Mastering Array Multiplication in Programming
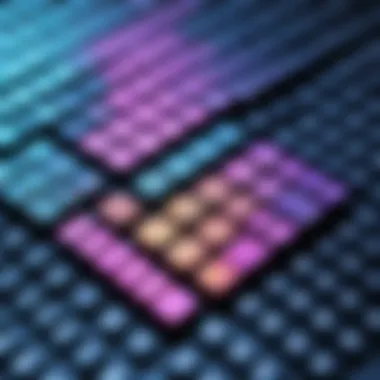
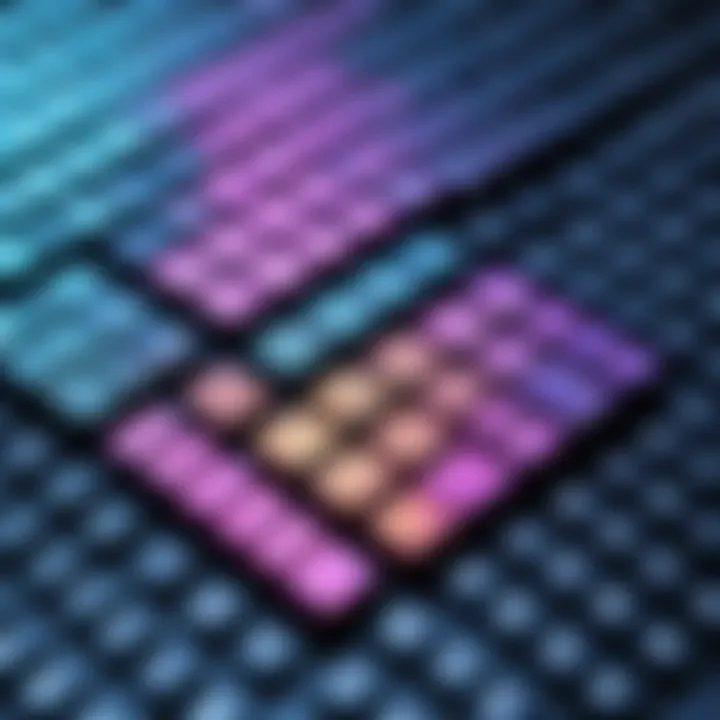
Intro
Multiplication of arrays plays a crucial role in programming and applications. Grasping this concept enables developers to manipulate data effectively. Learning to multiply arrays opens doors to advanced data handling techniques. This article aims to unpack the intricacies of this concept.
Coding Challenges
Developers frequently encounter coding challenges related to array multiplication. Comprehensive understanding can facilitate solutions. Participating in such challenges enhances skills and promotes learning.
Weekly Coding Challenges
To practice your skills, weekly challenges provide a structured approach. Problems focus on various scenarios, including how to handle different array sizes and shapes. These challenges encourage the exploration of different solving strategies.
Problem Solutions and Explanations
Analyzing solutions enhances understanding. Each problem usually has multiple solutions. Teaching notable techniques helps in grasping intricate concepts. This can include row by column multiplication in two-dimensional arrays. In two-dimensional arrays, yet again, this concept becomes fundamental:
- Validate the sizes to check if multiplication is possible.
- Calculate the dot product carefully to ensure accuracy.
It's relevant to add discussions about mistakes programmers often make with this topic.
Tips and Strategies for Coding Challenges
Developers can gain output by employing smart strategies:
- Understand the problem fully before diving into coding.
- Re-read the specifications to avoid basic mistakes.
- Break down problems into smaller, manageable parts.
Using these strategies, coders can navigate challenges more efficiently, aiding survival in the competitive landscape.
Community Participation Highlights
Active developers benefit from collaboration in coding communities. Diverse discussions help refine techniques and understanding. Platforms like Reddit and Discord offer valuable insights and critiques on problem-solving methods.
Engagement in community coding activities broadens perspectives on array multiplication and identifies new approaches to existing problems.
Coding Resources
To further expand your knowledge, utilize various coding resources designed for better comprehension. It includes manuals and tutorials across several platforms, notably:
- Programming Language Guides: Offered by diverse sites, such knowledge is essential.
- Tools and Software Reviews: Review notes on popular IDEs aids programmer workflow and productivity.
- Tutorials and How-To Articles: Focused specifically on learning multiplication approaches enhances your repertoire.
- Online Learning Platforms Comparison: A rich landscape for courses in programming.
Each resource can provide unique advantages, thereby enhancing understanding of multiplication in practice.
Computer Science Concepts
Delving into adjacent computer science concepts elevates high-level comprehension. Several major areas directly link to array multiplication include:
Algorithms and Data Structures Primers
Understanding algorithms enhances the efficiency of array handling. Knowing data structures aids in proper input organization. Fostering an environment for best practices empowers programmers.
Artificial Intelligence and Machine Learning Basics
In machine learning, data feature representation often uses arrays. Synthesizing arrays through multiplication can yield datasets that support algorithm functioning in complex scenarios.
Networking and Security Fundamentals
Efficient data processing matters in networking, dominating how array multiplication supports security features navigating various protocols.
Quantum Computing and Future Technologies
Though still evolving, quantum computing promotes unprecedented array manipulation opportunities, opening thoughtful explorations for programmers.
To summarize, grasping array multiplication holds tremendous worth. It provides a fundamental requirement across various applications in computer science. Understanding coding challenges, utilizing effective resources, and connecting to broader concepts is vital to becoming a proficient programmer.
Prolusion to Arrays
Understanding arrays is crucial for both novice and experienced programmers. Arrays serve as foundational data structures that allow for organized storage and access to multiple data points under a single name. They provide efficiency, enabling faster data manipulation and improved time complexity in various applications. Comprehending how arrays function sets the stage for more complex concepts, especially when exploring their multiplication, a core concern in a range of programming tasks.
Definition of an Array
An array is a collection of elements identified by index or key. It can hold data types like integers, strings, or even other arrays. This unique structure allows for quick access to an individual element when its index is known. The simplicity and effectiveness of arrays make them essential in programming, particularly for managing large datasets. Their fixed size typically facilitates memory management and prevents overhead, making accessing elements more efficient compared to standard data structures like lists.
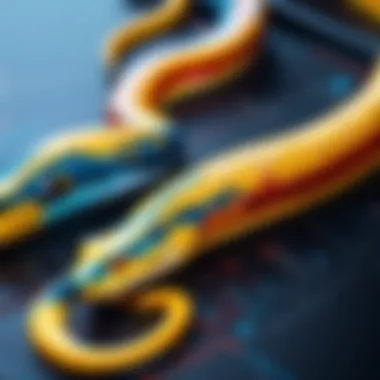
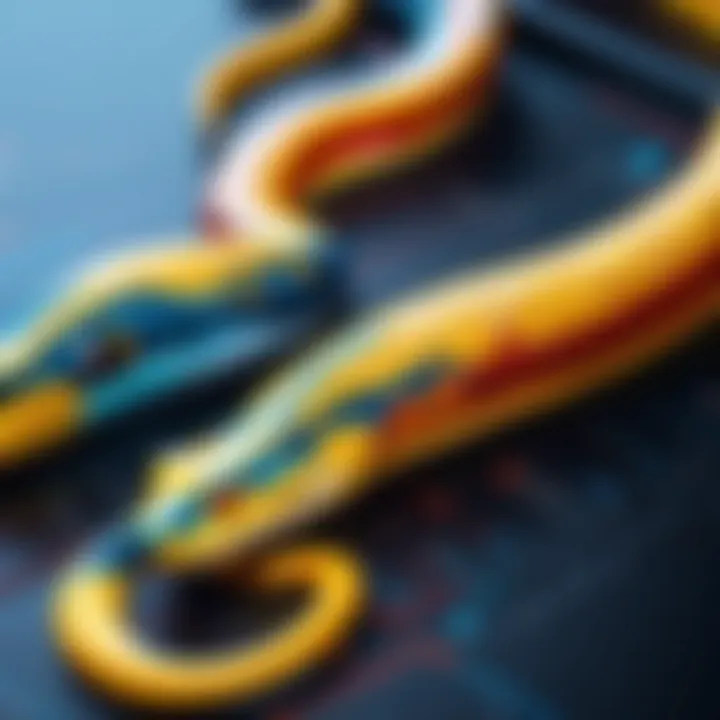
Types of Arrays
When it comes to arrays, variety plays an important role in catering to specific programming needs. Each type of array has distinct capabilities, ideal use cases, and features that shape their application.
One-Dimensional Arrays
One-dimensional arrays are the most straightforward form of arrays, consisting of a single row of elements. These arrays are not only easy to implement but also quite efficient in terms of memory allocation. A notable characteristic of one-dimensional arrays is their linear structure, allowing for rapid access to elements by referring to their index.
This type of array serves as a popular choice in many programming contexts due to its simplicity. When handling basic lists such as names or numbers, one-dimensional arrays offer a clear solution without additional complexity. However, they do have limitations, especially when we need to represent more complex data or relationships.
Multidimensional Arrays
Multidimensional arrays extend the capabilities of one-dimensional arrays, allowing developers to work with data in a grid-like fashion. This raises their usability across different scenarios, such as representing matrices or more complex datasets that require multiple dimensions.
What makes multidimensional arrays particularly powerful is their ability to manage data as collections, thus providing a structured method for addressing vast amounts of information. For instance, they shine in mathematical computations, simulations, or even dealing with images where multiple values must be monitored simultaneously. Nevertheless, their complexity can lead to a steeper learning curve for those unfamiliar with their structure and manipulation.
Jagged Arrays
Jagged arrays, often called
Mathematical Principles of Array Multiplication
The mathematical principles governing array multiplication are critical to algorithms used in programming and data analysis. Understanding these principles not only enhances a programmer's ability to manipulate data effectively but also underpins the efficiency of more complex operations. This foundation promotes rigorous thinking, which is essential in various fields such as machine learning, data processing, and scientific computing.
Since arrays serve as containers for numerous data types, their multiplication can perform more than mere arithmetic. It translates data from one form to another, facilitating tasks like transformations and aggregations. Overall, to master array manipulation, a solid grasp of its mathematical principles is invaluable.
Basic Concepts
At the core of array multiplication are fundamental mathematical concepts such as dimensions, indices, and operations specific to arrays. Knowing how the dimensions correlate during multiplication is crucial. For instance, in a matrix multiplication scenario, if a matrix A corresponds to dimensions (m x n), then a matrix B must have dimensions (n x p). The outcome will yield a new matrix C with dimensions (m x p).
Some key aspects to grasp include:
- Shape Compatibility: Ensure that the number of columns in the first matrix matches the number of rows in the second matrix.
- Element Arrangement: The arrangement of elements in arrays plays a crucial role; it affects the sequence and way products are derived.
- Indexing: Effective manipulation requires precise knowledge of index use, where each entry's position determines its contribution to the final result.
Matrix Multiplication
Matrix multiplication is a prime example of how array multiplication operates in mathematical contexts. Its method is not to multiply corresponding elements but to sum the products of elements from rows and columns.
To carry out matrix multiplication mathematically:
- Take the first row of the first matrix A and the first column of the second matrix B.
- Multiply each corresponding element together and sum those products.
- Repeat these steps for every row in A against every column in B.
Example: Let matrix A be:
And matrix B be:
The result of A * B:
The end product will yield:
This concept underscores the significance of dimensions as well as the resultant configuration during any array multiplication operation, making it essential knowledge for effective programming within technical frameworks like Python or Java.
Array Multiplication in ++
In C++, array multiplication should follow manual implementation as well. The language provides speed, which is an advantage, yet requires careful handling of pointers and memory. Libraries such as Eigen offer extensive features for a range of array manipulations.
Here’s a straightforward implementation using bare arrays:
In this case, the function multiplies two matrices and stores the result in another matrix using three nested loops. C++ offers direct control but requires strict adherence to the language's rules concerning memory management.
Additional Considerations:
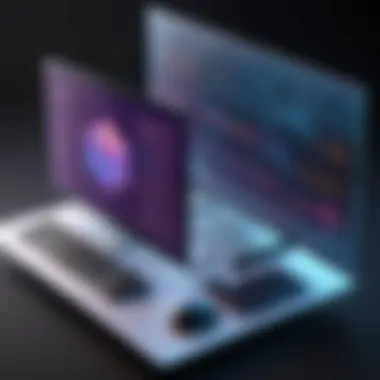
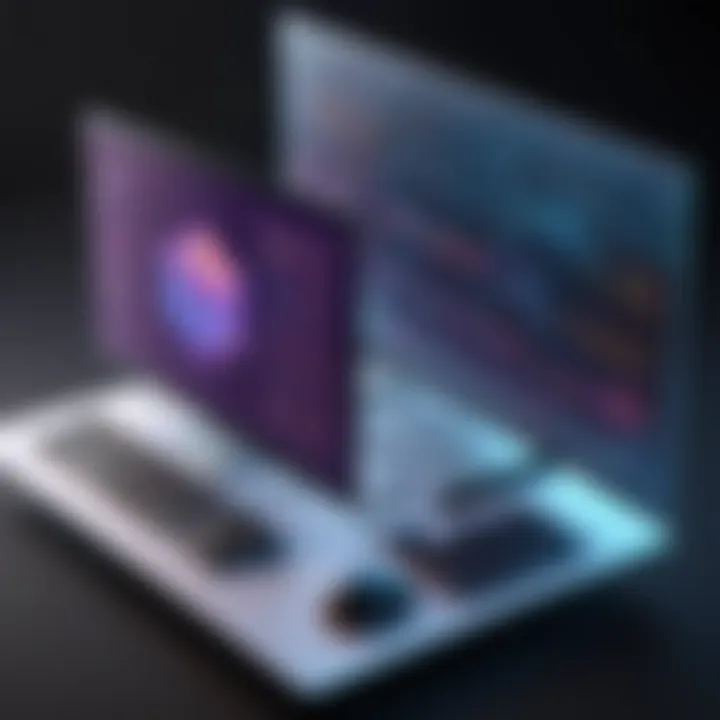
- Use appropriate array sizes to avoid overflow, especially with more extensive calculations.
- Efficiency increases with N-dimensional matrix libraries or optimized algorithms, which can further assist in handling more complex multiplication.
Challenges in Array Multiplication
Understanding the challenges in array multiplication is crucial for programmers at any level. This section focuses on potential pitfalls when working with arrays. A clear grasp of these challenges can lead to more efficient coding practices and better problem-solving skills. It also highlights the significance of rigorous testing to avoid common errors that can arise during implementation.
Common Errors
Common errors are one of the first obstacles encountered when working with array multiplication. These errors can cause unexpected results or even failures in code execution. Being aware of the issues makes it easier to preemptively tackle problems.
Dimension Mismatch
Dimension mismatch is especially pertinent when multiplying arrays. This error occurs when the dimensions of the arrays do not align appropriately. For example, an array with dimensions 2x3 cannot be multiplied directly with a 3x2 array without adjustments. This is fundamental in understanding how arrays interact with one another. The key characteristic of this issue is its potential to render an operation meaningless without creating valid dimensional alignment. Correctly addressing dimension mismatch is important for preventing runtime errors which can halt programs abruptly, disrupting workflows.
Some common solutions to dimension mismatch include reshaping an array or choosing different arrays. While reshaping can optimize a solution, users might overlook specific resizing restrictions that can inhibit overall efficiency.
Data Type Errors
Data type errors are another aspect that may lead to coding challenges. If the elements of the arrays involve incompatible types, the multiplication cannot proceed as expected. For instance, multiplying integers with strings creates logical issues. Understanding data types is vital because efficient programming involves accurate manipulation of various data models. Therefore, enforcing type matching frameworks when designing code can guard against this kind of flaw.
As benefits unfold in consistent type usage, programmers should appreciate the importance of strong typing. Potential downsides could arise when stricter rules require additional data conversions which also consume resources, leading to unnecessary complexity.
Performance Issues
Performance issues must be taken into account when engaging with array multiplication. Efficiency, both in terms of time and space, significantly influences the success of computation routines. Technologies that quickly process algorithms contribute positively to the evolution of programming, aiding in overall user experience.
Time Complexity
Time complexity describes how the execution time of an algorithm changes with the input size. When multiplying large arrays, it is foundational to understand the subtle variations in time complexity formulations. Knowing whether the sorting mechanism is O(n), O(n log n), or even higher influences choice in optimal programming techniques and data structures. This awareness helps programmers trade-off between speed and clarity in codebase while emphasizing efficient execution.
Commencing the early design stage of coding with time complexity in sight permits foresight into optimizing processes.
Space Complexity
Space complexity reflects the manner in which memory usage scales concerning the input size of an algorithm. While multiplying arrays, added dimensions multiply the requirements for holding data. The complexity measures both sum and use of temporary storage during operations. Finding a balance between time and space efficiencies becomes imperative while addressing bottlenecks in operations.
Implementing algorithms that respect space limitations can lead to vast improvements in speed and responsiveness, especially as applications scale.
Understanding these challenges leads to greater overall coding finesse and precision.
Optimization Techniques
Optimization techniques in array multiplication are vital for enhancing performance, reducing resource utilization, and ensuring output efficiency in computational tasks. Given that array operations, especially multiplication, can become intensive with larger data sets, knowing how to optimize these operations is essential. Optimum performance allows developers to create scalable solutions that are applicable in many scenarios, from scientific computations to machine learning.
Efficiency is not simply about quicker execution. It entails minimizing memory allotment as well. Both aspects directly relate to how arrays are manipulated and multiplied, where poorly optimized code may lead to unpredicted delays or crashes under load.
For any programmer tackling array multiplication, exploring optimization methods can reveal ways to streamline their processes. Algorithmic improvements often focus on refining existing procedures to reduce the complexity of multiplication operations.
Algorithmic Improvements
Improving algorithms for array multiplication involves finding faster or more resource-efficient methods to carry out calculations. Traditional approaches often use nested loops, which may be sufficient for smaller arrays but slow down considerably as size increases.
Efficient algorithms utilize advanced mathematical concepts. One such improvement is the Strassen algorithm, which reduces the number of necessary multiplications. Instead of relying solely on the overall complexity dictated by three nested loops, this method modifies how elements interact, cutting time consumption noticeably.
The Winograd algorithm also enhances performance compared with simple methods. While these improved algorithms increase complexity in understanding, the payoff is significant in medicine, finance, and larger artificial intelligence applications. Conclusively, seeking out such improvements affords satisfying outcomes.
Use of Libraries
Numpy (Python)
Numpy is a strong library often utilized for working with large arrays in Python. Its built-in functions for array multiplication divvy up complex tasks into simpler, more optimized steps. One key characteristic of Numpy is its low-level workspace, which frequently utilizes parallel processing techniques and SIMD (Single Instruction, Multiple Data) operations. This enhances performance far more effectively than standard Python capabilities.
A unique feature of Numpy is broadcasting. Broadcasting permits operations among arrays of differing sizes and dimensions without explicit replication of arrays. This attribute saves memory and execution time, allowing more extensive datasets to be processed effortlessly by the CPU.
Though Numpy benefits users substantially, it has disadvantages. These can include steep learning curves for novices, and the library does not operate efficiently with non-numeric data types, limiting use in concurrent development settings.
Apache Commons Math (Java)
Apache Commons Math is accomplished harnamer for Java. This library is oriented towards both developers as well as end-users who perhaps necessitate comprehensive mathematical functionalities. Its contribution toward enhancing array multiplication lays in its ability to provide prebuilt functions and more complex number manipulation vistas. One hallmark aspect is how its classes proficiently manages multidimensional arrays.
A crucial unique feature of Apache Commons Math is its array utilities. Such techniques can handle transforming, manipulating, and processing values with high execution speed, which further complements improving work with larger arrays.
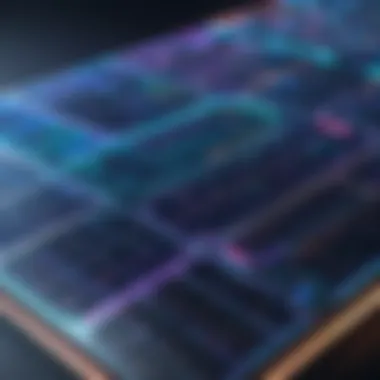
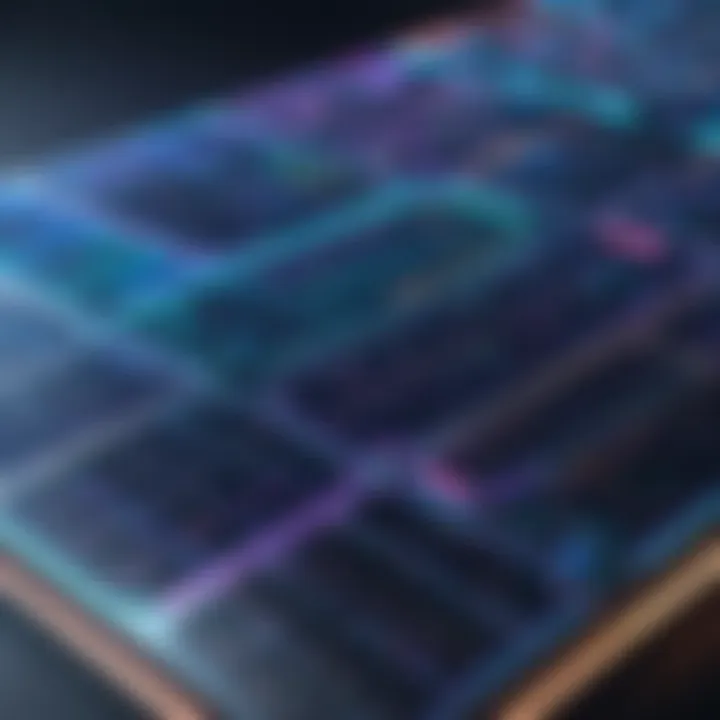
While the library is advantageous for performance-driven applications, it may be bulky and confusing for casual users. Moreover, its extensive arsenal leads to potential cognitive overload, pulling attention away from clarity and swift project execution.
In the landscape of array multiplication, efficiently these libraries speaks to a programmer's core requirement—performance and clarity optimized for best use across varying scopes and sizes of data.
In synthesizing the aforementioned, utilizing libraries like Numpy or Apache Commons Math elevates programmers' ability to handle array multiplication gracefully, consequently bridging theory with engaged practice in diverse programming languages while enhancing algronithm efficiency.
Practical Applications of Array Multiplication
Array multiplication plays a critical role in various fields of technology. Its applications spread across data processing and machine learning, both of which are essential to enhancements in data analysis and predictive modeling. Understanding these practical applications is vital for both aspiring and experienced programmers. The ability to employ array multiplication effectively can greatly influence the scope and speed of computational tasks.
Data Processing
Array multiplication significantly accelerates data processing tasks. In essence, it allows the manipulation of large datasets efficiently. For instance, when handling big data, the ability to multiply matrices comes into play when working with datasets that are conceptually multidimensional.
When multiple data inputs are processed, using array multiplication can achieve feats such as:
- Matrix transformations: It reduces the computational load and speeds up processing time.
- Statistical calculations: Many statistical operations for variance, covariance often require matrix manipulations.
- Simulations: In scenarios like financial modeling and risk analysis, forecasting can involve repeated multiplications of an large data array.
Here is a simple example using Python's NumPy library:
This code multiplies two matrices to produce new results that feed into further computation, demonstrating how array multiplication streamlines data workflows.
Machine Learning
In machine learning, array multiplication is indispensable. Most algorithms rely on identical operations to boost predictive performance. Models like neural networks extensively utilize arrays for weight applications and data inputs.
The impact of array multiplication in machine learning can be outlined as follows:
- Feature scaling: Data is often transformed in specific formats. Flattening data and applying matrix multiplications standardizes efficiently.
- Neural networks: Activation functions require consistent use of multiplication across layers, where arrays represent weights and inputs.
- Gradient descent: During optimization, the objective function is adjusted using derivatives with arrays. Efficient computation allows for quicker convergence toward optimal solutions.
For a basic view in TensorFlow, here’s how we see its use:
This action reflects transitioning upon layered-based structures formed when developing machine learning solutions. The necessity for rapid array computation confirms the significance of mastering array multiplication to tread the evolving space of artificial intelligence and data analysis.
Understanding array multiplication does not only accelerate computations; it fundamentally influences advancements in data-centric approaches across technologies.
Advanced Topics
Understanding advanced topics associated with array multiplication is crucial for programmers who wish to enhance their skills in computer science. This section aims to elucidate multidimensional arrays and their influence on algorithm efficiency. These concepts hold fundamental importance in various applications and areas of study in computing and data processing.
Multidimensional Arrays
Multidimensional arrays are an extension of the basic array unit, enabling developers to store data in more than one dimension. This additional complexity allows for more sophisticated data structures, like matrices, which can be beneficial in areas such as scientific computing, graphics, and machine learning.
- Structure and Representation: Multidimensional arrays are often represented as arrays of arrays. For example, a two-dimensional array can be visualized as a table, where each row represents a different dataset. This structure is helpful in keeping related data grouped systematically.
- Memory Layout: The way multidimensional arrays are stored in memory impacts the computational efficiency. Different programming languages, such as C++ and Java, store these arrays in different formats – either row-major or column-major order. Understanding the layout allows for optimizing memory access, thus increasing performance.
- Applications: The practical applications of multidimensional arrays are diverse. In image processing, for instance, an image can be stored as a two-dimensional array where each entry corresponds to a pixel's color value. In biological studies, multidimensional arrays are leveraged for handling complex datasets, such as genomic data.
Impact on Algorithm Efficiency
The impact of array multiplication on the efficiency of algorithms can be significant. When working with large datasets, the choice of algorithm and data structures can critically affect overall system performance. Here are key considerations:
- Parallel Processing: Array multiplication often benefits from parallelism. Using frameworks like OpenMP in C++ or multiprocessing libraries in Python can drastically decrease computation time. In modern computing environments, vectorized instructions in CPUs excel at handling array operations, leveraging parallel computation effectively.
- Algorithm Complexity Analysis: The time complexity associated with multiplying multidimensional arrays can be high, typically O(n^3) for matrix multiplication using naive methods. However, more sophisticated algorithms, like Strassen's algorithm, can reduce this to approximately O(n^2.81), thus demonstrating notable performance potential for large matrices.
- Space Complexity: Additionally, one must consider the space complexity when dealing with multidimensional arrays. Redundant storage of array data or temporary variables can lead to inefficiencies in memory usage. It is important to manage memory allocation skillfully to maintain overall system responsiveness.
The understanding and application of array multiplication in fields such as machine learning, computer graphics, and data analysis not only enhance algorithm efficiency but also make it possible to work with complex data types easily.
In summary, the advanced topics related to multidimensional arrays and their impact on algorithm efficiency play a pivotal role in shaping the performance and feasibility of programming solutions. These topics merit further exploration for developers aiming for proficiency in algorithm utilization and data manipulation.
Culmination
Understanding array multiplication is crucial for anyone working in programming and computer science. This article discussed various aspects of arrays, emphasizing their significance in computational applications. The key focus points included implementation, challenges, optimization, and practical applications. These elements provide a framework for deeper comprehension of how array multiplication functions within different programming contexts.
Effective utilization of array multiplication can lead to enhanced efficiency in data processing tasks, particularly in fields such as data science and machine learning. The emphasis on optimization techniques reveals how programmers can refine their approaches to handle vast datasets more efficiently. By acknowledging potential challenges, such as common errors and performance issues, one can take proactive steps to mitigate these problems before they impact the code's function.
Utilizing existing libraries like Numpy in Python or Apache Commons Math in Java brings additional benefits like speed and reliability to operations involving arrays. These resources embody best practices that can significantly improve a programmer's workflow.
The integral focus on both fundamentals and advanced topics ensures that readers, regardless of their skill level, can grasp essential ideas and strategies, preparing them for future challenges in their programming careers.
Recap of Key Points
- Definition and Types of Arrays: Arrays are collections of elements organized to facilitate efficient data management.
- Mathematical Concepts: Understanding of matrix operations enhances capabilities in manipulating arrays.
- Programming Implementations: Practical examples showed implementation in popular programming languages such as Python, Java, and C++.
- Common Challenges: Awareness of errors regarding dimensions and data types underlines the care required in these operations.
- Optimization Strategies: Use of libraries and improved algorithms highlight best practices for better performance.
- Practical Applications: Fields such as machine learning and data processing rely heavily on effective array multiplication.
Future Directions
The future of array multiplication is intertwined with advancements in programming languages and resources. As technology evolves, libraries will likely expand to introduce more streamlined methods for handling complex multidimensional data. Notably, the integration of artificial intelligence in programming may offer more intuitive ways for developers to manage these arrays. Furthermore, exploration into more advanced computational techniques, such as tensor processing, could lead to entirely new paradigms for understanding and executing mathematical operations.
The incorporation of machine learning frameworks can also increase the complexity of array operations, treating them as fundamental units of AI modeling. Continuing development in this area is very promising, pointing towards more sophisticated applications of theoretical concepts learned today.