Implementing OAuth in ReactJS: A Comprehensive Guide
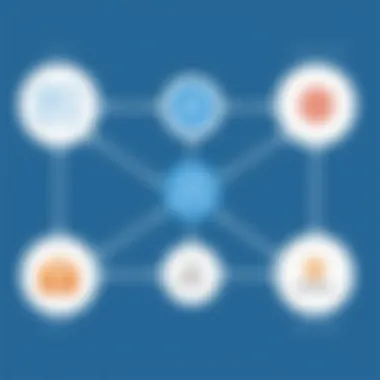
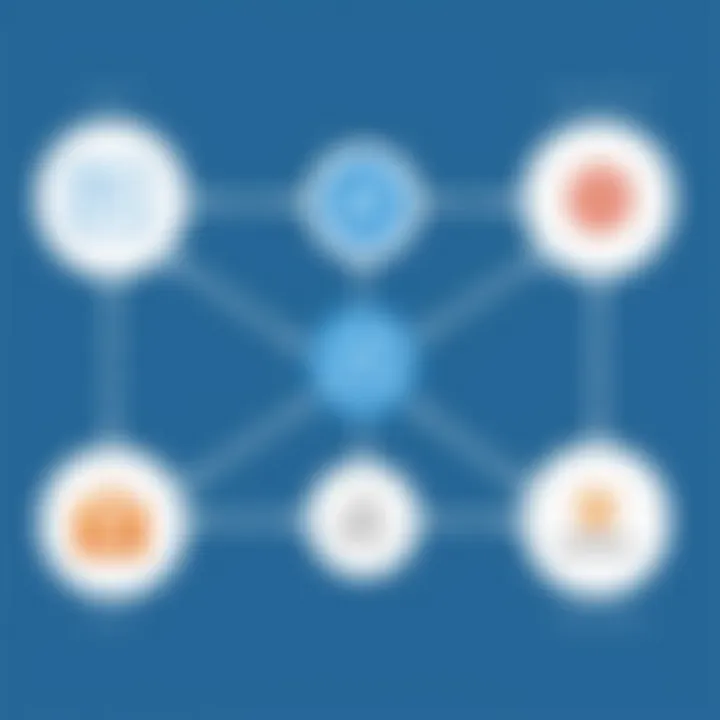
Intro
Implementing OAuth in ReactJS applications is increasingly vital for developers who prioritize secure user authentication. With numerous data breaches and privacy concerns, the need for robust authentication mechanisms is more pertinent than ever. OAuth serves as a widely accepted standard that allows for secure authorization without sharing credentials. This article aims to explore the various dimensions of OAuth in ReactJS, offering insights into its implementation, configurations, and best practices.
In the evolving landscape of web development, understanding OAuth is crucial for creating secure applications. This guide will walk you through every step of the process, starting from basic principles to complex integrations. It is tailored for both aspiring and experienced programmers, alongside technology enthusiasts and IT professionals, ensuring that it addresses the needs of a discerning audience.
Key Highlights of This Guide
- Understanding OAuth Protocols: An in-depth look at how OAuth works and its relevance.
- Configuration Steps: Detailed instructions for setting up OAuth seamlessly within ReactJS applications.
- Practical Examples: Real-world scenarios and examples guiding the integration process.
- Security Best Practices: Recommendations to fortify security and enhance performance.
- Troubleshooting Common Issues: Insights into resolving obstacles during the implementation phase.
By the end of this guide, readers will possess a comprehensive understanding of OAuth in the context of ReactJS, equipping them with the tools needed to enhance their applications with secure authentication mechanisms.
Intro to OAuth
OAuth, which stands for Open Authorization, plays a pivotal role in modern web applications, particularly in the context of user authentication within ReactJS applications. The demand for secure authentication mechanisms has surged as online services proliferate and user privacy concerns heighten. This guide presents a detailed examination of OAuth, highlighting its functionality, significance, and the benefits it brings to developers and users alike.
Implementing OAuth enables applications to access user data without directly exposing sensitive information like passwords. This introduces an extra layer of security. When an application uses OAuth, the user grants permissions without sharing their credentials with other applications. This separation of concerns not only enhances security but also streamlines the user experience.
Furthermore, OAuth has evolved into a standard protocol that numerous major platforms, such as Google, Facebook, and Twitter, utilize for authentication. An understanding of this protocol is essential for any developer working on applications that involve user data. The knowledge of OAuth contributes to implementing best practices in user authentication that enhance app security and user trust.
In this section, we will explore the core concepts of OAuth and its historical context. This lay the foundation for the subsequent sections, where we will delve into the different OAuth flows and the specific implementation practices within ReactJS.
Understanding OAuth Flow
Understanding the OAuth flow is essential for a successful implementation of secure authentication in ReactJS applications. OAuth provides a framework that allows applications to obtain limited access to user accounts on an HTTP service, such as Google or Facebook, without exposing user credentials. Mastering the OAuth flow clarifies how these processes occur, which can significantly enhance application security and user experience.
The OAuth protocol functions through several flows, each tailored for specific use cases. Familiarity with these flows is critical. Each flow's structure determines how a user authorizes an application, leading to distinct security outcomes. Choosing the correct flow based on your application's requirements ensures that sensitive data is handled appropriately and reduces vulnerability to attacks.
Authorization Code Flow
The Authorization Code Flow is the most common OAuth flow used for server-side applications. This flow requires an intermediate code generated upon user authentication, which is then exchanged for an access token. Here’s how it works step by step:
- User is redirected to the OAuth provider's authorization endpoint, requesting access to their data.
- User authenticates and grants permission for the application to access their information.
- Authorization code is sent back to the application via a redirect URI.
- Application exchanges the authorization code at the token endpoint for an access token and, optionally, a refresh token.
This flow is secure due to the client secret which is exchanged only on the server. It mitigates risks of access tokens being exposed as the tokens are not handled by the user's browswer.
Implicit Flow
The Implicit Flow is designed for applications running in a user's browser, particularly single-page applications. Unlike the Authorization Code Flow, it does not involve the intermediary step of providing a code. Instead, the flow directly returns an access token as part of the redirect URI. Here’s how it operates:
- User is redirected to the authorization server.
- Upon authentication, the server redirects the user back to the application's redirect URI, including the access token in the URI fragment.
- The application extracts the access token from the URL fragment for use in API calls.
While the Implicit Flow is simpler, it has inherent security risks. Access tokens are exposed in the URL, which can be intercepted. Therefore, it is recommended for applications where security is less critical.
Resource Owner Password Credentials Flow
The Resource Owner Password Credentials Flow is a less common flow used in highly trusted applications where the user can safely give their credentials to the client. This flow allows the application to directly request the user's credentials. The steps are:
- User provides a username and password directly to the application.
- The application submits these credentials to the OAuth server.
- Upon validation, the server returns an access token.
While convenient, this flow has serious security concerns. The application must be fully trusted by the user to handle their credentials. As a result, this flow should be limited to trusted first-party applications only.
Client Credentials Flow
The Client Credentials Flow is geared toward machine-to-machine communication. It allows applications to authenticate to the OAuth server using their own credentials rather than user credentials. The steps include:
- The application requests an access token using its own client ID and client secret.
- The OAuth provider validates the credentials and issues an access token.
This flow is useful for applications, like backend services, that need access to APIs without user involvement. It's essential to restrict access based on granted scopes to ensure security in this scenario.
Each OAuth flow has its own advantages and trade-offs. Selecting the right flow based on the context of the application is paramount for both security and functionality. As applications become increasingly complex, understanding these flows and their implementation can lead to more robust and secure authentication processes.
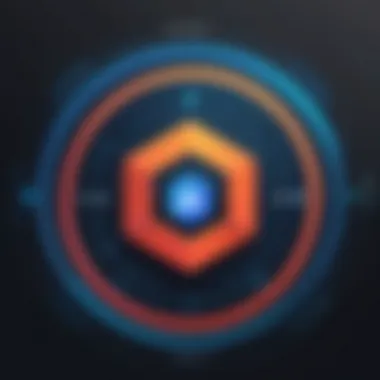
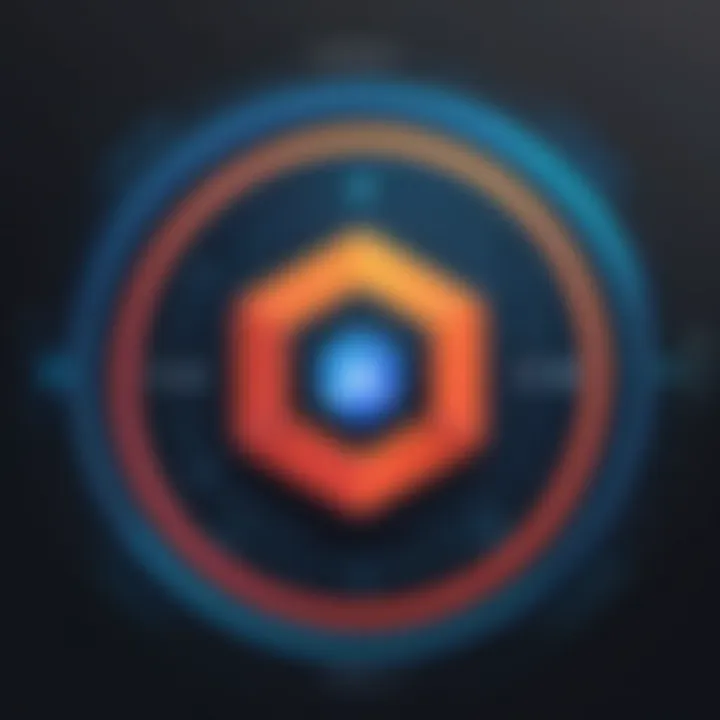
Setting Up a ReactJS Application
Setting up a ReactJS application is a fundamental step when implementing OAuth. The importance of this phase cannot be understated. It forms the groundwork for how you will integrate the authentication process into your application. A clean setup helps to manage dependencies, organize the project structure, and ensures a smoother development experience. Each part of the application should work harmoniously to create a secure environment for user authentication.
Moreover, a well-structured React application enhances both scalability and maintainability. The right setup can save time in the long run and improve the overall efficiency of the application. This section will guide the reader through the process of creating a new React project and integrating crucial libraries needed for implementing OAuth.
Creating a New React Project
To start, you need to create a new React project. This can be achieved simply using Create React App, which is a popular tool for setting up React applications quickly. It sets up the environment with the necessary configurations and scripts, easing the initial setup process. To create a new project, you can execute the following command in your terminal:
Here, is the name of the application. After executing this command, a new folder will be created containing all the essential files and dependencies. This structure follows modern development practices, which is beneficial as it prepares you for future enhancements.
Once the project is created, navigate to your project folder and start the development server with the command:
This command will open your new React application in the default web browser, allowing you to see changes in real time as you develop.
Integrating Necessary Libraries
After creating a React project, the next step is integrating essential libraries that facilitate easier implementation of OAuth. There are three pivotal libraries to consider: React Router, Axios, and OAuth-specific libraries. Each serves a distinct purpose in ensuring that your application can handle user authentication securely and efficiently.
React Router
React Router is crucial for navigating within a React application. It allows developers to define multiple routes and link them seamlessly. This is important in a scenario involving OAuth, where you need to redirect users to the authentication provider and handle redirects back to the application after authentication.
A key characteristic of React Router is its declarative routing approach. This makes managing routes straightforward and concise. You can define routes in a simple JSX syntax, which is a beneficial aspect for maintainability. React Router supports dynamic routing, which can be a unique feature when creating paths based on user roles or states. However, it can be complex when deeply nested routes are involved, leading to potential confusion if not managed properly.
Axios
Axios is a promise-based HTTP client for making requests to APIs. In the context of OAuth, Axios is vital for communicating with both the OAuth provider and your backend service. Its ease of use and ability to handle requests and responses effectively makes it popular among React developers.
One notable feature of Axios is its ability to intercept requests and responses. This can be handy for attaching authentication tokens to requests before they are sent. Another strength lies in its automatic JSON data transformation, simplifying data handling. However, one disadvantage may be the addition of a size overhead due to bundled dependencies, impacting loading times slightly.
OAuth Libraries
Integrating OAuth libraries is essential for streamlining the authentication process. Libraries such as OAuth.js or React-OAuth-Login provide pre-built methods to handle OAuth flows. This reduces the complexity of implementing these flows yourself.
The key characteristic of these libraries is that they abstract away much of the boilerplate code typically required for OAuth connections. This allows developers to focus on their application logic instead of the intricacies of the OAuth protocol. A unique feature is the ability to maintain sessions seamlessly without needing to refresh tokens manually, thus, improving user experience. Nevertheless, some OAuth libraries may limit flexibility, potentially forcing developers to adapt their strategies to fit the framework’s defined patterns.
Setting up your ReactJS application with these libraries establishes a secure base for implementing OAuth. With the right setup, you pave the way for a smooth authentication experience, ensuring users can log in seamlessly and securely.
OAuth Provider Configuration
OAuth provider configuration is a crucial step in implementing OAuth within a ReactJS application. This section discusses the key elements of this process and highlights its significance in establishing a secure authentication system. Proper configuration with the OAuth provider ensures that the application can authenticate users effectively, maintain security, and reduce the risk of potential vulnerabilities.
Registering Application with OAuth Provider
When starting with OAuth, the initial step involves registering your application with an OAuth provider. This procedure may seem straightforward, but it plays a pivotal role in ensuring secure interactions between your application and the provider. Each OAuth provider has its specific registration requirements, yet the general process involves visiting the provider's developer portal and filling in required details about your application. Details often include the application's name, description, and website URL. Also, you will need to specify the redirect URI which is necessary for handling callbacks after a user successfully authenticates.
- Visit the OAuth provider’s developer portal. Popular options include Google, Facebook, and GitHub.
- Create a new application in their system. This usually requires basic information about your app.
- Specify required permissions or scopes depending on what data you want to access.
- Set the redirect URI to ensure the authorization response returns to your application correctly.
Ensuring that the information is accurate during registration expedites the approval process. Keep in mind that some providers might take longer for the application to be approved. Thus, it’s prudent to initiate this process early in development.
Understanding Client and Client Secret
After successfully registering your application, you’ll receive a client ID and client secret. These components are unique identifiers for your application and are critical for authenticating API requests. The client ID serves as a public identifier for your application, while the client secret is a confidential key that should be kept secure. If anyone gains access to the client secret, they may impersonate your application and make unauthorized API calls.
- The Client ID is used to identify your application during the OAuth flow.
- The Client Secret, however, should be treated like a password. It must not be hardcoded in the front-end code or exposed to client-side access.
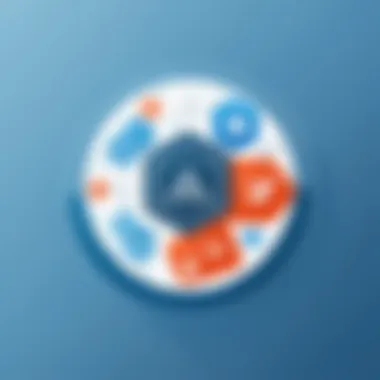
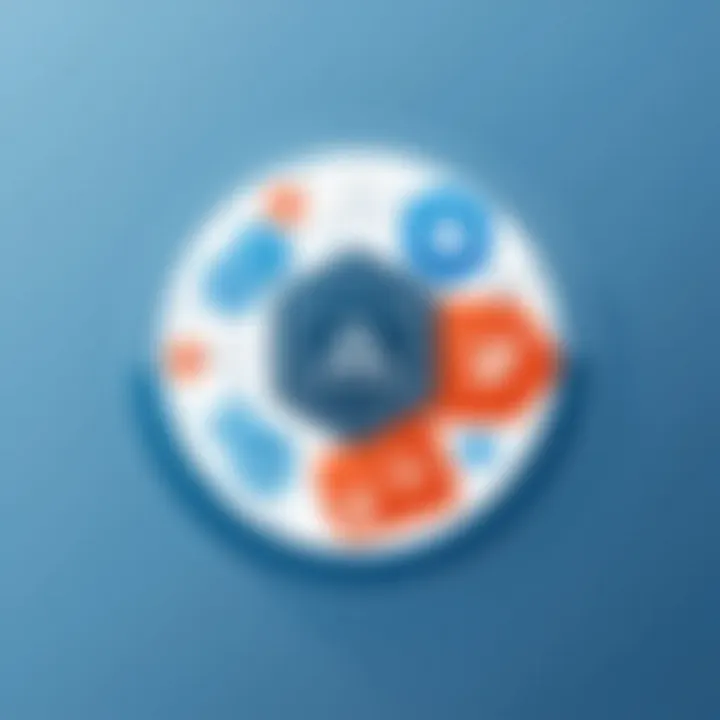
Including sensitive information such as a client secret in repositories or frontend code increases the risk of data breaches. Therefore, it is advisable to use environment variables or secure vaults in production settings.
Important: Always ensure that you protect your client secret, as it serves as a gatekeeper to your application's permissions.
By understanding these identifiers, you not only prepare your application for secure interactions with the OAuth provider but also establish a foundation for protecting user data during the authentication process. In summary, proper OAuth provider configuration is integral for successful user authentication, ensuring that your users' data remains secure.
Implementing OAuth in React
Implementing OAuth in React is crucial for modern web application security, particularly when it involves user authentication. OAuth protocols allow developers to delegate authorization to third-party services without exposing sensitive user credentials. This integration is vital not just for user safety but also for enhancing the overall user experience. By employing OAuth, applications can authenticate users seamlessly through well-known platforms such as Google or Facebook, significantly reducing friction during sign-ins.
In this context, there are specific elements to consider when incorporating OAuth into a React application. Developers must focus on building a robust authentication flow, securely storing tokens, and regularly refreshing them to maintain session integrity. Additionally, understanding the differentiation between various OAuth flows is beneficial for choosing the right strategy that aligns with the application's requirements. Overall, implementing OAuth in React not only fortifies security but also facilitates broader user engagement and satisfaction.
Building the Authentication Flow
Building the authentication flow is a foundational step in implementing OAuth in a React application. This process involves several key actions, from redirecting users to the OAuth provider to handling callbacks.
Redirecting Users to OAuth Provider
Redirecting users to an OAuth provider is a pivotal action that initiates the authentication process. Users must be directed to the OAuth service they wish to authenticate through. This step not only serves to enhance security but also improves usability. By leveraging a familiar login interface, users can access their accounts without having to create new ones.
A key characteristic of this redirection is the implementation of specific URL endpoints provided by OAuth services. This is a beneficial choice, as it shifts the responsibility for user authentication to trusted entities, mitigating risks associated with password management.
The unique feature of redirecting users is its automation of the authentication process. Developers do not need to manage sensitive credentials directly. Instead, users input their credentials on the OAuth provider's platform and are subsequently redirected back to the application with authorization codes. While this approach is generally secure, it does introduce possible vulnerabilities if redirect URIs are not properly configured.
Handling Callback and Tokens
Handling callbacks and tokens is integral to the authentication flow. Once the user has authenticated with the OAuth provider, the provider redirects them back to the application. At this stage, the application must capture the authorization code returned.
This capability is essential, as it allows the application to request access tokens. A critical aspect of handling callbacks is to ensure that the tokens are stored securely and dealt with appropriately. By effectively managing tokens, the application can maintain a user's session without requiring repeated login attempts.
A unique feature of handling callbacks is the ability to obtain refresh tokens as well. This can extend user sessions without requiring them to log in again. However, improper handling can expose tokens to security threats, emphasizing the need for careful development practices.
Storing Tokens Securely
Securely storing tokens is paramount to ensure the integrity and privacy of user data. When tokens are stored insecurely, they become vulnerable to theft and misuse.
There are several methods to store tokens, including local storage, session storage, and cookies. Each has its own set of advantages and disadvantages, which developers must consider based on the application's requirements. Storing tokens in memory is often the most secure, as it limits the exposure window to the duration of the session.
Encrypting tokens before storing is also a valuable practice. This ensures that even if unauthorized access occurs, the tokens remain protected. Moreover, adhering to the principle of least privilege when storing tokens can further bolster security. By minimizing the lifespan and scope of stored tokens, developers can reduce the risk associated with unauthorized access.
In summary, effectively implementing OAuth in React requires a comprehensive understanding of the authentication flow, including redirecting users, handling callbacks, and securely managing tokens. With these elements in place, developers can build robust applications that prioritize both security and user experience.
Best Practices for OAuth in ReactJS
Understanding and implementing OAuth within your ReactJS applications is only part of the equation. To truly harness its potential, you need to follow best practices, ensuring secure authentication mechanisms while delivering a smooth user experience. Addressing these considerations bolsters your application’s security posture and helps avoid common pitfalls. Focusing on best practices can significantly impact how effectively users authenticate, especially in a landscape where security threats are ever-present. This section will cover three key practices: secure storage of access tokens, reducing token exposure, and regular token refresh strategies.
Secure Storage of Access Tokens
Secure storage of access tokens is a critical element of maintaining security in OAuth implementations. Access tokens are the keys that provide access to protected resources. If these tokens are compromised, attackers could gain unauthorized access, putting user data at risk.
There are several methods to secure access tokens:
- Use Secure HTTP Only Cookies: Storing tokens in cookies flagged as HttpOnly can protect them from being accessed by JavaScript, thereby reducing the risk of cross-site scripting (XSS) attacks.
- Local Storage vs. Session Storage: Both give access to JavaScript, but they have different lifetime considerations. Session storage persists until the tab or window is closed, while local storage persists even after the browser is closed. This distinction can guide decisions based on the application's needs.
- Secure Environment Variables: If tokens must be stored server-side, ensure they are kept in secure environment variables to mitigate exposure.
Protecting access tokens must be taken seriously. Using these practices can prevent common vulnerabilities and create a sturdier security framework for your application.
Reducing Token Exposure
Reducing token exposure is another best practice that developers should prioritize. The less an access token is exposed, the lower the chance of it being intercepted. Here are strategies to consider:
- Limit Scope and Lifespan of Tokens: When obtaining tokens, always request the least privilege necessary. This means asking for only the permissions needed for the current operation. Similarly, setting a short expiry time on tokens limits their lifespan, thus reducing their value if stolen.
- Implement Token Binding: Tie access tokens to the client or device they were issued to. This can be achieved through techniques such as incorporating client information in the token users receive.
- Use HTTPS: Always serve your application over HTTPS. This prevents attackers from intercepting requests and gaining unauthorized access to access tokens while they are in transit.
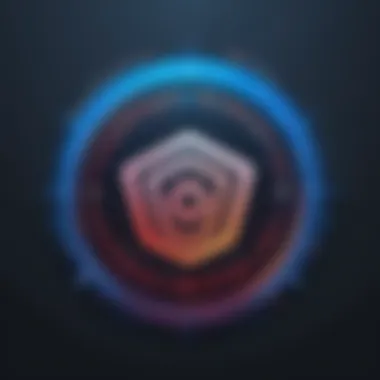
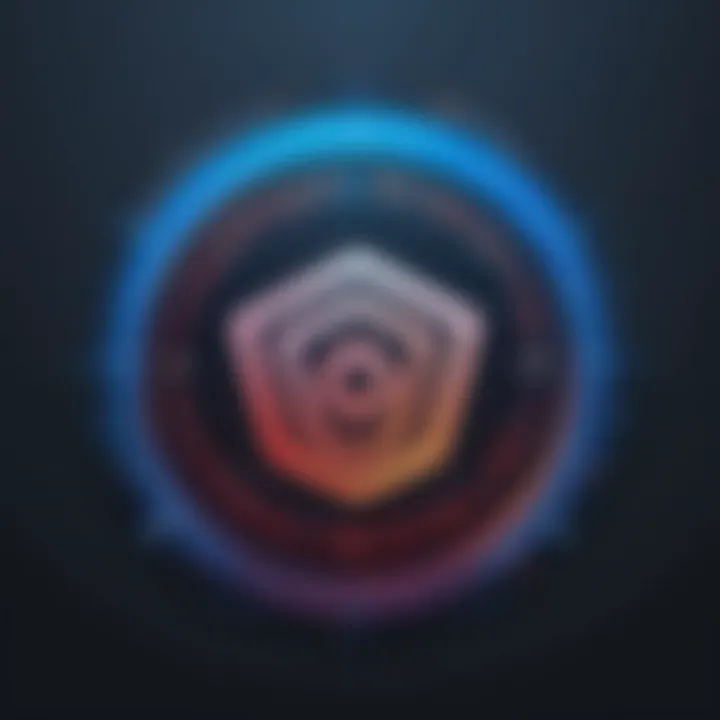
By employing these strategies, you can drastically decrease the opportunities for tokens to be exposed to malicious actors.
Regular Token Refresh Strategies
Token refresh strategies are essential for maintaining a user’s session while keeping security tight. Regularly refreshing tokens helps to ensure that access tokens do not linger long after they’ve been used.
- Refresh Tokens: Use refresh tokens to obtain new access tokens without requiring the user to log in again. This method provides a seamless experience while enhancing security.
- Automatic Token Refreshing: Implement an automatic process where your application checks the validity of an access token before making API requests. If the token is close to expiry, refresh it proactively. This helps ensure that users maintain a smooth interaction with your application.
- Graceful Handling of Expiration: Be ready for scenarios where an expired token might lead to an API call failure. Your application should handle this gracefully, prompting users to log in again if necessary.
Through careful consideration of token refresh strategies, one can maintain user sessions securely while minimizing user friction in the experience.
Following these best practices in OAuth implementation ensures that your ReactJS applications are robust and secure. Whether it's managing access tokens or reducing exposure, these actions contribute to a more secure user experience, which is essential in today's digital landscape.
Troubleshooting Common OAuth Issues
In any implementation of OAuth, challenges can arise that test your knowledge and skills in handling authentication effectively. This section dives into the common issues developers face when integrating OAuth in their applications. Understanding these challenges is crucial because effective troubleshooting can prevent downtime and improve user experience. Recognizing problems early and addressing them can streamline the authentication process, ensuring users are secure and satisfied.
Token Expiration and Renewal Failures
Token expiration is an inherent aspect of OAuth protocols. Access tokens are valid for a limited time to prevent unauthorized access. If a token is expired, attempts to use it will result in errors. This can create friction for users who expect seamless access to resources. To manage this, implementing a proper renewal mechanism is vital. The refresh token can be used to obtain a new access token without requiring user intervention. If the refresh token fails, it signifies a misconfiguration, an expired refresh token, or an improper scope.
To address this issue, consider the following steps:
- Ensure that you are storing tokens securely.
- Verify that the expiration time is considered in your logic.
- Check server responses for renewal errors and handle them appropriately.
If your application fails to renew access tokens properly, the user will inevitably face authorization issues. Being vigilant about token management is essential.
Redirect URI Mismatch Errors
Redirect URI mismatch errors occur when the URI specified in the OAuth provider's configuration does not match the URI provided during the authentication request. This mismatch leads to a denial of access, causing confusion and frustration for both developers and users.
To prevent this error, ensure the following:
- Confirm the redirect URI registered with the OAuth provider matches the one used in your application.
- Maintain consistent casing since some providers treat URIs as case-sensitive.
- Review your application's environment; sometimes, development and production URIs differ.
Regularly updating your configurations and testing them can prevent these issues from arising during critical user interactions.
Handling API Call Failures Post-Authentication
After successful authentication, an application often needs to make API calls. However, users may still encounter failures when trying to access data. These failures can arise from various sources, like invalid tokens, expired permissions, or server-side issues.
To effectively manage this scenario, consider these practices:
- Implement error handling that identifies the type of failure and responds accordingly; this could mean re-authenticating users or informing them of necessary actions.
- Monitor API responses for consistent issues, which may hint at broader service disruptions.
- Keep clear logs of user actions before failures to identify patterns.
The End
In this section, we reflect on the overall significance of implementing OAuth within ReactJS applications. OAuth stands as a critical protocol, enabling secure user authentication across various platforms. As we have discussed throughout this article, its implementation is not merely a technical requirement but a pathway toward building trust with users. When an application utilizes OAuth, it assures users that their sensitive information is handled securely, reducing the risk of unauthorized access.
The key elements of OAuth implementation that developers should consider include secure token storage, regular token refresh strategies, and vigilant handling of potential issues. Each of these components plays a vital role in maintaining the integrity and security of user data. Effective handling of OAuth not only enhances your application's security but also improves user experience by providing seamless authentication flows.
Furthermore, the benefits extend beyond just user trust. A well-implemented OAuth system can simplify the authentication process for developers, allowing for easier management of user identities across various platforms. This convenience translates into enhanced productivity and focus on core application features rather than being bogged down by the intricacies of user management.
"OAuth simplifies the process of authenticating users while ensuring their data remains secure."
Summarizing Key Takeaways
To aid in the retention of critical information, here are the main points covered in this article:
- Understanding OAuth: Knowing what OAuth is and its history enables developers to appreciate its importance.
- OAuth Flows: Familiarity with various OAuth flows helps in selecting the right approach for different scenarios.
- ReactJS Integration: Setting up and configuring React applications for OAuth requires attention to detail, especially with libraries like React Router and Axios.
- Best Practices: Secure storage of tokens and reducing token exposure are essential for protecting user data.
- Troubleshooting Common Issues: Awareness of common problems aids in faster resolutions when issues arise post-authentication.
- Future of OAuth: Staying updated with trends ensures that applications remain secure and relevant.
Future Directions in OAuth Implementation
As we look ahead, several trends and advancements in OAuth implementation within ReactJS applications warrant discussion:
- Improved Standards: The ongoing development of OAuth standards aims to enhance security measures and ease the implementation process. This will help developers adopt modern practices with greater confidence.
- Integration with Emerging Technologies: As technologies such as blockchain gain popularity, integrating OAuth with these frameworks might offer novel solutions to user identity management.
- Focus on User Experience: Providing a transparent and less intrusive authentication flow remains an essential goal. The emphasis on user-centric design will likely shape future OAuth implementations.
- Enhanced Security Protocols: With the rising threat landscape, further innovations around secure authentication will become critical. Implementing stronger encryption and better validation methods will be paramount.
- Community Collaboration: As developers contribute to open-source projects, collaboration will drive collective improvements in libraries and tools used for OAuth.
The future of OAuth looks promising, filled with opportunities for enhancing security and usability in ReactJS applications. Keeping these directions in mind will ensure that developers remain at the forefront of secure web application development.