Understanding NumPy in Python: A Comprehensive Guide
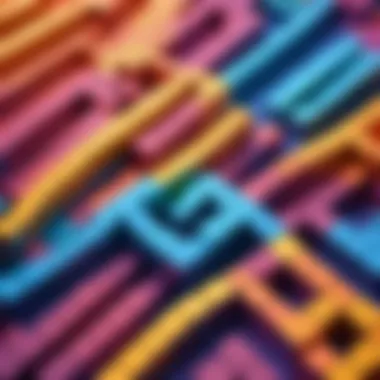
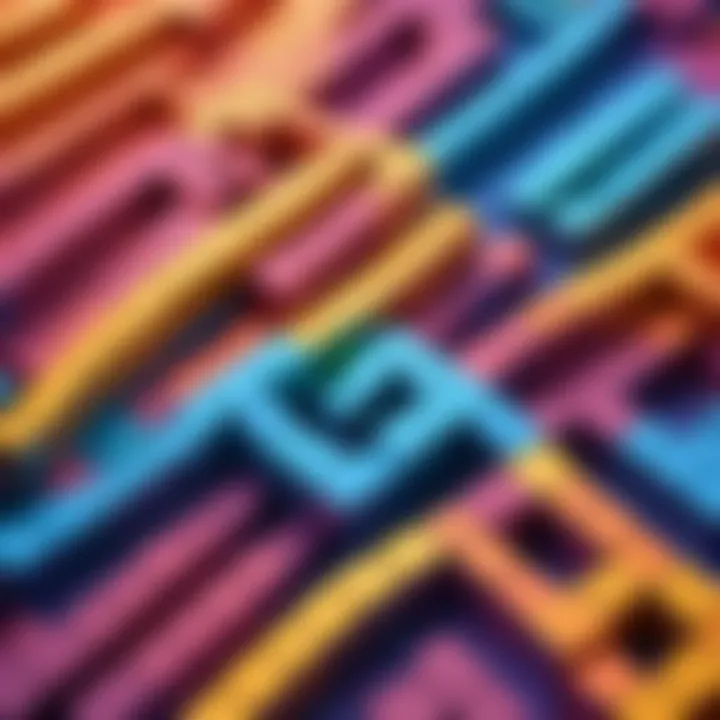
Intro
NumPy is a comprehensive library essential for numerical computations and data manipulation in Python. Developing from its origins in the early 2000s, its evolution reflects the demands of the data science community. NumPy simplifies handling arrays and matrices, and prioritizes performance through its optimized codebase. In this exploration, we will uncover the features that make NumPy indispensable, not only for academics but also for industries focusing on data analysis and machine learning.
Coding Challenges
Coding challenges often serve as practical applications of NumPy’s functions. These problems can range from basic array manipulations to complex data analyses. Engaging in coding challenges can enhance financial computing skills, making it valuable for job seeker hoping to establish expertise in this field.
Weekly Coding Challenges
Each week, programmers from various backgrounds gather online to tackle challenges. These tasks can help both aspiring and experienced coders to solve problems involving NumPy operations. Weekly contests allow permission of skill-building through real-world applications. Examples might include:
- Manipulating data arrays
- Performing matrix algebra
- Implementing statistical functions
Problem Solutions and Explanations
Effective learning occurs by practicing coding problems and analyzing different approaches to solutions. Understanding why a particular solution works is just as important as implementing it. Each solution provided usually comes with detailed explanations, tracing back to the fundamental NumPy abilities used, ensuring readers comprehensively grasp the logic.
Tips and Strategies for Coding Challenges
To excel in these challenges using NumPy, consider the following strategies:
- Read problem specifications carefully. Failing to understand the problem can lead to awkward assumptions.
- Break down complex problems into smaller ones. Tackling pieces one at a time simplifies equation resolutions.
- Practice common NumPy functions and methods. Familiarity with tools can increase efficiency.
- Join discussion forums. Platforms like Reddit are excellent for knowledge sharing and tutorials that refine your approach from experience.
Community Participation Highlights
The online coding community thrives on collaboration. Many tech forums allow users to submit solutions and discuss the process. Participation can unveil different methods of leveraging NumPy, which broadens networking and encourages collective learning.
Epilogue
Mastering NumPy requires practice and engagement. From solving weekly challenges to understanding various techniques, real-world skills can develop significantly. In the sphere of Python programming, gaining competency and confidence in using NumPy yields numerous opportunities for technological innovation and professional growth.
What is NumPy?
Understanding NumPy is essential for those who work with data in Python. It offers efficient numerical capabilities which are crucial for various computational tasks. In environments such as data science and machine learning, NumPy's functionality supports a range of applications from simple calculations to complex mathematical operations.
Definition and Overview
NumPy, short for Numerical Python, is a powerful open-source library that is foundational in the realm of scientific computing with Python. It establishes a high-performance multidimensional array object, which constitutes the core of its functionality. NumPy allows users to perform seamless operations on these arrays, enabling efficient processing and manipulation of numerical data.
The structure of arrays in NumPy is quite different from standard Python lists. Arrays are designed to accommodate a single data type, which optimizes speed and memory usage. Furthermore, the programmed operations, called Universal Functions or ufuncs, apply operations element-wise on these arrays, enhancing performance.
The Purpose of NumPy in Python
NumPy serves as a critical cornerstone in Python's ecosystem by providing high-level functionalities for mathematical computations. Its primary role addresses the limitations of Python's built-in data types by delivering faster and more capable replacements for array operations.
There are multiple benefits of using NumPy in Python:
- Performance: Due to its efficient implementation in C, NumPy has minimal overhead compared to native Python operations. This results in faster execution for array calculations.
- Convenience: NumPy supports broadcasting, allowing automatic expansion of arrays for arithmetic operations, circumventing the need for manual alignment of shapes.
- Compatibility: It pairs well with a multitude of other libraries, establishing a cohesive environment for data analysis. For instance, its integration with Pandas and Matplotlib further enhances functionalities.
Here is an important insight about NumPy:
NumPy can dramatically improve the efficiency of code execution in tasks that involve large datasets.
History of NumPy
The history of NumPy holds significant importance in understanding its role within the broader Python ecosystem. NumPy, known for providing support for large, multi-dimensional arrays and matrices, is integral to the field of numerical computing. Comprehending this history not only highlights user engagement with the tool but also showcases its evolution alongside the development of scientific computing needs in Python. Key aspects to consider include its origins, development timeline, and contributions from its community.
Origins and Development
NumPy originated from an earlier library called Numeric, which was created by Jim Hugunin in the mid-1990s. Shortly after this, another library, Numarray, was developed by a group dedicated to scientific computation. However, these early libraries exhibited various limitations, which led to a thought of merging their features into a single, improved library.
In 2005, Travis Olliphant took the lead in this project and initiated the development of NumPy as it exists today. The aim was to create a robust foundation for array computing, unifying many of the data types and functionalities previously disparate. By utilizing an efficient implementation in C, NumPy enabled rapid numerical computations. This improved performance set a strong standard within Python's data science community.
Key Contributors and Milestones
Many contributors played a critical role in NumPy's development, enhancing its functionality throughout the years. Some of the key contributors include:
- Jim Hugunin: The creator of Numeric, whose early work paved the way for association between Python and numerical computations.
- Travis Olliphant: Central to the birth of NumPy, he incorporated ideas from Numeric and Numarray to enhance usability and performance.
- Other collaborators: Various scientists and programmers contributed countless updates and bug fixes, relentlessly improving the library.
Milestones achieved during this journey include:
- The effective uniting of two previously separate libraries into a highly efficient tool by 2006.
- Wide adaptation of NumPy in scientific computing and data analysis fields due to its efficiency and user-friendly nature.
- Continued development and optimization efforts, addressing any performance issues reported by users with subsequent releases.
Understanding the history of NumPy offers insight into its features and underscore its significance for users.
By grasping the historical context, users can appreciate not only how NumPy sustains current endeavors in data science but also what its future developments may hold.
Key Features of NumPy
NumPy is the cornerstone of numerical computing in Python. Its key features serve as a potent foundation for various applications, especially in data science and machine learning. The ability to work efficiently with large datasets is crucial in these domains, and NumPy exhibits several features that optimize performance and functionality. This section expounds upon three pivotal aspects of NumPy: N-dimensional arrays, universal functions, and broadcasting rules.
N-dimensional Arrays
N-dimensional arrays, often called ndarrays, are at the heart of NumPy. Unlike traditional Python lists, which are limited to one-dimensional data handling, ndarrays allow for storage and manipulation of multi-dimensional data.
The significance of these arrays is evident in the versatility they provide. Users can create arrays of various dimensions, such as 1D, 2D, or higher. This means that activities like matrix manipulations, datasets processing, and even computational simulations can be handled more effectively.
For instance, the following code snippet demonstrates how to create a 2D ndarray:
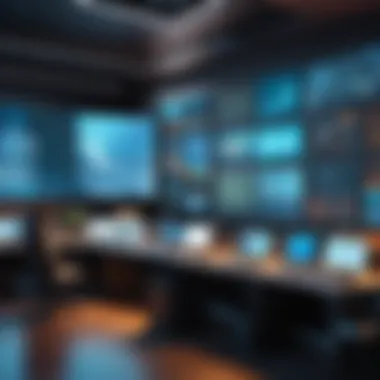
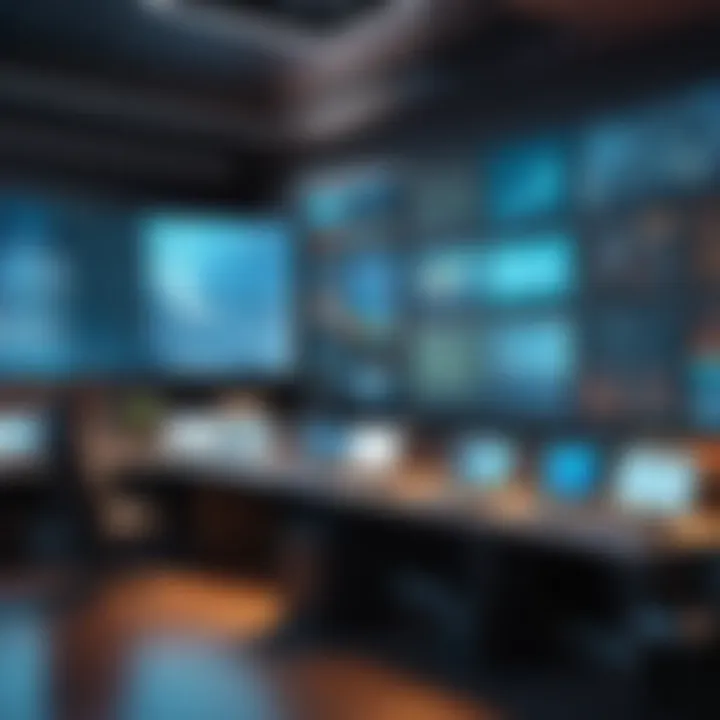
This creates a simple 2x3 matrix, showcasing the power of arrays in representing complex data structures. Managing such structures becomes easier, contributing to efficient algorithm implementations.
Universal Functions
Universal functions, or ufuncs, are another notable feature of NumPy. These are functions that operate element-wise on arrays, allowing for fast computations across entire datasets without looping through individual elements. This leads to optimized performance as compared to traditional Python functions.
Key highlights of ufuncs include:
- Speed: They are implemented in C, promoting increased computation speed.
- Broadcasting: Ufuncs can handle input arrays of different shapes, making them highly adaptable for various operations.
An example of using a universal function is hte following, which calculates the square of each element of an array:
This example illustrates the efficiency of applying functions across multidimensional datasets seamlessly.
Broadcasting Rules
Broadcasting is a powerful feature that allows NumPy to handle arithmetic operations on arrays of different shapes. This is done by
Installation of NumPy
Installing NumPy correctly is essential for utilizing its capabilities in numerical analysis and data manipulation. An improper installation can lead to various issues ranging from module import errors to performance problems. This section will touch on two prevalent methods for NumPy installation: using and setting up through Anaconda. Each method offers its own advantages, making it viable to choose based on your system requirements and personal preferences.
Using pip for Installation
Using , the Python package installer, is one of the simplest ways to install NumPy. This method is particularly beneficial for users who prefer a lightweight setup.
To install NumPy using , follow these steps:
- Open your command prompt or terminal. If you're on Windows, you can run , and on macOS or Linux, simply open .
- Run the following command:
- Verify the installation: You can check that the installation took place successfully by running this Python command:If there are no errors, it indicates that NumPy has been installed correctly.
Using also allows for easy updates. If you want to upgrade to the latest version of NumPy in the future, just run:
This simplicity and efficiency make using an attractive option for Python users.
Setting Up Anaconda
Anaconda is a widely adopted distribution for managing Python packages, especially in the data science and machine learning communities. NumPy may be included in the default Anaconda installation, simplifying usage significantly.
To set up NumPy with Anaconda, do the following:
- Download Anaconda Distribution from the official website.
- Follow the installation instructions for your operating system. The installer guides you step-by-step, making it simple to get started.
- Open Anaconda Navigator: It provides an easy-to-use interface for managing packages and environments.
- Install NumPy by creating a new environment or using an existing one:
You can use the following command in the Anaconda Prompt: - Check NumPy Installation: Like with pip, you can verify your installation using:
Utilizing Anaconda for your NumPy setup offers additional advantages, such as environment management tools and package version compatibility between dependencies, reducing potential conflicts.
Overall, the installation method is critical for leveraging NumPy's rich features. Before diving into your data analytic projects, ensure you have successfully installed NumPy to avoid obstacles.
Basic Operations with NumPy
Basic operations with NumPy are crucial for anyone using this library. These operations form the foundation of manipulating data and performing computations effectively within the Python environment. Understanding these basics enables programmers to harness the power of NumPy and enhances their ability to analyze, transform, and visualize data.
Creating Arrays
Creating arrays is the first step in using NumPy. An array is the primary structure for data in NumPy. It allows for efficient storage and manipulation. Arrays can be created in multiple ways, such as from existing data, using NumPy functions or through special functions tailored for creation. Examples of these include , , and . Each method serves different purposes:
- allows conversion from lists or tuples to an array format. This is great for transforming raw data into a structured form.
- creates an array filled with zeros. Useful for initializing data.
- generates a range of numbers, accommodating increments as specified.
For instance, to create a one dimensional array:
Indexing and Slicing
Indexing and slicing are critical for accessing and extracting portions of data from an array without altering the original data set. NumPy supports slicing, similar to Python lists, allowing for flexible selection patterns and manipulations of data structures.
You can access specific values with indexing. Starting with 0, the first element of an array could be access by the code below:
Slicing allows users to select a subset of the array through specifying range. For example:
Mastering indexing and slicing empowers users to manipulate large data sets efficiently. It provides a more flexible approach to handling and retrieving data patterns while keeping code clean and concise.
Array Reshaping
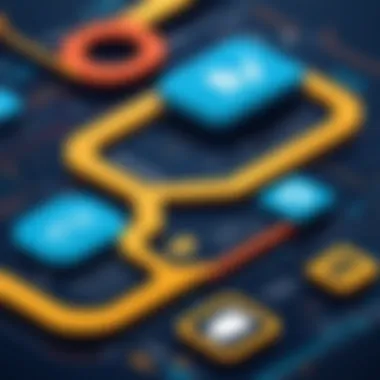
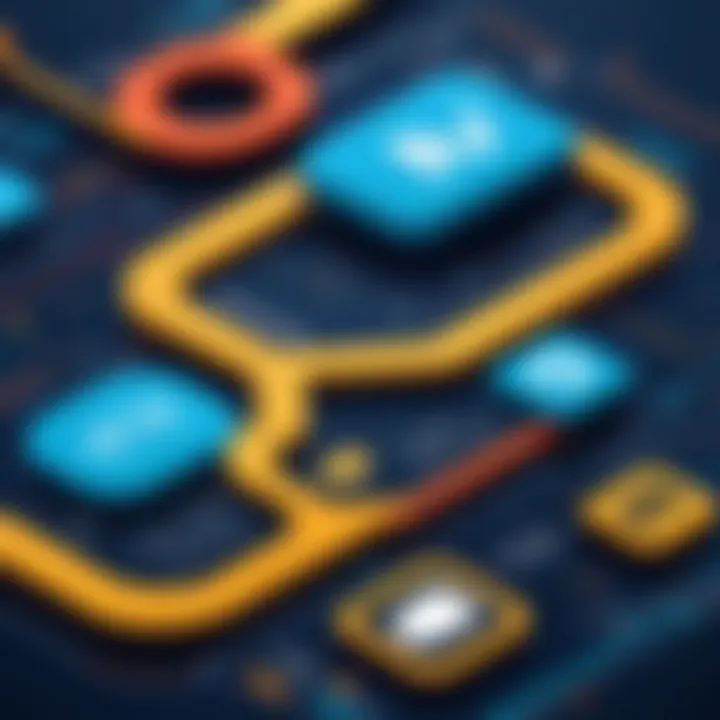
Reshaping arrays is another significant aspect of using NumPy. This allows users to change the shape or dimensions without altering the data. When data is reorganized physically, it can lead to improved workflows depending on the analysis required.
Changing the shape uses the method. This enables the construction of multi-dimensional arrays from one-dimensional ones. For example:
This results in:
Reshaping can enhance compatibility between operations. However, it should be careful, since the total number of elements must remain the same.
Basic operations like creating arrays, indexing, slicing, and reshaping are essential to understanding and harnessing the power of NumPy. The further your skills with these operations grow, the more robust and resourceful your data manipulation becomes.
These core concepts lay a solid groundwork, allowing users to explore more advanced NumPy features with confidence.
Advanced NumPy Functions
Advanced NumPy functions play a critical role in numerical computing and data manipulation. Understanding these functions is essential for anyone aiming to harness NumPy’s full potential in their projects. They provide the tools needed for complex mathematical operations and statistical analysis, enhancing the power of data-driven applications. Expanding your knowledge of these functions paves the way for efficient coding and robust outcomes, not just in simple array operations, but also in substantial applications like scientific research and machine learning.
Linear Algebra
Linear algebra is a core aspect of many domains, including computer science, engineering, economics, and physics. NumPy provides various functions to perform linear algebra operations effectively. These functions enable users to work with matrices and vectors, facilitating operations such as dot products, matrix multiplication, eigenvalue computation, and solutions to linear equations.
Key functions in this category include:
- : Computes the dot product of two arrays.
- : Finds the inverse of a given square matrix.
- : Determines the eigenvalues and eigenvectors of a matrix.
Using these functions, you can solve equations or transform data easily.
For example, to compute the dot product between two arrays:
This basic knowledge of linear algebra functions can solve more complicated tasks involving matrices.
Statistics Functions
Statistics also forms an important foundation for data analysis, and NumPy compiles a set of functions to simplify these calculations. These functions allow programmers to derive meaningful insights from datasets. With NumPy, you can compute various statistical metrics that are vital in real-world applications.
Some useful statistical functions in NumPy are:
- : Calculates the average of an array.
- : Computes the standard deviation.
- : Provides the Pearson correlation coefficients for arrays.
These functions can help in efficiently analyzing data trends or carrying out hypothesis testing.
For instance, to calculate the mean of an array:
Integration of NumPy with Other Libraries
Integration of NumPy with other libraries is crucial for optimizing operations in Python, especially in data manipulation and analysis. NumPy’s powerful array processing capabilities serve as the foundation for several other libraries, including Pandas and many machine learning frameworks. This interconnectivity permits an effective pooling of resources, maximizing efficiency and speed across various applications.
NumPy and Pandas
Pandas is a well-known data analysis library that leverages NumPy arrays in its operations. The ease of integrating these two libraries plays a pivotal role in manipulating large data datasets.
While NumPy offers a more efficient means to work with multi-dimensional arrays, Pandas adds additional layers for data structures, focusing more on labeled data.
- DataFrames and Series: Pandas utilize NumPy’s ndarray as the underlying structure for the DataFrame and Series objects. This facilitates complex data operations and statistical analyses.
- Improved Performance: The use of NumPy arrays allows Pandas to perform batch operations more efficiently than regular Python lists.
- Data Manipulation: Functions derived from NumPy, like vectorized operations, make it seamless to manipulate and analyze datasets.
Furthermore, both libraries often share similar syntax. Hence, developers experienced in NumPy can readily adapt to using Pandas without steep learning curves. The combination streamlines several analytical concepts, making the learning experiences more efficient.
NumPy in Machine Learning
In machine learning applications, seamless integration of NumPy is key. Several popular libraries, including TensorFlow and Scikit-learn, build upon NumPy. Here’s why this integration is ingrained into the machine learning workflow:
- Efficient Computation: Many machine learning algorithms depend heavily on linear algebraic computations, which are fundamentally optimized in NumPy. This ensures that models train faster.
- Feature Foundations: Raw data is often converted into NumPy arrays for models. This acts as a conduit to prepare features that convert data comprehensively.
- N-dimensional Manipulation: NumPy’s multidimensional arrays support the complex data shapes needed in machine learning models, such as large convolution operations in neural networks.
Overall, the integration of NumPy into various libraries elevates the capabilities in not just theoretical pursuits but real-world applications in data science and artificial intelligence.
Thus, the role of NumPy as a backbone becomes apparent. It occupies a significant position in the Python ecosystem, enhancing the relevance and efficiency of other vital libraries in performance optimizing tasks.
Performance Optimization with NumPy
Optimization in NumPy is fundamental for maximizing performance, especially when dealing with large datasets or computational tasks that require efficiency. It is essential to understand that running mathematical operations in NumPy's framework can often be more efficient than using conventional Python loops. NumPy is optimized for operations on arrays, but recognizing how to structure your code for optimal performance can make all the difference in execution time.
The specific elements include methods like vectorization and efficient memory management. These approaches are not optional but rather integral components that skilled programmers leverage to enhance performance.
Effective performance optimization can significantly reduce runtime and increase responsiveness, a critical factor in today's compute-intensive applications.
Using Vectorization
Vectorization refers to the ability of NumPy to execute operations on entire arrays instead of via individual elements or loops. This results in cleaner and often faster code because it relies on the highly optimized C libraries that NumPy uses under the hood. One key benefit of vectorization is reducing overhead associated with loops in Python. For instance, consider the array operation below:
In this example, the addition operation on arrays and computes regardless of their size, while a typical Python for loop would take much longer due to Python's interpreted nature. This not only makes your code easier to read but further empowers complex analyses and data manipulation tasks with significantly reduced complexity and run time.
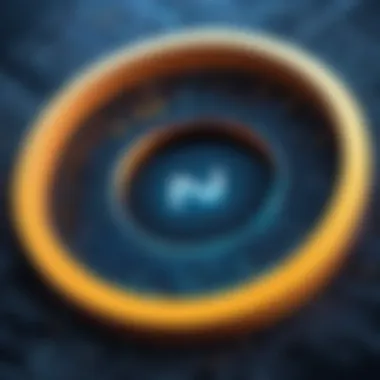
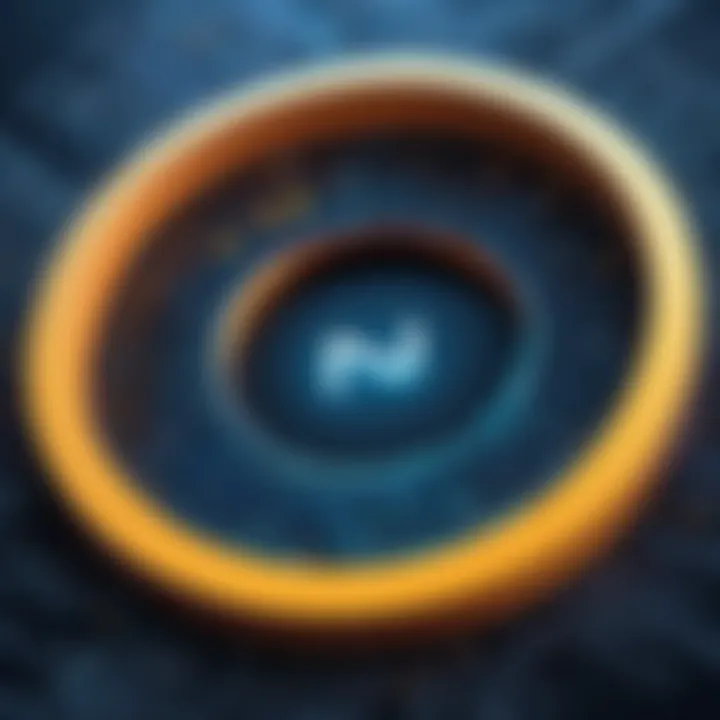
Memory Management
Memory management in NumPy is about how memory is allocated and accessed. NumPy Arrays are more memory efficient compared to Python listsbecause they store data in contiguous memory locations. There are a few key aspects to consider when managing memory efficiently:
- Avoid creating copies: This can be expensive. Functions like create a genuinely independent copy, while assigning a NumPy array to another variable simply creates a view.
- Data types: Choose specific data types according to your needs. For instance, using uses less memory than when appropriate.
- In-place operations: Functions that modify the original data, rather than returning a new array, can save memory as well.
Memory management won’t just guard against inefficiency; it safeguards against problems that arise from memory consumption issues.
In optimizing with NumPy, considering vectorization and memory management will ensure you extract the robust capabilities wrapped within the library, pushing the boundaries of what you can accomplish with numerical data processing.
Common Pitfalls and Challenges
Understanding NumPy also involves recognizing the common pitfalls that can impede the user experience and performance. While NumPy offers substantial advantages in numerical computing, there are several challenges that individuals, both new and experienced to Python programming, may encounter. An awareness of these pitfalls enhances the efficacy of NumPy by minimizing unexpected errors and maximizing performance. By addressing these challenges head-on, users can fully exploit the capabilities of this powerful library.
Errors in Array Manipulation
When working with NumPy, problems often arise through unexpected behavior in array manipulations. This is especially true for those new to the library who may misinterpret how arrays function. Common errors include:
- Shape Misalignment: Trying to perform operations on arrays that do not share the same dimensions can lead to runtime errors. Operations such as addition or multiplication of incompatible shapes can cause silent failures or unexpected results. It is vital to scrutinize the shapes of your arrays before conducting arithmetic or matrix operations.
- Immutable Objects: Unlike standard Python lists, NumPy arrays are fixed in their size. Whilst reshaping is possible, resizing an array without creating a new object will not yield the expected results. This misunderstanding can lead to attempts to modify an array without using appropriate methods, resulting in inefective code.
To mitigate these errors, working with NumPy’s built-in functions to check array properties before manipulation is prudent. Utilizing proper checks and balances enables more reliable code, preventing hours of frustrating debugging.
Performance Misunderstandings
Another area of confusion for users relates to the performance capabilities of NumPy. While NumPy optimizations vastly improve efficiency over standard Python, misunderstanding when and where to apply optimizations can lead to subpar performance. Following are some aspects to consider:
- Vectorization Misusage: A common mistake occurs when users cling to traditional for-loops rather than opting for more efficient vectorized operations. NumPy's speed comes primarily from operating on whole arrays, so neglecting this feature leads to sluggish performance. Learning to refactor code from for-loops to employ NumPy array operations makes a significant difference.
- Excessive List Conversions: Another notable pitfall concerns the conversion between Python lists and NumPy arrays. This conversion can be minimally performant. If large lists are frequently being transformed into arrays, minimizing these actions can generate huge performance improvements. It is prudent to establish your data in the correct format before initiating complex errands, to avoid skipping out on the advantages NumPy provides.
Users should familiarize themselves with optimal performance practices applicable to NumPy. Understanding the relationship between data structures and operations is key for harnessing the full potential of the library. When executed correctly, NumPy can attain impressive speed and efficiency, but misapplications can thwart its advantages.
Keeping these pitfalls in mind can enhance not only your understanding of NumPy but also your capabilities in numerical computing. It reflects preparation and foresight in a field where precision matters.
Taking the time to comprehend and navigate around these challenges pays dividends in both performance and satisfaction with using one of Python's foremost libraries for numerical analysis.
Applications of NumPy
NumPy stands as a pillar in the Python programming ecosystem. Its applications extend across diverse fields such as data analysis, scientific computing, and machine learning. Understanding NumPy’s applications aids users in fully capitalizing on its capabilities, allowing for not only efficient computation but also strategic development within various domains.
Data Analysis
Data analysis is one of the most prominent applications of NumPy. The library serves as a bedrock for many data analysis tools. It enables users to manage and manipulate large datasets effortlessly. By leveraging NumPy's powerful features, users can conduct complex operations that would otherwise be cumbersome if using standard Python lists.
Several key benefits come with using NumPy in data analysis:
- Efficiency: NumPy's focus on array processing allows operations to be executed faster than Python's native lists. This results in improved performance, especially when handling large amounts of data.
- Convenience: Functions such as array reshaping, broadcasting, and slicing are vital in analyzing data. NumPy makes these operations not only possible but straightforward.
- Integration: NumPy integrates seamlessly with other libraries like Pandas and Matplotlib, enhancing its utility in data analysis pipelines.
In essence, leveraging NumPy in data analysis leads to significant time-saving while improving the rigor of numerical computations.
Scientific Computing
In the realm of scientific computing, NumPy plays a critical role in solving many numerical problems. With its n-dimensional arrays and mathematical functions, it is suitable for tasks across various scientific fields. Researchers and engineers rely on NumPy to perform rigorous calculations that demand accurate results and high performance.
Some benefits of using NumPy in scientific computing include:
- Numerical Stability: Algorithms built with NumPy are generally more stable than those created using other libraries, thanks to the low-level optimizations.
- Versatile Functions: Mathematical functions provided by NumPy make it easier to compute complex equations or simulations needed in scientific research.
- Performance: Computational efficiency allows simulations to run quickly, which is essential in research where iterative calculations are common.
For anyone developing applications in physical sciences, engineering, or computational biology, NumPy is often an indispensable tool.
Machine Learning and Artificial Intelligence
Machine learning and artificial intelligence (AI) increasingly tap into NumPy's functionality for handling data and performing fast computations. These fields require various matrix and vector operations, and NumPy excels at this. It empowers developers to build and refine algorithms effectively, impacting model training and prediction accuracy.
Below are several key considerations in applying NumPy in machine learning:
- Array Manipulations: NumPy provides an efficient framework for manipulating multi-dimensional arrays, crucial for handling feature sets and training datasets.
- Optimization Framework: It helps implement gradient descent and other optimization algorithms that benefit significantly from fast matrix computations.
- Prototyping Speed: Machine learning practitioners often need to experiment with different models quickly, and NumPy allows for building initial prototypes expeditiously due to its straightforward API.
As a cornerstone library, NumPy enhances the ability of developers and researchers to create more sophisticated models that can handle and derive insights from vast data sets in the evolving landscape of machine learning. Advancements in AI often depend on mastering and optimizing these computations, which reinforces the importance of mastering NumPy.
Future of NumPy
The Future of NumPy holds great significance as it aligns with the ever-evolving demands of computational tasks and data analysis. Understanding where NumPy is heading can help both aspiring and experienced programmers prepare for changes in their workflow. Major ongoing enhancements indicate a commitment to improving performance, usability, and integration with emerging technologies.
Upcoming Features and Enhancements
NumPy's future capabilities are focused on functionality improvements and extended reach into other areas of tech. One anticipated enhancement is the ongoing support for faster operations as close to hardware performance as is feasible. With JAX, Dask, and other libraries introduced to conquer larger data sets, NumPy's ambition will provide efficiency.
Furthermore, the incorporation of type annotations aims to support modern Python development trends and reduce errors. The emphasis on user experience is critical. Other proposals include:
- More abstract data types for harmonious compatibility with libraries like CuPy.
- Extension of FFI (Foreign Function Interface) increasing use cases.
- Inclusion of API compatibility enhancements that improve links to SciPy and other important libraries.
These features reflect flexibility and continuous growth that addresses a climate of advanced data usage and unique programming bisogaciens.
Community and Contributions
The strength of NumPy also derives from its community. Contributions flow in from experts and novices alike, shaping the future of the library. A variety of sources guide users to maintain stability and authority in guiding user practices.
Critical aspects of diversity and involvement entail:
- Development proposals through GitHub where users can submit requests or contribute code enhancements.
- A user feedback loop sanctioned through forums on Reddit and its official discussion platforms.
- Collaborative events like NumPy Sprint, communal brainstorms decipher improvements, and wish lists directly influenced by user pain points.
A thriving ecosystem of integrations with AI, Machine Learning, and Deep Learning paradigms fosters a space where NumPy’s evolution proceeds intertwined with modern solutions in technology. This levven contributes to accelerating learning trajectories and optimizing problem-solving solutions blocd fairness.
As NumPy moves forward, it stands to not only enhance its performance metrics but also redefine its relevance in data-centric ecosystems.
The interoperability with innovators keeps the library quick to pivot toward novel trends and breakthroughs in computation.