First things first, you’ll need to establish that connection. In Python, you typically do this with the help of a connector library, such as the MySQL Connector/Python. This library allows Python scripts to communicate seamlessly with the MySQL database. A basic connection setup looks something like this:
This snippet effectively lays down the groundwork for interacting with the database. Remember to replace , , , and with your specific details.
It’s crucial to note that, besides the basic connection parameters, additional options may come handy based on your setup. For instance, if you're dealing with remote servers, you'll need to ensure that the connection parameters include the correct IP address and possibly port number. The importance here is simplicity; keep your connection string straightforward to avoid confusion.
Handling Connection Errors
Now, no one likes to run into hurdles, but errors are part and parcel of working with databases. Handling connection errors gracefully can make a world of difference in your application’s robustness. The MySQL Connector raises exceptions that can provide insights into what went wrong, be it a failed login, unreachable host, or a missing database.
Here’s how you can catch these connection errors:
This approach allows your application to continue running, offering a more pleasant user experience. It’s wise to log these errors so you can troubleshoot effectively. Remember, error handling isn't just about preventing crashes; it's also about maintaining the integrity of your processes.
Using Context Managers for Connections
When you're done working with your database, appropriately closing connections is just as crucial as opening them. One way to ensure this happens consistently is by utilizing context managers in Python. Context managers allow for automatic resource management, which is beneficial in preventing memory leaks and ensuring connections are closed properly, even if errors arise.
Here’s an example of how to leverage a context manager for your database connection:
In this code, the statement guarantees that the object is closed once the block is exited. It mechanizes a clean-up routine that shields you from potential chaos down the line. Using context managers provides clarity and keeps your code base cleaner.
By establishing proper connections and handling them correctly, you pave the way for smooth interaction between your Python scripts and MySQL databases.
Throwing yourself into these details might seem like a lot at first, but mastering them lays down the solid foundation for developing database-driven applications. Stay focused, and good luck as you climb the ladder of database management!
Performing CRUD Operations
When it comes to working with databases, understanding the Performing CRUD Operations is the backbone of effective data management. CRUD stands for Create, Read, Update, and Delete, and these operations form the fundamental building blocks for any application that interacts with a database. Mastering these operations allows developers to handle data in a way that is both systematic and efficient. Without the ability to effectively manage records through these operations, the capability of a system diminishes significantly.
Each CRUD component serves distinct purposes and presents unique challenges. For instance, creating records involves inserting new data, while reading data centers on extracting that information. Updates modify existing details, and deletion removes records that are no longer necessary. Understanding how these components work together can vastly improve the robustness and performance of an application.
Engaging with CRUD operations not only provides insight into the daily functions of database management but also teaches developers about optimizing queries for better efficiency. It reveals the common pitfalls to avoid, such as executing non-parameterized queries that pave the way for SQL injection attacks. As we discover the nuances of each operation, it becomes evident why a solid grounding in CRUD is imperative for any developer working with MySQL and Python.
Creating Records
Creating records in a database is the first step in data management. It involves inserting new entries into tables, making it essential for any application that needs to collect or store information. With the statement in SQL, you can add an entire row of data or specific columns. The key here is understanding the structure of the database and ensuring that data adheres to defined schema constraints such as data types and unique identifiers.
A common example could be inserting a new user into a user table. It’s not as straightforward as it looks; you need to make sure that the necessary fields are populated and that no rules set by the schema are violated. This operation becomes especially important when handling data integrity and consistency across applications.
Reading Data
Being able to read and access data is just as crucial as collecting it. In this process, SQL's statements play a significant role in retrieving relevant data from MySQL databases.
Executing SELECT Statements
The statements are arguably the most powerful tools in database querying. They allow for the extraction of data based on specified criteria. Developers can control which columns to fetch and even which rows to include using WHERE conditions. This means you can precisely tailor your queries to meet specific needs, whether it’s fetching all records from a table or picking just a few based on certain conditions.
The key characteristic that makes statements so popular is their flexibility. From simple queries to complex ones involving joins and aggregations, they serve a wide range of purposes. The unique advantage of using is that it maintains performance even in large datasets when optimized properly. However, poorly structured queries can lead to slower response times and heavier loads on the server.
A well-formed statement is not just an advantage; it’s a necessity for any application requiring data retrieval.
Fetching Results
Fetching results from executed queries captures the essence of how applications read data. After running a query, you can obtain results in various formats. Libraries like MySQL Connector make it easy to fetch one record at a time, or you can opt to gather all records simultaneously.
A major characteristic of this operation is speed and efficiency. The fetching mechanism directly impacts how quickly a user can interact with the data. For instance, fetching results using cursors can be more efficient than fetching all results at once, especially with large data sets. The unique feature here is the choice of retrieval method, which can have implications on both speed and memory usage in your application.
Updating Records
Updating records is where developers must tread carefully. The ability to modify existing data is critical for applications that allow user-generated content or updated information. The statement is used for making these changes, but it requires precision to avoid unintended data loss or corruption. Knowing how to set the correct clause is essential in ensuring that only the intended records are affected.
Deleting Records
The last part of managing data involves cleaning up or removing entries that are no longer relevant. Executing the statement is a straightforward procedure, but similar to updates, it must be done with caution. An incorrectly executed delete operation can lead to irreversible data loss.
Hence, being well-versed in CRUD operations establishes a foundation for future database interaction, allowing developers to manage their data efficiently and securely.
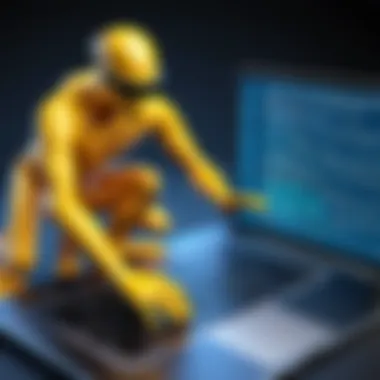
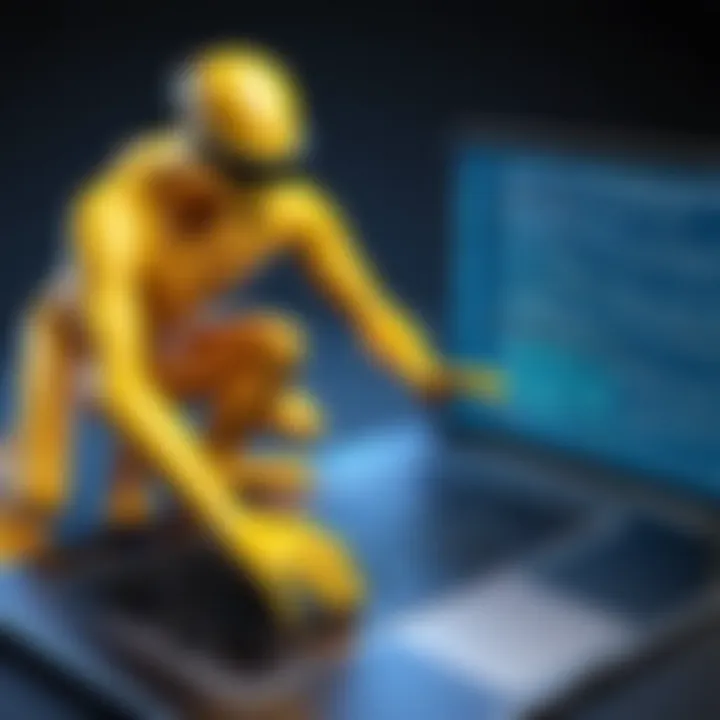
Advanced Database Management Techniques
In the realm of database management, integrating advanced techniques into your MySQL interactions with Python can significantly enhance both performance and reliability. These methods not only streamline processes but also bolster security and maintainability of your applications. Every programmer, from the novice to the seasoned veteran, must grasp these concepts to unlock the full potential of database systems.
Using Transactions
Transactions are crucial for ensuring data integrity. When you perform multiple actions that modify the database, it is vital that all these operations succeed or fail as a single unit. This all-or-nothing approach is encapsulated in the world of transactions.
In a practical sense, if you're updating funds in a banking application, you wouldn't want the debit operation to succeed while the corresponding credit fails. Hence, using transactions helps avoid inconsistencies and ensures that your application behaves predictably under various conditions. Python's library makes handling transactions straightforward with its support for commit and rollback operations. Here's a simplified example:
This example uses a structure to ensure any errors during the transaction lead to a rollback, avoiding half-done operations.
Prepared Statements and Parameterized Queries
Prepared statements are a hallmark of secure and performance-oriented database programming. They allow developers to define a SQL statement template in advance and use parameters to substitute the actual values at runtime. This has two significant benefits: it enhances security by protecting against SQL injection attacks and it can improve performance by facilitating query execution plan reuse by the database.
Imagine you're building an API that queries user details based on input from an external source. Instead of constructing SQL strings dynamically (which leaves you vulnerable), you prepare the statement:
Here, is safely passed as a parameter. Any attempt at SQL injection is thwarted because the database knows to treat the parameter as data, not executable code. This is a must-learn for any developer wanting to safeguard their applications.
Executing Batch Operations
When dealing with large datasets, individual insertions or updates can be painfully slow. Batch operations tackle this issue head-on by allowing multiple SQL commands to be sent to the database at once. This reduces the number of network round trips and can drastically improve performance.
Using the , running bulk inserts can transform your application's efficiency. For example:
This method not only saves time but also makes your database interactions more efficient. Some databases can also handle bulk operations via specialized commands, further optimzing your performance.
Proper management techniques in database systems are not just mere options; they are essential pillars that uphold the stability and efficiency of any application.
Optimizing Database Performance
Optimizing database performance is essential in any application where data integrity and speed are paramount. In our context of integrating MySQL with Python, focusing on database performance can significantly influence user experience, data retrieval times, and overall system efficiency. As your project scales, so does the necessity for a well-optimized database. Thus, understanding effective optimization techniques is crucial.
Indexing Strategies
Indexes are like road signs on a highway; they guide the database engine to retrieve data efficiently. Implementing proper indexing strategies can drastically reduce the time taken to fetch data.
- Types of Indexes:
- B-tree indexes: These are appropriate for most situations and excel in handling a wide range of queries.
- Full-text indexes: Useful for searching long strings of text, like articles or blog posts.
- Hash indexes: Efficient for key-value lookups, though less versatile for complex queries.
When creating an index, it’s vital to consider the specific queries most likely to run against the data. If you frequently search for users by their email addresses, creating an index on that column will enhance performance. However, over-indexing can be counterproductive, as it adds overhead during data insertion or updates.
Analyzing Query Performance
Analyzing the performance of your queries is another pillar of optimizing database performance. Without evaluation, you might be navigating blind in the labyrinth of data management. Using the built-in EXPLAIN statement in MySQL can reveal how a query executes, highlighting slow operations and areas in need of improvement.
- Things to look for:
- Join types: Identifying whether the database is using nested loops or optimized joins affects speed.
- Table scans: Full scans are costly; if you observe many, it's time to address indexing.
- Query plans: Reviewing the execution plan helps in understanding how efficiently queries are structured.
Regular performance tuning is integral. Maintain a documented process of query performance measurement to track changes over time, ensuring that modifications lead to genuine improvements.
Caching Strategies in Application Development
Caching is like having a speedboat on a lazy river; it saves time in getting to where you need to be. Implementing effective caching strategies can enhance the performance of your MySQL queries and minimize database load.
- Application-level caching: Utilize frameworks like Flask or Django, which offer built-in caching mechanisms. This allows frequently requested data to be stored in memory, reducing database lookups.
- Object caching: Solutions like Redis or Memcached can store data objects, such as user sessions or query results, to deliver faster responses for repeated data requests.
- Database query caching: MySQL has its own query cache feature for repeated read operations. While it won’t replace need for optimization, it can reduce read overhead per individual query.
"Caching is not just an optimization; it’s a necessity for performance in modern applications."
As with all strategies, it’s essential to monitor and analyze the effectiveness of the caching implemented, making adjustments based on evolving application demands.
By honing in on indexing strategies, analyzing query performance, and applying effective caching, you can ensure your database operates at peak performance. This not only makes your applications faster but also keeps user satisfaction high, ultimately leading to a more robust system.
Security Considerations
In the digital age, safeguarding sensitive data has never been more crucial. As applications become increasingly interconnected, ensuring robust security measures in your database is essential. Integrating MySQL with Python offers flexibility and power in managing data; however, it also presents potential security vulnerabilities. By acknowledging these risks and implementing security considerations, developers can protect both their data and their applications from malicious threats.
This section explores the best practices for database security and highlights how to effectively prevent common vulnerabilities, such as SQL injection attacks.
Best Practices for Database Security
Creating a secure database environment involves more than just implementing one-off fixes. It requires building a culture of security awareness and adherence to best practices. Here are some key recommendations:
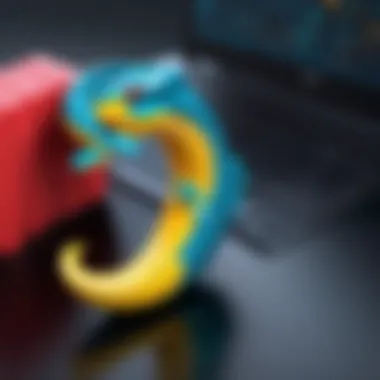
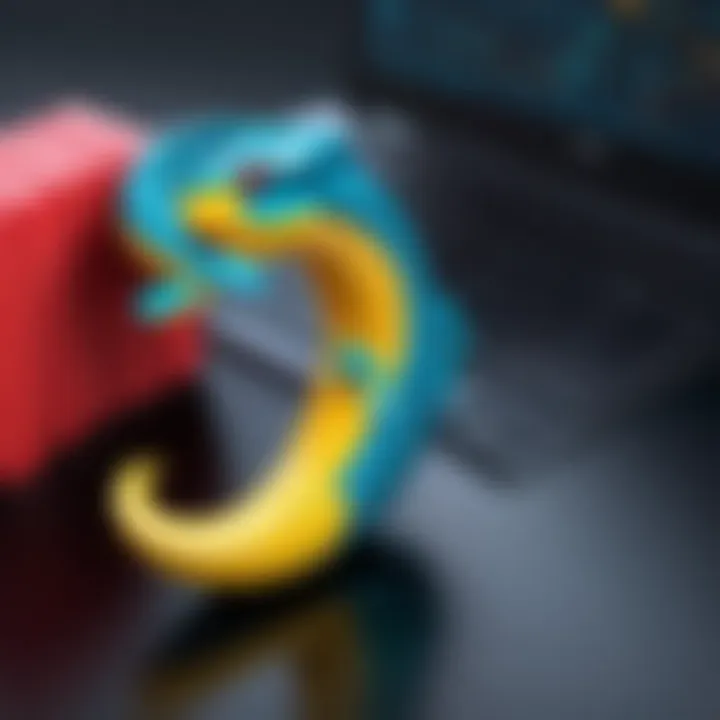
- Use Strong Passwords: Always employ complex passwords for database users. Avoid default passwords and regularly update them.
- Limit User Privileges: Grant users the minimal privileges they need to perform their tasks. This principle of least privilege minimizes the risk of unauthorized data access.
- Regular Backups: Keep consistent and reliable backups of your data. Should an incident occur, you can restore your database to a functional state.
- Apply Security Updates: Stay informed about the latest security patches for MySQL and your Python environment. Applying updates promptly is fundamental to mitigating potential vulnerabilities.
- Monitor Database Activity: Implement logging to track database access and changes. This can help detect abnormal access patterns that may signify a breach or attempted attack.
By incorporating these best practices, developers can create a more secure database environment and be better positioned to counteract threats.
Preventing SQL Injection Attacks
SQL injection is a notorious attack type where malicious SQL code is injected into a query, potentially exposing or compromising your database. Understanding how to prevent such attacks cannot be overstated. Here are some effective strategies to fortify your database against SQL injection:
- Use Parameterized Queries: Instead of directly interpolating user inputs into SQL statements, use parameterized queries. For instance, in Python:
This method separates code from data, effectively neutralizing any malicious code within user inputs.
- Sanitize Input: Always validate and sanitize user input. Employ whitelisting techniques and reject any suspicious entries. For instance, if expecting a username, confirm that it conforms to expected patterns.
- Error Handling: Ensure that error messages do not expose sensitive information about the database schema or queries. Custom error messages can help obscure the inner workings of your application.
- Web Application Firewalls: Deploying a firewall can help filter out malicious traffic, adding another layer of security in front of your database.
["Security is not a product, but a process."] This quote rings especially true in the context of database security. Regular assessments and updates are crucial in maintaining a secure environment.
Achieving a secure integration of MySQL with Python involves a concerted effort and proactive measures. By adhering to these strategies, developers not only protect their data but also bolster the integrity and trustworthiness of their applications.
Real-World Applications and Case Studies
Integrating MySQL with Python unlocks a world of possibilities for developers and companies striving for efficient data management. This section highlights how these technologies work hand-in-hand in real-world scenarios, offering practical insights on their implementation.
One significant focus in industry today is application development. Many organizations use Python with MySQL to build applications that require robust data storage and retrieval capabilities. For instance, e-commerce platforms might leverage this integration to manage product inventories and customer orders seamlessly. The ability to perform CRUD operations quickly means that changes to stock levels or customer information can be reflected in real time, enhancing user experience.
Consider another practical case: a data analytics company using MySQL to store large data sets from various sources while employing Python for analysis and insights extraction. This combination facilitates complex calculations and data visualizations, allowing businesses to make informed decisions based on current data patterns.
Moreover, the flexibility of Python libraries, such as Pandas and NumPy, can enrich data exploration and manipulation when fetching data from MySQL. Projects like these not only demonstrate an application of technical knowledge but also show how MySQL together with Python equips businesses with tools to adapt to changing landscapes.
Real-world applications of MySQL and Python improve data access speed, efficiency, and management reliability.
Through various enterprise-level applications, this section showcases how combining MySQL with Python isn't just a theory but rather a practical approach to tackle everyday business challenges.
Building a Simple CRUD Application
Building a simple CRUD (Create, Read, Update, Delete) application serves as a foundational project for anyone wanting to understand the practicalities of using MySQL with Python. This type of application is essential because it covers basic operations that are core to data management.
In the initial phase, setting up a MySQL database involves defining tables and relationships. For instance, an organization may have a user table to store personal information and another table for tasks that are related to each user. This initial structuring sets the stage for various relational operations.
Next, utilizing Python libraries like MySQL Connector or SQLAlchemy, one can easily facilitate interactions between the application and the database. The coding structure usually looks something like this:
In this example, a function is defined to create a new task. It's a simple but effective way to establish a contact point between application and database.
Applications like this help programmers and data enthusiasts better understand the dynamics of database operations and the attention to detail required in a live environment.
Integrating with Web Frameworks
Bringing together Python with web frameworks enables developers to create dynamic applications that can leverage MySQL for backend storage. Two prominent web frameworks that offer powerful integrations are Flask and Django.
Flask Integration
Flask is known for its lightweight and minimalist design, which makes it a popular choice for developers looking to build web applications rapidly. Flask aligns well with dynamic database interactions because it allows for flexibility in how applications are structured. This characteristic leads developers to enjoy a simplified setup process while still being robust enough to manage CRUD operations effectively.
A distinctive feature of Flask is its support for extensions. Developers can easily incorporate various modules into their applications to add features such as form validation, authentication, or even RESTful API functionalities. This adaptability means that when a Flask-based application integrates with MySQL, it can scale seamlessly as project requirements evolve.
However, Flask also comes with its challenges. As it provides less built-in functionality than a more heavyweight framework, developers must be cautious to ensure their code remains organized and maintainable over time.
Django and MySQL
Django stands in contrast as a full-stack framework, providing everything needed out of the box to build web applications with minimal configuration. Its ORM (Object-Relational Mapping) system is an impressive feature that simplifies database interactions significantly. With Django, developers can work more efficiently by translating Python objects into database tables without writing SQL queries explicitly.
The key advantage of using Django with MySQL lies in its speed of development. Projects can be kicked off in no time, and the framework's structure often leads to cleaner, maintainable code.
On the downside, such a comprehensive framework can sometimes feel overwhelming for simple applications. It requires a more in-depth understanding of its components, which can be an adjustment for some developers.
Data Analysis with MySQL and Python
Combining MySQL and Python for data analysis results in a powerful toolkit for handling large datasets. Python's extensive libraries, like Pandas and Matplotlib, strengthen the analysis process by enabling data manipulation and visualization with ease.
Data analysts often depend on MySQL to handle storage and retrieval efficiently. Once loaded into Python, datasets can be transformed and manipulated to extract insights quickly.
For instance, a data analyst could execute a SQL query that aggregates sales data by month and store the results in a Pandas DataFrame for further analysis. This kind of operation highlights the synergy of using both tools: MySQL for storage and management, and Python for analysis and interpretation.
Utilizing Python's library for visualizing the data adds another layer of understanding. Creating graphs and charts can help to tell stories that raw numbers alone can’t convey, thereby making informed decisions more accessible for stakeholders.
In summary, the applications of MySQL with Python range from full-fledged web applications to agile data analysis setups. Each scenario highlights the individual strengths of these technologies while showcasing their combined effectiveness in responding to real-world demands.
Ending
Integrating MySQL with Python is more than just a technical endeavor; it’s about enhancing the capability of applications to handle data effectively. As we stand back to examine all that has been covered, it's essential to appreciate the profound impact this integration plays in the realm of programming and data management.
First and foremost, the summary of key concepts expressed in this article serves as an essential anchor for aspiring and seasoned programmers alike. Each step, from installation to executing complex queries, underscores the critical role relational databases hold in applications. A strong grasp of CRUD operations and advanced management techniques empowers developers to build robust solutions that cater to real-world demands. Without a solid foundation in these areas, any application may struggle with efficiency and reliability, potentially hindering user experience.
Moreover, as we transition into future trends, it's clear that the integration of MySQL with Python will continue to evolve, particularly with advancements in technology and a growing emphasis on data analytics. Recognizing these trends is vital for staying relevant in a fast-paced industry. Enhanced tools and methods will likely arrive, offering developers even greater ease in handling complex data operations. Being ahead of the curve means developers can harness the newest innovations that drive efficiency and performance.
When considering the future of MySQL and Python integration, it's important to acknowledge the blend of data security and performance optimization. The techniques shared in this guide reinforce both operational prowess and necessary precautions in database management.