Mastering Conditional Types: A Deep Dive into Programming
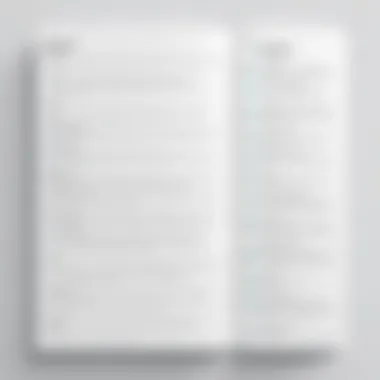
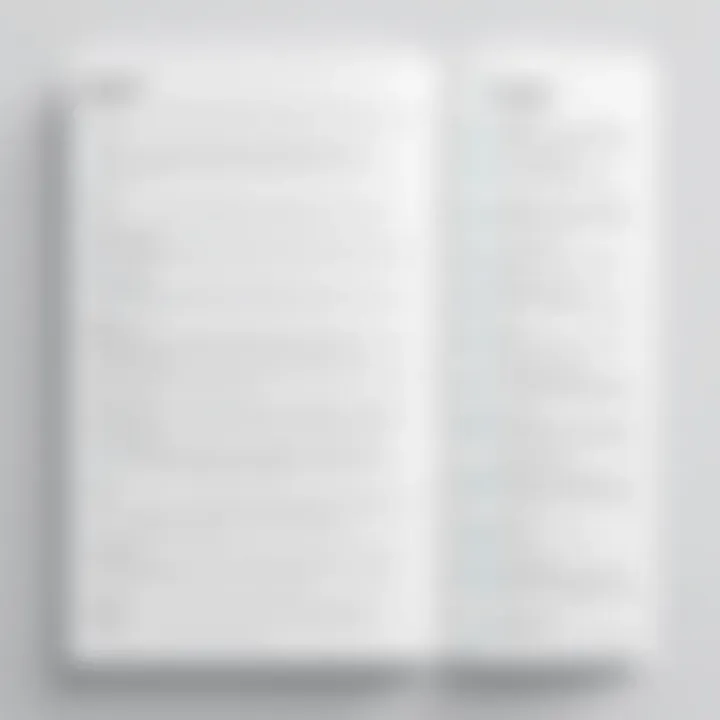
Intro
This article serves as an articulate exploration of conditional types, a significant advancement in programming languages. Understanding the implications of conditional types cannot be understated. As programmers, grasping these concepts enhances our ability to write dynamic, flexible, and efficient code. We will analyze how conditional types function, their syntax, and their practical applications in real-world scenarios. This knowledge is crucial for programmers who wish to stay relevant in continually evolving technology landscapes.
Coding Challenges
There are many challenges faced by developers when implementing programming concepts. Conditional types present both advantages and obstacles. To help programmers enhance their skills, we will examine coding challenges that focus on these types. This will include simulations that mimic real-world situations where conditional types can be effectively utilized.
Weekly Coding Challenges
Engaging in weekly coding challenges pushes developers to think outside the box and apply their knowledge of conditional types. Each challenge could require writing functions that leverage these types, thereby reinforcing the learnings from this article. To track progress, one might use online platforms such as GitHub or Stack Overflow and participate in community forums.
Problem Solutions and Explanations
After facing challenges, understanding the solutions is vital. Detailed explanations support the learning process, illuminating how conditional types worked within specific challenges. For instance, a problem could involve data filtering using conditional types, showcasing how to dynamically determine an output type based on specific inputs.
Tips and Strategies for Coding Challenges
Adopting smart strategies enhances performance in tackling coding challenges. Some useful tips include:
- Break down problems: Simplify complex issues into manageable parts.
- Practice regularly: Frequent engagement with different problems strengthens comprehension and skill.
- Utilize resources: Leverage forums, documentation, and tutorials to seek clarity on difficulties encountered.
Community Participation Highlights
Interacting with community members fosters a deeper understanding of conditional types. Discussing solutions with peers or engaging with experts in forums can unveil new insights. Platforms like Reddit and GitHub provide spaces for collective brain-storming and support as programmers navigate coding challenges involving conditional types.
By experiencing coding challenges firsthand, developers improve their proficiency in using conditional types and expand their problem-solving skills.
The End
Understanding and applying conditional types significantly enriches one's programming skills. As the field evolves, being adaptable becomes essential. These concepts not only contribute to effective coding but prepare developers for more complex programming tasks ahead.
Prelims to Conditional Types
Conditional types represent an advanced feature in programming languages that empower developers to create adaptively constructed types based on conditions. A deeper grasp of this concept forms the bedrock of modern type systems, particularly in languages such as TypeScript. Here, one can appreciate how conditional types enable more flexible and safer coding practices.
Definition
Conditional types utilize a form of conditional logic to alter type inheritance depending on certain conditions. In practice, a conditional type is often arranged using the syntax , where is checked against . If this condition is satisfied, the outcome is ; if not, it returns . This logic serves as a fundamental building block for crafting types that are context-sensitive, dynamically adjusting to different use cases within code.
Importance in Modern Programming
In today's programming landscape, developing robust and maintainable code is paramount. Conditional types facilitate this need through several key benifts:
- Type Safety: By leveraging conditional types, coders can enforce stricter type checks which prevent many common programming errors ' in advance. This proves particularly valuable in large code bases or complex systems where inconsistencies can lead to significant logical flaws.
- Enhanced Flexibility: Conditional types create adaptable code that can shift to different contexts seamlessly. Developers can write functions capable of accepting diverse input without sacrificing type integrity, addressing the growing demand for dynamic programming paradigms.
- Improved Readability: Although the syntax may initially appear complex, conditional types can actually aid in making code more explicit regarding intended use cases. This clarifies logic flow and intent, lending itself to cleaner coding practices.
Given the increasing sophistication of software and the languages that support them, understanding conditional types becomes increasingly critical for striking a balance between functionality and maintainability. As programmers dive deeper into higher-order types and generic programming, the shortening of potential error spans and clear computational logic rendered by conditional types begins to shine. Therefore, mastery over this feature can lead to markedly improved software systems.
"Conditional types represent a union of logic and type safety, creating opportunities for innovative coding practices."
Syntactical Structure of Conditional Types
Conditional types exhibit unique syntactical elements that are crucial to their functionality within programming languages. Understanding this structure forms the foundation for using conditional types effectively. A firm grasp of these elements allows developers to leverage their full potential, increasing code flexibility and robustness in modern software engineering.
Basic Syntax
At its core, the basic syntax of conditional types consists of three parts: the condition, the true branch, and the false branch. It is often framed as . Here, represents the type you evaluate against . If fulfills the criterion, the type resolves to ; otherwise, it reverts to .
This simple yet powerful structure plays an essential role in type inference and can create complex type relationships. For example, consider a conditional type that switches behavior based on whether an input is a string or not:
In this code snippet, generates a new type based on whether is truly a string. Such concise expressions allow developers to create code that adapts dynamically, adhering to the broader and evolving needs during application development.
Advanced Syntax Features
Beyond the basic syntax, more advanced features provide greater capabilities in shaping types. For instance, you can nest conditional types within each other, allowing for multi-tiered evaluations. This offers a pathway for considerable customization based on more intricate conditions.
Example: Nested Conditional Type
Within nested structures, you can refine behavior even further:
In this example, the evaluation layers allow checking explicitly against various expected types. Moreover, the concept of 'distributive conditional types' emerges when a conditional type is applied over union types. When scanned, these types act conditionally on each member of the union. For instance:
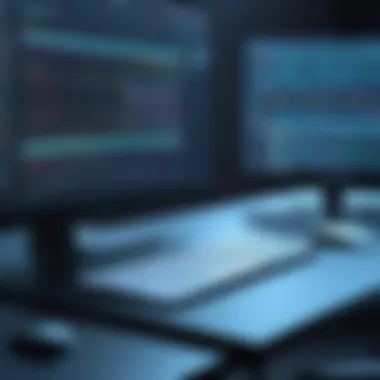
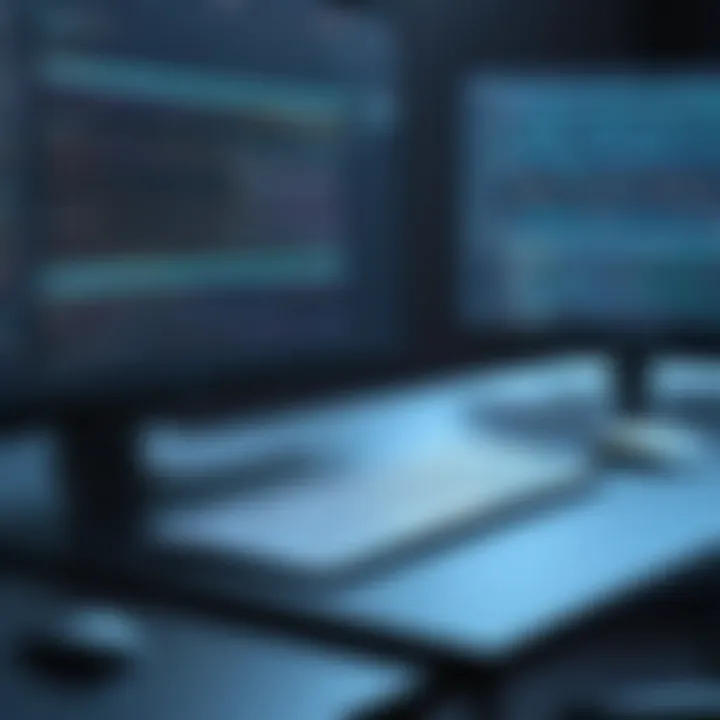
- If represents , it is evaluated as either correspondence to the resulting types.
Structured correctly, advanced syntax features make code expressive and maintainable while demonstrating what may seem like complex transitions between types. As such, developers are empowered to create efficient type maps that reflect true specifications of their applications. Understanding these constructs contributes greatly to effective programming practices, substantially prolonging code utility and enhancement opportunities.
Conditional types provide a robust mechanism for expressing logic in type-level programming, effectively expanding functionality without compromising simplicity.
Differences Between Conditional Types and Other Type Systems
Conditional types represent a unique construct within programming languages, significantly diverging from traditional type systems. To grasp the relevance of this topic, one must recognize how conditional types allow for greater flexibility in code while standard systems enforce more rigid structures. Understanding the nuances between conditional types, union types, and intersection types can enhance the programming capability and thorough comprehension of type manipulation.
Comparing to Union Types
Union types encapsulate multiple types within a single definition, providing a level of flexibility to handle different data types. This feature is advantageous when a function accepts various input types. For example, in TypeScript, a variable might be declared as , allowing it to store either string or number data. However, union types lack the dynamism found in conditional types.
Conditional types, such as , build upon the concept of unions but take it a step further by enabling type evaluation based on conditions. This dynamic attribute allows developers to write cleaner, more context-sensitive code that can adjust based on the input types during compile time.
For instance, consider the implementation of a function that adjusts its return type based on the presence of method properties.
This function smartly infers the correct return type on usage rather than solely relying on listed union types. This differentiation enhances type safety and significantly reduces possible runtime errors.
Main takeaways from comparing union types and conditional types:
- Flexibility: Union types allow for multiple types but lack conditional evaluation. Conditional types evaluate and adapt based on input.
- Type Safety: Conditional types provide enhanced assertions that align strictly with input types, minimizing mistakes.
Contrasting with Intersection Types
Intersection types provide the means to create a type that combines multiple other types into one single object. This plays a crucial role in scenarios requiring traits from multiple sources. For instance, a person might be defined by , allowing one to hold properties of both structures.
While intersection types assert a collective enforceable relationship, conditional types challenge that paradigm. They avoid the need for establishing strict type adherence as seen in intersections—instead, they choose behavior based on the type being passed. Just like with union types, this evaluation works at conditional levels.
For clarity, here's a hypothetical scenario:
- Consider a function base that returns specialized behavior based on object attributes. A conditional type approach can let a developer create customized return types effortlessly without needing explicit intersection guidelines.
Some considerations when contrasting intersecting and conditional types include:
- Dynamic Behavior: Conditional types can result behavioral changes based on the context whereas intersection types create a static, enforceable requirement.
- Readability: Using conditional types can sometimes result in more readable code. Decisions happen based on the type, minimizing complex structures.
As you understand the differences between conditional types, union types, and intersection types, consider how these constructs influence your coding. They present opportunities to optimize your systems in handling types based on conditions rather than adhering strictly to rigid paradigms. This approach not only aids code organization but ultimately influences software effectiveness.
Conditional types demand understanding yet offer substantial power to define relationships in a nuanced manner, possibly reshaping your coding efficiency.
Practical Implementations of Conditional Types
Conditional types present practical benefits in programming. Their flexibility and adaptability streamline development processes. In various programming languages, these types enhance efficiency while reducing boilerplate code. Understanding their implementations helps programmers leverage conditional types effectively in real-world applications.
Use Cases in Development
Conditional types find effective application across multiple development environments. They serve multiple purposes, such as:
- Function Overloading: Developers can create functions that act according to the input type. This capability allows for better reuse of functions without cluttering the codebase.
- Type Mappings: Conditional types make it easier to map one type to another. This is increasingly relevant in frameworks such as React.js where types need alignment with various state management schemes.
- Mocking and Testing: Both mocking libraries and testing frameworks can take advantage of conditional types to infer and manage type dependencies seamlessly. Developers often utilize conditional types to describe relationships between types clearly, making tests both more robust and easier to write.
Example of how conditional types are utilized:
This demonstrates twisted return types based on input condition.
Role in Generic Programming
In generic programming, conditional types play a crucial role. They allow programmers to define types that behave dynamically based on user inputs. This flexibility fosters cleaner and more concise code. A few considerations include:
- Type Inference: When defining generic functions or classes, conditional types enable automatic inferencing based on so that controls on derived types are possible, making programming smoother.
- Scalability: Systems that rely on generic programming benefit significantly when combined with conditional types. This enhances scalability, making it simpler for codebases to adapt to changes or to support new features.
- Utility Types Library: Many programming languages introduce utility types using conditional constructs, providing a standard method for type manipulation. Libraries in TypeScript, like and , enhance type flexibility.
As generics continue to shape software development paradigms, understanding conditional types becomes paramount for written dynamic quality code. Effective use can ensure developers maximize results, while handling complex data interactions seamlessly.
Advantages of Using Conditional Types
Conditional types provide several benefits that significantly contribute to the efficiency and clarity of code. Their adoption can lead to enhanced coding practices and mitigate the complexities often encountered in software development. Below are some of the critical advantages associated with conditional types:
Enhanced Type Safety
One of the most important advantages of conditional types is the enhancement of type safety. In programming, ensuring that variables, functions, and data structures behave as expected is crucial. Conditional types allow the definition of types that depend on the values of other types.
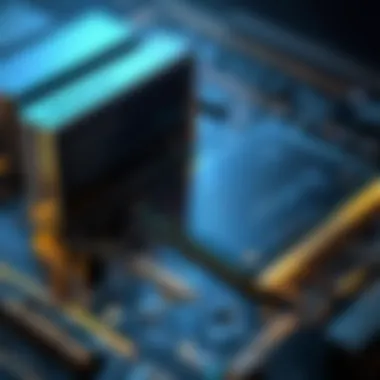
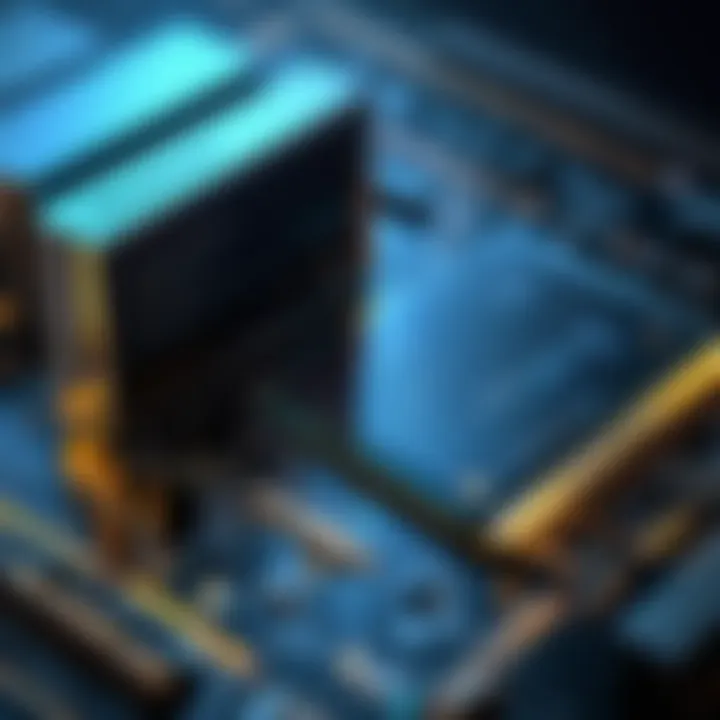
For example:
The type evaluates whether a given type is a string and returns the appropriate response. This mechanism may notably prevent errors that could occur at runtime by catching them early during compilation, therefore improving overall reliability.
The use of conditional types reduces the likelihood of type mismatches. Mismanagement of data types can result in subtle bugs that are hard to track. By introducing conditions, programmers can create stricter constraints that guide the development process more conscientiously. More security in type boundaries generally leads to more stable applications.
Improved Code Readability
Code readability is key to maintainability and collaboration in programming projects. Conditional types enhance how code is understood, ensuring that other developers can follow the intention behind different declarations easily.
When conditional types are used effectively, code that would have been complex and convoluted can be made clearer. Here's a brief example:
On the surface, it quickly informs the reader that extracts the from an object type . This explicit declaration can help others comprehend the developer’s goals without extensive comments or documentation, reducing cognitive overhead.
In contrast to traditional type systems where types can feel abstract, conditional types ground these abstractions, presenting them in a more dderectf and understandable manner. As a result, teams spend less time deciphering intentions behind functions, thereby fostering efficient collaboration and speeding up the development cycle.
Facilitating Code Reusability
Code reusability remains an ever-important principle in software development. Conditional types contribute significantly to building components that can adapt to different use cases without rewriting logic. This adaptability inherently promotes the creation of more flexible frameworks and libraries.
Imagine a component that behaves differently based on the type of data that it processes. For instance, you can define a generic function utilizing conditional types to manipulate input dynamically:
This example shows how programming constructs can remain flexible while being broad enough to facilitate various implementations. The richness of this capability not only conserves development time but also strives for consistency in how developers build components.
In summary, conditional types do more than just offer unique coding mechanisms. They present underlying support for both basic and complicated applications in programming. Improved safety, clarity in code, and the capacity for reusability are key counts in a developer's toolkit, setting the framework for achieving high-quality products.
Challenges and Limitations of Conditional Types
Conditional types, while offering remarkable flexibility and power in type systems, are not without their challenges. Understanding these limitations is crucial for programmers who seek to utilize conditional types effectively. Addressing the potential complexity and performance implications can provide a balanced view of how to implement conditional types in a practical context.
Complexity in Understanding
One of the primary hurdles with conditional types is their complexity. For novice developers, the concept of conditional types may be bewildering. The syntax can be intricate, demanding a solid foundation in TypeScript or similar programming languages. Because conditional types involve evaluation of true or false statements, predicting their behaviors in different contexts can be formidable.
Consider this simple example:
In this code, determining whether a given type is a string introduces an overlay of logic. When using this in larger projects, such complexity can lead to misunderstandings or misconfigurations if developers do not fully grasp the implications.
- Adjustments to the mental model: Developers must accommodate this additional cognitive load, complicating type inference and debugging efforts.
- Documentation gaps: In some cases, the documentation around these types is sparse. Failing to locate adequate resources makes wrapping one's head around conditional types even more difficult.
This complexity is compounded when advanced conditional types are considered. Combinatory promises and advanced conditional flows can escalate difficulty levels further, creating a learning curve that might dissuade some programmers from mastering these tools.
It is essential to engage with practical examples and in-depth resources to overcome the challenges complexity presents with conditional types.
Performance Considerations
Another aspect that carries significance is the potential performance impact. While conditional types introduce powerful features into the programming toolkit, they can inadvertently lead to degraded performance. This situation arises particularly during type checking and compilation.
During the type-checking phase, complex conditional types may cause longer processing time. Rigid conditional types interact with generics which further complicates how TypeScript achieves resolution:
- Increased compilation time: An overload of heavy conditional evaluations can lead to increased times as the compiler must assess every potential combination.
- Runtime penalties: Depending on implementation, too many conditions can negatively impact runtime bicycles, ultimately leading to less efficient code.
When considering widespread use of conditional types, programmers ought to benchmark performance outcomes. Running tests specifically focused on conditional types will clarify their impact relative to simpler implementations.
Thus, while conditional types provide superior flexibility, programmers must be wary of the implications from a performance standpoint.
Overall, it is stimulating to recall that every tool has its limitations. Fully understanding conditional types involves not just recognizing their benefits but actively considering the equal significance of challenges that accompany them. As we continue our exploration of conditional types in modern programming, these considerations form an essential part of crafting thoughtful, optimized code.
Examples of Conditional Types
Examining examples of conditional types is crucial to understanding their functionality and versatility in programming languages. Conditional types enable dynamic the evaluation of types at compile time, resulting in better type safety and improved code structure. Through these examples, developers can see practical implementations that illustrate the benefits of using conditional types in real-world scenarios.
Simple Conditional Type Example
A straightforward way to understand conditional types is by considering a basic use case. In TypeScript, for instance, we can create a simple conditional type that demonstrates how types can change based on other types. The general syntax for conditional types is:
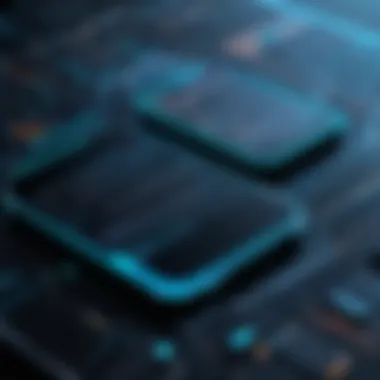
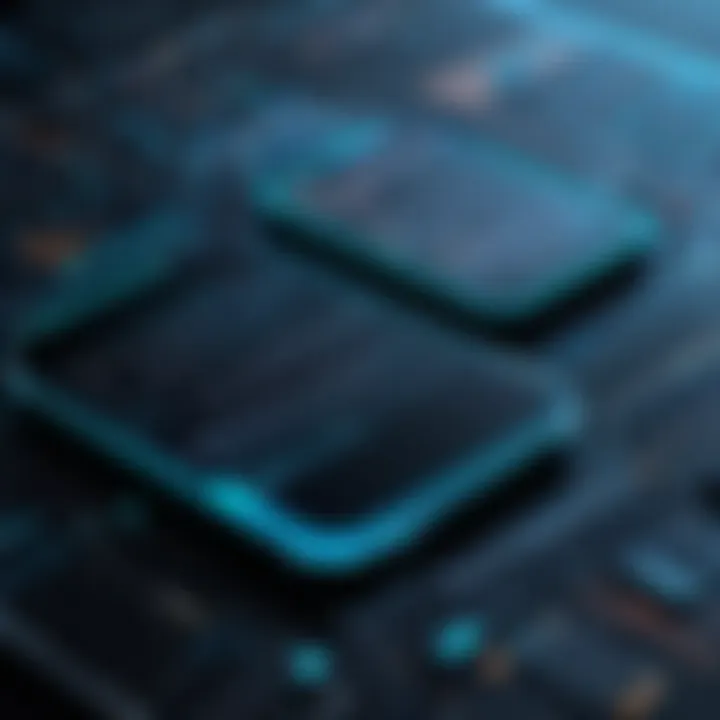
Here, is the type being examined. If extends , it results in type ; otherwise, it results in type .
Example: Suppose we want to create a type that identifies whether a type is a string or not. This could look like:
Here, would evaluate to 'No, it is not a string', while would evaluate to 'Yes, it is a string'. This simple exercise encapsulates a real use of conditional types, as it allows checking for type characteristics while improving type safety.
Complex Scenario Implementation
Moving beyond simple checks, conditional types can take different forms in more complex scenarios, such as in building advanced data structures or a delegated logic in a program.
Consider an instance where we build an API response handler that varies based on the request type. Below is an advanced implementation demonstrating conditional types and integrated generics:
In this example, conditionally returns different types based on the request method. If is 'GET', we receive an object with and . If 'POST', a structure confirming success. This allows for clearer expectations when implementing functions to handle various response types.
Such illustrations point to the power of conditional types. Leveraging these practices can lead to creating compact yet readable code, where developers can make better use of static typing to identify errors catch during compile time.
The Role of Conditional Types in TypeScript
Conditional types play a pivotal role in TypeScript by introducing powerful abstractions into type systems. This feature allows developers to express complex relationships between types based on certain conditions, transforming how type safety and flexibility are managed in JavaScript. As developers increasingly seek robust ways to handle dynamic types in large applications, conditional types offer significant advantages.
Integration with TypeScript
TypeScript, being a superset of JavaScript, carries the flexibility of JavaScript but adds a layer of static typing. Conditional types harness this flexibility by enabling type definitions that can change based on some condition. The syntax for a conditional type follows the pattern , where T is the type being checked, U is the type constraint, and X and Y are resultant types depending on whether T fits U or not.
Utilizing conditional types in TypeScript aids in creating more robust and adaptable types, minimizing potential errors and misunderstandings several complexity levels up. This syntax is important for implementing advanced functionalities, allowing more concise and readable declarations, where required types adjust based on the presence or absence of properties or interfaces. Developers are encouraged to embrace this feature as it evolves with TypeScript 4.x and beyond.
Real-life Applications
The practical usage of conditional types in TypeScript extends into various spheres of development. Below are some significant aspects:
- Flexible API design: Conditional types enhance the safety of APIs by making return types dependent on input, allowing better type inference and usage correct expectations.
- Default values for generics: They simplify the management of default types when generic types do not match specified constraints, bypassing complexity in implementation levels.
- Type-safe state management: In state management frameworks, conditional types help establish refined state types that rely on runtime data constraints. This results in error reduction and better overall state management.
Including examples forms a central role in deepening understanding. Utilizing tools, such as TypeScript Playground, provides immediate inline illustrations.
In summary, the role of conditional types in TypeScript significantly empowers developers to manage and manipulate types intelligently. As coding continues to projet improvements in quality and readability, optional conditions enable an extensive array of applications and cultivate an environment for safe coding practices.
Future Trends in Conditional Types
As programming languages continue to evolve, the relevance of conditional types evolves alongside them. This feature allows developers to set conditions that determine the resulting type based on certain criteria. This principle is being widely embraced in modern programming languages, enhancing flexibility in code written by engineers and developers. Not only does it empower type design but it also emphasizes type safety in software architecture.
Emerging Language Features
In recent years, many programming languages have started embracing conditional types with greater integration and advanced capabilities. Languages like TypeScript, Kotlin, and Swift are extending their type systems to utilize the principles tied to conditional types. By supporting complex conditional statements within type definitions, these languages allow developers to build more robust applications.
For instance, TypeScript has introduced symbolic conditional types, helping developers create dynamic type behaviors by utilizing features like inferencing and constraint expressions. Therefore, where interfacing and data manipulation are concerned, the need for clear conditions in types is transforming.
Some key features being seen in emerging languages are:
- Type inference enhancements: Language implementations are focusing on the intelligent recognition of used types, leading to automated type tracking.
- Rich macros and type expressions: More expressive facilities are introduced for creating complex conditional types without verbosity.
- Error handling improvements: Better feedback mechanisms on type mismatches are now becoming common practice.
"The emergent emphasis on conditional types signals not only an evolution in programming languages, but also a shift towards leaner and type-correct applications."
Influence on Software Development Practices
As conditional types gain prominence, they are fundamentally reshaping software development methodologies. Their incorporation ensures increased flexibility, thereby fostering cleaner and more maintainable code. By marrying logic with types, conditional types encourage a design-first approach towards coding that shapes best practices.
The adoption of conditional types impacts practices such as:
- The rise of type-oriented programming: Developers are encouraged to think critically about how types might interact dynamically based on conditions at compile time, improving decision making as projects escalate in size.
- Increased emphasis on test-driven development: Robust type systems help drive tests themselves since conditions become a basis for unit tests, assuring outcomes against expected behaviors.
- Robustness in API design: By leveraging conditional types, API developers achieve intelligent load handling, ensuring a clear expectation on the data structures being handled internally and externally.
Overall, emerging trends surrounding conditional types signify a broader commitment toward higher quality and reliable software solutions. Agile methodologies increasingly leverage these features, resonating in both education and the profession.
Culmination
The culmination of our exploration into conditional types bears significant pedagogical value for both aspiring and experienced programmers alike. Understanding the subtleties necessary parofconditional types provides programmers with a indispensable tool for building more dynamic, scalable, and maintainable code. Recognizing conditional types as integral rather than simply supplementary encourages a more robust approach to programming that better aligns with contemporary development practices.
Summary of Key Insights
Throughout this article, we navigated various aspects of conditional types, emphasizing their syntax, application, and their distinctive role among type systems in languages such as TypeScript. Here are the primary insights:
- Dynamic Typing Benefits: Conditional types leverage actual values to determine type assignments at compile time, enhancing flexibility without sacrificing safety.
- Real-word Applications: Familiarity with conditional types exposes programmers to advanced patterns, maximizing efficiency in functions and class components.
- Readability & Reusability: The adoption of conditional types not only aids in maintaining code cleaniness but also paves the way for high degrees of code reuse, fundamentally vital for modern software engineering.
- Performance Trade-offs: It is crucial to comprehend some practical limitations and the potential performance overhead that emerges from their peer complexity.
Final Thoughts on Conditional Types
Conditional types herald a notable shift in how type systems are viewed, where concepts of adaptability and flexibility become paramount in modern programming paradigms.
By integrating these transitional insights on conditional types into their methodologies, programmers can heighten their contributions to projects by wielding greater autonomy over types amidst complexity.