Exploring Java Classes: A Comprehensive Guide for Programmers
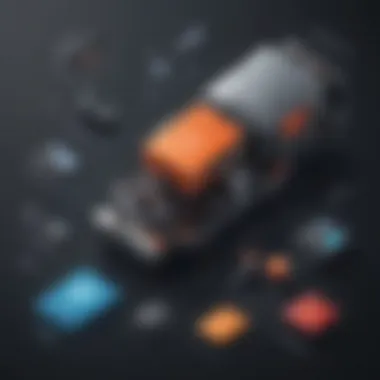
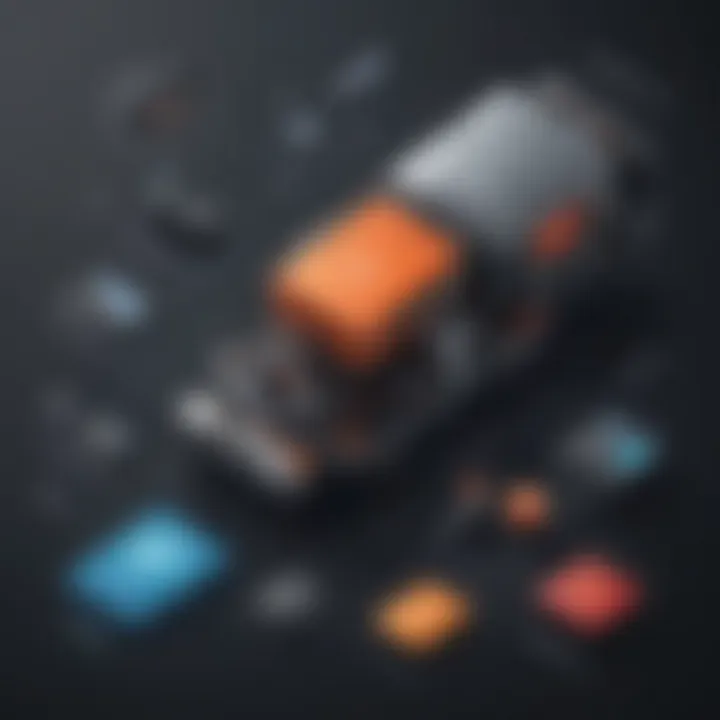
Coding Challenges
When delving into the intricate world of Java programming, understanding classes is essential for building a strong foundation in object-oriented programming. Classes serve as the blueprints for creating objects, encapsulating their properties, and defining their behavior. Whether you're a novice exploring the basic concepts or an experienced programmer honing your skills, comprehending classes in Java is a stepping stone towards mastering the language. Throughout this comprehensive guide, we will unravel the core aspects of classes, including inheritance, polymorphism, and encapsulation, offering insights and practical examples to enhance your understanding.
Technology Trends
Java, being a cornerstone in the realm of software development, continually evolves to align with technological advancements and industry demands. Understanding the trends and innovations surrounding Java classes can greatly influence your coding practices and project developments. Keeping abreast of the latest features, design patterns, and best practices in Java programming will not only enhance your efficiency but also elevate the quality and scalability of your applications. Gain a deeper insight into how Java classes integrate with emerging technologies, such as cloud computing, microservices architecture, and Internet of Things (IoT), shaping the future landscape of software development.
Coding Resources
While grasping the intricacies of Java classes is pivotal, having access to efficient coding resources can significantly expedite your learning and problem-solving abilities. From comprehensive language guides to advanced IDEs and debugging tools, leveraging the right programming resources can streamline your development workflow and enhance productivity. Explore a myriad of tutorials, code repositories, and online platforms tailored to Java programming, equipping yourself with essential knowledge and practical insights to navigate the diverse challenges and opportunities in software development.
Computer Science Concepts
Beyond just learning Java classes, delving into broader computer science concepts can broaden your horizons and deepen your understanding of programming principles. Dive into algorithms and data structures primers to sharpen your problem-solving skills, or explore the foundations of artificial intelligence and machine learning to unlock new avenues of innovation. Understand the significance of networking and security fundamentals in today's digital landscape, as well as the potential of quantum computing and other futuristic technologies reshaping the field of computer science. By immersing yourself in diverse concepts and technologies, you can expand your expertise and stay ahead in the dynamically evolving tech industry.
Introduction to Classes
In the realm of Java programming, the concept of classes stands as a pivotal element, laying the foundation for object-oriented programming paradigms. Understanding classes in Java is akin to acquiring the keys to constructing intricate software systems with utmost efficiency and organization. By delving into the intricate details of classes, programmers can streamline their code, enhance reusability, and maintain a structured approach towards software development. As we embark on this journey to comprehend classes in Java, we will unravel the essence of class definition, creation, and utilization, opening doors to limitless programming possibilities.
Definition of Classes
What is a class in Java?
A class in Java serves as a blueprint for creating objects, encapsulating attributes, and defining behaviors. It acts as a template that specifies the data and methods that constitute objects of that class. The essence of a class lies in its ability to model real-world entities or abstract concepts, providing a means to represent and manipulate data seamlessly. With a class, programmers can instantiate multiple objects sharing the same attributes and behaviors, fostering code reusability and structure. The elegance of a class in Java lies in its capacity to promote modularity, inheritance, and data encapsulation, essential features for developing scalable and maintainable software systems.
Role of classes in object-oriented programming
Classes play a fundamental role in the realm of object-oriented programming, serving as the building blocks for organizing and structuring code. They facilitate the principles of encapsulation, inheritance, and polymorphism, key tenets of object-oriented design. By encapsulating data within classes, developers can safeguard information and control its access, enhancing data security and integrity. Moreover, classes enable the concept of inheritance, allowing subclasses to inherit properties and methods from parent classes, fostering code reuse and extension. In essence, classes in object-oriented programming form the bedrock for creating robust, scalable, and modular software systems, empowering developers to craft elegant solutions to complex problems.
Creating a Class
Syntax of defining a class
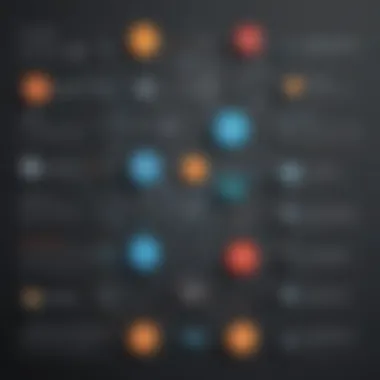
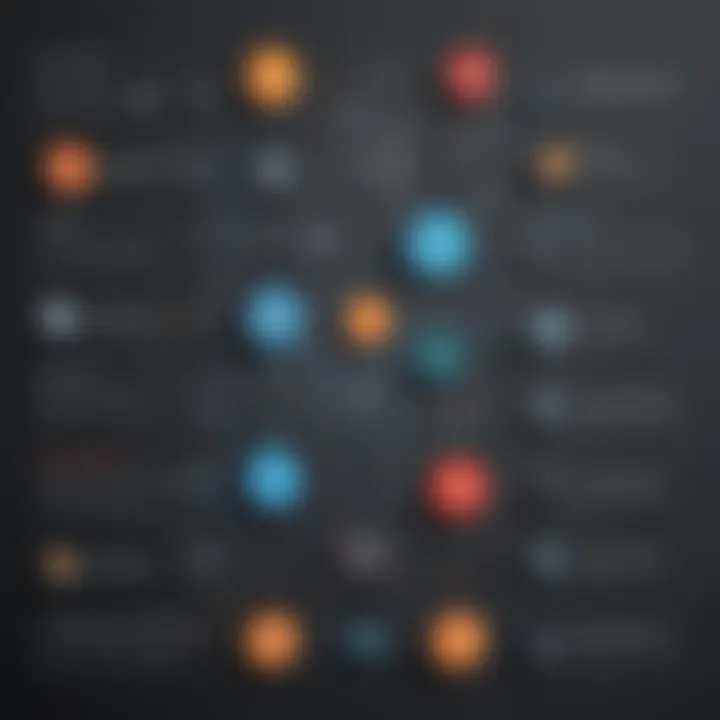
The syntax of defining a class in Java adheres to a strict structure, comprising modifiers, class name, superclass (if applicable), and class body enclosed within curly braces. By defining a class, programmers delineate the blueprint for creating objects of that type, specifying attributes, constructors, and methods that characterize the class. This syntactical precision ensures clarity and consistency in code organization, enabling developers to craft robust and maintainable software solutions. Embracing the syntax of defining a class is paramount for fostering code reusability, maintainability, and extensibility, laying the groundwork for scalable and structured software development.
Class structure and components
The structure of a class encompasses various components, including fields, constructors, methods, and nested classes, each playing a crucial role in defining the behavior and characteristics of the class. Fields represent the attributes or properties of the class, storing data that defines the state of objects. Constructors serve as special methods responsible for initializing objects upon creation, while methods encapsulate the behaviors and actions that objects can exhibit. Nested classes within a class provide a means to group related functionalities, enhancing code organization and readability. Understanding the structure and components of a class is essential for mastering Java programming, facilitating the creation of versatile and efficient software modules.
Class Members and Methods
In the vast landscape of Java programming, understanding class members and methods is imperative. As fundamental components of object-oriented programming, class members and methods play a crucial role in defining the behavior and attributes of objects. By delving into the specifics of class members and methods, programmers can effectively encapsulate data and functionality within their Java applications. This section will explore the intricacies of instance variables and methods, shedding light on their significance in crafting robust and efficient Java programs.
Instance Variables
Understanding instance variables
The concept of instance variables holds tremendous importance within the realm of Java programming. Instance variables are unique to each object instance, providing a mechanism for storing and retaining specific data related to an object. By encapsulating state within objects through instance variables, programmers can ensure data integrity and facilitate modular design. This section will delve into the nuances of understanding instance variables, highlighting their pivotal role in maintaining the state of objects throughout their lifecycle.
Declaring and initializing variables
In Java programming, declaring and initializing variables is a fundamental aspect of working with instance variables. By declaring variables, programmers define the data type and name of the variable, laying the groundwork for storing values within the object. Initialization involves assigning an initial value to the variable, initializing its state for future usage. By examining the process of declaring and initializing variables, programmers can establish a solid foundation for constructing object-oriented Java programs, ensuring clarity and consistency in data manipulation.
Methods in a Class
Defining methods in Java classes
Methods serve as the primary means of encapsulating behavior within Java classes, enabling objects to exhibit functionality and respond to stimuli. Defining methods in Java classes involves specifying the method signature, return type, and method body to outline the behavior of the function. By encapsulating logic within methods, programmers can achieve code reusability and maintainability, adhering to the principles of object-oriented design. This section will shed light on the essence of defining methods in Java classes, emphasizing their role in structuring and enhancing the functionality of Java applications.
Method overloading and overriding
Method overloading and overriding are advanced concepts in Java that contribute to polymorphism and code flexibility. Method overloading allows multiple methods with the same name but different parameters, enabling versatility in method invocation. On the other hand, method overriding involves redefining a method in a subclass to provide specific implementation, altering the behavior inherited from the superclass. By exploring method overloading and overriding, programmers can leverage the power of polymorphism to create efficient and adaptable Java programs, enriching their coding repertoire.
Inheritance and Polymorphism
In the realm of Java programming, understanding the concepts of inheritance and polymorphism is paramount. Inheritance, which involves creating new classes based on existing ones, plays a pivotal role in code reusability and structuring programs efficiently. It allows developers to derive characteristics and behaviors from existing classes, promoting a hierarchical organization of code. On the other hand, polymorphism enables objects to be treated as instances of their parent class, enhancing flexibility and extensibility in software design. By allowing multiple methods to have the same name but different implementations, polymorphism facilitates elegant solutions to complex programming challenges.
Concept of Inheritance in Java
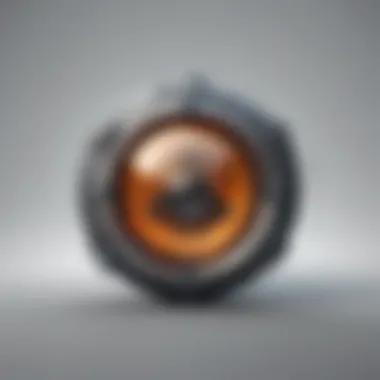
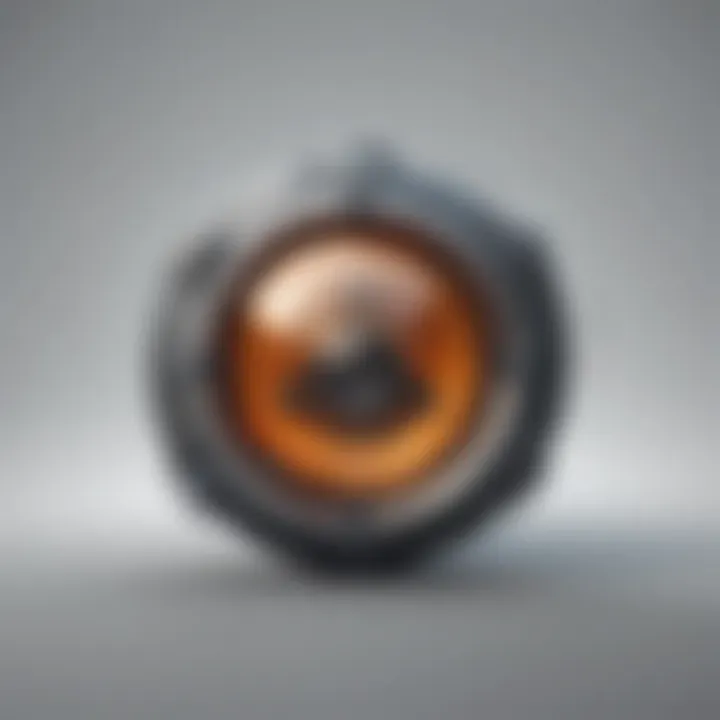
The concept of inheritance in Java embodies the idea of deriving new classes from pre-existing ones, fostering a parent-child relationship among classes. It allows child classes to inherit attributes and methods from their parent class, promoting code reusability and minimizing redundancy. This hierarchical structure enables developers to build upon existing code without reinventing the wheel, streamlining the development process and ensuring a more organized codebase. However, reliance on inheritance should be balanced with considerations of tight coupling and the potential for hierarchies to become overly complex, necessitating a judicious approach to class design.
Extending Classes and Superclass
Extending classes and working with superclasses in Java empowers developers to leverage the functionality of existing classes while introducing new features and behaviors unique to the subclass. By extending a class, developers can inherit attributes and methods from the superclass, enriching the subclass with additional functionalities tailored to specific requirements. This practice promotes modularity and facilitates the maintenance and scalability of codebases by encapsulating related functionalities within specific classes. However, excessive class extensions can lead to deep class hierarchies, potentially introducing complexity and maintenance challenges that may hinder code readability and robustness.
Polymorphism
In the context of Java programming, polymorphism serves as a crucial mechanism for enhancing the flexibility and adaptability of object-oriented code. Understanding polymorphism enables developers to write more concise and modular code by allowing different classes to be treated uniformly through a common interface. By leveraging polymorphism, developers can write code that is more flexible and easier to maintain, adapting efficiently to changing requirements and ensuring code extensibility. However, the misuse of polymorphism or excessive method overrides may lead to code obfuscation and reduced code clarity, highlighting the importance of careful design and implementation decisions.
Method Overriding and Dynamic Dispatch
Method overriding and dynamic dispatch represent key facets of polymorphism in Java, facilitating the execution of the most specific implementation of a method at runtime. Method overriding allows subclasses to provide a specific implementation of a method declared in the superclass, enabling customized behavior while adhering to a common interface. Dynamic dispatch, also known as runtime polymorphism, ensures that the correct method implementation is invoked based on the actual class of the object during program execution. This dynamic behavior enhances code flexibility and adaptability, enabling developers to write code that is robust and easily extensible. However, the intricacies of method overriding and dynamic dispatch require careful consideration to avoid unintended side effects and ensure the correctness and maintainability of object-oriented systems.
Encapsulation and Abstraction
Encapsulation and Abstraction in Java play a pivotal role in enhancing the modularity, security, and reusability of code. These concepts are fundamental to object-oriented programming as they allow developers to hide sensitive data within a class and only expose necessary information to the outside world. By encapsulating data, developers can prevent unauthorized access and modification, thus improving the robustness of the codebase. On the other hand, abstraction enables the creation of abstract classes and interfaces, easing the implementation of complex systems by providing a blueprint for concrete classes to follow.
Encapsulation
Encapsulating data in Java classes: Encapsulation involves bundling data (variables) and methods that operate on that data within a single unit, i.e., a class. By encapsulating data in Java classes, developers can ensure data integrity and prevent accidental modification from external sources. This not only enhances security but also promotes code maintenance and scalability. Encapsulation is a cornerstone of object-orientation, promoting the principle of encapsulation in modules. It allows for clear separation between implementation and interface, resulting in more manageable and organized code.
Access modifiers and data hiding: Access modifiers control the visibility and accessibility of class members within Java. By using access modifiers such as public, private, protected, and default, developers can restrict access to certain variables or methods, enhancing data hiding. Data hiding, a key aspect of encapsulation, ensures that critical data is not exposed to unintended manipulation. This helps in maintaining code integrity and reduces the risk of unexpected behavior. While access modifiers offer enhanced security and encapsulation benefits, they also impose constraints on class design and potential reuse, requiring careful consideration in Java development.
Abstraction
Abstract classes and interfaces: Abstract classes and interfaces provide a way to define abstract types in Java, allowing developers to create templates for concrete classes to implement. Abstract classes can contain both concrete and abstract methods, while interfaces only include abstract method signatures. This feature facilitates code reusability and design flexibility, as classes implementing interfaces can conform to specific behavior without the need for hierarchical inheritance. Abstract classes and interfaces serve as blueprints for defining contracts and structure within applications, leading to better maintainability and extensibility.
Implementing abstraction in Java: Implementing abstraction in Java involves creating abstract classes or interfaces to define common behaviors and characteristics that subclasses can inherit. By implementing abstractions, developers can achieve a level of decoupling between the client code and the implementation details. This results in code that is less prone to modifications and easier to scale. Implementing abstraction promotes a modular design approach, allowing for interchangeable components and reducing dependencies between modules. However, overuse of abstraction can lead to unnecessary complexity and decrease code readability, requiring a balanced approach in Java development.
Class Relationships and Packages
In the vast landscape of Java programming, understanding class relationships and packages plays a pivotal role. The relationship between classes in Java signifies how they interact and collaborate within a program. By delving into class relationships, developers can comprehensively design and structure their code, enhancing its readability, maintainability, and reusability. Packages, on the other hand, serve as containers for classes, allowing for logical organization and modular design. Efficiently utilizing packages can streamline project management and facilitate code sharing among team members. Moreover, grasping class relationships and packages is essential for developing scalable and robust Java applications, making it a core aspect of this comprehensive guide.
Association and Composition
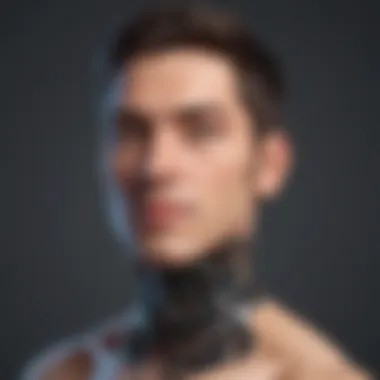
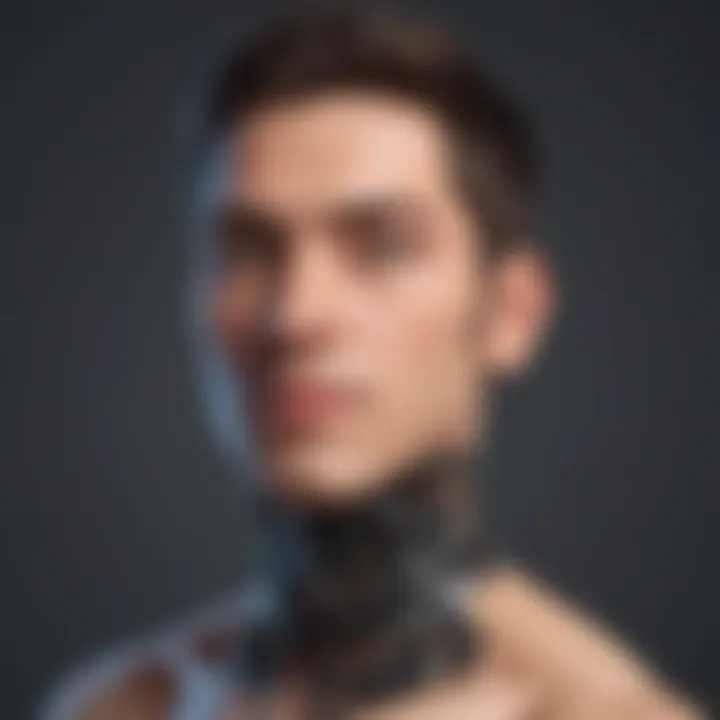
Understanding Class Relationships:
When exploring class relationships, the focus lies on how classes are interconnected and dependent on each other. Understanding these relationships provides insight into the flow of data and functionality within a program. By defining associations between classes, developers can establish links, enabling information exchange and collaboration. This promotes a structured design approach that enhances code clarity and modularity. Appreciating the nuances of class relationships is crucial for building complex systems where multiple classes interact seamlessly to achieve overarching software functionality.
Distinguishing Association and Composition:
Distinguishing between association and composition is vital in Java programming. While association signifies a relationship where classes are connected but can exist independently, composition represents a stronger bond where one class comprises the other. Recognizing this disparity is key to designing scalable and efficient systems. Associations offer flexibility, allowing for loosely coupled components, while composition ensures a tighter integration, often leading to complex yet cohesive entities. Deciphering between these two paradigms empowers developers to architect robust applications tailored to specific design requirements, a valuable skill emphasized within this article.
Java Packages
Organizing Classes in Packages:
The practice of organizing classes in packages imparts a structured approach to Java development. Packages serve as containers for related classes, enabling developers to categorize and manage large codebases effectively. Through logical grouping, packages facilitate code navigation and enhance project clarity. Organized packages promote code reusability and streamlining of development workflows. Embracing this practice fosters code maintainability and collaboration among team members, integral for the success of Java projects discussed in this guide.
Importing and Using Package Classes:
Importing and utilizing package classes is an indispensable aspect of Java programming. This process involves incorporating external classes into a project to leverage their functionalities. By importing classes from packages, developers can access predefined code modules, saving time and effort in development. Utilizing package classes promotes code modularization and simplifies project management. However, prudent consideration is required to minimize namespace conflicts and ensure efficient utilization of imported classes. Mastering this skill empowers developers to harness the full potential of Java's extensive library ecosystem, aligning with the in-depth coverage provided in this comprehensive guide.
Class Design Principles
In the realm of Java programming, understanding class design principles is paramount for ensuring the efficiency, scalability, and maintainability of your codebase. These principles serve as guiding lights, facilitating the creation of robust and flexible software solutions. By adhering to class design principles, developers can streamline the development process, enhance code quality, and promote reusability. Whether you are a novice or a seasoned programmer, grasping these foundational principles is essential for mastering Java programming.
SOLID Principles
Single Responsibility Principle
The Single Responsibility Principle (SRP) is a cornerstone of object-oriented design, emphasizing the importance of a class having only one reason to change. By focusing on a single responsibility, classes become more cohesive, easier to maintain, and less prone to code bloat. Implementing SRP results in modular, readable code that enhances code understanding and facilitates future modifications. adhering to SRP fosters code that is easier to test and debug, ultimately contributing to a more efficient and scalable codebase within the context of this article.
OpenClosed Principle
The OpenClosed Principle (OCP) advocates for classes to be open for extension but closed for modification. This principle encourages developers to design classes in a way that allows for extending functionality without altering existing code. By adhering to OCP, developers create code that is more resilient to changes, reducing the risk of introducing errors in the existing codebase. Embracing OCP leads to code that is more adaptable, less prone to bugs, and easier to maintain, providing significant advantages for the topics discussed in this article.
Design Patterns
Common Design Patterns in Java classes
Common design patterns in Java classes are established solutions to recurring design problems encountered during software development. These patterns encapsulate best practices, fostering code that is more modular, scalable, and maintainable. By incorporating common design patterns into Java classes, developers can expedite the development process, improve code readability, and enhance code maintainability. Understanding and leveraging these design patterns is crucial for developing robust and efficient Java applications relevant to the scope of this article.
Applying Design Patterns Effectively
Applying design patterns effectively involves implementing design patterns in a manner that aligns with the specific requirements and goals of a software project. Effectively applying design patterns requires a deep understanding of their internal workings, trade-offs, and implications. By leveraging design patterns effectively, developers can design code that is more flexible, extensible, and resilient to changes. This strategic application of design patterns plays a pivotal role in developing high-quality Java applications, as discussed in this article.