Unraveling the Importance of Python Tuples: A Detailed Guide
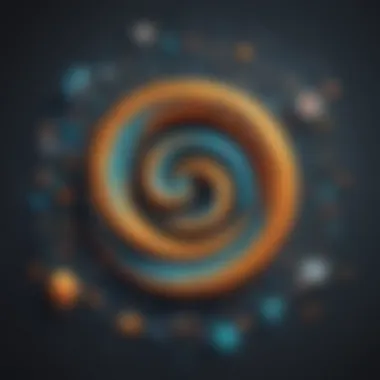
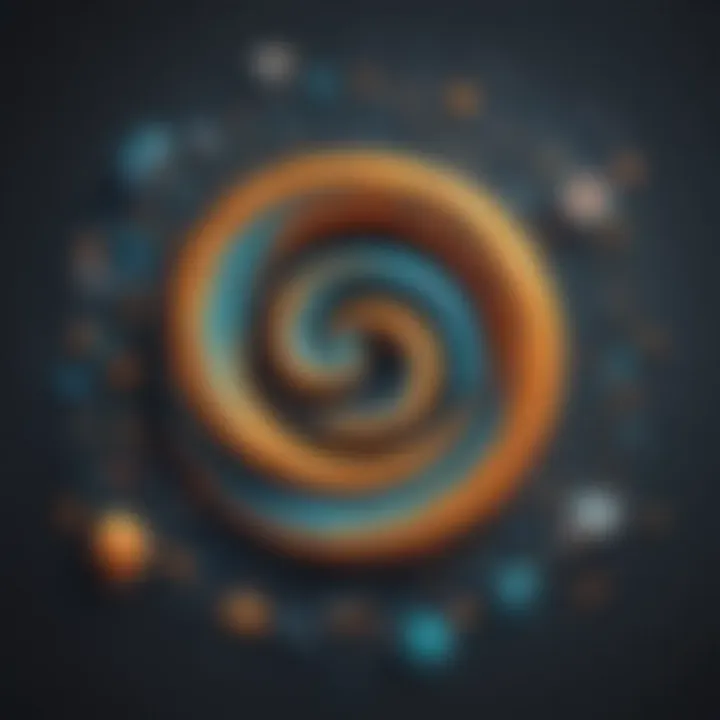
Coding Challenges
Tuples in Python can present fascinating challenges for both novice and seasoned programmers. Understanding the nuances of tuples, as immutable sequences, is essential for effective data manipulation and storage in Python. This section will delve into various coding challenges revolving around tuples, from handling nested tuples to exploring advanced tuple methods.
Weekly Coding Challenges
Each week, we will present a new coding challenge centered around tuples in Python. These challenges will test your ability to manipulate tuples efficiently, deepening your understanding of their unique characteristics and functionalities. Stay tuned for exciting puzzles and solutions that will sharpen your programming skills.
Problem Solutions and Explanations
Accompanying our coding challenges will be detailed solutions and explanations. Dive into the intricacies of each problem, unraveling the step-by-step process to arrive at the optimal tuple-based solution. Gain insights into best practices and clever techniques for working with tuples effectively.
Technology Trends
As technology continually evolves, the role of tuples in Python remains significant within the programming landscape. This section explores the latest technological trends shaping the use of tuples, alongside expert opinions on their impact and relevance in modern programming paradigms.
Latest Technological Innovations
Discover how tuples are integrated into the latest technological innovations across various industries. From data science to web development, explore cutting-edge applications where tuples play a pivotal role in streamlining processes and enhancing efficiency.
Emerging Technologies to Watch
Stay ahead of the curve by examining emerging technologies that leverage tuples for enhanced performance and scalability. Uncover how tuples intersect with machine learning, artificial intelligence, and blockchain technologies, offering versatile solutions for complex computational tasks.
Coding Resources
Empower your programming journey with a wealth of resources dedicated to mastering tuples in Python. From comprehensive guides to insightful tutorials, this section provides curated content that caters to your informational needs and skill development.
Programming Language Guides
Unravel the intricacies of tuples through comprehensive programming language guides. Explore in-depth explanations, code snippets, and practical examples that illuminate the versatility and practicality of tuples in Python programming.
Tools and Software Reviews
Discover essential tools and software designed to optimize tuple manipulation and utilization. From IDE recommendations to specialized libraries, enhance your programming workflow with insightful reviews and comparisons.
Computer Science Concepts
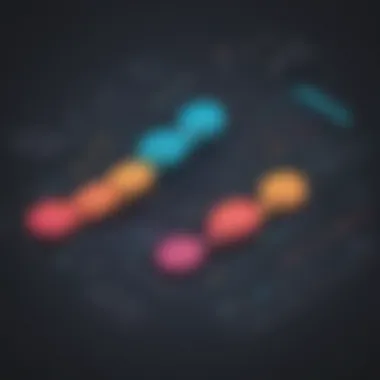
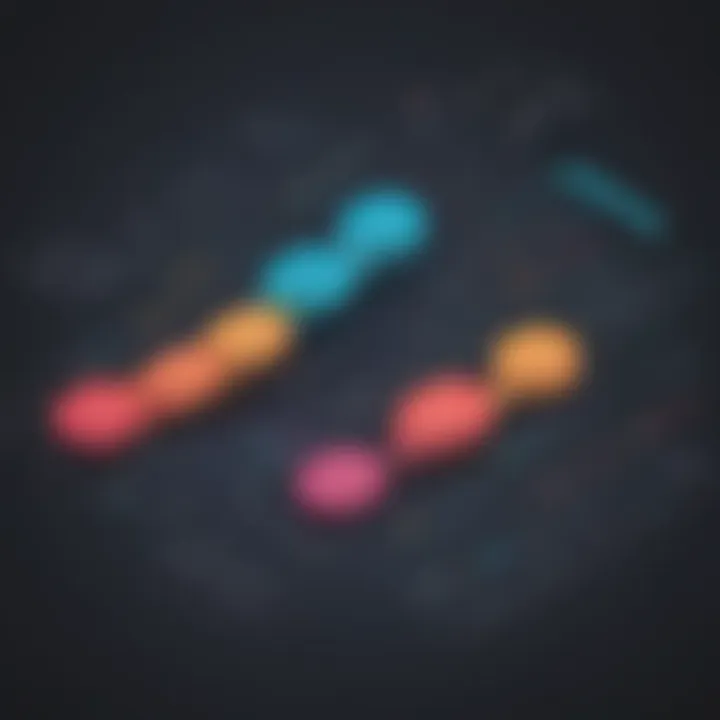
Delve into the fundamental principles of computer science through the lens of tuples in Python. This section elucidates key concepts such as algorithms, data structures, and artificial intelligence, demonstrating how tuples serve as foundational elements within the digital ecosystem.
Algorithms and Data Structures Primers
Understand how tuples intersect with core algorithms and data structures, forming the backbone of efficient computational processes. Explore the role of tuples in sorting algorithms, graph theory, and dynamic programming, enhancing your comprehension of fundamental CS concepts.
Artificial Intelligence and Machine Learning Basics
Embark on a journey through artificial intelligence and machine learning concepts intertwined with tuples. Investigate how tuples facilitate data representation, model interpretation, and feature engineering within AI algorithms, underscoring their crucial role in advanced computational frameworks.
Introduction to Tuples
In the expansive realm of Python programming, understanding tuples holds paramount significance. Tuples, as immutable sequences, play a pivotal role in data manipulation and storage within Python's domain. Delving into the intricacies of tuples allows programmers to grasp their essence and utilize them effectively in various programming scenarios. This section serves as a foundational exploration of tuples, shedding light on their defining characteristics and practical applications.
What are Tuples?
Definition of Tuples
The Definition of Tuples encapsulates a key aspect of tuple data structures in Python. Tuples are ordered collections of elements that are immutable, meaning their values cannot be changed after creation. This immutability feature ensures data integrity and reliability, making tuples a popular choice for scenarios requiring stable and unchangeable data. The static nature of tuples enhances the security and consistency of data storage, contributing significantly to their utility within Python programming.
Immutable Characteristics
Immutable Characteristics refer to the fundamental attribute of tuples that sets them apart from other data structures. The immutability of tuples guarantees that once created, their elements remain constant, offering a robust solution for preserving data integrity. While this unalterable nature provides consistency and security to stored information, it also limits the flexibility of dynamic data manipulation, posing a trade-off between stability and adaptability in programming contexts.
Syntax and Declaration
Tuple Creation
Tuple Creation signifies the process of initializing tuples in Python. Tuples are created by enclosing elements within parentheses, separated by commas. This simple syntax allows programmers to define tuples efficiently, leveraging their immutable properties for secure data storage. The ease of tuple creation aids in rapid prototyping and structured data organization, enhancing the readability and maintainability of codebases across diverse Python projects.
Accessing Tuple Elements
Accessing Tuple Elements refers to retrieving specific values from tuples for manipulation or analysis. Elements within tuples are accessed using indexing, with each element assigned a unique position starting from zero. This direct indexing approach empowers programmers to extract data seamlessly from tuples, enabling swift data retrieval and processing operations. By utilizing efficient element access methods, developers can harness the full potential of tuples for optimizing data-centric tasks.
Tuple Operations
Concatenation
Concatenation operation involves merging two or more tuples to create a new tuple with combined elements. This process concatenates the elements in the specified order, facilitating the consolidation of data from multiple sources into a single tuple. The ability to concatenate tuples enhances data aggregation and structuring capabilities, facilitating streamlined data manipulation and integration tasks within Python projects.
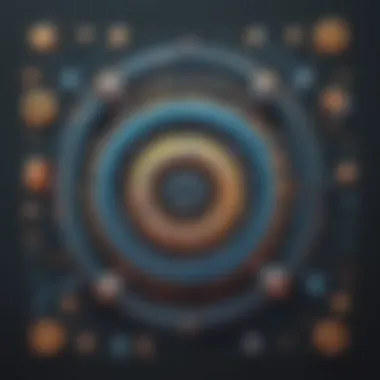
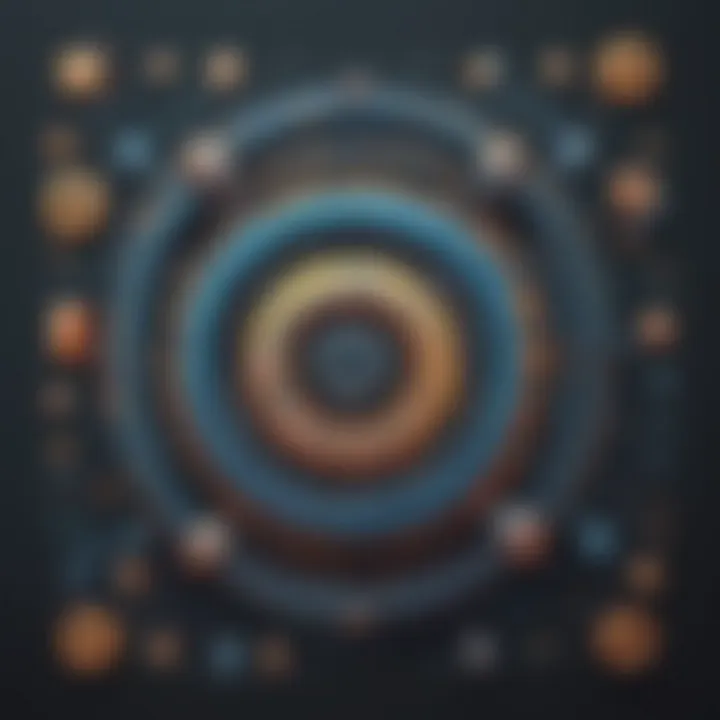
Repetition
Repetition operation enables the duplication of tuple elements a specified number of times to generate a larger tuple with repeated values. This function aids in scaling up data sets or creating patterns within tuples, offering flexibility in data arrangement and presentation. While repetition enhances data consistency and patterning, excessive use may lead to redundancy and inflated data sizes, warranting judicious application for optimal tuple management.
Understanding Tuple Methods
Importance of Understanding Tuple Methods in Python:
In this article, a focused examination of tuple methods is paramount to grasp the intricate functionality of tuples in Python. Understanding tuple methods sheds light on the manipulative capabilities of tuples, offering programmers a toolkit to enhance data manipulation and retrieval. By exploring common methods and their applications, readers can elevate their proficiency in working with tuples, underscoring their significance in scripting and programming.
Common Methods
count():
The count() method, a fundamental function within tuple operations, plays a pivotal role in determining the occurrence of a specific element within a tuple. This method underpins the identification of duplicate elements and facilitates statistical analysis by providing a straightforward means to quantify element repetitions. Its reliable and efficient nature renders it a popular choice in handling data analysis and categorization tasks, essential in diverse programming scenarios.
index():
A crucial element in tuple manipulation, the index() method allows users to swiftly locate the index of a specified element within a tuple. By enabling rapid and accurate retrieval of element positions, index() streamlines data processing and facilitates targeted element extraction. This method's precision and speed make it indispensable for tasks requiring precise element referencing, enhancing the overall efficiency of tuple handling in Python.
Appending and Updating Tuples
Immutable Nature:
The immutable nature of tuples ensures data integrity and consistency by prohibiting direct modification after creation. This inherent characteristic guarantees data stability and prevents inadvertent alterations, crucial for preserving the original dataset's sanctity. Although immutability restricts dynamic updates, it assures the reliability and consistency of tuple-based operations, aligning with Python's design principles of data security.
Workarounds for Modification:
To overcome the constraints imposed by tuple immutability, programmers employ workarounds for modification, such as tuple concatenation or conversion to mutable types for temporary modifications. These strategies enable selective alterations while preserving the tuple's overall structural integrity, striking a balance between data security and operational flexibility. Workarounds for modification furnish programmers with tailored solutions to cater to specific data manipulation requirements with deliberation and precision.
Practical Applications of Tuples
In the realm of Python programming, understanding the practical applications of tuples holds significant importance. Tuples, being immutable sequences, offer a unique set of benefits and considerations that make them essential in various programming tasks. One key element to note is that tuples provide a reliable means of preserving data integrity. This characteristic ensures that once data is stored in a tuple, it remains unchanged, thereby enhancing the security and reliability of information. Additionally, tuples are often utilized for function return values due to their efficiency in handling and conveying multiple pieces of data. This feature streamlines programming processes and enhances the readability of code. Furthermore, the memory management aspect of tuples plays a crucial role in optimizing performance and resource allocation within Python programs. By understanding and leveraging the practical benefits of tuples, programmers can enhance the robustness and efficiency of their codebase.
Data Integrity
Data integrity, a fundamental aspect of utilizing tuples in Python, plays a pivotal role in ensuring the correctness and reliability of stored information. By maintaining data in an immutable state, tuples prevent unintended modifications or alterations, thereby safeguarding the accuracy and consistency of data throughout the program execution. The immutable nature of tuples guarantees that once data is assigned, it remains unchangeable, reducing the likelihood of errors or inconsistencies. This feature makes tuples a popular choice for scenarios where data consistency is a critical requirement, such as handling configuration settings or maintaining historical records. While the inability to modify tuple elements may pose limitations in certain contexts, the advantages of preserving data integrity outweigh any potential drawbacks, making tuples a preferred data structure for maintaining data consistency and reliability.
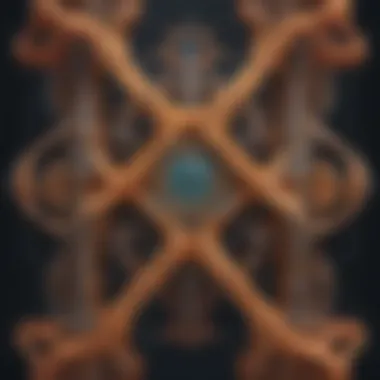
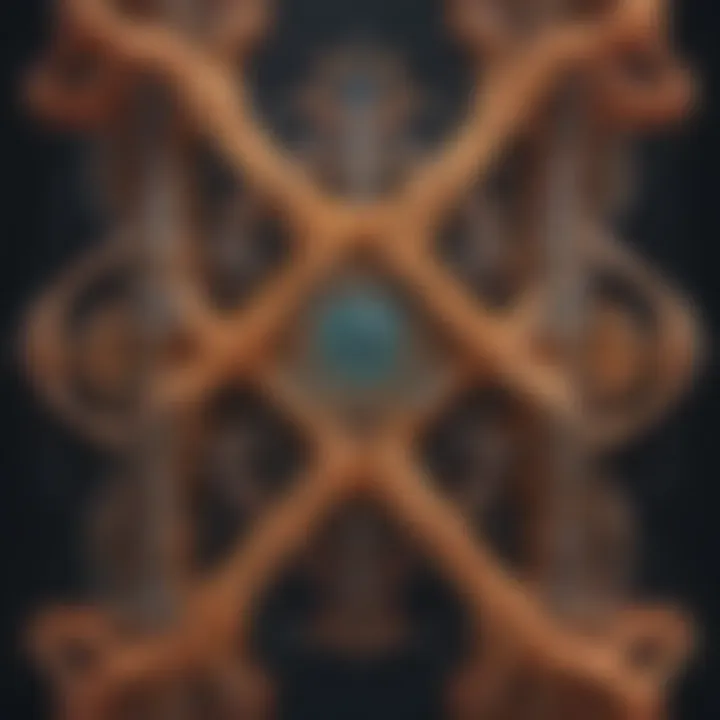
Function Return Values
Function return values represent another vital aspect of tuples in Python, offering a convenient way to return multiple values from a function call. By structuring function outputs as tuples, programmers can encapsulate and transmit multiple data elements seamlessly, enhancing code readability and organization. This approach simplifies the process of handling and unpacking returned values, especially in scenarios where functions need to convey diverse outcomes or parameters. The ability to return tuples as function results contributes to improved code clarity and maintainability, allowing developers to streamline their programming logic and communication. While the immutable nature of tuples may restrict the direct modification of return values, this characteristic promotes data consistency and prevents inadvertent alterations, aligning with best practices for function output handling.
Performance Benefits
Exploring the performance benefits associated with tuples in Python unveils valuable insights into their efficiency and resource management capabilities. Efficiency in processing tasks stands as a significant advantage of utilizing tuples within programming contexts. The inherent structure of tuples facilitates swift data manipulation and retrieval, contributing to enhanced algorithm efficiency and execution speed. By leveraging tuples for processing-intensive operations, programmers can optimize computational resources and improve the overall performance of their applications. Furthermore, memory management represents a critical aspect of tuple usage, influencing the allocation and deallocation of system resources. Tuples excel in memory efficiency by requiring minimal overhead for storing data elements, thereby reducing memory footprint and enhancing system responsiveness. This efficient memory utilization fosters streamlined program execution and enables developers to build resource-efficient applications with optimized memory usage.
Comparing Tuples with Other Data Structures
In the realm of Python programming, comparing tuples with other data structures holds immense significance. Tuples, being immutable sequences, differ notably from lists and dictionaries in terms of mutability and key-value pair characteristics. Understanding these variances is crucial for programmers to make informed decisions regarding data manipulation and storage. Tuples, characterized by their immutability, provide a stable and secure means of data storage, contrasting with the mutable nature of lists and the key-value structure of dictionaries. While lists offer flexibility for data modification and dictionaries excel in key-based data retrieval, tuples stand out for their constant and reliable data preservation.
Tuples vs. Lists
- Mutability Contrast: The key aspect of mutability contrast lies in the fundamental difference between tuples and lists. Tuples, once created, cannot be changed, making them ideal for scenarios requiring data integrity and consistency. On the other hand, lists allow for dynamic changes to elements, offering versatility but potentially compromising data stability. The immutable nature of tuples ensures that data remains fixed, preventing unintended modifications that could impact program behavior.
- Use Case Differentiation: Understanding the use case differentiation between tuples and lists is essential for effective programming choices. Tuples, with their immutable structure, are well-suited for situations where data should not be altered or tampered with. Lists, on the contrary, are preferred for scenarios requiring frequent element modifications. By leveraging tuples for safeguarding critical information and lists for fluid data manipulation, programmers can optimize data management processes and enhance code reliability.
Tuples vs. Dictionaries
- Key-Value Pair Analysis: The distinctive feature of key-value pair analysis in tuples and dictionaries lies in their organizational logic. While dictionaries excel in associating keys with corresponding values for efficient data retrieval, tuples provide a straightforward structure without key dependencies. Key-value pairs enable quick data access in dictionaries, making them ideal for scenarios emphasizing rapid information retrieval based on specific identifiers.
- Data Retrieval Efficiency: Highlighting the significance of data retrieval efficiency unveils the operational prowess of dictionaries compared to tuples. Dictionaries, by nature of their key-based retrieval system, offer swift and targeted data access, enhancing overall program performance. Tuples, while lacking the key-value pair mechanism, excel in preserving data integrity and coherence, albeit at the expense of direct key-linked information retrieval.
In essence, comprehending the nuanced distinctions between tuples and other data structures empowers programmers to make informed decisions aligned with their specific data handling requirements. By weighing the advantages and disadvantages of each structure, developers can strategically employ tuples, lists, or dictionaries based on the desired outcome and operational efficiency.
Best Practices and Recommendations
When delving into the realm of tuples in Python, understanding best practices and recommendations becomes paramount for efficient and effective programming. Optimal tuple usage requires a meticulous approach to data handling and storage. By adhering to well-defined practices, programmers can streamline their code and enhance its resilience. Implementing best practices ensures that tuple operations are carried out smoothly, without unnecessary complications. To achieve optimal tuple usage, practitioners must consider factors such as data integrity, performance efficiency, and memory management. By following established recommendations, programmers can harness the full potential of tuples in Python.
Optimal Tuple Usage
Selective Data Storage
Selective data storage plays a pivotal role in optimizing tuple usage within Python programming. This approach entails storing only essential data elements within tuples, enhancing data organization and access efficiency. Selective data storage minimizes unnecessary clutter and reduces the risk of data redundancy, leading to more streamlined code and improved performance. The key characteristic of selective data storage lies in its ability to prioritize critical information while omitting non-essential details. This deliberate selection process ensures that tuples remain concise and purposeful, contributing to more effective data management within Python applications.
Constant Data Preservation
Constant data preservation is another crucial aspect of optimal tuple usage in Python. By preserving data in a constant manner within tuples, programmers can ensure data consistency and reliability throughout program execution. This practice prevents inadvertent modifications to critical data elements, maintaining data integrity and program stability. The key feature of constant data preservation is its ability to safeguard essential information from unintended alterations or updates. Although this approach restricts data mutability, it reinforces the security and consistency of data stored in tuples, making it a preferred choice for applications requiring data immutability.
Coding Guidelines
Naming Conventions
Naming conventions play a significant role in dictating how variables, functions, and other program entities are named within the codebase. In the context of tuple usage, adhering to standardized naming conventions enhances code readability and maintainability. By following consistent naming practices, programmers can easily identify the purpose and context of tuples used in their code. The key characteristic of naming conventions lies in their ability to establish a uniform naming scheme across the codebase, facilitating collaboration and code comprehension among developers. While naming conventions promote code clarity, improper adherence may lead to confusion and ambiguity in tuple usage within Python applications.
Error Handling Strategies
Effective error handling strategies are essential for managing exceptions and unforeseen situations in Python programs utilizing tuples. By implementing robust error handling mechanisms, programmers can gracefully address errors and prevent program crashes. The key aspect of error handling strategies is their capacity to anticipate potential failures and provide corrective actions or fallback mechanisms. By incorporating comprehensive error handling techniques, programmers can enhance the robustness and reliability of their code when working with tuples. While error handling strategies mitigate the impact of unexpected scenarios, they also introduce additional complexity to the codebase, requiring careful consideration to maintain an optimal balance between error prevention and code simplicity.